Arithmetic Operation Resulted In An Overflow
Arithmetic operations are fundamental in programming and mathematical calculations. However, there are instances when these operations can result in an overflow, leading to unexpected outcomes and potential risks. In this article, we will explore what an arithmetic operation overflow is, its causes, consequences, and risks, as well as methods to detect and prevent it. We will also examine examples of arithmetic operation overflows in different programming languages and discuss strategies for handling them.
## What is an Arithmetic Operation Overflow?
An arithmetic operation overflow occurs when the result of a mathematical calculation exceeds the maximum or minimum value that can be stored in a given data type. Typically, computers allocate a fixed number of bits to represent numbers, and when the result exceeds the number of bits available, an overflow happens. This can lead to incorrect or unpredictable outcomes and, in some cases, even system crashes.
## Causes of Arithmetic Operation Overflow
There are several reasons why an arithmetic operation can result in an overflow. Some common causes include:
1. Insufficient data type size: Choosing an inadequate data type with limited size to store the result of an operation can lead to an overflow. For example, using a 16-bit integer to store the sum of two large numbers might result in an overflow if the result is too large to fit within 16 bits.
2. Integer division: Dividing a larger number by a smaller one using integer division can also lead to an overflow. Integer division truncates the decimal part of the result, which can cause unexpected results if the quotient exceeds the range of the data type.
3. Incorrect input validation: Failing to properly validate input values can introduce the possibility of an overflow. If user input is not properly checked or sanitized, it could contain values that result in arithmetic operations producing overflow.
## Consequences and Risks of Arithmetic Operation Overflow
An arithmetic operation overflow can have several consequences and introduce potential risks:
1. Incorrect results: When an overflow occurs, the result of the operation may be incorrect or inaccurate. This can lead to erroneous calculations and potentially compromise the integrity of the program or system.
2. Data corruption: If the overflow is not handled properly, it can corrupt data or overwrite critical memory sections. This can result in data loss, crashes, and security vulnerabilities.
3. System crashes or hangs: In some cases, an arithmetic operation overflow can cause the entire system to crash or hang. This can lead to disruption of services, loss of user data, and significant financial or reputational damage.
4. Security vulnerabilities: If an overflow is not well-handled, it can be exploited by attackers to execute malicious code, gain unauthorized access, or compromise the system’s security.
## How to Detect and Prevent Arithmetic Operation Overflow
To prevent arithmetic operation overflows, it is essential to implement proper detection mechanisms and preventative measures. Here are some strategies to consider:
1. Choose appropriate data types: Ensure that you select data types with enough capacity to store the expected results. For example, if you anticipate large values, consider using a larger data type such as a long integer instead of a standard integer.
2. Validate user input: Implement robust input validation to ensure that user-supplied values fall within acceptable ranges and prevent malicious inputs that may lead to an overflow.
3. Implement error checking and handling: Utilize appropriate error checking mechanisms to detect potential overflows during calculations. This involves checking for the limits of the data type and handling any overflow situations gracefully, rather than letting them propagate silently.
4. Use libraries or frameworks with built-in checks: Many modern programming languages and frameworks offer libraries or built-in functions that handle arithmetic operations with automatic overflow detection and prevention. Utilize these features whenever possible to reduce the likelihood of an overflow.
5. Test and verify extensively: Thoroughly test your code to identify potential overflow scenarios and ensure the correct handling of these situations. Use appropriate test cases to cover a wide range of input values and check the resulting output for expected behavior.
## Examples of Arithmetic Operation Overflow in Different Programming Languages
Let’s explore a few examples of arithmetic operation overflows in different programming languages:
### Arithmetic Operation Resulted in an Overflow C#
“`csharp
int a = int.MaxValue; // Largest possible value for an int
int b = 1;
int result = a + b; // Overflow occurs
“`
### Arithmetic Operation Resulted in an Overflow Excel
“`excel
=A1 + 1
“`
When cell A1 contains the maximum value that Excel supports, adding 1 to it will result in an overflow.
### Arithmetic Operation Resulted in an Overflow SQL
“`sql
DECLARE @a INT = 2147483647; — Maximum value for an integer
DECLARE @b INT = 1;
DECLARE @result INT;
SET @result = @a + @b; — Overflow occurs
“`
### Arithmetic Operation Resulted in an Overflow Visual Studio 2019
In Visual Studio 2019, if you attempt to perform an arithmetic operation that exceeds the data type’s range, the IDE will raise an overflow exception. This helps developers detect and handle such situations during development.
