Arduino Int To String
Arduino is a popular microcontroller platform that is widely used in electronic projects and prototyping. One common task in Arduino programming is converting an integer (int) value to a string. This article will provide a comprehensive guide on various methods to accomplish this task, as well as troubleshooting common issues that may arise during the conversion process.
Declaring variables and setting up libraries
Before we delve into different methods for converting an int to a string in Arduino, it is crucial to set up the necessary libraries and declare the required variables. The libraries that will be used for integer and string conversions are
Using the built-in helper functions
Arduino provides built-in helper functions that can be used to convert an int to a string. Two such functions are itoa() and dtostrf().
The itoa() function allows you to convert an integer to a string representation. Its syntax is as follows:
“`c++
itoa(integer_value, char_array, base);
“`
The `integer_value` is the integer that you want to convert, `char_array` is the character array that will store the resulting string, and `base` is the numerical base for the conversion.
The dtostrf() function, on the other hand, is specifically designed to convert floating-point numbers to string representations. However, it can also be used to convert an integer to a string by omitting the decimal portion. Its syntax is as follows:
“`c++
dtostrf(float_value, width, precision, char_array);
“`
The `float_value` is the floating-point value that you want to convert, `width` and `precision` specify the formatting of the resulting string, and `char_array` is the character array that will store the string.
Constructing a custom conversion function
If you prefer to have more control over the conversion process, you can create a custom function to convert an int to a string. Below is an example of a custom function that handles negative numbers and zero values:
“`c++
void intToString(int value, char* result) {
int i = 0;
bool isNegative = false;
if (value < 0) {
isNegative = true;
value = -value;
}
while (value > 0) {
result[i++] = value % 10 + ‘0’;
value /= 10;
}
if (isNegative) {
result[i++] = ‘-‘;
}
result[i] = ‘\0’;
// Reverse the string
int length = strlen(result);
for (int j = 0; j < length / 2; j++) {
char temp = result[j];
result[j] = result[length - 1 - j];
result[length - 1 - j] = temp;
}
}
```
Using the String class methods
Arduino also provides a String class that offers various methods for manipulating and converting strings. Two methods of interest when converting an int to a string are toString() and concat().
The toString() method can be called on an Integer object to convert it to a string representation. Here's an example:
```c++
int value = 42;
String str = String(value).toString();
```
The concat() method, on the other hand, allows you to concatenate an integer with an existing string. Here's an example:
```c++
int value = 42;
String str = "The answer is: ";
str.concat(value);
```
Applying the sprintf() function
The sprintf() function is a standard C library function that allows you to format a string by inserting values into placeholders. It can also be used to convert an integer to a string. Here's an example of how to use sprintf() in Arduino:
```c++
int value = 42;
char str[10];
sprintf(str, "%d", value);
```
In this example, the `%d` placeholder is used to indicate the position where the integer value should be inserted.
Converting int to string with the String constructor
Another approach to convert an integer to a string in Arduino is by utilizing the String constructor. The constructor allows you to create a String object by passing an integer as an argument. Here's an example:
```c++
int value = 42;
String str(value);
```
The String constructor also supports converting integers of different bases to strings. For example, to convert an integer to a hexadecimal string, you can pass `HEX` as the second argument:
```c++
int value = 42;
String str(value, HEX);
```
Handling memory allocation and optimization
When converting an int to a string, it is essential to manage memory efficiently, especially on memory-constrained Arduino boards. Avoiding unnecessary memory consumption can be achieved by using methods that don't involve string concatenation, such as itoa() or custom conversion functions. Additionally, be cautious when using the String class, as it may lead to memory fragmentation and unnecessary memory allocation if used improperly.
Troubleshooting common issues
During the int to string conversion process, several common issues may arise. One issue is overflow errors, which typically occur when the integer value is too large to be represented as a string. To avoid this, ensure that the character array used to store the string has enough memory to accommodate the resulting string. Another issue is unexpected output or incorrect string conversions, which can occur if the conversion method or formatting is incorrect. Double-check your syntax and formatting to troubleshoot such issues.
Examples and practical applications
Now that you have a comprehensive understanding of how to convert an int to a string in Arduino, let's explore some examples and practical applications.
Example 1: Arduino String Concatenation
```c++
int value1 = 42;
int value2 = 23;
String str = String(value1) + String(value2);
```
In this example, the values 42 and 23 are concatenated into a single string using the '+' operator.
Example 2: Arduino String Array
```c++
int values[] = {42, 23, 17};
String str;
for (int i = 0; i < 3; i++) {
str += String(values[i]) + " ";
}
```
In this example, an array of integers is converted to a string and concatenated with a space character.
These examples highlight how int to string conversions can be used in practical scenarios, such as displaying sensor data or constructing messages for communication protocols.
Conclusion
Converting an int to a string is a common task in Arduino programming. This article has provided a comprehensive guide on different methods to accomplish this task, including using built-in helper functions, constructing custom conversion functions, utilizing the String class methods, applying the sprintf() function, and using the String constructor. Additionally, we discussed troubleshooting common issues that may arise during the conversion process. By mastering int to string conversion techniques, you can enhance your Arduino projects and effectively handle various real-world scenarios.
Dtostrf() With Arduino – Convert Float To String
How To Convert Int To String On Arduino?
Working with numbers is a common task in Arduino programming. However, there may be instances when you need to convert an integer (int) to a string for different purposes such as displaying values on an LCD screen, sending data over a serial connection, or even storing them in a file. In this article, we will explore various methods for converting int to string on Arduino and discuss their pros and cons.
Method 1: Using the String Class
Arduino provides a built-in class called String, which makes string manipulation quite straightforward. To convert an int to a string using this method, you can use the String() constructor or the toString() method. Let’s take a look at both options:
1. Using the String() Constructor:
“`cpp
int number = 1234;
String strNumber = String(number);
“`
2. Using the toString() method:
“`cpp
int number = 1234;
String strNumber = String();
strNumber = number.toString();
“`
These methods are quick and easy to use, especially if you’re already familiar with the String class. However, keep in mind that excessive string operations can lead to memory fragmentation, so if you’re working on a memory-constrained Arduino board, it’s advisable to avoid using the String class extensively.
Method 2: Using snprintf()
The snprintf() function is a handy tool for converting numeric values to strings. It allows you to format the output string and control the number of characters used for the conversion. Here’s an example of using snprintf() to convert an int to a string:
“`cpp
int number = 1234;
char strNumber[6];
snprintf(strNumber, sizeof(strNumber), “%d”, number);
“`
By specifying “%d” as the format specifier, snprintf() converts the integer to a string and stores it in the character array strNumber. Keep in mind that you should allocate enough space in the array to hold the converted value. In this case, we allocate 6 bytes to accommodate the number and the null terminator.
