Add To List C#
Lists are a fundamental data structure in programming, and they play a crucial role in C# programming. A list is a collection of elements of a specific datatype, and it provides various operations for manipulating and managing the elements stored within it. In C#, the List class is part of the System.Collections.Generic namespace and provides a versatile and efficient way of working with collections of objects.
Creating a List in C#
To start working with a list in C#, you first need to define the List datatype and then instantiate a List object. The datatype can be any valid C# datatype or a custom object. Here’s how you can create a list of integers:
“`
List
“`
In this example, we create an empty list called “numbers” that will store integers. Once you have created a list, you can start adding elements to it.
Adding Elements to the List
To add elements to a list, you can use the Add method, which is available with the List class. The Add method appends an element to the end of the list. Here’s an example:
“`
numbers.Add(10);
numbers.Add(20);
numbers.Add(30);
“`
In this example, we add three integers to the “numbers” list. The list now contains the elements 10, 20, and 30.
Accessing Elements in the List
You can access elements in a list using their indexes. The index starts from 0, which means the first element in the list is at index 0, the second element is at index 1, and so on. To access an element, you simply use the index as shown in the example below:
“`
Console.WriteLine(numbers[1]); // Output: 20
“`
In this example, we access the element at index 1, which is 20, and display it on the console.
Modifying Elements in the List
Lists support modifying elements at specific positions by assigning a new value to the indexed element. Here’s an example:
“`
numbers[0] = 5;
Console.WriteLine(numbers[0]); // Output: 5
“`
In this example, we modify the element at index 0 and assign a new value of 5. We then access the modified element and display it on the console.
Common List Operations in C#
In addition to adding, accessing, and modifying elements, lists provide several other common operations, including sorting, searching, removing, and clearing. Let’s explore these operations in more detail:
Sorting Elements in a List
The Sort method allows you to sort the elements in a list in ascending order. Here’s an example:
“`
numbers.Sort();
“`
In this example, we sort the “numbers” list in ascending order. After sorting, the list will contain the elements 5, 20, and 30.
Searching for Elements in a List
To search for elements in a list, you can use the Find method. The Find method takes a condition as a parameter and returns the first element that satisfies the condition. Here’s an example:
“`
int number = numbers.Find(x => x > 10);
“`
In this example, we search for the first element in the “numbers” list that is greater than 10. The Find method returns the element 20.
Removing Elements from a List
To remove elements from a list, you can use the Remove method. The Remove method removes the first occurrence of a specified element from the list. Here’s an example:
“`
numbers.Remove(20);
“`
In this example, we remove the first occurrence of the element 20 from the “numbers” list. After removing, the list will contain the elements 5 and 30.
Clearing the Entire List
If you want to remove all elements from a list, you can use the Clear method. The Clear method removes all elements from the list, leaving it empty. Here’s an example:
“`
numbers.Clear();
“`
In this example, we clear all elements from the “numbers” list. After clearing, the list will be empty.
Checking the Size of a List
To obtain the number of elements in a list, you can use the Count property. The Count property returns the number of elements in the list. Here’s an example:
“`
int size = numbers.Count;
“`
In this example, we store the number of elements in the “numbers” list in the variable “size”.
List Methods and Properties in C#
In addition to the common list operations, the List class provides a wide range of useful methods and properties for manipulating lists. Here are some of the frequently used ones:
Count Property: Obtaining the number of elements in a List
The Count property returns the number of elements in a list. It is commonly used to check the size of a list or to iterate over all elements of a list using a loop.
“`
int count = numbers.Count;
“`
Contains Method: Checking if a specific element exists in the List
The Contains method checks if a specific element exists in the list. It returns a Boolean value indicating whether the element is present.
“`
bool contains = numbers.Contains(20);
“`
Find Method: Searching for an element using a specific condition
The Find method searches for an element in the list that satisfies a specific condition. It returns the first element that meets the condition.
“`
int evenNumber = numbers.Find(x => x % 2 == 0);
“`
Insert Method: Adding an element at a specific position in the List
The Insert method allows you to add an element at a specific position in the list. It takes the index and the element as parameters.
“`
numbers.Insert(1, 15);
“`
Remove Method: Removing the first occurrence of a specific element in the List
The Remove method removes the first occurrence of a specific element from the list. It returns a Boolean value indicating whether the removal was successful.