### Arithmetic Operation Resulted in an Overflow C# Sum
“`csharp
int[] numbers = { 1000000000, 1000000000 };
int sum = numbers.Sum(); // Overflow occurs during the sum calculation
“`
### Arithmetic Operation Resulted in an Overflow C# Byte Array
“`csharp
byte[] data = { 255, 255, 255, 255 };
byte[] result = new byte[4];
Buffer.BlockCopy(data, 0, result, 0, data.Length); // Overflow occurs during the copy operation
“`
### Arithmetic Operation Resulted in an Overflow VB.NET
“`vb.net
Dim a As Integer = Integer.MaxValue ‘ Maximum value for an Integer
Dim b As Integer = 1
Dim result As Integer
result = a + b ‘ Overflow occurs
“`
## Strategies for Handling Arithmetic Operation Overflow when it Occurs
When encountering an arithmetic operation overflow, it is crucial to handle it properly to ensure the stability and integrity of your program or system. Here are some strategies for handling overflows:
1. Throw an exception: In languages that support exceptions, you can throw an overflow exception and catch it at an appropriate level in your code. This allows you to handle the overflow gracefully by providing an error message to the user or taking corrective actions.
2. Use data type limits: Check the limits of the data type being used and compare the result of the operation against those limits. If the result exceeds the limits, take appropriate action, such as setting the result to the maximum or minimum value allowed by the data type.
3. Implement saturation arithmetic: Saturation arithmetic is a technique that clamps the result of an operation to the maximum or minimum value of the data type when an overflow is detected. This ensures that the result stays within the allowable range, preventing unexpected behavior.
4. Scale or normalize values: If the range of values is known or limited, you can scale or normalize the inputs to prevent overflows. This involves dividing or multiplying the values by a suitable factor to bring them within the desired range before performing the operation.
5. Use bitwise operations: In some cases, bitwise operations can be used to handle arithmetic operation overflows. By manipulating the bits of a number, you can detect and handle overflows without relying on exceptions or additional checks.
## FAQs (Frequently Asked Questions)
1. Q: Can an arithmetic operation overflow occur in non-programming scenarios?
A: Yes, arithmetic operation overflows can occur in various domains beyond programming, such as financial calculations, scientific simulations, or digital circuit design.
2. Q: Are all programming languages equally prone to arithmetic operation overflows?
A: No, different programming languages have different data types and mechanisms to handle arithmetic operation overflows. Some languages, like Python, automatically adjust the data type depending on the result’s magnitude, reducing the chances of overflows. Others, like C or C++, require explicit handling.
3. Q: Are there any tools available for automatically detecting arithmetic operation overflows?
A: Yes, there are several static analysis and code review tools that can help identify potential arithmetic operation overflows in your codebase. These tools analyze your code’s control flow and data dependencies to highlight potential overflow scenarios.
4. Q: Can arithmetic operation overflows occur in floating-point calculations?
A: Yes, arithmetic operation overflows can occur in both integer and floating-point calculations. Floating-point overflows typically manifest as the result becoming infinite or NaN (Not-a-Number).
5. Q: Are there any performance implications for handling arithmetic operation overflows?
A: Depending on the chosen strategy for handling overflows, there may be a slight performance overhead. Techniques like data type checks, saturation arithmetic, or scaling values may introduce additional computation steps. However, the benefits of preventing overflows far outweigh the small performance impact.
By understanding what an arithmetic operation overflow is, recognizing its causes and potential consequences, and employing preventative measures, programmers can ensure the reliability and security of their code. By utilizing the examples and strategies discussed in this article, developers can handle arithmetic operation overflows confidently and effectively, ultimately leading to more robust and dependable software solutions.
Sql Arithmetic Overflow Error Converting Int To Data Type Numeric
What Does Arithmetic Operation Resulted In An Overflow Mean?
Arithmetic operations are the fundamental building blocks of mathematics and play a vital role in various fields, including computer science, engineering, and finance. These operations may involve addition, subtraction, multiplication, and division. However, sometimes these operations can result in an overflow, a concept that is important to understand.
An overflow occurs when the result of an arithmetic operation exceeds the maximum value that can be represented by the data type used for the calculation. In computer systems, data is stored in a fixed number of bits, and each bit can represent a certain range of values. For example, an 8-bit integer can represent values from -128 to 127. If an arithmetic operation exceeds these bounds, an overflow occurs.
