Attributeerror ‘Numpy.Ndarray’ Object Has No Attribute ‘Append’
To understand this error and find alternative approaches, it is essential to grasp the concepts of Python objects and attributes. In Python, everything is an object, and objects have attributes, which are variables or functions that belong to the object. Each object in Python is associated with a specific type, and those types define what attributes and methods the object can have.
The ‘numpy.ndarray’ object is a fundamental data structure provided by the NumPy library for handling n-dimensional arrays. It is often used in scientific and mathematical computations due to its efficiency and flexibility. However, one important limitation of the ‘numpy.ndarray’ object is that it does not have a direct ‘append’ attribute.
The ‘append’ attribute is commonly found in other data structures, such as lists, and is used to add an element to the end of the data structure. This attribute allows for dynamic expansion of the data structure and is a convenient feature for many programming tasks.
Unfortunately, the absence of the ‘append’ attribute in the ‘numpy.ndarray’ object means that you cannot append elements directly to it. This is because ‘numpy.ndarray’ objects are designed to have fixed sizes and shapes, making them more suitable for mathematical operations that require consistent dimensions.
However, there are alternative approaches to achieve the desired result of appending elements to an ‘numpy.ndarray’ object. One option is to use the ‘concatenate’ function provided by the NumPy library. The ‘concatenate’ function allows for the combination of two or more arrays along a specified axis. By using this function, you can effectively append elements to the ‘numpy.ndarray’ object.
To use the ‘concatenate’ function, you need to ensure that the arrays you want to append have compatible shapes along the specified axis. For example, if you want to append elements along the rows, the number of columns in both arrays must match.
While using the ‘concatenate’ function can achieve the desired result, it is important to consider the advantages and disadvantages it entails. One advantage is that the ‘concatenate’ function allows for efficient memory allocation and can handle large arrays effectively. However, it is important to keep in mind that concatenating arrays repeatedly can lead to unnecessary memory usage, as each concatenation creates a new array that takes up additional memory.
Apart from the ‘concatenate’ function, the NumPy library also provides other functions for modifying arrays, such as ‘insert’, ‘delete’, and ‘resize’. These functions offer more flexibility in array manipulation and can be used as alternatives to the ‘append’ attribute. However, it is essential to understand the specifics of each function and evaluate their suitability for the task at hand.
In some cases, it may be necessary to convert the ‘numpy.ndarray’ object to a list before appending elements. Converting an ‘numpy.ndarray’ object to a list can be done using the ‘tolist()’ method provided by NumPy. This method creates a new list object that contains the same elements as the ‘numpy.ndarray’, allowing for the use of the ‘append’ attribute.
However, it is important to consider the performance implications of such conversions. Converting large arrays to lists can consume a significant amount of memory and may result in a decrease in performance. Therefore, it is advisable to only convert arrays to lists when necessary and when the size of the array is manageable.
Once the ‘numpy.ndarray’ object is converted to a list, appending elements becomes straightforward. The ‘append’ attribute can be used as usual, allowing for the desired dynamic expansion of the data structure.
In conclusion, while the ‘numpy.ndarray’ object does not have a direct ‘append’ attribute, there are several alternative approaches to append elements to the array. These include using the ‘concatenate’ function, utilizing other array modification functions provided by NumPy, or converting the ‘numpy.ndarray’ object to a list before appending. It is important to consider the specific requirements of your task and the performance implications of each approach to ensure efficient and effective array manipulation.
FAQs
Q: Why does the AttributeError ‘numpy.ndarray’ object has no attribute ‘append’ occur?
A: The ‘numpy.ndarray’ object does not have a direct ‘append’ attribute because it is designed to have fixed sizes and shapes, suitable for mathematical operations. As a result, attempting to use the ‘append’ attribute on a ‘numpy.ndarray’ object will generate an AttributeError.
Q: Can elements be appended to an ‘numpy.ndarray’ object?
A: No, elements cannot be directly appended to an ‘numpy.ndarray’ object. However, there are alternative approaches such as using the ‘concatenate’ function or converting the array to a list and then appending elements.
Q: What is the difference between ndarrays and lists in Python?
