Add To Array C#
Arrays are an essential data structure in programming that allow us to store multiple values of the same data type in a single variable. In C#, arrays can be used to effectively manage and organize large amounts of data, making it easier to process and manipulate information.
Declaring and Initializing an Array
To declare an array in C#, you first need to specify the type of data it will store, followed by the square brackets [] to indicate that it is an array. Here’s an example of declaring an array of integers:
int[] numbers;
Initializing an array involves assigning values to its elements. This can be done using the “new” keyword followed by the array data type and the desired number of elements in square brackets. For example:
int[] numbers = new int[5];
This initializes an array of integers with 5 elements, all of which are automatically set to their default values (0 in the case of integers).
Adding Elements to an Array
To add elements to an array, you can assign values directly to its individual elements using their respective indices. The index represents the position of an element in the array, starting from 0. For example, to add the value 10 to the first element of the array, you would do:
numbers[0] = 10;
Adding Elements at the Beginning of an Array
In C#, arrays have a fixed size and cannot be directly resized. However, you can simulate adding elements at the beginning of an array by shifting all existing elements to the right and then assigning the new element to the first index. Here’s an example:
int[] numbers = new int[5] { 1, 2, 3, 4, 5 };
int[] newArray = new int[numbers.Length + 1];
newArray[0] = 0;
for (int i = 1; i < newArray.Length; i++) { newArray[i] = numbers[i - 1]; } This code creates a new array called "newArray" with a length one more than the original array "numbers". It then assigns the value 0 to the first index of "newArray" and shifts the elements of "numbers" to the right by one position. Adding Elements at a Specific Index in an Array To add an element at a specific index in an array, you need to shift all existing elements to the right starting from the desired index and then assign the new element to that index. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int[] newArray = new int[numbers.Length + 1]; int index = 2; // Index at which to add the element int elementToAdd = 10; for (int i = 0; i < index; i++) { newArray[i] = numbers[i]; } newArray[index] = elementToAdd; for (int i = index; i < numbers.Length; i++) { newArray[i + 1] = numbers[i]; } This code creates a new array called "newArray" with a length one more than the original array "numbers". It adds the element 10 at position 2 and shifts the elements of "numbers" to the right starting from that index. Adding Elements at the End of an Array To add an element at the end of an array, you can make use of the Array class's Resize method to increase the size of the array by one and then assign the new element to the last index. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; Array.Resize(ref numbers, numbers.Length + 1); numbers[numbers.Length - 1] = 10; The Resize method is passed the array to be resized as a ref parameter, as it modifies the original array directly. It increases the size of the array by one and then assigns the value 10 to the new last index. Adding Multiple Elements to an Array To add multiple elements to an array, you can make use of the Array class's Concat method to join two arrays together. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int[] newElements = new int[3] { 6, 7, 8 }; numbers = numbers.Concat(newElements).ToArray(); The Concat method concatenates the two arrays, creating a new array with the combined elements. The original array "numbers" is then assigned this new array. Increasing the Size of an Array As mentioned earlier, arrays in C# have a fixed size and cannot be directly resized. To increase the size of an array, you need to create a new array with the desired size, copy the elements from the original array to the new array, and then assign the new array to the original array. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int newSize = numbers.Length + 1; int[] newArray = new int[newSize]; Array.Copy(numbers, newArray, numbers.Length); numbers = newArray; This code increases the size of the array "numbers" by one. It creates a new array called "newArray" with the desired size and uses the Array.Copy method to copy the elements from "numbers" to "newArray". Finally, the reference of "numbers" is assigned the new array. Avoiding Common Pitfalls and Best Practices When working with arrays in C#, it's important to keep in mind the following best practices: - Ensure that the index used to access array elements is within the valid range (0 to array.Length - 1) to avoid runtime errors. - Be cautious when resizing arrays, as it can have performance implications, particularly with large arrays. Consider using dynamic data structures like Lists or ArrayLists if you frequently need to add or remove elements. - Initialize arrays with meaningful default values to avoid unexpected behavior. For example, if using an array of reference types, initialize each element to null. - Use a foreach loop to iterate over array elements, as it provides a simplified and safer way to access the elements without worrying about index bounds. FAQs Q: How can I add an array to another array in C#? A: To add one array to another in C#, you can use the Concat method of the Array class. Here's an example: int[] array1 = new int[3] { 1, 2, 3 }; int[] array2 = new int[3] { 4, 5, 6 }; int[] newArray = array1.Concat(array2).ToArray(); This code creates a new array called "newArray" by concatenating the elements of "array1" and "array2". The resulting array will have a length of 6, containing the elements {1, 2, 3, 4, 5, 6}. Q: How do I add an element to the end of an array in C#? A: To add an element to the end of an array in C#, you can make use of the Array class's Resize method to increase the size of the array by one and then assign the new element to the last index. