Typeerror: Unhashable Type: ‘Slice’
TypeError is a common error that Python programmers come across when working with different data types. It occurs when an operation or function is performed on an object of the wrong type. This can happen when trying to perform unsupported operations or when passing arguments of incorrect types to functions or methods.
Key Concepts in Python TypeErrors
– TypeErrors indicate that an operation or function is being used on an object of the wrong type.
– Python is a dynamically typed language, which means that variable types are determined at runtime.
– TypeErrors can occur when performing operations such as arithmetic, indexing, slicing, or calling methods on objects.
Common Causes of TypeErrors in Python
There are several common causes for TypeErrors in Python:
1. Mixing incompatible types: TypeErrors often occur when trying to perform operations between objects of different types.
2. Incorrect function or method arguments: Passing incorrect types of arguments to functions or methods can also result in TypeErrors.
3. Accessing elements that do not exist: TypeErrors can occur when trying to access elements of a data structure, such as a list or dictionary, that do not exist.
4. Improper use of operators: Using operators incorrectly, such as trying to perform arithmetic with non-numeric values, can result in TypeErrors.
Dealing with ‘unhashable type: ‘slice” TypeError
The ‘unhashable type: ‘slice” TypeError is a specific type of TypeError that occurs when trying to use a ‘slice’ object as a key in a dictionary or as an element in a set. In Python, ‘slice’ objects are not hashable, which means they cannot be used as keys or elements in hash-based data structures.
To deal with this TypeError, a common approach is to convert the ‘slice’ object into a tuple or a list before using it as a key or element. This can be done using the ‘tuple()’ or ‘list()’ functions, respectively. Here is an example:
“`python
my_slice = slice(1, 5)
my_tuple = tuple(my_slice)
“`
In this example, the ‘slice’ object ‘my_slice’ is converted into a tuple ‘my_tuple’, which can now be used as a key in a dictionary or an element in a set without raising the ‘unhashable type: ‘slice” TypeError.
Exploring the ‘slice’ Type in Python
In Python, the ‘slice’ type is used to represent a range of indices in a sequence, such as a string, list, or tuple. It provides a convenient way to specify a subset of elements in a sequence without explicitly iterating over them. The syntax for creating a ‘slice’ object is ‘[start:stop:step]’.
– ‘start’ represents the starting index of the slice (inclusive).
– ‘stop’ represents the ending index of the slice (exclusive).
– ‘step’ represents the step or increment between indices.
Here is an example of using a ‘slice’ object to extract a subset of elements from a list:
“`python
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
my_slice = slice(1, 5, 2)
subset = my_list[my_slice] # [2, 4]
“`
In this example, the ‘slice’ object ‘my_slice’ represents the range of indices [1:5:2], which includes the elements at indices 1 and 3 (2 and 4, respectively). The resulting subset of elements is [2, 4].
Methods to Avoid or Handle ‘unhashable type: ‘slice” TypeError
To avoid or handle the ‘unhashable type: ‘slice” TypeError, you can use the following methods:
1. Convert the ‘slice’ object into a tuple or a list using the ‘tuple()’ or ‘list()’ functions respectively, as mentioned earlier.
2. If you need to use a ‘slice’ object as a key in a dictionary, consider using a different data structure, such as a list or a tuple.
3. If you need to perform operations that require hashable types on a ‘slice’ object, consider using a different approach without using ‘slice’ objects.
Debugging Techniques for TypeErrors in Python
When encountering TypeErrors in Python, it is important to identify the source of the error. Here are some debugging techniques to help you:
1. Check the error message: The error message usually provides information about the type involved and the operation causing the TypeError.
2. Review the code: Check the code that is causing the TypeError and verify whether the operations or arguments are used correctly.
3. Use print statements: Insert print statements to display the values of variables and objects involved in the erroneous operation. This can help identify the source of the TypeError.
4. Apply divide and conquer: If the code is complex, try to isolate the problematic part by gradually commenting out or simplifying sections of the code until the TypeError is resolved.
Best Practices to Prevent TypeErrors in Python
To prevent TypeErrors in your Python code, consider the following best practices:
1. Use type annotations: Type annotations provide hints about expected types, allowing you to catch potential TypeErrors during development using static analysis tools.
2. Perform input validation: Before using any data or arguments, perform input validation to ensure they conform to the expected types.
3. Use descriptive variable names: Naming variables according to their intended purpose can help prevent mixing incompatible types.
4. Write unit tests: Writing comprehensive unit tests can help identify any TypeErrors before they occur in production.
5. Follow Python’s coding conventions: Adhering to Python’s coding conventions, such as using meaningful variable names and avoiding unnecessary type conversions, can prevent potential TypeErrors.
FAQs
Q: What does the ‘unhashable type: ‘slice” TypeError mean in Python?
A: The ‘unhashable type: ‘slice” TypeError occurs when trying to use a ‘slice’ object as a key in a dictionary or as an element in a set. ‘Slice’ objects in Python are not hashable and cannot be used as keys or elements in hash-based data structures.
Q: How can I deal with the ‘unhashable type: ‘slice” TypeError in Python?
A: To deal with this TypeError, you can convert the ‘slice’ object into a tuple or a list using the ‘tuple()’ or ‘list()’ functions respectively before using it as a key or an element. Alternatively, consider using different data structures or approaches that do not involve ‘slice’ objects.
Q: What are some common causes of TypeErrors in Python?
A: Some common causes of TypeErrors in Python include mixing incompatible types, passing incorrect arguments to functions or methods, accessing elements that do not exist, and improper use of operators.
Q: How can I prevent TypeErrors in Python?
A: To prevent TypeErrors, you can use type annotations, perform input validation, use descriptive variable names, write unit tests, and follow Python’s coding conventions. These practices help catch potential TypeErrors during development and ensure the code is robust and error-free.
In conclusion, the ‘unhashable type: ‘slice” TypeError is a specific type of TypeError that occurs when trying to use a ‘slice’ object as a key in a dictionary or as an element in a set. By understanding the underlying concepts of TypeErrors in Python and following the best practices, you can effectively prevent, handle, and debug TypeErrors in your code.
Python How To Fix Typeerror: Unhashable Type: ‘List’ (Troubleshooting #2)
What Is Unhashable Type Slice?
In the world of programming, developers often come across the term “unhashable type slice.” This concept is specifically related to Python programming language and understanding it can greatly enhance your coding skills. Essentially, an unhashable type slice refers to the inability to hash a particular data structure known as a slice.
To comprehend what an unhashable type slice is, we first need to grasp the concept of hashable and unhashable types. In Python, a hashable type is an object that has a hash value which remains constant throughout its lifetime. This hash value is used as an identifier to quickly compare and look up objects, making it a crucial aspect of efficient programming. Examples of hashable types in Python include integers, floats, and strings.
On the other hand, an unhashable type is an object that does not have a fixed hash value or cannot be hashed at all. In Python, two types are inherently unhashable: mutable types (such as lists, sets, and dictionaries) and any object that contains mutable types. This is because mutable types can be changed, which would consequently alter their hash value, making them unusable for hashing purposes.
Now, let’s focus on the term “slice” in the context of Python programming. In Python, a slice is defined as a portion or subset of a sequence object (e.g., a list, tuple, or string). It allows you to extract a specific range of elements from the original sequence without modifying it. A slice is represented using a colon-separated syntax, such as `my_list[start:end]`.
However, slices themselves are not hashable types in Python. This is due to the fact that slices are dynamic and can change based on the original sequence. If the original sequence is modified, it may result in a different subset of elements within the slice. As a result, Python treats slices as unhashable types, preventing them from being used as keys in dictionaries or elements in sets.
