An Object Reference Is Required To Access Non-Static Field
In Java, object reference is a variable that points to an object in memory. It allows us to access and manipulate the data and behavior of the object. An object reference is necessary to interact with non-static fields, methods, or properties of an object.
Explaining the Distinction between Static and Non-static Fields
In Java, fields can be either static or non-static. Static fields belong to the class itself and are shared among all instances of the class. Non-static fields, on the other hand, are unique to each instance of the class and are not shared.
The Need for an Object Reference to Access Non-static Fields
When accessing non-static fields, methods, or properties of an object, an object reference is required. This is because non-static members are associated with individual instances of a class, and the object reference points to a specific instance.
Without an object reference, it is not possible to access the non-static members of an object. This is because non-static members do not exist independently and rely on the state of a particular instance.
How Object References are Created and Used in Java
Object references in Java are created by instantiating a class using the “new” keyword. For example, to create an object reference of the class “Person”, we would write:
Person person = new Person();
Here, “person” is the object reference that points to a new instance of the class “Person”. This object reference can then be used to access the non-static members of the “Person” class.
Examples of Non-static Fields and Their Access through Object References
Let’s consider an example where we have a class named “Car” with a non-static field called “color”. We can access this field through an object reference as follows:
Car car1 = new Car();
car1.color = “Red”;
In this example, we create an object reference “car1” of the class “Car” and assign the value “Red” to its “color” field. We are able to access the “color” field through the object reference.
Common Errors and Exceptions Related to Non-static Field Access
One common error when accessing non-static fields is the “Object reference not set to an instance of an object” error. This occurs when an object reference is used to access a non-static field, but the object reference has not been initialized or set to an instance of the object.
Another common error is the “An object reference is required for the non-static field, method, or property” error. This error typically occurs when trying to access a non-static member without using an object reference.
Best Practices for Managing Object References and Non-static Fields
When working with non-static fields in Java, it is essential to properly manage object references. Here are some best practices to consider:
1. Always initialize object references before using them to avoid null reference errors.
2. Use meaningful names for object references to improve code readability.
3. Limit the scope of object references to minimize potential bugs or confusion.
4. Update object references when necessary to ensure they point to the correct instances.
5. Be aware of memory management when creating and disposing of object references.
Exploring the Benefits and Limitations of Non-static Fields
Non-static fields offer several benefits in Java programming. They allow us to store unique data for each instance of a class and maintain the state of an object. Non-static fields also enable us to create dynamic and customizable objects.
However, non-static fields also have some limitations. They consume more memory compared to static fields as each instance has its own copy. Additionally, non-static fields require an object reference to access them, which adds an extra step in the code.
Frequently Asked Questions (FAQs)
Q: What is the difference between static and non-static fields in Java?
A: Static fields belong to the class itself and are shared among all instances, while non-static fields are unique to each instance of the class.
Q: Why do we need an object reference to access non-static fields?
A: Non-static fields are associated with individual instances of a class, and an object reference is needed to point to a specific instance and access its non-static members.
Q: What are the common errors related to non-static field access?
A: The “Object reference not set to an instance of an object” error and the “An object reference is required for the non-static field, method, or property” error are common errors related to non-static field access.
Q: What are some best practices for managing object references and non-static fields?
A: Best practices include initializing object references before use, using meaningful names, limiting scope, updating references when necessary, and managing memory.
Q: What are the benefits and limitations of non-static fields?
A: Non-static fields allow for unique data storage, maintaining object state, and creating dynamic objects. However, they consume more memory and require an object reference to access.
Additional Resources for Further Learning
1. Java Documentation: https://docs.oracle.com/javase/8/docs/
2. Java Object-Oriented Programming Tutorial: https://www.javatpoint.com/java-oops-concepts
3. Java – Static and Non-Static Variables: https://www.geeksforgeeks.org/java-static-and-non-static-variable-instantiation-issues/
Cs0120: An Object Reference Is Required For The Non-Static Field, Method, Or Property… Easy Fix
Can Object Reference Is Required For A Non Static Field?
In Java, the concept of static and non-static fields is essential to understand the behavior of variables within a class. A static field belongs to the class itself, while a non-static field is associated with individual instances or objects of the class. When it comes to accessing and modifying non-static fields, a crucial consideration is whether an object reference is required.
