Base64 Decode In C#
What is Base64 encoding?
Base64 encoding is a process where binary data is converted into a string of ASCII characters. This encoding scheme uses a set of 64 characters – A-Z, a-z, 0-9, and “+” and “/” as the 63rd and 64th characters. The encoding process takes three octets of binary data and divides them into four 6-bit groups. Each of these 6-bit groups is then converted into a corresponding character from the 64-character set. The resulting string is a representation of the original binary data in ASCII format.
What is Base64 decoding?
Base64 decoding is the reverse process of Base64 encoding. It takes a Base64 encoded string and converts it back into its original binary representation. The decoding algorithm converts each character in the Base64 string back to its corresponding 6-bit binary value. These binary values are then combined to reconstruct the original binary data.
Why do we need to decode Base64 in C#?
There are several reasons why we may need to decode Base64 in C#. One common use case is when receiving data encoded in Base64 format over a network or from a file. In order to use the data in its original form, we need to decode it. Another scenario is when working with data stored in a database or file system, where the data is encoded using Base64. In such cases, decoding is necessary to obtain the original binary data.
Understanding the Base64 decoding process
The Base64 decoding process in C# involves converting each character in the Base64 string to its corresponding 6-bit binary value. This can be achieved using various techniques and classes available in C#. We will explore three common methods of decoding Base64 in C#: using the Convert class, using the MIME Convert class, and implementing a custom decoder.
Using the Convert class to decode Base64 in C#
The Convert class in C# provides a simple way to decode Base64 strings. The Convert class includes a method called FromBase64String that takes a Base64 encoded string as input and returns the decoded binary data as a byte array. Here’s an example of how to use this method:
“`csharp
string base64String = “SGVsbG8gd29ybGQh”; // Base64 encoded string
byte[] bytes = Convert.FromBase64String(base64String); // Decoding Base64
string decodedString = Encoding.UTF8.GetString(bytes); // Converting byte array to string
Console.WriteLine(decodedString); // Output: “Hello world!”
“`
Using the MIME Convert class to decode Base64 in C#
The MIME Convert class in C# provides additional functionalities for handling Base64 encoding and decoding. It includes a method called FromBase64String that works similar to the Convert class method we discussed earlier. Here’s an example of how to use the MIME Convert class:
“`csharp
string base64String = “SGVsbG8gd29ybGQh”; // Base64 encoded string
byte[] bytes = System.Security.Cryptography.FromBase64Transform.FromBase64String(base64String); // Decoding Base64
string decodedString = Encoding.UTF8.GetString(bytes); // Converting byte array to string
Console.WriteLine(decodedString); // Output: “Hello world!”
“`
Decoding Base64 with a custom decoder implementation
If you want more control over the decoding process or need to handle non-standard Base64 variations, you can also implement your own Base64 decoder in C#. This gives you the flexibility to handle custom characters or padding options. Here’s an example of a custom decoder implementation:
“`csharp
static byte[] CustomBase64Decode(string input)
{
string base64Chars = “ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/”;
int padding = input.EndsWith(“==”) ? 2 : input.EndsWith(“=”) ? 1 : 0;
int length = input.Length * 6 / 8 – padding;
byte[] output = new byte[length];
for (int i = 0, j = 0; i < length; i += 3, j += 4)
{
int b0 = base64Chars.IndexOf(input[j]);
int b1 = base64Chars.IndexOf(input[j + 1]);
int b2 = base64Chars.IndexOf(input[j + 2]);
int b3 = base64Chars.IndexOf(input[j + 3]);
output[i] = (byte)((b0 << 2) | (b1 >> 4));
if (i + 1 < length)
output[i + 1] = (byte)(((b1 & 0x0F) << 4) | (b2 >> 2));
if (i + 2 < length)
output[i + 2] = (byte)(((b2 & 0x03) << 6) | b3);
}
return output;
}
```
Best practices for Base64 decoding in C#
When working with Base64 decoding in C#, keep the following best practices in mind:
1. Handle potential exceptions: While decoding, it's essential to handle exceptions that may occur, such as malformed Base64 strings or unsupported characters.