For instance, consider the following code snippet:
“`
my_list = [1, 2, 3, 4, 5]
my_dict = {my_list[1:3]: “slice object”}
“`
Executing this code will generate a `TypeError` with the message “unhashable type: ‘slice’.” This occurs because the slice `my_list[1:3]` is being used as a key for the dictionary `my_dict`, which is not allowed due to its unhashable nature.
FAQs
Q: Can I ever use a slice as a key in Python dictionaries?
A: No, slices cannot be used directly as keys in Python dictionaries. You need to convert them to hashable types, such as tuples, to use them as keys.
Q: Are slices unhashable only in Python?
A: Slices being unhashable is specific to Python. Other programming languages may have different rules regarding the hashability of slices or similar constructs.
Q: How can I convert a slice into a hashable type?
A: To convert a slice into a hashable type, you can wrap it in a tuple. For example, `(my_list[1:3],)` would create a hashable representation of the slice `my_list[1:3]`.
Q: Can I modify a slice in Python?
A: No, slices are read-only and do not allow modifications to the original sequence. Any attempt to modify a slice directly will raise a `TypeError`.
Q: What are the alternatives to using slices as dictionary keys?
A: Instead of using slices as keys, you can consider using other hashable types like tuples, strings, or integers. Alternatively, you can restructure your code to not rely on slices as keys in the first place.
In conclusion, an unhashable type slice in Python refers to the inability to hash slices due to their dynamic nature. By understanding the concept of hashable and unhashable types, as well as the characteristics of slices, developers can write more efficient and error-free code. Remember that when dealing with slices in Python, it’s crucial to convert them into hashable types before using them as dictionary keys.
What Is Unhashable Type Type Error?
In programming, it is common to encounter various types of errors while writing code. One such error is the “Unhashable Type Error.” This article aims to explain what an unhashable type error is, how it occurs, and how to resolve it.
Understanding Hashing
To comprehend the unhashable type error, it is first necessary to have a basic understanding of hashing. Hashing is a fundamental concept in programming that involves turning an input (such as a string or object) into a fixed-size value. This value, known as a hash, is unique to the input provided. The idea behind hashing is to create a faster way to access data or determine equality.
Hashable Types
In Python, hashable types are those that can be converted into a hash value effortlessly. Immutable data types such as numbers (integers, floats), strings, and tuples fall under the category of hashable types. On the other hand, mutable data types like lists and dictionaries are not hashable since their contents can be changed, making it difficult to maintain a consistent hash value.
The Unhashable Type Error
When attempting to use an unhashable type within a context that requires hashable types, Python raises the “unhashable type error.” This error occurs when dynamic data structures that require hashable elements, such as sets or dictionaries, encounter an unhashable object.
For instance, consider the following code snippet:
my_set = {1, 2, 3}
my_list = [4, 5, 6]
my_set.add(my_list)
In the above example, an attempt is made to add a list (unhashable) to a set (hashable). Since lists are mutable in Python, they are unhashable and cannot be added to a set. This will result in a “Type Error: unhashable type: ‘list'” being raised.
Similarly, if we were to use a dictionary as a key for another dictionary or a set, we would encounter the same unhashable type error.
How to Resolve the Unhashable Type Error
To resolve the unhashable type error, it is necessary to ensure that only hashable types are used when dealing with hash-dependent data structures. Here are a few approaches you can take:
1. Change the unhashable type: If the unhashable object used is mutable, consider modifying it to make it hashable. For example, if a list is causing the error, consider converting it to a tuple, a hashable alternative.
2. Use hashable elements: When using hash-dependent data structures, ensure that all elements within them are hashable types. This may involve reevaluating the data or using a different type of data structure.
3. Implement custom hashing: If the unhashable type is an instance of a custom class, you can define a custom hash function by overriding the __hash__() method. This allows you to specify how an object of that class should be hashed.
4. Consider alternative data structures: If your code requires mutable objects and hash functions simultaneously, it might be worth considering alternative data structures that accommodate your specific needs, such as lists or custom implementations.
FAQs about Unhashable Type Error
Q: Can I hash any Python object?
A: No, not all Python objects are hashable. Only immutable objects, such as numbers, strings, and tuples, can be hashed.
Q: Can I hash a dictionary?
A: No, dictionaries in Python are mutable and, therefore, unhashable. However, you can use tuples as keys instead.
Q: How do I know if an object is hashable?
A: You can use the built-in function hash() on the object. If it successfully returns a hash value, then the object is hashable. Otherwise, an error will be raised.
Q: I am getting an unhashable type error, but I’m not using a hash-dependent data structure. Why?
A: Although the error is commonly encountered when using hash-dependent structures like sets or dictionaries, it can also occur in other cases. Some libraries or functions may internally rely on hashability, leading to this error.
Q: Why does Python require hashable types for certain operations?
A: Hashing allows for efficient data retrieval and comparison. By requiring hashable types, Python ensures consistent and predictable behavior for hash-dependent data structures.
In Conclusion
The unhashable type error is a common error in Python that occurs when an unhashable type is used in a context that requires hashable types. By understanding the concepts of hashing and hashable types, you can effectively troubleshoot and resolve this error. Remember to ensure all elements used in hash-dependent structures are hashable, or consider alternatives if mutable objects are necessary.
Keywords searched by users: typeerror: unhashable type: ‘slice’ Unhashable type slice pika, Slice dictionary Python
Categories: Top 38 Typeerror: Unhashable Type: ‘Slice’
See more here: nhanvietluanvan.com
Unhashable Type Slice Pika
When programming in Python, you may come across the dreaded “TypeError: unhashable type: ‘slice'” error. This error typically occurs when you try to use a slice object as a key in a dictionary or a set. In this article, we will explore this error in detail, understand why it occurs, and explore possible solutions.
To fully grasp the unhashable type slice error, it is crucial to understand what hashable types are in Python. A hashable type is an object that has a hash value which remains constant throughout its lifetime. Immutable objects like integers, strings, and tuples are hashable, as they do not change their values once created. On the other hand, objects like lists, dictionaries, and sets are mutable and not hashable.
Slices, denoted using the colon (:) operator, are often used to extract a portion of a sequence, such as a list or a string. Since slices, by nature, work on mutable sequences, they themselves are not hashable. Therefore, attempting to use a slice as a key in a dictionary or set will raise the “unhashable type slice” error.
Here’s an example scenario to clarify the error:
“`python
my_dict = {(1, 2): “Hello”, [3:5]: “World”}
“`
Upon executing the above code, you will encounter the unhashable type slice error, as Python is unable to hash the slice object [3:5]. To resolve this, you must find an alternative strategy.
One common solution is to convert the slice object into a tuple or a string, which are hashable types. This can be achieved by applying the tuple() or str() functions to the slice object, respectively. Consider the following updated code:
“`python
my_dict = {(1, 2): “Hello”, tuple([3:5]): “World”}
“`
In this revised example, we have converted the slice [3:5] into a tuple using the tuple() function, allowing us to use it as a key in the dictionary. However, it is important to note that this approach will only work if the slice does not change frequently.
Alternatively, if you have a situation where you must frequently modify the slice, you can consider replacing the slice with a tuple or another hashable object altogether. By designing your code around hashable objects, you can avoid the unhashable type slice error altogether.
Now, let’s move on to a section covering some frequently asked questions related to the unhashable type slice error:
Q1. Can I use a slice as a key in a dictionary or set?
A1. No, a slice object is not hashable and cannot be directly used as a key. You need to convert the slice into a hashable type, such as a tuple or a string, before using it as a key in a dictionary or a set.
