Bash Check If Variable Is Set
Bash, or the Bourne Again SHell, is a popular command-line interpreter for Unix-like operating systems. It provides a powerful scripting language that allows developers to automate tasks and write robust scripts. In Bash, variables play a crucial role in storing and manipulating data. However, in certain situations, it becomes necessary to check whether a variable is set or not. This article will explore various methods to determine if a variable is set in Bash.
What does it mean for a variable to be “set” in Bash?
Before we dive into the methods of checking if a variable is set, it’s important to understand what it means for a variable to be “set” in Bash. In simple terms, a variable is considered set if it has been assigned a value. This value could be anything, such as a string, a number, or even an empty value. On the other hand, an unset variable is one that has not been assigned any value at all.
Checking if a variable is set using the -v option
One way to check if a variable is set in Bash is by using the -v option in the test command. The test command, denoted by the brackets ([ ]), allows you to perform various tests and conditionals in Bash scripts. To use the -v option, simply wrap the variable inside double square brackets and check if it is true or false:
“`
if [[ -v variable ]]; then
echo “Variable is set”
else
echo “Variable is unset”
fi
“`
If the variable is set, the script will print “Variable is set.” Otherwise, it will print “Variable is unset.”
Using the -z option to check if a variable is set and empty
In addition to checking if a variable is set, you may also need to determine if it is both set and empty. This can be achieved using the -z option in the test command. Here’s an example:
“`
if [[ -z $variable ]]; then
echo “Variable is set but empty”
else
echo “Variable is either unset or non-empty”
fi
“`
If the variable is set and empty, the script will display “Variable is set but empty.” Otherwise, it will indicate that the variable is either unset or non-empty.
Testing if a variable is set using the -n option
Another way to check if a variable is set in Bash is by using the -n option in the test command. This option tests whether the length of the string inside the variable is non-zero. Here’s an example:
“`
if [[ -n $variable ]]; then
echo “Variable is set”
else
echo “Variable is unset”
fi
“`
If the variable is set, regardless of its content, the script will output “Variable is set.” Otherwise, it will indicate that the variable is unset.
The difference between testing if a variable is set and testing if it is empty
It’s important to clarify the distinction between testing if a variable is set and testing if it is empty. When checking if a variable is set, you are only concerned with whether the variable has been assigned any value at all. On the other hand, when testing if a variable is empty, you specifically want to determine if the variable has been assigned an empty value. Two different options, -z and -n, are used for these respective checks.
Checking if a variable is set using the -o nounset option
In Bash, you can also enable the nounset option to treat unset variables as errors. This means that if you attempt to use an unset variable, a script will terminate with an error message. To enable this option, use the following command:
“`
set -o nounset
“`
After enabling the nounset option, any unset variable will result in an error. This can be useful for ensuring variables are always initialized before use, preventing potential issues or bugs.
Alternatives to checking if a variable is set in Bash
Apart from the methods discussed above, there are a few alternatives to check if a variable is set in Bash. Here are some common scenarios:
1. Bash script check if variable exists: You can use the command `declare -p variable >/dev/null 2>&1` to check if a variable exists. It will return true if the variable exists and false otherwise.
2. If variable bash: Another option is to use the `if` statement and check if the variable exists before executing certain commands. For example:
“`
if [ -n “${variable+set}” ]; then
echo “Variable exists”
else
echo “Variable does not exist”
fi
“`
3. Check variable is number bash: To determine if a variable contains a number, you can use the regex pattern matching feature in Bash. Here’s an example:
“`
if [[ $variable =~ ^[0-9]+$ ]]; then
echo “Variable is a number”
else
echo “Variable is not a number”
fi
“`
4. Bash script check environment variable exists: To check if an environment variable exists, you can use the -z option in the test command, like this:
“`
if [[ -z ${ENV_VAR+x} ]]; then
echo “Environment variable does not exist”
else
echo “Environment variable exists”
fi
“`
5. Bash check if variable is set using a boolean expression: If you wish to use a boolean expression to determine if a variable is set, you can leverage the AND (&&) operator in Bash. For example:
“`
if [ -n “$variable” ] && [ “$variable” == “value” ]; then
echo “Variable is set and matches the expected value”
else
echo “Variable is either unset or does not match the expected value”
fi
“`
FAQs
Q: How can I check if a variable is set and if it is a number in Bash?
A: You can use regex pattern matching to check if the variable contains a number. Alternatively, you can use the -n option to check if the variable is set, and then use a conditional statement to determine if it is a number.
Q: What is the difference between testing if a variable is set and testing if it is empty?
