Bash Split String To Array
In Bash scripting, it is often necessary to split a string into separate elements, forming an array. This allows for easy manipulation and processing of the individual components. The process of splitting a string into an array in Bash can be achieved through various techniques, each suited for different scenarios. This article aims to provide a comprehensive understanding of these techniques and how they can be employed effectively.
1. Understanding string splitting in Bash
String splitting refers to the process of dividing a string into multiple parts or elements based on a specific delimiter. The resulting parts are then stored as individual entities within an array. This splitting process is a fundamental operation in many programming languages and is equally important in Bash scripting.
2. Using the Internal Field Separator (IFS) to split a string into an array
Bash offers a built-in variable called the Internal Field Separator (IFS) that determines the delimiter used to split strings into array elements. By manipulating the value of IFS, we can split the string accordingly. For example, setting IFS to a space character (IFS=’ ‘) allows splitting the string by spaces.
3. Splitting a string with a specific delimiter into an array
In addition to the IFS method, Bash provides a variety of tools to split a string using a specified delimiter. One such tool is the “read” command, which can read input and split it into separate variables. By storing these variables within an array, we can achieve string splitting.
4. Handling multiple delimiters while splitting a string into an array
Sometimes, we encounter scenarios where a string contains multiple delimiters. Bash offers various approaches to handle such situations. One approach involves using the “tr” command to replace the multiple delimiters with a single delimiter, and then using the mentioned techniques for splitting the string into an array of elements.
5. Removing empty elements from the resulting array after splitting a string
When splitting a string into an array, it is possible to have empty elements in the resulting array. These empty elements may arise from consecutive delimiters or leading/trailing delimiters. To handle this, we can utilize the “mapfile” command (also known as “readarray”) with the “-t” option, which automatically removes trailing newlines and empty elements.
6. Splitting a string into an array and accessing its elements
Once a string has been successfully split into an array, it becomes crucial to access and manipulate its individual elements. This can be achieved by iterating over the array using a loop or accessing specific elements using their respective indices.
7. Iterating over an array created from a split string in Bash
Iterating over an array created from a split string allows us to perform actions on each element of the array. This can be done using a “for” loop or a “while” loop. By accessing each element within the loop, we can perform desired operations and achieve versatile functionality.
8. Practical examples and use cases for splitting strings into arrays in Bash
Splitting strings into arrays holds immense value in various real-world scenarios. Some practical examples include:
– Parsing command-line arguments: Splitting the input string, containing command-line arguments, into an array can help access and process each argument separately.
– Reading from a file: Splitting a file’s content, which is read into a string, into an array allows easy manipulation and analysis of individual lines or words.
– Data processing: Splitting data from comma-separated values (CSV) or tab-separated values (TSV) files into an array enables efficient data manipulation and extraction.
FAQs
Q: How can I split a string into an array in Bash?
A: There are multiple approaches to accomplish this task, such as using the IFS variable, the “read” command, or tools like “tr” and “awk”. Each technique has its own advantages depending on your specific requirements.
Q: How can I handle multiple delimiters while splitting a string into an array?
A: One approach is to use the “tr” command, which replaces multiple delimiters with a single delimiter. Alternatively, you can utilize the power of regular expressions with tools like “awk”.
Q: Can I remove empty elements from the array after splitting a string?
A: Yes, you can remove empty elements from the resulting array by using the “mapfile” command with the “-t” option, which automatically eliminates trailing newlines and empty elements.
Q: How can I access individual elements of the array created from a split string?
A: You can access individual elements of the array by iterating over it using a loop, such as a “for” loop or a “while” loop, and accessing the elements through their respective indices.
Q: What are some practical use cases for splitting strings into arrays in Bash?
A: Splitting strings into arrays is valuable in scenarios such as parsing command-line arguments, reading from files, and processing data from CSV or TSV files.
In conclusion, splitting a string into an array in Bash is a crucial skill for efficient data manipulation and processing. By employing the different techniques and tools available in Bash, you can harness the power of arrays to enhance your scripting capabilities and handle complex scenarios with ease.
Splitting Strings Into Arrays – Bash – Linux
Keywords searched by users: bash split string to array Bash split string into array, Bash save output to array, Bash array length, Readarray bash, Bash script split string by space, Read file to array Bash, Split in cshell, Bash loop array
Categories: Top 57 Bash Split String To Array
See more here: nhanvietluanvan.com
Bash Split String Into Array
Bash scripting, a powerful tool in the Linux environment, offers a wide range of functionalities to automate tasks and improve productivity. One frequently encountered task is splitting a string into an array, which enables better manipulation and processing of data. In this article, we will explore various techniques to split a string into an array in Bash, going into depth to ensure a comprehensive understanding of the topic.
1. Using readarray command:
The readarray command allows for easy conversion of a string into an array in Bash. This command reads lines from standard input and assigns them to elements of an array. To split a string into an array using readarray, you can redirect the string into the command or use a process substitution.
Here’s an example using redirection:
“`
string=”Hello World”
readarray -t array <<< "$string"
```
And here's an example using process substitution:
```
string="Hello World"
readarray -t array < <(echo "$string")
```
The `-t` option is used to trim newlines from each line read into the array, if desired.
2. Using IFS (Internal Field Separator):
IFS, the Internal Field Separator, determines how Bash splits words into fields. By changing the value of IFS, you can split a string into an array using a specific delimiter. After splitting, each field becomes an element of the array.
Here's an example splitting a string using a space as the delimiter:
```
string="Hello World"
IFS=" " read -ra array <<< "$string"
```
In this example, the `-r` option ensures that backslashes are not interpreted. The `-a` option assigns the inputs to an array.
3. Using string manipulation and a loop:
You can also split a string into an array by utilizing string manipulation operations like substring extraction, regex matching, and loop constructs. While this approach may require more code, it offers flexibility to handle complex string splitting scenarios.
Here's an example utilizing substring extraction through iteration:
```bash
string="Hello World"
array=()
while [[ $string ]]; do
array+=( "${string%% *}" )
string=${string#* }
done
```
In this example, the substring `${string%% *}` extracts the substring before the first space, and `${string#* }` removes the substring before the first space.
FAQs (Frequently Asked Questions):
Q1: Can we split a string into an array using a specific character as the delimiter?
A1: Yes, by utilizing the IFS (Internal Field Separator) technique mentioned earlier, you can split a string into an array using any character as the delimiter.
Q2: Is it possible to split a string into an array without trimming leading or trailing whitespaces?
A2: Yes. By omitting the `-t` option in the readarray command or using `read -a` without the `-r` option, the leading or trailing whitespaces will not be trimmed.
Q3: What if we want to split a string into an array by multiple delimiters?
A3: One approach would be to use the regex matching technique. You can define a regular expression pattern that matches all the desired delimiters and use `grep` or `sed` to split the string accordingly.
Q4: Can we split a string into an associative array?
A4: No, Bash does not support associative arrays for direct string splitting. However, you can associate values with specific keys after splitting a string into a regular array.
Q5: Are there any performance considerations when splitting strings into arrays?
