You Cannot Call A Method On A Null-Valued Expression
In programming, errors can occur when trying to perform operations on null-valued expressions. One of the most common errors is known as “You cannot call a method on a null-valued expression.” This article aims to provide a comprehensive understanding of this error and how to avoid it in various programming contexts, particularly within the PowerShell environment.
Definition of a Null-Valued Expression:
A null-valued expression refers to a variable or object that has not been assigned a value, or has been explicitly set to null. In programming languages like PowerShell, null represents the absence of a value. When attempting to call a method on a null-valued expression, an error occurs because there is no object or variable to perform the desired action on.
Common Causes of Null-Valued Expressions:
1. Uninitialized Variables: If a variable is declared but not assigned a value before being used in a method call, it will be considered null.
2. Incorrect Assignment: Assigning an incorrect value or forgetting to assign a value to a variable or object can result in a null-valued expression.
3. Unavailable Data: In some cases, data retrieved from external sources may not be available or may return null, leading to null-valued expressions.
4. Failed Function Calls: If a function fails to execute correctly or returns null, subsequent method calls on the result may produce this error.
Implications of Calling a Method on a Null-Valued Expression:
Calling a method on a null-valued expression can have several implications, depending on the programming language and context. Some common consequences include:
1. Runtime Errors: The program will terminate abruptly, displaying the error message, which can disrupt execution.
2. Unexpected Behavior: If the program continues execution after encountering the error, it may produce unexpected or incorrect results.
3. Data Loss: In certain cases, the error may lead to loss or corruption of data if the program does not handle the error appropriately.
How to Avoid Calling a Method on a Null-Valued Expression:
To prevent calling a method on a null-valued expression, programmers can employ the following techniques:
1. Null Checks: Before calling a method on an object or variable, perform a null check to ensure that it is not null. This can be achieved by using conditional statements or a null coalescing operator. For example, in PowerShell, you can use the “-eq $null” comparison to check if a variable is null before calling a method on it.
“`
if ($variable -eq $null) { Write-Host “Variable is null.” }
else { $variable.MethodCall() }
“`
2. Defensive Programming: Practice good coding habits by initializing variables with default values or explicitly handling null cases. This can help prevent null-valued expression errors before they occur.
“`
$variable = $null
$variable = “Default Value” # Avoids null-valued expression error
“`
3. Error Handling: Implement proper error handling techniques, such as try-catch blocks, to gracefully deal with null-valued expression errors. This enables you to provide meaningful error messages and take appropriate actions when encountering null values.
Handling Null-Valued Expressions using Conditional Statements:
Conditional statements are an effective way to handle null-valued expressions in programming. They allow you to check the value of an object or variable before performing any method calls. In PowerShell, you can use an “if” statement to conditionally execute code. Here’s an example of handling a null-valued expression using conditional statements:
“`powershell
if ($variable -eq $null) {
Write-Host “Variable is null.”
} else {
$variable.MethodCall()
}
“`
By checking if the variable is null before calling the method, you prevent the error message and handle the null value appropriately.
Error Handling Techniques for Null-Valued Expressions:
When dealing with null-valued expressions, it’s crucial to implement robust error handling techniques. In PowerShell, you can use the “try-catch” block to catch and handle exceptions gracefully. Here’s an example of error handling for null-valued expressions:
“`powershell
try {
$result = $variable.MethodCall()
} catch {
Write-Host “An error occurred: $($_.Exception.Message)”
# Handle the error appropriately
}
“`
By enclosing the potentially error-prone method call within a “try” block, you can catch any exceptions that occur during execution. The “catch” block allows you to handle the error, display a custom error message, and take corrective action.
Frequently Asked Questions (FAQs):
Q1. What is the “get-windowsautopilotinfo you cannot call a method on a null-valued expression” error?
The “get-windowsautopilotinfo you cannot call a method on a null-valued expression” error typically occurs when calling the “Get-WindowsAutoPilotInfo” method in PowerShell, passing a null-valued expression as an argument. This error indicates that the provided argument contains null.
Q2. How can I set a variable in PowerShell without encountering a null-valued expression error?