2. Validate input: Before decoding, ensure that the input string is a valid Base64 encoded string to avoid unnecessary decoding errors or security vulnerabilities.
3. Consider performance: When decoding large Base64 strings, consider using optimized libraries or techniques to improve performance.
4. Validate decoded data: After decoding, validate and verify the integrity of the decoded data using appropriate validation methods or techniques.
FAQs:
Q: What is the difference between Base64 encoding and decoding?
A: Base64 encoding converts binary data into a string representation, while Base64 decoding converts the encoded string back to its original binary form.
Q: Can I decode Base64 using other programming languages, such as C, Python, or C++?
A: Yes, Base64 encoding and decoding are widely supported across different programming languages and can be implemented using their respective libraries or built-in functions.
Q: How can I decode Base64 using OpenSSL?
A: OpenSSL provides a command-line tool called "openssl" that can be used to decode Base64. The command "openssl enc -d -base64" can be used to decode a Base64 encoded file or string.
Q: Is it possible to encode and decode Base64 using STM32 microcontrollers?
A: Yes, STM32 microcontrollers provide libraries and functions to encode and decode Base64 data.
Q: How can I implement Base64 encoding in C#?
A: The Convert.ToBase64String method in C# can be used to encode binary data into Base64 format.
In conclusion, Base64 decoding in C# is a crucial process for converting Base64 encoded strings back to their original binary representation. Whether you use the Convert class, MIME Convert class, or a custom implementation, understanding the decoding process and following best practices ensures the accurate and secure handling of Base64 encoded data in your C# applications.
Base64 Encoding
What Is Base64 In C?
Base64 encoding is a popular technique used for converting binary data into ASCII text. It is widely used in computer science and communication protocols where binary data needs to be safely transmitted over text-based systems. In the context of the C programming language, Base64 is commonly used for storing and transmitting data in a human-readable form, ensuring reliable and accurate data transmission.
Understanding Base64 Encoding:
Base64 encoding works by converting three bytes (24 bits) of binary data into four ASCII characters (each character represented by 6 bits). The resulting text is a combination of uppercase and lowercase letters, numbers, and two special characters, typically ‘+’, and ‘/’. This process ensures that the resulting text is compatible with most communication protocols and can be easily transmitted over text-based systems such as email or HTTP.
The initial binary data is divided into groups of three bytes (3×8=24 bits). If the total number of binary bytes is not divisible by three, padding is added at the end to ensure the consistency of the encoded data. Each group of three bytes is then split into four 6-bit sections. These 6-bit sections are converted into decimal values and used as indices to select a character from a predefined table of 64 characters. The resulting characters are concatenated to form the Base64 encoding of the original binary data.
Advantages of Base64 Encoding in C:
1. Portability: Base64 encoding in C is platform-independent and can be easily implemented and understood across different operating systems, making it a reliable choice for data transmission in various environments.
2. Efficiency: Base64 encoding in C is a computationally efficient technique that utilizes simple bitwise operations, making it suitable for resource-constrained environments or systems with limited processing power.
3. Data Integrity: Base64 encoding allows the accurate transmission of binary data over text-based systems, ensuring data integrity during transmission. The encoding process avoids the use of characters that could be misinterpreted or corrupted during transmission.
4. Security: While Base64 encoding is not intended as a security mechanism, it can provide a certain level of obfuscation as the encoded data is not immediately recognizable as the original binary data. However, it should be noted that Base64 encoding is not a secure method for protecting sensitive information.
Implementing Base64 Encoding in C:
To implement Base64 encoding in the C programming language, there are various approaches and libraries available that facilitate the encoding and decoding process. Several open-source libraries, such as OpenSSL, also provide Base64 encoding support.
One approach to implementing Base64 encoding in C is by manually implementing the encoding algorithm using bitwise operations and lookup tables. This method allows for a deeper understanding of the encoding process and provides flexibility for customization. However, it can be more time-consuming and error-prone.