“`
bool removed = numbers.Remove(20);
“`
Working with Generic Lists in C#
Generic Lists in C# provide the flexibility to work with different data types in a single list. You can create lists of various datatypes such as integers, strings, custom objects, and more. Here are some aspects to consider when working with generic lists:
Using Generic Lists with Different Data Types
You can create generic lists with different data types by specifying the datatype in the angle brackets while defining the List. For example:
“`
List
List
“`
Creating a List of Custom Objects
You can create a list of custom objects by defining a class and instantiating a list with that class as its datatype. Here’s an example:
“`
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
List
“`
In this example, we create a custom class called “Person” with properties for name and age. We then create a list of Person objects.
Overriding Equality and Comparison Operators for Custom Objects in a List
When working with custom objects in a list, you may need to override the equality and comparison operators to define how the objects are compared and sorted. This allows you to customize the behavior of the list for your specific object type.
Sorting and Searching in Generic Lists
Generic lists support sorting and searching using the Sort method and the Find method, as mentioned earlier. These methods work with generic lists the same way they do with lists of built-in data types.
Limitations and Considerations of Using Generic Lists
While generic lists offer flexibility and convenience, there are some limitations to be aware of. Generic lists cannot store value types and are not suitable for storing large amounts of data due to performance considerations. In such cases, alternate data structures like arrays or specialized collections may be more appropriate.
Benefits and Advantages of Lists in C#
Lists provide numerous benefits and advantages when working with collections of data in C#. Here are some key advantages:
Dynamic Resizing
Lists automatically manage underlying memory allocation, enabling dynamic resizing as elements are added or removed. This eliminates the need for manual memory management and provides convenience in handling variable-sized collections.
Efficient Element Access
Elements in a list can be accessed by their index, allowing for quick retrieval of elements in constant time. This makes lists efficient for applications that involve frequent or random access to elements.
Versatility
Lists are suitable for various data storage and manipulation scenarios, making them highly versatile. They can store elements of different datatypes and can be easily modified to accommodate changing requirements.
Flexibility
Lists provide the flexibility to add, remove, and modify elements without fixed length constraints. This makes them ideal for scenarios where the size of the collection may change dynamically.
Enhanced Functionality
Lists come with a wide range of built-in methods and properties, which simplify list manipulation tasks. This includes operations such as sorting, searching, removing, and more, saving developers time and effort.
List vs. Array in C#
Lists and arrays are both commonly used data structures in C#. While both provide ways to store collections of elements, they have some important differences. Here are a few key distinctions:
Usage and Functionality
Lists are dynamic collections that can grow or shrink in size, while arrays have a fixed length determined at the time of declaration. Lists provide additional operations and methods for list manipulation, such as sorting or searching, which are not available in arrays.
Advantages of Using Lists over Arrays
Lists offer advantages over arrays in scenarios where the size of the collection is not known in advance or may change dynamically. Lists also provide higher-level functionalities that simplify common list operations, making them easier to work with.
Performance Considerations
Arrays generally offer better performance when it comes to memory allocation and element access. Since arrays have a fixed size, they have a more compact memory representation compared to lists. Array element access is also slightly faster than accessing elements in a list using their index.
Trade-offs between Memory Efficiency and Dynamic Operations
Arrays are more memory-efficient compared to lists since they do not require additional metadata for resizing and maintaining the collection. However, lists provide dynamic operations such as resizing and dynamic memory allocation, which can be convenient in certain situations.
Converting Lists to Arrays and Vice Versa
C# provides methods and properties to convert lists to arrays and vice versa. The ToArray method can be used to convert a list to an array, and the Array.ToList method can be used to convert an array to a list. These conversions can be useful when you need to perform specific operations or pass the data to functions that accept arrays or lists.
In conclusion, lists play a vital role in C# programming, offering a powerful and flexible way to work with collections of objects. They provide numerous methods and properties for common list operations, along with benefits such as dynamic resizing, efficient element access, and enhanced functionality. While lists have advantages over arrays in certain scenarios, it is important to consider the specific requirements and constraints of your application when choosing between them.
FAQs
Q: Can I add different data types to a single List in C#?
