Typeerror Unhashable Type Numpy.Ndarray
The TypeError ‘unhashable type: numpy.ndarray’ is a common error encountered by programmers when working with the NumPy library in Python. This error occurs when attempting to use a NumPy ndarray object as a key in a dictionary or as an element in a set, which requires the object to be hashable. In this article, we will explore the reasons behind this error, provide an explanation of the NumPy ndarray, delve into the reasons why it is unhashable, and discuss possible ways to resolve the TypeError.
Explanation of TypeError:
Before diving into the specifics, let’s first understand what a TypeError is in Python. A TypeError is raised when an operation or function is attempted on an object of an inappropriate type. It indicates that the types of operands or arguments used in a particular context are not compatible, resulting in an inability to perform the desired operation.
Reasons for TypeError:
TypeError occurs in various scenarios and can have multiple causes. In the case of the error message ‘unhashable type: numpy.ndarray’, it is specifically related to the hashability of the NumPy ndarray object. The concept of hashability is a crucial aspect of Python’s built-in data structures, such as dictionaries and sets. These data structures rely on hash functions to efficiently store and retrieve elements.
Understanding Unhashable Types:
In Python, a hashable object is one that has a hash value that never changes throughout its lifetime and can be compared to other objects. Immutable objects like integers, floats, strings, and tuples are hashable by default, as their values cannot be modified once created. On the other hand, mutable objects like lists, dictionaries, and NumPy ndarrays are unhashable.
Explanation of Numpy.ndarray:
NumPy, short for Numerical Python, is a powerful Python library widely used for scientific computing and numerical operations. It provides a multidimensional array object called ndarray, which is the fundamental building block for various mathematical and scientific computations. An ndarray represents a grid of values, where each element is of the same data type.
Reasons for Numpy.ndarray to be Unhashable:
NumPy ndarrays are mutable objects, meaning their contents can be modified after creation. This mutability prevents them from being hashable. If ndarray objects were allowed as dictionary keys or set elements, they could potentially change their values, which would lead to inconsistencies in the hash tables or sets. To maintain the integrity of hash-based operations, NumPy ndarrays are deliberately designed to be unhashable.
The inability to use ndarrays as keys or elements in dictionaries and sets might be inconvenient in certain situations, especially when dealing with large datasets. However, this design choice ensures the consistency and reliability of hash-based operations in Python.
How to Resolve TypeError:
1. Convert to a Hashable Type: If you need to use an ndarray as a key or element, you can convert it to a hashable type. One possible solution is to convert the ndarray to a tuple using the ‘tuple()’ function, as tuples are immutable and hashable. Keep in mind that this conversion might lead to increased memory usage.
2. Use Another Data Structure: If the use of a dictionary or set is not essential, consider using alternative data structures that do not rely on hashability. For example, a list can be used instead of a set to store ndarrays without encountering the TypeError.
3. Revisit the Design: Since NumPy ndarrays are intentionally designed to be unhashable, it might be worth reconsidering the design of your program or algorithm. Try to find alternative approaches that do not require the use of ndarrays as keys or elements.
Frequently Asked Questions (FAQs):
Q: Can I use an ndarray as a key in a dictionary if it is immutable?
A: No, even if the ndarray’s values are immutable, the object itself is mutable, making it unhashable. Only hashable objects, such as strings or tuples, can be used as dictionary keys.
Q: Why does Python require dictionary keys and set elements to be hashable?
A: Hash-based data structures like dictionaries and sets require hashability for efficient insertion, retrieval, and membership testing operations. Hash functions depend on the object’s hash value, which remains constant for hashable types.
Q: Are there any performance implications when converting ndarray to a tuple?
A: Converting an ndarray to a tuple can be memory-intensive, as it creates a new object in memory. It is recommended to consider the memory impact when working with large arrays.
Q: Can I modify the NumPy library to make ndarrays hashable?
A: Modifying the NumPy library to make ndarrays hashable is possible, but highly discouraged. It can introduce inconsistencies and undermine the functionality and reliability of hash-based operations throughout the entire Python ecosystem.
In conclusion, the TypeError ‘unhashable type: numpy.ndarray’ occurs when attempting to use a NumPy ndarray as a key in a dictionary or element in a set. This error is due to the inherent mutability of ndarrays and their deliberate design choice to be unhashable. To resolve this issue, one can convert the ndarray to a hashable type, utilize alternative data structures, or reconsider the program’s design. Understanding the reasons behind this error and the concepts of hashability in Python will help programmers navigate and address this common issue effectively.
Python How To Fix Typeerror: Unhashable Type: ‘List’ (Troubleshooting #2)
Why Is Numpy Array Not Hashable?
NumPy is a popular Python library widely used for scientific computing and numerical operations. It provides powerful data structures, such as arrays and matrices, along with a vast collection of functions for mathematical and statistical computations. However, one limitation of the NumPy library is that its arrays are not hashable. This means that NumPy arrays cannot be used as keys in dictionaries or elements in sets. In this article, we will explore the reasons behind this limitation and its implications in various scenarios.