Method 3: Using itoa()
The itoa() function is specifically designed for converting integers to strings. However, it’s important to note that itoa() is not standardized and may not be available on all Arduino boards. If you’re using a board that doesn’t support itoa(), you can use the standard library function sprintf() as an alternative. Here’s an example of how to use itoa():
“`cpp
int number = 1234;
char strNumber[6];
itoa(number, strNumber, 10);
“`
The itoa() function takes three arguments: the integer to convert, the character array to store the result, and the base of the number system (in this example, the decimal system is used).
FAQs:
Q: Why should I convert an int to a string on Arduino?
A: Converting an int to a string is useful for various purposes, such as displaying values on an LCD screen, transmitting data over a serial connection, or storing values in a file.
Q: Can I convert a negative int to a string using these methods?
A: Yes, all the methods mentioned above work for both positive and negative integers.
Q: Which method should I use for converting an int to a string?
A: The choice of method depends on your specific application. If you’re already using the String class extensively, you can stick to the first method. Otherwise, snprintf() or itoa() can be good alternatives, depending on the supported functions on your Arduino board.
Q: Are there any limitations when using the String class for conversions?
A: Yes, excessive string operations using the String class can cause memory fragmentation, especially on memory-constrained Arduino boards.
Q: Can I convert float or double values to a string using these methods?
A: No, the methods discussed in this article are specifically for converting integers (int) to strings. To convert float or double values, you can use the dtostrf() function or other external libraries like ArduinoJson or sprintf().
In conclusion, converting an int to a string on Arduino is a frequent requirement in many projects. By understanding the various methods available, you can choose the most suitable approach for your application. Whether it’s using the built-in String class, snprintf(), or itoa(), each method has its own advantages and considerations.
How Can I Convert Int To String?
In the world of programming, it is common to encounter scenarios where a programmer needs to convert an integer (int) into a string data type. This conversion can be necessary for a variety of reasons, such as displaying numerical values as strings or concatenating them with other strings. Fortunately, most programming languages provide built-in functions or methods to facilitate this conversion process seamlessly. In this article, we will explore different approaches to converting int to string in various popular programming languages.
1. Converting int to string in Python:
Python, a widely-used and beginner-friendly programming language, provides a simple method called `str()` to convert an integer to a string. Here is an example:
“`python
num = 42
str_num = str(num)
print(type(str_num), str_num)
“`
The output will be:
“`
“`
2. Converting int to string in Java:
Java, another popular programming language, offers a method called `Integer.toString()` or using the `String.valueOf()` conversion function. Both methods will accomplish the task effectively. Here are examples demonstrating both approaches:
Using `Integer.toString()`:
“`java
int num = 42;
String strNum = Integer.toString(num);
System.out.println(strNum);
“`
Using `String.valueOf()`:
“`java
int num = 42;
String strNum = String.valueOf(num);
System.out.println(strNum);
“`
Both approaches will produce the same output:
“`
42
“`
3. Converting int to string in C++:
C++ provides several ways to convert an int to a string. One popular approach is to use the `std::to_string()` function. Here is an example:
“`cpp
#include
#include
int main() {
int num = 42;
std::string strNum = std::to_string(num);
std::cout << strNum << std::endl;
return 0;
}
```
The output will be:
```
42
```
4. Converting int to string in JavaScript:
JavaScript, the language of the web, offers multiple ways to convert an int to a string. One of the simplest methods is to concatenate the integer value with an empty string. Here is an example:
```javascript
let num = 42;
let strNum = num + "";
console.log(typeof strNum, strNum);
```
The output will be:
```
string 42
```
Another approach is to use the `toString()` method provided by JavaScript's Number class:
```javascript
let num = 42;
let strNum = num.toString();
console.log(typeof strNum, strNum);
```
The output will be the same as the previous example:
```
string 42
```
FAQs:
Q: Can I convert negative integers to strings using these methods?
A: Absolutely! All the methods mentioned in this article support converting both positive and negative integers to strings effectively.
Q: Can I convert floating-point numbers to strings using the same methods?
A: No, these methods are designed to convert integers to strings. For floating-point numbers, most programming languages offer different functions or methods, such as `std::to_string()` in C++ or `float.parseFloat()` in Java, to achieve the same conversion.
Q: Will the output string preserve leading zeros, if present in the integer value?
A: Yes, the conversion methods demonstrated will preserve any leading zeros present in the input integer value.
Q: Are there any performance considerations when converting int to string?
A: The performance impact of converting an int to a string is generally negligible. However, it's advisable to analyze the specific programming language and implementation details for potential performance pitfalls when handling large numbers or performing bulk conversions.
In conclusion, converting an int to a string is a fundamental operation in programming. This article explored how to accomplish this task in Python, Java, C++, and JavaScript using various built-in functions and methods. By following the approaches outlined here, you can confidently convert int to string and fulfill your programming requirements efficiently.
Keywords searched by users: arduino int to string arduino string concatenation, arduino string variable, arduino string example, arduino string format, arduino string functions, arduino string array, arduino string to char, Int to string Arduino
Categories: Top 78 Arduino Int To String
See more here: nhanvietluanvan.com
Arduino String Concatenation
Introduction:
String concatenation is a fundamental operation in programming that allows you to combine or join multiple strings into one. In the context of Arduino programming, understanding how to concatenate strings is crucial for manipulating and organizing data effectively. In this article, we will delve into the intricacies of Arduino string concatenation, exploring the syntax, approaches, and best practices to master this essential skill.
I. Understanding String Concatenation in Arduino:
String concatenation in Arduino involves merging two or more strings together to form a single string. This operation is particularly useful when building dynamic messages, displaying data, or constructing larger strings from smaller components. In Arduino, strings are represented by the String class, which provides various methods for manipulating and concatenating strings effortlessly.
II. Concatenating Strings with the “+” Operator:
The simplest way to concatenate strings in Arduino is by using the “+” operator. This operator allows you to combine two or more strings into one.
Example 1:
“`
String greeting = “Hello, “;
String name = “Arduino”;
String message = greeting + name;
Serial.println(message);
“`
Output:
“`
Hello, Arduino
“`
In Example 1, we created three strings: “greeting,” “name,” and “message.” By using the “+” operator, we concatenated the strings “greeting” and “name” into “message.” Then, we printed the concatenated message to the Serial Monitor.
III. Concatenating Strings using the concat() Method:
The Arduino String class also provides the `concat()` method for concatenating strings. This method allows you to concatenate strings directly without relying on the “+” operator.