A: Testing if a variable is set determines whether it has been assigned any value at all, regardless of whether it is empty or not. Testing if a variable is empty specifically checks if it has been assigned an empty value.
Q: How can I check if an environment variable exists in Bash?
A: You can use the -z option in the test command to check if an environment variable exists.
In conclusion, checking if a variable is set in Bash is a crucial step in ensuring the reliability and robustness of your scripts. By using options such as -v, -z, and -n in the test command, you can easily determine if a variable has been assigned a value or not. Additionally, understanding the difference between testing if a variable is set and testing if it is empty allows you to handle different scenarios appropriately.
Check If A Variable Is Set/Empty In Bash
Keywords searched by users: bash check if variable is set Bash script check if variable exists, If variable bash, Check variable is number bash, If var bash, Bash script check environment variable exists, Bash check, Bash if and, Boolean bash
Categories: Top 42 Bash Check If Variable Is Set
See more here: nhanvietluanvan.com
Bash Script Check If Variable Exists
Bash, short for “Bourne Again Shell,” is a popular command-line interpreter and scripting language used primarily in Unix-based operating systems. With its powerful features and flexibility, it allows users to automate various tasks efficiently. One common requirement when writing Bash scripts is to check if a variable exists. In this article, we will explore different methods to determine variable existence in Bash scripts. So, let’s dive in!
Methods to Check if a Variable Exists:
1. Using the -v flag:
The -v flag in Bash checks if a variable is set and not empty. You can use it with the ‘if’ statement to conditionally execute a block of code. Consider the following example:
“`
if [ -v myVariable ]; then
echo “myVariable exists”
else
echo “myVariable does not exist”
fi
“`
This method is straightforward and reliable. If the variable is set and not empty, the code inside the ‘if’ block will be executed; otherwise, the ‘else’ block will be executed.
2. Using the -z flag with parameter substitution:
The -z flag checks if a variable is empty. By combining it with parameter substitution, we can also determine if a variable is set. Here’s an example:
“`
if [ -z “${myVariable+x}” ]; then
echo “myVariable does not exist”
else
echo “myVariable exists”
fi
“`
In this approach, the parameter substitution `${myVariable+x}` expands to the value of ‘myVariable’ if it is set; otherwise, it expands to an empty string. The -z flag then checks if the result is an empty string, indicating that the variable does not exist.
3. Using the declare command:
The ‘declare’ command in Bash allows us to define variables with various attributes. By using the ‘-p’ option, we can check if a variable is defined. Consider the following example:
“`
declare -p myVariable &> /dev/null
if [ $? -eq 0 ]; then
echo “myVariable exists”
else
echo “myVariable does not exist”
fi
“`
Here, we redirect the output of `declare -p myVariable` to `/dev/null` to discard it. The `$?` variable holds the exit code of the previous command, and a value of 0 indicates success, confirming the existence of the variable.
4. Using the ${!variable} syntax:
If you want to check if a variable exists and also get its value, you can use the `${!variable}` syntax. It expands to the value of the variable whose name is stored in ‘variable.’ If the variable does not exist, it expands to an empty string. Here’s an example:
“`
if [ -n “${!myVariable}” ]; then
echo “myVariable exists and its value is ${!myVariable}”
else
echo “myVariable does not exist”
fi
“`
In this method, the -n flag checks if the expanded variable is a non-empty string, confirming the existence of the variable.
Frequently Asked Questions:
Q1. How can I check if a variable is set but empty?
To check if a variable is set but empty, you can modify the second method explained above. Instead of using the -z flag, use the -n flag, which checks if the variable is a non-empty string. Here’s an example:
“`
if [ -n “${myVariable+x}” ]; then
echo “myVariable is set but empty”
else
echo “myVariable is not set or not empty”
fi
“`
Q2. Can I use the same methods to check if an array exists?
Yes, the same methods can be used to check the existence of an array. However, you need to access the array elements accordingly. For example, you can check if the first element of the array ‘myArray’ exists using the -v flag:
“`
if [ -v myArray[0] ]; then
echo “myArray[0] exists”
else
echo “myArray[0] does not exist”
fi
“`
Q3. How can I handle multiple variables in a single check?
If you want to check if multiple variables exist, you can use any of the provided methods in conjunction with logical operators like ‘&&’ (AND) or ‘||’ (OR). Here’s an example using the -v flag:
“`
if [ -v var1 ] && [ -v var2 ]; then
echo “Both var1 and var2 exist”
elif [ -v var1 ] || [ -v var2 ]; then
echo “Either var1 or var2 exist”
else
echo “Neither var1 nor var2 exist”
fi
“`
In conclusion, checking if a variable exists in Bash scripts is a crucial aspect of writing robust and error-free code. By using the methods mentioned above, you can easily handle variable existence in your scripts. Remember to consider the specific scenario and requirements while choosing the appropriate method.
