Ansible Combine Two Lists
Ansible, an open-source automation tool, provides various ways to manipulate and combine lists. Combining lists allows you to aggregate data, merge dictionaries, concatenate strings, and perform other operations. In this article, we will explore different methods and filters in Ansible to combine two lists effectively.
Using the “combine” filter is one of the simplest ways to merge two lists in Ansible. The “combine” filter takes two or more lists as input and returns a new list containing all elements from the input lists. Let’s see an example:
“`yaml
– hosts: localhost
tasks:
– name: Merge two lists
set_fact:
merged_list: “{{ list1 | combine(list2) }}”
vars:
list1:
– item1
– item2
list2:
– item3
– item4
– debug:
var: merged_list
“`
In the above example, we define two lists, `list1` and `list2`, and then use the “combine” filter to merge them into a new list called `merged_list`. Finally, we print the merged list using the `debug` module.
Using the “union” filter
The “union” filter in Ansible is another method to merge lists. Similar to the “combine” filter, it takes two or more lists and returns a new list without duplicate elements. Here’s an example:
“`yaml
– hosts: localhost
tasks:
– name: Merge two lists
set_fact:
merged_list: “{{ list1 | union(list2) }}”
vars:
list1:
– item1
– item2
list2:
– item2
– item3
– debug:
var: merged_list
“`
In this example, `list1` contains “item1” and “item2”, while `list2` contains “item2” and “item3”. When we merge these lists using the “union” filter, the duplicate element “item2” is removed from the final merged list.
Using the “+” operator to merge lists
The “+” operator in Ansible allows us to concatenate or merge two lists. Unlike the “combine” and “union” filters, the “+” operator preserves duplicate elements in the resulting list. Take a look at the following example:
“`yaml
– hosts: localhost
tasks:
– name: Merge two lists
set_fact:
merged_list: “{{ list1 + list2 }}”
vars:
list1:
– item1
– item2
list2:
– item2
– item3
– debug:
var: merged_list
“`
In this case, both “item2” elements are included in the merged list because the “+” operator doesn’t remove duplicates.
Handling duplicate values while combining lists
If you want to remove duplicate values while combining lists, you can use the “unique” filter in Ansible. The “unique” filter takes a list as input and returns a new list with unique elements. Here’s an example:
“`yaml
– hosts: localhost
tasks:
– name: Merge two lists and remove duplicates
set_fact:
merged_list: “{{ (list1 + list2) | unique }}”
vars:
list1:
– item1
– item2
list2:
– item2
– item3
– debug:
var: merged_list
“`
In this example, the “unique” filter is used after merging `list1` and `list2`. As a result, the duplicate element “item2” is removed from the merged list.
Using the “zip” filter to combine lists in parallel
The “zip” filter in Ansible enables us to combine multiple lists in parallel. It takes two or more lists as input and returns a new list containing elements pairs from the input lists. If the input lists have different lengths, the resulting list will have the length of the shortest input list. Let’s see an example:
“`yaml
– hosts: localhost
tasks:
– name: Combine lists in parallel
set_fact:
combined_list: “{{ list1 | zip(list2) | list }}”
vars:
list1:
– item1
– item2
list2:
– value1
– value2
– debug:
var: combined_list
“`
In this example, the “zip” filter is used to combine `list1` and `list2` in parallel. The resulting list, `combined_list`, will contain [(“item1”, “value1”), (“item2”, “value2”)]. If one list is shorter than the other, only the corresponding elements will be included in the resulting list.
Using loops to combine lists dynamically
Ansible allows us to use loops to combine lists dynamically. By iterating over a list, we can dynamically generate and merge multiple lists. Here’s an example:
“`yaml
– hosts: localhost
vars:
lists_to_combine:
– [1, 2, 3]
– [4, 5, 6]
– [7, 8, 9]
tasks:
– name: Combine lists dynamically
set_fact:
combined_list: “{{ combined_list | default([]) + item }}”
loop: “{{ lists_to_combine }}”
– debug:
var: combined_list
“`
In this example, we define a variable `lists_to_combine` that contains multiple lists. Using a loop, we iterate over each list in `lists_to_combine` and add its elements to the `combined_list`. Finally, we print the resulting `combined_list` using the `debug` module.