A5: While splitting small to medium-sized strings won't have a noticeable impact on performance, splitting large strings or processing a large number of strings may consume more system resources. It is advisable to assess the specific use case and optimize the code accordingly if performance is a concern.
In conclusion, the ability to split a string into an array in Bash is an essential skill for efficiently handling and manipulating data within scripts. By using techniques like the readarray command, IFS manipulation, or string manipulation with loops, you can effectively split strings into arrays and perform a wide range of operations. Understanding these techniques and their appropriate use cases will enable you to write more powerful and flexible Bash scripts.
Bash Save Output To Array
The first step in saving output to an array is to understand what an array is. In Bash, an array is a variable that can hold multiple values simultaneously. Each value in the array is assigned a unique index, starting from zero. This allows for efficient storage and retrieval of data.
To save output to an array, we can use command substitution, a feature of Bash that allows us to capture the output of a command and use it as part of a larger expression. Using command substitution, we can assign the output of a command to a variable, and then populate an array with the contents of that variable.
Let’s take a look at a simple example to illustrate the process:
“`bash
output=$(ls) # Save output of ‘ls’ command to ‘output’ variable
arr=($output) # Populate array ‘arr’ with contents of ‘output’
“`
In this example, we are using the `ls` command to list the contents of the current directory. The output of the command is saved to the `output` variable using command substitution. We then assign the contents of `output` to the `arr` array.
Now that we have saved the output to an array, we can easily access and manipulate the data. Let’s explore some common operations:
– Accessing array elements:
– Individual elements can be accessed using the index within square brackets, starting from zero. For example, `${arr[0]}` would retrieve the first element in the array.
– Alternatively, we can access all elements in the array using the `[*]` or `[@]` special characters. For example, `${arr[*]}` would return all elements of the array as a single string.
– Looping through array elements:
– We can use a for loop to iterate over each element in the array. Here’s an example:
“`bash
for element in “${arr[@]}”; do
echo “$element”
done
“`
This loop will print each element of the array on a new line.
– Modifying array elements:
– To modify an element in the array, we can simply assign a new value to the corresponding index. For example, `${arr[0]}=”new value”` would change the first element in the array.
– Finding the length of an array:
– The length of the array (i.e., the number of elements it contains) can be obtained using the `${#arr[@]}` syntax. For example, `length=${#arr[@]}` would assign the length of the array to the variable `length`.
Now that we have covered the basics of saving output to an array in Bash, let’s address some frequently asked questions:
Q: Can I save the output of a command directly to an array without using a variable?
A: Yes, you can save the output of a command directly to an array. Just substitute the variable assignment with the name of the array. For example, `arr=($(ls))` would save the output of `ls` directly to `arr`.
Q: Can I save multi-line output to an array?
A: Yes, Bash arrays can hold multi-line output. Each line will be treated as a separate element in the array.
Q: How do I delete an element from an array?
A: You can delete an element by using the `unset` command followed by the array index. For example, `unset arr[2]` would delete the third element of the array `arr`.
Q: Can I use commands with pipe (|) in command substitution to save output to an array?
A: Yes, you can use commands with pipe in command substitution to save output to an array. For example, `arr=($(ls | grep “*.txt”))` would save the output of `ls | grep “*.txt”` to `arr`.
Q: Can I save output to an associative array?
A: No, Bash does not support associative arrays. It only supports indexed arrays.
Saving output to an array in Bash provides a flexible and efficient way to store and manipulate data. By understanding the basics and mastering the different operations, you can leverage the power of arrays in your Bash scripts. Experiment, practice, and explore the possibilities to maximize the potential of this powerful Bash feature.
Bash Array Length
Bash arrays are a powerful feature of the Bash shell scripting language that allow you to store multiple values in a single variable. With arrays, you can easily perform operations on a group of elements, such as searching, sorting, or manipulating data. As arrays grow in complexity, it becomes essential to know the length of an array to control its behavior effectively. This article explores the concept of Bash array length in detail, addressing common questions and offering practical examples.
Understanding Bash Arrays:
Before diving into array length, let’s briefly review Bash arrays. Array elements are assigned to a variable using the `=` operator, surrounded by parentheses and separated by spaces. Here’s an example of how you can declare an array and assign values to it:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
“`
In the above example, the array `my_array` is created, and its elements are assigned as strings. It is worth noting that Bash arrays can store not only strings but also integers, variables, and even other arrays.
Calculating Bash Array Length:
To find the length of an array in Bash, you need to rely on a few techniques. There are multiple ways to determine the length of an array, and we will explore three common approaches.
1. Using the `${#array[@]}` Syntax:
One straightforward method to obtain the length of an array is by using the `${#array[@]}` syntax. The `${#array[@]}` construct returns the number of elements in the given array. Here’s an example to illustrate this:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
length=${#my_array[@]}
echo “The length of the array is: $length”
“`
The output will be: `The length of the array is: 4`.
2. Using the `${#array[*]}` Syntax:
Similar to the previous method, you can also use the `${#array[*]}` syntax to obtain the length of an array. The only difference is that the `[*]` notation treats the entire array as a single element. Here’s an example showcasing this syntax:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
length=${#my_array[*]}
echo “The length of the array is: $length”
“`
The output in this case will also be: `The length of the array is: 4`.
3. Iterating Over Array Elements:
Another indirect method to determine the length of an array is by looping over its elements. By iterating over each element and keeping a count, you can find the length. Here’s a script to demonstrate this approach:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
length=0
for element in “${my_array[@]}”; do
((length++))
done
echo “The length of the array is: $length”
“`
This script also yields an output of `The length of the array is: 4`.
Frequently Asked Questions (FAQs):
Q1. Can I assign a value directly to an array element during declaration?
Yes, you can assign a value directly to a specific array element during declaration. Here’s an example:
“`bash
my_array[0]=”apple”
my_array[1]=”banana”
“`
Q2. Can I add elements to an array after its declaration?
Yes, you can add elements to an array using the `+=` operator. Here’s an example:
“`bash
my_array=(“apple” “banana”)
my_array+=(“cherry” “date”)
“`
Q3. How do I access a specific element from an array?
To access a specific element from an array, you can use the index of that element within square brackets. Here’s an example:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
echo “${my_array[2]}” # Output: cherry
“`
Q4. Can I find the length of a multidimensional array?
No, Bash does not provide any direct method to determine the length of a multidimensional array. However, you can calculate the length of each dimension separately.
Q5. How do I remove an element from an array?
To remove an element from an array, you can use the `unset` command. Here’s an example:
“`bash
my_array=(“apple” “banana” “cherry” “date”)
unset my_array[1]
“`
In conclusion, understanding the length of a Bash array is crucial for effective array management. Whether you’re working with small or large arrays, knowing the number of elements helps you manipulate data efficiently. By utilizing syntax like `${#array[@]}` or iterating over elements, you can calculate the length of an array effortlessly. With these techniques at your disposal, you can unlock the full potential of Bash arrays in your shell scripts.