Q2. Why are slices not hashable in Python?
A2. Slices are not hashable because they are mutable. Hashable types in Python must be immutable, meaning they cannot change their values after creation. Slices are designed to work on mutable sequences, hence making them mutable as well.
Q3. Are there any alternatives to using slices in Python?
A3. Yes, there are alternatives to using slices in Python. You can consider using list comprehensions or functional programming constructs like map or filter to achieve similar results. It ultimately depends on the specific use case and the level of mutability required.
Q4. Can I use other mutable objects as keys in a dictionary or set?
A4. No, you cannot directly use other mutable objects like lists or dictionaries as keys in a dictionary or set. Their mutability makes them unhashable. However, you can convert them into hashable types, such as tuples, before using them as keys.
In conclusion, the unhashable type slice error can be a daunting obstacle when working with slices in Python. By understanding its cause and utilizing the appropriate strategies, such as converting slices into hashable types, you can overcome this error and continue writing robust Python code. Remember, adapting your code to work with hashable objects is key to solving the unhashable type slice error, allowing for smoother execution of your Python programs.
Slice Dictionary Python
Python has gained popularity among developers for its simplicity and versatility. One of the powerful features it offers is the ability to slice and manipulate data structures, including dictionaries. In this article, we will explore the concept of slice dictionaries in Python, delve into various techniques to slice dictionaries, and discuss some frequently asked questions to help you understand and use this feature effectively.
Understanding Dictionaries in Python
Before diving into slicing dictionaries, let’s have a brief overview of dictionaries in Python. A dictionary is an unordered collection of key-value pairs enclosed in curly brackets {}. Each key within a dictionary must be unique, and it can be of various data types, including integers, strings, or even tuples. Values associated with the keys can be of any data type, making dictionaries a flexible and powerful tool for managing data.
Slicing Dictionaries: The Basics
Slicing dictionaries allows us to extract a subset of key-value pairs based on specific criteria. It can be done using the slice notation [start:stop:step], where start, stop, and step are optional arguments. However, unlike slicing lists or strings in Python, dictionaries are inherently unordered, so slicing operations do not consider the ordering of key-value pairs.
Techniques for Slicing Dictionaries
To effectively slice dictionaries, there are various techniques available in Python. Let’s explore some of them below:
1. Slice based on keys:
We can extract a subset of key-value pairs by specifying a range of keys. This can be achieved using the built-in function “sorted” on the dictionary, followed by slicing the resulting list. For example:
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3, ‘d’: 4, ‘e’: 5}
sorted_keys = sorted(my_dict)
sliced_dict = {k: my_dict[k] for k in sorted_keys[1:3]} # Slice from the second key to the third key
“`
In the above code, the resulting sliced_dict will contain key-value pairs corresponding to keys ‘b’ and ‘c’, excluding the first and the last keys.
2. Slice based on values:
We can slice dictionaries based on the values they hold. This involves using the “items” method, which returns a collection of key-value pairs. We can then apply a condition to select values of interest. Let’s consider an example:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘grape’: 10, ‘mango’: 7}
sliced_dict = {k: v for k, v in my_dict.items() if v > 5} # Slice where values are greater than 5
“`
In the above code, sliced_dict will contain the key-value pairs where the values are greater than 5.
3. Slice using keys and values simultaneously:
We can combine the previous techniques to create complex slices based on both keys and values. For instance:
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3, ‘d’: 4, ‘e’: 5, ‘f’: 6}
common_keys = [‘b’, ‘c’, ‘d’] # Select specific keys
sliced_dict = {k: v for k, v in my_dict.items() if k in common_keys and v % 2 == 0} # Slice using keys and even values
“`
In the above code, we select specific keys using the common_keys list and select key-value pairs where the value is even, resulting in the sliced_dict.
FAQs: Frequently Asked Questions
Q1. Can we change the order of key-value pairs while slicing a dictionary?
Answer: No, dictionaries in Python are unordered, and slicing operations do not consider the ordering of key-value pairs. To maintain order, we can use alternative data structures like OrderedDict.
Q2. Are there any restrictions on the data types of keys in a sliced dictionary?
Answer: No, the data types of keys in a sliced dictionary remain the same as the original dictionary. However, keep in mind that keys within a dictionary must be unique.
Q3. Is it possible to slice nested dictionaries in Python?
Answer: Yes, nested dictionaries can be sliced using similar techniques by applying conditions on multiple levels. However, the complexity increases as you need to navigate through nested keys.
Q4. Can we add or remove key-value pairs while slicing?
Answer: Technically, it is not slicing if you add or remove key-value pairs. Slicing is the process of extracting a subset of data, not modifying it. You can create a new dictionary with the desired key-value pairs instead.
Q5. Are there any built-in functions specifically designed for slicing dictionaries?
Answer: No, Python does not provide built-in functions exclusively for slicing dictionaries. However, by utilizing the methods provided, such as “sorted”, “items”, and certain conditional statements, effective slicing operations can be achieved.
Conclusion
Slicing dictionaries in Python provides a flexible and efficient way to extract specific subsets of key-value pairs based on certain criteria. By using techniques like slicing based on keys or values, or combining conditions, we can effectively manipulate dictionaries. However, it is important to remember that dictionaries are unordered, and slicing operations do not consider the original ordering of key-value pairs. With these insights, you can harness the power of slicing dictionaries in Python to efficiently handle complex data structures.