Understanding Hashable Objects:
Before diving into the reasons why NumPy arrays are not hashable, it is essential to grasp the concept of hashable objects. In Python, hashable objects are those whose internal state can never change during their lifetime. Additionally, hashable objects must provide the `__hash__()` method, which allows them to be hashed.
The hash value of an object is an integer that represents its internal state. Hash values are used for efficient retrieval of objects in dictionaries and set operations. Hashable objects can be used as dictionary keys because their hash values remain constant, enabling efficient lookups. On the other hand, unhashable objects, like NumPy arrays, lack a fixed hash value.
Reasons for Unhashability of NumPy Arrays:
1. Mutability: One of the primary reasons why NumPy arrays are not hashable is their mutability. Mutable objects are those whose internal state can be modified after their creation. Unlike the built-in Python types, such as integers and strings, NumPy arrays can be altered by modifying elements or reshaping the array. Since hashable objects must have an immutable state, NumPy arrays do not meet this requirement.
2. Varying Internal Data Layout: NumPy provides support for arrays with various data types and dimensionalities. This flexibility requires arrays to have varying internal data layouts and representations, making it challenging to define a generic hash function. The calculation of a hash value would need to consider the specific data type, shape, and other characteristics of each array. Since different arrays would have different hash functions, it becomes impractical to support hashability for all possible array configurations.
3. Performance Implications: Hashing objects is a computationally expensive operation. Python dictionaries and sets heavily rely on efficient hashing algorithms to ensure speedy lookups and operations. Supporting hashability for NumPy arrays would require additional computational overhead to calculate the hash values for arrays, impacting the overall performance of dictionary and set operations.
Implications and Workarounds:
The unhashability of NumPy arrays can have various implications in practical scenarios. Here are a few situations where this limitation becomes apparent:
1. Dictionary Keys and Sets: As mentioned earlier, NumPy arrays cannot be directly used as keys in dictionaries or elements in sets due to their unhashability. If you attempt to use a NumPy array as a dictionary key, you will encounter a `TypeError` stating that the array is unhashable. Similarly, adding a NumPy array to a set will raise a `TypeError` as well.
Fortunately, there are workarounds available to overcome this limitation. One approach is to convert the NumPy array into an immutable object, such as a tuple. Tuples are hashable as long as all their elements are hashable. By converting the array to a tuple, you can work around the unhashability of NumPy arrays while retaining the necessary information. However, this conversion can incur additional memory and computational costs, so it should be used judiciously.
2. Caching and Memoization: Hashing plays a vital role in caching and memoization, which are important techniques for optimizing function calls by storing previously computed results. In scenarios where functions use NumPy arrays as inputs, their unhashability can hinder the effective use of caching mechanisms. Consequently, functions that operate on large NumPy arrays might need to rely on alternative caching mechanisms or modify their designs to accommodate arrays as inputs.
3. Unique Element Identification: Hashability is commonly used for identifying unique elements in a collection. While NumPy arrays can still be compared for equality, they cannot be directly used as keys in dictionaries or sets for element unicity checks. In such cases, it becomes necessary to explore alternative data structures or techniques, such as hashing derived features of the arrays or using custom classes to wrap the array data.
FAQs:
Q1. Can I use NumPy arrays as keys in my custom classes?
A1. Yes, you can use NumPy arrays as keys in your custom classes if you define a custom hashing mechanism for your classes. By implementing the `__hash__()` method for your class, you can ensure that specific NumPy arrays within your class have fixed hash values.
Q2. Are there any plans to make NumPy arrays hashable in the future?
A2. While NumPy continues to evolve, there are currently no plans to make NumPy arrays hashable. The reasons mentioned earlier, such as mutability and varying internal data layouts, pose challenges to implementing a generic hash function for all possible array configurations.
Q3. Are any alternative libraries available that offer hashable array-like structures?
A3. Yes, some libraries provide hashable alternatives to NumPy arrays. One such library is the `array` module from the Python standard library. However, the functionality and performance of these alternatives may differ from NumPy. It is essential to consider your specific requirements before switching to an alternative library.
In conclusion, NumPy arrays are not hashable primarily due to their mutability, varying internal data layouts, and performance implications. Although this limitation can introduce challenges in certain scenarios, workarounds exist to handle this unhashability effectively. By understanding the reasons behind this limitation, developers can navigate the complexities of working with NumPy arrays in Python successfully.
What Is Numpy Ndarray In Python?
NumPy is a powerful library in Python, widely used for numerical computing. It stands for Numerical Python and is a fundamental package for scientific computing with Python. One of its key features is the NumPy ndarray, short for n-dimensional array. The ndarray allows efficient computation and manipulation of large datasets, making it a crucial tool in data analysis, machine learning, and scientific research.
The ndarray object in NumPy is a multidimensional array of elements, all of the same type. It is similar to a Python list, but with additional capabilities and optimizations for numerical operations. The array can have any number of dimensions, allowing for complex mathematical computations on large datasets.