A: No, a single List can only store elements of a specific datatype. To store different data types in a List, you can use a list of objects or consider using a generic List with a base class or interface.
Q: What happens if I access an element in a List using an invalid index?
A: If you try to access an element in a List using an invalid index (e.g., an index that is out of range), it will throw an IndexOutOfRangeException.
Q: How can I check if a List is empty?
A: You can check if a List is empty by using the Count property and checking if it is equal to zero.
Q: Can I sort a List in descending order?
A: Yes, you can sort a List in descending order by using the Reverse method after sorting it in ascending order.
Q: What is the difference between List
A: The Remove method removes the first occurrence of a specific element from the List, while the RemoveAt method removes an element at a specified index in the List.
Q: Can I use Lists with non-generic types in C#?
A: Yes, C# provides non-generic List types such as ArrayList and ListDictionary, but it is recommended to use generic Lists for type safety and performance benefits.
Q: Can I nest lists within lists in C#?
A: Yes, you can nest lists within lists in C#. This allows you to create multi-dimensional lists or lists of lists, which can be useful for handling structured data.
Q: Are Lists in C# thread-safe?
A: No, Lists in C# are not thread-safe by default. If you need to access a List from multiple threads simultaneously, you should use synchronization techniques such as locks or concurrent collections.
Adding Elements To A Linked List
How To Add Element To A List In C?
Lists are fundamental data structures that store a collection of elements in a sequential manner. In the C programming language, there are several ways to add an element to a list, depending on the specific implementation. In this article, we will explore different methods to accomplish this task and provide a comprehensive guide on adding elements to lists in C.
1. Array Implementation
One of the simplest ways to create a list in C is by using an array. To add an element to an array-based list, you need to follow these steps:
a. Declare an array with a sufficient size to hold the elements.
b. Initialize the array with the desired initial elements.
c. Determine the index of the position where you want to add the new element.
d. Shift the elements from the chosen index to the right to create space for the new element.
e. Assign the new element to the chosen index.
Here is a code snippet that demonstrates this process:
“`c
#include
#define MAX_SIZE 100
int main() {
int list[MAX_SIZE] = {1, 2, 3, 4, 5};
int size = 5;
int index = 2; // Add element at index 2
int newElement = 10;
// Shift elements to the right
for (int i = size; i > index; i–) {
list[i] = list[i-1];
}
// Assign the new element
list[index] = newElement;
size++; // Increment the size of the list
printf(“List after adding an element: “);
for (int i = 0; i < size; i++) {
printf("%d ", list[i]);
}
return 0;
}
```
2. Linked List Implementation
Linked lists offer a dynamic method for creating lists in C. In this implementation, elements are stored in individual nodes, and each node contains a pointer to the next node. To add an element to a linked list, you can follow the following steps:
a. Define a structure to represent a node.
b. Create an empty linked list by assigning NULL to the head pointer.
c. Allocate memory for a new node using the malloc() function.
d. Assign the new element to the data field of the new node.
e. Set the next pointer of the new node to point to the next node in the list.
f. Update the last node's next pointer to point to the new node.
g. Update the head pointer if necessary.
Here is an example implementation of adding an element to a linked list:
```c
#include
#include
typedef struct Node {
int data;
struct Node* next;
} Node;
void addNode(Node** head, int newData) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = newData;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
int main() {
Node* head = NULL;
// Initial list: 1->2->3->4->5
for (int i = 1; i <= 5; i++) {
addNode(&head, i);
}
// Add new element at the end
addNode(&head, 10);
printf("List after adding an element: ");
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
return 0;
}
“`
FAQs:
Q1: Can I add an element to a list in C without knowing the index?
A1: Yes, you can add an element to the end of an array-based list by simply assigning the new element to the next available index. In the case of a linked list, you can add an element without knowing the index by traversing the list until you find the last node and then appending the new element.
Q2: How do I add an element to the beginning of a linked list?
A2: To add an element to the beginning of a linked list, you need to follow these steps:
a. Create a new node and assign the new element.
b. Set the next pointer of the new node to the current head.
c. Update the head pointer to point to the new node.
Q3: Is it possible to add an element to a list using a specific index?
