Bash Check If Command Exists
Requirements for Checking if a Command Exists in a Bash Script:
Before diving into the different methods, it is essential to understand the basic requirements for checking if a command exists in a bash script. The primary requirement is the availability of the command being checked. If the command is not installed or cannot be found in the system’s PATH variable, it will be considered non-existent.
It is also important to have a basic understanding of the bash scripting language and familiarity with the command line interface. Additionally, some of the methods described here may require specific shell options or built-in commands, so it is crucial to check the scripting environment’s compatibility.
Now let’s explore the various methods for checking command existence in a bash script:
1. Using the “which” Command:
The “which” command is commonly used to locate the executable file associated with a given command. It searches the directories listed in the PATH variable to find the command. To check if a command exists, we can use the output of the “which” command.
Syntax:
“`
which command-name
“`
Example:
“`
if which git >/dev/null 2>&1; then
echo “Git is installed”
else
echo “Git is not installed”
fi
“`
Limitations:
– The “which” command may not work properly if the PATH variable is not set correctly or if the command is not in the directories listed in the PATH.
– It may not be available in some minimal or restricted shell environments.
2. Using the “type” Command:
The “type” command is used to determine the type of a command. It can be used to verify if a command is an alias, a built-in command, a shell function, or an executable file.
Syntax:
“`
type command-name >/dev/null 2>&1
“`
Example:
“`
if type python >/dev/null 2>&1; then
echo “Python is installed”
else
echo “Python is not installed”
fi
“`
Limitations:
– The “type” command may not work correctly if the command is defined as an alias or if the PATH variable is not set correctly.
3. Using the “-v” Option with the “command” Command:
The “command” command is a built-in command in the bash shell. When used with the “-v” option, it prints a description of the command, verifying its existence.
Syntax:
“`
command -v command-name >/dev/null 2>&1
“`
Example:
“`
if command -v make >/dev/null 2>&1; then
echo “Make is installed”
else
echo “Make is not installed”
fi
“`
Limitations:
– The “-v” option may not be available in some other shells or older versions of bash.
4. Using the “hash” Built-in Command:
The “hash” command is a built-in command in the bash shell that remembers and reuses the pathnames of commands found in directories listed in the PATH variable. By checking if the command is hashed, we can determine its existence.
Syntax:
“`
hash command-name >/dev/null 2>&1
“`
Example:
“`
if hash awk >/dev/null 2>&1; then
echo “Awk is installed”
else
echo “Awk is not installed”
fi
“`
Limitations:
– The “hash” command may not work as expected if the command is not in the directories listed in the PATH or if the command was recently added or removed.
5. Using the “command -v” Syntax:
The “command -v” syntax is an alternative to the “type” command for checking command existence. It prints the path to the command if it exists, or nothing if the command does not exist.
Syntax:
“`
[ -x “$(command -v command-name)” ]
“`
Example:
“`
if [ -x “$(command -v curl)” ]; then
echo “Curl is installed”
else
echo “Curl is not installed”
fi
“`
Limitations:
– This syntax may not be compatible with older versions of bash or other shell environments.
6. Using Shell Functions to Check Command Existence:
Shell functions can be built to check the existence of a command. By creating a shell function and calling it with the command name, we can determine if the command exists or not.
Syntax:
“`
command_exists() {
type “$1” >/dev/null 2>&1
}
if command_exists gcc; then
echo “GCC is installed”
else
echo “GCC is not installed”
fi
“`
Limitations:
– Shell functions may add overhead in terms of performance and readability.
7. Error Handling for Nonexistent Commands:
It is important to handle errors gracefully when a command does not exist. This can be done through different strategies like signal handling, exit codes, and custom error messages.
Signal Handling:
By trapping the specific signals generated when a command is not found, we can handle the error and perform necessary actions.
Exit Codes:
When a command does not exist, the exit code of the command represents the error. By checking the exit code, we can determine the existence of the command.
Error Messages:
Custom error messages can be produced to inform the user when a command is not found. These messages can be displayed using the “echo” command or redirected to a log file.
8. Practical Use Cases for Command Existence Checks:
Command existence checks have multiple practical use cases, including:
– Automation Scripts: Checking command existence ensures that the required commands are available before executing the script, avoiding errors and unexpected behavior.
– Dependency Management: Verifying the existence of dependencies before executing commands ensures that the required software or libraries are installed.
– Cross-Platform Compatibility: Checking command existence is particularly useful when writing scripts that need to be compatible with different operating systems or platforms.
9. Best Practices for Command Existence Checks:
Here are some best practices to consider when checking command existence in bash scripts:
– Avoiding Arbitrary Commands: It is recommended to avoid relying on specific commands that may vary between systems or platforms. Use universal or widely available commands instead.
– Considering Alternatives: If a command is not found, consider alternative approaches or fallback options to achieve the desired functionality.
– Preemptive Checking: Check for command availability before executing the command to prevent errors and unexpected behavior.
10. Conclusion:
In conclusion, checking if a command exists in a bash script is a crucial task to ensure the smooth operation of the script and avoid errors. There are various methods available, such as using the “which,” “type,” “-v” option with “command,” “hash,” “command -v” syntax, and shell functions. Each method has its syntax, examples, limitations, and best practices, which should be considered when implementing command existence checks in a bash script.
FAQs:
Q: How can I check if a file exists in a shell script?