Images related to the topic typeerror: unhashable type: ‘slice’
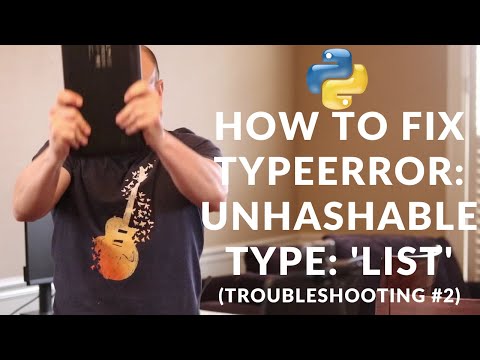
Found 24 images related to typeerror: unhashable type: ‘slice’ theme

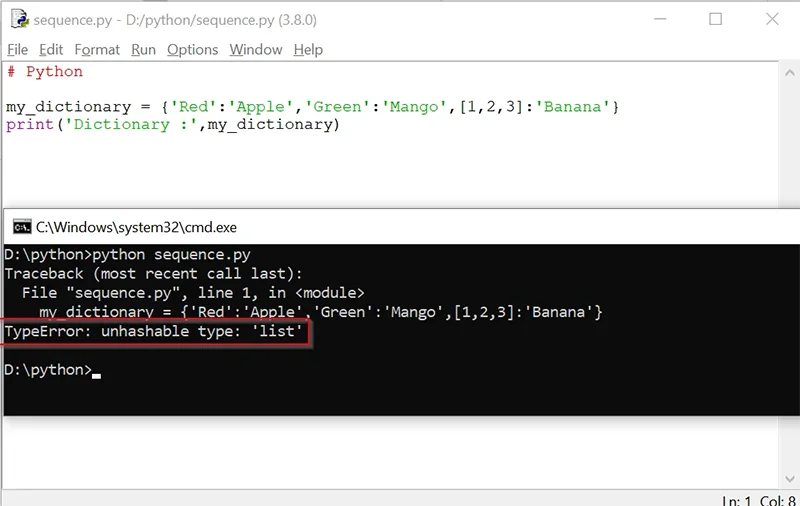
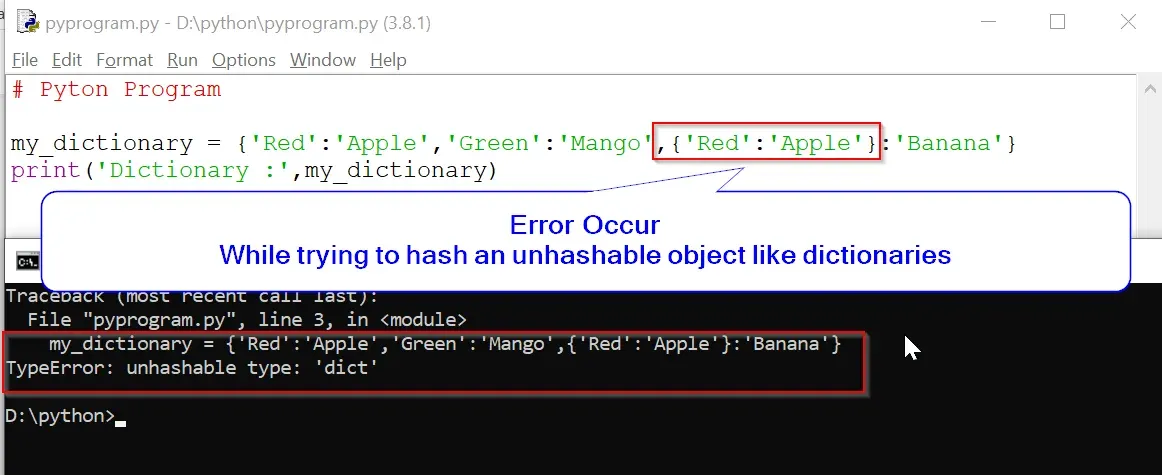

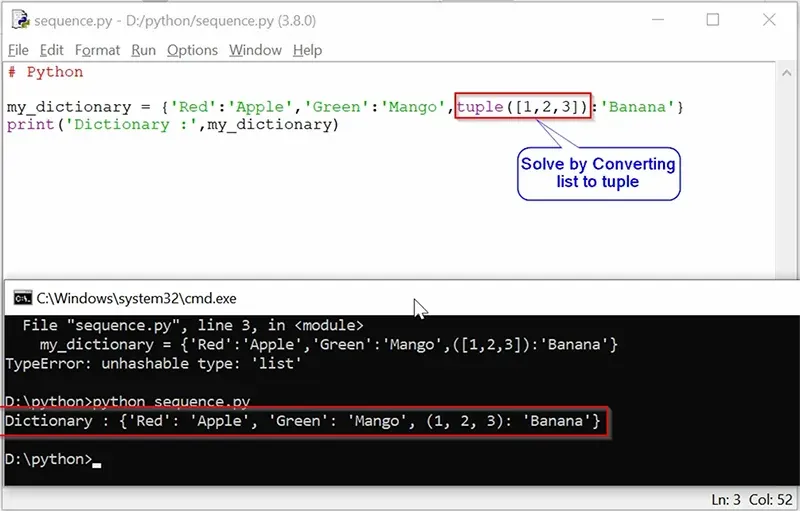

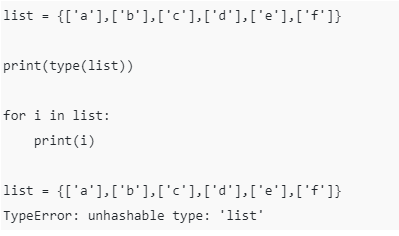
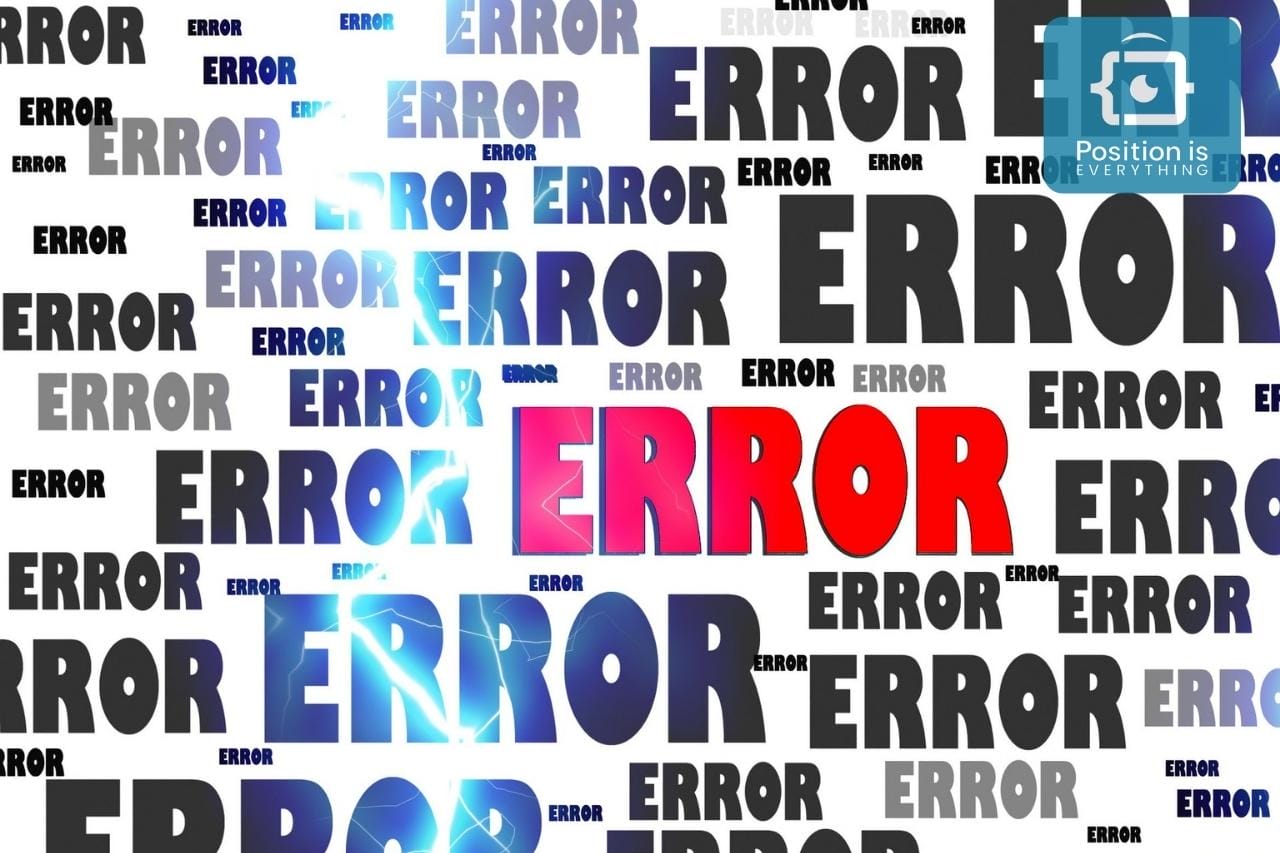
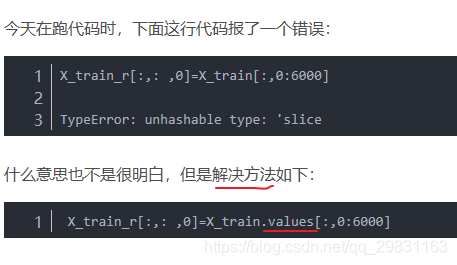