A3: Yes, it is possible to add an element to a list using a specific index. For array-based lists, you need to shift the elements from the chosen index to the right before assigning the new element. Similarly, for linked lists, you need to traverse the list until you reach the desired index and then rearrange the pointers accordingly.
Q4: Can I add elements of different types to a list in C?
A4: In C, lists are typically designed to store elements of the same type. If you want to add different types of elements to a list, you can define a structure that contains multiple data fields representing different types and add nodes of this structure type to the list.
In conclusion, adding an element to a list in C can be accomplished using different approaches. Array-based lists involve shifting elements and assigning the new element to the desired index, while linked lists require creating new nodes and updating pointers accordingly. By understanding these techniques, you can easily add elements to lists in C and manipulate the data as needed.
Can You Append To A List In C?
In the C programming language, manipulating arrays is a common task. Arrays are used to store a fixed number of elements of the same data type in a sequential manner. However, arrays have a fixed size, and adding or removing elements from an array is not as straightforward as it may seem.
To overcome this limitation and allow dynamic resizing of arrays, the concept of lists or linked lists comes into play. A list is a data structure that dynamically grows or shrinks as elements are added or removed. It offers more flexibility compared to arrays but requires more memory due to the additional overhead of maintaining the linked structure.
Appending, or adding an element to the end of a list, is a fundamental operation in list manipulation. Append operations ensure that new elements are properly inserted at the end of a list, maintaining its integrity and order.
In C, you can implement a list using either an array-based approach or a linked list approach. Let’s explore both methods and understand how appending works in each case.
1. Array-Based Approach
In the array-based approach, a list is implemented using an array, where elements are stored in consecutive memory locations. To append an element to the end of the list, you need to consider two scenarios:
a) If the list has available space, you can directly add the new element at the next index position.
b) If the list is full, you need to allocate a new larger array, copy the existing elements to the new array, and then add the new element.
Here’s an example code snippet using an array-based approach to append an element to a list in C:
“`c
#define MAX_SIZE 100
int main() {
int list[MAX_SIZE] = {1, 2, 3, 4, 5};
int element = 6; // Element to append
int size = 5; // Current size of the list
if (size < MAX_SIZE) {
list[size] = element;
size++;
printf("Element appended successfully!\n");
} else {
printf("List is full. Unable to append element.\n");
}
return 0;
}
```
2. Linked List Approach
In the linked list approach, each element of the list is stored in a separate node. Each node contains the data element and a pointer to the next node in the list. To append an element to the end of a linked list, you need to follow these steps:
a) Create a new node with the data element to be appended.
b) Traverse the list until you reach the last node.
c) Set the next pointer of the last node to point to the new node.
d) Update the new node's next pointer to NULL, indicating the end of the list.
Here's an example code snippet using a linked list approach to append an element to a list in C:
```c
#include
#include
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
void appendToList(ListNode** head, int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
ListNode* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
printf(“Element appended successfully!\n”);
}
int main() {
ListNode* head = NULL;
int element = 6; // Element to append
appendToList(&head, element);
return 0;
}
“`
FAQs:
Q1. Can I append elements to an array without using a list in C?
A1. No, arrays in C have a fixed size and cannot be directly extended. You will need to allocate a new array and copy the elements if you want to simulate appending behavior.
Q2. What is the advantage of using a linked list for appending elements?
A2. Linked lists provide dynamic resizing, allowing efficient appending of elements. They do not require reallocating memory and copying elements like arrays.
Q3. How does appending elements in a linked list differ from appending elements in an array?
A3. Appending elements in a linked list involves creating a new node, updating the pointers, and connecting it to the last node. In an array, appending requires checking if the list has available space or reallocating memory and copying elements.
Q4. Are there any limitations to appending elements in a linked list?
A4. Linked lists have a higher memory overhead due to the extra pointers required for maintaining the linked structure. Additionally, accessing elements in a linked list is less efficient compared to arrays due to the lack of direct indexing.
In conclusion, arrays have a fixed size and do not support direct appending of elements. However, both array-based and linked list approaches can be used in C to achieve appending behavior. The choice depends on the specific requirements and constraints of your program.