To avoid null-valued expression errors when setting a variable in PowerShell, ensure that you assign a valid value during initialization. For example:
“`powershell
$variable = “Default Value”
“`
Q3. Are there any alternative methods to print output in PowerShell without triggering null-valued expression errors?
Yes, there are alternative methods to print output in PowerShell. One commonly used method is “Write-Host,” which directly displays text on the console. Another option is the “Write-Output” cmdlet, which sends output to the pipeline for further processing.
Q4. How can I use conditional statements to avoid null-valued expression errors in PowerShell?
PowerShell allows you to use conditional statements, such as “if,” “switch,” and “try-catch” blocks, to handle null-valued expression errors. By checking if a variable is null before performing method calls, you can avoid triggering this error.
You Cannot Call A Method On A Null-Valued Expression. Invalidoperation:…
How To Fix You Cannot Call A Method On A Null Valued Expression?
If you have ever encountered the error message stating “You cannot call a method on a null valued expression” while programming, you’re not alone. It is a common issue that can occur in various programming languages, including Java, C#, JavaScript, and others. This article aims to provide you with a comprehensive guide on fixing this error by understanding its causes and suggesting valuable solutions.
Understanding the Error:
To begin, let’s first grasp the meaning behind the error message. The phrase “you cannot call a method on a null valued expression” typically suggests that you are trying to invoke a method or access a property on an object that is null, meaning the variable does not reference any object currently. Consequently, calling a method on a null object will result in a run-time error.
Causes of the Error:
1. Uninitialized Variables: One common cause of this error is when you forget to initialize the variable before invoking a method on it. For instance, suppose you declare a variable but fail to assign it any value before calling a method. This will result in a null valued expression error.
2. Null References: Another reason for encountering this error is when you pass null as a parameter to a method that cannot handle null values. The method then tries to perform an action on the null object, causing the error message to appear.
3. Inaccessible Objects: If you attempt to call a method on an object that is inaccessible or has been set to null intentionally, you will also encounter this error. Double-check the object’s accessibility and ensure it refers to an instantiated object before calling any methods.
Solutions to Resolve the Error:
1. Check for Null References: To fix the error, you must ensure there are no null references passed to methods or variables involved. Before invoking a method, validate if the object is null. If it is, handle the null scenario appropriately, either by assigning a default value or returning an error message.
2. Initialize Variables: A more preventive approach to avoid null valued expression errors is to initialize variables as soon as you declare them. Assign a default value or instantiate an object, ensuring it is not null when a method is called on it.
3. Implement Null Checks: Incorporate null checks strategically in your code to prevent null objects from causing runtime errors. Use conditional statements, such as if-else or try-catch blocks, to verify if an object is null before attempting to call a method on it.
4. Use Null-Safe Libraries: Some programming languages offer null-safe libraries specifically designed to handle null values gracefully. Explore these libraries compatible with your programming language, such as Optional in Java or Nullable Reference Types in C#, to simplify null value handling and prevent null reference errors.
5. Debugging and Testing: It’s crucial to perform thorough testing and debugging to identify the root cause of the error. Utilize debugging tools provided by your IDE (Integrated Development Environment) to trace the code execution and pinpoint where the null valued expression error occurs. Walk through the code step by step and analyze the values of variables and objects to ensure they are not null when needed.
FAQs:
1. What does the error “You cannot call a method on a null valued expression” mean?
This error message indicates that you are trying to invoke a method or access a property on an object that is currently null, resulting in a run-time error.
2. Why am I encountering this error?
The error can occur due to various reasons, including uninitialized variables, null references passed as parameters, or attempts to call methods on inaccessible or intentionally set null objects.
3. How can I fix the null valued expression error?
To fix this error, ensure there are no null references passed to methods, initialize variables when declaring them, implement null checks strategically, consider using null-safe libraries, and perform thorough testing and debugging.
4. Are there any programming languages where this error does not occur?
Although the exact error message may vary between programming languages, the concept of null valued expressions exists across languages. Hence, the error can occur in most programming languages, including Java, C#, JavaScript, and others.
In conclusion, encountering the “You cannot call a method on a null valued expression” error is a common challenge while programming. By understanding the causes behind this error and implementing the suggested solutions provided in this article, you can effectively resolve this issue and ensure smoother code execution. Remember to initialize variables, validate null references, and perform thorough testing to prevent such errors and create reliable code.