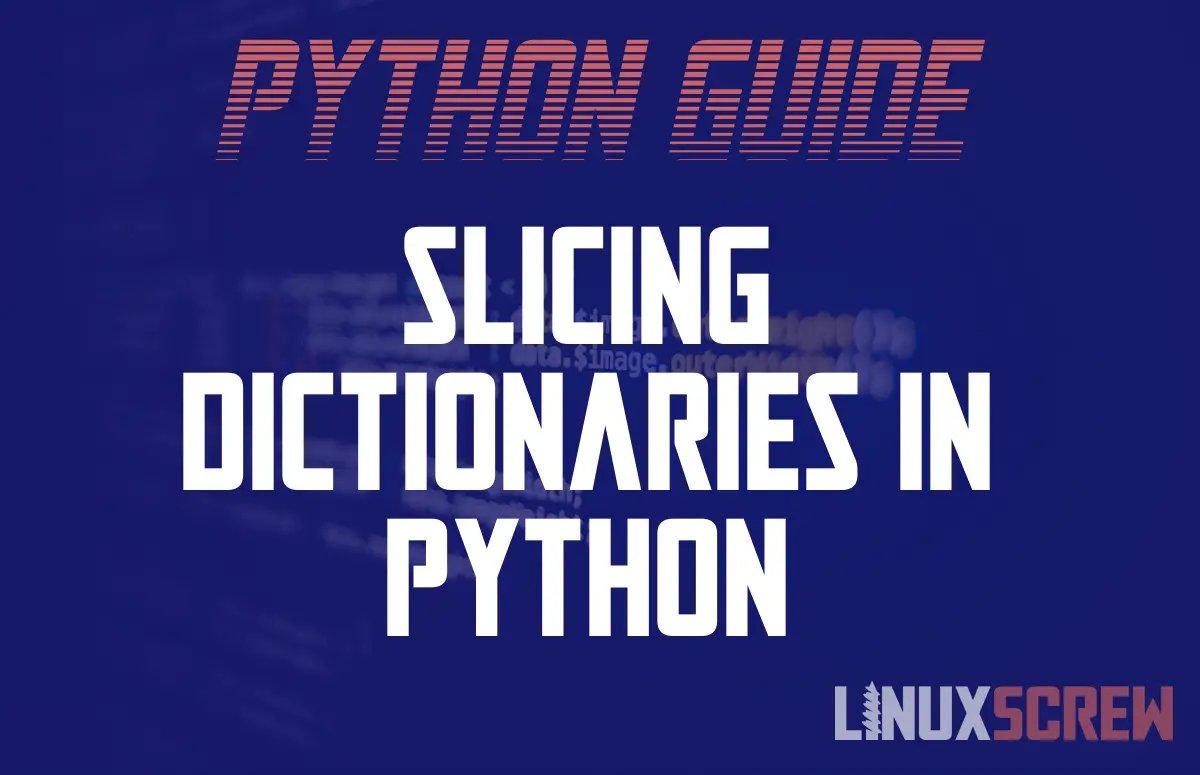
![Typeerror: unhashable type: 'series' [SOLVED] Typeerror: Unhashable Type: 'Series' [Solved]](https://itsourcecode.com/wp-content/uploads/2023/04/Typeerror-unhashable-type-series.png)
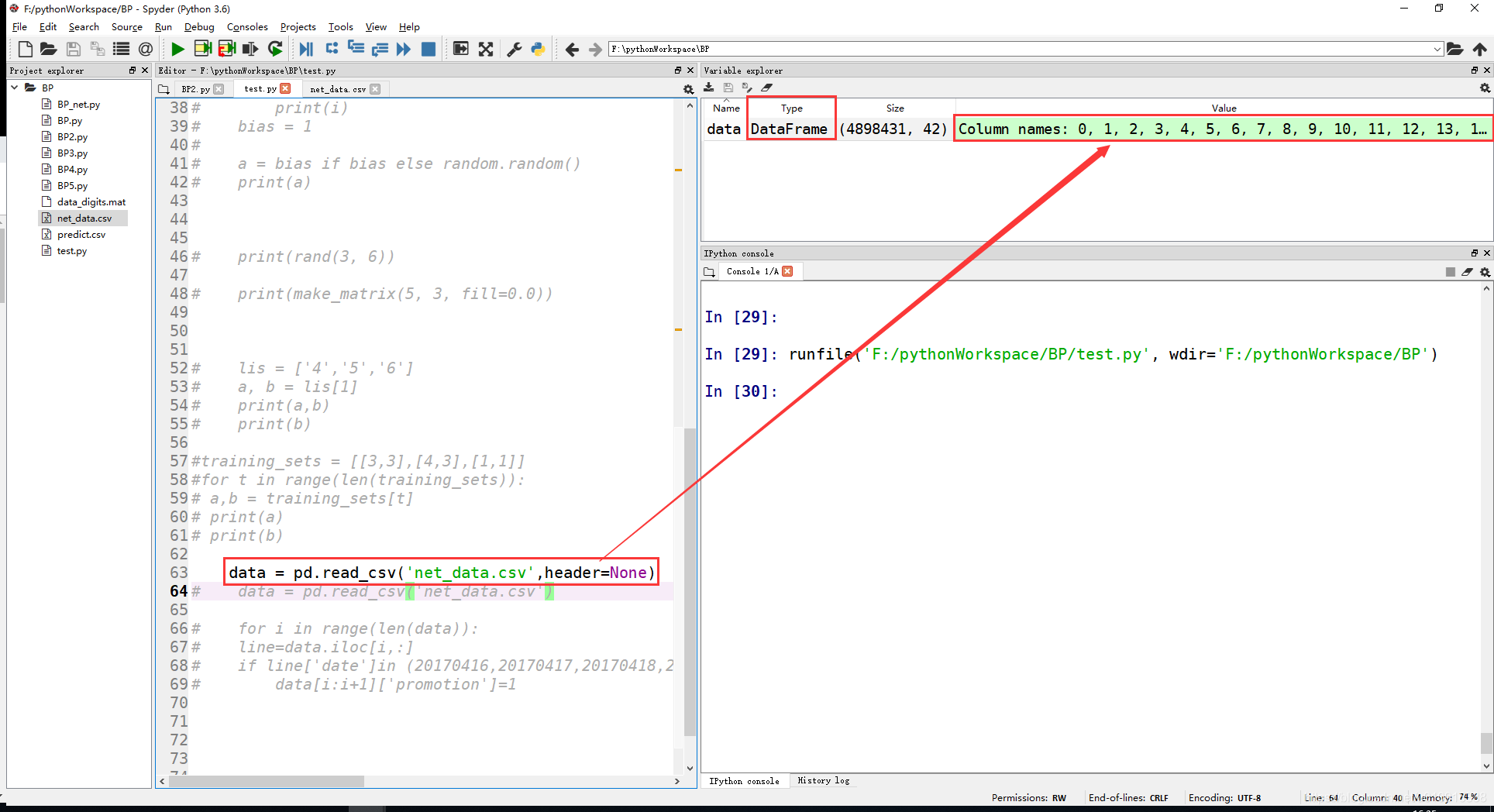
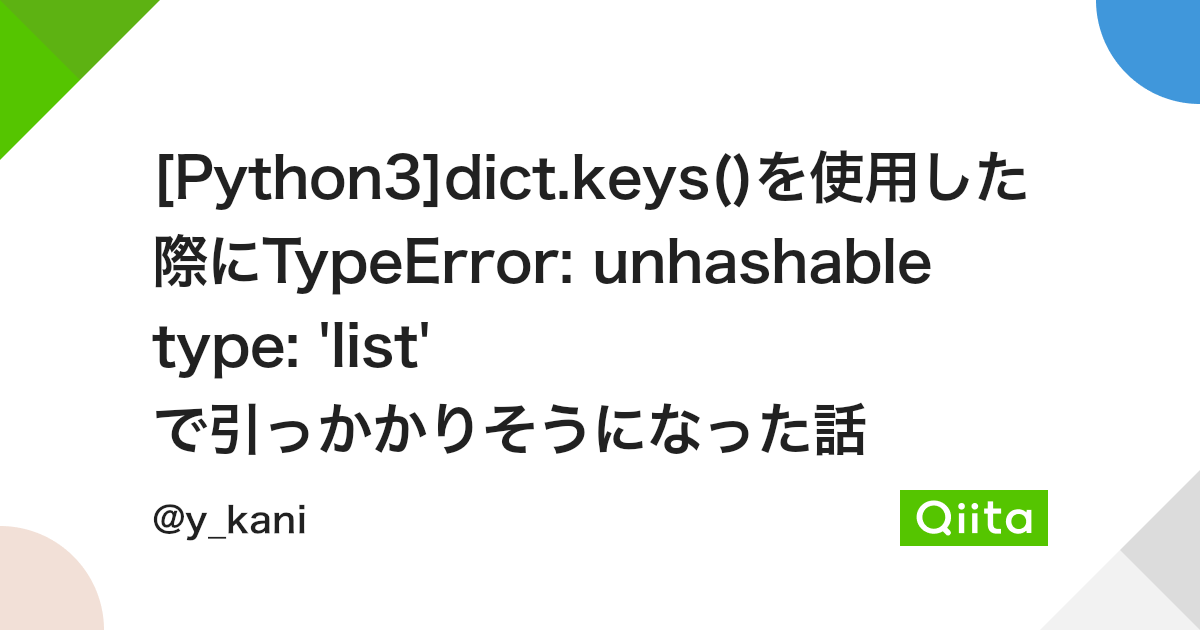
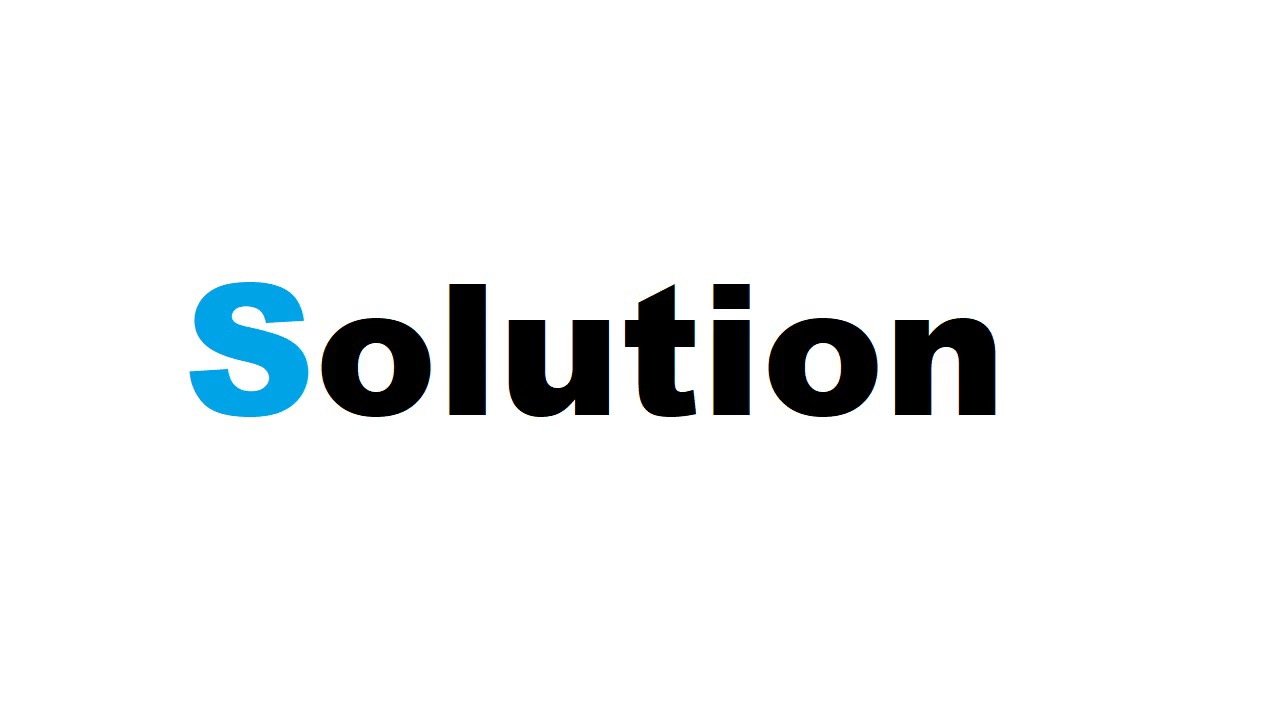

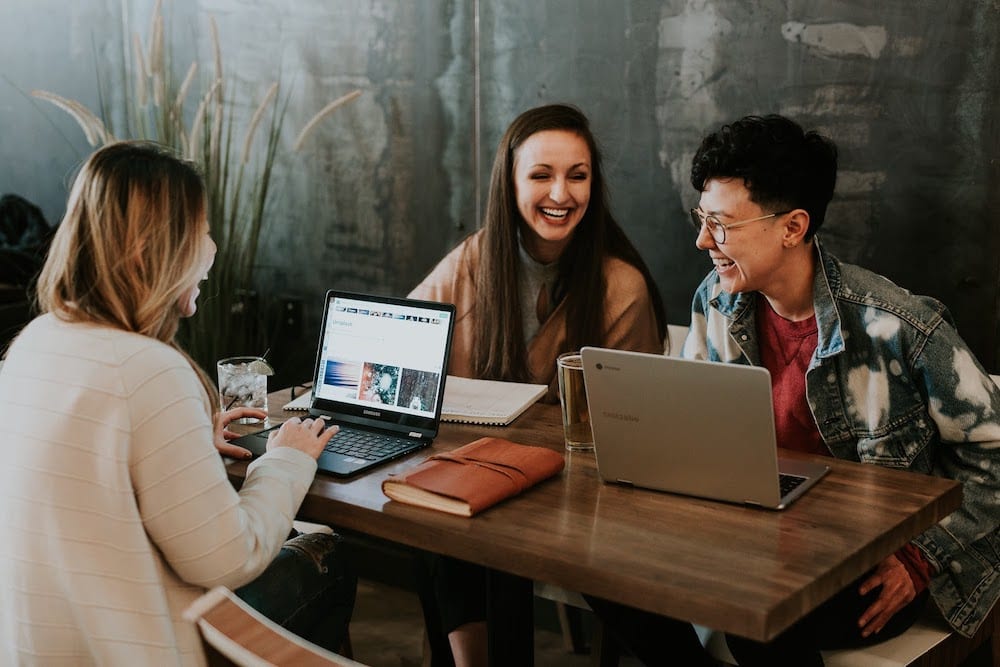
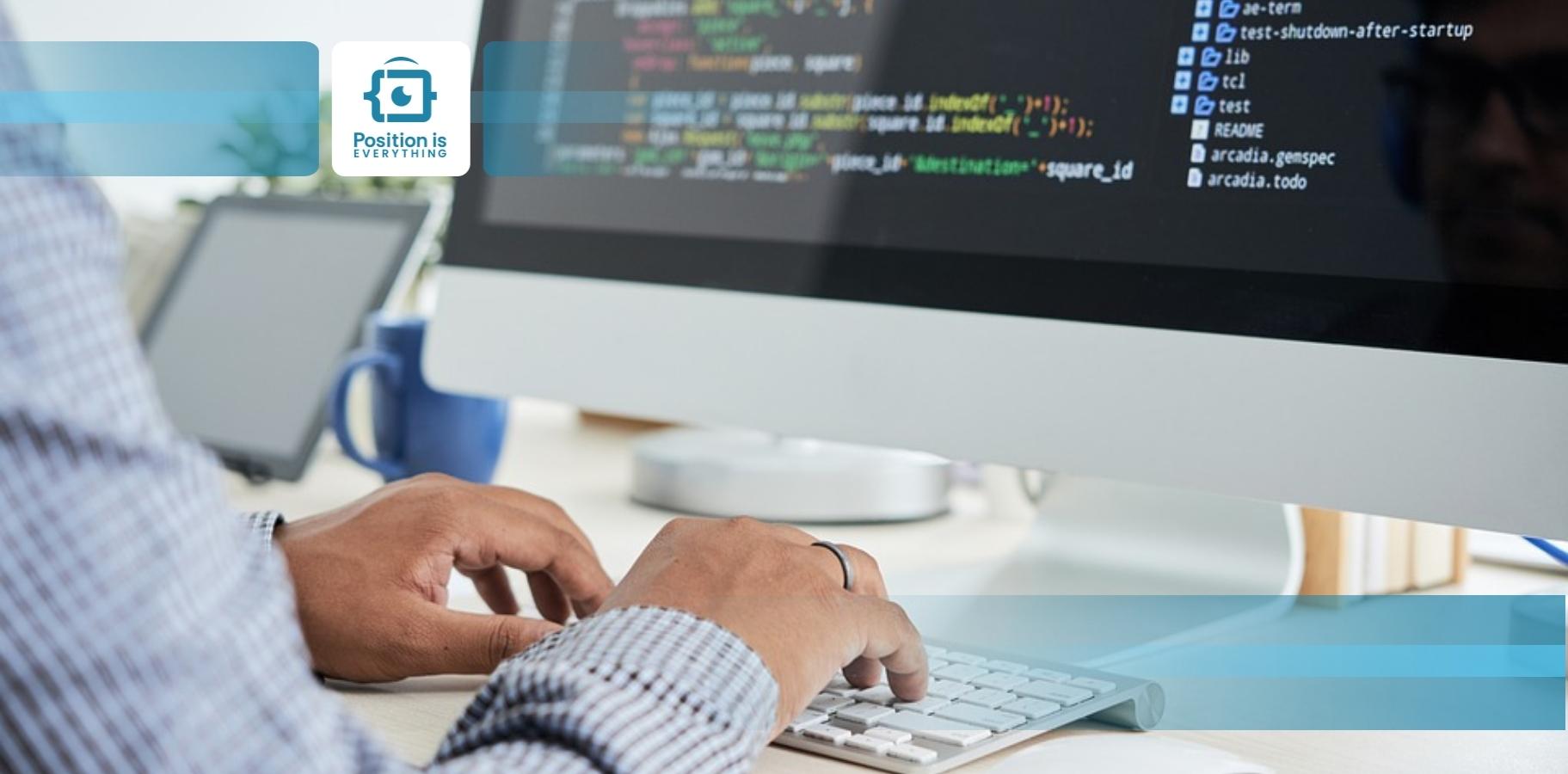