Using the “combine” filter with conditional statements
The “combine” filter can also be used with conditional statements to control the merging of lists based on certain conditions. Suppose you want to merge two lists only if a specific condition is met. Here’s an example that demonstrates this:
“`yaml
– hosts: localhost
vars:
list1:
– item1
– item2
list2:
– item3
– item4
condition: true
tasks:
– name: Merge two lists conditionally
set_fact:
merged_list: “{{ list1 | combine(list2) if condition else list1 }}”
– debug:
var: merged_list
“`
In this example, the `merged_list` will contain the result of merging `list1` and `list2` if the `condition` variable is true. Otherwise, it will contain `list1` as it is.
Using the “with_nested” loop to combine multiple lists
Sometimes, you may need to combine multiple lists, each containing different elements, into a single list of dictionaries. In such cases, the “with_nested” loop comes in handy. Let’s see an example:
“`yaml
– hosts: localhost
vars:
list1:
– value1
– value2
list2:
– item1
– item2
tasks:
– name: Combine lists into a list of dictionaries
set_fact:
combined_list: “{{ combined_list | default([]) + [{‘key1’: item.0, ‘key2’: item.1}] }}”
with_nested:
– “{{ list1 }}”
– “{{ list2 }}”
– debug:
var: combined_list
“`
In this example, the “with_nested” loop iterates over each combination of elements from `list1` and `list2`. For each combination, a new dictionary is created with keys “key1” and “key2” mapping to the elements from `list1` and `list2` respectively. The resulting list of dictionaries is stored in the `combined_list` variable.
FAQs
Q: What is the difference between the “combine” and “union” filters?
A: The “combine” filter merges two or more lists into a new list, preserving all elements, including duplicates. The “union” filter also merges lists, but it removes duplicate elements from the resulting list.
Q: How can I handle duplicate values while combining lists in Ansible?
A: To remove duplicate values, you can use the “unique” filter after merging the lists. The “unique” filter returns a new list with only unique elements.
Q: Can I use loops to combine lists dynamically in Ansible?
A: Yes, you can use loops to iterate over multiple lists and dynamically combine them. By iterating over a list, you can add its elements to a new list.
Q: How can I conditionally merge two lists in Ansible?
A: You can use the “combine” filter in conjunction with conditional statements to merge lists based on certain conditions. Use the conditional expression to decide whether to merge the lists or not.
Q: Can I combine multiple lists into a list of dictionaries in Ansible?
A: Yes, you can use the “with_nested” loop to combine multiple lists into a list of dictionaries. Each element in the resulting list will be a dictionary with keys and their corresponding values from the input lists.
Excel Magic Trick 381: Merge Two Lists Tables W Advanced Filter And Remove Duplicates Feature
Keywords searched by users: ansible combine two lists ansible merge lists unique, ansible combine list of dictionaries, ansible concatenate list to string, ansible loop two lists, ansible join list with commas, ansible lists, ansible combine filter, ansible merge dictionaries
Categories: Top 41 Ansible Combine Two Lists
See more here: nhanvietluanvan.com
Ansible Merge Lists Unique
Introduction:
Ansible is an open-source automation tool widely used for deploying, configuring, and managing systems. With its powerful features and flexibility, Ansible allows users to effortlessly execute tasks across multiple servers. In this article, we will focus on one specific aspect of Ansible: merging lists and selecting unique items, along with an FAQ section to address common queries.
Merging Lists in Ansible:
When working with Ansible, there may be scenarios where you need to merge two or more lists together. This can be achieved using the `combine` filter. The `combine` filter concatenates two or more lists and provides the combined list as output.