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; Array.Resize(ref numbers, numbers.Length + 1); numbers[numbers.Length - 1] = 10; This code increases the size of the array "numbers" by one and assigns the value 10 to the new last index. Q: How do I append data to an array in C#? A: In C#, arrays have a fixed size and cannot be directly resized. However, you can simulate appending data to an array by creating a new array with a larger size, copying the elements of the original array to the new array, and then adding the new elements. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int[] newElements = new int[3] { 6, 7, 8 }; numbers = numbers.Concat(newElements).ToArray(); This code combines the elements of "numbers" and "newElements" into a new array called "numbers" by using the Concat method of the Array class. Q: How do I define an array in C#? A: To define an array in C#, you need to specify the data type it will store, followed by the square brackets [] to indicate that it is an array. Here's an example: int[] numbers; This code declares an array of integers called "numbers". Q: How do I find the sum of an array in C#? A: You can find the sum of an array in C# by using the LINQ extension method called Sum, which is available for numeric types. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int sum = numbers.Sum(); This code calculates the sum of the elements in the array "numbers". The resulting sum will be 15. Q: How do I add a string to an array in C#? A: To add a string to an array in C#, you can assign the string directly to a specific index of the array. Here's an example: string[] names = new string[3] { "John", "Jane", "" }; names[2] = "Kate"; This code assigns the string "Kate" to the third index of the array "names", replacing its previous value (an empty string). Q: Can I have an array within an array in C#? A: Yes, you can have an array within an array in C#. This is known as a multidimensional or jagged array. Here's an example of a jagged array: int[][] numbers = new int[2][]; numbers[0] = new int[3] { 1, 2, 3 }; numbers[1] = new int[2] { 4, 5 }; This code creates a jagged array called "numbers" with two inner arrays. The first inner array has three elements, while the second inner array has two elements. Q: How do I insert an element at a specific position in an array in C#? A: To insert an element at a specific position in an array in C#, you need to shift all existing elements to the right starting from the desired position and then assign the new element to that position. Here's an example: int[] numbers = new int[5] { 1, 2, 3, 4, 5 }; int[] newArray = new int[numbers.Length + 1]; int index = 2; // Index at which to insert the element int elementToAdd = 10; for (int i = 0; i < index; i++) { newArray[i] = numbers[i]; } newArray[index] = elementToAdd; for (int i = index; i < numbers.Length; i++) { newArray[i + 1] = numbers[i]; } This code creates a new array called "newArray" with a length one more than the original array "numbers". It inserts the element 10 at position 2 and shifts the elements of "numbers" to the right starting from that position.
Sum The Values In An Array | C Programming Example
How To Add To Arrays In C?
Arrays, a fundamental concept in the world of programming, allow us to store a collection of elements of the same data type in contiguous memory locations. In C, manipulating arrays is an essential skill for any programmer. One common operation is adding elements to an array. In this article, we will explore various methods to add elements to arrays in C and delve into the intricacies of each approach.
Methods for Adding Elements to Arrays
1. Initializing the Array with Values
One straightforward method to add elements to an array is by initializing it with values directly. This approach is suitable when the size of the array and the values to be added are known in advance. For example:
“`c
int myArray[5] = {1, 2, 3, 4, 5};
“`
In this case, the array `myArray` is assigned the values 1, 2, 3, 4, and 5, respectively. However, the size of the array must match the number of elements provided.
2. Using a Loop
When the number of elements to be added to an array is not known upfront or dynamic, using a loop can be an effective approach. By iterating through the loop, we can prompt the user to enter values and add them to the array. Here’s an example:
“`c
int myArray[5];
for (int i = 0; i < 5; i++) {
printf("Enter element %d: ", i+1);
scanf("%d", &myArray[i]);
}
```
In this code snippet, the loop executes five times, asking the user to enter each element. The entered values are then stored in the `myArray` array.
