Bash Test If Command Exists
In Bash scripting, it is often necessary to check if a certain command exists before executing it. This can be useful to handle different scenarios depending on the availability of certain commands, or to ensure that a script can run on different systems without encountering errors. Fortunately, Bash provides several options for testing the existence of a command. In this article, we will explore four different approaches to accomplish this task: using the -v option, the which command, the command command, and the -n option.
Checking for a Command Using the -v Option
One way to test if a command exists in Bash is by using the -v option of the Bash command. The -v option prints a version of the command if it exists, or nothing if the command does not exist. The following is the syntax for using the -v option to check if a command exists:
“`bash
command -v
“`
In the above syntax, `
Let’s consider an example to better understand the usage of the -v option. Suppose we want to check if the `git` command exists. We can use the following command:
“`bash
if command -v git >/dev/null 2>&1; then
echo “Git is installed”
else
echo “Git is not installed”
fi
“`
In this example, the output of the `command -v git` command is redirected to the null device (`/dev/null`) to suppress any output. The redirection of standard error (`2`) and standard output (`1`) to `/dev/null` ensures that no error messages are displayed. If the `git` command exists, the output of `command -v git` will be non-empty, and the script will display “Git is installed”. Otherwise, it will display “Git is not installed”.
Using the which Command to Check for a Command
Another approach to test if a command exists in Bash is by using the `which` command. The `which` command searches for the specified command in the directories listed in the `PATH` environment variable and prints the path to the command if it is found. Here’s the syntax for running the `which` command to determine if a command exists:
“`bash
which
“`
Replace `
Let’s see an example to illustrate the usage of the `which` command. We want to check if the `curl` command exists. We can use the following command:
“`bash
if which curl >/dev/null 2>&1; then
echo “Curl is installed”
else
echo “Curl is not installed”
fi
“`
In this example, if the `curl` command exists, the output of `which curl` will be non-empty, and the script will display “Curl is installed”. Otherwise, it will display “Curl is not installed”.
Testing Command Existence with the command Command
The `command` command provides another way to check if a command exists in Bash. The `command` command executes the specified command, or displays information about the command if it is not executed. Here’s the syntax for using the `command` command to test the existence of a command:
“`bash
command
“`
Replace `
Let’s consider an example to better understand the usage of the `command` command. Suppose we want to check if the `ls` command exists. We can use the following command:
“`bash
if command ls >/dev/null 2>&1; then
echo “ls command exists”
else
echo “ls command does not exist”
fi
“`
In this example, if the `ls` command exists, the output of `command ls` will be displayed, and the script will display “ls command exists”. Otherwise, it will display “ls command does not exist”.
Using the -n Option to Check for a Command in Bash
The -n option is another way to test for command existence in Bash. It checks if a command name is not null, which implies that the command exists. Here’s an overview of the -n option for testing command existence:
“`bash
if [ -n “$(command -v
# command exists
else
# command does not exist
fi
“`
Replace `
For example, let’s check if the `grep` command exists using the -n option:
“`bash
if [ -n “$(command -v grep)” ]; then
echo “grep command exists”
else
echo “grep command does not exist”
fi
“`
In this example, if the `grep` command exists, the script will display “grep command exists”, otherwise it will display “grep command does not exist”.
FAQs:
Q: What is the purpose of checking command existence in Bash scripting?
A: Checking command existence is useful to handle different scenarios depending on the availability of certain commands, or to ensure that a script can run on different systems without encountering errors.
Q: How can I check if a command exists in Bash using the -v option?
A: You can use the `command -v
Q: What does the which command do in Bash?
A: The which command searches for the specified command in the directories listed in the `PATH` environment variable and prints the path to the command if it is found.
Q: How can I use the which command to check if a command exists?
A: Use the `which
Q: What is the purpose of the command command in Bash scripting?
A: The command command provides a way to execute a command or display information about the command if it does not exist.
Q: How can I test if a command exists using the command command?
A: Use the `command
Q: What does the -n option do in Bash command testing?
A: The -n option checks if a command name is not null, indicating that the command exists.
Q: How do I use the -n option to check for command existence?
A: Use the `[ -n “$(command -v
Shell Scripting – If \U0026 If/Else
Keywords searched by users: bash test if command exists Bash if else, Python check if command exists, Makefile check command exists, Bash if and, Bash check if package is installed, Shell script check command successful, Script check folder exists, Boolean bash
Categories: Top 63 Bash Test If Command Exists
See more here: nhanvietluanvan.com
Bash If Else
Introduction
In Bash scripting, conditional statements play a crucial role in making decisions based on certain conditions. One such conditional construct is the if-else statement, which enables you to execute different blocks of code depending on the evaluation of a given condition. In this article, we will delve deep into the world of Bash if else statements, exploring their syntax, usage, and various scenarios where they prove to be highly useful. So, let’s dive in!
Syntax of Bash if else
The basic syntax of a Bash if else statement is as follows:
“`bash
if [ condition ]
then
# code to execute if the condition is true
else
# code to execute if the condition is false
fi
“`
The condition enclosed in square brackets can be any valid expression that evaluates to either true or false. If the condition evaluates to true, the code block following the `then` statement is executed; otherwise, the code block following the `else` statement (if present) is executed. Finally, the statement is terminated with the `fi` keyword.
Usage of Bash if else
1. Simple Comparison:
Bash if else statements are frequently used for performing simple comparisons. For instance, let’s assume we want to check if a given number is positive or negative:
“`bash
#!/bin/bash
read -p “Enter a number: ” num
if [ $num -gt 0 ]
then
echo “The number is positive.”
else
echo “The number is negative.”
fi
“`
Here, the script prompts the user to enter a number. If the number is greater than 0, it prints “The number is positive.” Otherwise, it prints “The number is negative.”