Example 2:
“`
String result;
result.concat(“The answer is: “);
result.concat(42);
Serial.println(result);
“`
Output:
“`
The answer is: 42
“`
In Example 2, we initialized an empty string called “result.” We then used the `concat()` method to concatenate the string “The answer is: ” and the integer 42. Finally, we printed the result to the Serial Monitor.
IV. Performance Considerations:
When concatenating strings, it’s essential to be mindful of memory usage and performance. The String class in Arduino is convenient but can be memory-intensive, leading to potential performance issues when dealing with large-scale applications. To mitigate this, consider the following best practices:
1. Avoid excessive concatenation within loops: Repeatedly concatenating strings within loops can quickly deplete available memory. If possible, concatenate strings outside the loop or use more memory-efficient data types like character arrays (`char`) if performance is a concern.
2. Prefer `concat()` over “+”: While the “+” operator is more concise, it creates temporary objects during concatenation, leading to unnecessary memory usage. Using the `concat()` method is typically more memory-efficient, especially when concatenating multiple strings.
FAQs:
Q1. Can I concatenate an integer or other data types with a string in Arduino?
Yes, Arduino’s String class allows you to concatenate various data types, including integers, floats, characters, and more. However, you might need to convert non-string data types using appropriate functions like `String()` or `String.valueOf()` to ensure successful concatenation.
Q2. Is there a limit to the length of the resultant concatenated string?
Arduino imposes a limit on the maximum length of a String object, typically around 2KB. Attempting to exceed this limit can result in memory allocation errors. If you anticipate creating very long strings, consider using character arrays (`char`) instead, as they offer greater flexibility and less memory consumption.
Q3. Can I concatenate more than two strings together?
Yes, you can concatenate as many strings as needed by chaining the `concat()` method or “+” operators together. This allows you to combine multiple strings into a single string seamlessly.
Conclusion:
String concatenation is a vital skill for Arduino programmers, facilitating the manipulation and organization of data. By exploring the various methods and best practices mentioned above, you can now confidently concatenate strings in your Arduino projects. Remember to consider memory usage and performance optimization to ensure efficient code execution. Happy coding!
Arduino String Variable
Introduction:
The Arduino programming language offers a range of data types to store and manipulate different kinds of information. One commonly used data type is the Arduino String variable. Strings, simply put, are sequences of characters, such as words or sentences. This article aims to provide a comprehensive exploration of the Arduino String variable, including how to declare and manipulate strings, common use cases, and frequently asked questions.
Understanding the Arduino String Variable:
In Arduino, the String variable is a powerful tool for handling textual data. It provides a more flexible and dynamic approach compared to traditional character arrays. The String variable offers numerous functions and methods that simplify operations like concatenation, substring extraction, and comparison. By using the Arduino String variable, programmers can efficiently manage and manipulate textual information within their Arduino sketches.
Declaring an Arduino String:
To declare a String variable in Arduino, simply use the following syntax:
String myString;
This line of code creates a String variable named “myString.” It is important to note that the initial value of an Arduino String variable is an empty string, meaning it contains no characters. However, you can assign a default value during declaration:
String myString = “Hello World!”;
Manipulating Arduino Strings:
The Arduino String variable provides several functions and methods that facilitate common string manipulations. Let’s explore a few key operations:
1. String Concatenation:
To concatenate two strings, use the ‘+’ operator:
String name = “John”;
String greeting = “Hello, ” + name;
The resulting String “greeting” will hold the value “Hello, John.”
2. Substring Extraction:
The substring() method allows you to extract a portion of a String variable based on index positions:
String longString = “The quick brown fox”;
String subString = longString.substring(4, 9);
The variable “subString” will now contain the value “quick.”
3. String Comparison:
The equals() method enables string comparison, allowing you to assess if two String variables are equal:
String password = “Password123”;
String userInput = “password123”;
if (password.equals(userInput)) {
// The passwords match!
}
Common Use Cases for Arduino String Variables:
1. User Input:
Arduino String variables are often used to handle user input. By storing user-generated text values in String variables, developers can process this input to trigger specific actions or manipulate data.
2. Displaying Data:
When working with LCD screens or other output devices, String variables facilitate the flexible display of various sensor readings, program statuses, and other important information.
3. Serial Communication:
The String variable’s versatility makes it ideal for parsing and composing messages in communication applications, including serial communication with other devices or software.
Frequently Asked Questions:
Q1: Can an Arduino String variable store numerical values?
A1: No, the String variable is specifically designed to handle textual information. If you need to store numerical values, consider using the appropriate data type, such as int or float.
Q2: What is the maximum size limit for an Arduino String variable?
A2: The maximum size limit of an Arduino String variable depends on the available memory on your Arduino board. However, it is generally recommended to avoid excessively long strings to prevent memory overflow issues.
Q3: How can I convert an Arduino String variable to a character array?
A3: Use the c_str() method to convert an Arduino String variable to a character array:
String myString = “Hello!”;
char charArray[10];
myString.toCharArray(charArray, 10);
Q4: Can I change the value of an Arduino String variable after declaring it?
A4: Yes, Arduino String variables are mutable, meaning their values can be changed at any time during the program execution. Use the assignment (=) operator to update the value.
Conclusion:
The Arduino String variable is an essential tool for handling textual information efficiently. By providing numerous operations to manipulate strings, it simplifies tasks like concatenation, substring extraction, and comparison. Its versatility makes it useful in various applications such as user input, data display, and communication. Understanding the capabilities of the Arduino String variable allows programmers to harness its power and enhance their Arduino projects.
