Append Multiple Items To List Python
In Python, a list is a versatile data structure that allows you to store and manipulate a collection of items. Lists are mutable, which means they can be modified after they are created. They can contain any combination of data types, including integers, strings, booleans, or even other nested lists.
Lists are defined and initialized using square brackets ([]), with the items separated by commas. Here’s an example of how to create a list:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
“`
In this example, the list `fruits` contains three elements: ‘apple’, ‘banana’, and ‘orange’.
Common Operations and Methods Associated with Lists
Lists offer various operations and methods that allow you to manipulate and work with the data they contain. Some common operations include accessing elements by their index, slicing to extract a subset of elements, and checking for membership using the `in` keyword.
Additionally, lists provide several built-in methods that simplify common tasks. Some common methods include `append()`, `extend()`, `insert()`, `remove()`, `pop()`, `sort()`, and `reverse()`, among others. These methods give you the flexibility to modify the list in different ways.
Appending Single Items to a List
The `append()` method is used to add a single element to the end of a list. It modifies the original list by adding the new item as the last element. For example:
“`
fruits = [‘apple’, ‘banana’]
fruits.append(‘orange’)
“`
After executing these lines of code, the list `fruits` will become `[‘apple’, ‘banana’, ‘orange’]`.
The syntax for the `append()` method is simple:
“`
list_name.append(item)
“`
The `item` is the element that you want to add to the list. It can be of any data type.
When you append a single item using the `append()` method, the length of the list increases by 1, and the new item becomes the last element. The order of the existing elements remains unchanged.
It is important to note that appending a single item using `append()` is an efficient operation, as it has a time complexity of O(1). This means that the time it takes to append an item does not depend on the size of the list.
Appending Multiple Items at Once Using `extend()`
In Python, you can add multiple items to a list at once using the `extend()` method. This method takes another list (or any iterable) as an argument and appends all the elements from the given list to the original list. Here’s an example:
“`
fruits = [‘apple’, ‘banana’]
more_fruits = [‘orange’, ‘grape’]
fruits.extend(more_fruits)
“`
After executing these lines of code, the list `fruits` will become `[‘apple’, ‘banana’, ‘orange’, ‘grape’]`.
The syntax for the `extend()` method is straightforward:
“`
list_name.extend(iterable)
“`
The `iterable` argument can be a list, tuple, set, or any other iterable object.
When using `extend()`, the order of the elements in the original list is preserved, and the new items are added at the end. The length of the list increases by the number of elements in the given iterable.
Working with different data types while extending a list is completely valid. For example, you can extend a list of integers with a list of strings without any issues.
Comparing Performance and Efficiency of `append()` vs. `extend()` for Multiple Items
When it comes to adding multiple items to a list, both `append()` and `extend()` have their advantages and trade-offs.
Using `append()` in a loop to add multiple items can result in poor performance, especially when dealing with large lists. Each call to `append()` requires some overhead to update the list’s internal structure.
On the other hand, `extend()` allows you to add multiple items in a single operation, making it more efficient. It updates the list’s internal structure only once, reducing the overhead.
Combining Lists Using the + Operator
In addition to using the `append()` and `extend()` methods, you can combine two or more lists using the + operator. The + operator concatenates the elements of the lists together to create a new list. Here’s an example:
“`
fruits = [‘apple’, ‘banana’]
more_fruits = [‘orange’, ‘grape’]
all_fruits = fruits + more_fruits
“`
After executing these lines of code, the list `all_fruits` will become `[‘apple’, ‘banana’, ‘orange’, ‘grape’]`.
The syntax for combining lists using the + operator is straightforward:
“`
new_list = list1 + list2
“`
The resulting list will contain the elements from `list1` followed by the elements from `list2`. The order of the elements is preserved, and the length of the final list is the sum of the lengths of the original lists.
Combining lists using the + operator can be advantageous in scenarios where you want to keep the original lists intact and create a new list with all the elements. It provides a concise syntax for list concatenation.
Efficiently Appending Items in Large Lists
Appending multiple items to a large list efficiently requires some careful considerations. Here are some strategies to improve the performance:
1. Utilize List Comprehension: List comprehension is a concise way to generate lists. Instead of appending items individually, you can use list comprehension to create a new list directly. This approach can be faster than appending items using a loop.
2. Avoid Unnecessary Copying or Resizing: Whenever possible, try to estimate the final size of the list in advance. Preallocating a larger list can reduce the need for resizing or copying during appending.
3. Consider Memory Usage vs. Time Complexity: Depending on your requirements, you may need to find a balance between memory usage and time complexity. For example, if memory is a concern, you may prefer to append items individually using the `append()` method rather than `extend()`.
Common Pitfalls and Best Practices
Appending items to lists in Python is generally straightforward, but there are some common pitfalls to avoid:
1. Modifying the List While Iterating: If you’re iterating over a list and modifying it at the same time, you may run into unexpected behaviors or errors. To avoid this, consider creating a separate list for appending the new items or use a while loop.
2. Handling Index Out of Range: When using indexing to append items to a specific position in the list using `insert()`, be cautious of the index range. Accessing an index beyond the list’s length will result in an IndexError.
3. Following Pythonic Coding Conventions: Python has a set of coding conventions and style guidelines called “Pythonic.” It’s important to follow these guidelines to write clean, readable, and maintainable code. This includes using meaningful variable names, writing docstrings, and following proper indentation.
4. Optimizing Append Operations: Depending on your specific use case and requirements, there might be additional optimizations you can make. For example, if you’re frequently appending items to the beginning of the list, using a different data structure like a deque may provide better performance.
Frequently Asked Questions
Q: Can I append multiple items using the `append()` method?
A: No, the `append()` method is designed to add only a single item to the end of a list. To add multiple items, you can use the `extend()` method or combine lists using the + operator.
Q: How can I remove multiple elements from a list?
A: To remove multiple elements from a list, you can use a combination of list slicing and using the `del` keyword. For example, to remove elements at indices 1 and 3, you can use `del my_list[1:4:2]`.
Q: How can I append all elements of a list to another list?
A: To append all elements of one list to another list, you can use the `extend()` method. For example, `list1.extend(list2)` will append all elements of `list2` to `list1`.
In conclusion, appending multiple items to a list in Python can be done efficiently using methods like `extend()` or by combining lists using the + operator. It is important to consider the performance implications, avoid common pitfalls, and follow best practices to ensure clean and optimized code.
How To Append To A List In Python
Keywords searched by users: append multiple items to list python Append multiple elements Python, Add new element to python list, Multiple list Python, python append multiple strings to list, List append list Python, Python append multiple times, Remove multiple elements from list Python, Append all elements of a list Python
Categories: Top 81 Append Multiple Items To List Python
See more here: nhanvietluanvan.com
Append Multiple Elements Python
Python is a versatile programming language that offers a wide range of functionalities. One such feature is the ability to append multiple elements to a list or array. This functionality allows programmers to efficiently add multiple items to a data structure, providing flexibility and convenience. In this article, we will delve into the process of appending multiple elements in Python, exploring various methods and examples. We will also address some frequently asked questions related to this topic.
Appending Elements to a List
In Python, a list is an ordered collection of elements, enclosed within square brackets []. To append a single element to a list, one can use the `append()` method. However, the `append()` method only appends one element at a time. So, how can we append multiple elements to a list?
1. Using the `extend()` Method:
The `extend()` method is used to append multiple elements to a list. It takes an iterable object, such as a list, tuple, or set, and adds its elements to the existing list. Here’s an example:
“`python
my_list = [1, 2, 3]
new_elements = [4, 5, 6]
my_list.extend(new_elements)
print(my_list)
“`
Output:
“`
[1, 2, 3, 4, 5, 6]
“`
2. Using the `+=` Operator:
The `+=` operator can also be used to concatenate two lists, resulting in the addition of elements from one list to another. Here’s an example:
“`python
my_list = [1, 2, 3]
new_elements = [4, 5, 6]
my_list += new_elements
print(my_list)
“`
Output:
“`
[1, 2, 3, 4, 5, 6]
“`
Appending Elements to an Array
While lists are widely used in Python, arrays offer a more memory-efficient way of storing homogeneous data types. The process of appending multiple elements to an array is slightly different from that of a list. Python provides a useful library called NumPy that allows us to perform various operations on arrays efficiently.