Creating an ndarray in NumPy is simple. We can utilize the ‘array’ function and pass the desired data to it. Here’s an example:
“`
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
“`
In this example, we created a one-dimensional ndarray containing the numbers 1 to 5. We can access individual elements of the array using indexing, just like we would with a regular Python list. In this case, ‘arr[0]’ would give us the value 1.
However, the real beauty of the NumPy ndarray lies in its ability to handle multi-dimensional data. We can create arrays with two or more dimensions using nested lists, as shown below:
“`
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
“`
In this example, we created a two-dimensional array with dimensions 2×3. We can access individual elements using indexing with multiple values, such as ‘arr[0, 1]’ to get the value 2.
The NumPy ndarray provides a wide range of functions and methods for data manipulation and computation. For example, we can perform mathematical operations on arrays, such as addition, subtraction, multiplication, and division. These operations are applied element-wise, which means that the corresponding elements in the arrays are operated on.
NumPy also offers built-in functions to perform common mathematical operations on arrays, like calculating the mean, median, or variance. These functions are optimized for performance, making them much faster compared to using pure Python implementations.
Furthermore, the ndarray allows easy reshaping and slicing of arrays, providing flexibility in handling complex data structures. We can reshape an array into different dimensions using the `reshape` method, enabling us to perform operations on different parts of the array separately. Slicing allows us to extract a subset of elements from an array based on specified index ranges.
Frequently Asked Questions (FAQs):
Q: What is the advantage of using NumPy ndarray over regular Python lists?