3. Expanding the Array Dynamically
In some cases, we may need to add elements to an array dynamically, i.e., when the size of the array is not predetermined. C does not provide a direct way to increase the size of an array, but we can simulate this behavior by creating a new, larger array and copying the existing elements into it. Here's an example using the `realloc()` function:
```c
int *myArray = malloc(5 * sizeof(int));
// Add new element
int element = 6;
int newSize = 6;
myArray = realloc(myArray, newSize * sizeof(int));
myArray[5] = element;
```
In this code, we first allocate memory to hold 5 integers using `malloc()`. Then, we add a new element by reallocating memory for 6 integers. Finally, we assign the value 6 to the last index of the expanded array.
4. Utilizing Dynamic Data Structures
If the array needs to grow dynamically, it may be more efficient to use dynamic data structures, such as linked lists or vectors. These data structures provide built-in mechanisms to handle resizing and adding elements efficiently.
Using VLA (Variable Length Array) Extension
In C99 and later, the Variable Length Array (VLA) extension allows us to define arrays whose size can be determined at runtime. This feature can be utilized to add elements to arrays dynamically. Here is an example:
```c
int size;
printf("Enter the size: ");
scanf("%d", &size);
int myArray[size];
for (int i = 0; i < size; i++) {
printf("Enter element %d: ", i+1);
scanf("%d", &myArray[i]);
}
```
In this code, the user is prompted to enter the desired size of the array. The array is then defined with the specified size, and the user can proceed to enter elements using a loop.
FAQs
Q: Can I add elements to an array without knowing its size in advance?
A: Yes, you can add elements to an array without knowing its size in advance. One way to accomplish this is by using a loop to prompt the user for input repeatedly until an exit condition is met.
Q: Can the size of an array be increased after its initialization?
A: No, the size of an array in C cannot be increased after its initialization. However, dynamic memory allocation techniques, such as reallocating memory or using dynamic data structures, can be employed to simulate the behavior of increasing the size of an array.
Q: Are there any limitations on the size of arrays that can be dynamically allocated?
A: The maximum size of an array (dynamically allocated or otherwise) depends on several factors, including the operating system and the available memory. If the requested size exceeds the system's limitations, the allocation will fail.
Q: Can I add elements to the middle of an array?
A: In C, arrays are fixed-size structures. If you want to add elements in the middle of an array, you will need to shift the existing elements to create space for the new elements. This process can be time-consuming and may not be suitable for large arrays.
In conclusion, adding elements to arrays in C can be achieved using various techniques. By understanding these methods, programmers can effectively manipulate arrays to meet their specific needs. Whether by initializing with values, using loops, dynamic memory allocation, or dynamic data structures, the ability to add elements to arrays empowers programmers to create versatile and efficient code.
How To Add Words To An Array In C?
An array is a data structure that allows you to store multiple elements of the same data type in a contiguous memory space. Adding words to an array in C can be done in a few different ways, depending on the specific requirements of your program. In this article, we will explore multiple methods to add words to an array and provide examples to help you understand the process better.
Method 1: Initializing the array with predefined words
One of the simplest ways to add words to an array is by initializing it with predefined values. Here’s an example:
“`c
#include
#define MAX_SIZE 5
int main() {
char words[MAX_SIZE][10] = {“apple”, “banana”, “orange”, “grape”, “melon”};
// Accessing the words
for (int i = 0; i < MAX_SIZE; i++) {
printf("%s\n", words[i]);
}
return 0;
}
```
In this example, we have initialized an array called `words` with a maximum size of 5 words, each having a length of 10 characters. The array is populated with the words "apple", "banana", "orange", "grape", and "melon". The `%s` format specifier is used to print each word.
Method 2: Adding words dynamically using user input
If you want to allow users to add words to an array during runtime, you can make use of loops and the `scanf` function to achieve this. Here's an example:
```c
#include
#define MAX_SIZE 5
int main() {
char words[MAX_SIZE][10];
// Adding words dynamically using user input
for (int i = 0; i < MAX_SIZE; i++) {
printf("Enter a word: ");
scanf("%s", words[i]);
}
// Accessing the words
for (int i = 0; i < MAX_SIZE; i++) {
printf("%s\n", words[i]);
}
return 0;
}
```
In this example, the user is prompted to enter a word, which is then stored in the array. The loop continues until the maximum size of the array is reached. Later, the words are printed using the same loop structure.