A: To check if a file exists in a shell script, you can use the “-f” option with the “test” command or the “-e” option with the square brackets “[ ]”. For example:
“`
if [ -f “filename” ]; then
echo “File exists”
else
echo “File does not exist”
fi
“`
Q: How can I use if-else statements in bash?
A: In bash, if-else statements are used to conditionally execute code based on certain conditions. The general syntax is:
“`
if condition; then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
“`
Q: How can I check if a command exists in a Makefile?
A: You can use the “shell” function in a Makefile to check if a command exists. For example:
“`
ifneq (“$(shell command -v gcc)”, “”)
# code to be executed if the command exists
endif
“`
Q: How can I check if a command exists in Python?
A: In Python, you can use the “subprocess” module to run a command and check its return code. If the return code is 0, the command exists; otherwise, it does not. For example:
“`python
import subprocess
def command_exists(command):
try:
subprocess.check_output([“which”, command])
return True
except subprocess.CalledProcessError:
return False
if command_exists(“git”):
print(“Git is installed”)
else:
print(“Git is not installed”)
“`
Q: How can I check if a package is installed in a bash script?
A: Package availability can be checked by using package managers like “dpkg” or “apt-get” in Debian-based systems, “rpm” in Red Hat-based systems, or “yum” in CentOS. For example:
“`
if dpkg -s “package-name” >/dev/null 2>&1; then
echo “Package is installed”
else
echo “Package is not installed”
fi
“`
Q: How can I get the exit code of a command in bash?
A: The exit code of a command in bash can be accessed using the special variable “$?”. After executing a command, its exit code can be checked or stored in a variable for further processing.
Q: How can I check if a command was successful in a shell script?
A: In bash, the success of a command can be determined by its exit code. If the exit code is 0, the command was successful, and if it is non-zero, the command failed. You can use the “$?” variable to access the exit code of the last executed command.
Q: How can I check the output of a command in a bash script?
A: The output of a command in a bash script can be captured and stored in a variable using command substitution or the “$(command)” syntax. For example:
“`
output=$(command)
echo “$output”
“`
Bash check if command exists is an important aspect of bash scripting to ensure the smooth execution of scripts and prevent errors. By understanding the various methods, their syntax, examples, limitations, and best practices, you can effectively check if a command exists in a bash script. Remember to consider the specific requirements of your script and choose the most suitable method accordingly.
Shell Script Check If Directory Exists – Folder – Linux – Bash – Tutorial
Keywords searched by users: bash check if command exists How to check FILE exists in shell script, Bash if else, Makefile check command exists, Python check if command exists, Bash check if package is installed, Get exit code of command Bash, Shell script check command successful, Check output of command bash
Categories: Top 89 Bash Check If Command Exists
See more here: nhanvietluanvan.com
How To Check File Exists In Shell Script
In shell scripting, it is common to work with files, whether it is reading, writing, or manipulating them. One crucial step before performing any operation on a file is to check whether the file exists or not. This verification is essential as it ensures that the script doesn’t encounter any errors when it tries to work with the file.
In this article, we will explore different methods to check the existence of a file in a shell script, along with some examples, and also address common FAQs related to this topic.
Methods to Check File Existence
Method 1: Using the ‘-f’ Flag
The simplest way to check if a file exists is by using the ‘-f’ flag with the ‘test’ command. The ‘test’ command is a built-in shell command that evaluates the expression and returns a true or false value.
Here is the syntax of using the ‘-f’ flag:
“`
if [ -f
echo “File exists!”
else
echo “File does not exist.”
fi
“`
In this block of code, replace `
Method 2: Using the ‘stat’ Command
The ‘stat’ command retrieves file or file system status. We can leverage its functionality to check if a file exists by redirecting the error message to ‘/dev/null’ and capturing the exit status.
Here is an example of using ‘stat’ command to check file existence:
“`
if stat
echo “File exists!”
else
echo “File does not exist.”
fi
“`
Replace `
Method 3: Using the ‘-e’ Flag
The ‘-e’ flag is another option that can be used with the ‘test’ command to directly check the existence of the file. It checks if the file exists regardless of its type.
Here is an example of using the ‘-e’ flag to check file existence:
“`
if [ -e
echo “File exists!”
else
echo “File does not exist.”
fi
“`
Replace `
Method 4: Using File Tests
Shell script also provides a set of file tests that allow you to check different file attributes. One such test is ‘-r’ flag that checks if the file is readable. Similarly, the ‘-w’ flag checks if the file is writable.
Here is an example of using file tests to check file existence:
“`
file_path=
if [ -r $file_path ] && [ -w $file_path ]; then
echo “File exists and is both readable and writable!”
elif [ -r $file_path ]; then
echo “File exists and is readable!”
else
echo “File does not exist or is not readable.”
fi
“`
Replace `
FAQs
Q1. Can I use variables to check if a file exists?
Yes, you can use variables to check if a file exists. Simply replace the `
Q2. What is the difference between ‘-f’ and ‘-e’ flags?
The ‘-f’ flag checks if the file exists and is a regular file, whereas the ‘-e’ flag checks if the file exists regardless of its type.
Q3. How can I check if a directory exists?
To check if a directory exists, you can use the ‘-d’ flag with the ‘test’ command. For example:
“`
if [ -d
echo “Directory exists!”
else
echo “Directory does not exist.”
fi
“`
Replace `
Q4. How can I check the existence of multiple files at once?
