Array Indices Must Be Positive Integers Or Logical Values.
Understanding array indices
An array is a data structure that allows storage of multiple values under a single variable name. Each element in an array is assigned an index, which helps in accessing and manipulating specific elements within the array. Array indices represent the position of elements within the array, with the first element typically assigned an index of 1.
The significance of positive integers as array indices
Positive integers are the most commonly used type of array indices. They provide a straightforward and intuitive way to access elements within an array. By using positive integers, programmers can easily iterate through an array using a for loop, as the indices act as a guide for traversing the elements sequentially.
Why logical values can be used as array indices
In addition to positive integers, logical values can also be used as array indices in certain programming languages like MATLAB. Logical values, represented by the keywords “true” and “false”, can help in selecting specific elements from an array based on certain conditions. This allows for more dynamic and flexible array operations.
Limitations with negative integers as array indices
The use of negative integers as array indices is generally not allowed in most programming languages. This is because negative indices would imply accessing elements from the end of the array, which can lead to confusion and errors. For example, if an array has a length of 5, using a negative index of -1 would refer to the last element. Such usage is prone to mistakes and can make code difficult to understand and maintain.
Implications of using non-integer values as array indices
Non-integer values, such as decimals or strings, are not appropriate for array indices. Array indices must be whole numbers or logical values to ensure the proper functioning of array operations. Using non-integer values as array indices can result in unexpected behavior and errors. It is essential to ensure that indices are valid and within the bounds of the array length.
Error handling for invalid array indices
When using array indices, it is crucial to handle errors that may arise. If an invalid index, such as a negative integer or a non-integer value, is used, the program should provide appropriate error messages to inform the programmer about the issue. Error handling mechanisms like exception handling can help in gracefully handling such situations.
Best practices for working with array indices
To ensure reliable and efficient array operations, it is recommended to follow certain best practices when working with array indices:
1. Always use positive integers or logical values as array indices.
2. Validate the indices to ensure they are within the bounds of the array length.
3. Handle errors and exceptions that may occur due to invalid indices.
4. Use meaningful variable and index names to improve code readability.
5. Comment code sections where array indices are used extensively to enhance code understanding and maintenance.
Examples of using logical values as array indices
In MATLAB, logical values can be used as indices to select specific elements from an array based on a logical condition. For example:
“`matlab
A = [1, 2, 3, 4, 5];
logical_indices = [true, false, true, false, true];
selected_elements = A(logical_indices);
“`
In this example, the logical_indices array selects the elements in A at the corresponding positions where the value is true. This allows for advanced filtering and manipulation of array elements.
Advantages and disadvantages of using logical values as array indices
Using logical values as array indices can have certain advantages and disadvantages. Some advantages include increased flexibility in extracting array elements based on logical conditions, simplification of complex indexing operations, and improved code readability. However, the use of logical indices can potentially result in slower array operations and increased memory usage.
FAQs:
Q: What programming languages allow the use of logical values as array indices?
A: Languages like MATLAB allow the use of logical values as array indices.
Q: Can negative integers be used as array indices?
A: Negative integers are generally not allowed as array indices in most programming languages.
Q: What happens if an invalid array index is used?
A: Using an invalid array index can result in errors or unexpected behavior. It is important to handle such situations properly through error handling mechanisms.
Q: What are some best practices for working with array indices?
A: Best practices include using positive integers or logical values as indices, validating indices, handling errors, using meaningful names, and commenting code sections involving array indices extensively.
Q: Are there any limitations when using non-integer values as array indices?
A: Non-integer values, such as decimals or strings, should not be used as array indices as they can lead to errors and unexpected behavior.
Trying To Fix A Problem In A Matlab Code (Array Indices Must Be Positive Integers Or Logical Values)
What Is Array Indices Must Be Positive Integers Or Logical Values?