2. File and Directory Operations:
Bash if else statements are also handy for manipulating files and directories. Let’s consider an example where we need to check if a file exists and is readable:
“`bash
#!/bin/bash
file_name=”sample.txt”
if [ -r $file_name ]
then
echo “The file exists and is readable.”
else
echo “The file either doesn’t exist or is not readable.”
fi
“`
In this case, the script checks if the file “sample.txt” exists and is readable. If the condition is satisfied, it prints “The file exists and is readable.” Otherwise, it prints “The file either doesn’t exist or is not readable.”
3. Nested if else statements:
Bash allows you to nest if else statements within each other, enabling you to handle more complex scenarios. Consider a scenario where we need to determine a student’s grade based on their score:
“`bash
#!/bin/bash
read -p “Enter the student’s score: ” score
if [ $score -ge 90 ]
then
echo “A”
elif [ $score -ge 80 ]
then
echo “B”
elif [ $score -ge 70 ]
then
echo “C”
elif [ $score -ge 60 ]
then
echo “D”
else
echo “F”
fi
“`
This script asks the user to input a student’s score. Depending on the score, it prints the corresponding grade (A, B, C, D, or F).
4. Multiple conditions:
In complex scenarios, you often need to evaluate multiple conditions using logical operators such as `&&` (AND) and `||` (OR). Take the following example, where we check if a number is both positive and even:
“`bash
#!/bin/bash
read -p “Enter a number: ” num
if [[ $num -gt 0 && $((num % 2)) -eq 0 ]]
then
echo “The number is positive and even.”
else
echo “The number is either negative or odd.”
fi
“`
In this script, if the entered number is both greater than 0 and divisible by 2 with a remainder of 0, it prints “The number is positive and even.” Otherwise, it prints “The number is either negative or odd.”
FAQs
Q1. Can I use multiple if else statements within a script?
Yes, you can have multiple if else statements within a script. Just make sure to maintain the proper syntax and logical flow.
Q2. Are if else statements case-sensitive?
No, if else statements in Bash scripting are not case-sensitive. However, the variables and strings used in the conditions may be.
Q3. Can I compare strings using if else statements?
Yes, you can compare strings in Bash using the `==` operator. For example, `if [ $string1 == $string2 ]`.
Q4. How can I check for the absence of a file?
To check if a file does not exist, you can use the negation operator `!` along with the file existence check condition. For instance, `if [ ! -f $file_name ]`.
Q5. Can I use curly braces instead of square brackets in the condition?
Yes, you can use curly braces `{}` instead of square brackets `[]`. However, using square brackets is a more conventional and widely accepted practice.
Conclusion
Conditional statements are fundamental to Bash scripting, and the if else statement is a powerful tool for decision-making within scripts. In this article, we’ve covered the syntax and various usage scenarios of Bash if else statements, equipping you with the knowledge to apply them effectively in your own scripts. So, go ahead, experiment, and make your Bash scripts smarter with if else statements!
Python Check If Command Exists
Python is a high-level programming language widely known for its simplicity and versatility. When working with Python, you may often find the need to check if a specific command or function exists before using it. By ensuring the presence of a command, you can handle scenarios where the command is unavailable or deprecated in certain Python versions. In this article, we will explore various methods to check if a command exists in Python, along with some frequently asked questions.
Checking If a Command Exists
Python provides several approaches to check the existence of a command or function. Let’s delve into some of the most common methods.
1. Using the “try-except” block:
One way to check if a command exists is by attempting to import the module that contains the command using the “try-except” block. If the import succeeds, it signifies that the command exists, and you can proceed with using it in your code. Here’s an example:
“`python
try:
import module_name
# Command exists, proceed with using it
except ImportError:
# Command does not exist or cannot be imported
“`
2. Using the “dir()” function:
Another method is to use the built-in “dir()” function. This function returns a list of attributes and methods associated with an object. By checking if the desired command is present in the resulting list, you can determine its existence. Consider the following code snippet:
“`python
command = “my_command”
if command in dir(module_name):
# Command exists, proceed with using it
else:
# Command does not exist
“`
3. Using the “hasattr()” function:
The “hasattr()” function can also be employed to verify the presence of a command or function. It takes two arguments: the object to check, and the name of the attribute or method to verify. If the function returns `True`, the command exists; otherwise, it does not. Here’s an example:
“`python
if hasattr(module_name, “my_command”):
# Command exists, proceed with using it
else:
# Command does not exist
“`
4. Using the “callable()” function:
To specifically check if a command is callable, meaning it can be invoked as a function or method, you can utilize the “callable()” function. This function returns `True` if the specified object is callable; otherwise, it returns `False`. Consider the following code snippet:
“`python
command = module_name.my_command
if callable(command):
# Command exists and is callable, proceed with using it
else:
# Command either does not exist or is not callable
“`
5. Using the “importlib” module:
Python’s “importlib” module provides additional capabilities to dynamically import modules and inspect their contents. The “import_module()” function from the “importlib” module allows you to import a module by its name as a string. You can then use other methods, such as “hasattr()”, to determine the existence of the desired command. Here’s an example:
“`python
import importlib
try:
module = importlib.import_module(“module_name”)
if hasattr(module, “my_command”):
# Command exists, proceed with using it
else:
# Command does not exist
except ImportError:
# Module does not exist or cannot be imported
“`
Frequently Asked Questions
Q: Why is it important to check if a command exists before using it?
A: Checking if a command exists before using it helps avoid issues such as runtime errors or unexpected behaviors. It ensures that your code executes smoothly without triggering exceptions or relying on unsupported features.