You can use a loop to check the existence of multiple files. Iterate over each file path and use one of the above methods inside the loop to check if each file exists.
Conclusion
Checking the existence of a file in shell scripting is an essential task before performing any operations on the file. In this article, we explored various methods to check if a file exists, such as using the ‘-f’ flag, the ‘stat’ command, the ‘-e’ flag, and file tests. By using these techniques, you can ensure that your script executes without encountering any unexpected errors related to file handling.
Bash If Else
Introduction
In shell scripting, one of the most fundamental concepts is conditional statements. These statements allow us to write scripts that make decisions based on certain conditions. One such conditional statement is an if-else statement, which enables us to execute different sets of instructions based on whether a certain condition is true or false. In this article, we will delve into the world of Bash if-else statements, exploring their syntax, capabilities, and common use cases.
Understanding the Syntax
The syntax of an if-else statement in Bash is relatively simple and follows the format:
“`
if [ condition ]
then
# Execute commands if condition is true
else
# Execute commands if condition is false
fi
“`
The `if` keyword introduces the conditional statement, followed by the condition enclosed in square brackets `[ ]`. If the condition evaluates to true, the commands within the `then` block are executed. Conversely, if the condition evaluates to false, the commands within the `else` block are executed. Finally, `fi` indicates the end of the if-else statement. It is crucial to maintain correct indentation and use proper syntax within each block.
Comparison Operators
In order to provide conditions within the if-else statement, we need to use comparison operators. Bash supports a variety of comparison operators, including but not limited to:
– `==` for equality comparison
– `!=` for inequality comparison
– `-gt` for greater than comparison
– `-lt` for less than comparison
– `-ge` for greater than or equal to comparison
– `-le` for less than or equal to comparison
For example, let’s consider a simple if-else statement that checks if an entered number is positive:
“`
read -p “Please enter a number: ” number
if [ $number -gt 0 ]
then
echo “The number is positive.”
else
echo “The number is non-positive.”
fi
“`
In this example, the entered number is compared using the `-gt` operator to check if it is greater than zero. Based on the result, the appropriate message is displayed.
Nested If-else Statements
Sometimes, we may need to include more complex conditions in our shell scripts. This can be achieved by employing nested if-else statements. A nested if-else statement is an if-else statement placed within another if or else block. This hierarchical structure allows for greater flexibility and complexity in decision-making.
Let’s consider an example where we need to classify a given number as positive, negative, or zero:
“`
read -p “Please enter a number: ” number
if [ $number -gt 0 ]
then
echo “The number is positive.”
elif [ $number -lt 0 ]
then
echo “The number is negative.”
else
echo “The number is zero.”
fi
“`
In this example, an additional `elif` statement is introduced, which stands for ‘else if’. This statement enables us to test multiple conditions sequentially. Only the block belonging to the first true condition encountered is executed, and subsequent conditions are skipped.
Logical Operators
Apart from comparison operators, Bash also provides logical operators to form more complex conditions. The commonly used logical operators are:
– `&&` for logical AND
– `||` for logical OR
These operators enable us to combine multiple conditions and evaluate them together.
For instance, suppose we want to determine whether a given number is both positive and even. We can utilize logical operators in the following manner:
“`
read -p “Please enter a number: ” number
if [ $number -gt 0 ] && [ $((number % 2)) -eq 0 ]
then
echo “The number is positive and even.”
else
echo “The number is either negative, odd, or both.”
fi
“`
In this example, the `-gt` operator checks if the number is greater than zero, while `$((number % 2))` checks if the number is divisible by 2, making it even. By combining these two conditions using the logical AND operator, we can ensure both conditions are true for the subsequent block to execute.
FAQs
1. Can I use string comparisons in Bash if-else statements?
Yes, Bash supports string comparisons as well. Instead of using numerical comparison operators, you can use `=` for equality and `!=` for inequality when comparing strings.
2. Is it necessary to enclose variables within double quotes in Bash if-else statements?
While enclosing variables within double quotes is often good practice to handle cases where the variable might contain spaces or special characters, it is not required for basic variable comparison.
Conclusion
In this article, we have explored Bash if-else statements, covering their syntax, comparison operators, nested structures, logical operators, and common use cases. Understanding these fundamental concepts of conditional statements in shell scripting is essential for writing robust and versatile scripts. The ability to make decisions within scripts based on specific conditions empowers shell script developers to create powerful, automated solutions for various tasks.
Makefile Check Command Exists
What is the Makefile check command?
The Makefile check command is a built-in functionality in the GNU Make tool that allows developers to run tests and perform checks on their software projects. It provides a reliable and efficient way to verify the correctness and stability of the codebase. Essentially, the check command serves as a gatekeeper, ensuring that the software meets the necessary quality standards before it is released.
Typically, the check command is used in conjunction with testing frameworks and tools such as JUnit, PyTest, or Mocha, depending on the programming language. These testing frameworks enable developers to write and execute automated tests, ensuring that the code behaves as expected.
Why is the Makefile check command important?
When working on software projects, it is crucial to have an automated process in place to verify the integrity of the codebase. Manually testing the entire project after each code change is not only time-consuming but also error-prone. The Makefile check command steps in to address this issue by automating the testing process.