Images related to the topic arduino int to string
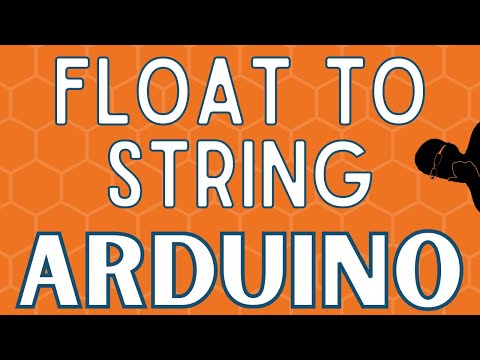
Found 36 images related to arduino int to string theme
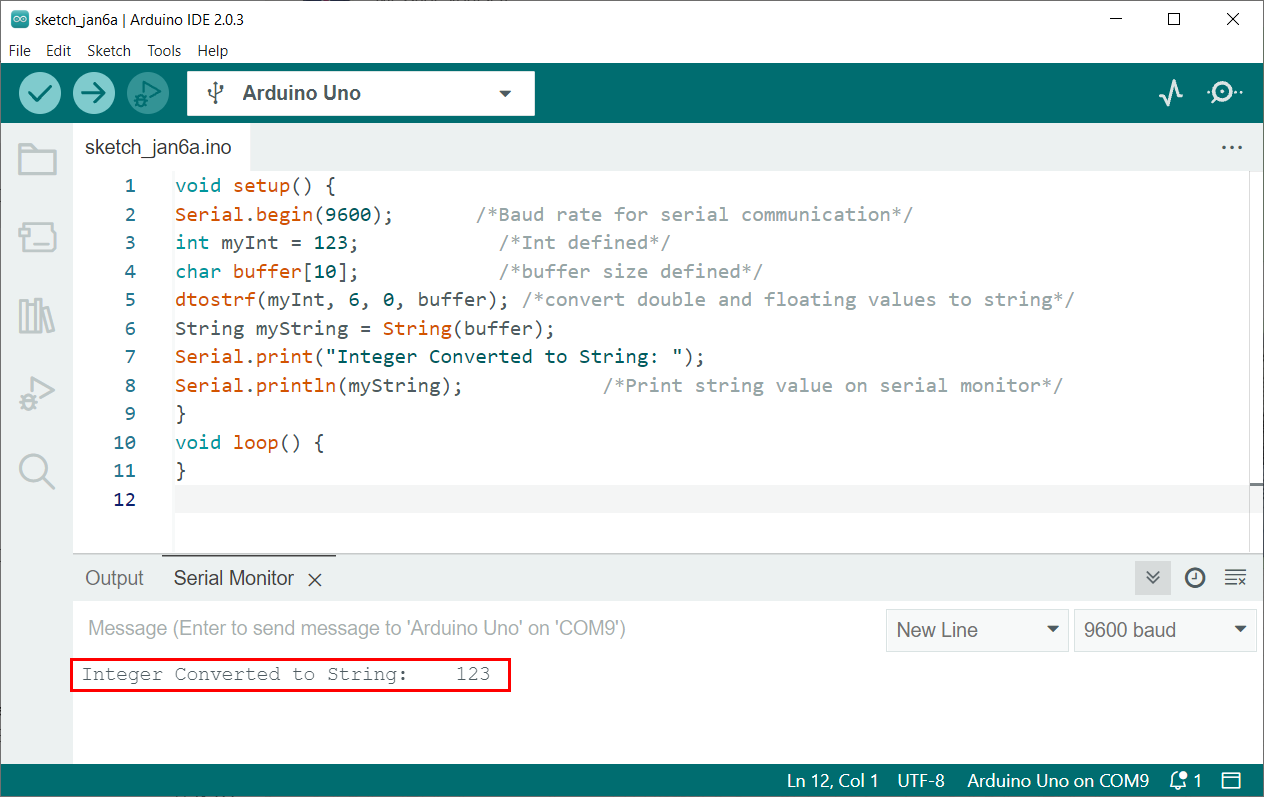
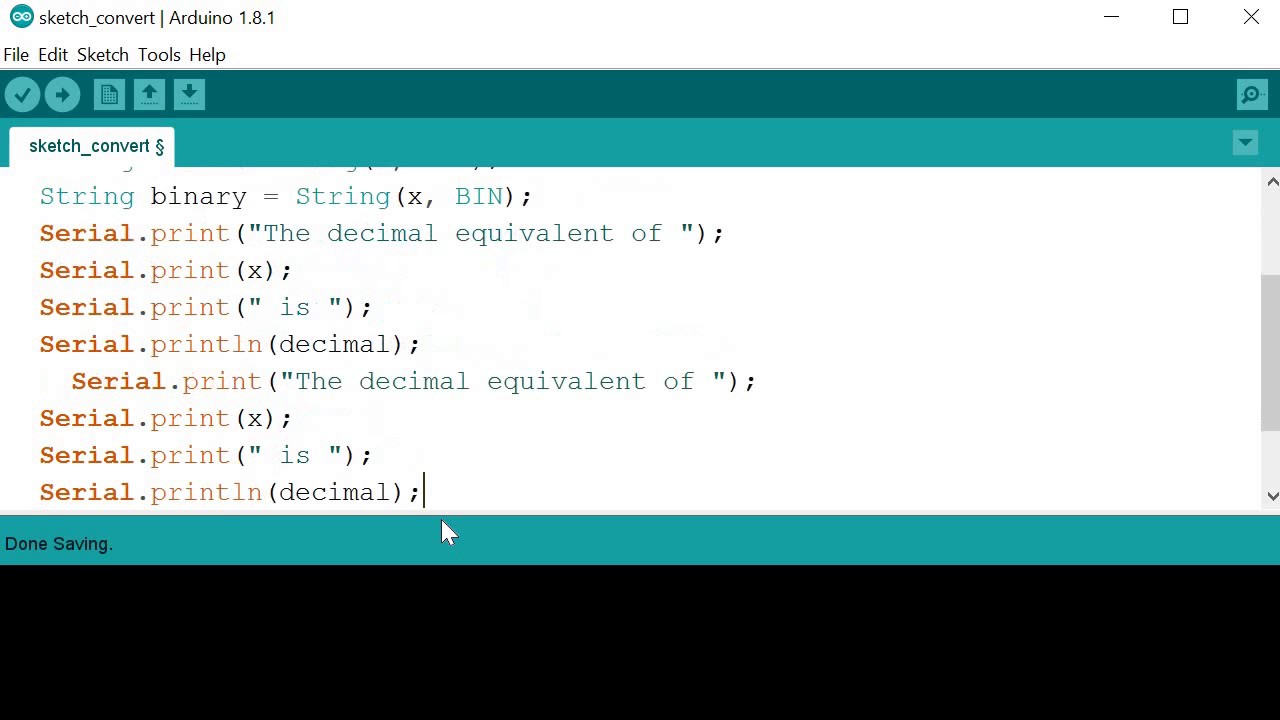

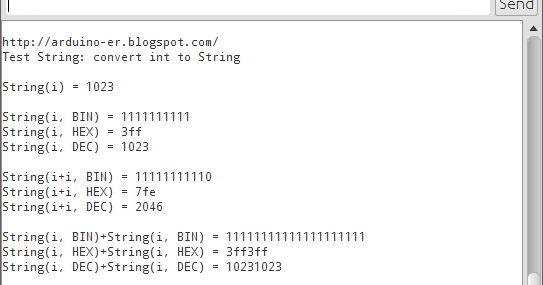


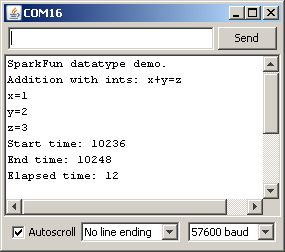