A: ‘ndarrays’ and lists are both data structures in Python but have different characteristics. ‘ndarrays’ are efficient for mathematical operations and have fixed sizes and shapes, while lists are more flexible and allow for dynamic resizing and appending.
Q: How can ‘ndarrays’ be modified without the append attribute?
A: ‘ndarrays’ can be modified without the append attribute by using alternative approaches such as the ‘concatenate’ function, other array modification functions, or converting the array to a list and then appending elements.
Q: What are the best practices for manipulating ‘ndarrays’ without append?
A: To effectively manipulate ‘ndarrays’ without the append attribute, it is advisable to avoid the need for appending by properly planning the data structure requirements. Additionally, understanding and utilizing the available NumPy functions for array modification can maximize efficiency and performance.
Attributeerror: ‘Numpy.Ndarray’ Object Has No Attribute ‘Append’
Keywords searched by users: attributeerror ‘numpy.ndarray’ object has no attribute ‘append’ Numpy append array to another array, Np append example, Numpy array append column, Add value to NumPy array, Numpy ndarray append, List to numpy array, Add item to array in Python, Np append not working
Categories: Top 36 Attributeerror ‘Numpy.Ndarray’ Object Has No Attribute ‘Append’
See more here: nhanvietluanvan.com
Numpy Append Array To Another Array
Appending arrays in NumPy can be done using the `numpy.append()` function. This function takes in two mandatory arguments: the array to which you want to append, and the array you want to append. Additionally, there is an optional argument called `axis`, which specifies along which axis the arrays should be appended. By default, `axis` is set to None, which means the arrays will be flattened before the append operation.
Let’s start by looking at a simple example. Suppose we have two arrays, `arr1` and `arr2`:
“` python
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
“`
To append `arr2` to `arr1` using the `numpy.append()` function, we can simply use the following code:
“` python
result = np.append(arr1, arr2)
print(result)
“`
The output will be: `[1 2 3 4 5 6]`. As you can see, the elements of `arr2` have been appended to `arr1` in a flattened manner.
If you want to append arrays along a specific axis, you can specify the `axis` argument. For example, if we have two two-dimensional arrays, `arr1` and `arr2`:
“` python
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
“`
To append `arr2` below `arr1`, we can use `axis=0`:
“` python
result = np.append(arr1, arr2, axis=0)
print(result)
“`
The output will be:
“`
[[1 2]
[3 4]
[5 6]
[7 8]]
“`
Similarly, if you want to append `arr2` to the right of `arr1`, you can use `axis=1`:
“` python
result = np.append(arr1, arr2, axis=1)
print(result)
“`
The output will be:
“`
[[1 2 5 6]
[3 4 7 8]]
“`
It is important to note that when appending arrays, the shapes along the specified axis must match. In the above example, both `arr1` and `arr2` have the same number of rows (2), allowing for a successful append operation.
Now, let’s discuss some FAQs regarding array appending in NumPy:
**FAQs:**
**Q: Can I append more than two arrays at once using `numpy.append()`?**
A: Yes, you can append multiple arrays at once by passing them as separate arguments to `numpy.append()`. For example:
“` python
result = np.append(arr1, arr2, arr3)
“`
**Q: How does `numpy.append()` handle data types?**
A: When appending arrays, `numpy.append()` attempts to maintain the data type of the resulting array. However, if the data types of the arrays being appended are different, NumPy will upcast the resulting array to a more general data type.
**Q: Is `numpy.concatenate()` the same as `numpy.append()`?**
A: No, `numpy.concatenate()` is a different function that concatenates arrays along a specified axis. While `numpy.append()` can be considered a specific case of `numpy.concatenate()`, the latter provides more flexibility and options.
**Q: What is the performance impact of using `numpy.append()`?**
A: It is worth noting that `numpy.append()` creates a new array each time it is called, which can be memory-intensive for large arrays. If performance is a concern, it is often more efficient to preallocate an array with sufficient space and then assign values directly, rather than appending sequentially.
**Q: Are there any alternatives to `numpy.append()` for array appending?**
A: Yes, you can use `numpy.concatenate()` as mentioned earlier. Alternatively, you can also use the `numpy.vstack()` function to vertically stack arrays, or `numpy.hstack()` to horizontally stack arrays. These functions offer more control and flexibility when it comes to array concatenation.