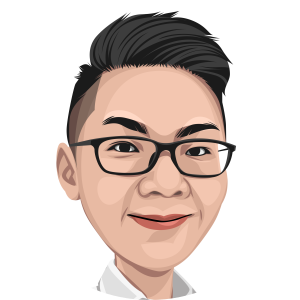
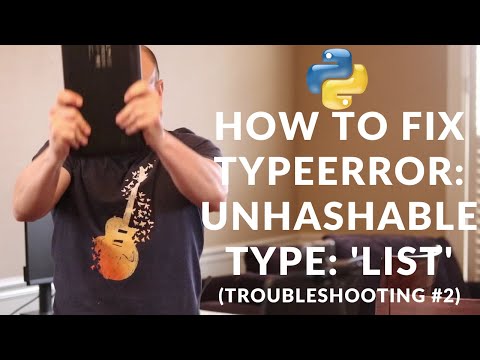

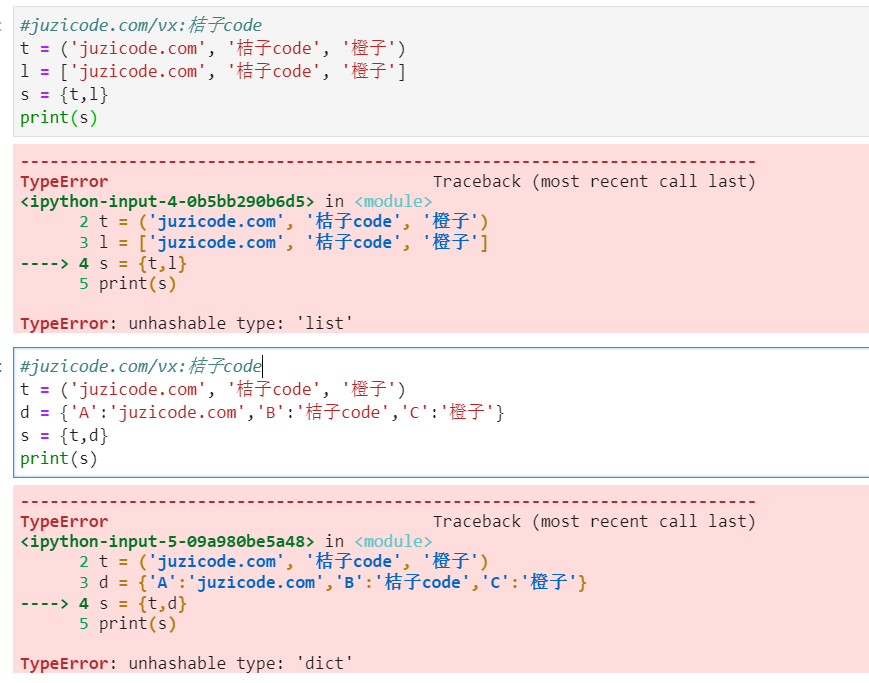
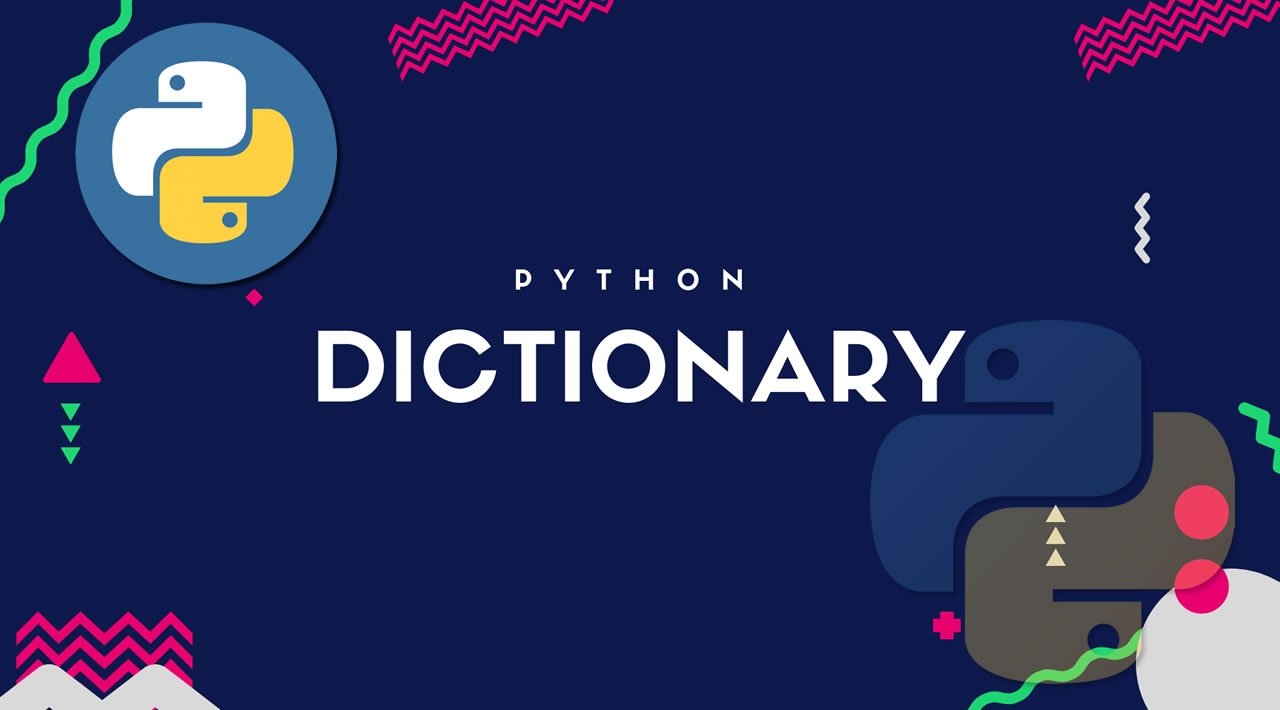

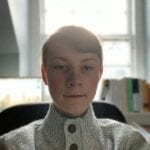
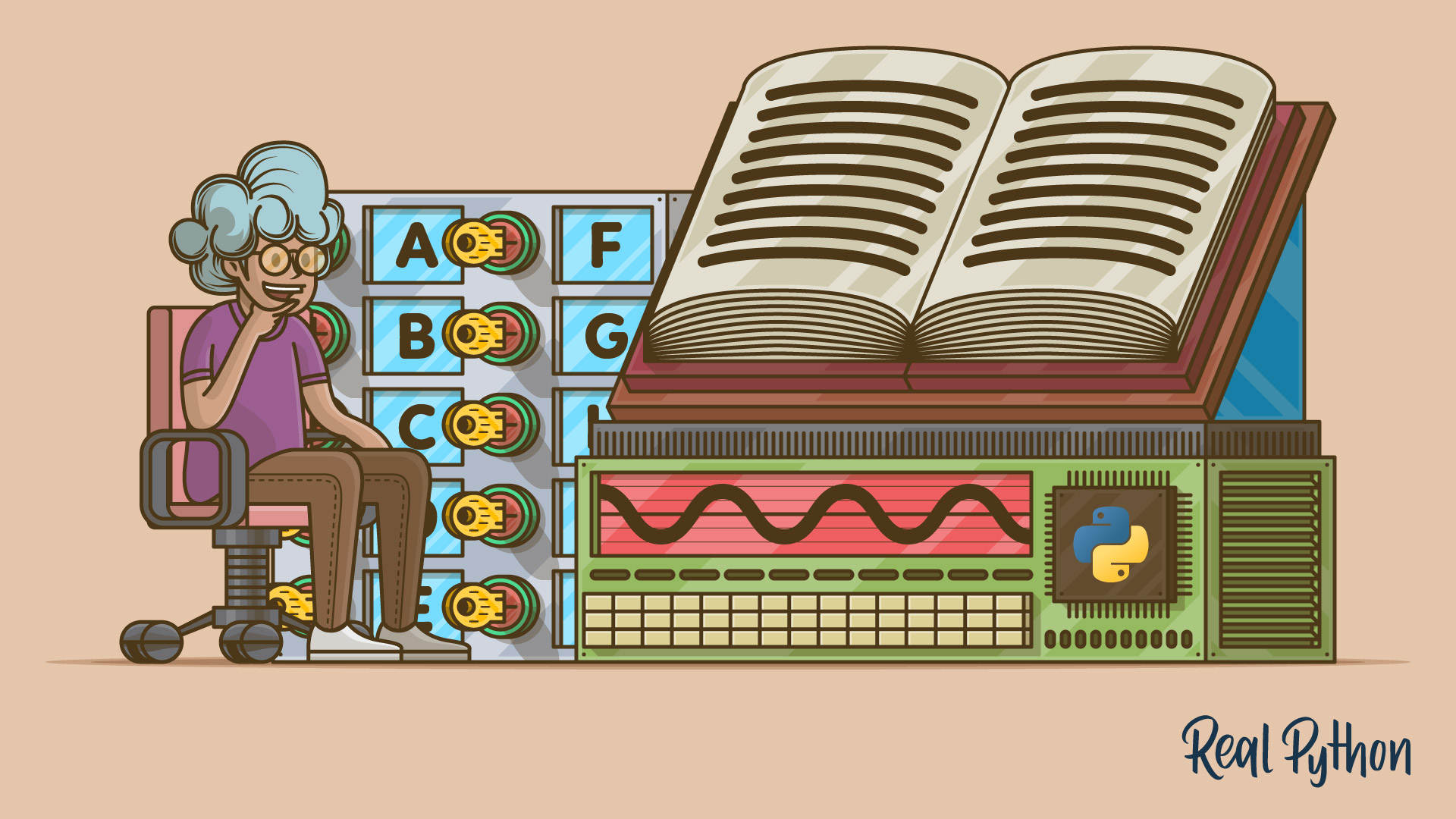
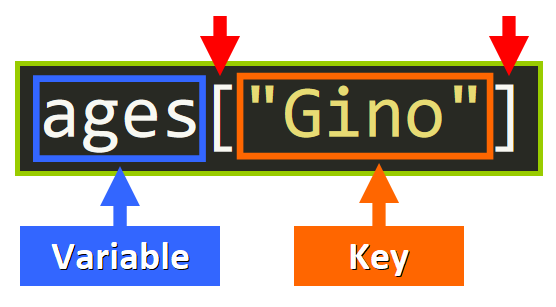
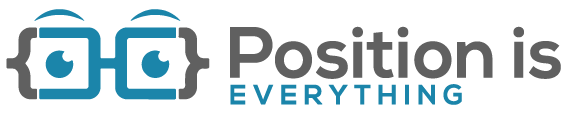
Article link: typeerror: unhashable type: ‘slice’.
Learn more about the topic typeerror: unhashable type: ‘slice’.
- Python TypeError: unhashable type: ‘slice’ Solution
- Python “TypeError: unhashable type: ‘slice'” for encoding …
- TypeError: unhashable type ‘slice’ in Python [Solved]
- [Solved] TypeError: Unhashable Type: ‘slice’ – Python Pool
- How to fix TypeError: unhashable type: ‘slice’ – sebhastian
- How to Handle TypeError: Unhashable Type ‘Dict’ Exception in …
- TypeError : Unhashable type – python – Stack Overflow
- How to fix TypeError: unhashable type: ‘slice’ – sebhastian
- How To Fix TypeError: unhashable type ‘slice’
- TypeError: Unhashable Type: Slice in Python – Delft Stack
- Typeerror: unhashable type: ‘slice’ – Itsourcecode.com
- GooglePlayValidator – unhashable type: ‘slice’ #78 – GitHub
- Unhashable type: ‘slice’ – fastai – fast.ai Course Forums
See more: https://nhanvietluanvan.com/luat-hoc