If Variable Bash
Introduction
Bash, also known as the Bourne Again Shell, is a widely used Unix shell and command language interpreter. It is the default shell in most Linux distributions and is also available on macOS and other Unix-like systems. One of the essential features of bash is its ability to manipulate variables. This article will focus specifically on the “if” variable in bash, exploring its various use cases, syntax, and common FAQs.
Understanding the “If” Variable in Bash
In bash scripting, the “if” statement is used to evaluate conditions and execute certain commands based on the result of the evaluation. With the “if” variable, you can check the status of a variable and perform actions accordingly. It provides conditional branching in your scripts, allowing for more dynamic and flexible execution.
Syntax of the “If” Variable
The basic syntax of an “if” statement in bash is as follows:
“`
if [ condition ]; then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
“`
Here, the square brackets `[ ]` are used to denote a conditional expression. The condition is placed within these brackets, and based on the result, the corresponding block of code will be executed. If the condition is true, the code inside the “if” block will be executed; otherwise, the code inside the “else” block will be executed.
Using Variables in the “If” Statement
To use variables in the “if” statement, you need to assign a value to a variable and then evaluate it. Here’s an example:
“`
age=25
if [ $age -gt 18 ]; then
echo “You are an adult.”
else
echo “You are underage.”
fi
“`
In this case, the variable `age` is assigned a value of `25`. It is then evaluated in the “if” statement using the `-gt` operator, which stands for “greater than.” If the value of `age` is greater than `18`, the script will print “You are an adult.” Otherwise, it will print “You are underage.” The output will be “You are an adult” in this case.
Common Use Cases of the “If” Variable
1. Checking file existence: You can use the “if” variable to check if a file exists or not before performing any actions on it. For example:
“`
if [ -f “$filename” ]; then
echo “File $filename exists.”
else
echo “File $filename does not exist.”
fi
“`
Here, the script checks if the file specified by the variable `filename` exists using the `-f` option. If it does, it displays the message “File $filename exists,” otherwise, it displays “File $filename does not exist.”
2. Comparing strings: The “if” variable can also be used to compare strings. For example:
“`
if [ “$string1” == “$string2” ]; then
echo “The strings are equal.”
else
echo “The strings are not equal.”
fi
“`
In this case, the script compares the values of `string1` and `string2` using the `==` operator. If they are equal, it displays “The strings are equal.” Otherwise, it displays “The strings are not equal.”
FAQs
Q1. Can I use multiple conditions in an “if” statement?
Yes, you can use multiple conditions by combining them using logical operators such as `&&` (AND) or `||` (OR). For example:
“`
if [ condition1 ] && [ condition2 ]; then
# code to be executed if both conditions are true
fi
“`
Q2. How can I check if a variable is empty?
To check if a variable is empty, you can use the `-z` option. For example:
“`
if [ -z “$my_variable” ]; then
echo “Variable is empty.”
else
echo “Variable is not empty.”
fi
“`
In this case, if the variable `my_variable` is empty, it will display “Variable is empty,” otherwise, it will display “Variable is not empty.”
Q3. What is the difference between `=` and `==` in string comparisons?
The `=` operator is used for string comparisons in most POSIX-compliant shells, including bash. However, the `==` operator is specific to bash and is considered safer to use. It is preferred when writing portable bash scripts.
Conclusion
The “if” variable in bash scripting allows for conditional execution of code based on the evaluation of specified conditions. By using the proper syntax and understanding its various use cases, you can create powerful and dynamic scripts. Whether you need to check file existence or compare strings, the “if” variable in bash provides a versatile and essential tool for your scripting needs.