Understanding the different types of overflows:
1. Integer Overflow: This type of overflow occurs when an arithmetic operation on integers results in a value that exceeds the maximum value that can be represented by the data type. For example, if two positive integers, both close to the maximum value of the data type, are added together, the result may be greater than the maximum value, causing an overflow.
2. Underflow: Although not commonly referred to as overflow, underflow is the reverse scenario where the result of an arithmetic operation is smaller than the minimum representable value of the data type. It occurs when the result falls below the lower bound. It is important to note underflow should not be confused with overflow; however, both cases lead to unexpected results.
3. Floating-Point Overflow: Floating-point numbers are used to represent real numbers and are commonly used in scientific calculations. Similar to integer overflow, floating-point overflow occurs when the result of an arithmetic operation, such as addition or multiplication, exceeds the maximum representable value for the given floating-point data type.
The consequences of overflow:
When an overflow occurs, it can lead to unexpected and incorrect results, which can have serious implications depending on the context in which it happens. In some cases, the overflow may be ignored, resulting in a wrap-around effect where the value wraps back to the lowest representable value in the data type.
In other cases, the overflow may trigger an error or exception, causing the program to terminate or produce abnormal behavior. These errors can be particularly problematic in critical systems like financial calculations or aerospace engineering, where precision and accuracy are crucial.
Common causes of overflow:
1. Inadequate data type choice: Choosing a data type that is unable to represent the range of values involved in an arithmetic operation can lead to overflow. It is important to select a data type that can accommodate the expected magnitude of the results.
2. Insufficient range checking: Failing to properly check the range of values during computations can escalate the possibility of overflow. This can be mitigated by implementing appropriate checks and validation in the code.
3. Accumulation of rounding errors: In floating-point arithmetic, repeated operations involving numbers with limited precision can accumulate rounding errors, eventually leading to an overflow.
Preventing and detecting overflows:
1. Use appropriate data types: To prevent overflows, it is crucial to choose data types that can represent the range of values involved accurately. For example, if you know that the result of an operation will always be positive, using an unsigned integer can eliminate the possibility of an overflow caused by negative values.
2. Range checking and validation: Implement proper range checking in your code by verifying that the operands and results of arithmetic operations fall within the acceptable range of values. This can help identify and handle potential overflows before they cause unexpected behavior.
3. Error handling: When an overflow is detected, it is essential to implement appropriate error handling mechanisms. This may include terminating the program or notifying the user about the exceptional condition, allowing for appropriate corrective actions to be taken.
Frequently Asked Questions:
Q1. What is the impact of an overflow in programming?
A1. Overflows can lead to unexpected and incorrect results, causing programs to crash, produce incorrect outputs, or even disrupt critical systems. In some cases, they can introduce security vulnerabilities that could be exploited.
Q2. Can all arithmetic operations result in overflow?
A2. No, not all arithmetic operations will result in an overflow. Overflows occur when the result exceeds the maximum or minimum representable value for a given data type.
Q3. Are there any benefits to overflow in programming?
A3. Overflow is generally considered an error or exceptional condition and is not desirable in most cases. However, in specific scenarios where wrapping behavior is expected and handled appropriately, overflow can simplify certain mathematical operations.
Q4. How can I prevent overflow in my code?
A4. Using appropriate data types, implementing range checks, and incorporating proper error handling mechanisms can help prevent and mitigate the risks associated with overflow.
Q5. What steps can be taken to detect and handle overflows?
A5. Implementing range checking, validating inputs and outputs, and using exception handling mechanisms can help detect and handle overflows effectively.
In conclusion, an overflow occurs when the result of an arithmetic operation exceeds the maximum value that can be represented by the data type used. Understanding the different types of overflows, the consequences of overflow, and practicing preventative measures can ensure accurate computation and prevent unexpected behavior in programming.
What Is An Arithmetic Overflow Error?
An arithmetic overflow error is a type of error that occurs in computer programs or systems when performing mathematical operations. It happens when the result of an arithmetic operation is too large to be represented within the numeric data type being used. This error can lead to unexpected and incorrect results, program crashes, or even security vulnerabilities if not handled properly.
Arithmetic overflow errors can occur in various programming languages, including C, C++, Java, Python, and many others. These languages provide different data types with fixed sizes to represent numerical values, such as integers, floating-point numbers, and decimal numbers. Each data type has a limited range of values that it can represent, and when an arithmetic operation exceeds this range, an overflow error is triggered.
To understand how an arithmetic overflow error occurs, consider the example of a 32-bit signed integer, which can represent values from -2,147,483,648 to 2,147,483,647. If a program attempts to add two large numbers that exceed this range, an overflow error will occur. For instance, adding 2,147,483,647 to 10 results in 2,147,483,657, which is outside the valid range for a 32-bit signed integer.