In programming, an array is a data structure that stores a collection of elements of the same type. Each element in an array is associated with an index, which represents its position within the array. These indices are crucial for accessing and manipulating the array’s elements. It is a fundamental rule in most programming languages that array indices must be positive integers or logical values.
Positive integers, also known as natural numbers, are whole numbers greater than zero. They are used as indices to access specific elements in an array. By using positive integers as indices, programmers can easily identify and retrieve elements based on their position in the array. This allows for efficient and organized data manipulation.
Logical values, on the other hand, refer to boolean values, which can be either true or false. In certain programming languages, such as MATLAB, logical values can also be used as array indices. This allows for conditional access to array elements based on certain criteria or conditions. For example, one can access elements in an array based on whether they meet a specific condition, such as being greater than a certain value or equal to a certain value.
The requirement for array indices to be positive integers or logical values ensures the predictability and consistency of array operations. If indices were not restricted to these types, it could lead to ambiguity and potential errors. For instance, if negative numbers were allowed as indices, it would introduce ambiguity in array indexing, as it would be unclear how to interpret a negative index (e.g., whether it means accessing elements from the end of the array). Similarly, allowing non-integer values as indices could create confusion and make it harder to determine which element is being accessed.
Furthermore, using positive integers or logical values as indices simplifies array operations. It follows a straightforward and intuitive numbering system, where the first element of an array is typically assigned an index of 1, the second element has an index of 2, and so on. This allows programmers to easily calculate the index of a desired element based on its position within the array. For example, accessing the fifth element of an array can be done by specifying an index of 5.
FAQs:
Q: Can array indices be negative integers?
A: No, array indices must be positive integers or logical values. Negative integers are not allowed as indices because they introduce ambiguity in array indexing.
Q: Can array indices be fractional or decimal numbers?
A: No, array indices must be positive integers or logical values. Fractional or decimal numbers are not allowed as indices because they are not discrete values that represent valid positions within an array.
Q: Can array indices be characters or strings?
A: In most programming languages, indices cannot be characters or strings. They must be positive integers or logical values. However, some languages may allow for character-based indexing, where individual characters within a string can be accessed using their positions.
Q: Why are logical values allowed as array indices?
A: Logical values provide a means of conditional indexing, allowing for access to array elements based on specific conditions or criteria. This can be useful in certain programming scenarios.
Q: Are there any exceptions to the rule of array indices being positive integers or logical values?
A: While the general rule is that indices must be positive integers or logical values, there may be some programming languages or specific scenarios where this rule can be relaxed or modified. It is important to consult the documentation or guidelines of the specific language being used to determine the exact rules and limitations regarding array indices.
What Is Array Indices In Matlab?
In MATLAB, an array is a fundamental data type that allows you to store and manipulate collections of values. Array indices in MATLAB refer to the position of an element within an array. Each element in an array is uniquely identified by its indices, which enable you to access and manipulate specific elements within the array.
Understanding how array indices work in MATLAB is crucial for performing various operations on arrays, such as accessing specific elements, modifying values, and performing mathematical computations. Therefore, let’s explore the concept of array indices in MATLAB in depth.
Understanding Array Indices:
In MATLAB, array indices are integers that specify the position or location of an element within an array. The indices of a MATLAB array are zero-based, meaning the first element is identified by an index of zero, the second element by an index of one, and so on.
To access a specific element in an array using its indices, you need to specify the indices within parentheses following the name of the array. For example, if you have an array named “A”, you can access its first element using the syntax “A(1)”.
Array indices in MATLAB can be positive integers, negative integers, or logical values. Positive indices represent elements of an array from the first to the last element, while negative indices indicate elements in reverse order, starting from the last element (-1 refers to the last element).
If you use a logical value as an index, MATLAB will evaluate it as either true or false, where true represents the indices that are selected, and false represents the indices that are not selected. This feature makes logical indices extremely powerful for selective operations on arrays.