Alternatively, several Base64 encoding libraries are readily available, providing reliable and optimized implementations. These libraries offer functions that can be easily integrated into existing C code, simplifying the implementation process and ensuring compatibility across platforms.
FAQs:
Q1. When should I use Base64 encoding in C?
Base64 encoding is commonly used in situations where binary data needs to be transmitted over text-based systems. It is particularly useful when working with data that contains non-printable characters, such as image files, cryptographic keys, or binary file attachments in email systems.
Q2. Can I use Base64 encoding for encryption or data security purposes?
Base64 encoding is not designed for encryption or ensuring data security. While it can provide some obfuscation, it is not a suitable method for protecting sensitive or confidential information. If data security is a concern, please refer to encryption algorithms specifically designed for that purpose, such as AES or RSA.
Q3. How can I decode Base64-encoded data in C?
Decoding Base64-encoded data in C can be achieved using similar methods as the encoding process. Either manually implementing the decoding algorithm or utilizing existing libraries can accomplish this task. Several libraries provide ready-to-use functions specifically for Base64 decoding, ensuring accuracy and efficiency.
Q4. Is Base64 encoding reversible?
Yes, Base64 encoding is reversible. The encoded data can be easily decoded back to its original binary form, assuming the decoding algorithm is correctly implemented or a reliable library function is used.
Q5. How can I handle Base64-encoded strings that contain padding characters?
Base64-encoded strings may contain padding characters (‘=’) at the end to ensure the correct alignment of the encoded data. When decoding such strings, it is important to consider and handle the padding characters correctly to ensure accurate decoding of the original binary data.
Conclusion:
Base64 encoding is a widely used technique for converting binary data into a human-readable form, facilitating its transmission over text-based systems. In the context of the C programming language, Base64 encoding offers portability, efficiency, and data integrity. Whether implementing the encoding algorithm manually or utilizing pre-existing libraries, Base64 encoding is a powerful tool for safely transmitting binary data. However, it is important to note that Base64 encoding is not intended for encryption or data security purposes and should not be relied upon as such.
How To Use Base64 Decode In C#?
Introduction:
Base64 is a widely used encoding scheme that represents binary data in an ASCII string format. It is commonly used for transferring and storing data that cannot be directly represented in a text-only format. In C#, decoding Base64 strings is a straightforward process that can be accomplished using built-in functionality. In this article, we will dive into the details of using Base64 decode in C#.
Understanding Base64 Encoding:
Before we proceed to discussing Base64 decoding, it is essential to understand how Base64 encoding works. Base64 encoding converts binary data into a string of ASCII characters. It achieves this by representing each set of three bytes from the binary data into four ASCII characters.
The encoding process starts by splitting the binary data into chunks of three bytes. Then, each set of three bytes is converted into four Base64 characters using a specific mapping table. If the binary data does not have enough bytes to form a complete three-byte chunk, padding characters (‘=’) are added at the end. These padding characters denote that the encoded data is not complete.
Using Base64 Decode in C#:
C# provides various ways to decode Base64 strings. Here, we will explore two commonly used methods: the Convert class and the System.Text.Encoding class.
1. Using the Convert class:
The Convert class in C# provides a simple way to perform Base64 decoding. It includes a method named FromBase64String(string s), which decodes a provided Base64 string and returns the original binary data in the form of a byte array.
Here is an example of decoding a Base64 string using the Convert class:
“`csharp
string base64String = “SGVsbG8gd29ybGQh”;
byte[] byteArray = Convert.FromBase64String(base64String);
string decodedString = Encoding.UTF8.GetString(byteArray);
Console.WriteLine(decodedString);
“`
In this example, we have a Base64 encoded string “SGVsbG8gd29ybGQh”, which represents the text “Hello world!”. We decode the string using the FromBase64String method, which returns a byte array. Finally, we convert the byte array into a string using the UTF8 encoding.
2. Using the System.Text.Encoding class:
The System.Text.Encoding class in C# provides support for various character encodings, including Base64. It includes methods for decoding Base64 strings into byte arrays.