To delve deeper into this topic, let’s first clarify the difference between static and non-static fields. Static fields are shared among all instances of a class, meaning they have only one copy that is accessible to all objects. On the other hand, each object or instance of a class has its own unique copy of non-static fields. This distinction plays a significant role in determining whether an object reference is necessary to interact with a non-static field.
When accessing a non-static field, an object reference is mandatory. Since non-static fields are specific to individual objects, they cannot be accessed directly through the class name. Instead, they require an instance of the class to provide the necessary reference to access and modify the field. This is because non-static fields are not stored in a common memory location like static fields; they are associated with the state of an instance.
To illustrate this concept, consider a simple Java class called “Person” with a non-static field called “name”:
“`java
public class Person {
public String name;
}
“`
To access and set the value of the “name” field, we need an object reference. Here’s an example:
“`java
Person person = new Person();
person.name = “John”;
“`
In the above code, we create an object of the “Person” class using the “new” keyword. By using the “.name” syntax, we access and modify the value of the non-static field “name” through the object reference “person”.
Without an object reference, attempting to access a non-static field will result in a compilation error. This error occurs because the field requires an instance of the class to be accessed, and without a reference, the Java compiler cannot determine which object’s field is being referred to.
Frequently Asked Questions (FAQs):
Q: Can a static field be accessed without an object reference?
A: Yes, a static field can be accessed without an object reference. Since static fields are associated with the class itself, they can be accessed directly using the class name. For example, in the “Person” class mentioned earlier, if we had a static field called “counter”, we could access it as follows: “Person.counter”.
Q: What happens if we try to access a non-static field without an object reference?
A: A compilation error will occur if we attempt to access a non-static field without an object reference. The Java compiler requires an instance of the class to provide the necessary reference to access and modify non-static fields.
Q: Can static and non-static fields have the same name?
A: Yes, static and non-static fields can have the same name without causing any conflicts. Since they are accessed differently (static fields through the class name and non-static fields through object references), there is no ambiguity in accessing these fields.
Q: Are non-static fields shared among objects of the same class?
A: No, non-static fields are unique to each object or instance of a class. Each object will have its own copy of non-static fields, and modifying them in one object will not affect the values in other objects.
Q: Can non-static fields be accessed from static methods?
A: Non-static fields cannot be accessed directly from static methods. This is because static methods do not have an associated instance of the class to access or modify non-static fields. To access non-static fields within a static method, an object reference must be passed as a parameter to the method.
In conclusion, when working with non-static fields in Java, it is crucial to understand that an object reference is required to access and modify them. Non-static fields are specific to individual objects and cannot be accessed through the class name alone. By utilizing object references, we can effectively interact with non-static fields and manipulate the state of individual instances.
How To Access Non Static Field In Static Method C#?
In C#, a static method is one that belongs to the class rather than to any specific instance of the class. This means that the static method can be called without creating an instance of the class. However, accessing non-static fields within a static method can be a challenge since non-static fields are specific to each instance of the class. In this article, we will explore different ways to access non-static fields within a static method in C#, providing you with a comprehensive guide on this topic.
Understanding Static Methods and Non-static Fields
Before delving into the ways to access non-static fields in a static method, it is imperative to understand the difference between these two concepts.
Static methods are commonly used to perform operations that do not require any specific object instance, such as mathematical calculations, utility methods, or global operations. These methods are associated with the class itself rather than with any particular instance of the class, allowing them to be called directly using the class name.
On the other hand, non-static fields are specific to each instance of the class. They are part of the object’s state and contain data that varies across different instances. Non-static fields cannot be accessed directly within a static method since they are not associated with the class itself but rather with the instances of the class.
Ways to Access Non-static Fields in Static Methods
1. Pass Object Reference as a Parameter
One way to access non-static fields within a static method is by passing the object reference as a parameter. By passing the reference of the object to the static method, you can access the non-static fields of that particular instance.
Here’s an example that demonstrates this approach:
“`csharp
class MyClass
{
public int nonStaticField;
public static void AccessNonStaticField(MyClass obj)
{
Console.WriteLine(obj.nonStaticField);
}
}
static void Main(string[] args)
{
MyClass instance = new MyClass();
instance.nonStaticField = 10;
MyClass.AccessNonStaticField(instance);
}
“`
In the above code, the static method `AccessNonStaticField()` accepts an instance of the class `MyClass` as a parameter. By accessing the `nonStaticField` within the method, we can print its value.