1. Using the `numpy.append()` Function:
The `numpy.append()` function is used to add multiple elements to an array. It takes the array, elements to be appended, and the axis (optional) as input parameters. This function returns a new array containing the appended elements. Here’s an example:
“`python
import numpy as np
my_array = np.array([1, 2, 3])
new_elements = np.array([4, 5, 6])
appended_array = np.append(my_array, new_elements)
print(appended_array)
“`
Output:
“`
[1 2 3 4 5 6]
“`
2. Using the `numpy.concatenate()` Function:
The `numpy.concatenate()` function can also be used to append arrays. Unlike the `numpy.append()` function, it concatenates arrays along an existing axis. Here’s an example:
“`python
import numpy as np
my_array = np.array([1, 2, 3])
new_elements = np.array([4, 5, 6])
appended_array = np.concatenate((my_array, new_elements))
print(appended_array)
“`
Output:
“`
[1 2 3 4 5 6]
“`
FAQs:
Q1. Can I append multiple elements of different data types to a list or array?
Python allows you to append elements of different data types to a list, as lists are capable of storing heterogeneous data. However, arrays typically require elements to be of the same data type. If you try to append elements of different data types to an array, it will automatically convert them to a common data type.
Q2. Can I append multiple elements at the beginning of a list or array?
Yes, you can prepend multiple elements to the beginning of a list or array. To achieve this, you can use the `insert()` method for lists or the `numpy.insert()` function for arrays. These methods allow you to specify the index at which the elements should be inserted.
Q3. What is the difference between `extend()` and `append()` for lists?
The `extend()` method and `append()` method differ in their functionality. The `extend()` method is used to add multiple elements to the end of a list, whereas the `append()` method is used to add a single element to the end of a list. The `extend()` method takes an iterable object, while the `append()` method takes a single object.
Q4. Are there any performance differences between using `extend()` and `+=` for lists?
There is no significant performance difference between using `extend()` and `+=` for lists. However, in some cases, the `+=` operator may provide a slight performance advantage as it avoids the method call overhead.
In conclusion, Python offers several methods for appending multiple elements to lists and arrays, providing flexibility and ease of use. By understanding these methods and their functionalities, programmers can efficiently manipulate data structures and enhance their programs. Whether using lists or arrays, Python’s versatility ensures that appending multiple elements is a simple and powerful operation.
Add New Element To Python List
In Python, lists are widely used for storing and managing collections of items. Being a versatile data structure, lists provide various methods to modify their contents. One of the common tasks is adding new elements to a list. In this article, we will explore different methods to add elements to a Python list and understand their advantages and use cases.
There are three primary approaches to adding new elements to a Python list:
1. Append method:
The append() method is the most straightforward way to add an element to the end of a list. It takes a single argument, the element to be added, and adds it to the end of the list. Here’s an example:
“`python
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
“`
The append() method is efficient when we only need to add elements to the end of a list. However, it may not be suitable if we want to insert elements at a specific position within the list. For such cases, we can explore other methods.
2. Insert method:
The insert() method allows us to add an element at a specific position within the list. It takes two arguments: the index at which the element is to be inserted and the element itself. Consider the following example:
“`python
my_list = [1, 2, 3]
my_list.insert(1, 4)
print(my_list) # Output: [1, 4, 2, 3]
“`
In this example, the number 4 is inserted at index 1, pushing the original element at that index and subsequent elements to the right. This method gives us the flexibility to add elements at any position within the list.
3. Concatenation and extend:
Another approach to add elements to a list is by concatenating two lists. We can use the ‘+’ operator to combine two lists into a new list. Here’s an example:
“`python
my_list = [1, 2, 3]
new_elements = [4, 5]
my_list = my_list + new_elements
print(my_list) # Output: [1, 2, 3, 4, 5]
“`
In this example, we create a new list consisting of the original list and the new elements. This method allows us to add multiple elements at once. However, concatenation creates a new list in memory, which may not be desirable if dealing with large lists.
Alternatively, we can use the extend() method to append elements from another iterable to the end of an existing list. This method takes an iterable (e.g., list, tuple, or set) as an argument. Here’s how it works:
“`python
my_list = [1, 2, 3]
new_elements = [4, 5]
my_list.extend(new_elements)
print(my_list) # Output: [1, 2, 3, 4, 5]
“`
In this example, the elements of the new_elements list are added to the end of the my_list using the extend() method. The extend() method is particularly useful when we want to append multiple elements from another iterable without creating a new list.
Frequently Asked Questions:
Q: Can we add elements to a specific index using the append() method?
A: No, the append() method can only add elements to the end of a list. If you need to add an element at a specific position, you should use the insert() method instead.
Q: How do we add multiple elements to a list at once?
A: There are multiple ways to add multiple elements to a list. You can use the concatenation operator ‘+’ to combine two lists, or use the extend() method to append elements from another iterable to the end of an existing list.
Q: What is the difference between the insert() and extend() methods?
A: The insert() method allows you to add an element at a specific position within a list, whereas the extend() method appends elements from another iterable to the end of an existing list.
Q: Are there any performance considerations when adding elements to lists?
A: Yes, performance considerations may arise when dealing with large lists. The append() method is the most efficient for adding elements to the end of a list, whereas concatenation and extend() methods can be costly for large lists as they involve creating a new list in memory.
Conclusion:
Adding new elements to a Python list is a common requirement when working with collections of data. Python provides several methods, including append(), insert(), and concatenation/extend, to achieve this task. The choice of method depends on the specific use case, such as adding elements to the end of the list, at a specific position, or appending from another iterable. Understanding these methods and their differences empowers developers to efficiently manage and modify list contents in Python.