Images related to the topic bash check if variable is set
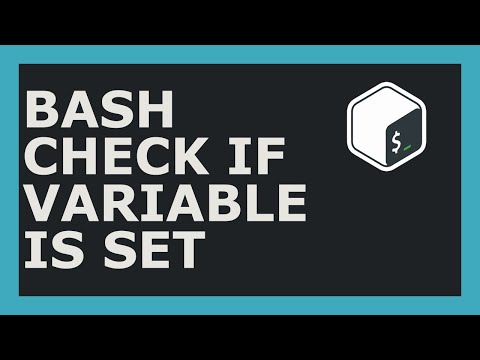
Found 14 images related to bash check if variable is set theme


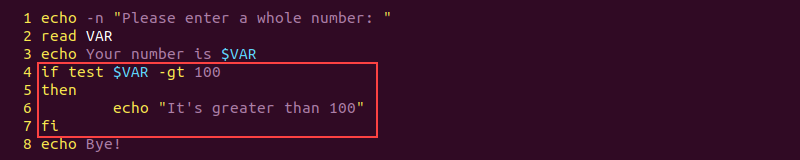
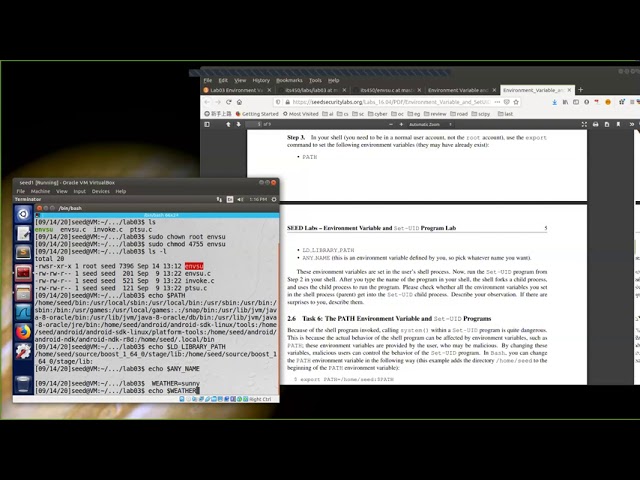
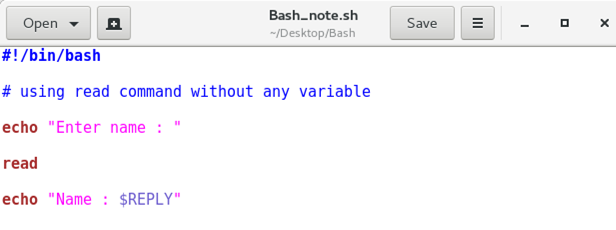

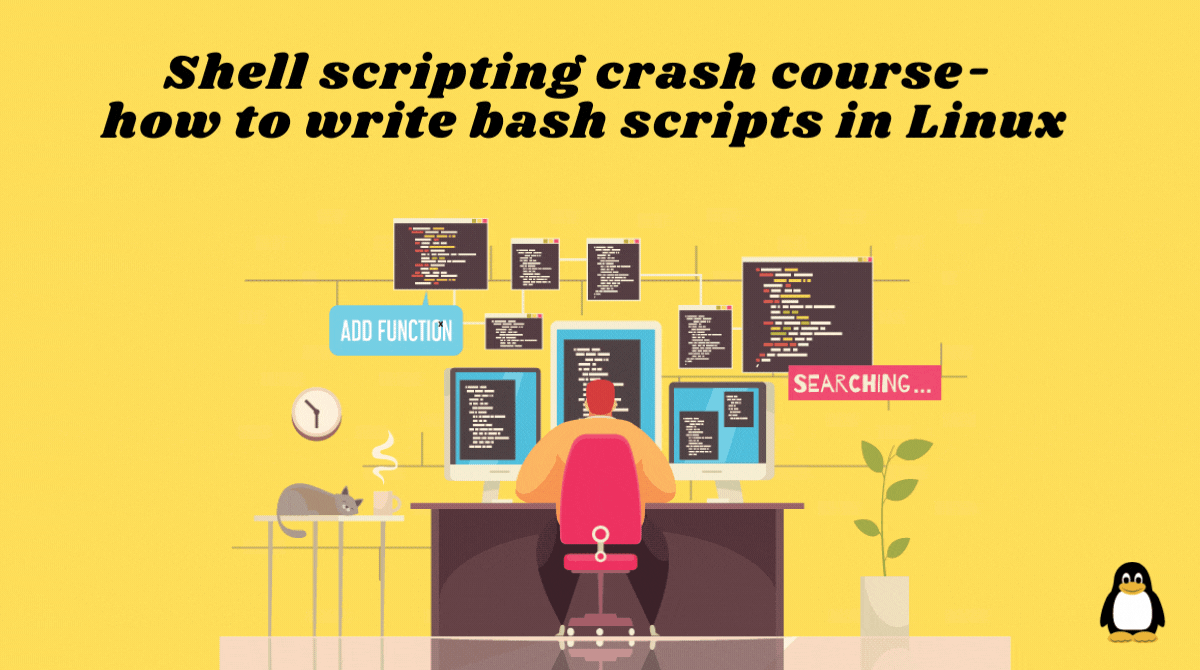



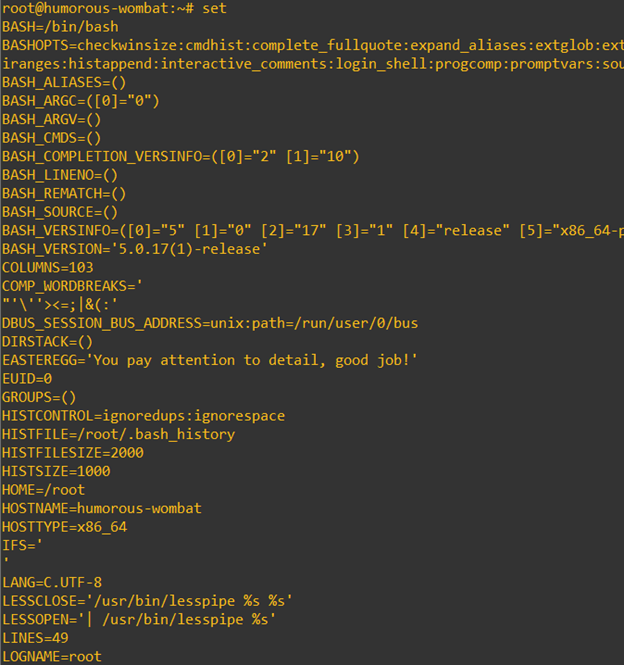
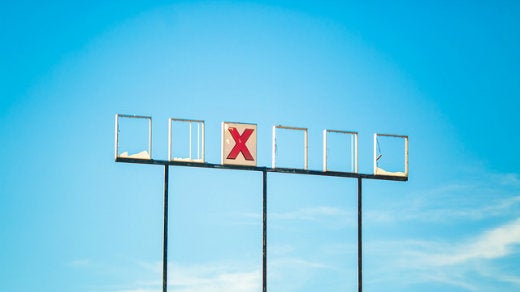

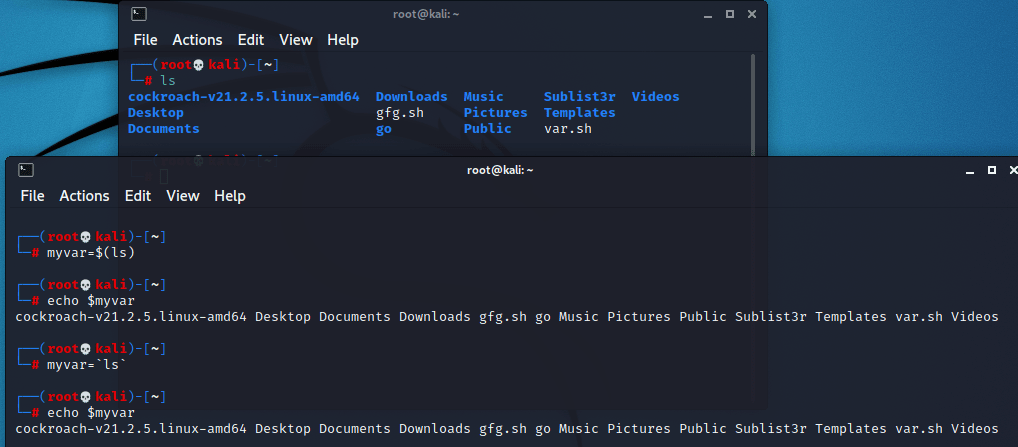

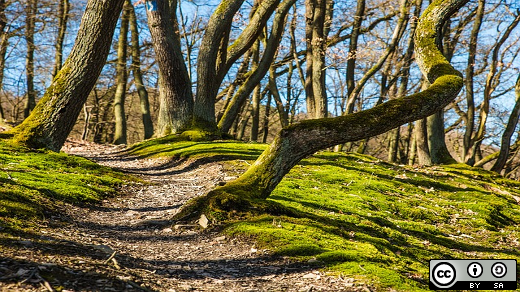
Article link: bash check if variable is set.
Learn more about the topic bash check if variable is set.
- How to check if a variable is set in Bash – Stack Overflow
- Bash – Check if a Variable is Set – Tutorial Kart
- How to check if bash variable defined in script – nixCraft
- Bash Check if Variable is Set – Javatpoint
- Bash Scripting – How to check If variable is Set – GeeksforGeeks
- Check if Variable Is Set in Bash | Delft Stack
- How to check the variable is set or empty in bash – Linux Hint
- How to check if a variable is set in Bash – Tuts Make
- How to check if a variable is set in Bash – Educative.io
- bash – How do I check if a variable exists in an ‘if’ statement?
See more: https://nhanvietluanvan.com/luat-hoc