Handling arithmetic overflow errors is crucial to ensure the correctness and reliability of computer programs. There are several strategies to address this issue, depending on the programming language and the specific requirements of the application.
One common approach is to use data types that have a larger range, such as 64-bit integers or floating-point numbers, to avoid arithmetic overflow errors. By utilizing these extended data types, programs can handle larger numbers without encountering overflow errors. However, this approach comes with the trade-off of increased memory consumption and computational overhead.
Another way to handle arithmetic overflow errors is to perform range checks before executing arithmetic operations. By validating the input data and ensuring that it falls within the acceptable range, programs can prevent overflows from occurring. If an overflow is detected, appropriate error handling mechanisms can be implemented, such as terminating the program or providing error messages to the user.
Additionally, some programming languages provide built-in mechanisms to handle arithmetic overflow errors automatically. For example, in Python, the ‘int’ data type automatically switches to ‘long’ if an overflow is detected, allowing operations on arbitrarily large numbers. Similarly, in languages like C# and Java, the ‘checked’ keyword can be used to enable runtime checks for overflow errors.
Frequently Asked Questions (FAQs):
Q: Can arithmetic overflow errors lead to security vulnerabilities?
A: Yes, arithmetic overflow errors can potentially lead to security vulnerabilities. In certain scenarios, attackers can exploit these errors to gain unauthorized access or perform malicious actions. For example, if an application stores financial transactions in a fixed-size data type and an overflow occurs, it may result in incorrect calculations, allowing attackers to manipulate or bypass certain security controls.
Q: How can I prevent arithmetic overflow errors?
A: To prevent arithmetic overflow errors, it is essential to choose appropriate data types with a wider range to accommodate the expected values. Performing range checks before executing operations can also help identify and handle potential overflows. Additionally, utilizing programming languages that offer built-in mechanisms to detect and handle overflow errors can reduce the risk of encountering such errors.
Q: Is it always necessary to handle arithmetic overflow errors?
A: Handling arithmetic overflow errors is crucial for ensuring the correctness and reliability of computer programs. Failing to handle these errors can lead to unexpected results, crashes, or security vulnerabilities. However, in certain cases where the risk of overflow is known to be negligible or the consequences are non-critical, handling every possible overflow scenario may not be necessary.
Q: Are arithmetic overflow errors limited to addition and subtraction operations?
A: No, arithmetic overflow errors can occur with various mathematical operations, including addition, subtraction, multiplication, division, and exponentiation. Any operation that exceeds the range of the data type being used can lead to an overflow error.
Q: Can floating-point numbers encounter arithmetic overflow errors?
A: While floating-point numbers have a larger range compared to integers, they can still encounter arithmetic overflow errors. Floating-point numbers use a scientific representation and have limited precision, which means that very large or very small numbers can be rounded or truncated, leading to loss of accuracy and potential overflow errors.
Keywords searched by users: arithmetic operation resulted in an overflow Arithmetic operation resulted in an overflow C#, Arithmetic operation resulted in an overflow excel, Arithmetic operation resulted in an overflow SQL, Arithmetic operation resulted in an overflow Visual Studio 2019, Arithmetic operation resulted in an overflow c# sum, Arithmetic operation resulted in an overflow c# byte array, Arithmetic operation resulted in an overflow vb net, C# sum overflow
Categories: Top 15 Arithmetic Operation Resulted In An Overflow
See more here: nhanvietluanvan.com
Arithmetic Operation Resulted In An Overflow C#
What is an Overflow in C#?
In C#, variables have a specific range within which they can hold values. This range is dictated by their data type and the number of bits allocated to each variable. When a value is assigned to a variable that exceeds its maximum representable value, an overflow occurs. This means that the variable cannot accommodate the assigned value within its defined range, leading to unpredictable and erroneous results.
The Causes of Overflow
Overflow usually occurs when arithmetic operations, such as addition, subtraction, multiplication, or division, are performed on variables that surpass their maximum value. For instance, if you have an integer variable (int) controlled by 32 bits, its maximum value is 2,147,483,647. Adding 1 to this value results in an overflow, leading the variable to wrap around to the minimum representable value (-2,147,483,648) and continue from there. Similarly, subtracting 1 from the minimum value causes an overflow in the opposite direction, looping back to the maximum value.