Q: Can I check if a specific Python function exists?
A: Yes, you can. The methods mentioned above can be used to check the existence of any Python command, including functions.
Q: How can I handle the case when a command does not exist?
A: You can utilize conditional statements (e.g., if-else) to handle scenarios where the command does not exist. This allows you to provide alternative actions or error messages to the user.
Q: Can I check the existence of a command in a specific Python version?
A: Yes, you can employ the aforementioned methods to check the availability of a command in a particular Python version.
Q: Is there any performance impact when using these checks?
A: The performance impact of these checks is generally negligible. However, it’s recommended to employ them only when necessary to avoid unnecessary complexity in your code.
Q: Can these methods be used for modules installed via pip?
A: Yes, these methods can be applied to check if commands or functions exist in any imported modules, including those installed via pip.
In conclusion, ensuring the existence of a command before using it is an essential practice in Python programming. By using the methods discussed above, you can handle scenarios where a command might be unavailable or deprecated, leading to more robust and reliable code. By familiarizing yourself with these techniques, you can write Python code that gracefully handles various runtime scenarios and provides a smooth user experience.
Images related to the topic bash test if command exists
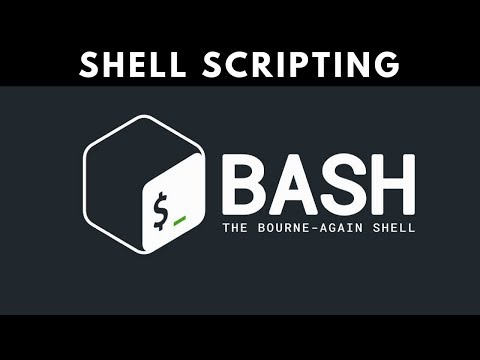
Found 24 images related to bash test if command exists theme

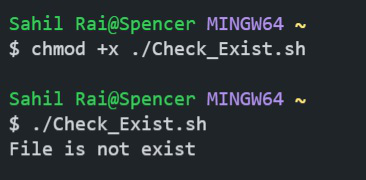
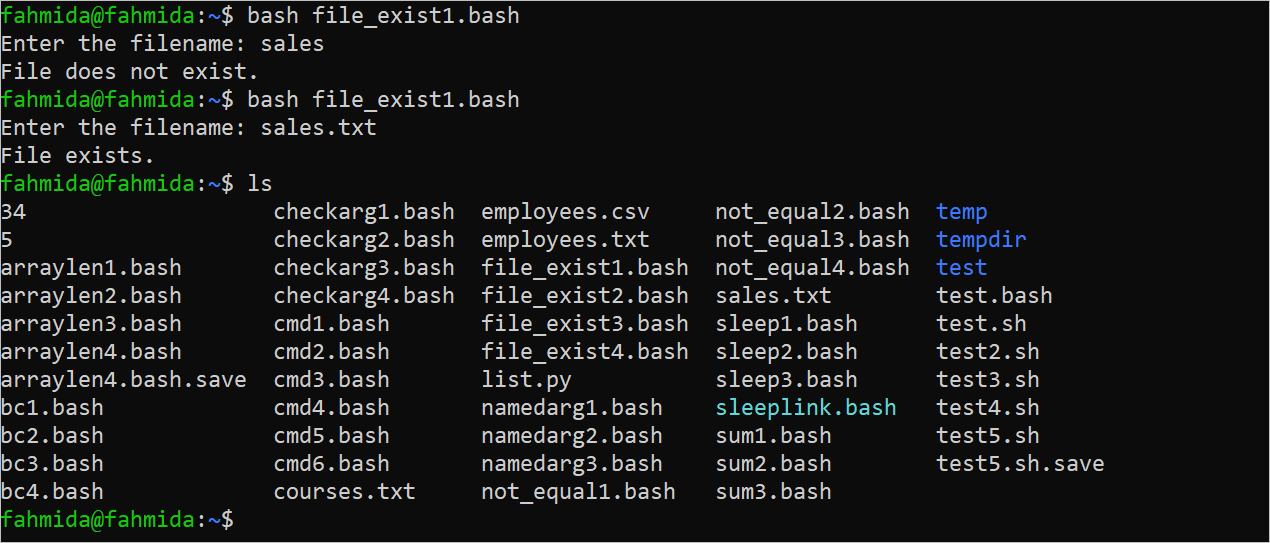
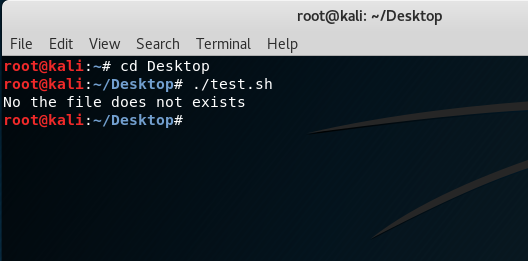
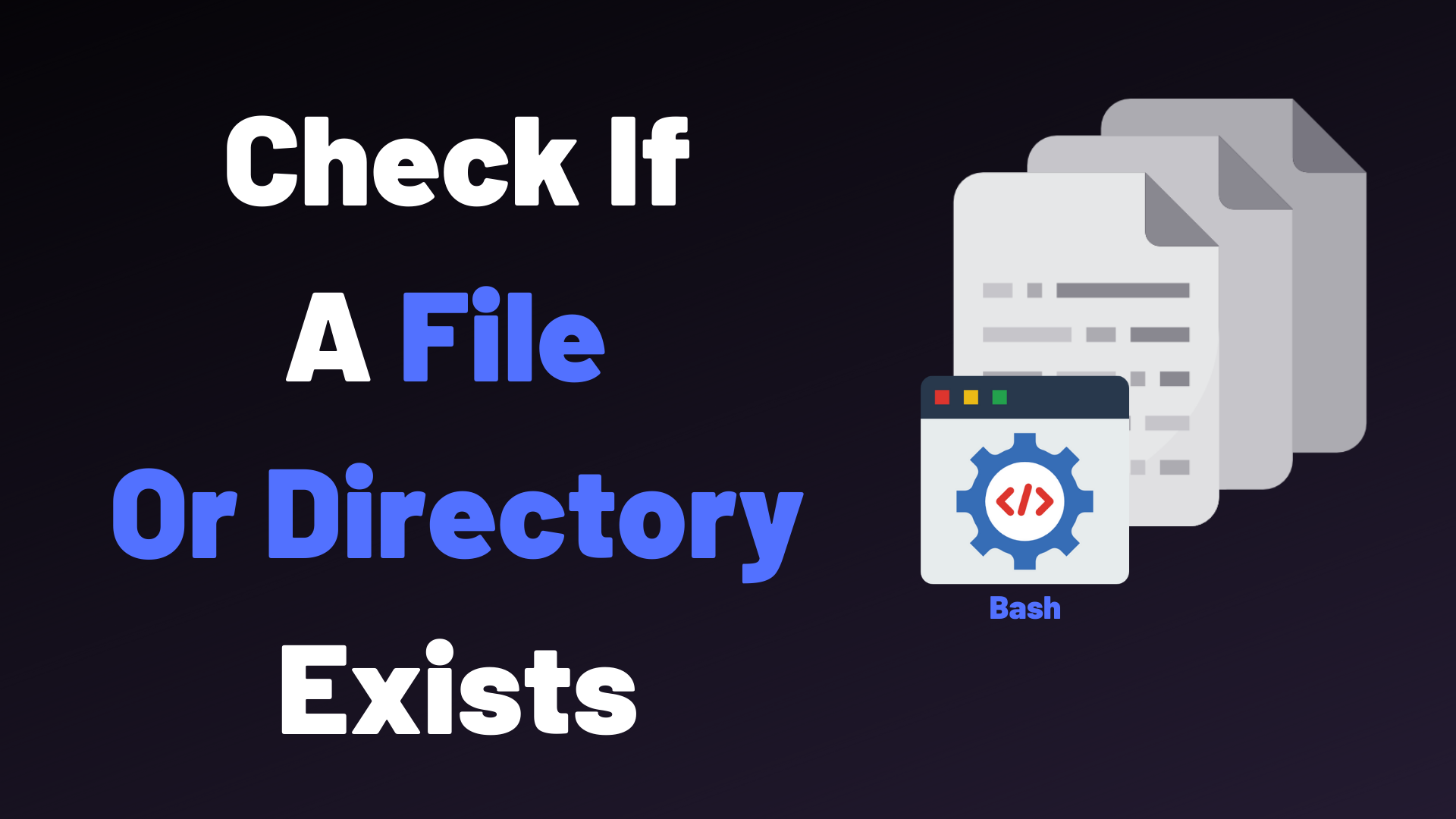

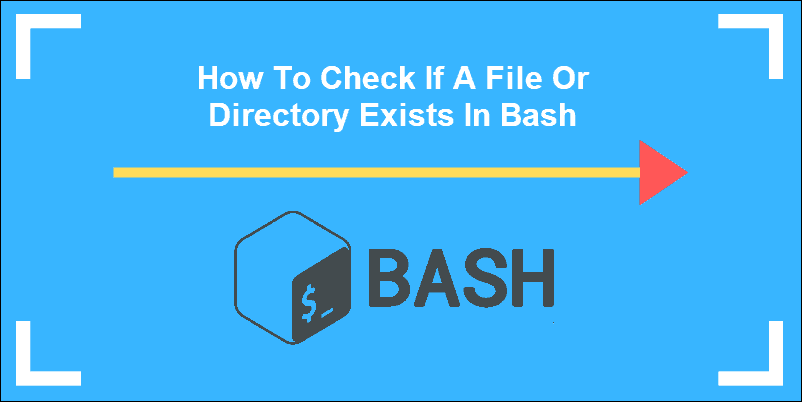
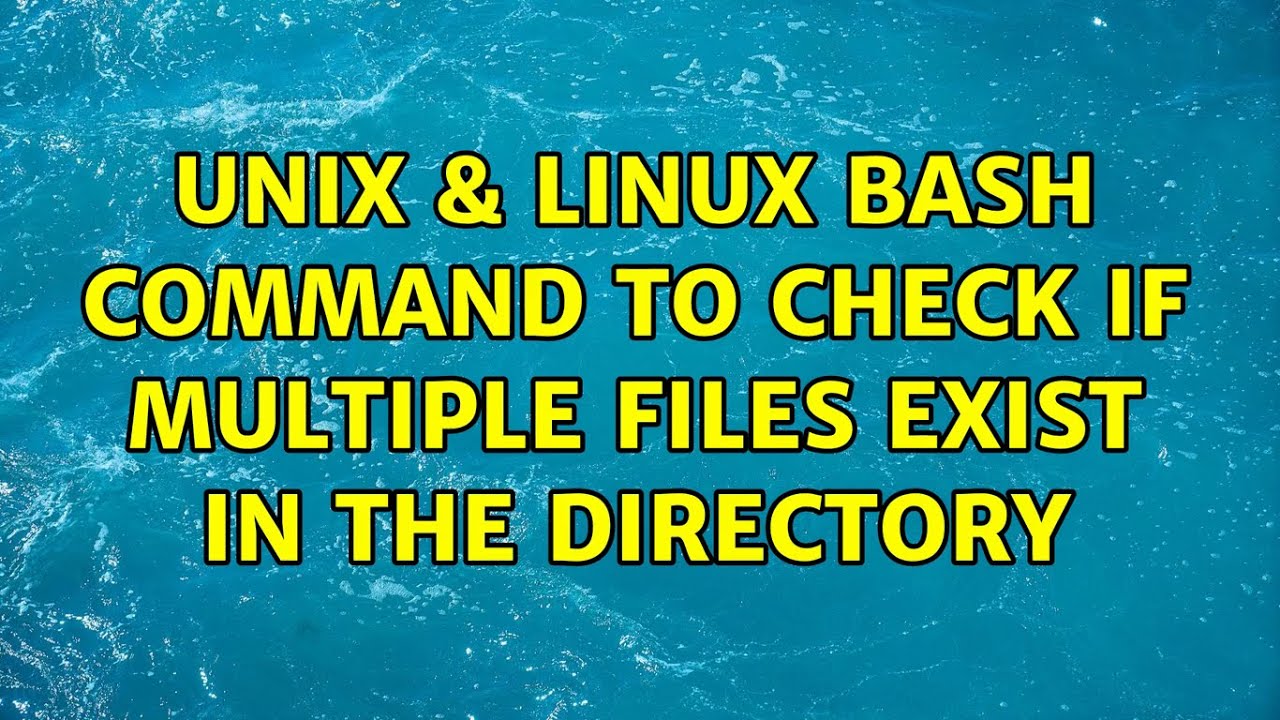
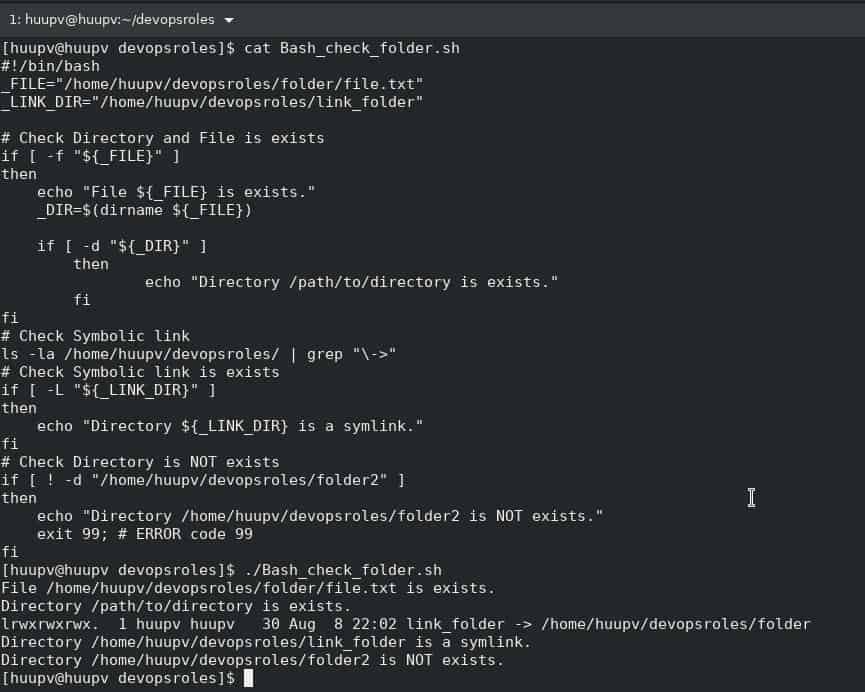