In conclusion, NumPy provides a convenient and efficient way to append one array to another using the `numpy.append()` function. We have explored the basic usage of this function, along with examples of appending along different axes. It is important to understand the concept of shape matching and consider the performance implications when working with large arrays. Additionally, it is worth exploring other alternatives like `numpy.concatenate()`, `numpy.vstack()`, and `numpy.hstack()` for more specific use cases.
Np Append Example
The Np append command is a popular and powerful tool used in various programming languages for adding elements to an existing array. This command allows developers to effortlessly append items to an array, thereby expanding its size and functionality. In this article, we will delve into the details of Np append example, explore its usage in different programming languages, and answer some frequently asked questions.
Np Append Example in Python:
Python is a versatile and widely-used programming language that supports Np append. Consider the following example:
“`python
import numpy as np
arr = np.array([1, 2, 3])
new_element = 4
new_arr = np.append(arr, new_element)
print(new_arr)
“`
In this example, we import the numpy library as np and create an array called `arr` with the values 1, 2, and 3. By using the `np.append()` function, we add a new element, 4, to the existing array. Finally, we print the new array, which now includes the appended element. The output will be `[1 2 3 4]`.
Np Append Example in R:
R is a statistical programming language that also provides support for Np append. Let’s look at a brief example:
“`R
library(data.table)
arr <- c(1, 2, 3) new_element <- 4 new_arr <- append(arr, new_element) print(new_arr) ``` Here, we use the `append()` function provided by the data.table library in R. Similar to Python, we define the array `arr` with the values 1, 2, and 3. After that, we append the new element, 4, using the `append()` function, and finally, we print the updated array. The output will be `[1] 1 2 3 4`. Np Append Example in MATLAB: MATLAB is a high-level programming language often used in scientific and engineering applications. Let's see how Np append works in MATLAB: ```matlab arr = [1, 2, 3]; new_element = 4; new_arr = [arr, new_element]; disp(new_arr) ``` In this MATLAB example, we define the array `arr` with the values 1, 2, and 3. Using square brackets, we concatenate the existing array with the new_element and assign it to new_arr. Finally, we use the `disp()` function to display the updated array. The output will be `[1, 2, 3, 4]`. FAQs: Q1. What is the difference between Np append and Np concatenate? A1. The `np.append()` function in Python and the `append()` function in R and MATLAB are used to add elements to an existing array. On the other hand, `np.concatenate()` is used to combine two or more arrays together, either along the rows or columns. The key difference is that `append()` adds elements to an existing array, while `concatenate()` combines arrays. Q2. Can I append multiple elements to an array using Np append? A2. Yes, you can append multiple elements to an array using Np append. In all the aforementioned programming languages, you can provide multiple elements separated by commas within the parentheses of the append function. Q3. Is it possible to append arrays of different sizes? A3. Yes, you can append arrays of different sizes. Np append automatically adjusts the size of the resulting array based on the elements being appended. However, keep in mind that the shape and dimensions of the arrays must still be compatible. Q4. Are there any performance considerations when using Np append? A4. Yes, appending elements to an array using Np append can be computationally expensive. This is because, in most cases, it involves creating a new array and copying the existing elements along with the appended elements. Therefore, if you need to perform a large number of appends, it is recommended to preallocate the array and fill it with elements instead of using Np append repeatedly. In conclusion, Np append is a handy command that enables developers to add elements to existing arrays effortlessly. This article covered Np append examples in popular programming languages such as Python, R, and MATLAB. It also addressed frequently asked questions related to Np append, clarifying differences with Np concatenate, explaining appending multiple elements, handling arrays of different sizes, and considering performance concerns. Hopefully, this comprehensive guide has shed light on the topic of Np append and its usage, empowering programmers to make effective use of this command in their respective projects.
Numpy Array Append Column
Numpy, short for Numerical Python, is a widely used library in the Python programming language for efficient numerical computations. One of its key features is the Numpy array, a powerful data structure that allows for fast and convenient manipulation of large datasets. In this article, we will delve deep into the topic of appending a column to a Numpy array, discussing its applications, implementation, and potential challenges.