Consequences of Overflow
When an overflow occurs, the resulting value can be unexpected and incorrect. This can have severe consequences, as calculations and subsequent decisions made based on faulty data may yield erroneous outputs or lead to program instability. The consequences may range from incorrect results in calculations to program crashes or even security vulnerabilities. Therefore, it is crucial to prevent or handle overflows effectively.
Preventing Overflow in C#
C# provides several mechanisms to prevent overflows when performing arithmetic operations. One of the popular approaches is the use of checked and unchecked keywords. By default, C# performs arithmetic operations unchecked, allowing overflows to occur without throwing any exceptions. However, by explicitly using the checked keyword, you enable overflow checking, which triggers an exception when an overflow occurs. This way, unchecked operations can be selectively marked for checking, reducing the likelihood of unnoticed overflows.
Another technique to prevent overflow is to use data types that can accommodate larger values than the default ones. C# offers data types like long, allowing larger numbers to be stored and calculated without experiencing overflow issues. By selecting an appropriate data type based on the expected range and precision of your variables, you can mitigate the risks of overflow.
Handling Overflow Exceptions
When an overflow exception is detected, it can be handled gracefully to prevent program termination or incorrect results. C# offers the try-catch statement for handling exceptions, which allows you to catch overflow exceptions specifically and execute alternative code paths. By employing try-catch blocks around arithmetic operations that may encounter an overflow, you can control the flow of your program and handle exceptions in a desired manner. For instance, you can decide to cancel the current calculation, notify the user, or perform alternative calculations instead.
Frequently Asked Questions (FAQs):
Q1: What happens if I don’t handle an overflow in C#?
If an overflow is left unhandled, the program will continue to execute with incorrect values. This can lead to erroneous results, program crashes, or even security vulnerabilities.
Q2: Are overflow exceptions costly in terms of performance?
Overflow exceptions can have a slight impact on performance due to the additional checking involved. However, the cost is usually negligible unless you are performing extensive arithmetic operations within performance-critical sections of your code.
Q3: Can I enable overflow checking globally in C#?
Yes, you can enable overflow checking globally by using the /checked compiler option. This instructs the compiler to perform overflow checking in all arithmetic operations within the corresponding code block.
Q4: Are there any alternative ways to handle overflow exceptions in C#?
Aside from using try-catch blocks, you can also utilize the OnOverflowContext method to define custom handling for overflow exceptions. This method allows you to specify custom actions or delegates to control the behavior in case of an overflow.
Q5: Are there any tools or libraries available to detect and prevent overflows automatically?
Some static analysis tools, like ReSharper, can help detect potential overflow issues during the development process. Additionally, libraries like “Math.NET Numerics” provide enhanced numerical types and operations, which can handle overflows more efficiently.
In conclusion, arithmetic operations resulting in an overflow can cause severe issues in C# programs. By understanding the causes and consequences of overflow and utilizing preventive measures, such as checked operations and appropriate data types, developers can minimize the risks associated with overflows. Furthermore, by handling overflow exceptions effectively using try-catch blocks, programmers can ensure graceful error recovery and maintain the stability and correctness of their programs.
Arithmetic Operation Resulted In An Overflow Excel
Arithmetic operations are a fundamental part of working with numbers in Excel. However, there are instances where these operations can result in an overflow. In this article, we will explore what an overflow is, why it occurs in Excel, and how to handle it effectively. We will also provide answers to commonly asked questions regarding this topic.
What is an Overflow?
In the context of Excel, an overflow refers to a mathematical operation that produces a result that is too large to be accurately represented within the limitations of the computer’s memory. When this occurs, Excel is unable to display the exact value and instead shows an error message, such as “#VALUE!”, “#NUM!”, or “#DIV/0!”. This is an indication that the result exceeds the maximum value that Excel can handle.
Why Does an Overflow Occur in Excel?
An overflow occurs in Excel when a numeric calculation exceeds the available bit space allocated to represent the numbers. In Excel, numbers are stored using a finite number of bits, which limits the range of values that can be accurately represented. The maximum value that Excel can handle depends on the data type used to store the number.
For example, if you attempt to multiply two large numbers together using the default data type, Excel may not have enough bits to store the result accurately, resulting in an overflow. Similarly, dividing a number by a very small value can also lead to an overflow, as Excel cannot accurately represent the result.
Preventing Overflows in Excel
To prevent overflows in Excel, it is crucial to be aware of the data type limitations and adjust accordingly. Excel provides different data types such as Integer, Long, Single, and Double, with varying ranges of values they can accurately represent. Choosing the appropriate data type for your calculations can help avoid overflow errors.