By utilizing the Makefile check command, developers can easily incorporate tests into their daily workflow. It encourages the practice of writing testable code and ensures that any new features or bug fixes do not introduce regressions. This way, software development teams can confidently ship their code, minimizing the risk of introducing defects into the final product.
How to use the Makefile check command?
To make use of the Makefile check command, you first need to have a Makefile set up in your project directory. This Makefile acts as a blueprint for building your software project and defines various commands, including the check command.
To write the check command in the Makefile, you typically need to define the necessary dependencies, set up the test environment, and execute the test commands. For instance, if you are using a Java project with JUnit for testing, your check command might look like this:
“`
check:
mvn test
“`
In this example, the check command uses the Maven build tool to run the tests defined within your project. Depending on your project setup, the command to execute the tests may differ. You can tailor the check command to suit your project’s needs and integrate it with your preferred testing framework, build tool, or command-line tool.
Once you have defined the check command in your Makefile, you can run it by executing the following command in your terminal:
“`
make check
“`
Executing this command will trigger the tests and perform the necessary checks defined in your check command.
Frequently Asked Questions (FAQs):
Q: Can I use the Makefile check command for non-test related checks?
A: Yes, definitely! While the check command is commonly used to run tests, it can also be extended to perform other checks like code linting, code formatting, or static analysis. By defining additional rules in your Makefile, you can incorporate various checks into your software development workflow.
Q: Are there any limitations to using the Makefile check command?
A: The limitations of the Makefile check command depend on the testing framework or tools you use. It is essential to note that the check command relies on the setup of your