Images related to the topic append multiple items to list python
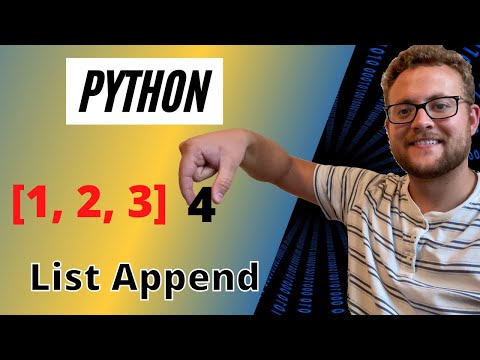
Found 40 images related to append multiple items to list python theme


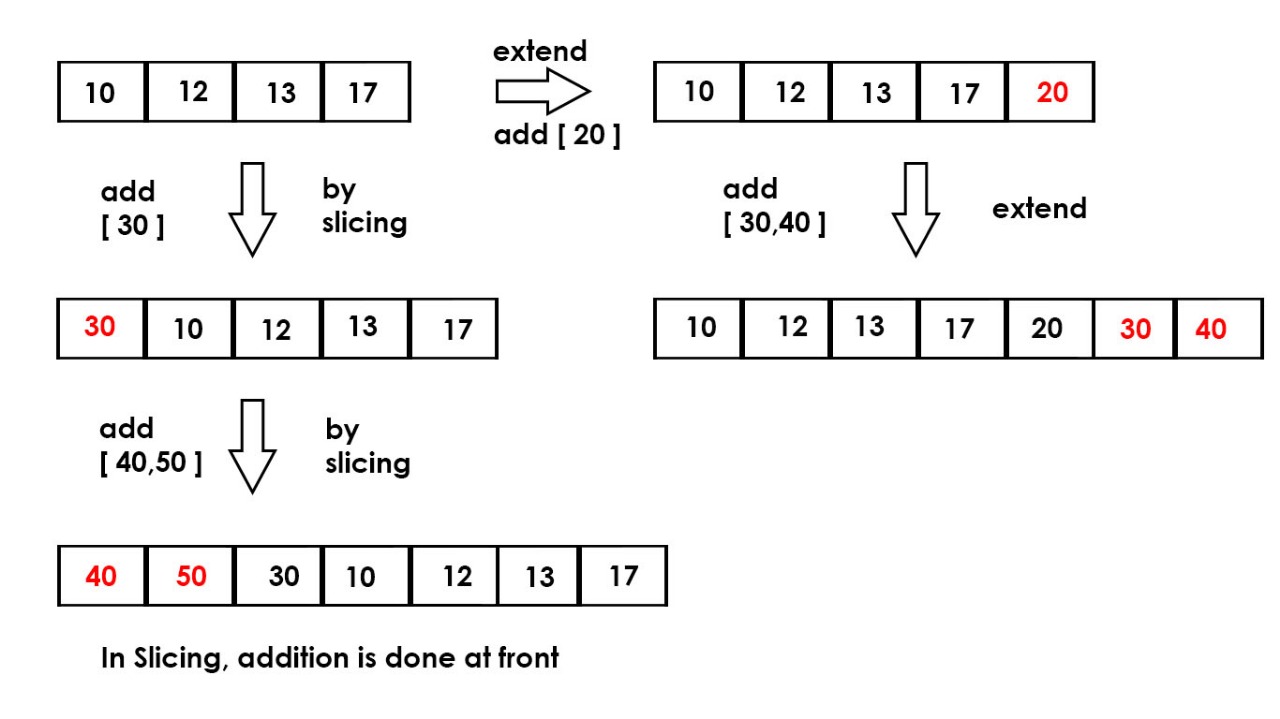
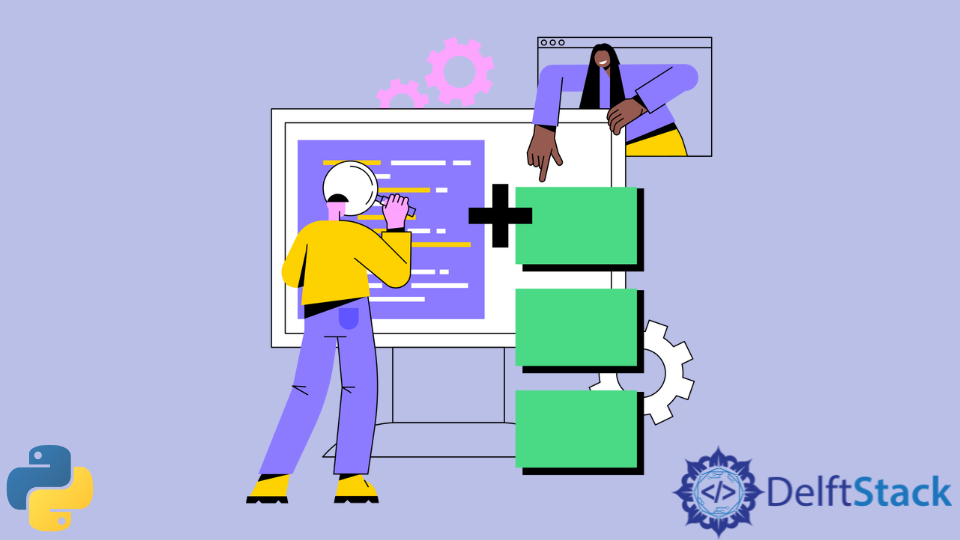



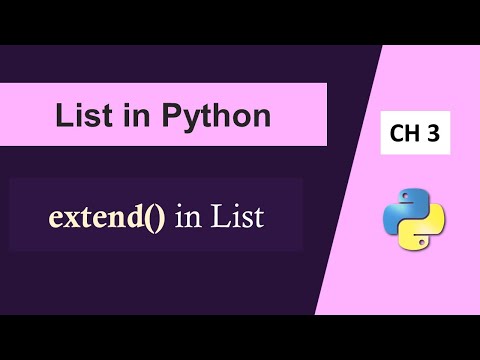