Method 3: Adding words from a file
In some scenarios, you might want to read words from a file and add them to an array. This can be achieved by using file handling functions such as `fopen`, `fscanf`, and `fclose`. Here's an example:
```c
#include
#define MAX_SIZE 100
int main() {
FILE *file_ptr;
char words[MAX_SIZE][10];
// Opening the file
file_ptr = fopen(“words.txt”, “r”);
if (file_ptr == NULL) {
printf(“Failed to open the file.\n”);
return 1;
}
// Reading words from the file
int i = 0;
while (fscanf(file_ptr, “%s”, words[i]) != EOF && i < MAX_SIZE) {
i++;
}
fclose(file_ptr); // Closing the file
// Accessing the words
for (int j = 0; j < i; j++) {
printf("%s\n", words[j]);
}
return 0;
}
```
In this example, we open the file "words.txt" in read mode using `fopen`. We then read words from the file using `fscanf` until either the end of the file or the maximum size of the array is reached. Finally, the accessed words are printed to the console.
Moreover, it is important to remember that the size of the array should be large enough to accommodate all the words you plan to add, or you should handle potential overflow gracefully.
FAQs:
Q: Can I add numeric values to the same array?
A: Yes, you can add numeric values to the same array as long as the data type of the array is compatible with the values you intend to store.
Q: How can I determine the number of words in the array?
A: To determine the number of words in the array, you can use a counter variable that increments each time a word is added to the array. Alternatively, you can keep track of the number of words using another variable or by inserting a special value (e.g., an empty string) to indicate the end of the words.
Q: Is it possible to add words to an array without specifying the maximum size?
A: Yes, you can dynamically allocate memory for an array using pointers and the `malloc` function, allowing you to add words without specifying a fixed size beforehand. However, this is a more advanced topic that goes beyond the scope of this article.
Q: What happens if I try to add more words than the array's maximum size?
A: If you attempt to add more words than the array's maximum size, it could lead to a buffer overflow, causing undefined behavior or even crashes in your program. It is important to ensure that your array can handle the expected maximum number of words or implement appropriate checks to prevent overflow.
In conclusion, adding words to an array in C can be done through various methods. Whether it's initializing the array with predefined words, taking user input, or reading from a file, it's essential to choose the approach that best suits your requirements. Remember to consider the maximum size of the array and handle potential issues like overflow to ensure the stability and correctness of your program.
Keywords searched by users: add to array c# Add array to array c, Add element to the end of array c, Append data to array C, Define array in C, Sum of array C, Add string to array c, Array in array C, Insert position in array in c
Categories: Top 48 Add To Array C#
See more here: nhanvietluanvan.com
Add Array To Array C
Arrays are an essential part of programming as they allow us to store and manipulate multiple values of the same data type efficiently. In C, arrays are widely used due to their simplicity and effectiveness. However, there may come a time when we need to combine or concatenate two arrays to perform specific operations. In this article, we will explore various methods to add one array to another in C, providing you with a comprehensive guide to tackle this common programming task.
1. Basic Concept of Array in C
To fully grasp the concept of adding arrays in C, let’s start with a brief overview of arrays. An array is a collection of similar data items of the same type, arranged in contiguous memory locations. Each element in the array can be accessed by its index value, starting from 0. For example, an integer array named “numbers” can be declared as follows:
“`c
int numbers[5] = {1, 2, 3, 4, 5};
“`
2. Adding One Array to Another Array
To add one array to another, we need to ensure that there is enough space in the destination array to accommodate the elements from both arrays. There are several methods to accomplish this, depending on the specific requirements of your program. Let’s explore three commonly used approaches:
2.1. Using a Separate Destination Array
One straightforward method involves creating a separate destination array that can hold the combined elements from both arrays. Here’s an example to demonstrate:
“`c
#include
int main() {
int array1[5] = {1, 2, 3, 4, 5};
int array2[3] = {6, 7, 8};
int size1 = sizeof(array1) / sizeof(array1[0]);
int size2 = sizeof(array2) / sizeof(array2[0]);
int destinationArray[size1 + size2];
for (int i = 0; i < size1; i++) {
destinationArray[i] = array1[i];
}
for (int i = 0; i < size2; i++) {
destinationArray[size1 + i] = array2[i];
}
// Print the combined array
for (int i = 0; i < size1 + size2; i++) {
printf("%d ", destinationArray[i]);
}
return 0;
}
```
In this example, we declare two arrays: `array1` containing five elements and `array2` containing three elements. We then calculate the sizes of both arrays using the formula `sizeof(array) / sizeof(array[0])`. We create a `destinationArray` with a size equal to the sum of `size1` and `size2`. Finally, we use two separate loops to copy the elements from `array1` and `array2` to the `destinationArray`, and then print the combined array.
