Asio Socket.Set_Option Failed With Std::System_Error
The Asio Library is an open-source C++ library that provides a cross-platform network programming framework. It offers a set of asynchronous I/O functions and classes that simplify the development of efficient and reliable network applications. One important aspect of network programming is socket configuration, which involves setting various options for a socket to control its behavior.
Understanding Socket Options and Set Option Function
Socket options allow developers to customize the behavior of a socket to suit their specific requirements. These options can control various aspects such as the socket’s timeout, buffer size, and protocol-specific behavior. The Asio Library provides a convenient way to manipulate these options using the `socket.set_option` function.
The `set_option` function is used to set the value of a specific socket option. It takes two arguments: the option to set and its corresponding value. The option is typically defined through an object that encapsulates its properties. For example, to set the timeout option, you would use the `boost::asio::socket_base::receive_timeout` object.
Common Causes of std::system_error When Using set_option
While using the `set_option` function, you might encounter the `std::system_error` exception. This exception indicates a failure in executing the `set_option` operation. There can be several reasons why this error occurs:
1. Permission issues: If the process does not have sufficient privileges to modify the socket options, a permission error can occur. For example, if the socket file is located in a directory that requires elevated permissions, the operation might fail with a “failed to unlink socket file” or “sock operation not permitted” error.
2. Incorrect or non-existent path: If the specified path for the socket file or any other related resource is incorrect or non-existent, the `set_option` operation can fail. This can manifest as a “nonexistentpath: data directory /data/db not found” error.
3. Address already in use: If the specified address is already being used by another socket, the `set_option` operation will fail. This can occur when multiple sockets are bound to the same address and port combination, resulting in a “socketexception: address already in use” error.
4. I/O errors: If there are any input/output errors during the execution of the `set_option` operation, it can result in a failure. This can be caused by various factors such as a network problem or a disk issue. Common errors in this scenario include “bootstrap failed: 5: input/output error” and “load failed: 5: input/output error”.
Troubleshooting std::system_error with set_option
When encountering an `std::system_error` with the `set_option` function, there are several steps you can take to troubleshoot and resolve the issue:
1. Check permissions: Ensure that the process has sufficient permissions to modify the socket options. If necessary, elevate the privileges or change the file permissions to allow the operation.
2. Validate paths and resources: Verify that the paths specified for the socket file or any other related resources are correct and exist. If not, update the paths accordingly.
3. Investigate conflicting addresses: Identify if there are any other sockets bound to the same address and port combination. If found, either reconfigure the sockets to use different addresses or terminate the conflicting sockets.
4. Check for I/O errors and connectivity: Examine the system logs and network connectivity to determine if there are any input/output errors or network issues that could be causing the failure. Address any underlying problems that may exist.
Addressing Specific std::system_error Scenarios with set_option
Here are some specific scenarios and their respective resolutions that can help in addressing `std::system_error` during the `set_option` operation:
1. “failed to unlink socket file /tmp/mongodb-27017 sock operation not permitted”: Check file permissions for the specified socket file. Ensure that the process has the necessary permissions to unlink the file.
2. “nonexistentpath: data directory /data/db not found”: Verify that the specified data directory exists. If it doesn’t, create the directory or specify the correct path.
3. “socketexception: address already in use”: Identify the process using the conflicting address and terminate it or configure it to use a different address.
4. “bootstrap failed: 5: input/output error” or “load failed: 5: input/output error”: Investigate the underlying cause of the input/output error. It could be due to network connectivity or disk issues. Resolve the root cause to fix the problem.
Best Practices for Handling std::system_error in set_option
To handle `std::system_error` gracefully and improve the robustness of your code, consider the following best practices:
1. Use proper exception handling: Wrap the `set_option` call in a try-catch block and catch the `std::system_error` exception. This allows you to handle the exception appropriately without crashing the program.
2. Log and report errors: Include logging and reporting mechanisms to record the occurrence of `std::system_error` and any relevant details. This information can help in troubleshooting and diagnosing issues.
3. Provide meaningful error messages: When catching and handling `std::system_error`, display user-friendly error messages that explain the cause of the error. This enhances the user experience and facilitates understanding of the problem.
Advanced Use Cases and Alternative Approaches for set_option Error Handling
In certain advanced use cases, you may need to employ alternative approaches to handle errors related to `set_option`. Some possible approaches include:
1. Retry mechanism: Implement a retry mechanism to automatically retry the `set_option` operation in case of transient errors. This can help overcome temporary issues and improve the resilience of your code.
2. Graceful termination: In scenarios where the errors are critical and cannot be resolved easily, consider terminating the application gracefully. Provide appropriate error messages and close any open resources before exiting.
3. Alternative socket libraries: If you continue to face persistent `std::system_error` issues with `set_option`, consider exploring alternative socket libraries that provide better error handling mechanisms or more specific options for your use case.
Conclusion
Understanding and effectively handling `std::system_error` exceptions during the `socket.set_option` operation is crucial for developing robust and reliable network applications using the Asio Library. By following the troubleshooting steps, addressing specific scenarios, and adopting best practices, you can enhance the error handling capabilities of your code and ensure the smooth operation of your network applications.