Here is an example of using the System.Text.Encoding class to decode a Base64 string:
“`csharp
string base64String = “SGVsbG8gd29ybGQh”;
byte[] byteArray = Convert.FromBase64String(base64String);
string decodedString = Encoding.UTF8.GetString(byteArray);
Console.WriteLine(decodedString);
“`
In this example, we perform the same steps as in the previous example. We decode the Base64 string using the FromBase64String method and convert it into a byte array. Finally, we convert the byte array into a string using the UTF8 encoding.
FAQs about Base64 decode in C#:
Q: Can I decode non-ASCII characters using Base64 in C#?
A: Yes, Base64 encoding/decoding is not limited to ASCII characters only. It can be used to encode/decode any binary data, including non-ASCII characters such as UTF-8 encoded text or images.
Q: What happens if the Base64 string is not properly padded?
A: Base64 strings should always be properly padded to a multiple of four characters. If a string is not correctly padded, the decoding process may not work correctly, resulting in a corrupted output.
Q: Can I decode Base64 strings with different character encodings?
A: Yes, C# provides various character encodings through the System.Text.Encoding class. You can specify the desired encoding when converting the byte array into a string.
Q: What should I do if the Base64 string contains an invalid character?
A: If the Base64 string contains invalid characters (characters not recognized in the Base64 alphabet), the decoding process will raise an exception. Make sure the provided string only contains valid Base64 characters.
Conclusion:
Base64 decoding is a fundamental process when working with encoded data in C#. By following the methods provided by the Convert and System.Text.Encoding classes, you can easily decode Base64 strings into their original binary representation. It is crucial to ensure proper padding and handle exceptions when dealing with Base64 data. With this knowledge, you can confidently handle Base64 encoding and decoding in your C# applications.
Keywords searched by users: base64 decode in c# Base64 in c, Openssl base64 decode, Base64 source code c, Base64 decode Python, Stm32 Base64 encode, Base64 C++, Implement base64 encoding, Base64 decode C
Categories: Top 38 Base64 Decode In C#
See more here: nhanvietluanvan.com
Base64 In C
Introduction to Base64
Base64 is a widely used encoding format that allows binary data to be safely transmitted over channels that only support textual data. It is especially useful for encoding complex data structures or binary files such as images, documents, or audio files. The concept behind Base64 encoding is to convert binary data into a set of 64 characters, consisting of alphanumeric characters and additional special characters, without any control characters or whitespace.
In this article, we will explore the fundamentals of Base64 encoding and decoding in the C programming language, covering its implementation, benefits, and common use cases. Additionally, we will address some frequently asked questions related to Base64 in C.
Implementing Base64 Encoding and Decoding in C
To implement Base64 encoding and decoding in C, we need to utilize the ASCII character set. The ASCII characters are represented by numeric values from 0 to 127, and Base64 encoding converts these values into a range of 64 distinct characters. The C programming language provides built-in functions for character manipulation, making it relatively simple to convert between ASCII and Base64.
To encode data in Base64, we need to follow a series of steps:
1. Retrieve the binary data that needs encoding.
2. Break the binary data into 6-bit chunks.
3. Convert each 6-bit chunk into decimal representation.
4. Map the decimal values to corresponding Base64 characters.
5. Append padding characters if necessary to ensure a multiple of 4 characters.
Similarly, to decode Base64-encoded data, we follow these steps:
1. Retrieve the Base64-encoded data.
2. Map each Base64 character back to its decimal representation.
3. Combine the decimal values of each character to form a sequence of 6-bit chunks.
4. Reconstruct the binary data from the 6-bit chunks.
Both the encoding and decoding process require manipulating bits and characters in C, utilizing bitwise operators, shift operations, and logical operations.
Benefits and Use Cases
There are several benefits to using Base64 encoding in C. Firstly, Base64 provides a reliable method for transmitting binary data over channels that only accept textual data, such as JSON or XML. By converting binary data into a textual representation, it becomes safe from corruption during transmission or processing.
Secondly, Base64 encoding is widely supported by different programming languages, frameworks, and applications. This uniformity makes it an ideal choice when dealing with interoperability between different platforms or systems.