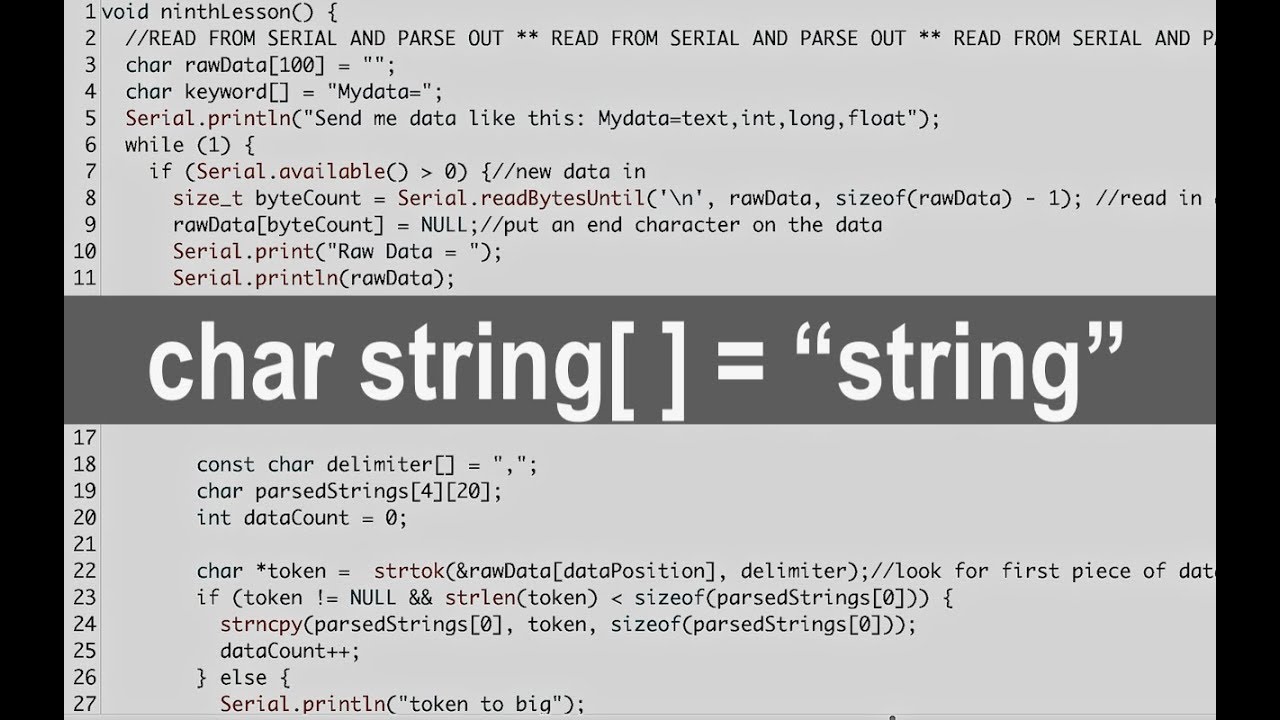



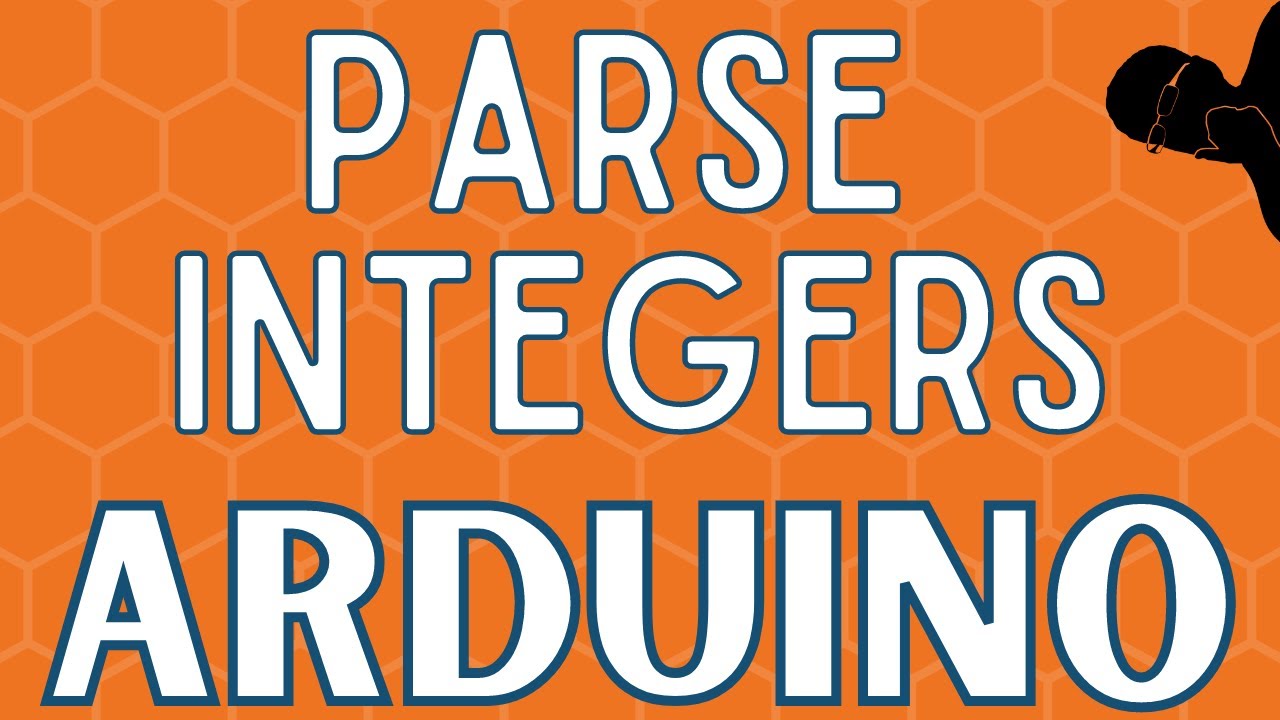

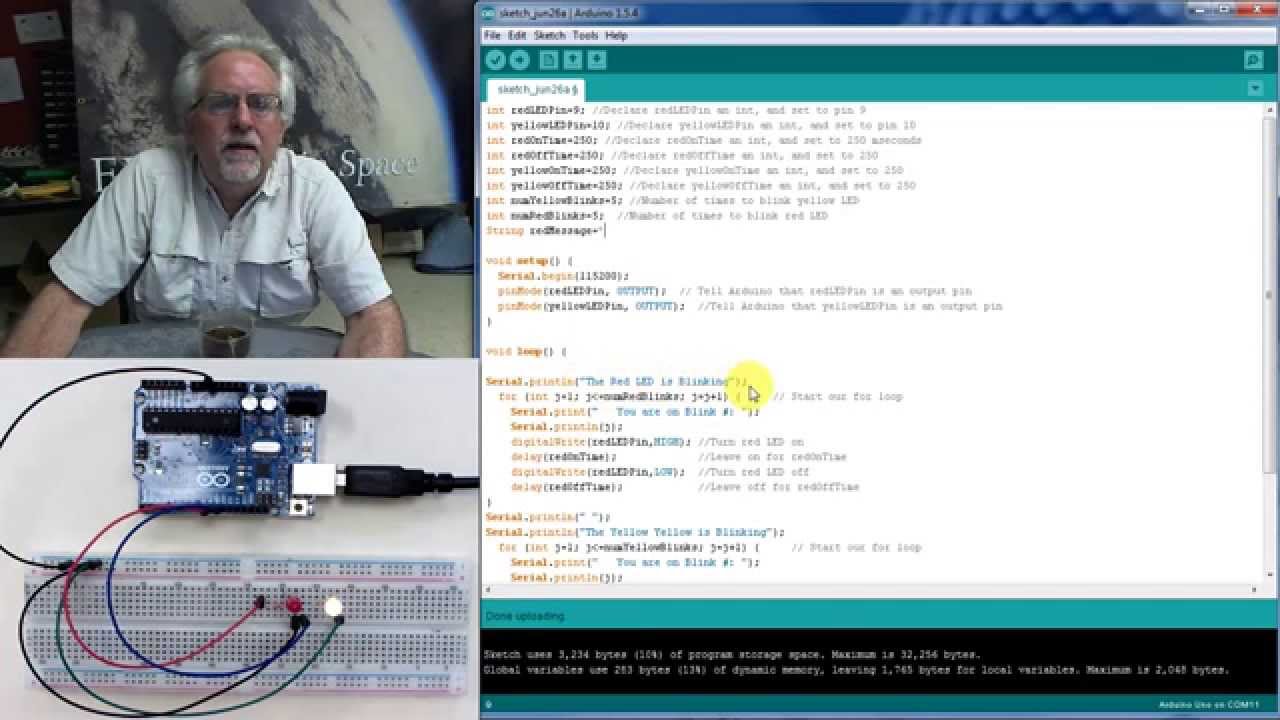


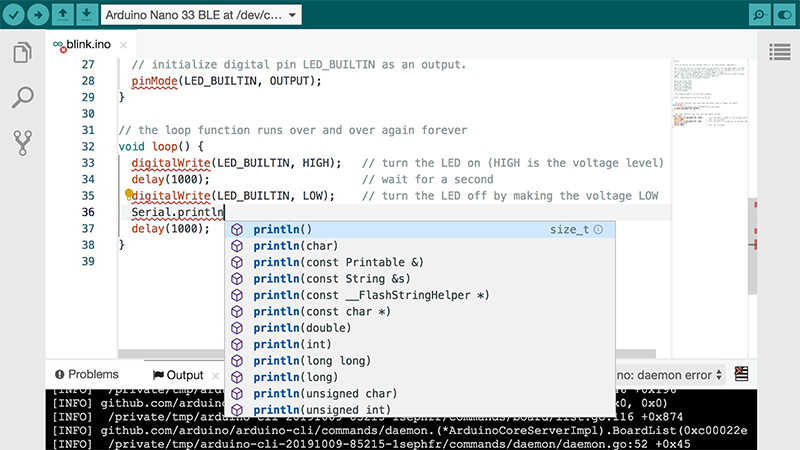

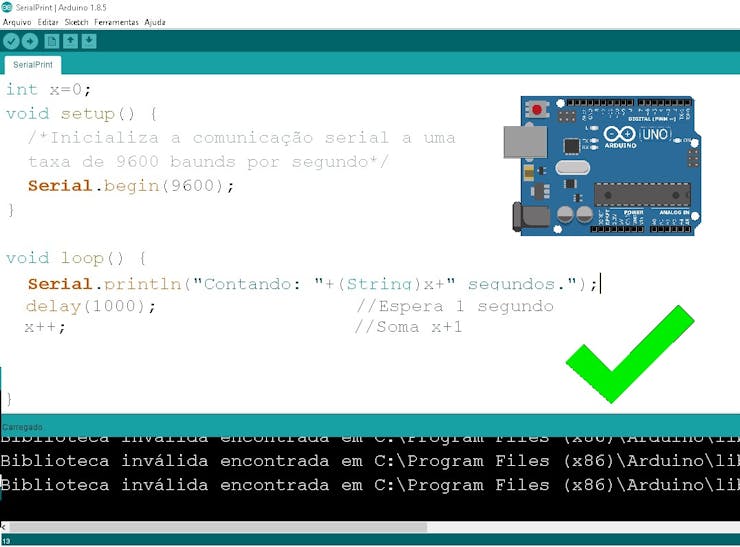
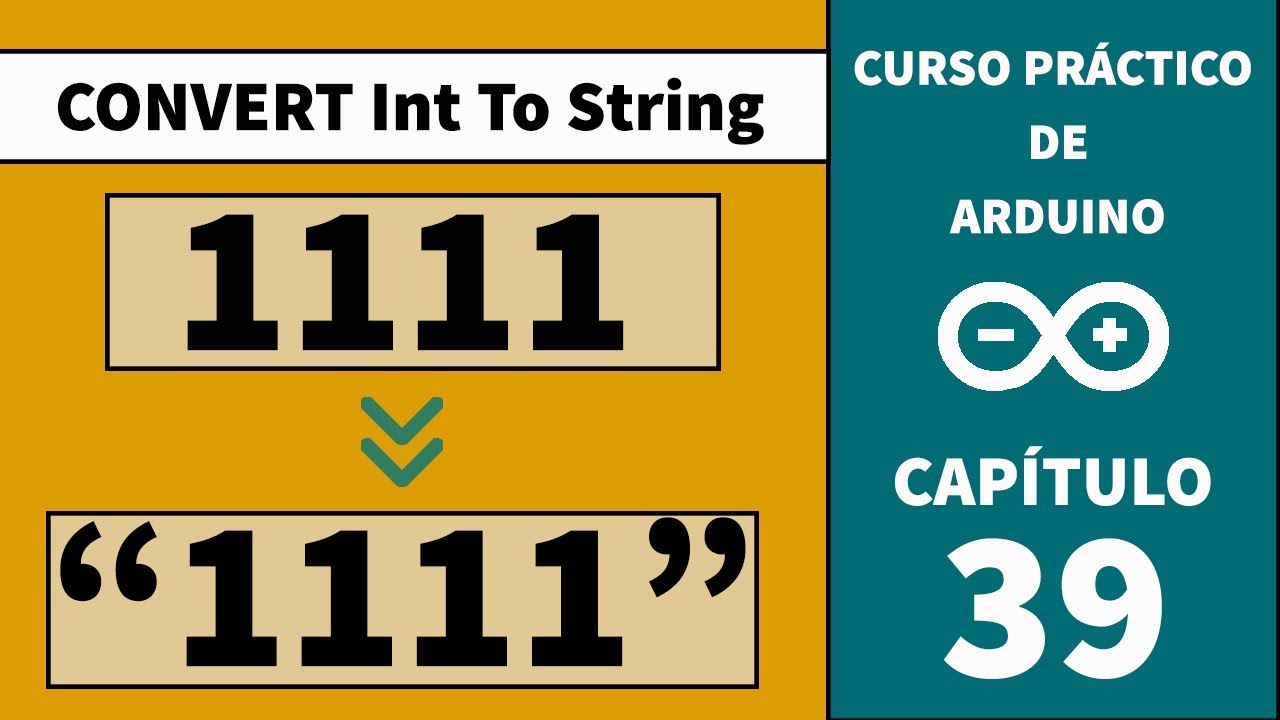





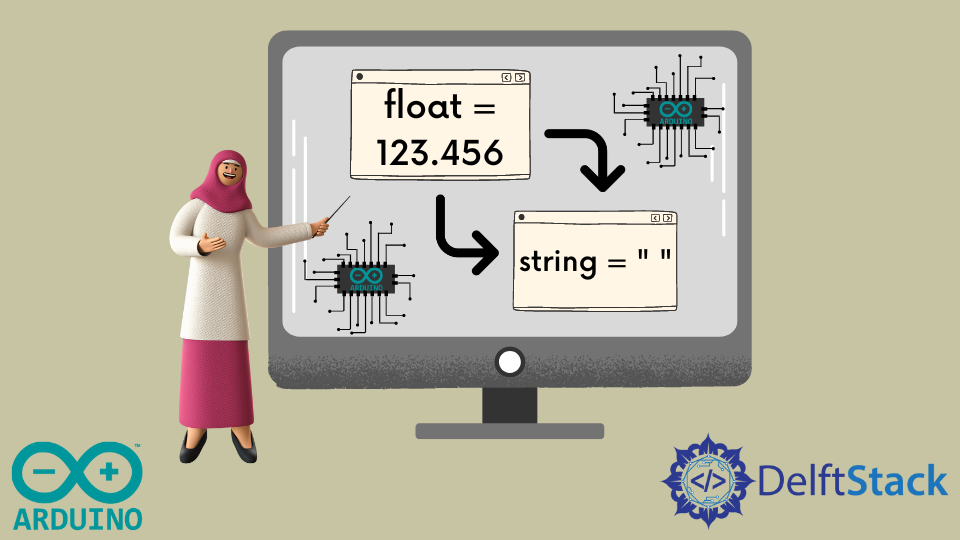