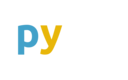
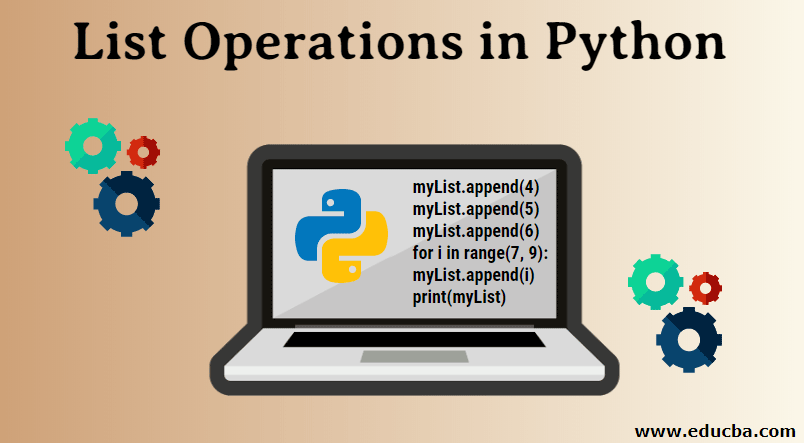
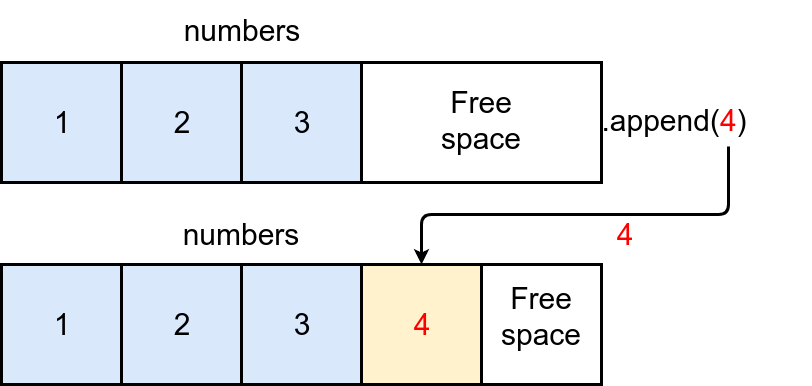
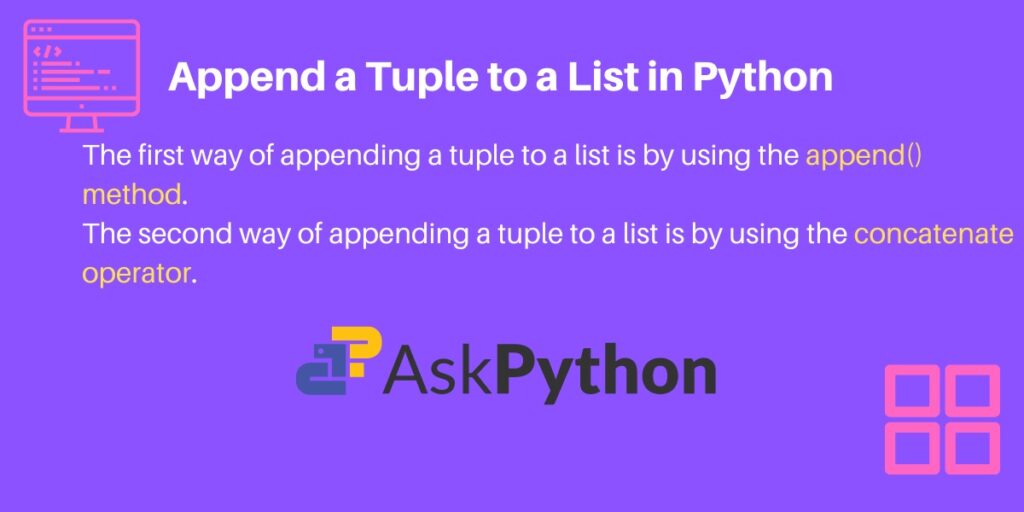


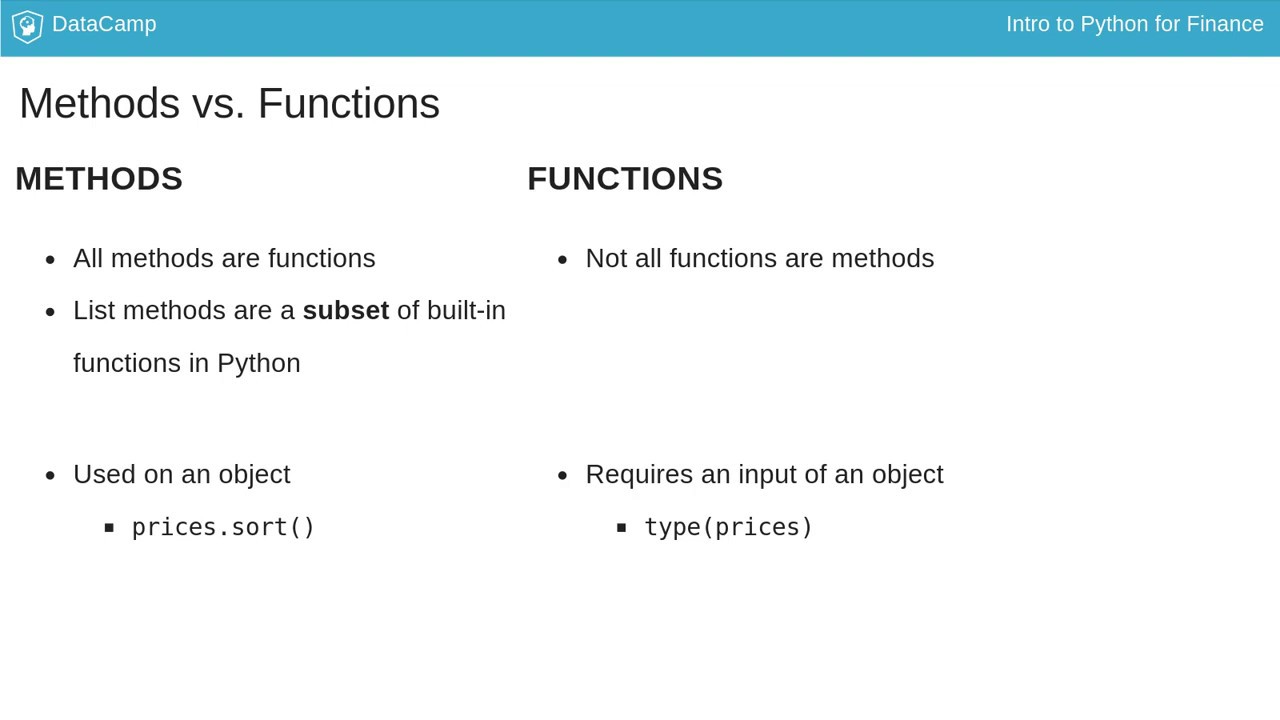
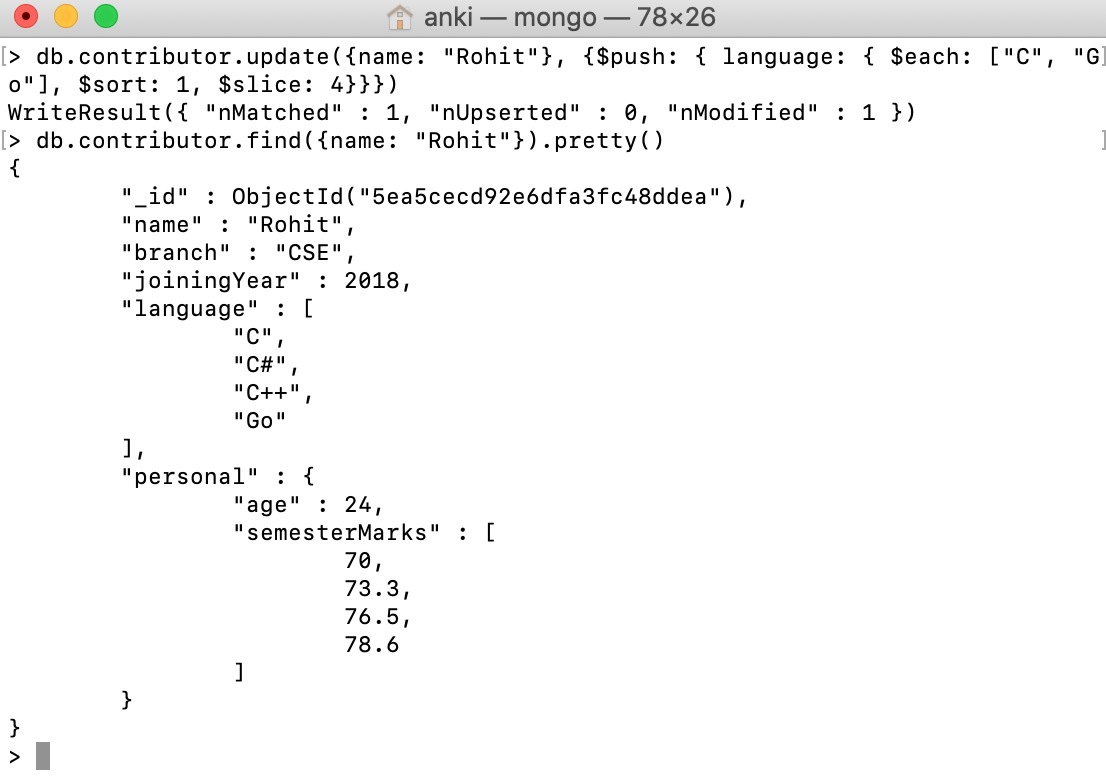

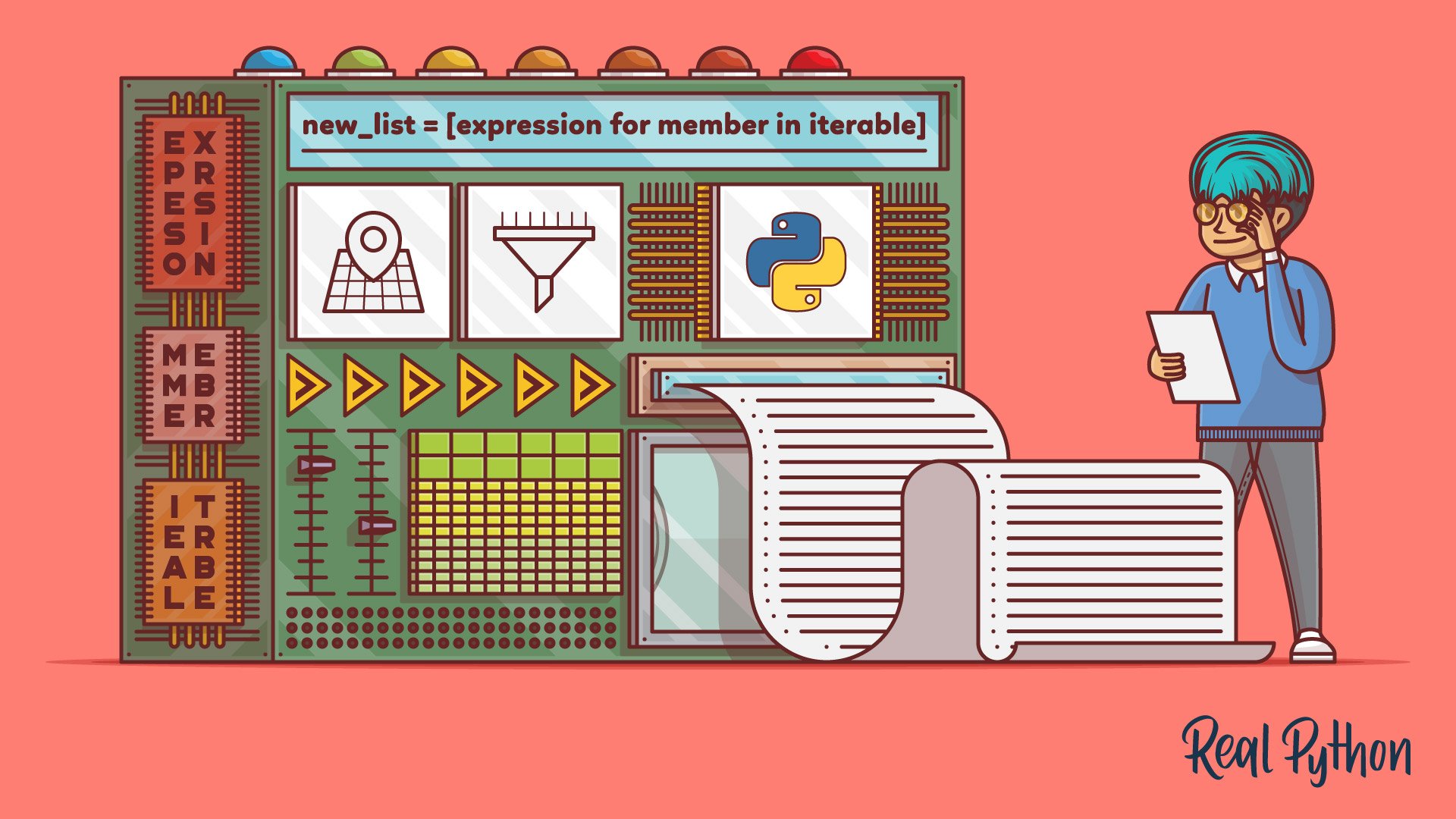
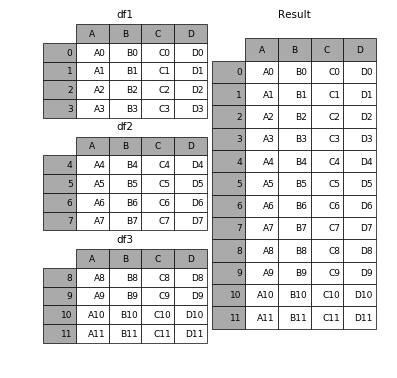