Additionally, Base64 encoding is often applied when embedding binary files within text-based formats, such as HTML emails or CSS stylesheets. By encoding binary data into a text format compatible with these systems, it becomes easier to transmit and handle these files.
Furthermore, Base64 encoding can be used for data obfuscation. Though it doesn’t provide encryption or security against determined adversaries, converting data into Base64 can deter casual or unintended human readers from understanding the original content.
Frequently Asked Questions (FAQs)
Q1: Is Base64 encryption?
A1: No, Base64 is not an encryption mechanism. It is merely an encoding format that converts binary data into a textual representation. Base64 does not provide any confidentiality or data protection.
Q2: Can Base64 be reversed back to the original data?
A2: Yes, Base64 encoding can be reversed through the decoding process, successfully retrieving the original binary data. However, Base64 is not suitable for encryption purposes as the encoded data can be easily decoded by anyone with access to the encoded data.
Q3: How large is the size increase when using Base64 encoding?
A3: Base64 encoding increases the size of the data by approximately 33%. This increase is due to the fact that every 3 bytes of binary data are converted to 4 bytes of Base64-encoded data.
Q4: Is Base64 encoding case-sensitive?
A4: No, Base64 encoding is case-insensitive. The character set used for mapping binary values to characters includes both uppercase and lowercase letters, but they are considered interchangeable during decoding.
Q5: Can Base64 be used for large binary files?
A5: While Base64 encoding can be used for large binary files, it is not the most efficient choice due to the increase in size. Encoding or decoding large files can be computationally expensive and may impact performance. In such cases, alternatives like binary file transfer or compression techniques should be considered.
Conclusion
Base64 encoding and decoding in the C programming language offer a versatile method for transmitting binary data in a textual format. By following the steps highlighted in this article, you can easily encode and decode binary data using Base64. The benefits of using Base64 include compatibility, safe transmission over textual channels, and data obfuscation. However, it is essential to understand that Base64 is not suitable for encryption purposes, as the encoded data can be easily reversed.
Openssl Base64 Decode
In the realm of cryptography and secure communication, OpenSSL plays a crucial role by providing a robust framework for various operations, including encryption, decryption, digital signatures, and certificate management. One particular functionality OpenSSL offers is Base64 decoding, which allows the conversion of Base64-encoded data back into its original format. In this comprehensive guide, we will delve into the intricacies of OpenSSL Base64 decode, its applications, implementation, and some frequently asked questions.
Understanding Base64 Encoding
Before diving into OpenSSL’s Base64 decoding capabilities, it is crucial to comprehend the basics of Base64 encoding itself. The Base64 encoding scheme is employed to transform binary data, such as images or binary files, into ASCII characters. It achieves this by converting three bytes of binary data into four ASCII characters.
Base64 encoding is widely used in various applications, including email attachments, data transmission over email protocols, and encoding binary data within XML or JSON files. Decoding Base64-encoded data is an essential step to retrieve and utilize such information effectively.
The Role of OpenSSL in Base64 Decoding
OpenSSL, a versatile open-source library that provides cryptographic protocols and tools, extends its support for Base64 decoding. With OpenSSL, developers have a straightforward mechanism to decode Base64 data and extract the original binary information effortlessly.
Implementation of Base64 Decoding using OpenSSL
To perform Base64 decoding using OpenSSL, developers can utilize OpenSSL’s ‘bio’ (BIO) objects. ‘BIO’ stands for Basic Input/Output, which acts as a bridge between OpenSSL and various data sources or sinks. Here’s an example of using OpenSSL BIO to decode a Base64-encoded string:
“`C++
#include
#include
// Encoded Base64 string
const char* encodedData = “SGVsbG8gV29ybGQ=”;
// Set up input BIO
BIO *bio = BIO_new_mem_buf(encodedData, -1);
BIO *base64 = BIO_new(BIO_f_base64());
bio = BIO_push(base64, bio);
// Set decoding mode
BIO_set_flags(bio, BIO_FLAGS_BASE64_NO_NL);
// Allocate memory for decoded data
char decodedData[256];
memset(decodedData, 0, sizeof(decodedData));
// Perform the Base64 decoding
int decodedLen = BIO_read(bio, decodedData, strlen(encodedData));
// Cleanup
BIO_free_all(bio);
// Use the decoded data
printf(“Decoded data: %s\n”, decodedData);
“`
This example demonstrates the essential steps to perform Base64 decoding using OpenSSL’s ‘bio’ objects in the C programming language. By leveraging the BIO functions, developers can easily decode Base64-encoded data and retrieve the original information.