Images related to the topic bash split string to array
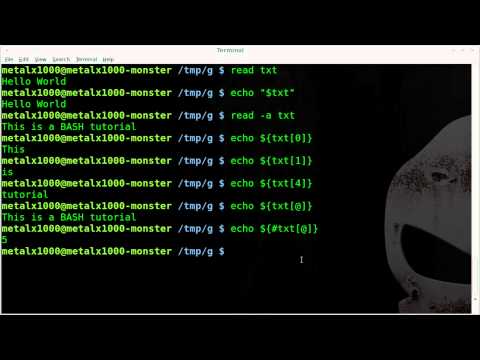
Found 44 images related to bash split string to array theme
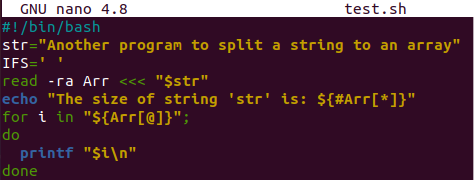
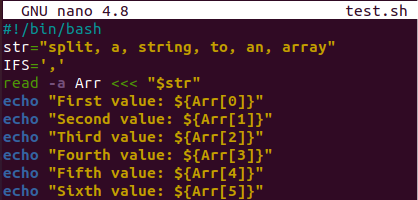
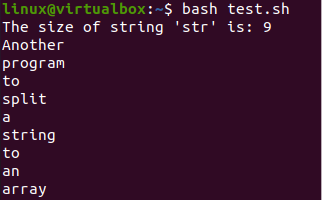
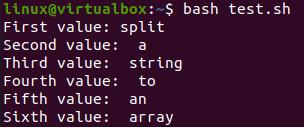
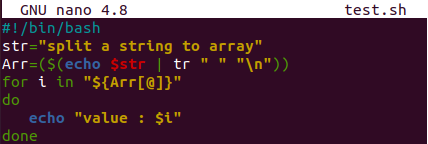

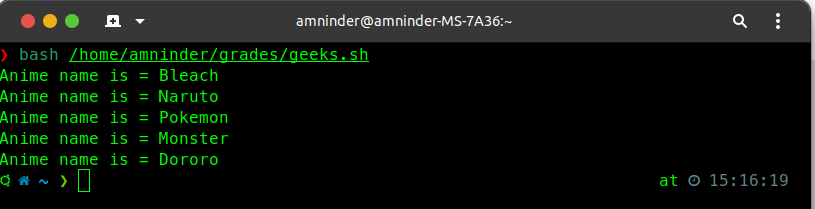
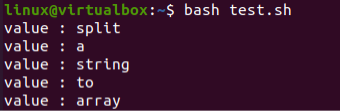