Let’s consider an example where we have two lists: `list1` and `list2`.
“`
list1:
– item1
– item2
list2:
– item3
– item4
“`
To merge these two lists, we can use the following Ansible task:
“`
– name: Merge lists
set_fact:
merged_list: “{{ list1 | combine(list2) }}”
“`
The `merged_list` will now contain all the items from `list1` and `list2`:
“`
merged_list:
– item1
– item2
– item3
– item4
“`
We can use this approach with any number of lists and merge them together.
Selecting Unique Items:
Sometimes, you may also want to filter out duplicate items from a merged list and obtain only the unique ones. Ansible provides the `unique` filter to accomplish this task. Let’s suppose we have a merged list `merged_list` containing multiple occurrences of the same item.
“`
merged_list:
– item1
– item1
– item2
– item3
– item3
“`
To extract only the unique items from this list, we can use the `unique` filter as shown below:
“`
– name: Select unique items
set_fact:
unique_items: “{{ merged_list | unique }}”
“`
Upon executing this task, the `unique_items` list will contain only the unique elements:
“`
unique_items:
– item1
– item2
– item3
“`
By employing the `unique` filter, you can easily eliminate duplicate entries and obtain a refined list.
Frequently Asked Questions:
Q1: Can lists with dictionary items be merged in Ansible?
Ans: Yes, lists containing dictionary items can be merged using the `combine` filter just like regular lists. However, it is important to ensure that the dictionaries have distinct keys to avoid data conflicts.
Q2: How can I merge multiple lists into one list using Ansible?
Ans: To merge multiple lists into one, you can use the `combine` filter repeatedly. For example, if you have three lists: `list1`, `list2`, and `list3`, the following task can be used:
“`
– name: Merge lists
set_fact:
merged_list: “{{ list1 | combine(list2) | combine(list3) }}”
“`
Q3: How can I merge two lists without creating duplicate entries?
Ans: To avoid duplicate entries when merging two lists, you can use the `unique` filter after merging. For instance:
“`
– name: Merge and select unique items
set_fact:
merged_list: “{{ list1 | combine(list2) }}”
unique_items: “{{ merged_list | unique }}”
“`
Q4: Can I merge and select unique items at the same time?
Ans: Yes, you can merge lists and select unique items simultaneously. We can achieve this by chaining the `combine` and `unique` filters together. Here’s an example:
“`
– name: Merge and select unique items
set_fact:
unique_merged_list: “{{ list1 | combine(list2) | unique }}”
“`
Conclusion:
In this article, we discussed how to merge lists in Ansible using the `combine` filter and select unique items using the `unique` filter. Merging lists enables us to consolidate data from various sources, while selecting unique items helps in reducing redundancy. By mastering these techniques, you can significantly improve your Ansible automation workflows and system management capabilities.
FAQ Section:
Q1: Can lists with dictionary items be merged in Ansible?
Ans: Yes, lists containing dictionary items can be merged using the `combine` filter.
Q2: How can I merge multiple lists into one using Ansible?
Ans: To merge multiple lists into one, you can use the `combine` filter repeatedly.
Q3: How can I merge two lists without creating duplicate entries?
Ans: To avoid duplicate entries when merging two lists, use the `unique` filter after merging.
Q4: Can I merge and select unique items at the same time?
Ans: Yes, you can merge lists and select unique items simultaneously by chaining the `combine` and `unique` filters together.
Ansible Combine List Of Dictionaries
Introduction:
Ansible, an open-source automation tool, has gained widespread popularity for its ability to simplify complex tasks and streamline IT operations. With its declarative and agentless approach, it enables administrators to manage and configure large-scale infrastructures effortlessly. One of Ansible’s powerful features is the ability to combine lists of dictionaries, allowing for fine-grained control and efficient automation. In this article, we will explore the process of combining lists of dictionaries in Ansible, its benefits, and provide practical examples to illustrate its usage.