Applications of Appending a Column
Appending a column to a Numpy array is a fundamental operation in many data analysis tasks. It can be particularly useful when we need to expand the data structure to accommodate additional information. For instance, when working with a dataset representing student grades, we may want to add a column to store the student’s average score.
Moreover, appending a column becomes indispensable when merging or concatenating multiple arrays. In tasks like data preprocessing or feature engineering, it is often necessary to combine multiple datasets to have a holistic view of the problem under consideration. By appending columns, we can easily join information from different sources into a single array, facilitating subsequent analysis.
Implementation
Implementing column appending in Numpy is fairly straightforward and efficient. Let’s consider a hypothetical scenario where we have an existing Numpy array called `my_array` with shape `(m, n)`, where `m` is the number of rows and `n` is the number of columns. To append a column to this array, we can use the `numpy.append` function.
The syntax for appending a column is as follows:
“`python
import numpy as np
new_column = np.array([1, 2, 3, 4]) # Creating the column to be appended
my_array = np.append(my_array, np.expand_dims(new_column, axis=1), axis=1)
“`
In this example, we create a new column `new_column` as a Numpy array. Then, using `numpy.append`, we append this new column to the existing array `my_array`. The `np.expand_dims` function is used to reshape the new column to have the proper dimensions before appending.
Challenges and Considerations
While appending a column to a Numpy array may seem uncomplicated, there are some considerations to keep in mind. One important factor to be aware of is the potentially large memory consumption when dealing with sizable datasets. Appending a column results in the creation of an entirely new array, requiring extra memory allocation. This operation can become resource-intensive when working with big data, leading to increased execution time and potential out-of-memory errors.
To mitigate these challenges, it is recommended to preallocate the array with the necessary dimensions before appending columns. This allows for more efficient memory usage and avoids the overhead of dynamically resizing the array during each append operation.
Another aspect to consider is the performance impact of looping over rows when appending columns incrementally. Iteratively appending columns row by row can be time-consuming, especially with large datasets. In such cases, using vectorized operations, such as concatenating columns in a single pass, can significantly enhance performance.
FAQs
Q: Can I append multiple columns at once using the `numpy.append` function?
A: Yes, the `numpy.append` function can be used to append multiple columns together. Simply pass a 2D Numpy array containing the columns to be appended, ensuring that the dimensions match the original array.
Q: Is it possible to append a column to a Numpy array without creating a new array?
A: No, appending a column always involves creating a new array. Numpy arrays are immutable, meaning their size cannot be modified after creation. Therefore, appending a column requires creating a new array and copying the existing data along with the new column.
Q: Are there any alternatives to the `numpy.append` function for column appending?
A: Yes, there are alternative methods for column appending in Numpy, such as using the `numpy.concatenate` function or directly modifying the arrays in-place. However, it is crucial to be cautious when modifying arrays in-place to avoid unintended side effects and ensure data integrity.
In conclusion, understanding how to append a column to a Numpy array is a valuable skill for any data analyst, scientist, or engineer working with numerical data. By mastering this operation, you can efficiently manipulate arrays, expand datasets, and perform complex computations. With the information provided in this article, you are now equipped to tackle column appending tasks with confidence and optimize your data workflows.