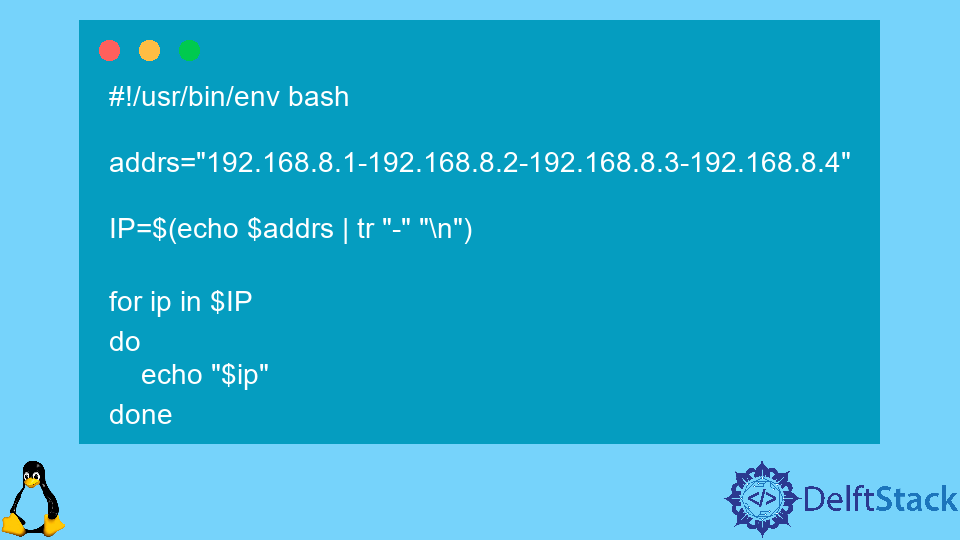


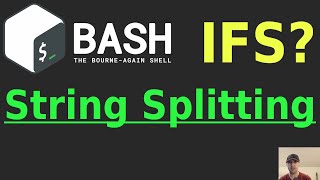
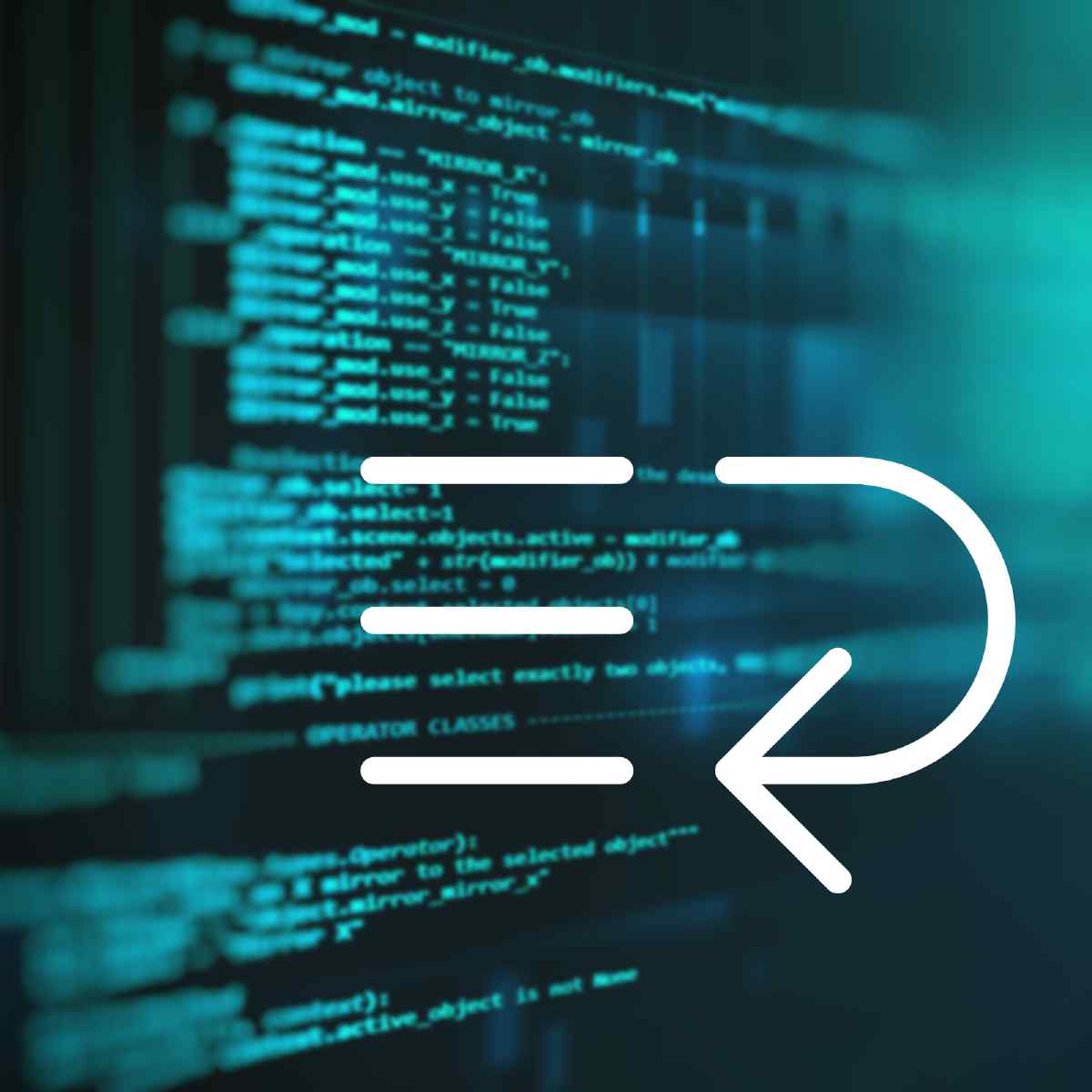
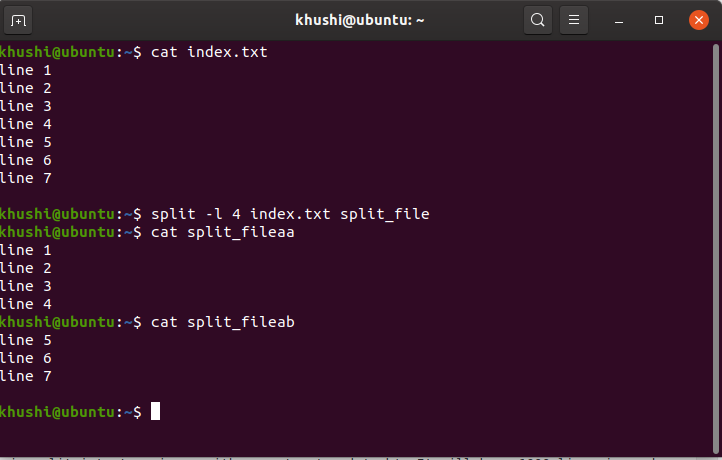
![How to Split String into Array in Bash [Easiest Way] How To Split String Into Array In Bash [Easiest Way]](https://linuxhandbook.com/content/images/2020/08/bash-beginner-series.jpg)

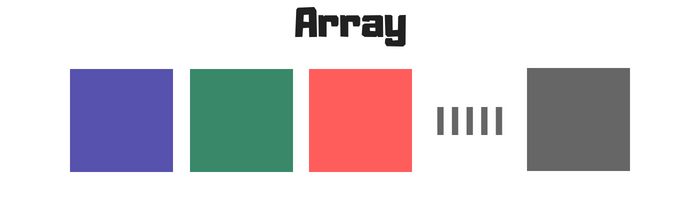