I. Understanding Lists and Dictionaries in Ansible:
Before diving into combining lists of dictionaries, it is essential to understand the two data structures: lists and dictionaries. In Ansible, a list is an ordered collection of items, while a dictionary is an unordered collection of key-value pairs. Lists are denoted by square brackets ([ ]) and dictionaries by curly braces ({ }). Here’s an example to illustrate the syntax:
“`
my_list = [item1, item2, item3]
my_dict = {key1: value1, key2: value2, key3: value3}
“`
Lists can contain elements of any type, while dictionaries can have different values assigned to their respective keys. These data structures provide flexibility and enable efficient organization and retrieval of information.
II. Combining Lists of Dictionaries:
When working with complex infrastructures and automation tasks, it is often necessary to combine multiple lists of dictionaries. Ansible provides several methods to accomplish this, including the use of filters, loops, and built-in functions.
1. Filter: The `combine` filter in Ansible allows you to merge dictionaries together, overriding values in case of conflicts. To combine lists of dictionaries, you can use the filter in a loop construct. Here’s an example:
“`
– name: Combine lists of dictionaries
set_fact:
combined_list: “{{ combined_list|default([]) + my_list }}”
loop: “{{ my_lists }}”
“`
In this example, `my_list` is a list of dictionaries, and `my_lists` is a list containing several lists of dictionaries. The `combine` filter merges the dictionaries from `my_list` into the `combined_list` variable.
2. Loop: Another approach is to iterate over each list of dictionaries using the `with_items` loop. Here’s an example:
“`
– name: Combine lists of dictionaries
set_fact:
combined_list: “{{ combined_list|default([]) + item }}”
with_items:
– “{{ my_list1 }}”
– “{{ my_list2 }}”
– “{{ my_list3 }}”
“`
In this example, `my_list1`, `my_list2`, and `my_list3` are lists of dictionaries. The loop iterates over each list and appends its content to the `combined_list` using the `+` operator.
3. Built-in Functions: Ansible also provides various built-in functions to combine lists of dictionaries. The `union` function, for instance, merges dictionaries without overriding conflicting values. Here’s an example:
“`
– name: Combine lists of dictionaries
set_fact:
combined_list: “{{ my_list1|union(my_list2, my_list3) }}”
“`
In this example, `my_list1`, `my_list2`, and `my_list3` are lists of dictionaries. The `union` function combines them into a single `combined_list`, ensuring no values are overridden.
III. Benefits of Combining Lists of Dictionaries:
Combining lists of dictionaries in Ansible brings several benefits, contributing to efficient automation:
1. Simplified Data Management: By combining related information from multiple sources, lists of dictionaries provide a unified and comprehensive view of the data to be processed. This eliminates the need for manual consolidation and simplifies the management of complex automation tasks.
2. Enhanced Flexibility: Combining lists of dictionaries allows for flexible automation, enabling granular control over individual items within a unified data structure. Complex configurations, such as multi-environment deployments, can be streamlined and easily managed.
3. Improved Reusability: The ability to combine lists of dictionaries promotes code reuse. Automation playbooks or roles can utilize standardized dictionaries, enhancing maintainability and reducing redundancy across multiple automation tasks.
4. Streamlined Reporting and Analytics: Combining lists of dictionaries enables easy extraction and analysis of specific data elements. This facilitates the generation of comprehensive reports and insightful analytics, providing valuable insights into system behavior and performance.
FAQs:
Q1. Can I combine more than three lists of dictionaries in Ansible?
Ans: Absolutely! Ansible allows you to combine any number of lists of dictionaries using the mentioned techniques. You can iterate over the lists, apply filters, or utilize built-in functions to achieve this.
Q2. What happens if there are conflicting keys while combining dictionaries?
Ans: By default, when combining dictionaries, Ansible overrides the values of conflicting keys with the last occurrence. However, you can utilize specific filters or functions like `union` to handle conflicts differently, ensuring all values are preserved.
Q3. How can I access specific values within the combined list of dictionaries?