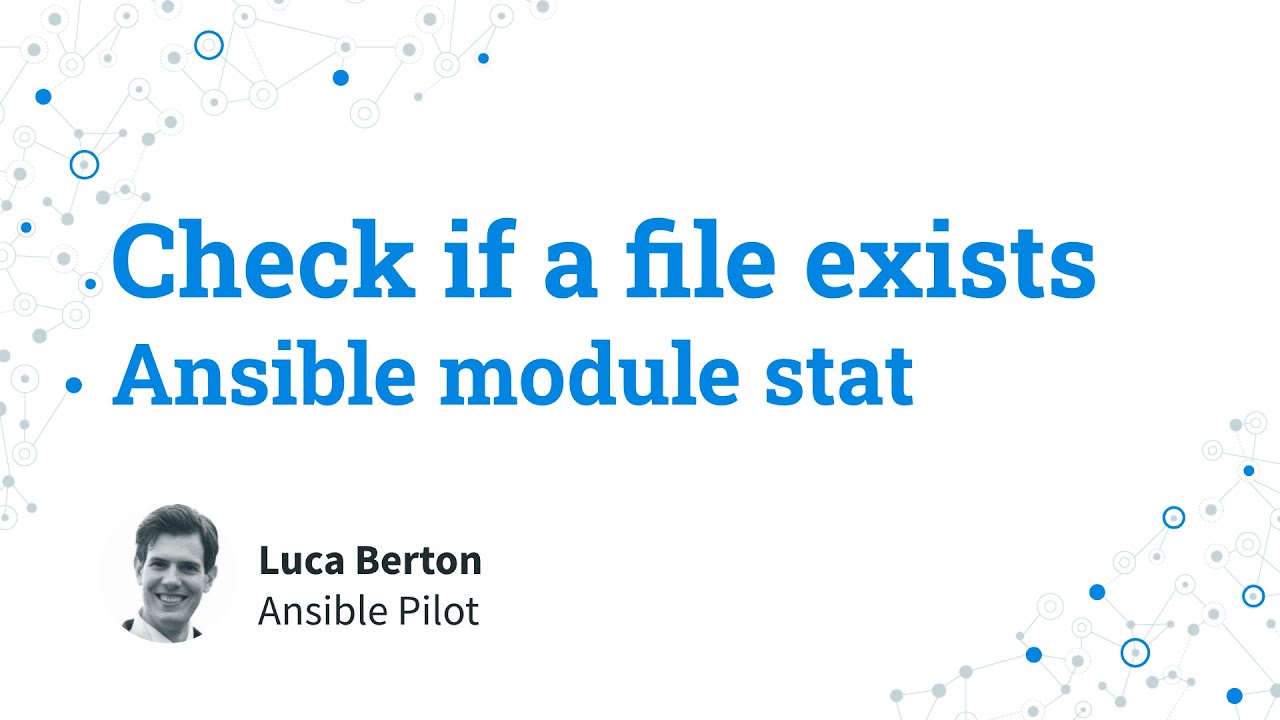
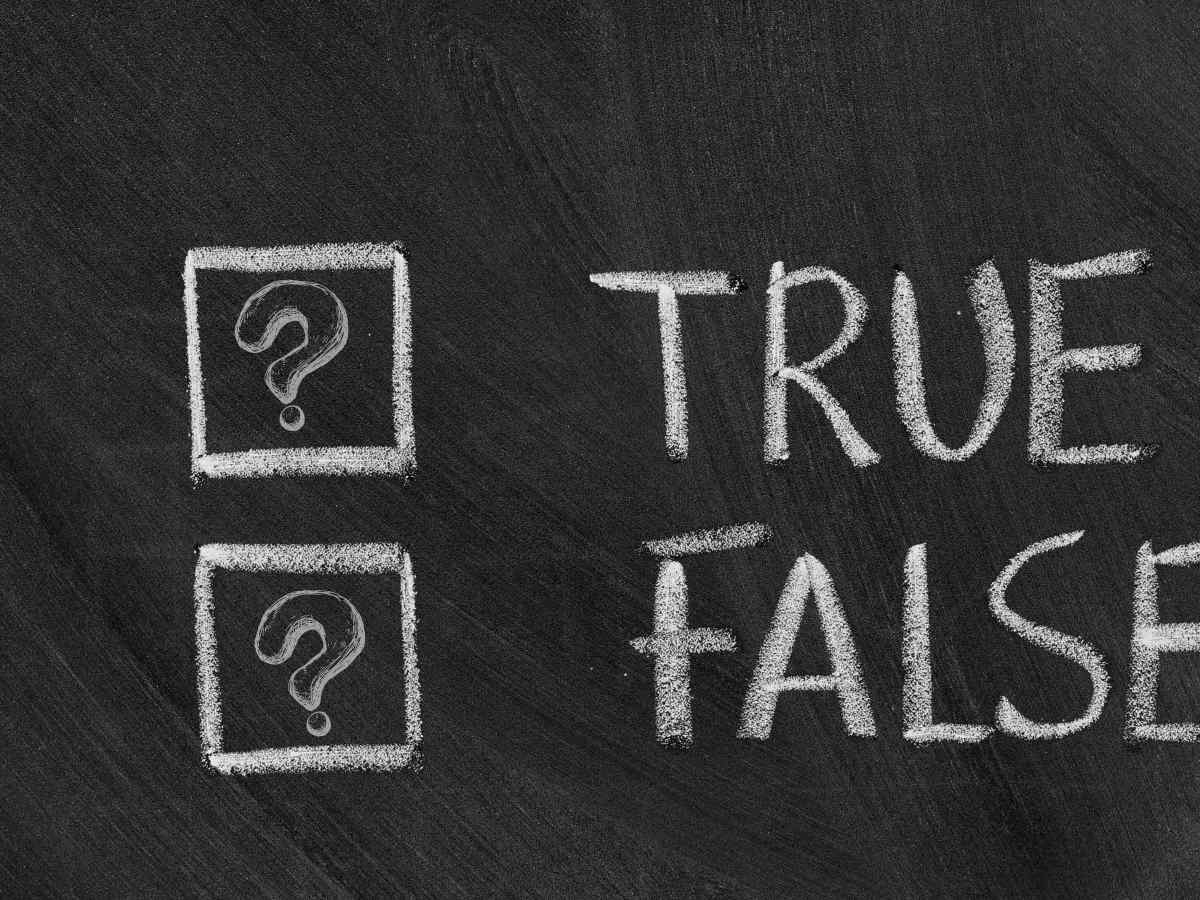
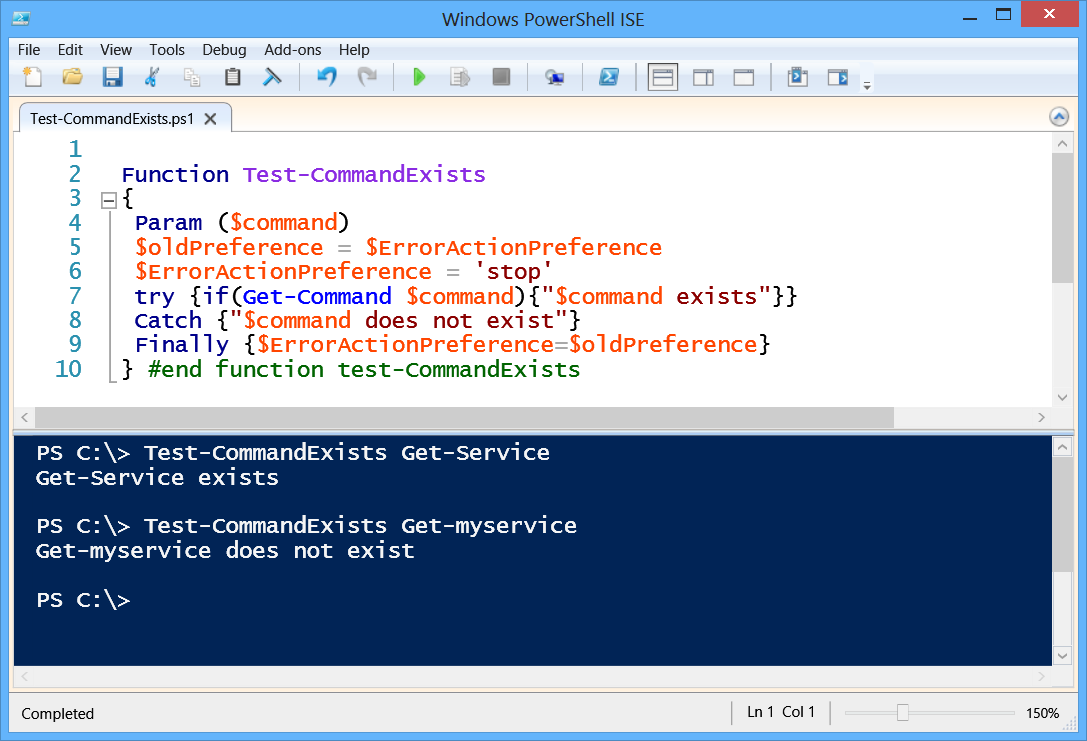

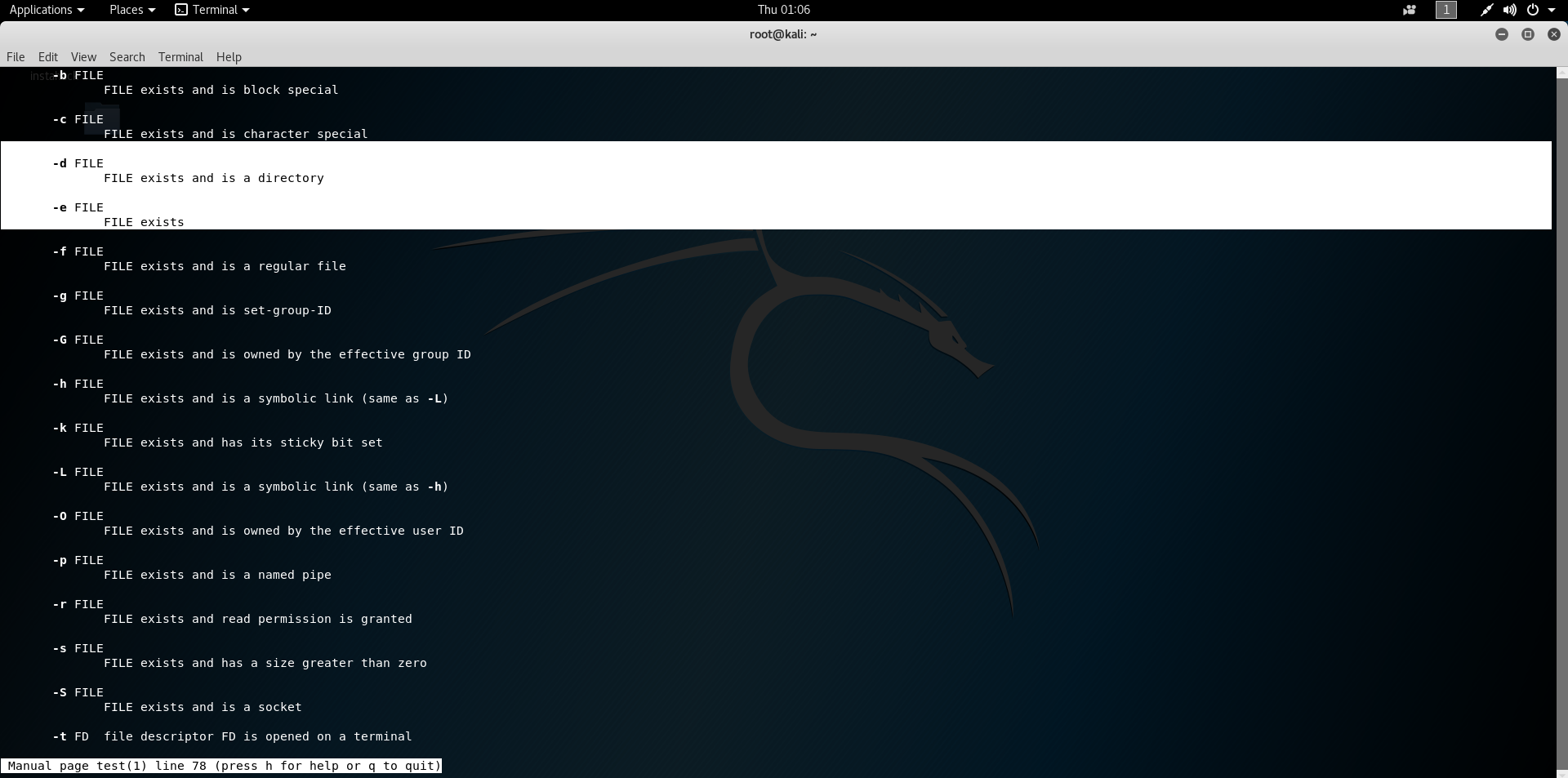
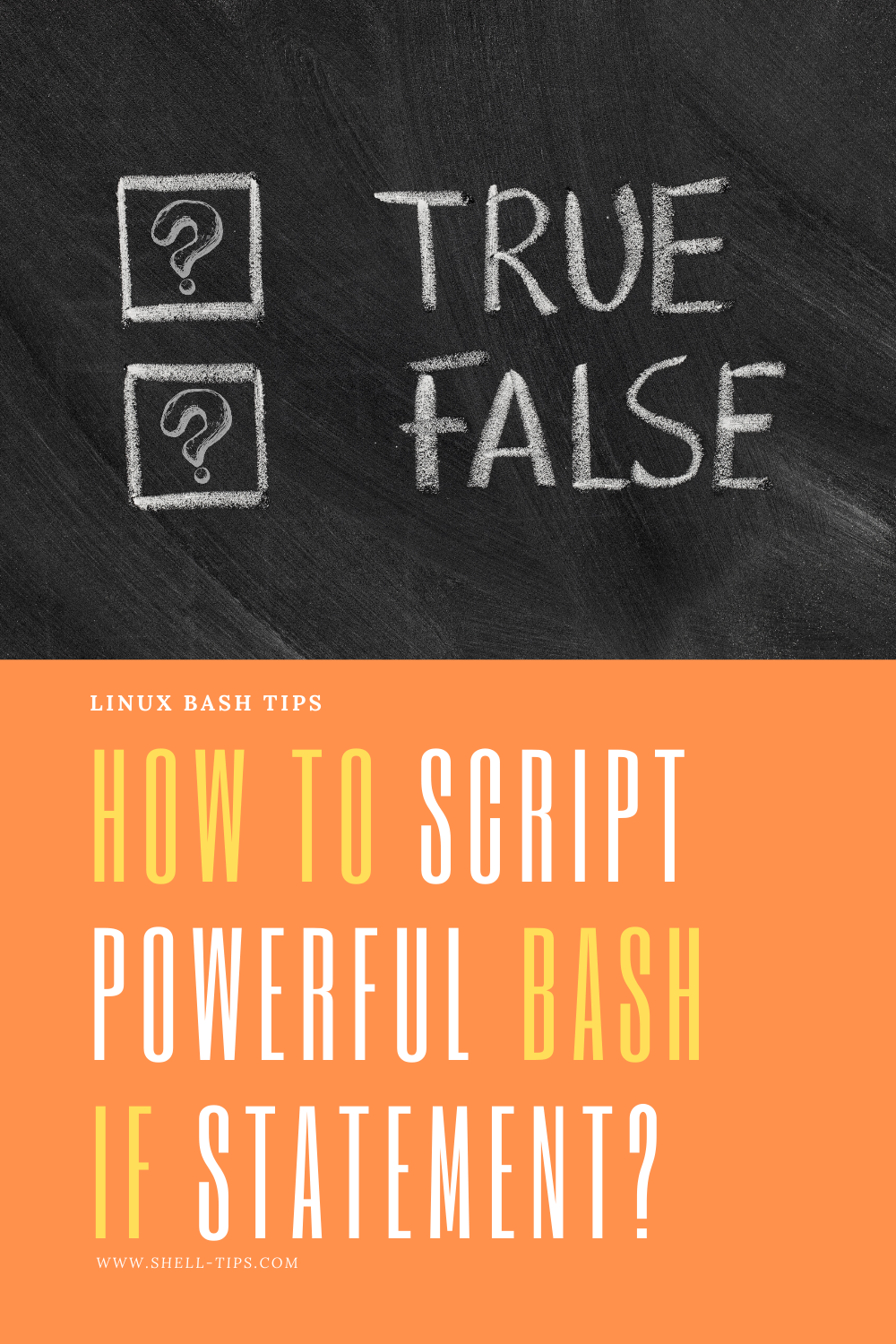
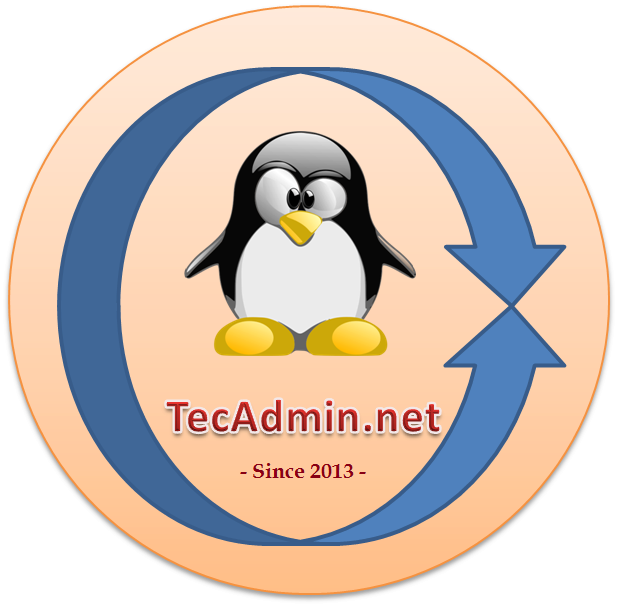
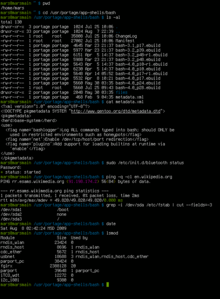
Article link: bash test if command exists.
Learn more about the topic bash test if command exists.
- Check if a Command Exists in Bash – Delft Stack
- How can I check if a program exists from a Bash script?
- How to Check if Program Exists in Shell Script – Fedingo
- Bash Shell Find Out If A Command Exists On UNIX / Linux …
- Check if a command exists revisited – Unix Stack Exchange
- How to Check if a Program Exists in Linux – TecAdmin
- How to Check if the program exists from a Bash script – Reactgo
- 7.1. Introduction to if
- Speed Test: Check the Existence of a Command in Bash and …
- Conditions in bash scripting (if statements) – Pluralsight
See more: https://nhanvietluanvan.com/luat-hoc/