Keywords searched by users: add to list c# add to list c#, c add to array, list in c, Add object to List c#, dynamic list in c, List add List C#, list of lists in c, Add List C#
Categories: Top 44 Add To List C#
See more here: nhanvietluanvan.com
Add To List C#
Introduction:
In the realm of programming, efficiency is a crucial aspect that developers constantly strive for. An essential tool in achieving efficiency is the ‘Add to List’ feature in C# programming language. This powerful functionality allows developers to efficiently manage collections of data, simplifying processes and enhancing the overall performance of applications. In this article, we will delve into the intricacies of C#’s ‘Add to List’ feature, exploring its benefits, implementation techniques, and providing a comprehensive FAQ section to address common queries.
I. Understanding ‘Add to List’ Feature:
The ‘Add to List’ feature in C# provides a convenient and efficient way to store data as elements in a list collection. It allows for dynamic addition of elements, ensuring a flexible and scalable solution. As a developer, working with lists can significantly simplify code maintenance and increase productivity.
1. Benefits of ‘Add to List’ feature:
a. Flexibility: The ‘Add to List’ feature provides developers with the ability to handle collections that can dynamically grow or shrink as per requirements. This flexibility facilitates easier data manipulation and processing.
b. Improved Performance: List collections enable optimized data retrieval and insertion operations, resulting in faster and more efficient algorithms when performing searches, sorting, or filtering operations.
c. Enhanced Maintainability: By utilizing ‘Add to List’ functionality, developers can easily manage and manipulate data, simplifying code structure and improving code readability. This, in turn, enhances the maintainability of the application over time.
II. Implementation Techniques for ‘Add to List’ feature:
1. Declaring and Initializing a List:
To begin utilizing the ‘Add to List’ feature, it is essential to declare and initialize the list. By following the syntax “List
2. Adding Elements to the List:
After the initial list creation, elements can be added using the “Add()” method. The syntax follows as follows: “list_name.Add(element);”. This method allows developers to add data of any valid data type to the list.
3. Iterative Addition:
Developers often need to populate a list with multiple elements iteratively. This can be achieved by utilizing loops or conditionals statements, such as ‘for’ or ‘while’ loops. Within the loop, each element can be appended to the list using ‘Add()’ method.
4. Accessing and Manipulating Elements:
Accessing and manipulating elements within a list is straightforward. Elements can be accessed using their assigned index, starting from ‘0’. Manipulations can include modifying, removing, or sorting elements based on specific requirements.
III. Frequently Asked Questions (FAQs):
Q1. Can I add elements of different data types to a list?
A1. No, lists in C# are strongly typed, meaning they can only store elements of a specific data type defined during initialization.
Q2. How can I check if an element already exists in a list before adding it?
A2. You can use the ‘Contains()’ method to check if an element is already present in the list before adding it. The method returns a Boolean value, indicating whether the element exists or not.
Q3. Is there a maximum limit to the number of elements I can add to a list?
A3. Though the practical limits depend on system specifications, C# lists support adding a large number of elements without any predefined limit.
Q4. What is the difference between an array and a list?
A4. While arrays have a fixed length once initialized, lists in C# provide dynamic resizing capabilities. This allows lists to handle variable amounts of data, making them more flexible than arrays.
Q5. Are there any performance considerations when using ‘Add to List’ feature extensively?
A5. When adding a large number of elements to a list, the performance may decrease compared to other specialized collection data structures, such as dictionaries or sets. Consider the specific requirements and choose the appropriate collection type accordingly.
Conclusion:
The ‘Add to List’ feature in C# is a powerful tool that allows developers to efficiently manage collections of data. By leveraging this feature, developers can create more flexible, scalable, and maintainable applications. Understanding the benefits, implementation techniques, and frequently asked questions related to this feature will undoubtedly enhance developers’ efficiency and productivity in C# programming.
C Add To Array
Introduction:
Arrays are an essential part of any programming language, and C is no exception. They allow you to store multiple values of the same data type in a single variable, making it easier to manage and manipulate large sets of data. In this article, we will delve into the topic of adding elements to an array in the C programming language, covering both the theory and practical implementation.
Understanding Arrays in C:
Before understanding how to add elements to an array, it’s important to have a solid grasp of what arrays are and how they work in C. An array in C is a collection of elements, all of the same data type, grouped together under a single name. Each element in an array can be accessed using its index, starting from 0 for the first element and incrementing by one for each subsequent element.