Ans: Once you have combined the lists of dictionaries, you can access specific values using dot notation, such as `combined_list[0].key1`. You can also utilize Ansible’s powerful template language and filters to extract and manipulate data as needed.
Q4. Are there any performance considerations while combining large lists of dictionaries?
Ans: Combining large lists of dictionaries can impact performance, especially if done repeatedly within a loop. It is recommended to optimize your automation logic and utilize filters or functions wisely to minimize unnecessary computational overhead.
Q5. Can I modify the combined list of dictionaries during runtime?
Ans: Yes, Ansible allows you to modify the combined list of dictionaries during runtime. You can apply various transformations, filters, and operations according to your requirements. However, make sure to handle potential conflicts and maintain data integrity.
Conclusion:
Combining lists of dictionaries in Ansible is a powerful technique that greatly enhances automation capabilities. It simplifies data management, provides flexibility, promotes code reuse, and enables insightful analytics. By understanding the syntax and utilizing the appropriate filters, loops, or functions, you can leverage this feature to efficiently automate complex tasks and manage large-scale infrastructures with ease. So, embrace the power of combining lists of dictionaries in Ansible, and elevate your automation game to new heights.
Images related to the topic ansible combine two lists
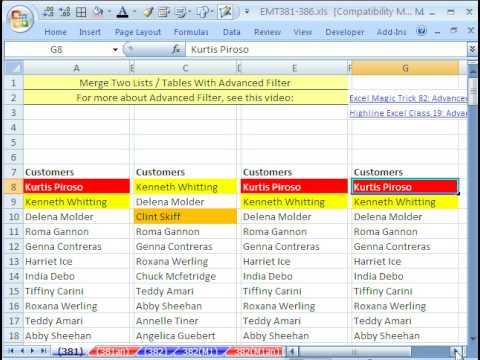
Found 20 images related to ansible combine two lists theme
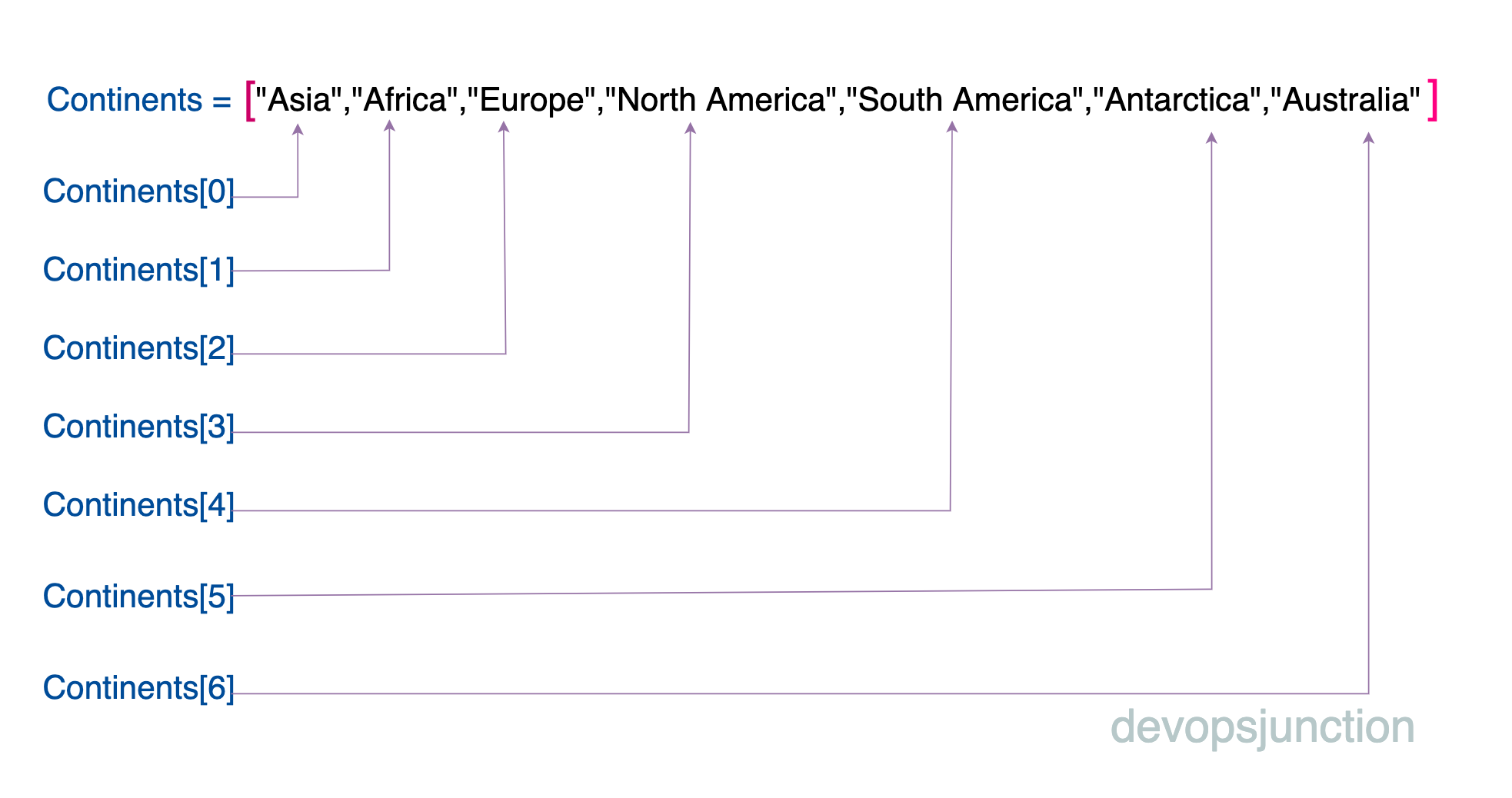


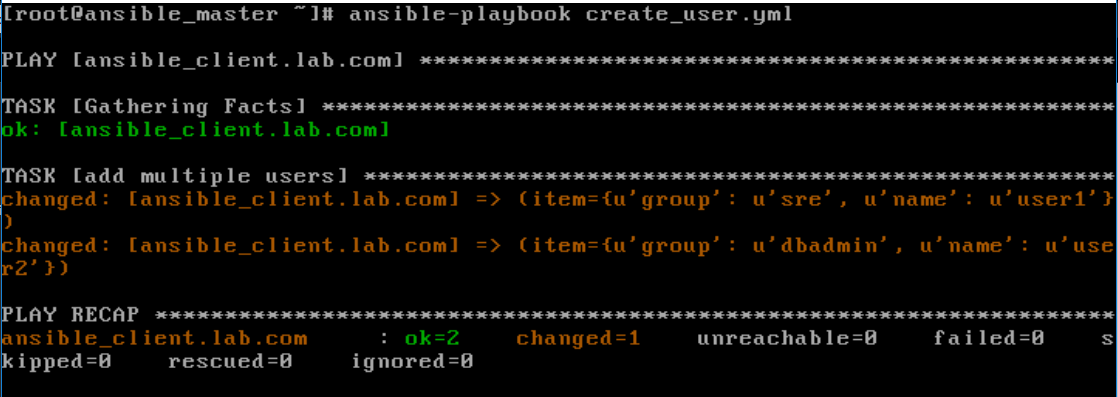


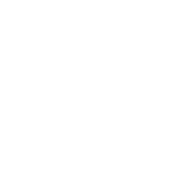

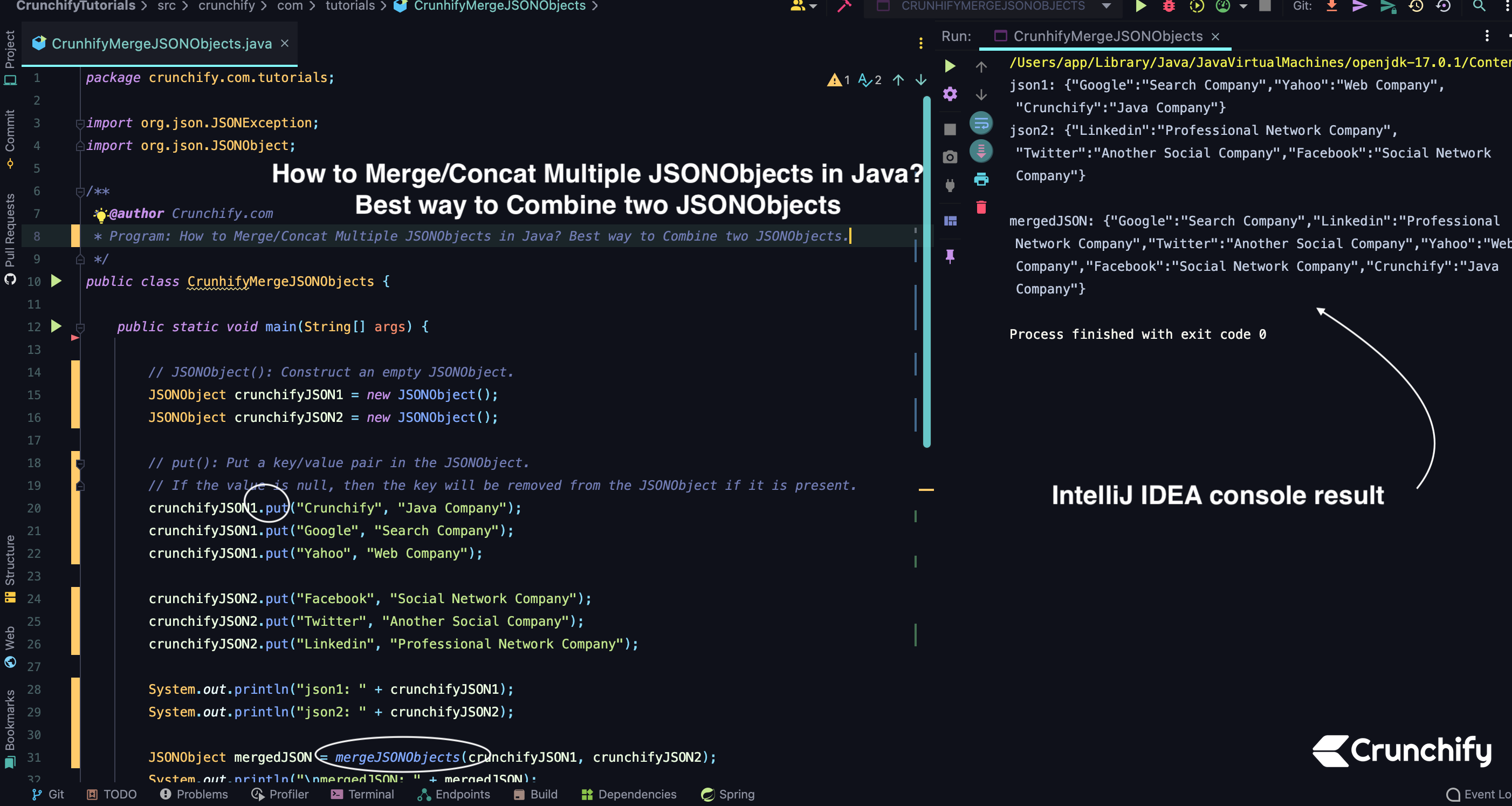
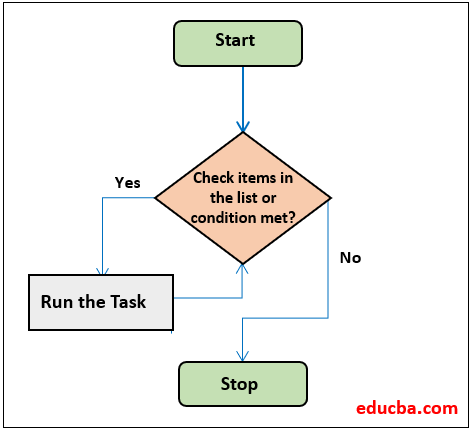