Networking In C++ Part #2: Mmo Client/Server, Asio, Sockets \U0026 Connections
Keywords searched by users: asio socket.set_option failed with std::system_error failed to unlink socket file /tmp/mongodb-27017 sock operation not permitted, nonexistentpath: data directory /data/db not found, socket exception mongodb, bootstrap failed: 5: input/output error, failed to set up listener: socketexception: address already in use, zsh: command not found: mongo, load failed: 5: input/output error, mongod –config /usr/local/etc/mongod conf
Categories: Top 63 Asio Socket.Set_Option Failed With Std::System_Error
See more here: nhanvietluanvan.com
Failed To Unlink Socket File /Tmp/Mongodb-27017 Sock Operation Not Permitted
When working with MongoDB, you may encounter an error message stating “failed to unlink socket file /tmp/mongodb-27017.sock: Operation not permitted.” This error indicates that there is a problem with the MongoDB server, specifically with the socket file in the /tmp directory. In this article, we will explore the possible causes of this error and provide potential solutions to fix it.
Understanding the error message
The error message “failed to unlink socket file /tmp/mongodb-27017.sock: Operation not permitted” suggests that the MongoDB server is unable to remove or unlink the socket file located at /tmp/mongodb-27017.sock. This socket file is a communication channel used by MongoDB to establish connections between the server and clients.
Possible causes
There are several potential causes for this error. Let’s explore some of the most common ones:
1. Insufficient permissions: The first possibility is that the user running the MongoDB server does not have sufficient permissions to modify or delete the socket file. This can occur if the server process is running under a user with limited privileges.
2. Incorrect ownership: Another cause for this error is incorrect ownership of the socket file. If the file is owned by a different user or group than the one running the MongoDB server, the server will not have the necessary rights to manipulate it.
3. Active server instance: If there is already an active MongoDB server instance running, it may prevent the new server instance from creating or removing the socket file. This can occur when multiple instances of MongoDB are running simultaneously.
4. Filesystem limitations: Some filesystems may impose restrictions on certain file operations, such as unlinking files. If the /tmp directory is located on a filesystem with such limitations, it can lead to the “Operation not permitted” error.
Solutions
Now that we have identified some potential causes for the error, let’s discuss possible solutions to resolve it:
1. Check permissions: Start by verifying the permissions of the socket file and the directory containing it. Ensure that the user running the MongoDB server has sufficient rights to modify or delete files in the /tmp directory. You can use the `ls -l` command to view the ownership and permissions of the files.
2. Adjust ownership and permissions: If the socket file is owned by a different user or group, you can change the ownership using the `chown` command. For example, `sudo chown mongodb:mongodb /tmp/mongodb-27017.sock` changes the ownership to the mongodb user and group. Additionally, ensure that the permissions allow for modification or deletion using the `chmod` command.
3. Stop existing instances: If there are multiple instances of MongoDB running, stop all active instances before starting a new one. You can use the `mongod` command followed by the `–shutdown` option to gracefully stop the server. Once all instances are stopped, attempt to start the MongoDB server again.
4. Use a different directory: If the /tmp directory is located on a restricted filesystem, consider changing the location of the socket file to a different directory. In the MongoDB configuration file (mongod.conf), update the `unixDomainSocket` option to specify a different directory for the socket file. Remember to grant the necessary permissions for the new directory.
FAQs
Q1. Why am I getting the “Operation not permitted” error when trying to start the MongoDB server?
A1. This error usually occurs when the MongoDB server does not have sufficient permissions to modify or delete the socket file located in the /tmp directory. It can also be caused by incorrect ownership or an already active MongoDB instance.
Q2. How do I check the permissions of a file or directory?
A2. You can use the `ls -l` command in the terminal to display the ownership and permissions of files and directories. The permissions are represented by a series of letters (e.g., rwxr-xr-x), indicating read, write, and execute permissions for the file owner, group, and others.
Q3. Can I change the location of the socket file?
A3. Yes, you can change the location of the socket file by modifying the MongoDB configuration file (mongod.conf). Update the `unixDomainSocket` option to specify a different directory for the socket file.
Q4. Do I need to restart the server after making changes to the socket file’s ownership or permissions?
A4. Yes, after modifying the ownership or permissions of the socket file, restart the MongoDB server for the changes to take effect.
Conclusion
The “failed to unlink socket file” error in MongoDB is often caused by insufficient permissions, incorrect ownership, active server instances, or filesystem limitations. By checking and adjusting the file permissions and ownership, stopping existing instances, or changing the socket file’s location, you can resolve this error and successfully start the MongoDB server. Remember to restart the server after making any modifications.
Nonexistentpath: Data Directory /Data/Db Not Found
Introduction:
For those who have ventured into the world of databases and are familiar with MongoDB, you may have encountered a peculiar error message: “NonexistentPath: Data directory /data/db not found.” This perplexing message can leave many scratching their heads, unsure of what steps to take next. In this comprehensive article, we will delve into the reasons behind this error, explore potential solutions, and address some frequently asked questions to help you navigate through this issue with ease.