2.2. Extending the First Array
Another approach to add one array to another is by extending the size of the first array to accommodate the additional elements from the second array. This method is useful if you want to modify the original array itself. Here's an example:
```c
#include
int main() {
int array1[5] = {1, 2, 3, 4, 5};
int array2[3] = {6, 7, 8};
int size1 = sizeof(array1) / sizeof(array1[0]);
int size2 = sizeof(array2) / sizeof(array2[0]);
array1[size1 + size2];
for (int i = 0; i < size2; i++) {
array1[size1 + i] = array2[i];
}
// Print the combined array
for (int i = 0; i < size1 + size2; i++) {
printf("%d ", array1[i]);
}
return 0;
}
```
In this example, we start with two arrays, `array1` and `array2`, as before. We calculate their sizes and extend `array1` by declaring a new size. Then, using a loop, we copy the elements from `array2` to the extended portion of `array1`. Finally, we print the combined array.
2.3. Using Dynamic Memory Allocation
If you want to add an array at runtime where the size is not predetermined, dynamic memory allocation can be a suitable approach. This involves using the `malloc()` or `calloc()` functions to allocate memory for the destination array. Consider the following example:
```c
#include
#include
int main() {
int* array1 = (int*) malloc(5 * sizeof(int));
int array2[3] = {6, 7, 8};
int size1 = 5;
int size2 = sizeof(array2) / sizeof(array2[0]);
// Store elements in array1
for (int i = 0; i < size1; i++) {
array1[i] = i + 1;
}
// Extend array1 to accommodate array2
int* tempArray = (int*) realloc(array1, (size1 + size2) * sizeof(int));
if (tempArray) {
array1 = tempArray;
for (int i = 0; i < size2; i++) {
array1[size1 + i] = array2[i];
}
// Print the combined array
for (int i = 0; i < size1 + size2; i++) {
printf("%d ", array1[i]);
}
free(array1);
} else {
printf("Memory reallocation failed.");
}
return 0;
}
```
In this example, we use dynamic memory allocation functions to allocate memory for `array1` and extend its size using `realloc()`. After extending `array1`, we copy the elements from `array2` to the extended portion of `array1`. Finally, we print the combined array and free the dynamically allocated memory.
FAQs:
Q1. Can I add arrays of different data types?
A1. No, in C, arrays can only store elements of the same data type. If you need to combine arrays of different data types, you can use structures or create a new array to hold the elements of different arrays.
Q2. Is it possible to add arrays of different sizes?
A2. When adding arrays, the sizes of the destination array and the array being added must be known. They must have enough space to accommodate all the elements. If the sizes are not known beforehand, you can use dynamic memory allocation, as shown in the example using `malloc()` and `realloc()`.
Q3. Can I add more than two arrays together?
A3. Yes, the methods described above can be extended to combine multiple arrays. You can use additional loops to copy elements from multiple arrays to the destination array.
Q4. How can I handle the case where the destination array is too small to accommodate both arrays?
A4. It is essential to ensure that the destination array has enough space to hold the combined elements. If it does not, you may encounter memory corruption or segmentation faults. The dynamic memory allocation method allows you to dynamically resize the destination array to accommodate the added elements.
In conclusion, adding one array to another is a common programming task that can be accomplished using various methods in C. Whether you choose to create a separate destination array, extend the size of the first array, or use dynamic memory allocation, these techniques will help you effectively combine arrays and perform operations on them. Understanding the concepts and implementing the appropriate method according to your program's requirements will enrich your C programming skills.
Add Element To The End Of Array C
Arrays are one of the fundamental data structures used in computer programming. They allow us to store multiple values of the same type in a single variable. While arrays have a fixed size, in some cases, we may find the need to add elements to the end of an existing array. In this article, we will explore various methods to add an element to the end of an array in the C programming language.
In C, arrays are declared with a specific size, which means that they cannot dynamically grow or shrink. Therefore, if we want to add an element to the end of an array, we need to create a new, larger array and copy all the existing elements to it, along with the new element. This process can be achieved through the following steps:
1. Determine the current size of the array:
Before we proceed, we need to know the current size of the array to properly allocate memory for the new array.