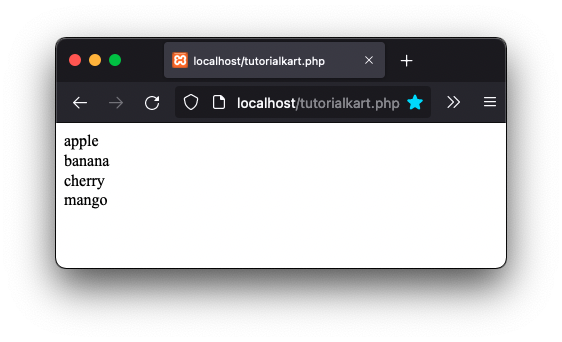
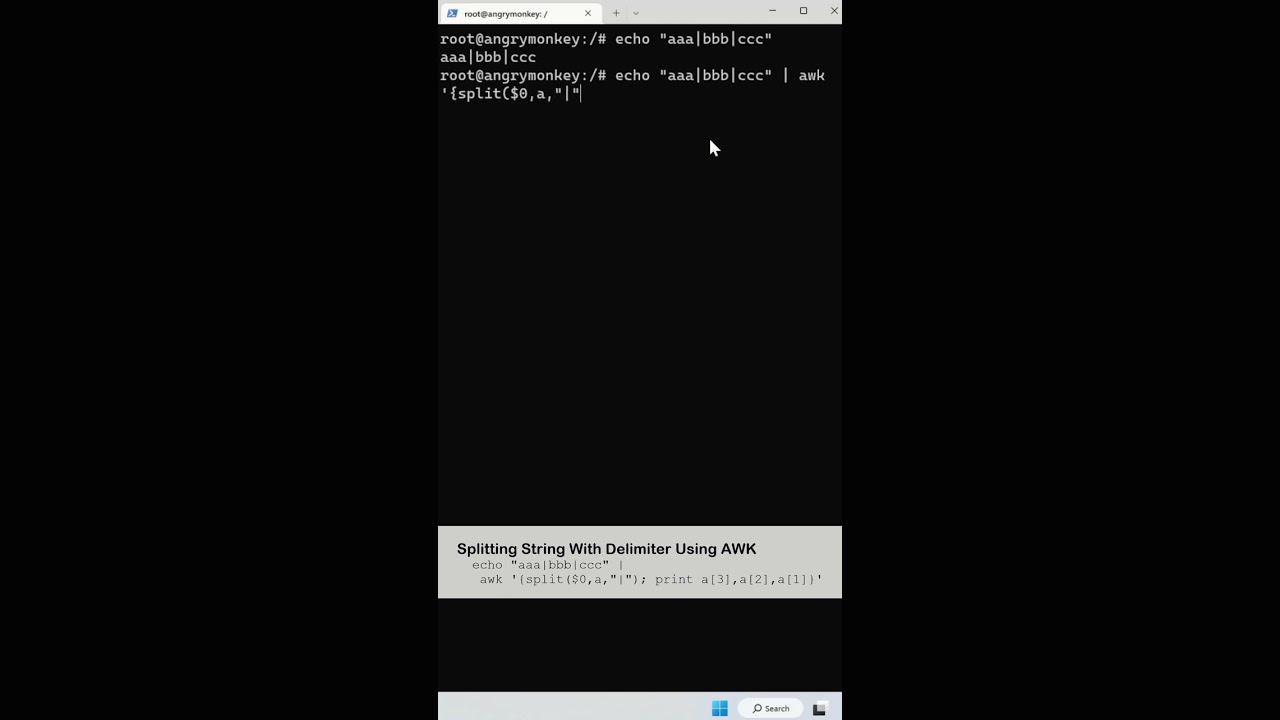
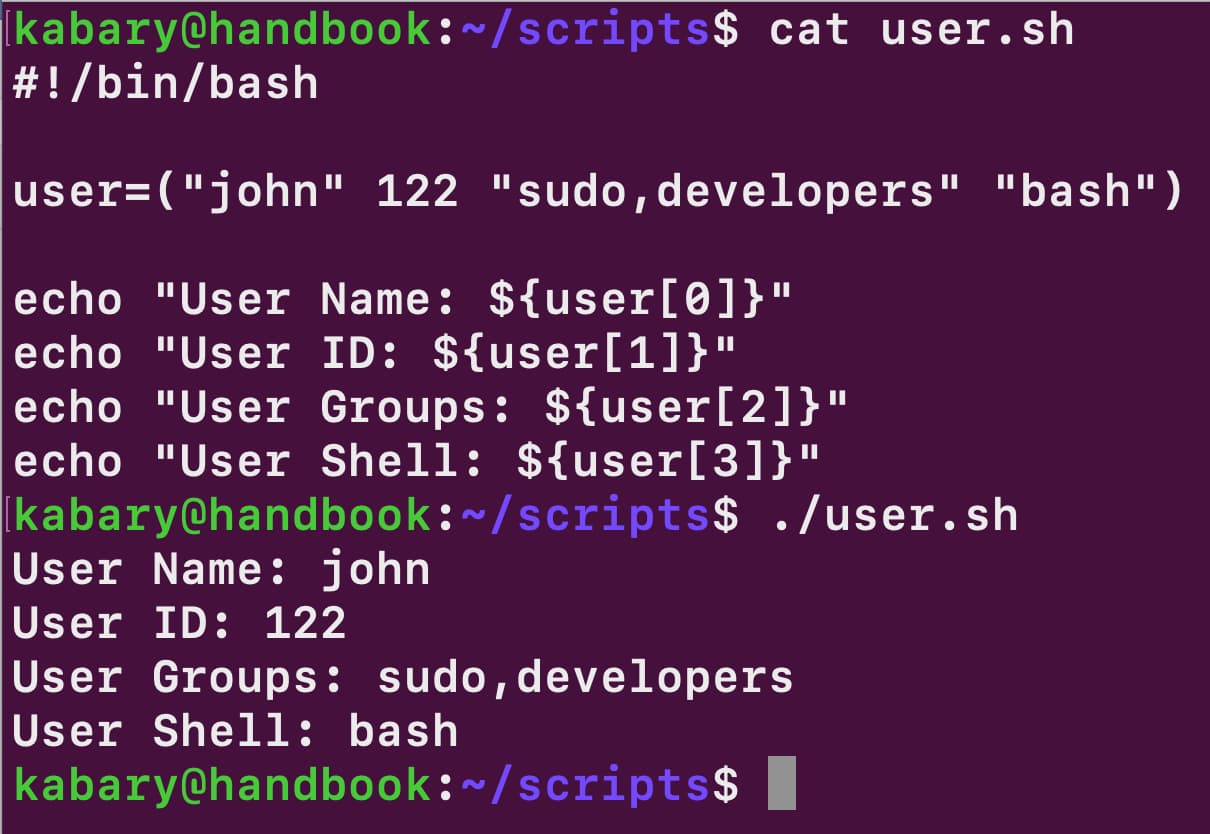
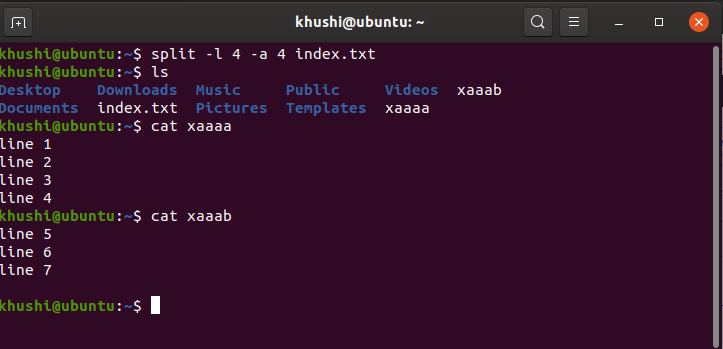
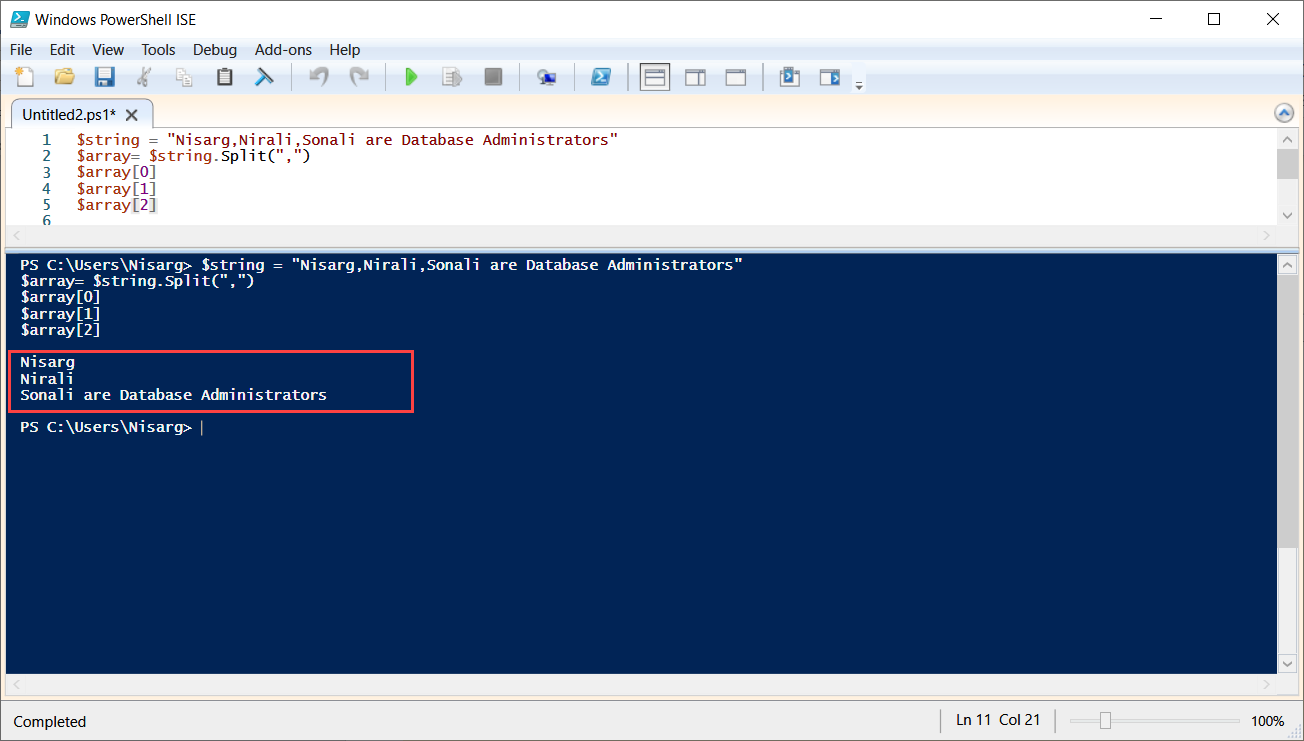
![Delete elements of one array from another array in bash [SOLVED] | GoLinuxCloud Delete Elements Of One Array From Another Array In Bash [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/bash-remove-array-elements.jpg)