What Is The Null Method In Powershell?
PowerShell is a powerful scripting language and shell environment framework developed by Microsoft for task automation and configuration management. It provides a wide range of features and functionalities to effectively manage and manipulate Windows operating systems. One of the useful methods in PowerShell is the null method, which helps handle situations where you may not want any output or need to discard the result of a command. In this article, we will explore the null method in PowerShell, its significance, and how it can be effectively used.
Understanding the null method:
The null method in PowerShell is denoted by `$null`, which represents an empty or undefined value. It is commonly used when you want to suppress the output of a command or expression within a script. When a command returns a result, you can assign it to the `$null` variable to discard the output entirely. This method is particularly handy to prevent unwanted or excessive output cluttering your PowerShell console.
Usage and Applications:
The null method is employed in various situations to improve script performance, reduce clutter, and handle specific scenarios. Let’s discuss some common use cases below:
1. Suppressing command output:
Often, when running a command or executing a script, you may not require the output to be displayed. For instance, when working with large datasets or executing lengthy scripts, printing output to the console may cause unnecessary performance overhead. Placing `$null =` in front of the command will discard the output, preventing it from being displayed. This method can significantly enhance script execution speed, especially when working with bulk operations.
Example:
“`powershell
$null = Get-Process
“`
2. Discarding unwanted information:
Sometimes, a command may return additional information that is not relevant to the task at hand. In such cases, using `$null` can help discard extraneous data, keeping your output concise and relevant. This is particularly useful when piping multiple commands together, as it enables you to filter and streamline information based on your requirements.
Example:
“`powershell
Get-ChildItem | Where-Object { $_.Length -ge 100kb } | $null
“`
In the above example, `$null` is used to discard the output of the `Where-Object` cmdlet, allowing the command to effectively filter files greater than or equal to 100KB.
3. Testing command success without displaying output:
Another important application of the null method is to test command success without cluttering the console with output. Often, script logic requires the result of a command to determine subsequent actions. When assigning the command result to `$null`, you can still evaluate the success or failure without displaying the output.
Example:
“`powershell
$null = Stop-Service -Name “ServiceName” -ErrorAction SilentlyContinue
if ($?) {
Write-Host “Service stopped successfully.”
}
“`
In the above example, `$null` is used to discard the output of the `Stop-Service` cmdlet. The `$?` variable is then used to check if the command was successful. If true, a success message is displayed.
FAQs:
Q: Can I assign a value to `$null`?
A: No, `$null` is a reserved value in PowerShell and cannot be changed or assigned a different value.
Q: What is the difference between `$null` and `$false`?
A: `$null` represents an empty or undefined value, while `$false` is a Boolean variable representing the value false.
Q: Can I use `$null` to discard output to a file?
A: No, using `$null` will simply discard the output on the console. To redirect output to a file, you can use the `>` or `>>` operators.
Q: Can I use the null method with functions or script modules?
A: Yes, the null method can be used within functions and script modules to discard unwanted output or evaluate the success of a command.
Q: Can `$null` be used with commands that require output for further processing?
A: It is generally not recommended to use `$null` with commands where the output is required for subsequent processing. Use it only for cases where the output is not relevant or needs to be discarded.
Keywords searched by users: you cannot call a method on a null-valued expression get-windowsautopilotinfo you cannot call a method on a null-valued expression, PowerShell variable, Function PowerShell, PowerShell echo, Set-Variable PowerShell, PowerShell parameter, Print PowerShell, Get-Content PowerShell
Categories: Top 27 You Cannot Call A Method On A Null-Valued Expression
See more here: nhanvietluanvan.com
Get-Windowsautopilotinfo You Cannot Call A Method On A Null-Valued Expression
Introduction:
The Get-WindowsAutoPilotInfo command in Windows PowerShell is a fundamental tool used to gather information about AutoPilot deployment. However, sometimes users encounter a cryptic error message that halts their progress: “You cannot call a method on a null-valued expression.” In this article, we will delve into the specifics of this error message, explore its causes, and provide effective troubleshooting methods to overcome it.