2. Create an Instance within the Static Method
Another way to work with non-static fields in a static method is by creating an instance of the class within the static method. This allows you to access the non-static fields through the instance.
Here’s an example illustrating this approach:
“`csharp
class MyClass
{
public int nonStaticField;
public static void AccessNonStaticField()
{
MyClass obj = new MyClass();
obj.nonStaticField = 10;
Console.WriteLine(obj.nonStaticField);
}
}
static void Main(string[] args)
{
MyClass.AccessNonStaticField();
}
“`
In the above code, the static method `AccessNonStaticField()` creates an instance of `MyClass` and assigns a value to its `nonStaticField`. We can then access the `nonStaticField` and print its value.
FAQs
Q1: Why can’t I directly access non-static fields in a static method in C#?
A1: Non-static fields are specific to each instance of the class, while static methods are associated with the class itself. Since non-static fields are not inherently part of the class, you cannot directly access them in a static method.
Q2: Can static methods access static variables?
A2: Yes, static methods can access static variables as they both belong to the class itself. Static variables are not specific to any instance and can be accessed directly within a static method.
Q3: Why would I need to access non-static fields in a static method?
A3: There may be situations where you need to perform operations on non-static fields within a static method. For example, you might have a static utility method to calculate statistics based on data stored in non-static fields.
Q4: Are there any alternatives to accessing non-static fields in a static method?
A4: If the non-static field is required by the static method frequently, it might be worth considering converting the non-static field to a static field. However, this approach should be carefully evaluated as it can impact the design and behavior of your application.
In conclusion, accessing non-static fields within a static method in C# can be achieved by passing the object reference as a parameter or by creating an instance of the class within the static method. It is essential to understand the differences between static methods and non-static fields to effectively access and manipulate data in your applications.
Keywords searched by users: an object reference is required to access non-static field An object reference is required for the non static field, method, or property, Lỗi An object reference is required for the non static field, method, or property, an object reference is required for the non-static field method or property c#, An object reference is required to access non static member, An object reference is required for the non static field, method, or property ‘HttpContext session, An object reference is required for the non static field method or property in asp net, An object reference is required for the non static field method or property Unity, Object reference not set to an instance of an object
Categories: Top 58 An Object Reference Is Required To Access Non-Static Field
See more here: nhanvietluanvan.com
An Object Reference Is Required For The Non Static Field, Method, Or Property
In the world of programming, encountering errors is not an uncommon occurrence. From syntax mistakes to logical errors, developers are constantly faced with challenges that require troubleshooting and debugging. One such error message that programmers often encounter is the infamous “An object reference is required for the non-static field, method, or property” error.
This error is commonly seen in object-oriented programming languages such as Java, C#, and C++. It occurs when a non-static member of a class is accessed or called without an instance of that class. To understand this error better, let’s delve into the concept of static and non-static members in programming.
Static vs Non-Static Members
In programming, members of a class can be categorized as static or non-static. Static members are associated with the class itself rather than individual objects or instances of that class. They can be accessed or called without creating an instance of the class. On the other hand, non-static members are specific to each instance of the class. They require an object reference to be accessed or called.
Understanding the Error Message
The error message “An object reference is required for the non-static field, method, or property” is thrown when a non-static member of a class is mistakenly accessed without an object reference. This error can occur in several scenarios, such as:
1. Attempting to access an instance variable without creating an instance of the class.
2. Trying to call a non-static method without an object reference.
3. Accessing a non-static property without an object reference.
Such mistakes often happen accidentally when developers overlook the need for an object reference while working with non-static members. The error message serves as a reminder to provide the necessary object reference.
Common Scenarios and Solutions
Let’s now explore some common scenarios where this error might occur and the corresponding solutions.
1. Accessing an instance variable without an object reference:
Consider the following code snippet:
“`java
public class MyClass {
private int count = 0;
public static void main(String[] args) {
System.out.println(count); // Error: An object reference is required
}
}
“`
In this example, the instance variable `count` is accessed without a corresponding object reference. To resolve this error, we need to create an instance of the `MyClass` and use that object reference to access the instance variable:
“`java
public class MyClass {
private int count = 0;
public static void main(String[] args) {
MyClass obj = new MyClass();
System.out.println(obj.count); // Output: 0
}
}
“`
2. Calling a non-static method without an object reference:
Consider the following code snippet:
“`csharp
public class MyClass {
public void display() {
Console.WriteLine(“Hello!”);
}
public static void main(String[] args) {
display(); // Error: An object reference is required
}
}
“`
In this example, the non-static method `display()` is called without a corresponding object reference. To correct this error, we need to create an instance of the `MyClass` and use that object reference to call the method:
“`csharp
public class MyClass {
public void display() {
Console.WriteLine(“Hello!”);
}
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.display(); // Output: Hello!