Multi-Dimensional Array Indices:
In MATLAB, you can create arrays with more than one dimension, known as multi-dimensional arrays. The concept of array indices extends to multidimensional arrays as well. For a two-dimensional array, the indices are used to specify both the row and column position of the element.
For example, if you have a two-dimensional array named “B”, you can access an element at the second row and third column using the syntax “B(2, 3)”. Similarly, for a three-dimensional array, you would need to specify the indices along each dimension.
FAQs:
Q: Can I use non-integer values as array indices in MATLAB?
A: No, MATLAB only supports integer values (positive, negative, or logical) as array indices.
Q: How can I access multiple elements from an array at once?
A: MATLAB allows you to specify an array of indices to access multiple elements simultaneously. For example, to access the first, third, and fifth elements of an array “C”, you can use the syntax “C([1, 3, 5])”.
Q: How can I modify elements in an array using their indices?
A: You can modify array elements by assigning new values to them using the index notation. For example, to change the value of the fourth element in an array “D” to 10, you can use the syntax “D(4) = 10”.
Q: Can I use variables as array indices in MATLAB?
A: Yes, MATLAB allows you to use variables as indices. The variable should contain an integer value that represents a valid index position within the array.
Q: Are array indices in MATLAB case-sensitive?
A: No, array indices are not case-sensitive in MATLAB. MATLAB treats uppercase and lowercase indices as equivalent.
Q: Can I perform mathematical operations on array indices in MATLAB?
A: While array indices are generally used for accessing and manipulating array elements, you can perform basic mathematical operations on them such as addition, subtraction, multiplication, and division.
In conclusion, array indices in MATLAB play a critical role in accessing, modifying, and manipulating elements within arrays. By understanding how to work with array indices, you can effectively harness the power of MATLAB arrays for various computational tasks. Remember to adhere to the zero-based indexing convention, and feel free to use negative or logical indices when appropriate.
Keywords searched by users: array indices must be positive integers or logical values. array indices must be positive integers or logical values. matlab for loop, array indices must be positive integers or logical values reddit, Index in position 2 is invalid array indices must be positive integers or logical values, Array indices must be positive integers or logical values, Index in position 1 is invalid array indices must be positive integers or logical values, array indices matlab, Index exceeds the number of array elements index must not exceed 1, matlab logical values
Categories: Top 100 Array Indices Must Be Positive Integers Or Logical Values.
See more here: nhanvietluanvan.com
Array Indices Must Be Positive Integers Or Logical Values. Matlab For Loop
Introduction:
MATLAB is a powerful programming language widely used for numerical computations and data analysis. One of the fundamental concepts in MATLAB is array indexing. When working with arrays, it is crucial to understand that array indices must be positive integers or logical values. This article will delve into the details of array indexing, particularly focusing on the for loop in MATLAB. By the end, you will have a comprehensive understanding of this essential concept.
Understanding Array Indexing:
Array indexing plays a vital role in accessing and manipulating elements within arrays in MATLAB. Arrays are one of the primary data structures in MATLAB, and they can be one-dimensional (vectors), two-dimensional (matrices), or multidimensional. Indexing allows you to specify the position of elements in an array to perform operations on them.
In MATLAB, array indices must be positive integers or logical values. A positive integer index refers to the position of the element within the array, starting from 1. On the other hand, a logical value index can be used to access elements of an array based on a logical condition. For example, you can use logical indexing to retrieve all elements in an array that satisfy a specific criterion.
The For Loop in MATLAB:
One of the primary ways to iterate over elements in an array is by using a for loop. A for loop repeatedly executes a block of code for a specified number of times, allowing you to access and manipulate different array elements. The syntax for a basic for loop in MATLAB is as follows:
“`
for index = starting_value:step_value:ending_value
% Code to be executed
end
“`
Let’s break down the components of the for loop:
– `index`: The loop variable that takes on different values during each iteration.
– `starting_value`: The initial value for the loop variable.