Images related to the topic attributeerror ‘numpy.ndarray’ object has no attribute ‘append’
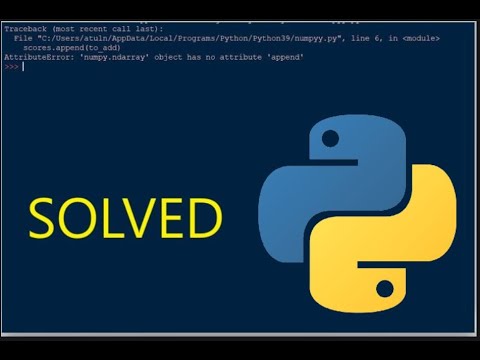
Found 20 images related to attributeerror ‘numpy.ndarray’ object has no attribute ‘append’ theme
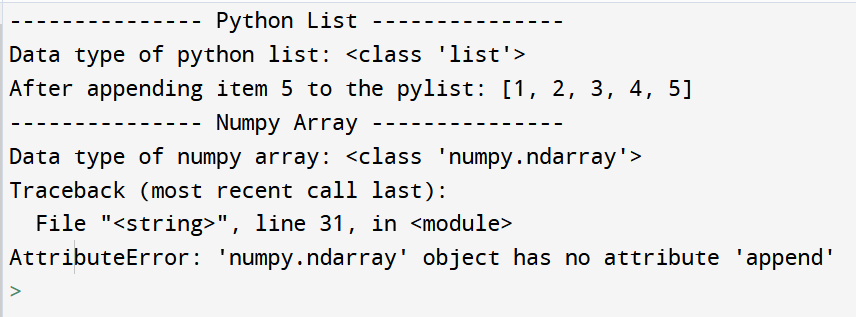
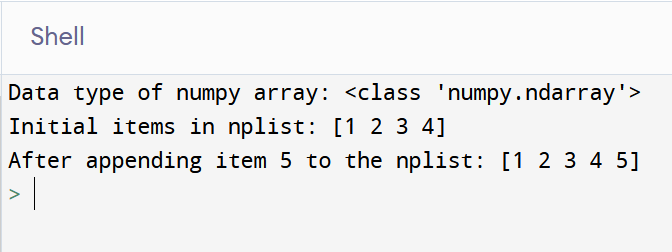

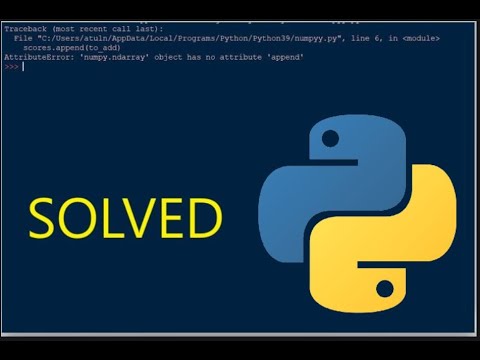
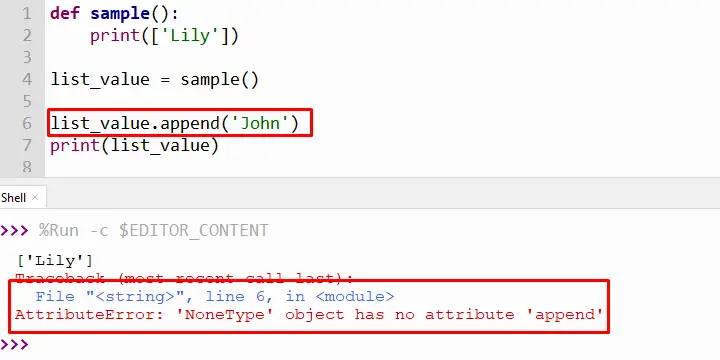
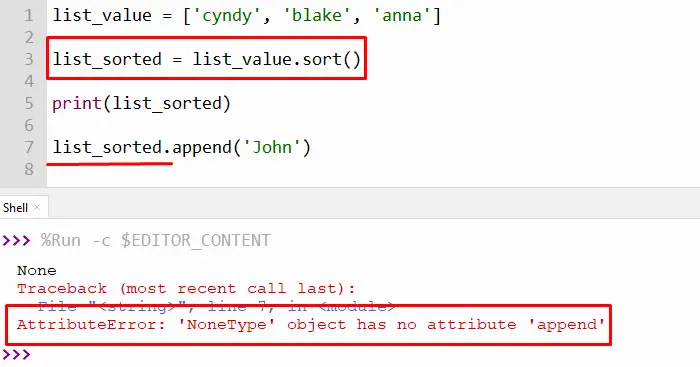

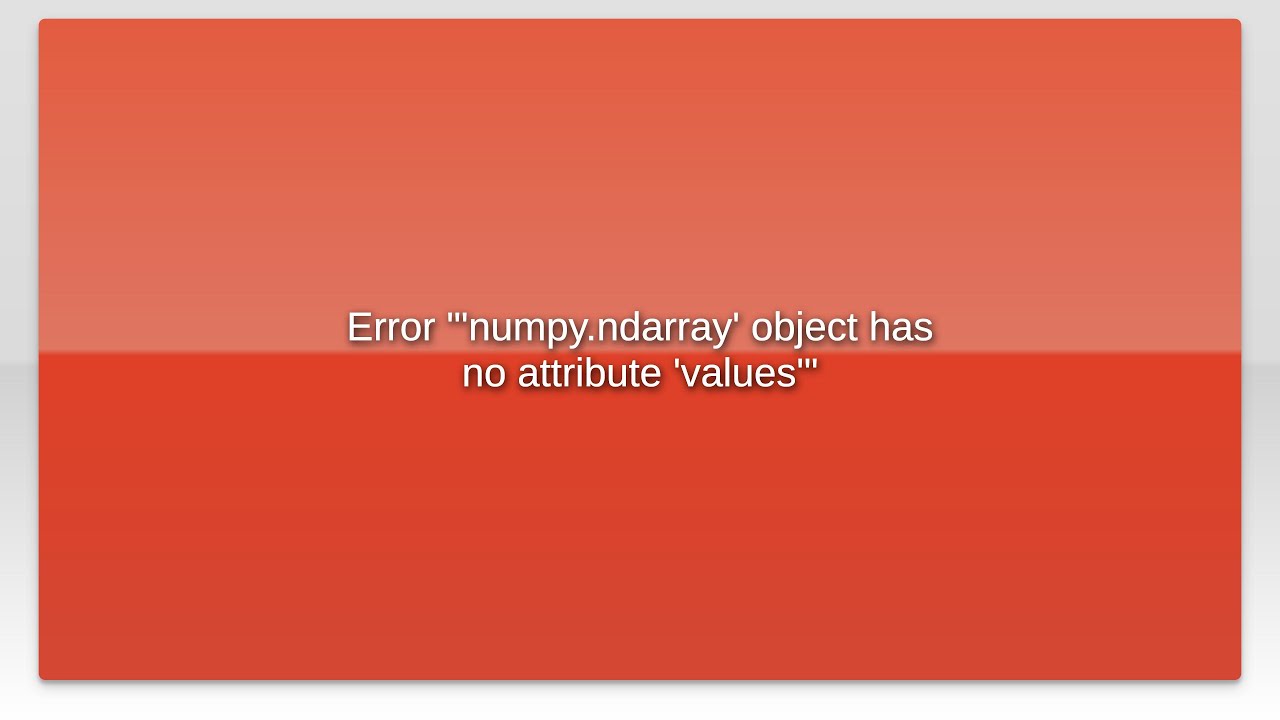

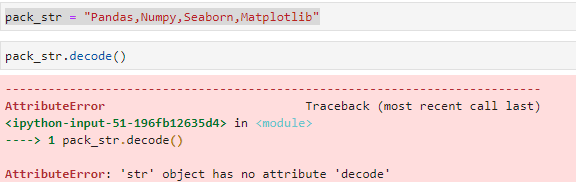