Here are a few tips to prevent overflows:
1. Use the appropriate data type: Make sure to choose a data type that can handle the range of values you expect to encounter in your calculations. For example, use the Long data type instead of Integer if you need to work with larger numbers.
2. Split complex calculations: If you have complex calculations involving large numbers, consider breaking them down into smaller, more manageable parts. By doing this, you can prevent intermediate results from exceeding the available memory space.
3. Use error handling functions: Excel provides several error handling functions that can help you manage overflow errors effectively. Functions like IFERROR, ISERROR, and ISNUMBER can be used to identify and handle overflow errors in your formulas.
4. Consider using scientific notation: In some cases, using scientific notation can help represent very large or very small numbers accurately. Excel supports scientific notation using the “E” or “e” character. For example, 1.23456E+10 represents 123,456,000,000.
Frequently Asked Questions (FAQs)
Q1. How can I identify if an overflow has occurred in Excel?
A1. When an overflow occurs, Excel displays an error message such as “#VALUE!”, “#NUM!”, or “#DIV/0!”. You can also use error handling functions like ISERROR or ISNUMBER to check for overflow errors in your formulas.
Q2. Can I change the default data type in Excel to avoid overflows?
A2. No, the default data types in Excel are predefined and cannot be changed. However, you can specify the data type explicitly when declaring variables or use appropriate functions to convert data types if needed.
Q3. What are the consequences of ignoring overflow errors in Excel?
A3. Ignoring overflow errors can lead to incorrect calculations, misleading results, and data loss. It is essential to address overflow errors appropriately to ensure accurate and reliable results.
Q4. Are there any performance implications of handling overflow errors in Excel?
A4. While handling overflow errors in Excel can impact performance slightly due to additional calculations, the benefits of accurate results far outweigh the minimal performance impact.
In conclusion, arithmetic operations in Excel can result in an overflow when the calculated result exceeds the available memory space. To avoid overflows, it is important to select the appropriate data type, split complex calculations, use error handling functions, and consider using scientific notation. By keeping these considerations in mind, you can effectively manage and prevent overflow errors in Excel, ensuring accurate results in your calculations.
Images related to the topic arithmetic operation resulted in an overflow
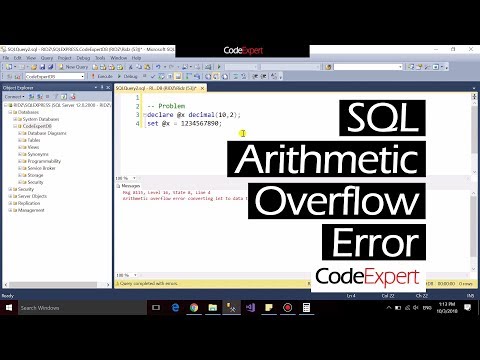
Found 40 images related to arithmetic operation resulted in an overflow theme


![Solved] - Arithmetic operation resulted in an overflow Solved] - Arithmetic Operation Resulted In An Overflow](https://1.bp.blogspot.com/-xb2SN44uSVs/XkYe7KzrZBI/AAAAAAAAD2M/xyC3e499HagY-oy2i-TYIKG00mG1mhVDwCLcBGAsYHQ/s1600/Arithmetic%2BError.png)