– `step_value`: The increment or decrement between consecutive loop variable values.
– `ending_value`: The final value for the loop variable.
Throughout each iteration, the loop variable can be used to access elements within an array using indexing. For example, if you have a vector `A` with `N` elements, you can access each element in the vector using `A(index)` inside the for loop.
FAQs about Array Indices and the For Loop in MATLAB:
Q: Can I use negative indices to access elements in an array?
A: No, MATLAB does not support negative indices. Array indices must always be positive integers or logical values.
Q: Can I use non-integer indices to access elements in an array?
A: No, array indices in MATLAB must be positive integers or logical values. Non-integer indices, such as floating-point numbers, are not valid.
Q: Can I change the step value in a for loop dynamically?
A: Yes, the step value can be a variable or an expression that is updated dynamically within the loop. However, it is essential to ensure that the loop termination condition can be reached.
Q: How can I access elements in a multidimensional array using a for loop?
A: MATLAB supports nested for loops for multidimensional arrays. You can use multiple loop variables to iterate over different dimensions of the array.
Q: Are there any alternatives to using for loops for array manipulation in MATLAB?
A: Yes, MATLAB offers various vectorized operations and functions that can efficiently manipulate arrays without the need for explicit loops. Vectorized operations make use of optimized algorithms to perform computations on entire arrays rather than individual elements.
Q: Can logical indexing be combined with a for loop?
A: Yes, you can use logical indexing within a for loop to conditionally access and manipulate elements in an array. Logical conditions can be based on any desired criterion.
Conclusion:
Understanding that array indices must be positive integers or logical values is crucial when working with arrays in MATLAB. The for loop is a powerful construct to iterate over array elements and enables you to perform operations on them efficiently. By adhering to the rules of array indexing, you can effectively manipulate arrays in MATLAB, unleashing the full potential of this popular programming language.
Array Indices Must Be Positive Integers Or Logical Values Reddit
Arrays, a fundamental data structure in computer programming, allow us to store and manipulate collections of data. In various programming languages, including popular ones like Python, Java, and C++, array indices must be positive integers or logical values. This article aims to provide a comprehensive understanding of this fundamental rule, explaining its significance, its implications, and addressing common queries surrounding this topic.
Why Must Array Indices be Positive Integers or Logical Values?
Arrays are designed to organize and access data efficiently. By using indices, we can refer to specific elements within an array. However, there is a fundamental reason why indices need to be positive integers or logical values. This is because indices serve as an address or location within the array, allowing for quick retrieval of data. Using positive integers helps establish a direct relationship between the index and the memory location where an element is stored. Logical values, such as true/false in languages like MATLAB, can also be used to support indexing in a structured manner.
1. Implications of Positive Integer Indices:
Using positive integers as indices provides a mapping that helps programmers access elements within an array quickly. This mapping allows for constant-time retrieval, as one simple arithmetic calculation can determine the memory location of an element based on its index. For example, if the starting memory address of an array is known, adding the index multiplied by the size of each element can directly point to the desired location. This simplicity and efficiency make positive integer indices crucial in array manipulation operations.
2. Implications of Logical Value Indices:
While positive integers indices are widely used, some programming languages also support logical value indices. For instance, in MATLAB, logical value indexing allows for flexible and concise syntax to select specific array elements based on certain conditions. These conditions can include checking for logical statements like “greater than,” “less than,” or “equal to.” Logical value indices offer a powerful mechanism for filtering and manipulating arrays, enhancing the functionality and expressiveness of the code.
Commonly Asked Questions:
Q1: What happens if we use negative or non-integer indices?
Using negative or non-integer indices typically leads to runtime errors, exceptions, or unpredictable behavior. Such behavior often arises due to the underlying implementation of arrays. Since arrays are allocated memory in a continuous and linear fashion, a negative or non-integer index would not correspond to a valid memory location. Consequently, accessing or modifying elements using these indices may result in memory violations, crashes, or data corruption. It is crucial to ensure that array indices conform to the required positive integer or logical value format to maintain program stability and correctness.