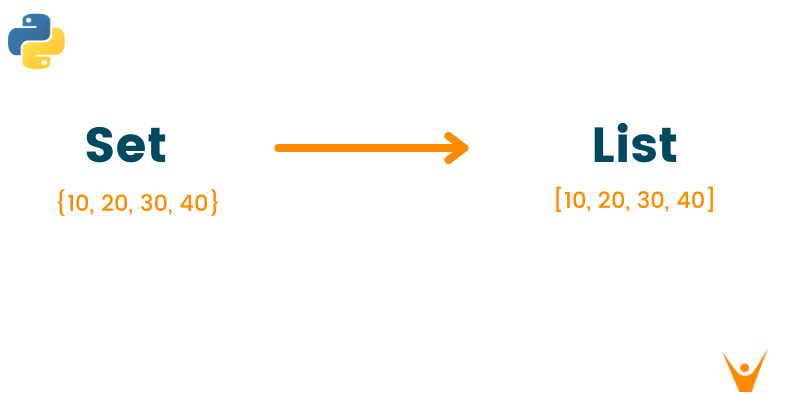
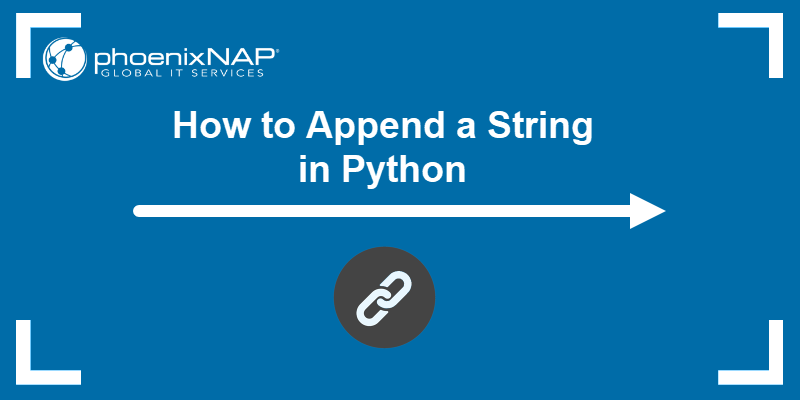

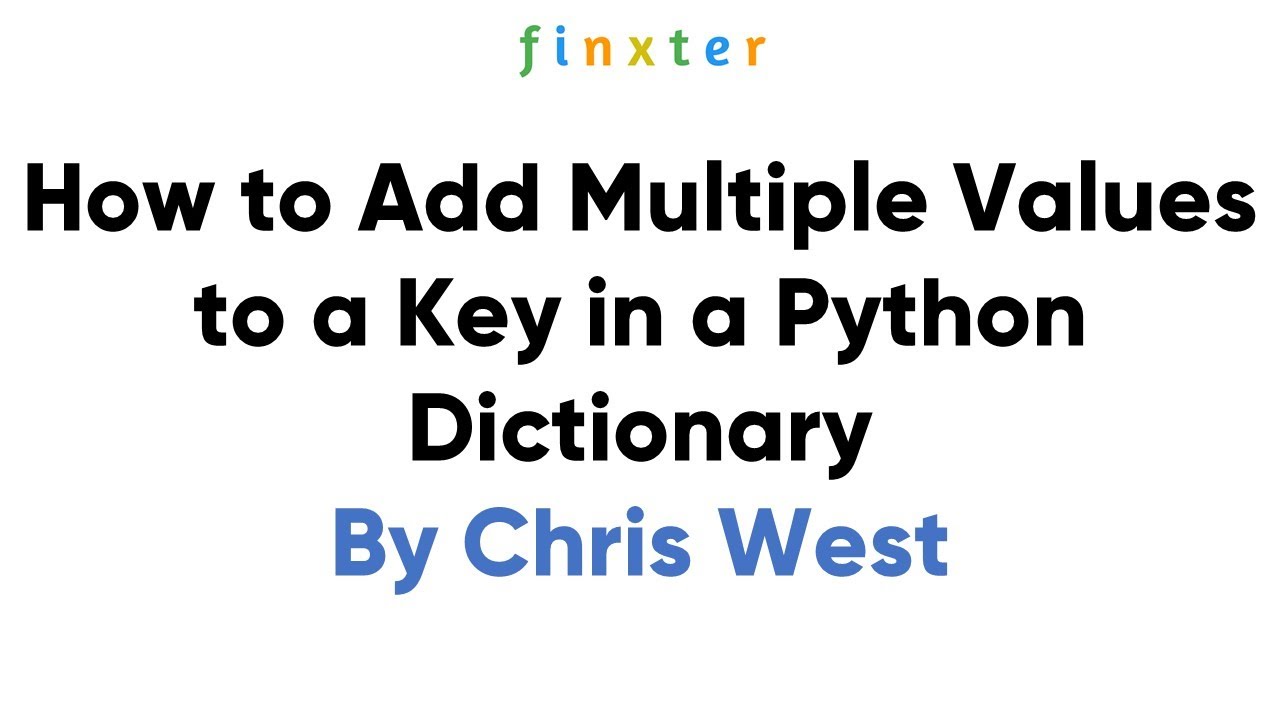


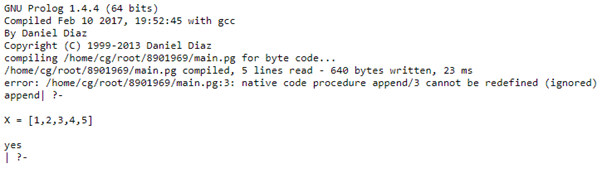
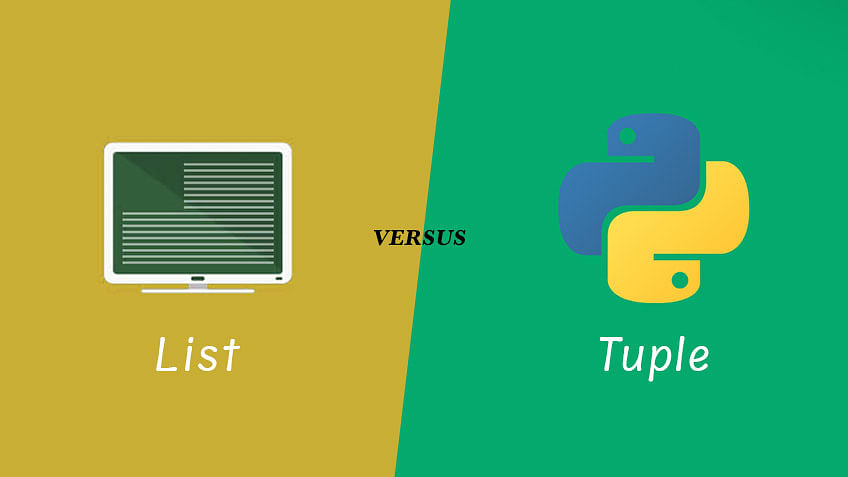
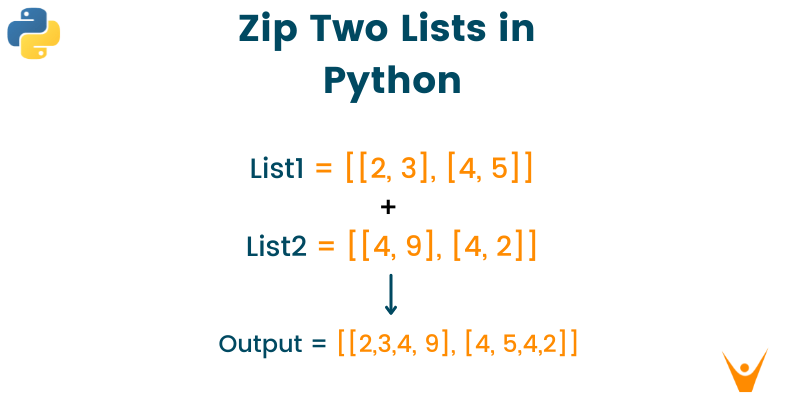
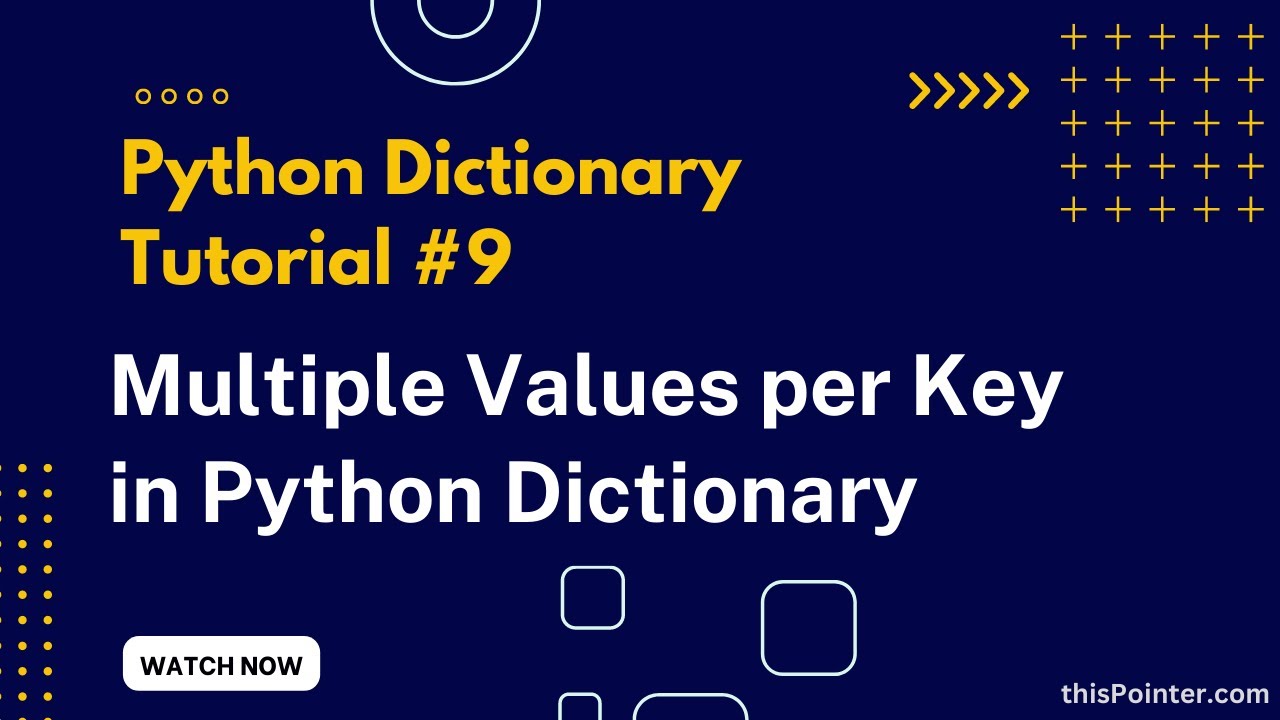