}
}
“`
3. Accessing a non-static property without an object reference:
Consider the following code snippet:
“`cpp
class MyClass {
public:
int age = 25;
};
int main() {
cout << age; // Error: An object reference is required
return 0;
}
```
In this example, the non-static property `age` is accessed without a corresponding object reference. To fix this error, we need to create an instance of the `MyClass` and use that object reference to access the property:
```cpp
class MyClass {
public:
int age = 25;
};
int main() {
MyClass obj;
cout << obj.age; // Output: 25
return 0;
}
```
FAQs
Q1. Can this error occur in other programming languages apart from Java, C#, and C++?
Yes, this error message can occur in any object-oriented programming language that supports both static and non-static members.
Q2. What is the significance of using static and non-static members in programming?
Static members are useful when you want to maintain shared variables or methods across all instances of a class. Non-static members, on the other hand, are unique to each instance of the class and allow individual objects to have their own set of variables and methods.
Q3. How can I avoid encountering this error?
Make sure to always provide the required object reference when accessing non-static members. Also, pay attention to the scope and visibility of your variables and methods.
Q4. Are there any tools available to automatically detect and fix this error?
Most modern integrated development environments (IDEs) provide tools that help identify such errors during coding and offer suggestions to fix them.
In conclusion, the error "An object reference is required for the non-static field, method, or property" is a common mistake that developers encounter while working with non-static members. By providing the necessary object reference, understanding the nature of static and non-static members, and practicing careful coding, this error can be easily avoided and resolved.
Lỗi An Object Reference Is Required For The Non Static Field, Method, Or Property
Understanding the Object Reference Error
When programming in an object-oriented language, we create classes as blueprints for creating objects. These objects have their own attributes (fields) and behaviors (methods), which can be accessed through instantiated objects. However, if we try to access non-static members directly from the class itself, this error will occur because non-static members belong to an instance of the class and require an object reference to access them.
For example, consider a class named “Car” with a non-static field called “color.” To access this field, we would typically create an instance of the Car class, such as “Car myCar = new Car();” and then access its color like this: “string myCarColor = myCar.color;”. Here, “myCar” is the object reference that provides access to the non-static field “color”.
Common Causes of the Object Reference Error
1. Accessing non-static members from a static context: Static methods or properties can be accessed without creating instances of a class. However, when trying to access non-static members from these static methods or properties, the error will occur. To resolve this, create an instance of the class or change the member to static if appropriate.
2. Mistakenly using the class name instead of an object reference: If we use the class name directly to access a non-static member instead of providing an object reference, this error will be thrown. Ensure that an object reference is used to access the non-static member.
3. Omitting the creation of an instance: Sometimes, developers forget to create an instance of a class and directly try to access its non-static members. A simple solution to this is to create an instance of the class before accessing the non-static members.
4. Mismatch between method signature and calling code: If the calling code or object reference does not meet the exact method signature required, this error can occur. Ensure that the calling code matches the method signature, including parameter types and return types.
5. Using non-static members in a static constructor: Static constructors don’t have an associated object instance, so we can’t access non-static members within them. Avoid using non-static members in static constructors or provide default values to static fields through static constructor arguments.
Frequently Asked Questions (FAQs) about the Object Reference Error:
Q1: How can I fix the “Object reference error” in C#?
A1: To fix this error, ensure that you are using an object reference to access non-static members. Create an instance of the class or change the member to static if appropriate.
Q2: Why do I get this error when accessing non-static members from a static method?
A2: Static methods or properties can be accessed without creating instances of a class. However, when accessing non-static members from static methods, this error will occur. To resolve, either create an instance of the class or change the member to static if appropriate.
Q3: How can I avoid this error when using non-static members in a static constructor?
A3: Static constructors don’t have an associated object instance, so accessing non-static members within them is not allowed. Avoid using non-static members in static constructors or provide default values to static fields through static constructor arguments.
Q4: Can I access non-static members directly from the class itself?