Understanding the Error Message:
The error message, “You cannot call a method on a null-valued expression,” typically occurs when calling the Get-WindowsAutoPilotInfo command in PowerShell. It signifies that the command tried to invoke a method on a variable or object that does not contain any data, leaving it empty or null.
Causes of the Error:
1. Insufficient Permissions: One common cause of this error is the absence of sufficient permissions to access the necessary resources or query the AutoPilot data. Ensure that the user account executing the command has the necessary privileges.
2. Incorrect Command Usage: Executing the Get-WindowsAutoPilotInfo command with incorrect parameters or syntax can result in a null response. Review the command syntax and ensure that all required parameters are provided correctly.
3. Incomplete or Inaccurate Input Data: This error may also occur when the command is unable to retrieve any valid data due to incomplete or inaccurate input. Review the input parameters, such as the CSV file containing hardware information, and ensure their accuracy.
4. Data Synchronization Issues: If the device records in the AutoPilot deployment service are not synchronized properly, the information requested by the Get-WindowsAutoPilotInfo command may not be available. Check the synchronization status and make sure all devices are up-to-date.
Troubleshooting Steps:
1. Validate User Permissions: Ensure that the user account executing the command has the necessary permissions to access AutoPilot data. Grant the required permissions using the Azure Active Directory (AAD) settings or consult with your administrator to verify the user’s access rights.
2. Double-Check Command Syntax: Review the Get-WindowsAutoPilotInfo command syntax and verify that all required parameters are provided accurately. Cross-reference the official Microsoft documentation or consult the help section within PowerShell.
3. Verify Input Data: Check the input data, such as the CSV file that contains the hardware information, to ensure its completeness and accuracy. Validate the file’s structure, correct column headers, and properly formatted content.
4. Execute the Command on a Domain-Joined PC: Attempt running the command on a domain-joined PC that has an active connection to the Internet. This ensures seamless communication with the AutoPilot deployment service and reduces the chances of errors related to connectivity.
5. Verify Data Synchronization: Ensure that the device information is synchronized between the AutoPilot deployment service, Azure AD, and Intune. Monitor the synchronization progress and confirm that all devices are up-to-date.
FAQs:
Q1. Can this error occur on any Windows operating system?
A1. Yes, the “You cannot call a method on a null-valued expression” error can occur on any Windows operating system where the Get-WindowsAutoPilotInfo command is executed.
Q2. How can I grant the necessary permissions to access AutoPilot data?
A2. To grant permissions, navigate to Azure Active Directory, locate the user in question, and assign them the required roles or privileges to access AutoPilot data.
Q3. Why is synchronization important in resolving this error?
A3. Synchronization ensures that the device records are up-to-date, providing accurate data for the Get-WindowsAutoPilotInfo command to retrieve. Syncing devices can help resolve this error.
Q4. Does this error impact the overall AutoPilot deployment process?
A4. This error primarily affects the Get-WindowsAutoPilotInfo command and does not halt the entire AutoPilot deployment process. However, resolving this error is crucial for collecting accurate AutoPilot data.
Conclusion:
The “You cannot call a method on a null-valued expression” error can be a roadblock when gathering AutoPilot deployment information using the Get-WindowsAutoPilotInfo command in PowerShell. By understanding the causes and following the troubleshooting steps provided in this article, users can overcome this error and continue their AutoPilot deployment smoothly. Remember to validate user permissions, review the command syntax, input data, and ensure proper data synchronization.
Powershell Variable
Introduction:
PowerShell is a command-line scripting language that is widely used by system administrators and IT professionals for automating administrative tasks in the Windows operating system. One of the most powerful features of PowerShell is its variable capabilities, which allow users to store and manipulate data. In this article, we will explore the concept of PowerShell variables, their various types, and how to effectively use them to streamline your scripting tasks.
Understanding PowerShell Variables:
In simple terms, a variable in PowerShell is a named storage location that holds a value or piece of information. These values can be anything from numbers, strings, dates, arrays, or even objects. Variables are essential for storing temporary or calculated data, enabling users to reference and update them throughout their script.