Q2: Are there any exceptions to this rule?
Yes, some programming languages provide advanced features, such as off-by-one indexing or zero-based indexing. These exceptions can affect the interpretation of array indices. For instance, in languages like C and C++, arrays are zero-indexed, meaning the first element is accessed using index 0. Similarly, other languages support arbitrary indexing where the programmer can customize the starting index. Despite these exceptions, the underlying requirement remains the same; indices must be positive integers or logical values, albeit with certain adjustments.
Q3: Why don’t all programming languages support arbitrary indexing?
While flexible indexing might seem appealing, it can introduce complexity and potential confusion. A standardized approach of using only positive integers or logical values simplifies the interpretation and implementation of array operations across different programming languages. Additionally, allowing arbitrary indexing could lead to inefficient memory usage, as separate memory allocations might be required to accommodate custom indexing schemes. Therefore, the consistency and accessibility provided by positive integer or logical value indices outweigh the benefits of arbitrary indexing in most scenarios.
Conclusion:
Understanding the requirement for positive integer or logical value indices in arrays is vital for writing reliable and efficient code. By adhering to this fundamental rule, programmers can harness the full potential of array-based data structures while avoiding runtime errors or unexpected behavior. Whether it’s simplifying array accessing or maximizing code expressiveness with logical value indices, the positive integer or logical value restriction ensures structured and predictable manipulation of arrays across various programming languages.
Images related to the topic array indices must be positive integers or logical values.
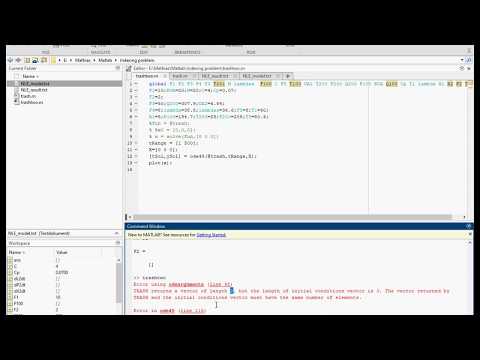
Found 49 images related to array indices must be positive integers or logical values. theme
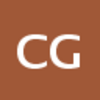


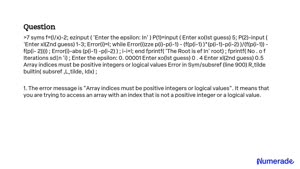


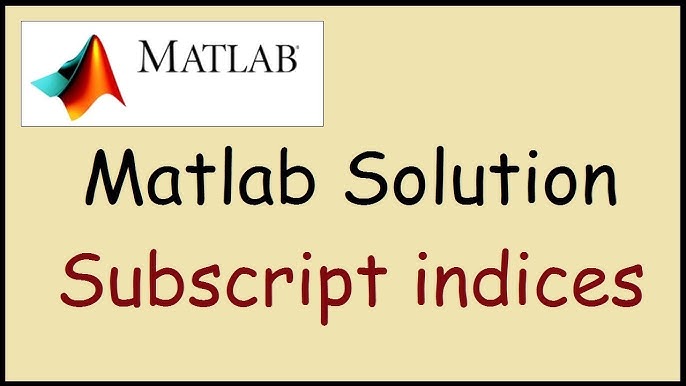