project and the availability of the necessary dependencies. For example, if your test framework has certain requirements, such as specific libraries or environmental configurations, you need to ensure that they are properly set up before executing the check command.
Q: Can I run multiple tests using the check command?
A: Absolutely! The check command can be expanded to include multiple test commands or even call a testing script. It provides the flexibility to combine various test suites or implement custom test scenarios tailored to specific project requirements.
Q: Is it possible to run specific tests with the Makefile check command?
A: Yes, you can specify which tests to run by either modifying the check command or via command-line arguments. Some testing frameworks allow you to use tags or annotations to categorize your tests, making it easier to specify subsets of tests to execute.
Q: Are there alternative ways to automate tests rather than using the Makefile check command?
A: Yes, there are alternative ways to automate tests. Many modern development frameworks offer built-in testing capabilities, such as Laravel’s PHPUnit integration or Django’s test framework. Additionally, there are dedicated test automation tools like Jenkins or CircleCI that can be used to set up continuous integration pipelines, automatically triggering tests whenever code changes are pushed.
In conclusion, the Makefile check command plays a vital role in automating software testing and ensuring code quality. By utilizing this command, developers can efficiently execute tests and checks within their workflow, minimizing manual efforts and reducing the risk of introducing defects. Combined with testing frameworks and other automation tools, the Makefile check command empowers software development teams to build robust and reliable applications.
Images related to the topic bash check if command exists
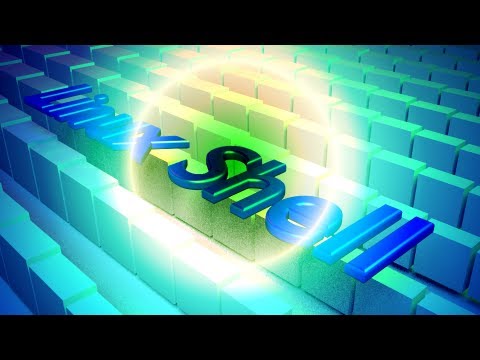
Found 35 images related to bash check if command exists theme

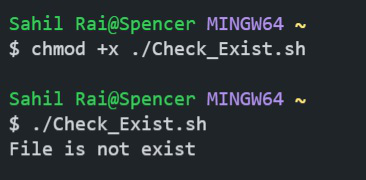
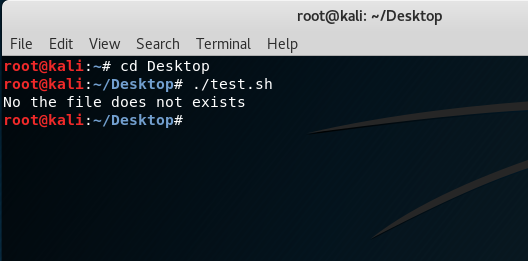
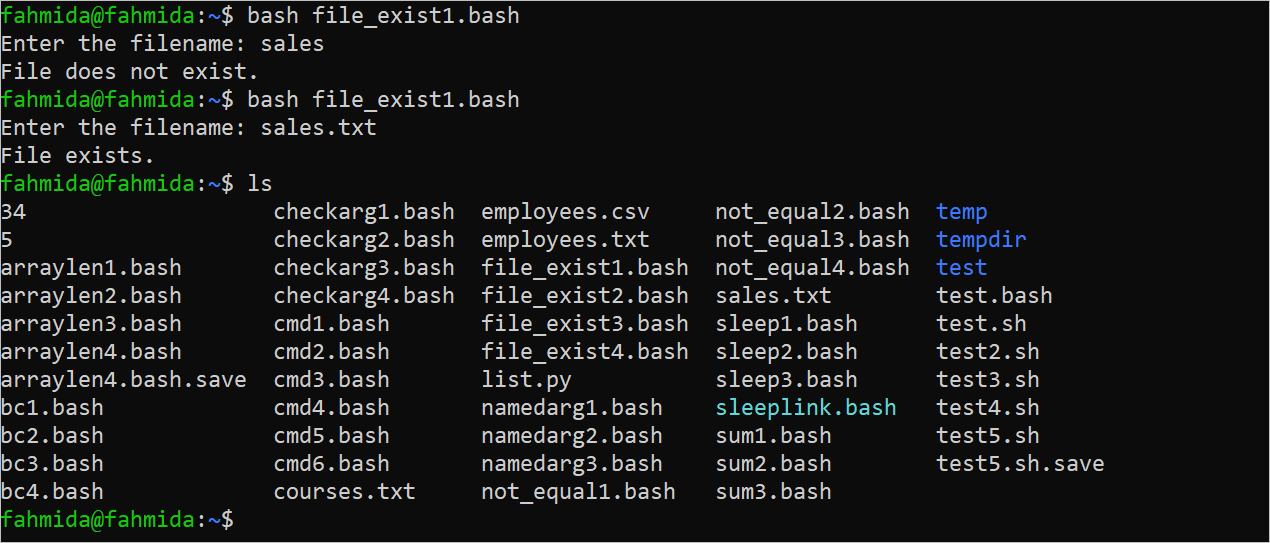
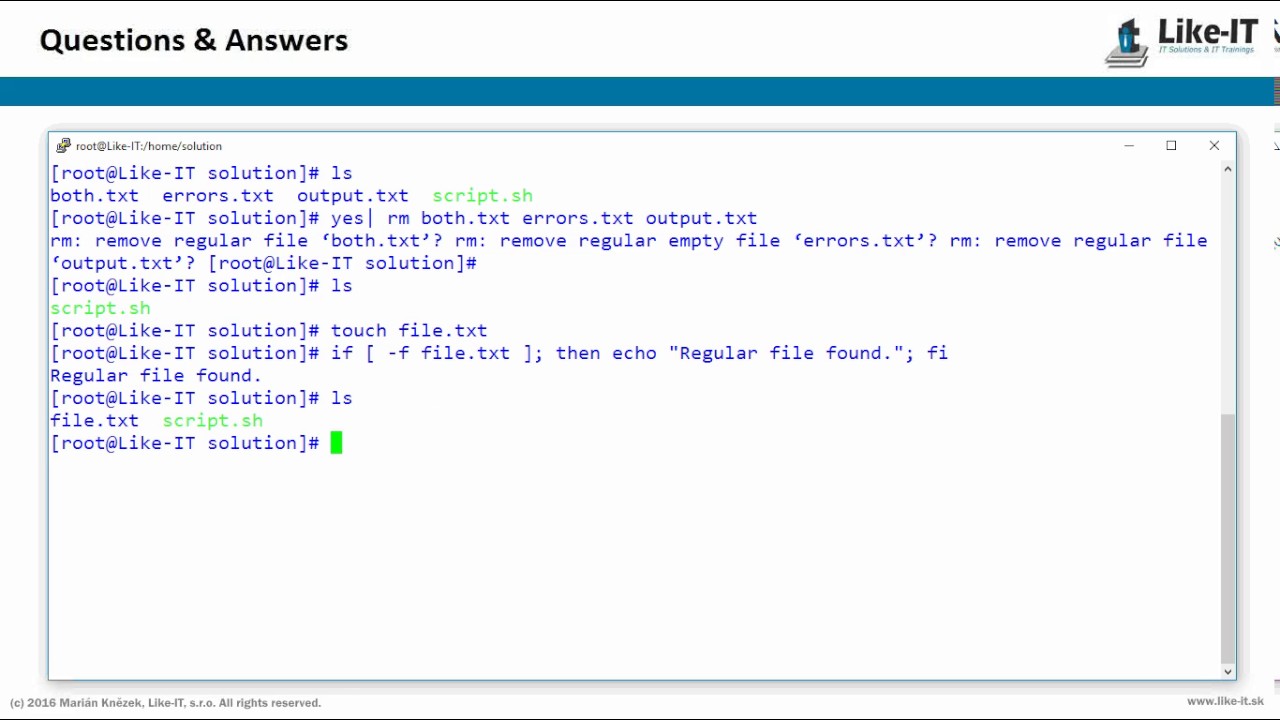