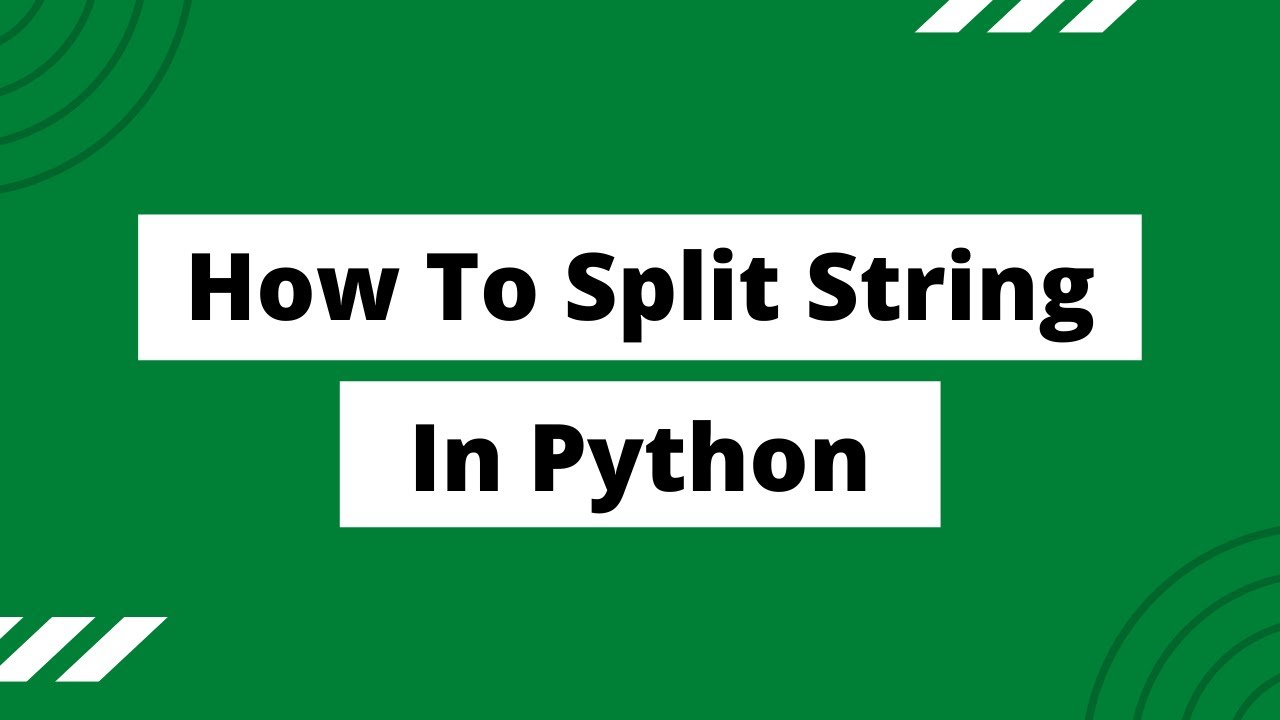
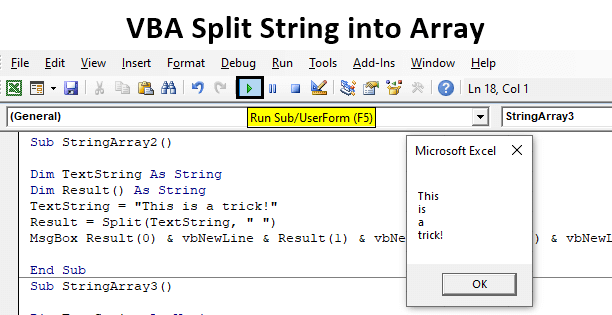

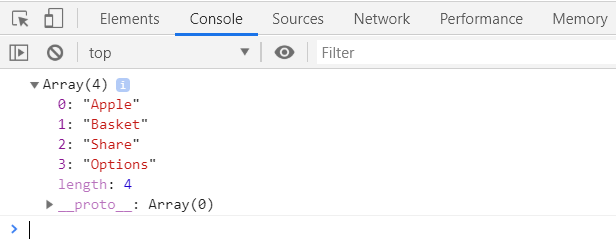
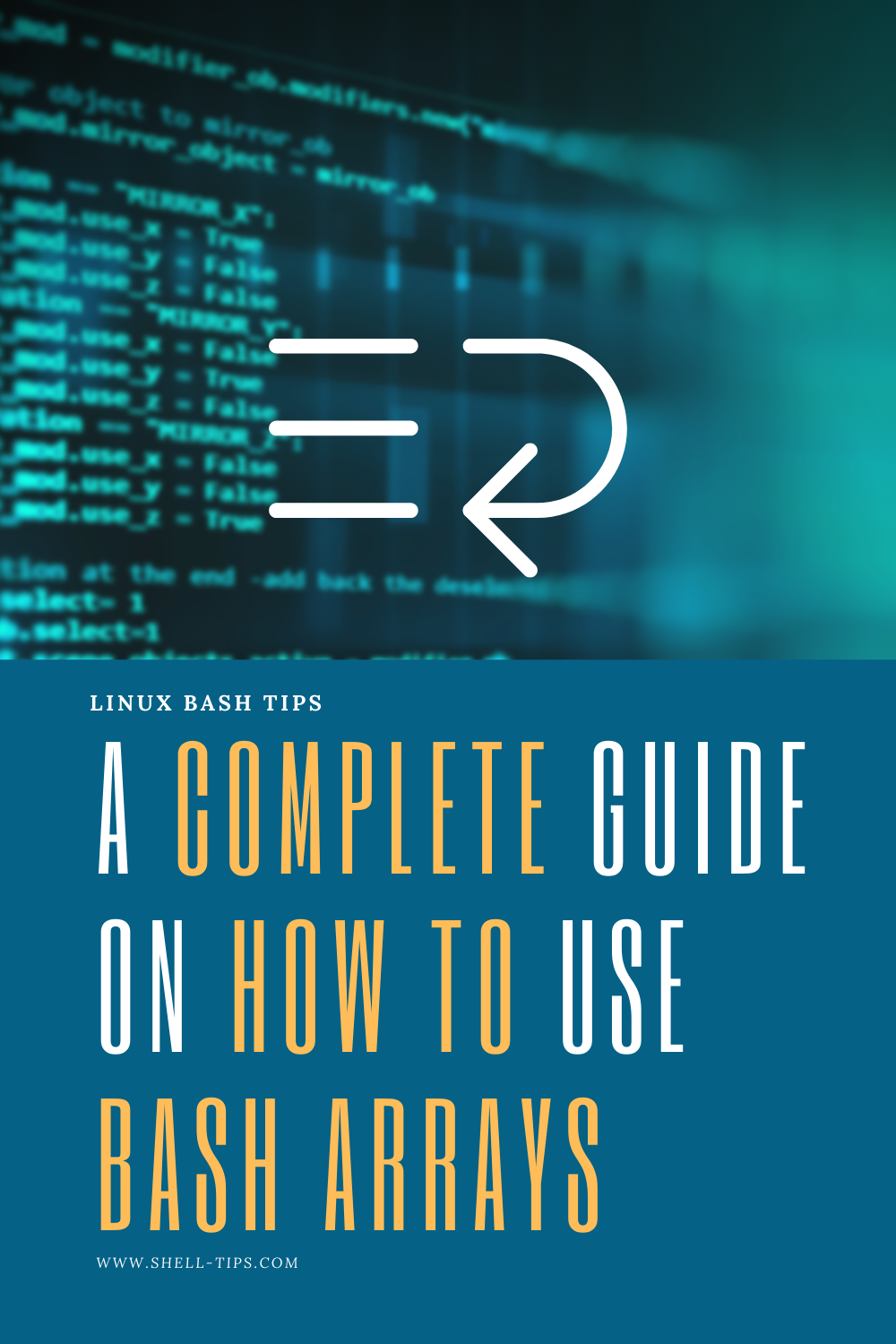
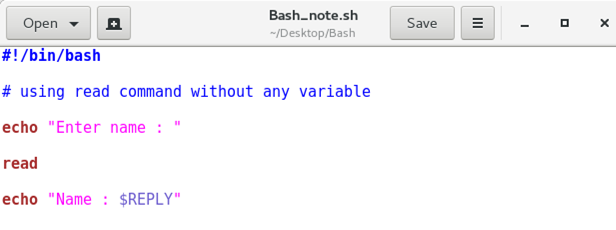
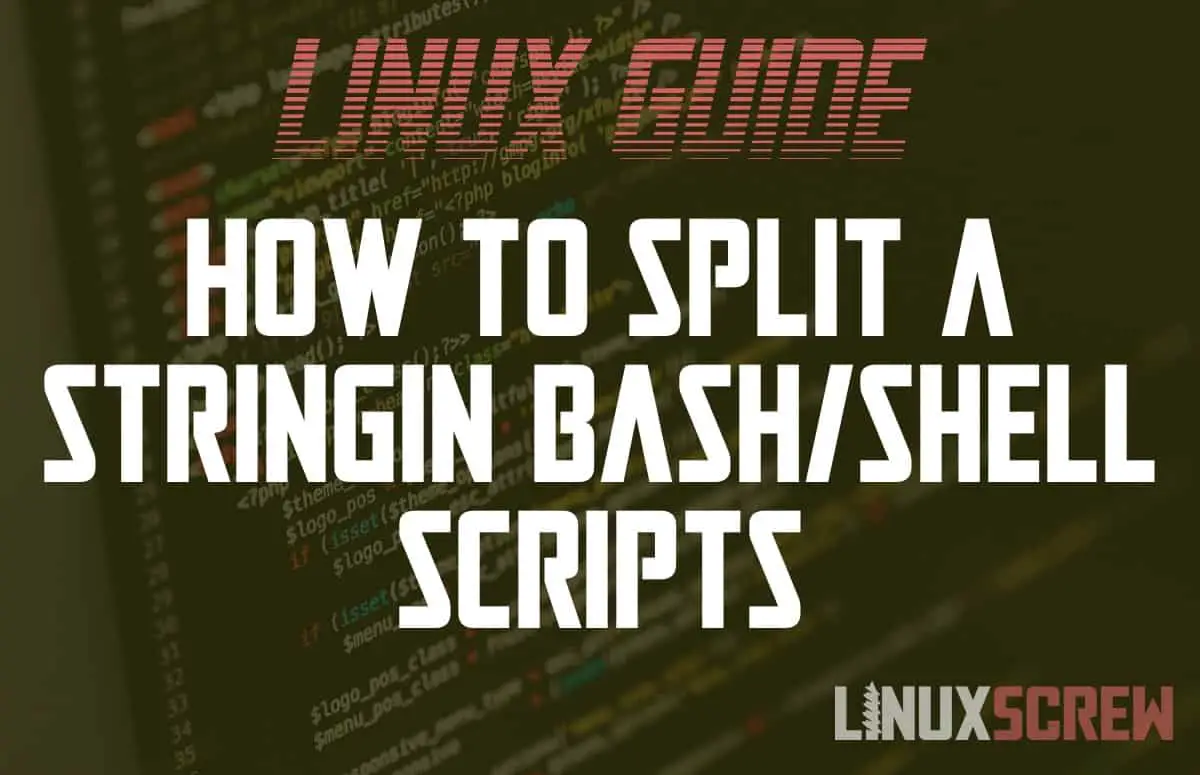
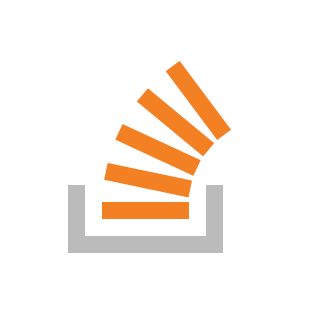
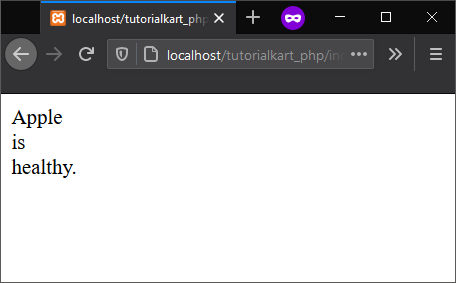
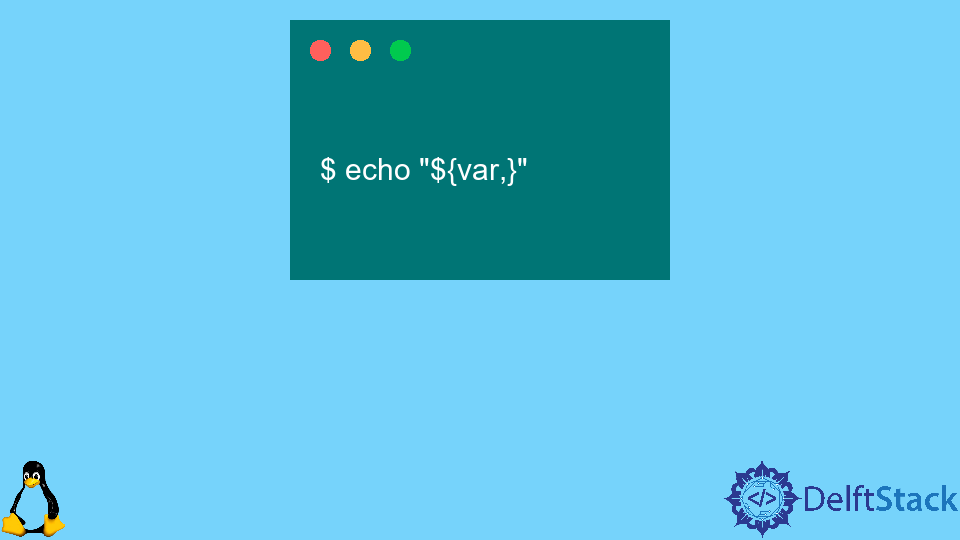
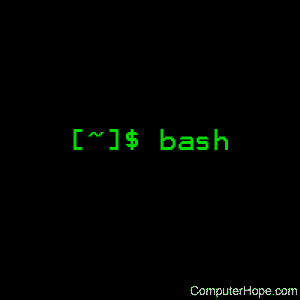