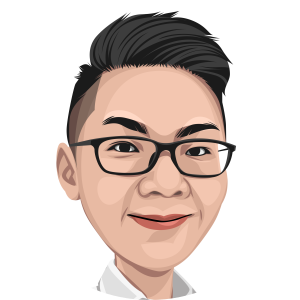



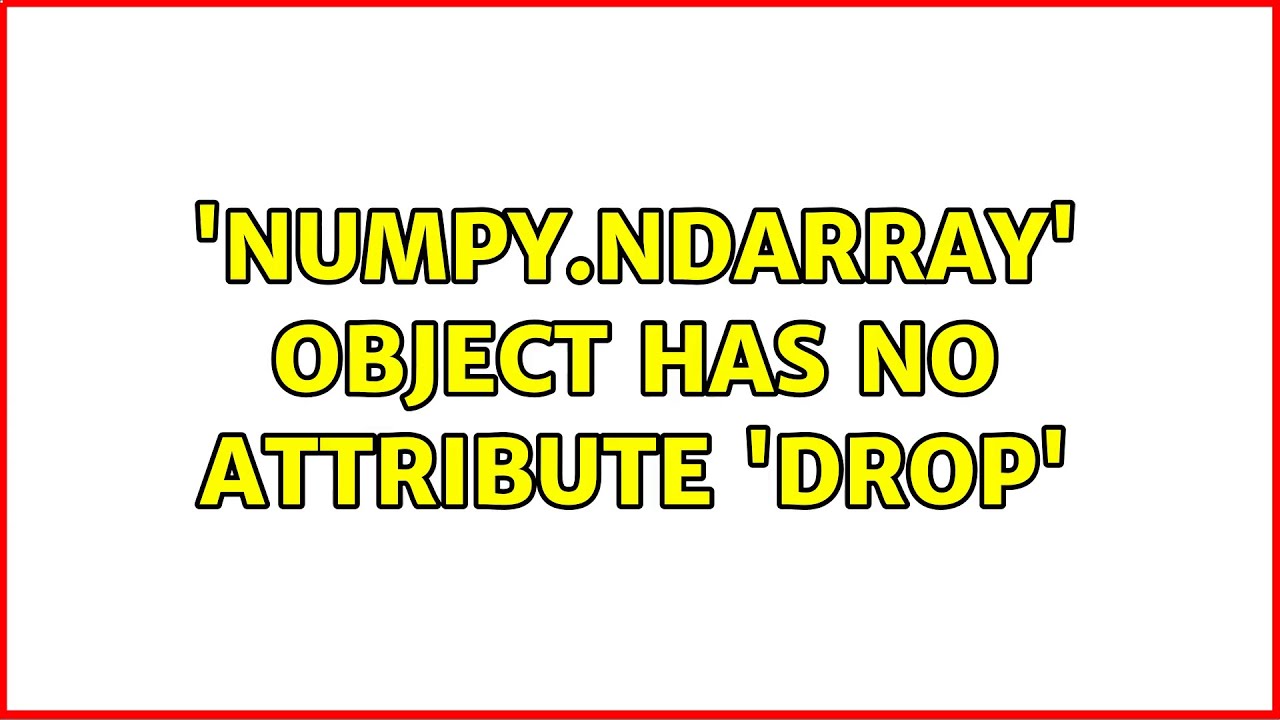
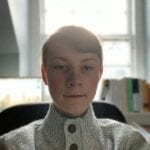


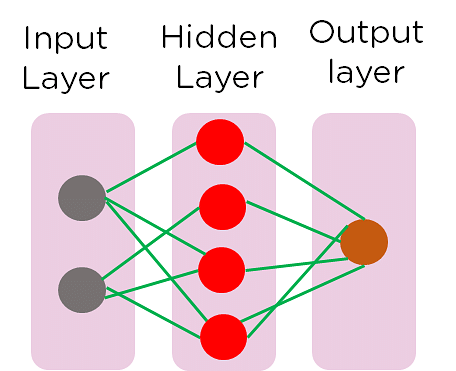
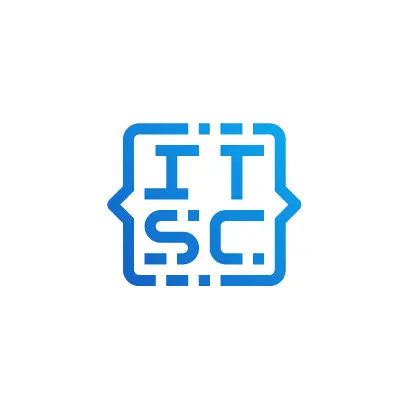

Article link: attributeerror ‘numpy.ndarray’ object has no attribute ‘append’.
Learn more about the topic attributeerror ‘numpy.ndarray’ object has no attribute ‘append’.
- How to Fix: ‘numpy.ndarray’ object has no attribute ‘append’
- AttributeError: ‘numpy.ndarray’ object has no attribute ‘append’
- numpy.ndarray object has no attribute append ( Solved )
- ‘numpy.ndarray’ object has no attribute ‘append’ Solution
- AttributeError: ‘numpy.ndarray’ object has no attribute ‘append’
- How to Fix: ‘numpy.ndarray’ object has no attribute ‘append’
- ‘numpy.ndarray’ object has no attribute ‘append’ – AppDividend
- (SOLVED) numpy.ndarray object has no attribute append
- numpy.ndarray object has no attribute append error
- ‘numpy.ndarray’ Object Has No Attribute ‘Append’ in Python
See more: nhanvietluanvan.com/luat-hoc