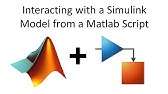
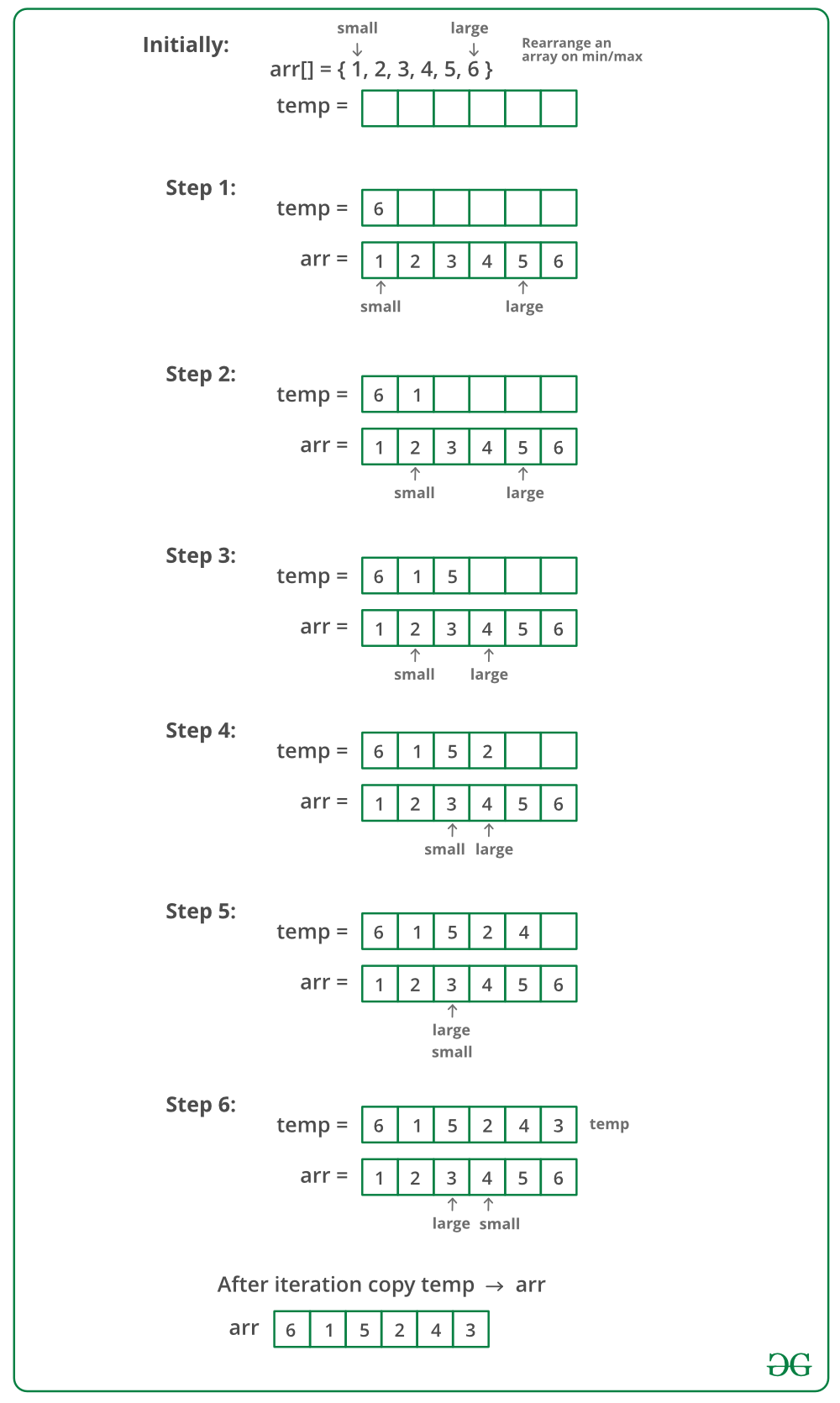
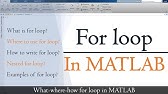
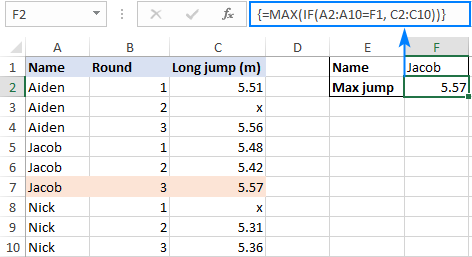
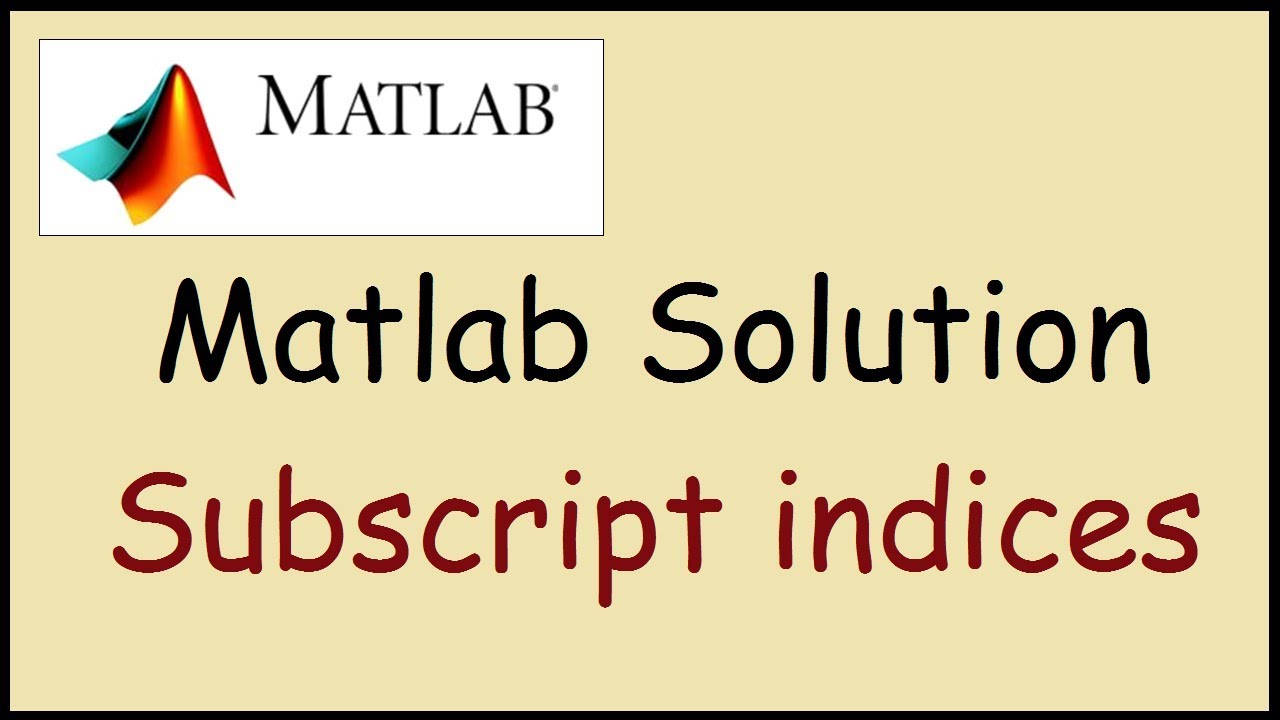
Article link: array indices must be positive integers or logical values..
Learn more about the topic array indices must be positive integers or logical values..
- Array indices must be positive integers or logical values
- 4.5: Indexing Errors – Engineering LibreTexts
- Array Indexing – MATLAB & Simulink – MathWorks
- Logical (Boolean) Operations – MATLAB & Simulink – MathWorks
- Resolve Error: Undefined Function or Variable – MATLAB & Simulink
- 4.5: Indexing Errors – Engineering LibreTexts
- How to fix error “Array indices must be positive integers or …
- in position 1 is invalid. Array indices must be positive integers …
- functions – “Index in position 1 is invalid. Array indices must be …
- 0 ref PS selected, Array indices must be positive integers or …
- in position 2 is invalid. Array indices must be positive integers …
See more: https://nhanvietluanvan.com/luat-hoc