Understanding the Error:
The error message “NonexistentPath: Data directory /data/db not found” typically occurs when MongoDB is unable to locate the specified data directory. This error signifies that the default data directory for MongoDB, which is ‘/data/db,’ is missing or inaccessible. MongoDB uses this directory to store its data files, including database collections, indexes, and other relevant data.
Common Causes:
1. Improper Installation: One of the primary reasons for encountering this error is an incorrect or incomplete installation of MongoDB. During the installation process, if the necessary directories and permissions are not properly set up, MongoDB may fail to find the data directory.
2. Insufficient Privileges: Another reason for this error may be insufficient privileges or incorrect file permissions on the data directory. MongoDB requires read and write access to the specified directory to function seamlessly. If the permissions are not set correctly, it may lead to this error.
3. Unspecified Custom Data Directory: By default, MongoDB expects the data directory to be in ‘/data/db.’ However, if you have specified a custom data directory during the setup process, MongoDB will search for data in the specified location. If the custom directory is not accessible or does not exist, the error message will be triggered.
Troubleshooting Steps:
1. Verify Data Directory Existence: Start by confirming the existence of the specified data directory. Open the command prompt or terminal and navigate to the default data directory (‘/data/db’) or the custom directory if one has been set. Check for any accidental deletions, renames, or issues with the disk that may have caused the directory to become inaccessible.
2. Check File Permissions: Ensure that the user running the MongoDB process has appropriate read and write permissions on the data directory. On Unix-like systems, you can use the ‘ls -ld /data/db’ command to view the directory’s permissions. If required, modify the permissions using ‘chmod’ to grant the necessary privileges.
3. Create Missing Data Directory: If the data directory is missing, you can create it manually. Use the ‘mkdir -p /data/db’ command to create the default data directory. Don’t forget to assign proper ownership and permissions to ensure MongoDB can access the newly created directory.
4. Configure Custom Data Directory: If you have specified a custom data directory during installation or configuration, make sure the path is correct. Verify that the custom directory actually exists and has the necessary permissions assigned to it. Adjust the MongoDB configuration file to reflect the custom data directory path, which typically resides within the ‘/etc’ directory.
Frequently Asked Questions:
Q1. Can I change the default data directory for MongoDB?
A1. Yes, MongoDB allows you to specify a custom data directory during the installation or in the configuration file. However, ensure the new directory exists and has the required permissions before making any changes.
Q2. I do not have administrative privileges. How can I resolve this issue?
A2. If you do not have administrative privileges to modify the data directory or permissions, reach out to your system administrator or IT department. They can assist you in creating or modifying the necessary directories and permissions.
Q3. Is it possible to recover data if the data directory is accidentally deleted?
A3. Unfortunately, if the data directory is deleted and no backup is available, it can be challenging to recover the data. Regularly backing up your MongoDB data is highly recommended to prevent data loss in such scenarios.
Q4. What other locations can be used as the data directory?
A4. While ‘/data/db’ is the default data directory for MongoDB, you can specify any valid directory as long as it has the correct permissions and is accessible by the MongoDB process.
Conclusion:
Encountering the “NonexistentPath: Data directory /data/db not found” error during MongoDB operations can be frustrating. However, armed with the knowledge gained from this article, you can identify potential causes and troubleshoot the issue effectively. By ensuring the correct data directory existence, verifying permissions, and properly configuring custom paths, you can overcome this error and resume working with MongoDB smoothly. Remember to regularly backup your data to minimize the risk of irreversible loss.
Images related to the topic asio socket.set_option failed with std::system_error
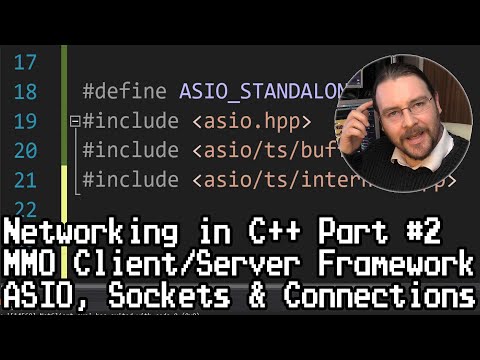
Found 19 images related to asio socket.set_option failed with std::system_error theme

Article link: asio socket.set_option failed with std::system_error.
Learn more about the topic asio socket.set_option failed with std::system_error.
- MongoDB 5.0 Fails to run in Mac OS with Homebrew Error …
- Unable to run MongoDB Community v6.1 after installation on …
- Cannot upgrade to latest v6.1.5 from 6.0.0 #56 – GitHub
- Cannot update / migrate 4.x database to 5.x or 6.x
- MongoDB Getting Started – Websoft9
- boost/asio/basic_socket.hpp – 1.63.0
- ‘mskalick pushed to mongodb (master). “Update to … – Marc.info
- Program Listing for File base_socket.ipp – stream-client
See more: nhanvietluanvan.com/luat-hoc