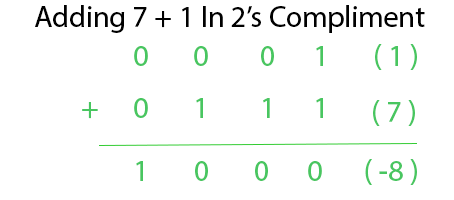
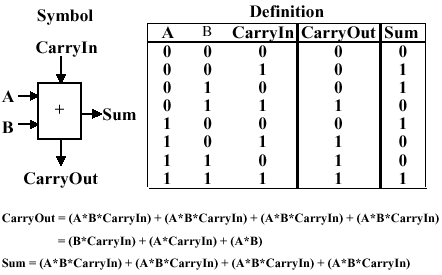

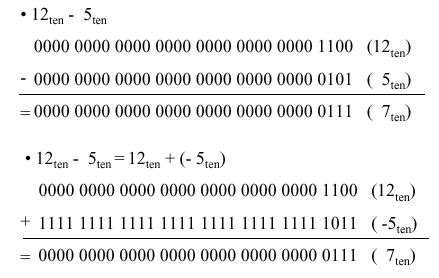
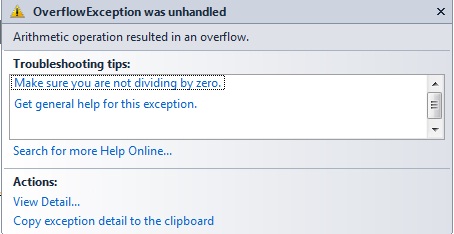
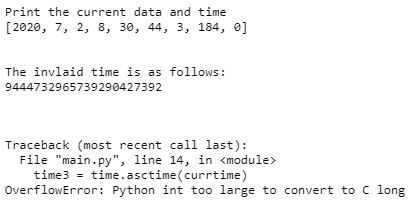


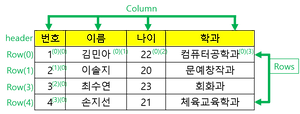


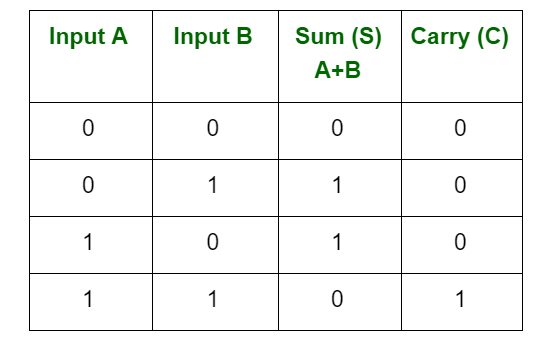


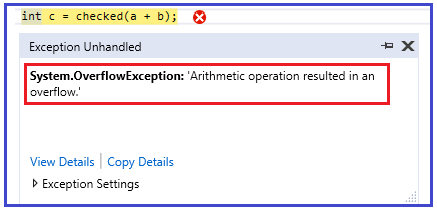
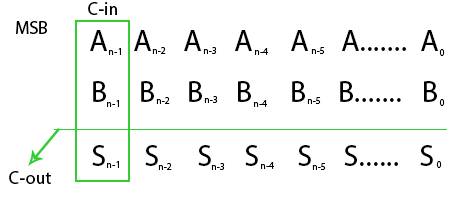
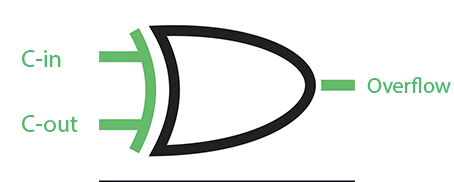



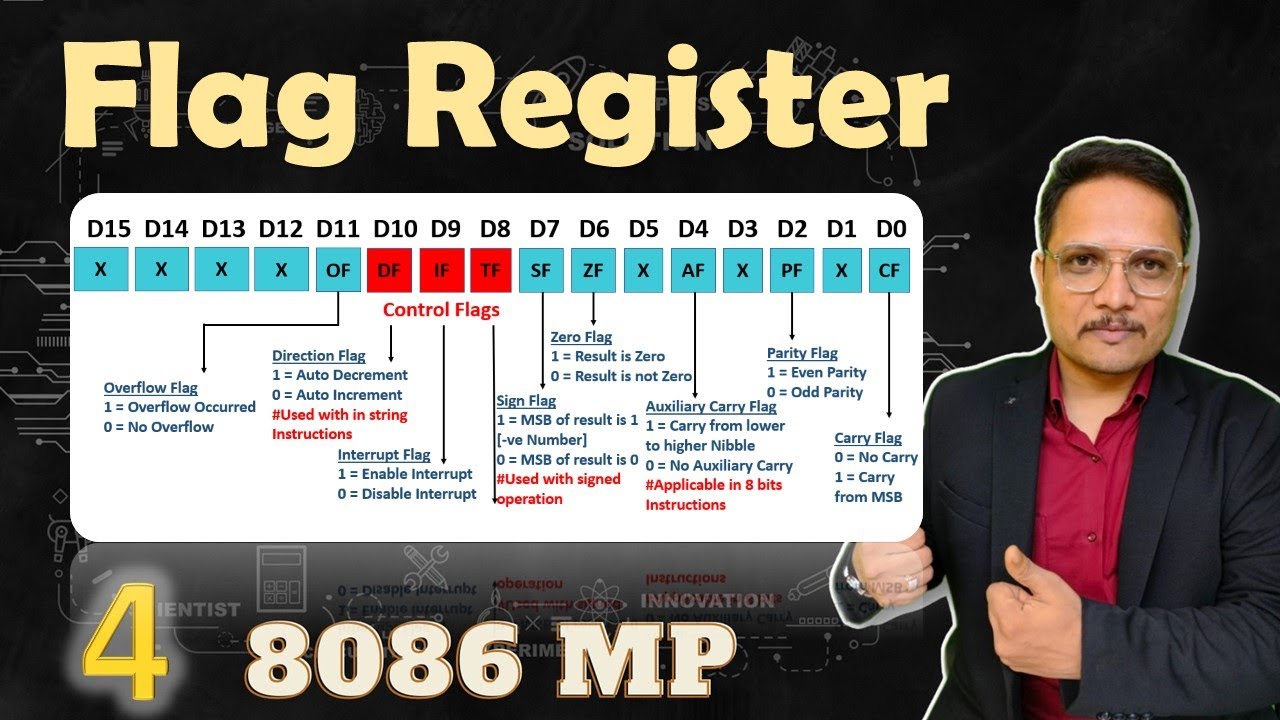
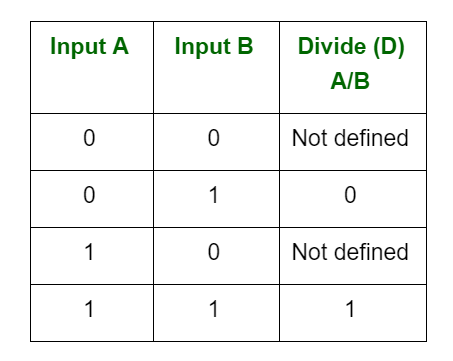
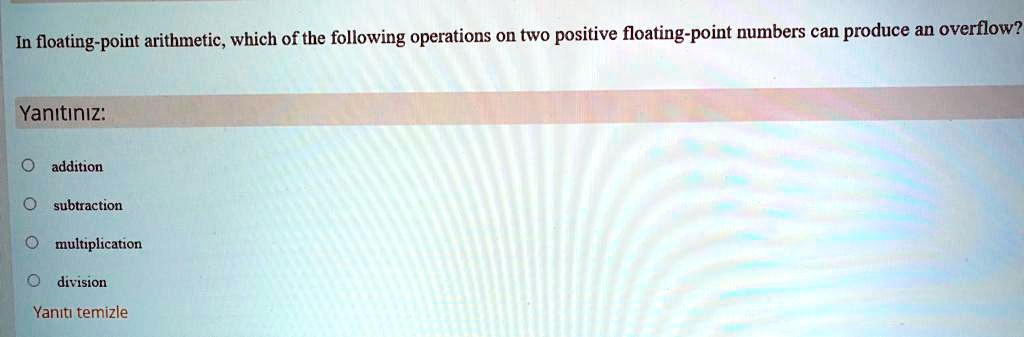

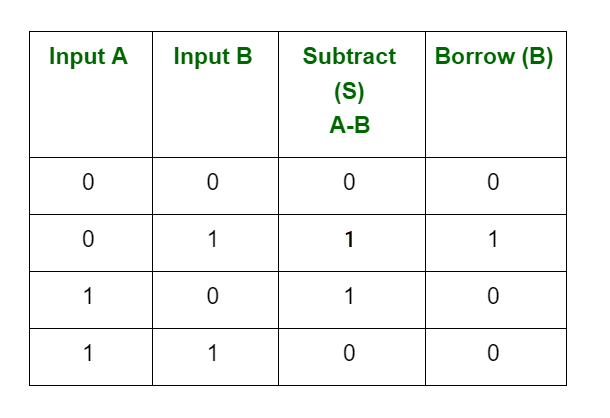
Article link: arithmetic operation resulted in an overflow.
Learn more about the topic arithmetic operation resulted in an overflow.
- Arithmetic operation resulted in an overflow. (Adding integers)
- Arithmetic overflow – C# Professional – Numbers – CodinGame
- Arithmetic Overflow Error T-SQL – how to debug – Microsoft Q&A
- Arithmetic underflow – Wikipedia
- Organization of Computer Systems: Computer Arithmetic – UF CISE
- Troubleshooting ** “Arithmetic operation resulted in an … – IBM
- Arithmetic operation resulted in an overflow – Help
- How to fix: “OverflowException occurred Arithmetic operation …
- Understanding Arithmetic Overflow And How Checked And …
- Database returned the error like Arithmetic Operation resulted …
- Arithmetic operation resulted in an overflow. : RIDER-72381
- Crash when zooming: System.OverflowException: Arithmetic …
See more: blog https://nhanvietluanvan.com/luat-hoc