Declaring an Array in C:
To declare an array in C, we need to specify the data type of the elements it will store, followed by the array identifier and the number of elements enclosed within square brackets []. For example, to declare an integer array of size 5, we would write:
int myArray[5];
Adding Elements to an Array in C:
Once an array is declared, you can populate it with elements using various methods. Here, we’ll discuss two commonly used approaches: initializing the array during declaration and assigning values to array elements later.
1. Initializing the array during declaration:
During array declaration, you can assign values to each element of the array. For example, let’s say we want to initialize an integer array called myArray with the values 1, 2, 3, 4, and 5. We would write:
int myArray[5] = {1, 2, 3, 4, 5};
In this way, the array is initialized with the specified values at the time of declaration itself.
2. Assigning values to array elements later:
If you want to assign values to an array after its declaration, you can do so by accessing each individual element using its index and assigning the desired value. For example, to assign the value 10 to the second element (index 1) of the myArray, we would write:
myArray[1] = 10;
Similarly, you can assign values to the other elements of the array as needed. It is important to ensure that you do not exceed the bounds of the array while assigning values, as it can lead to unexpected results or undefined behaviors.
FAQs:
Q1. Can I add elements to an array dynamically in C?
A1. No, arrays in C have a fixed size, which is determined at the time of declaration. Once an array is declared, its size cannot be changed. If you need a dynamic collection of elements, consider using other data structures like linked lists or dynamically allocating memory using pointer arithmetic.
Q2. How can I handle the situation when the array is full, and I need to add more elements?
A2. In such cases, you have a few options. One approach is to declare a new array of a larger size, copy the existing elements to the new array, and then add the additional elements. Alternatively, you can use dynamic memory allocation techniques to reallocate memory for the array using functions like `realloc()`.
Q3. How can I add elements to the end of an array without overwriting existing elements?
A3. To add elements to the end of an array, you need to keep track of the current number of elements in the array and use it as an index to assign the new value. For example, if the array already has n elements, you can add a new element at index n by accessing myArray[n] = newValue. After adding the new element, you should increment the count of elements in the array (i.e., n++).
Q4. How can I add elements to a specific index in an array?
A4. To add elements at a specific index, you need to shift all the existing elements from that index to one position ahead. For instance, if you want to add a new element at index i, you would need to move all the elements from index i to (n-1) to the right. Then, you can assign the desired value at index i.
Conclusion:
In this article, we explored the process of adding elements to an array in the C programming language. We discussed initializing an array during declaration and assigning values to array elements later. We also addressed frequently asked questions related to array manipulation in C. Arrays are a fundamental concept in C and mastering their manipulation techniques will significantly enhance your programming skills.
Images related to the topic add to list c#
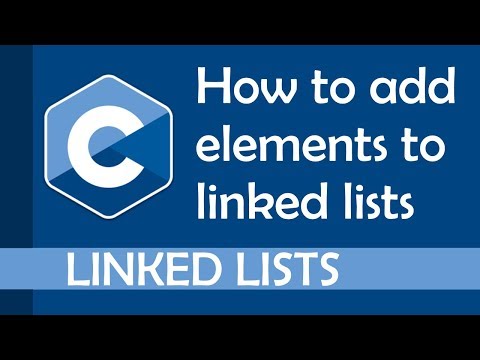
Article link: add to list c#.
Learn more about the topic add to list c#.
- Is there a way to add elements to a list/array? [C] [duplicate]
- how to add element in list c – W3schools.blog
- C program to Insert an element in an Array – GeeksforGeeks
- How to append a second list to an existing list in C – Tutorialspoint
- Sum of n numbers in C – Programming Simplified
- C# ArrayList (With Examples) – TutorialsTeacher
- How to Add Items to a C# List
- List
.Add(T) Method (System.Collections.Generic) - C program to insert an element in a singly linked list
- C# List Add Method (Append) – Dot Net Perls
- C# List
Collection – TutorialsTeacher - Adding an element to the List in C – Tutorialspoint
- How To add Elements to a List in Python – DigitalOcean
- C# List: Everything You Need to Know | Simplilearn
See more: blog https://nhanvietluanvan.com/luat-hoc