A4: No, you cannot access non-static members directly from the class itself. Non-static members require an object reference, which provides access to the instance-specific data and behavior of the class.
Q5: Is the Object Reference Error specific to any particular programming language?
A5: The error message “An object reference is required for the non-static field, method, or property” is commonly encountered in object-oriented programming languages like C#, Java, and similar languages which follow the same principles of accessing member variables and methods.
In conclusion, the “Object reference error” occurs when we try to access non-static members without creating an instance of a class or providing an object reference. By understanding the causes of this error and following the appropriate coding practices, developers can avoid and fix this error effectively.
Images related to the topic an object reference is required to access non-static field
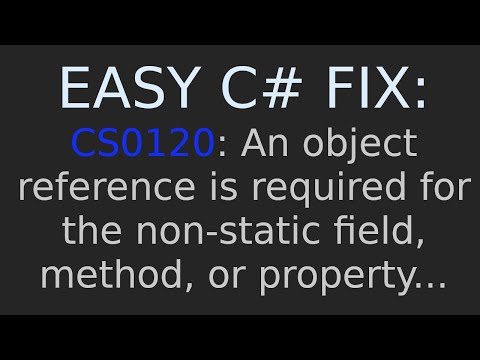
Found 42 images related to an object reference is required to access non-static field theme

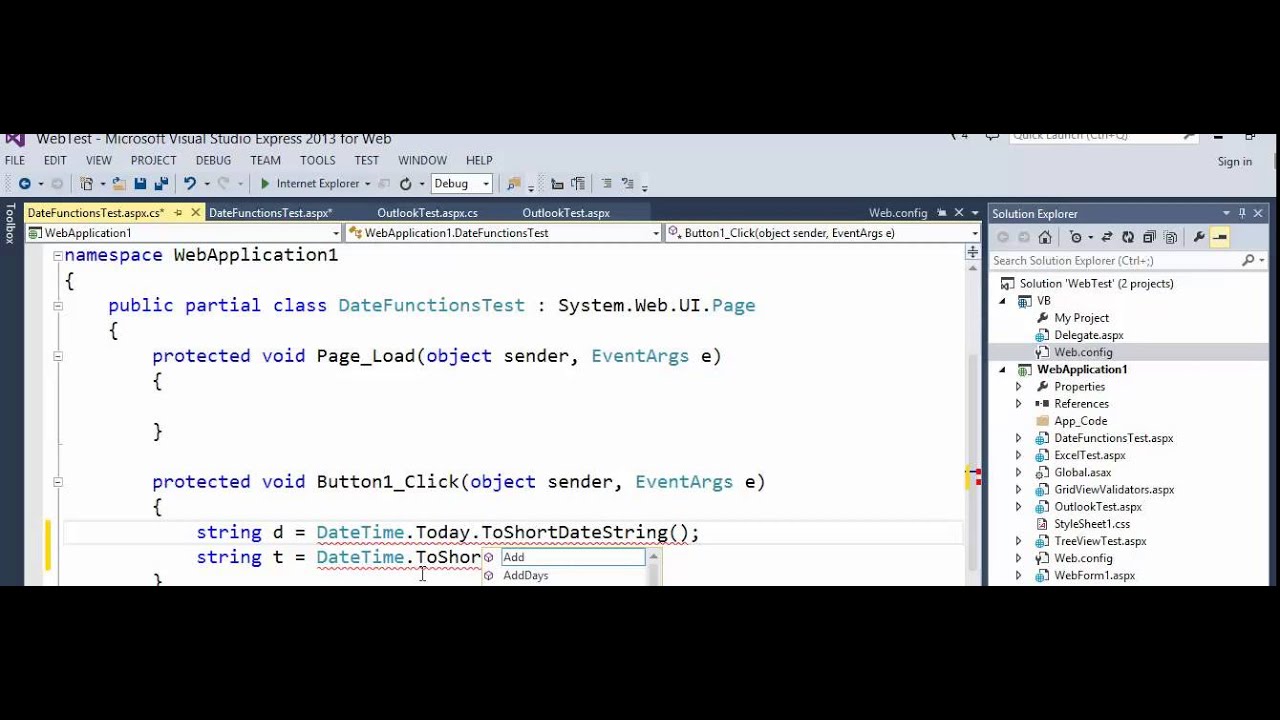



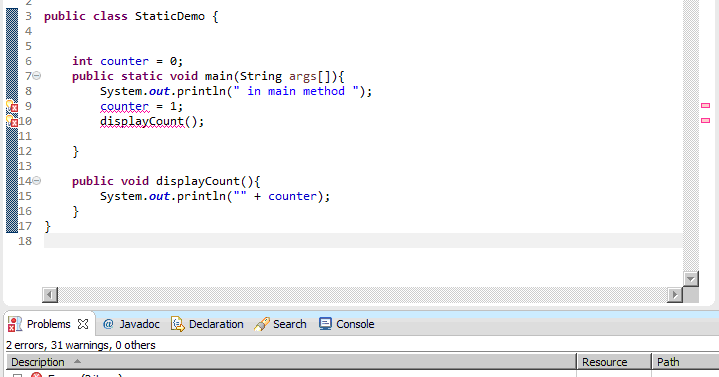

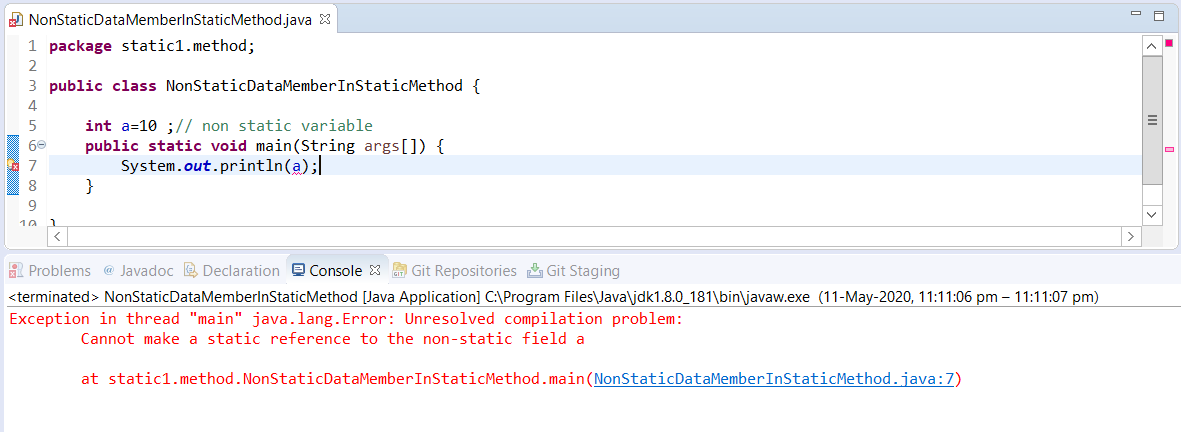
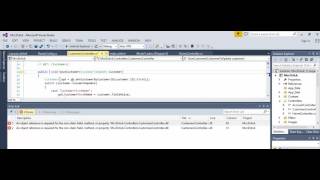





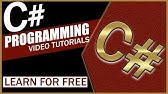
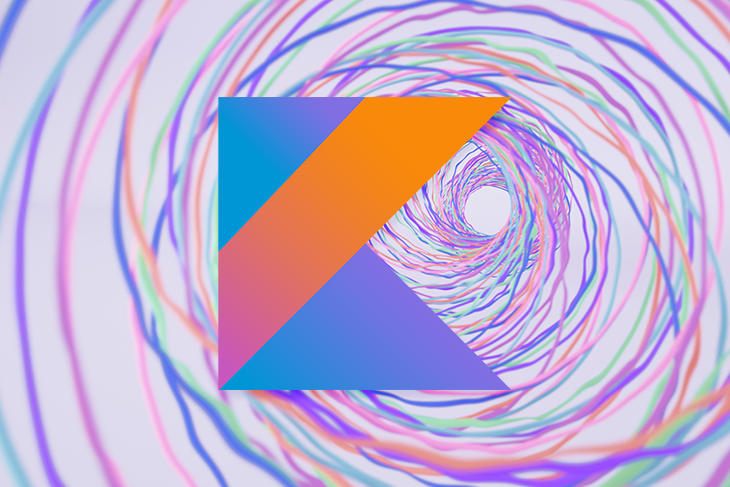

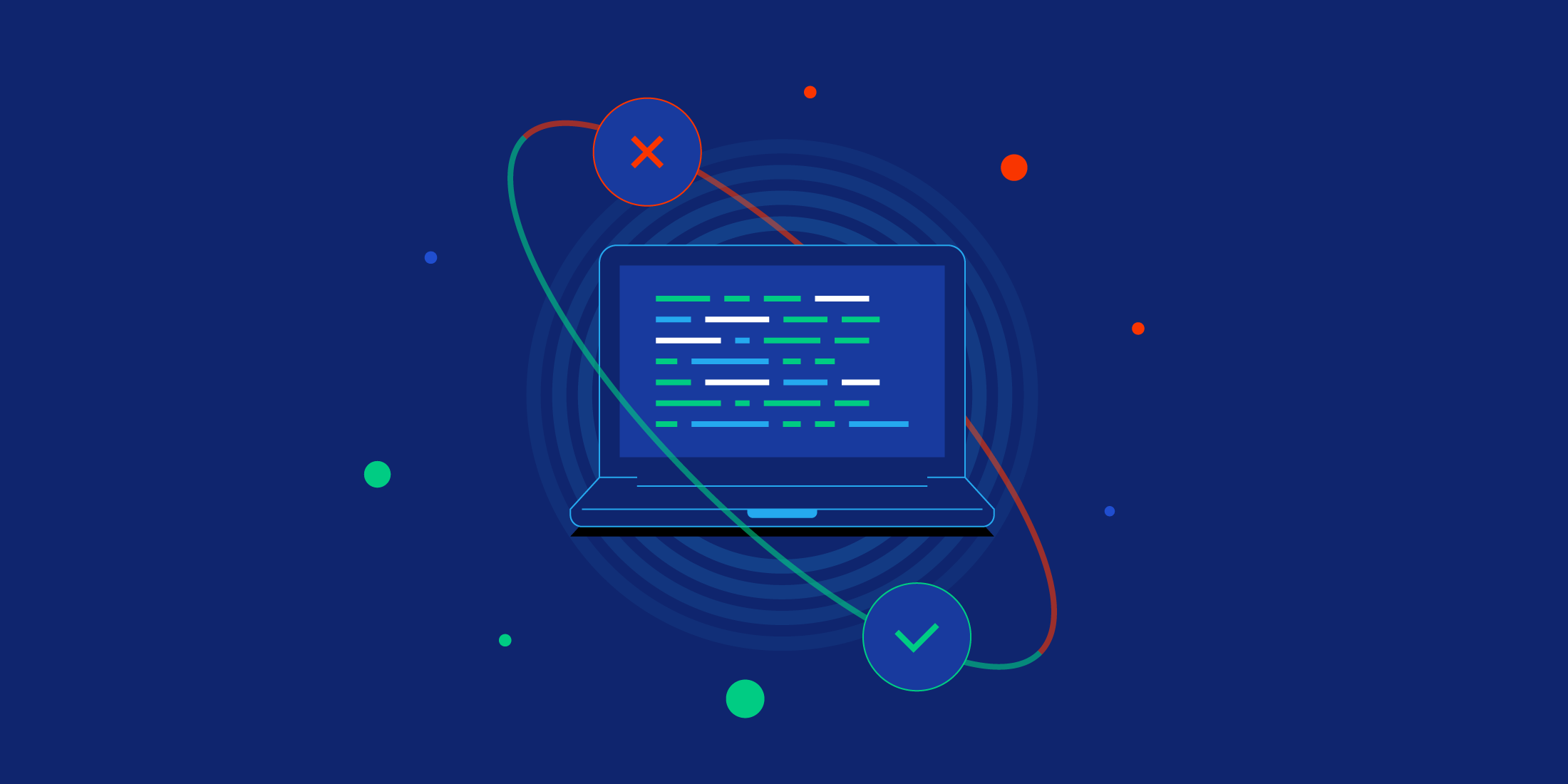
Article link: an object reference is required to access non-static field.
Learn more about the topic an object reference is required to access non-static field.
- Error: “an object reference is required for the non-static field …
- C# Error: How to Resolve an object reference is required for …
- Compiler Error CS0120 | Microsoft Learn
- C# Error: How to Resolve an object reference is required for …
- Static vs Non-Static Members in C# with Examples – Dot Net Tutorials
- Static Variable in Java with Examples – Scaler Topics
- Learn C#: References Cheatsheet – Codecademy
- error An object reference is required for the non-static field …
- Error CS0120 An object reference is required for the non-static …
- An object reference is required to access a non-static field
- How to resolve ‘Error an object reference is required … – Quora