A: NumPy ndarrays provide a more efficient and convenient way to work with numerical data. They are optimized for numerical operations, which makes them considerably faster compared to regular Python lists. Additionally, ndarrays support multi-dimensional data structures and provide a wide range of mathematical functions and methods for data manipulation.
Q: Can I perform mathematical operations between ndarrays of different dimensions?
A: No, mathematical operations between ndarrays require them to have compatible dimensions. The dimensions must either be equal or one of them must be 1, in which case the operation is applied across all elements.
Q: Can I mix different data types in a NumPy ndarray?
A: No, ndarrays require all elements to have the same data type. However, NumPy provides a wide range of data types to choose from, including integers, floating-point numbers, complex numbers, and more.
Q: How can I transpose a NumPy ndarray?
A: You can transpose an ndarray using the `T` attribute or the `transpose` function. For example, `arr.T` would transpose the array `arr`. The `transpose` function allows specifying the axes order.
Q: Can I save and load ndarrays to/from disk?
A: Yes, NumPy provides functions to save ndarrays to disk using formats like text, binary, or NumPy’s proprietary format. The `numpy.save` and `numpy.load` functions can be used for this purpose.
In conclusion, the NumPy ndarray is a powerful data structure for handling numerical data in Python. Its ability to efficiently manipulate and compute large datasets makes it an essential tool for various scientific and computational tasks. By leveraging its capabilities, data analysts, machine learning practitioners, and scientists can work with complex data structures easily, while achieving optimal performance and efficiency.
Keywords searched by users: typeerror unhashable type numpy.ndarray
Categories: Top 25 Typeerror Unhashable Type Numpy.Ndarray
See more here: nhanvietluanvan.com
Images related to the topic typeerror unhashable type numpy.ndarray