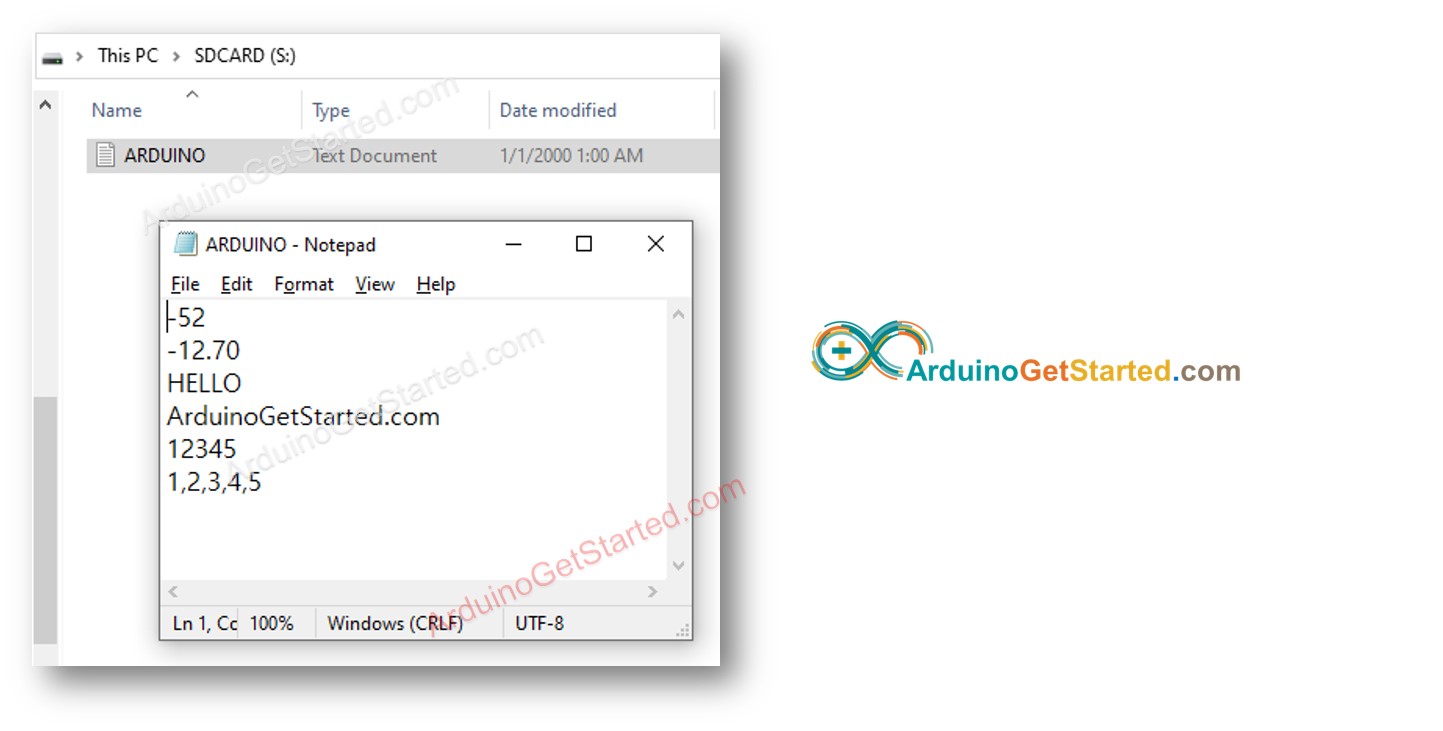
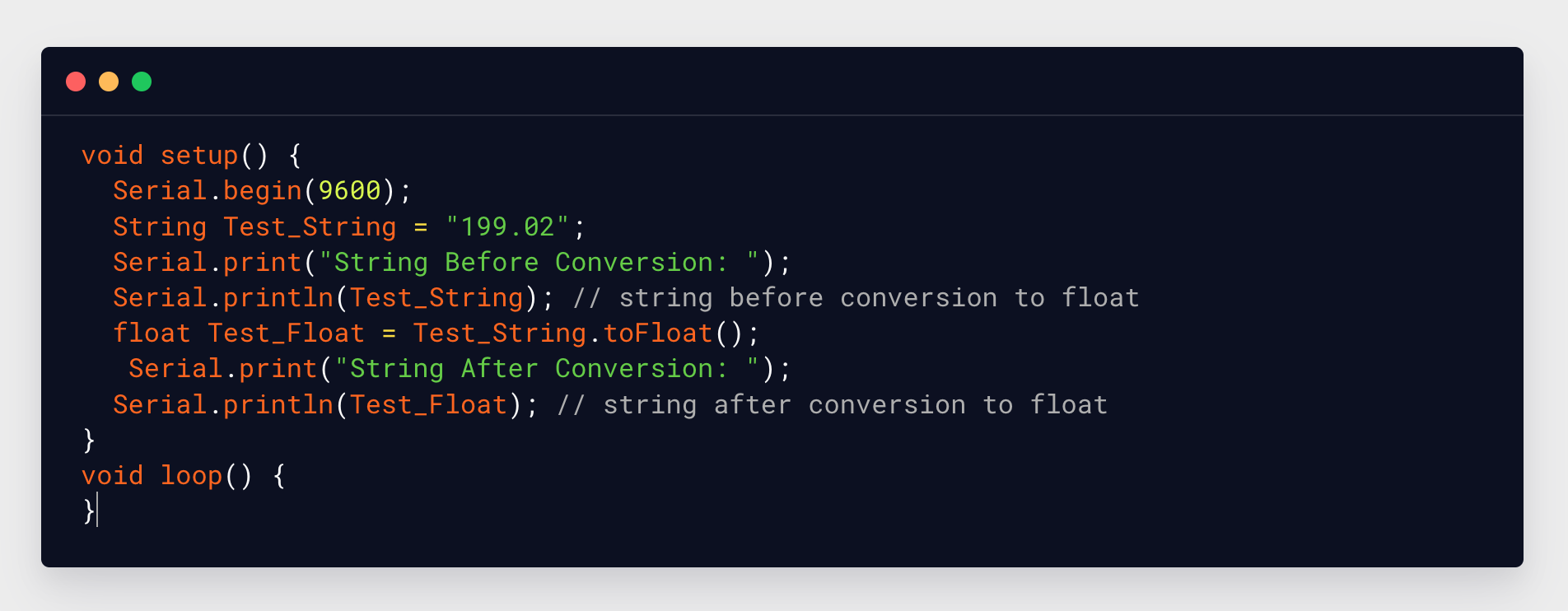
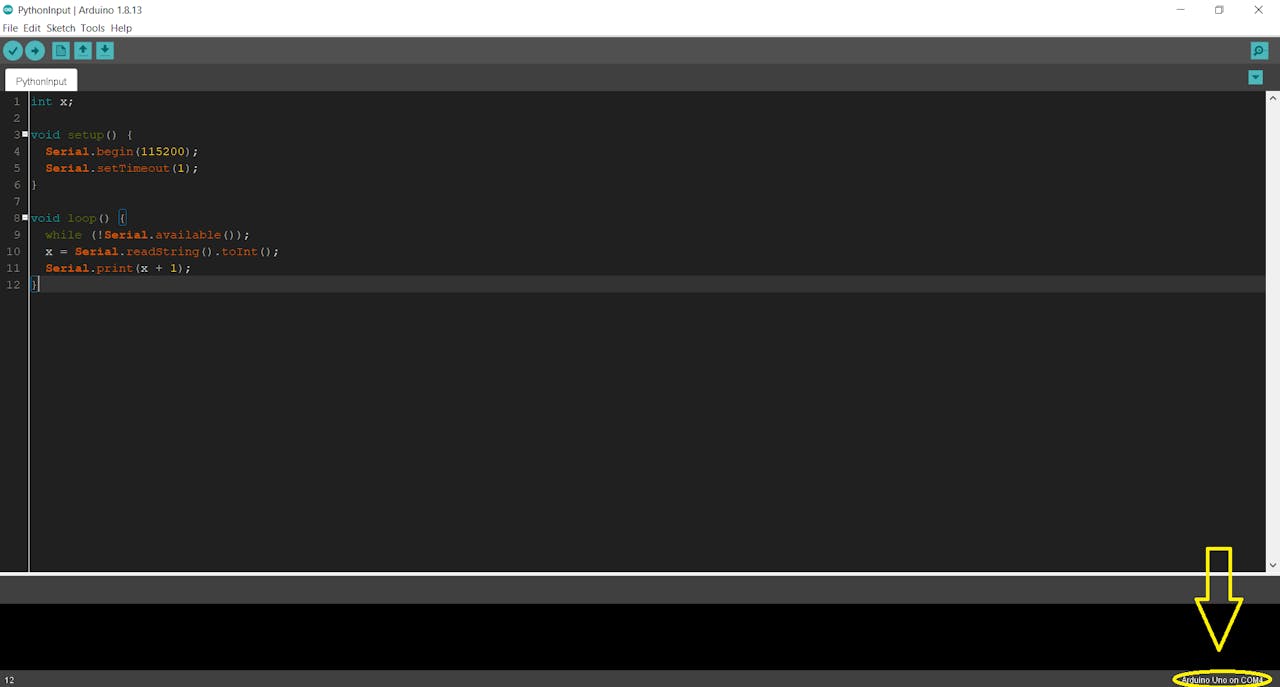



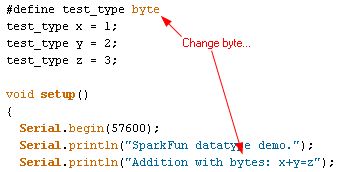
Article link: arduino int to string.
Learn more about the topic arduino int to string.
- How to Convert Integer to String Arduino – Linux Hint
- How to convert int to string on Arduino? – Stack Overflow
- How to convert integer to string and string to int on Arduino
- How to Convert Integer to String Arduino – Linux Hint
- Int to String in Java – How to Convert an Integer into a String
- Converting Integer to Character Arduino – Instructables
- Arduino String – JavaTpoint
- Arduino int to string: Arguments, function name and how it works
- Chuyển từ int sang string và string sang int trong Arduino
- Convert Integer to String in Arduino – Delft Stack
- Arduino String(), convert int to String – PlatformIO IDE
See more: https://nhanvietluanvan.com/luat-hoc