2. Create a new array with a larger size:
To add an element to the end of an array, we need to create a new array with a size larger than the original array. This can be done by calculating the new size, which equals the current size plus one.
3. Copy the existing elements to the new array:
Using a loop, we can traverse the original array and copy each element to the corresponding position in the new array.
4. Add the new element at the end of the new array:
Once we have copied all the existing elements, we can add the new element at the end of the new array.
5. Deallocate the memory occupied by the old array:
As we have created a new array, it’s necessary to deallocate the memory occupied by the old array to avoid memory leaks.
Let’s illustrate these steps with an example code snippet:
“`c
#include
int main() {
int c[5] = {1, 2, 3, 4, 5};
int size = sizeof(c) / sizeof(int); // Determine the current size of the array
int new_size = size + 1; // Calculate the new size of the array
int new_array[new_size]; // Create a new array with a larger size
for (int i = 0; i < size; i++) { new_array[i] = c[i]; // Copy existing elements to the new array } int new_element = 6; // The element to be added new_array[new_size - 1] = new_element; // Add the new element at the end of the new array // Print the elements of the new array for (int i = 0; i < new_size; i++) { printf("%d ", new_array[i]); } return 0; } ``` In this example, we have an array `c` with five elements. We first determine the current size of the array, which is five. Then, we calculate the new size by adding one to the current size. We create a new array `new_array` with the new size and use a loop to copy all the existing elements from `c` to `new_array`. Finally, we add the new element `6` at the end of `new_array`. The resulting array is then printed to confirm that the new element has been added successfully. FAQs: Q: Can we add elements to the end of an array without creating a new, larger array? A: No, in C, arrays have a fixed size and cannot dynamically grow or shrink. Adding an element to the end of an array requires creating a new array that can accommodate the additional element. Q: Can we use functions to add elements to the end of an array? A: Yes, by defining a function that accepts an array and a new element as arguments, you can encapsulate the process of creating a new array, copying elements, and adding the new element into a single function. This allows for more modular and reusable code. Q: Is there a limit to how many elements an array can hold? A: Yes, the maximum number of elements an array can hold is determined by the size of the array, which is specified during declaration. If the array is declared as `int c[10]`, it can hold a maximum of ten elements. Q: What if the array is already at its maximum size and we want to add more elements? A: When an array is already at its maximum size, it is not possible to add more elements. In such cases, alternative data structures like linked lists or dynamically resizing arrays can be used. Q: Are there any library functions available to add elements to the end of an array? A: The standard C library does not provide specific functions to add elements to the end of an array. However, there are libraries like `malloc` and `realloc` that can be used for dynamic memory allocation, which can be utilized to achieve similar functionality. In conclusion, while arrays in C have a fixed size, we can add elements to the end of an array by creating a new, larger array and copying all the existing elements along with the new element. Although this process may seem indirect, it allows us to extend the capabilities of arrays and adapt them to our programming needs. Remember to consider the limitations of arrays in C and explore alternative data structures when necessary.
Images related to the topic add to array c#
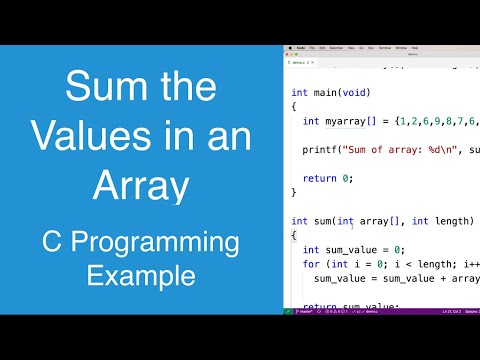
Article link: add to array c#.
Learn more about the topic add to array c#.
- Can someone explain how to append an element to an array …
- C program to Insert an element in an Array – GeeksforGeeks
- C program to Insert an element in an Array – GeeksforGeeks
- How to store words in an array in C? – GeeksforGeeks
- Inserting an element in an array | Practical C Programming
- How to Find the Size of an Array in C with the sizeof Operator
- Inserting elements in an array using C Language – Tutorialspoint
- Add Element in Array in C – Javatpoint
- C Arrays – W3Schools
- C Program to Insert an Element in an Array – W3schools
- How can I append to an array in C? – Quora
- Display Append and Insert Elements in an Array
- C program to find sum of array elements – Log2Base2
- C Arrays (With Examples) – Programiz
See more: blog https://nhanvietluanvan.com/luat-hoc