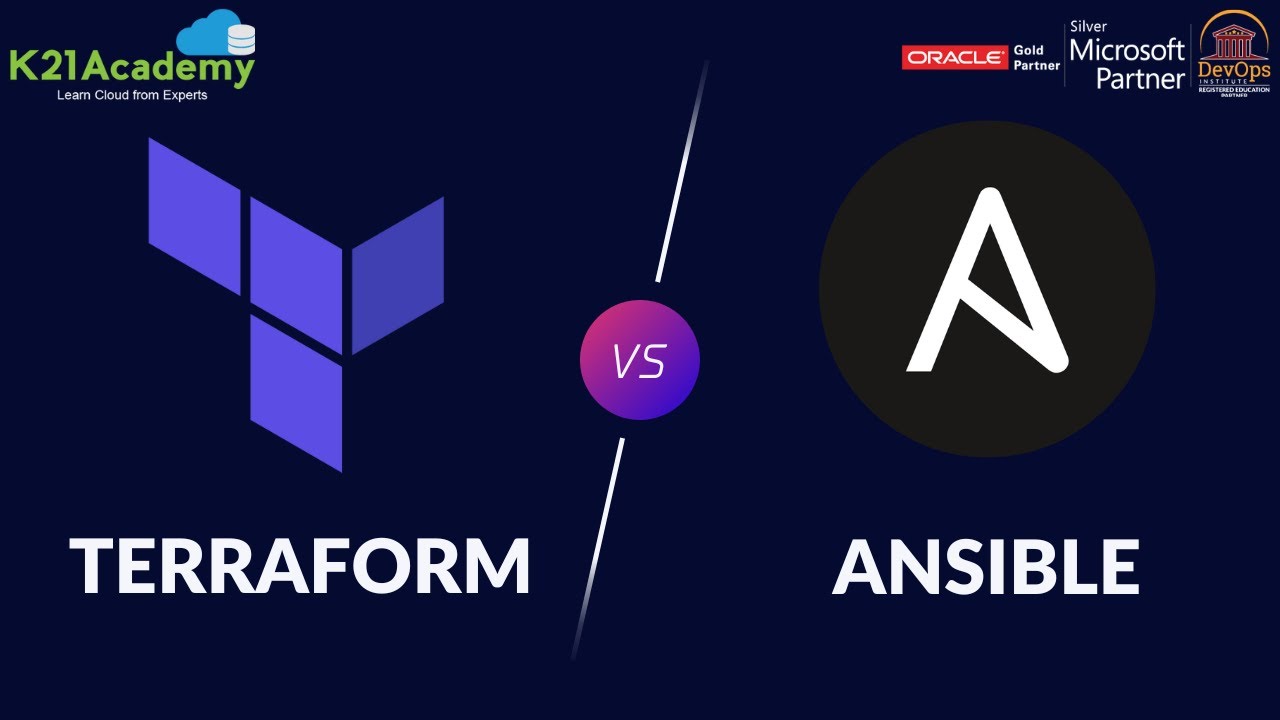
Article link: ansible combine two lists.
Learn more about the topic ansible combine two lists.
- Append list variable to another list in Ansible – Stack Overflow
- How to merge multiple lists in ansible
- How to combine two lists? – ansible – Server Fault
- Combine and Merge Lists… How? : r/ansible – Reddit
- community.general.lists_mergeby filter – Merge two or more …
- Ansible: Merge two lists
- How to append to lists in Ansible – Crisp’s Blog
- Ansible Combine or Merge a List using plus or zip – FreeKB
See more: blog https://nhanvietluanvan.com/luat-hoc