Declaring PowerShell Variables:
To declare a variable in PowerShell, you simply assign a name to it using the “$” sign, followed by the variable name and an equals sign. For example, to declare a variable called “myVariable” with the value 5, you would use the following command:
$myVariable = 5
PowerShell Variables and Data Types:
PowerShell is a dynamically typed language, meaning you don’t have to specify a specific data type when declaring a variable. It automatically assigns the appropriate data type based on the assigned value. However, it is helpful to understand the various data types to properly manipulate and utilize variables.
1. String: A string variable holds a sequence of characters, enclosed in either single or double quotes. For example:
$name = “John”
2. Integer: An integer variable stores whole numbers with no decimal places. For example:
$age = 25
3. Float: A float variable stores numbers with decimal places. For example:
$piValue = 3.14
4. Boolean: A boolean variable holds either a “true” or “false” value. For example:
$isOnline = $true
5. Array: An array variable allows storing multiple values in a single variable. For example:
$fruits = “apple”, “banana”, “orange”
Common Operations with PowerShell Variables:
Now that we understand the basics of declaring variables in PowerShell, let’s delve into some common operations associated with variables.
1. Assigning a New Value: You can assign a new value to an existing variable simply by reassigning it. For example:
$age = 30
2. Concatenation: You can concatenate strings using the plus (+) operator. For example:
$greeting = “Hello, ” + $name
3. Arithmetic Operations: PowerShell supports various arithmetic operations such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). For example:
$result = $num1 + $num2
4. Updating Variable Values: PowerShell provides shortcuts for updating variable values. For instance, you can increment a numerical variable using the “++” operator or concatenate strings using the “+=” operator. For example:
$counter++
$stringVariable += ” new content”
Frequently Asked Questions (FAQs):
Q1. Can I change the data type of a variable after declaration?
A1. No, once a variable is declared, changing its data type is not possible. However, you can reassign a new value with a different data type.
Q2. Can I use spaces while declaring variable names?
A2. While it is technically possible to use spaces in variable names, it is not recommended. It is better to use camel case or underscores for readability and alignment with coding best practices.
Q3. Can I use variable values in PowerShell command arguments?
A3. Yes, PowerShell allows using variable values directly within command arguments. Simply reference the variable using the “$” sign. For example: `Get-Process -name $processName`
Q4. How can I check if a variable is empty?
A4. You can use the `If` statement along with the `-eq` comparison operator to check if a variable is empty. For example: `If ($variable -eq $null) { }`
Conclusion:
PowerShell variables are an integral part of the scripting language, providing a way to store, manipulate, and reference data. By understanding the various data types and operations associated with variables, you can effectively utilize them to automate administrative tasks and streamline your scripting workflow. Experiment with PowerShell variables and unleash the power of automation in your IT operations.
Images related to the topic you cannot call a method on a null-valued expression
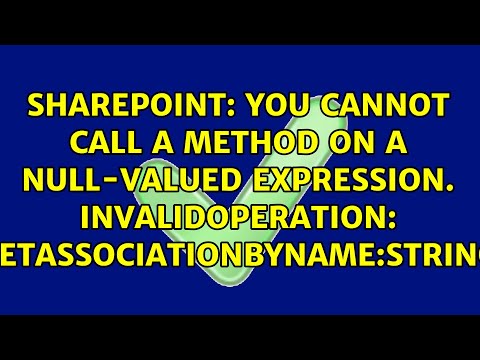
Found 22 images related to you cannot call a method on a null-valued expression theme


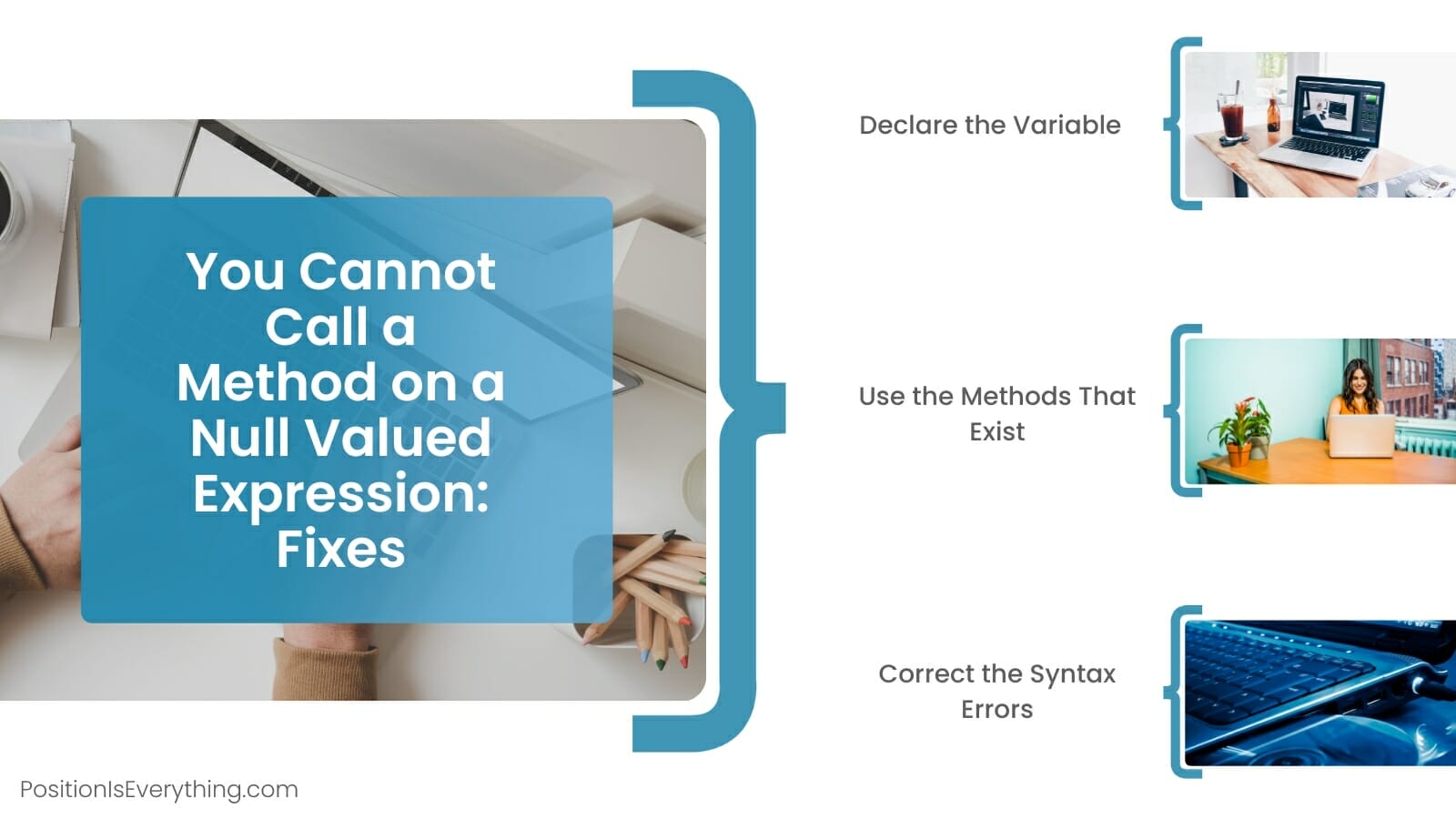



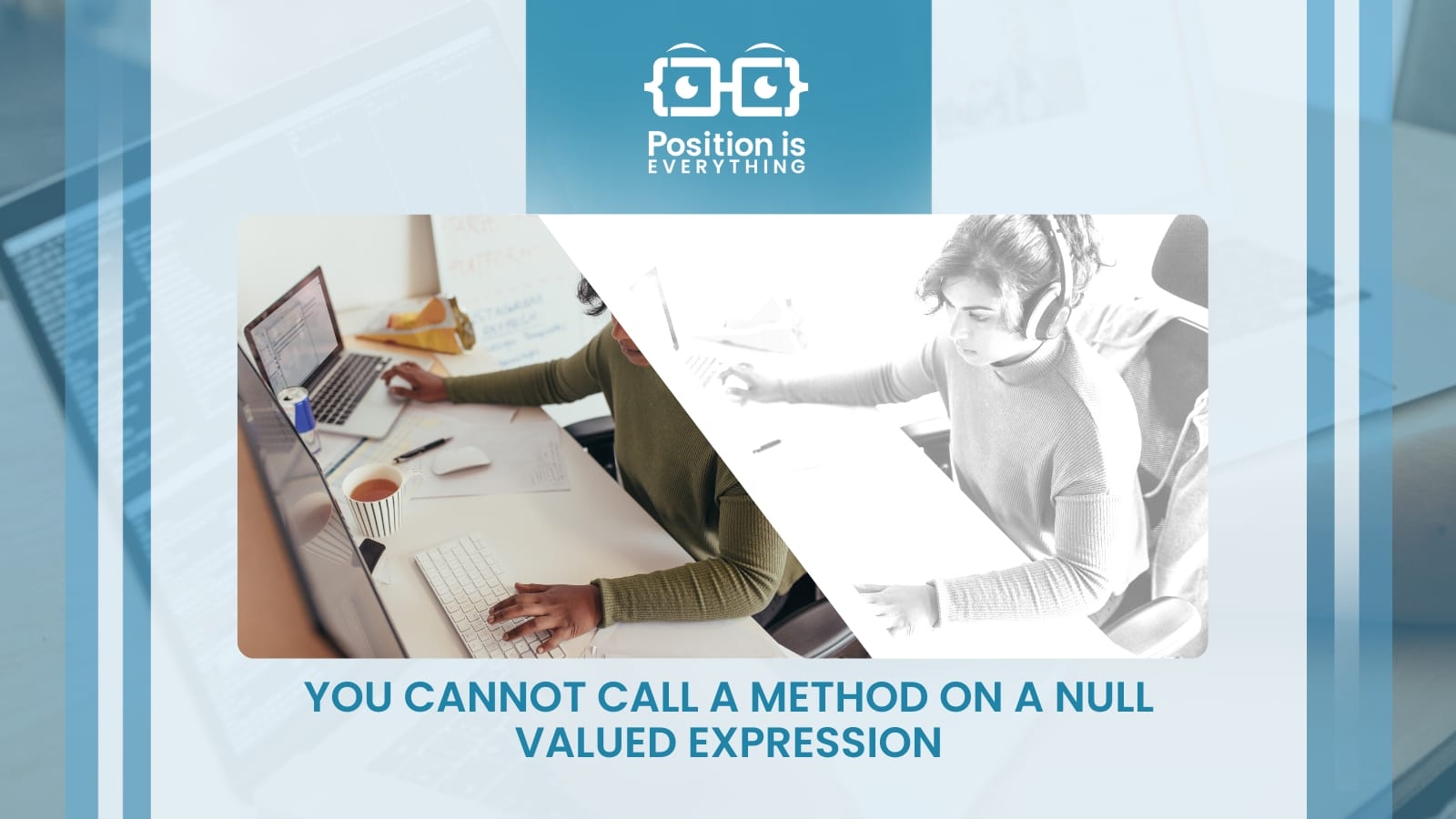



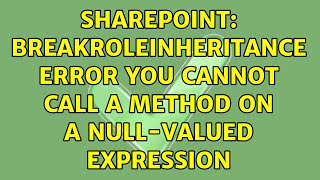

![You cannot call a method on Null valued expression at: $File = $Web.GetFile([Guid]$Result.Id) - YouTube You Cannot Call A Method On Null Valued Expression At: $File = $Web.Getfile([Guid]$Result.Id) - Youtube](https://i.ytimg.com/vi/YpnHaLN7zJU/maxresdefault.jpg)



Article link: you cannot call a method on a null-valued expression.
Learn more about the topic you cannot call a method on a null-valued expression.
- You cannot call a method on a null-valued expression
- You Cannot Call a Method on a Null Valued Expression: Guide
- You Cannot Call a Method on a Null Valued Expression: Guide
- Everything you wanted to know about $null – PowerShell | Microsoft Learn
- Understanding The Null Valued Expression Error: Calling A …
- [SOLVED] Cannot Call a Null Method – Spiceworks Community
- Error during provisioning: “You cannot call a method on a null …
- TFS Certificate – ou cannot call a method on a null-valued …
See more: nhanvietluanvan.com/luat-hoc