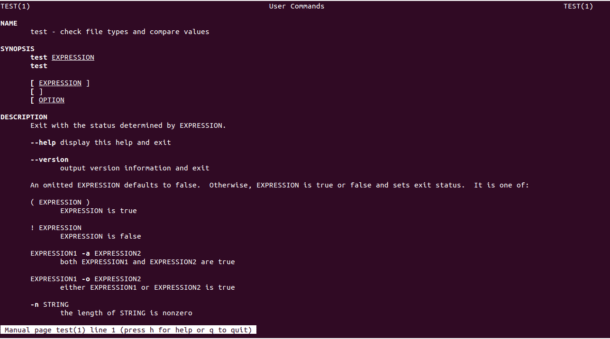
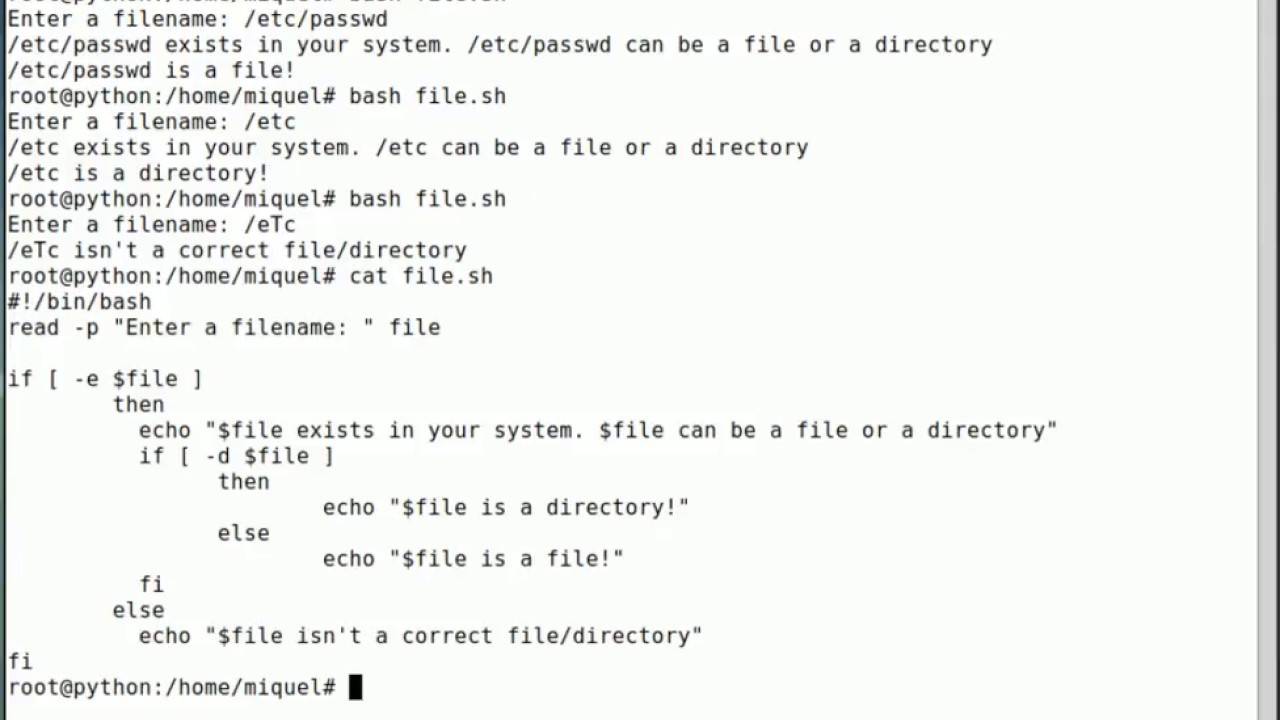
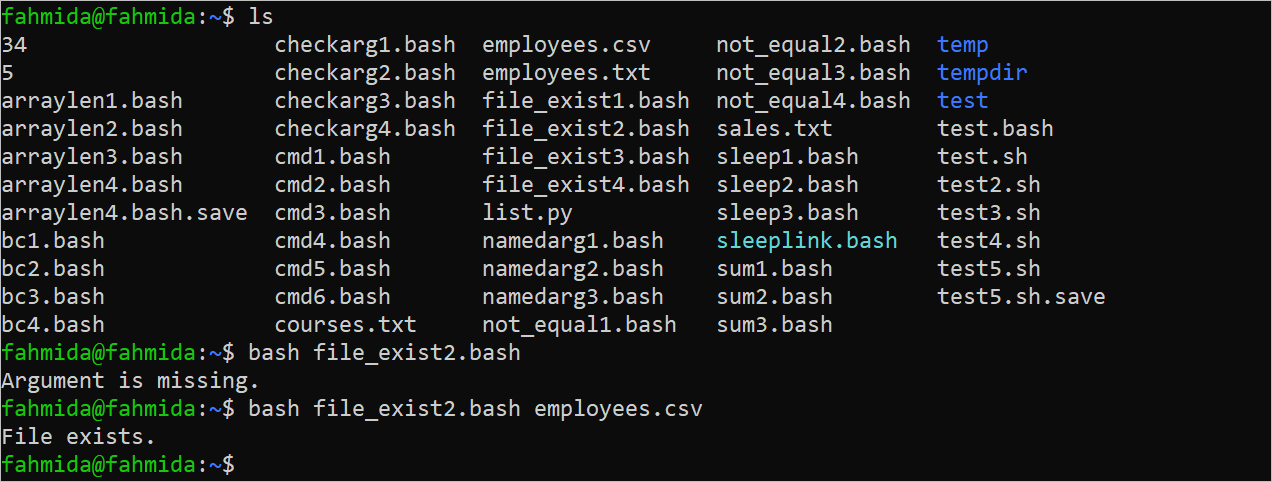
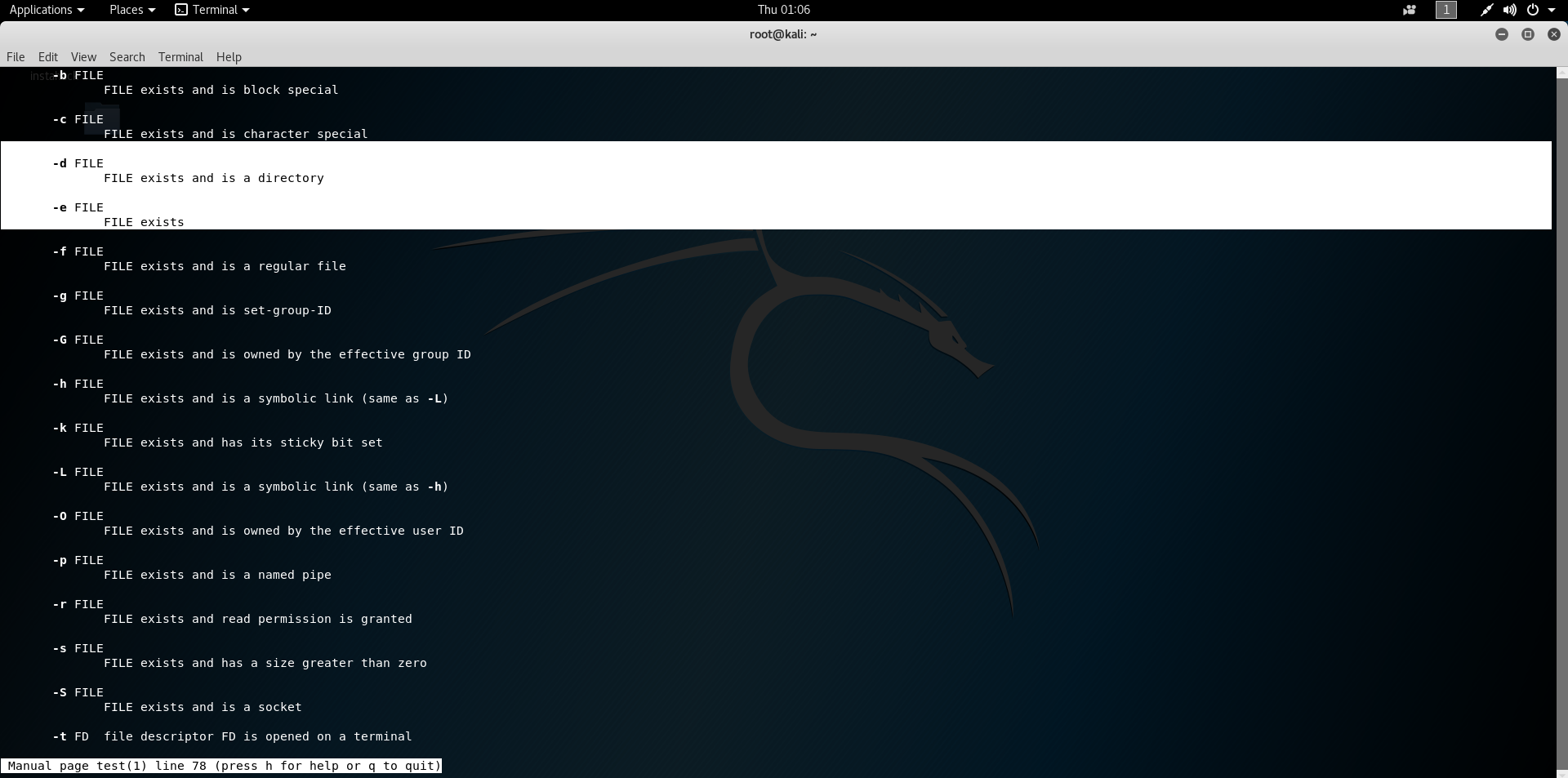


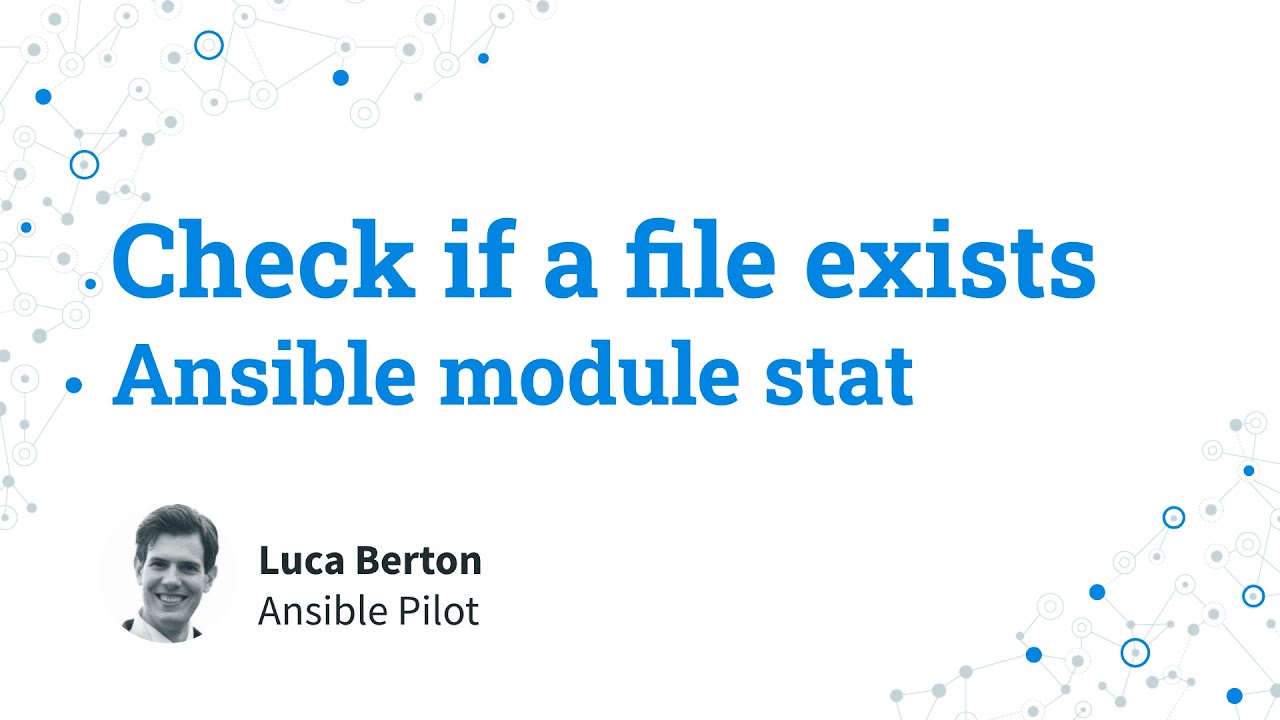
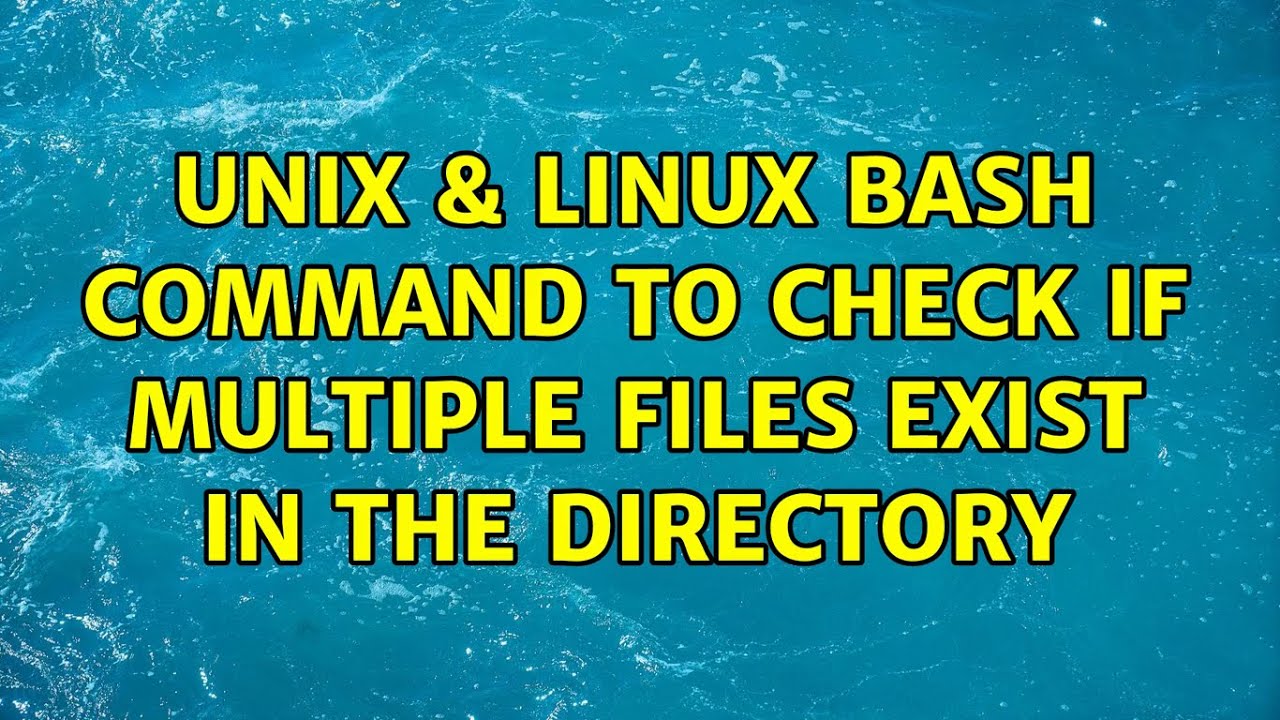
![Golang] Check If A Program (Command) Exists Golang] Check If A Program (Command) Exists](https://i.stack.imgur.com/qN5s4.png)

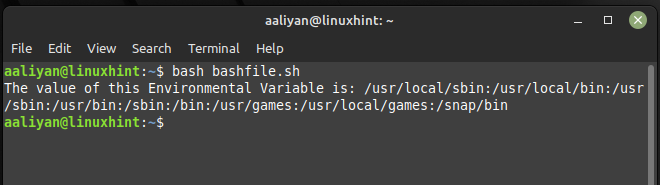
Article link: bash check if command exists.
Learn more about the topic bash check if command exists.
- How can I check if a program exists from a Bash script?
- Check if a Command Exists in Bash – Delft Stack
- Check if Command Exists in Bash [3 Ways] – Java2Blog
- How to Check if Program Exists in Shell Script – Fedingo
- Bash Shell Find Out If A Command Exists On UNIX / Linux …
- Check if a command exists revisited – Unix Stack Exchange
- How to Check if a Program Exists in Linux – TecAdmin
- How to Check if the program exists from a Bash script – Reactgo
- How to check if a command exists – Bash – OneLinerHub
- Bash: Check if Directory or File Exists – Stack Abuse