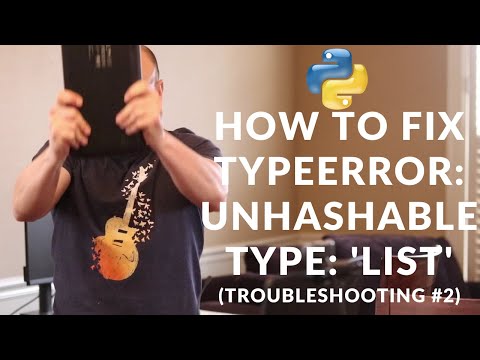
Found 10 images related to typeerror unhashable type numpy.ndarray theme


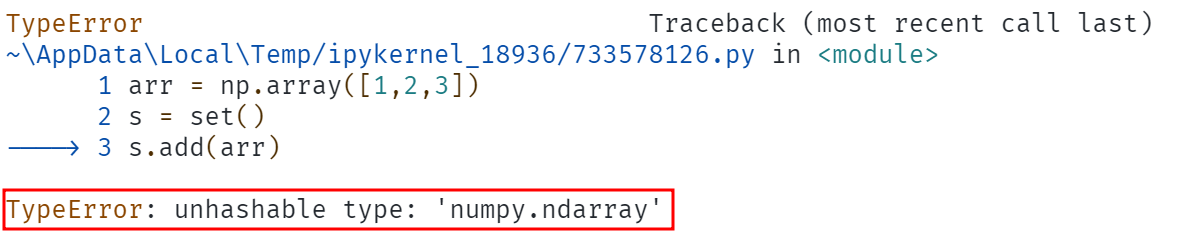
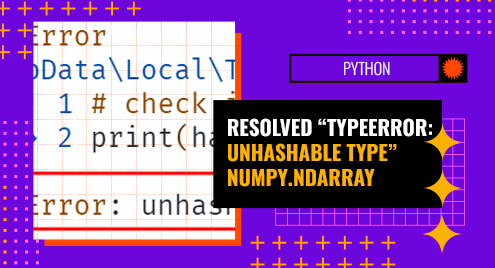


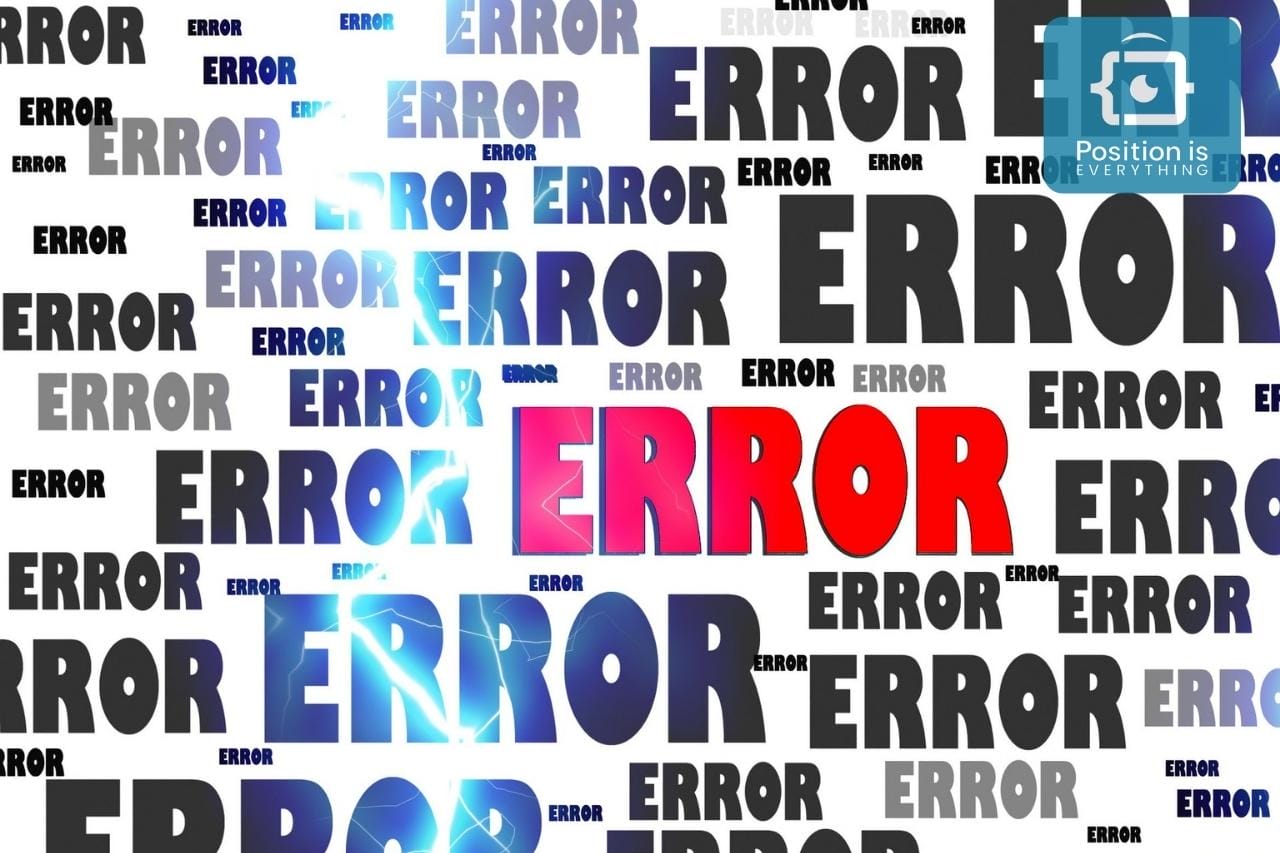


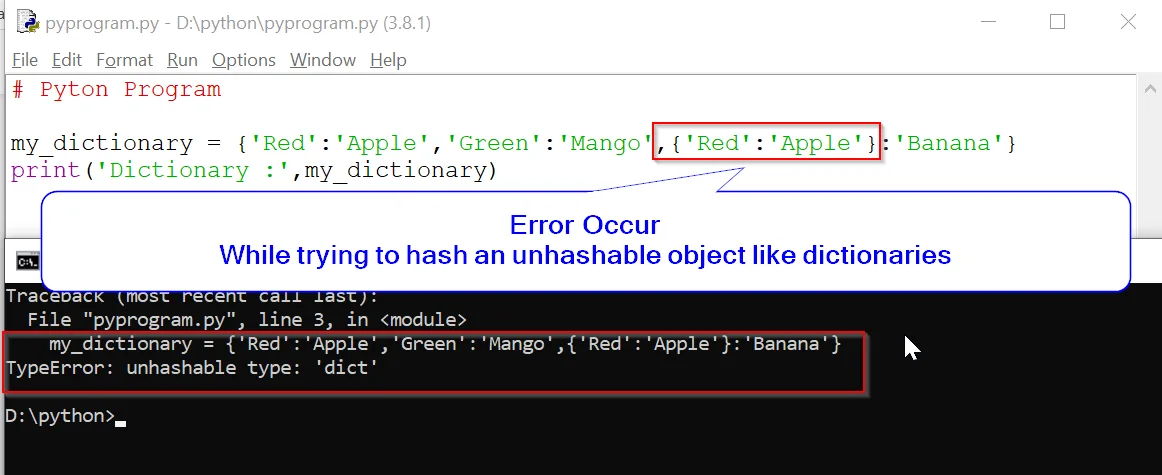
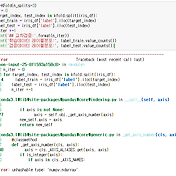




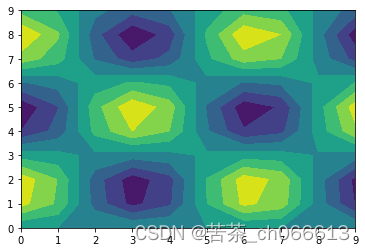

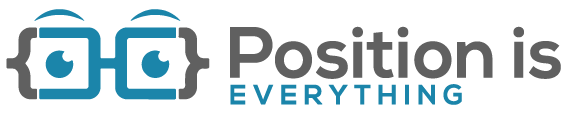
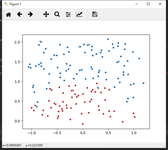
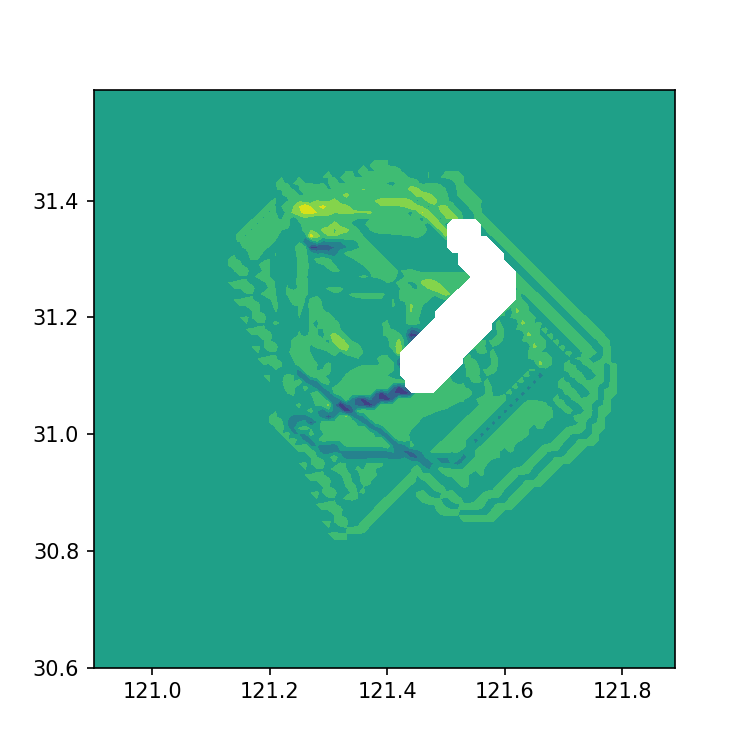
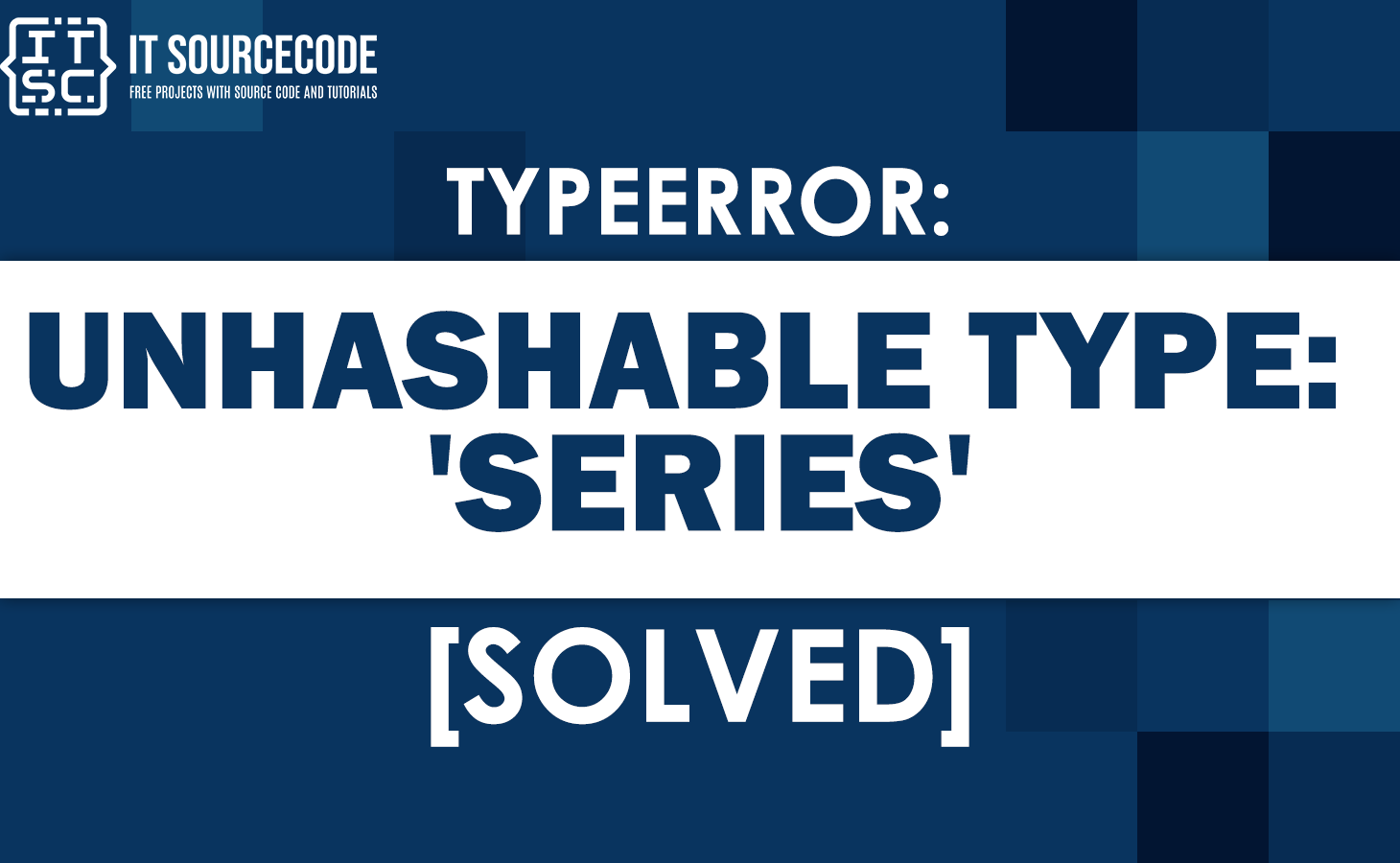



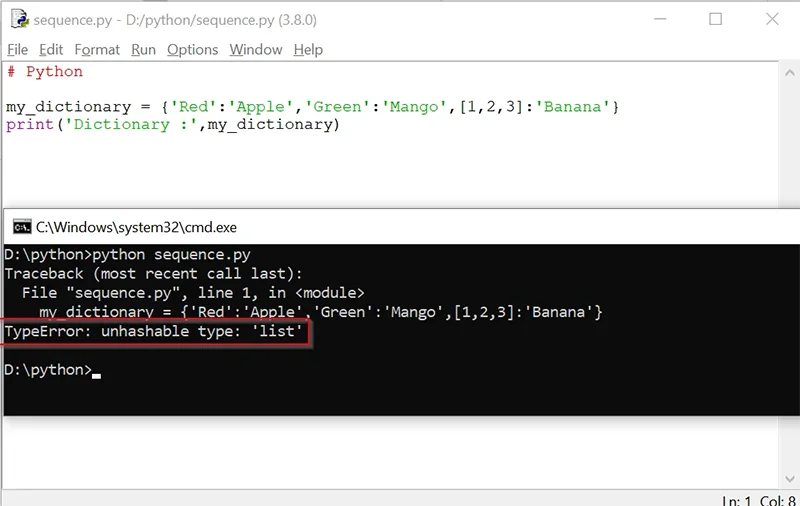

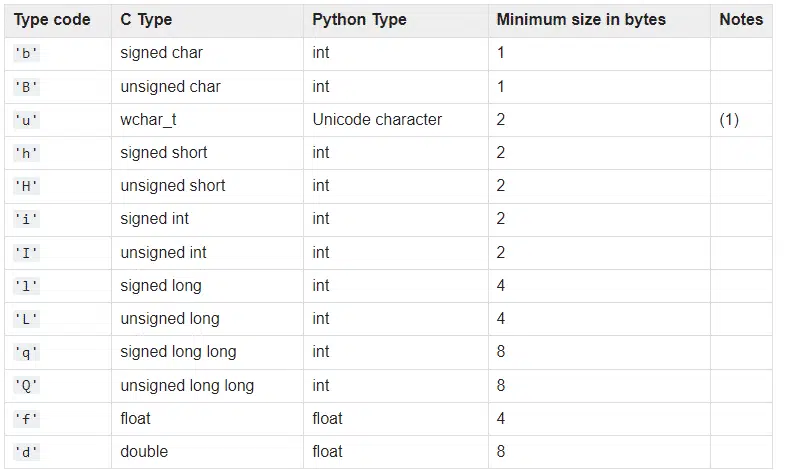
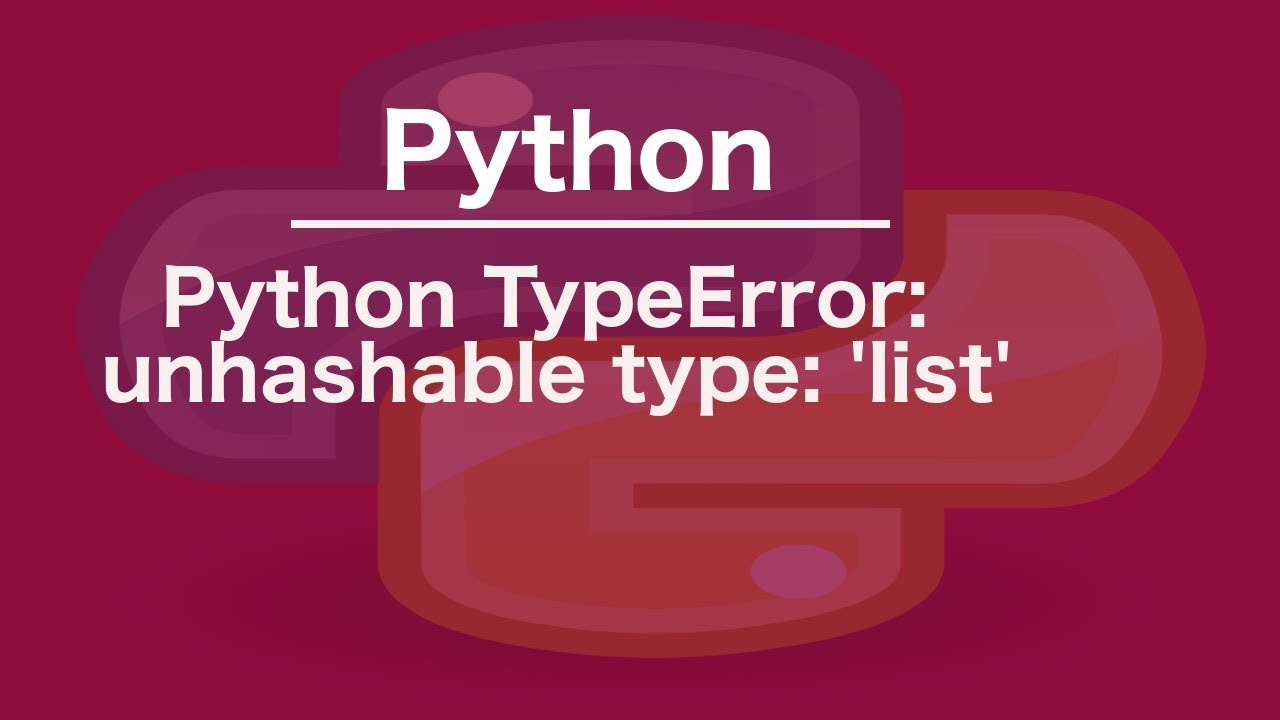


![SOLVED] TypeError: Solved] Typeerror:](https://www.pythonpool.com/wp-content/uploads/2022/04/TypeError-int-Object-Is-Not-Callable.webp)
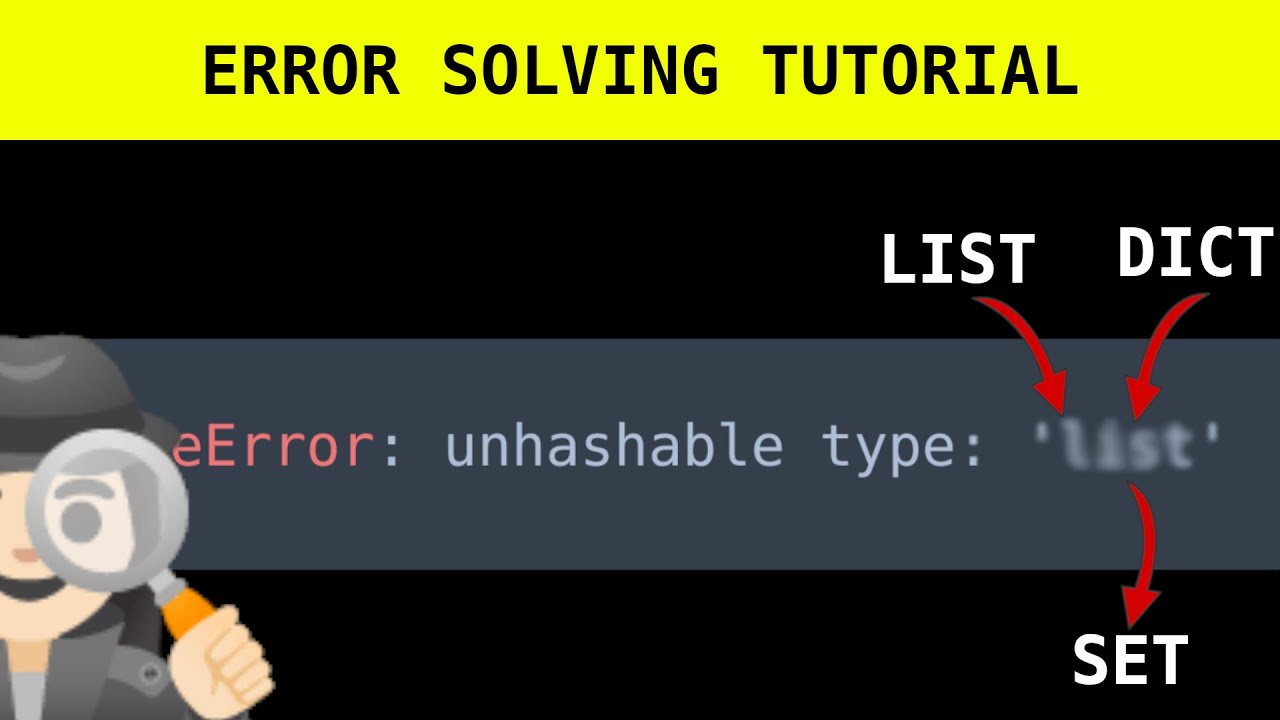

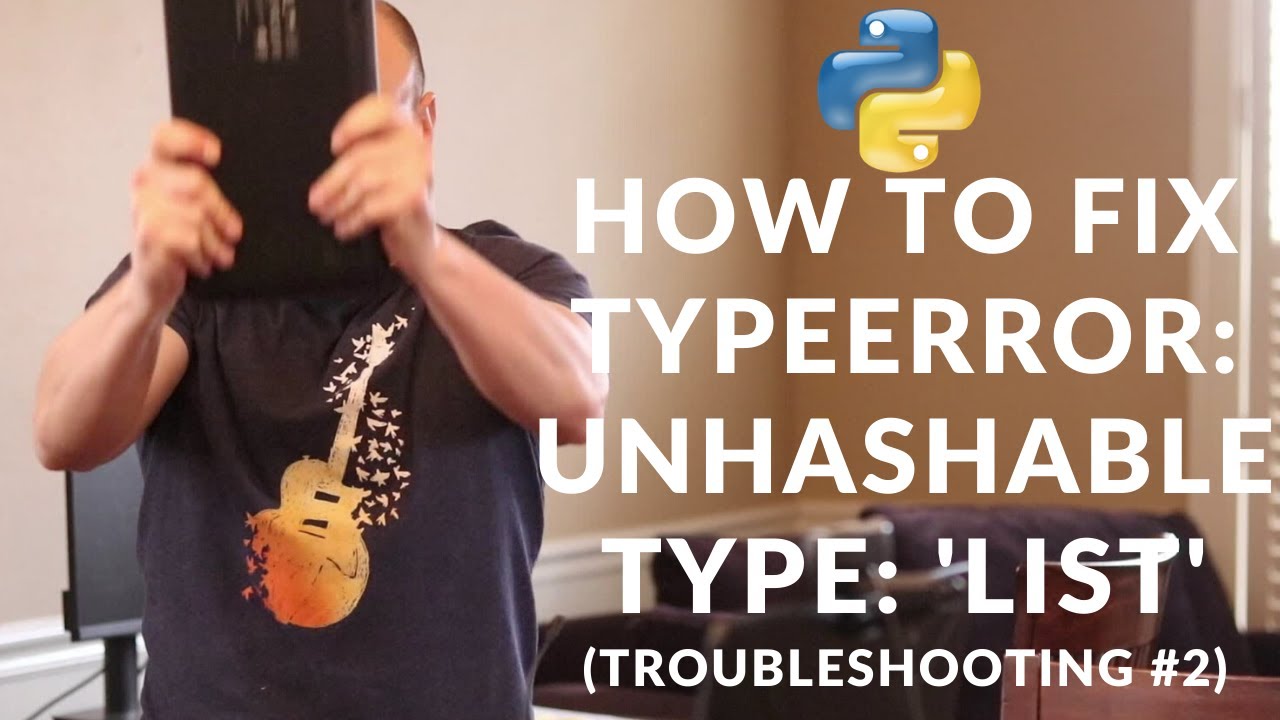
![Typeerror: unhashable type: 'numpy.ndarray' [SOLVED] Typeerror: Unhashable Type: 'Numpy.Ndarray' [Solved]](https://itsourcecode.com/wp-content/uploads/2021/01/IT-SOURCECODE_ICON-07.jpg)
Article link: typeerror unhashable type numpy.ndarray.
Learn more about the topic typeerror unhashable type numpy.ndarray.
- TypeError: unhashable type: ‘numpy.ndarray’ – Stack Overflow
- Resolved “TypeError: Unhashable Type” Numpy.Ndarray
- 3 Easy Ways to Fix TypeError: unhashable type: ‘numpy.ndarray’
- How to Fix the TypeError: unhashable type: ‘numpy.ndarray’?
- Typeerror: Unhashable Type: ‘numpy.ndarray’: Debugged and …
- Fix the Unhashable Type numpy.ndarray Error in Python
- How to make a tuple including a numpy array hashable?
- Numpy | ndarray – GeeksforGeeks
- How to Handle TypeError: Unhashable Type ‘Dict’ Exception in …
- Test whether the elements of a given NumPy array is zero or not in Python
- Typeerror: unhashable type: ‘numpy.ndarray’ – Itsourcecode.com
- unhashable type: ‘numpy.ndarray’ – AI Search Based Chat
- TypeError: unhashable type: ‘numpy.ndarray’ [closed]
See more: nhanvietluanvan.com/luat-hoc