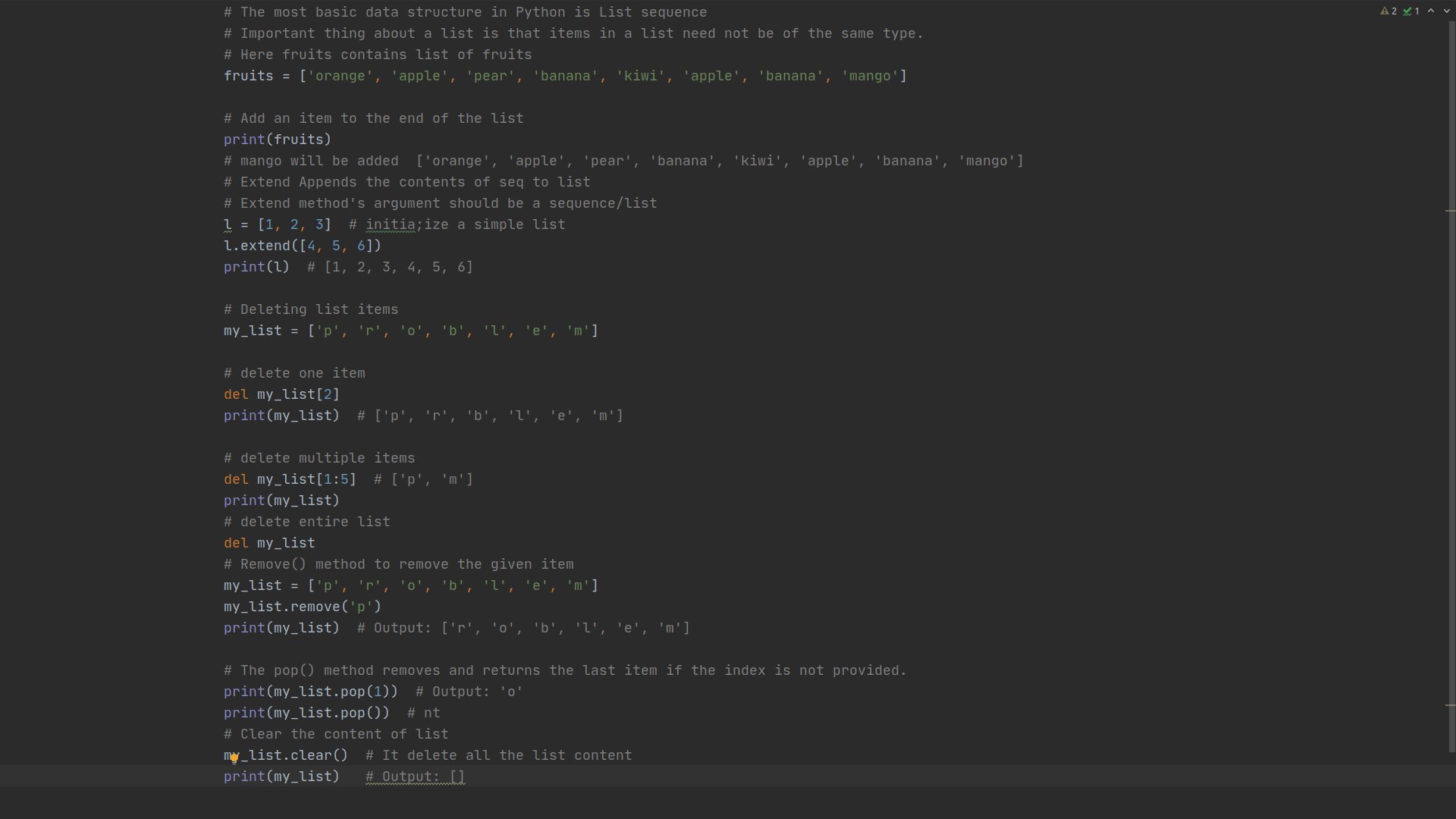

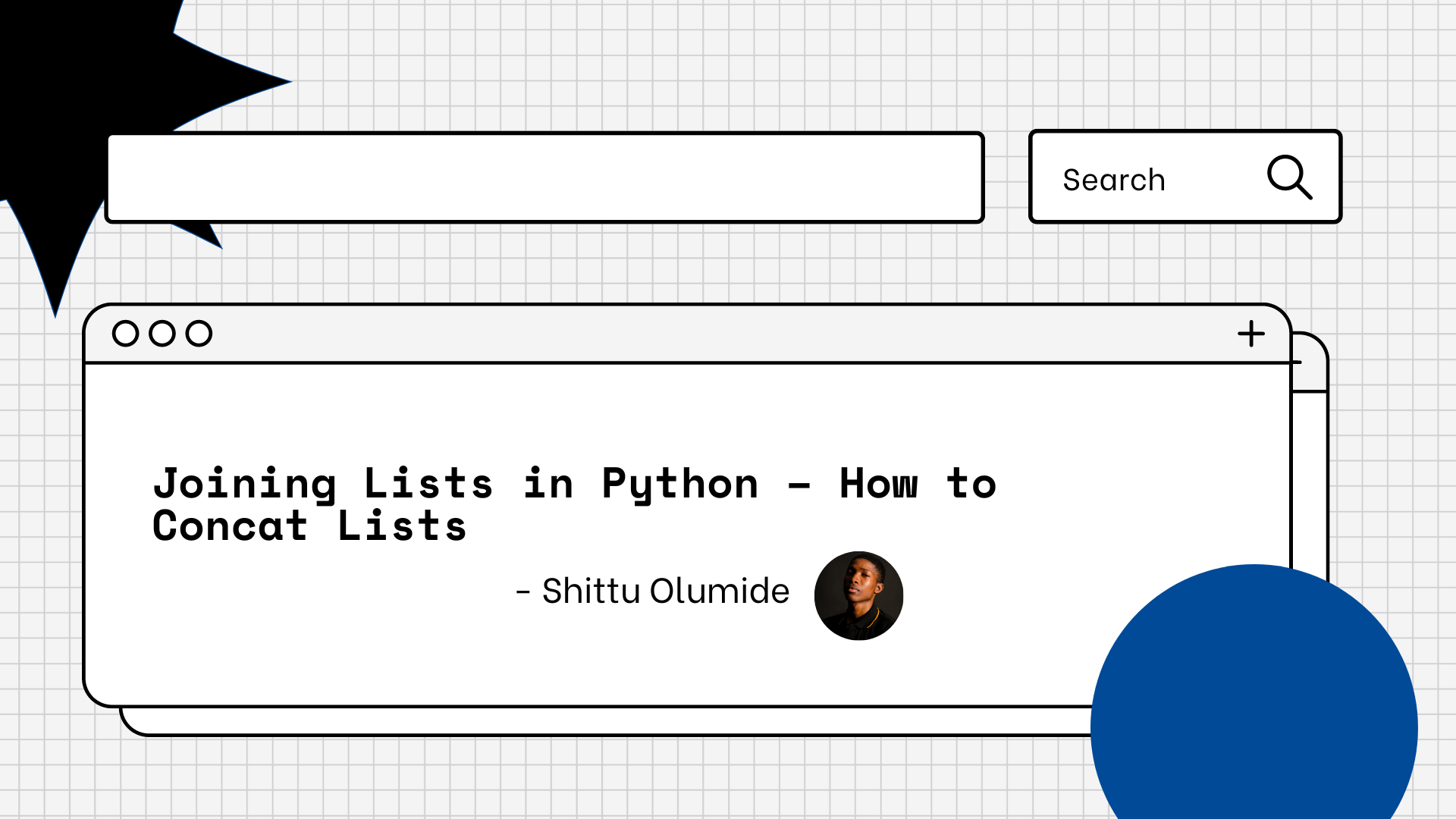
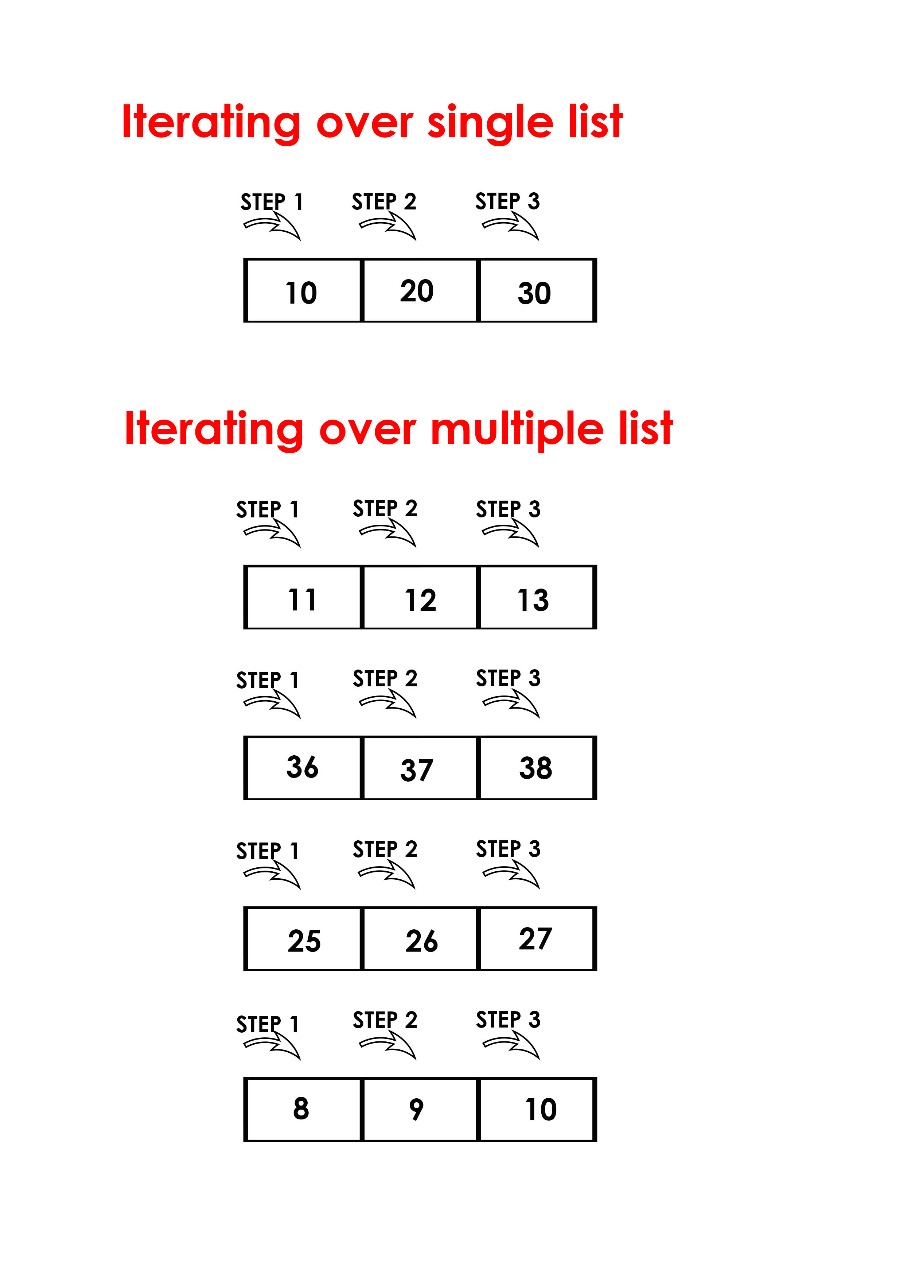
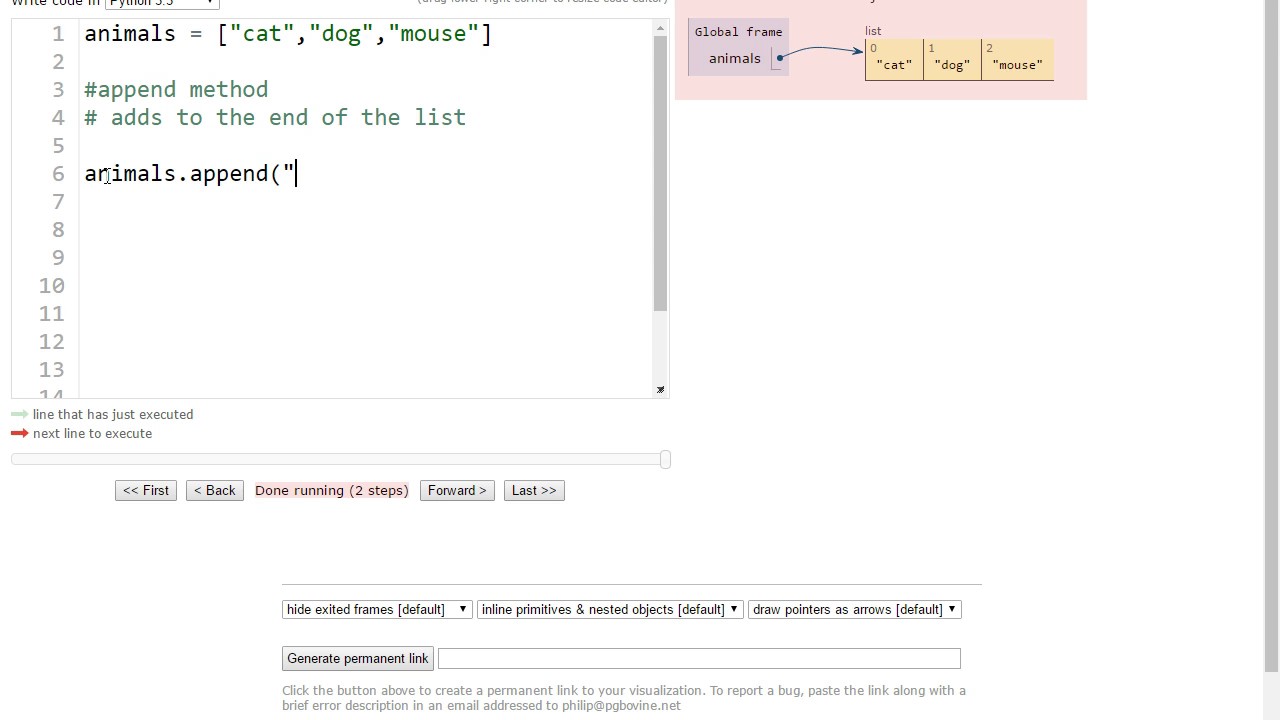
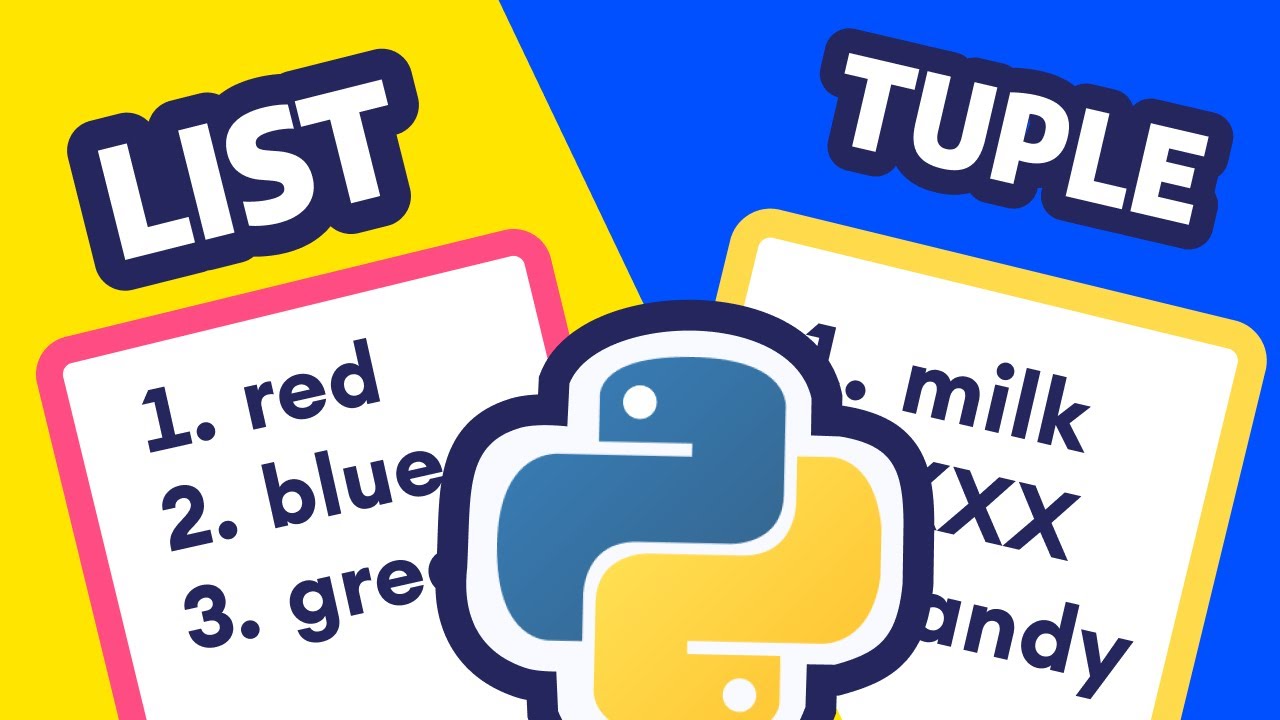

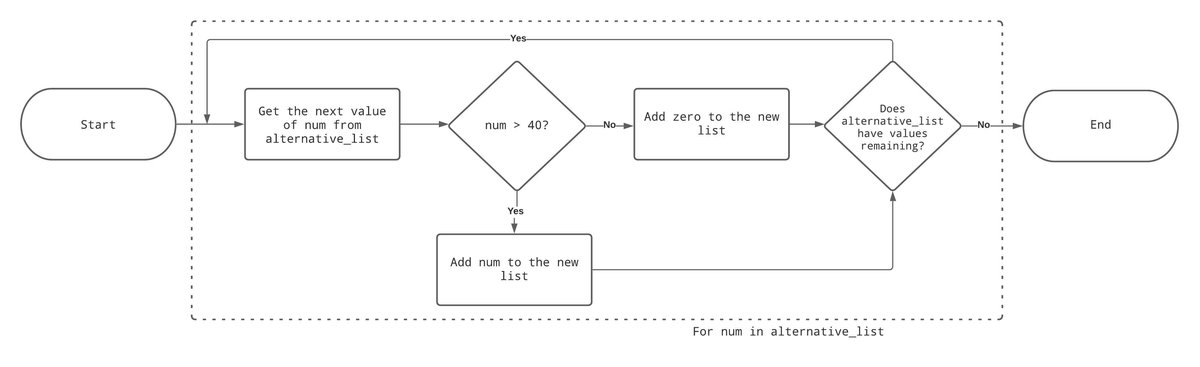
Article link: append multiple items to list python.
Learn more about the topic append multiple items to list python.
- How to append multiple values to a list in Python
- Python Append Items Elements to List – Spark By {Examples}
- How to append multiple Values to a List in Python – bobbyhadz
- How to Append Multiple Items to List at Once in Python
- How to Append Multiple Items to List in Python – PyTutorial
- How To add Elements to a List in Python – DigitalOcean
- Append Multiple Elements to List in Python | Delft Stack
- Python Tutorial: How to append multiple items to a list in Python
- Python List Extend: Append Multiple Items to a List – Datagy
- Python Append Multiple Items To List – AppDividend