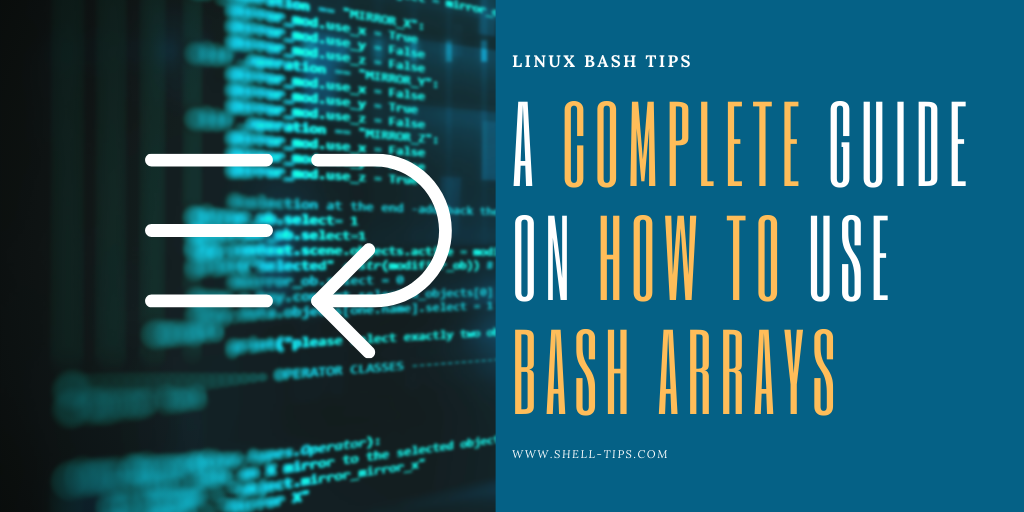

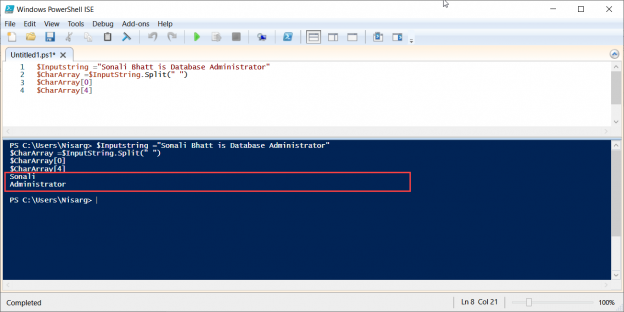
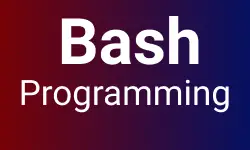

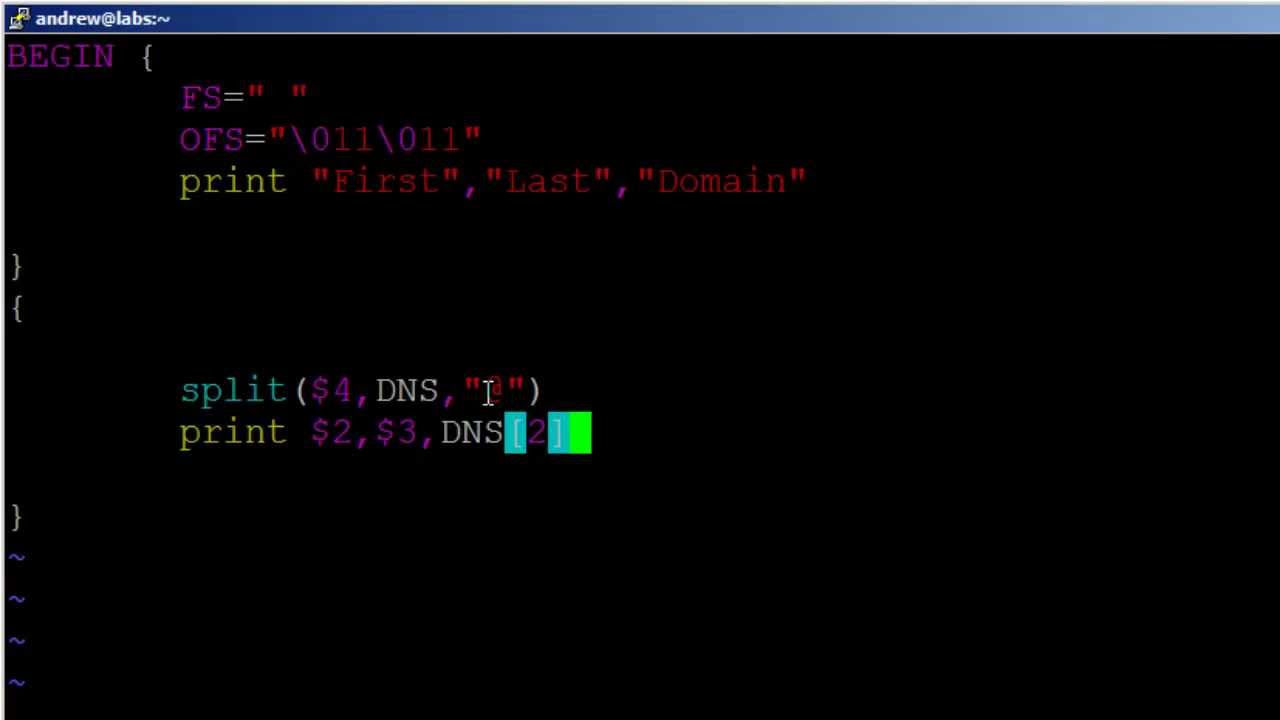


Article link: bash split string to array.
Learn more about the topic bash split string to array.
- How to split a string into an array in Bash? – Stack Overflow
- Bash split string into array using 4 simple methods
- Bash Split String into Array – Linux Hint
- How to Split String in Bash Script – Linux Handbook
- Split String Into Array in Bash – Delft Stack
- Bash Split String – Javatpoint
- Split string into an array in Bash – Essential Programming Books
- Bash Tutorial => Split string into an array in Bash – RIP Tutorial
- Splitting Strings by a Delimiter with IFS or Bash’s String Replace
- Bash Scripting – Split String – GeeksforGeeks
See more: https://nhanvietluanvan.com/luat-hoc/