FAQs
Q: Is OpenSSL Base64 decoding restricted to C programming language only?
A: No, OpenSSL is a versatile library that supports various programming languages such as Python, Java, C++, and more. Developers can find OpenSSL bindings or libraries tailored for their preferred programming language and utilize the Base64 decoding functionality accordingly.
Q: Can I decode Base64-encoded data directly from a file using OpenSSL?
A: Absolutely! OpenSSL provides support for reading data from files using ‘BIO’ objects. By utilizing BIO objects as inputs, developers can read Base64-encoded data directly from files and perform the necessary Base64 decoding operations with ease.
Q: Are there any limitations to the size of data that OpenSSL can decode?
A: OpenSSL can handle Base64 decoding operations for data of various sizes. However, it is essential to consider memory limitations when dealing with large files or significant amounts of Base64-encoded data. Avoid reading large files entirely into memory at once and instead use streaming techniques to handle decoding efficiently.
Q: Are there any performance considerations when using OpenSSL Base64 decoding?
A: OpenSSL is known for its exceptional performance and optimized implementations. However, the encoding/decoding speed may vary depending on the system’s processing power, memory, and the size of the data being decoded. Developers should leverage proper optimization techniques and utilize OpenSSL recommendations to achieve the best performance possible.
Q: Can I use OpenSSL Base64 decoding in combination with other cryptographic operations?
A: Absolutely! OpenSSL provides a comprehensive suite of cryptographic functionalities, including symmetric and asymmetric encryption, hashing, and digital signatures. Combining Base64 decoding with other cryptographic operations provides developers with a powerful toolkit for secure data manipulation and transmission.
In conclusion, OpenSSL’s Base64 decoding capabilities enable developers to easily convert Base64-encoded data back into its original format. By leveraging OpenSSL’s BIO objects, developers can efficiently decode Base64-encoded data in various programming languages. Understanding and utilizing OpenSSL’s vast cryptographic functionalities facilitates secure data manipulation and encrypted communication across various applications.
Images related to the topic base64 decode in c#
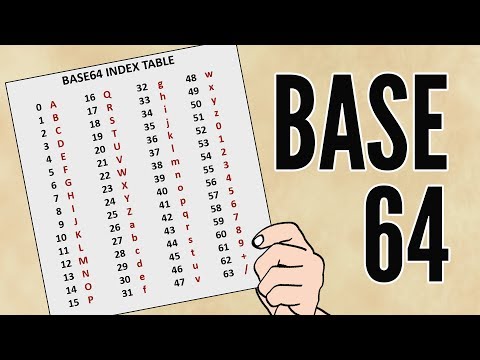
Article link: base64 decode in c#.
Learn more about the topic base64 decode in c#.
- How do I base64 encode (decode) in C? – Stack Overflow
- Base64 Encode and Decode in C – John’s Blog
- jwerle/b64.c: Base64 encode/decode – GitHub
- C Implementation of Base64 Encoding and Decoding
- C Implementation of Base64 Encoding and Decoding
- How to encode and decode a base64 string in C# – Dofactory
- C program to encode and decode string – Joymon V/S Code
- Why does a base64 encoded string always end with? – Quora
- Base64
- 4.6. Performing Base64 Decoding – O’Reilly
- base64.c Source Code – Base64 encoding scheme
- base64.c – Apple Open Source
- Base64 Encode And Decode In C# – C# Corner
- tests encoding/decoding strings with base64 in ‘C’ – FM4DD
See more: nhanvietluanvan.com/luat-hoc