Typeerror Float Object Is Not Subscriptable
Python is a popular programming language known for its simplicity and versatility. However, like any other programming language, it is not without its challenges. One common error that programmers may come across is the “TypeError: float object is not subscriptable” error. This error occurs when you try to access an index or slice a float object, which is not possible since float objects are not subscriptable. In this article, we will explore the causes of this error, common scenarios where it may occur, and how to handle and prevent it in Python programming.
Defining subscriptable objects and their usage in Python
Before diving into the “TypeError: float object is not subscriptable” error, let’s first understand what subscriptable objects are and their usage in Python.
Subscriptable objects in Python refer to objects that support the concept of indexing or slicing. In simple terms, subscripting allows us to access individual elements within a collection of objects. Common examples of subscriptable objects include lists, strings, and tuples.
For instance, with a list, you can access elements by specifying their index position using square brackets ([]):
“`python
my_list = [1, 2, 3]
print(my_list[0]) # Output: 1
“`
Similarly, you can also retrieve a range of elements using slicing:
“`python
my_string = “Hello World”
print(my_string[0:5]) # Output: “Hello”
“`
Introduction to float objects and their limitations
Float objects, on the other hand, are not subscriptable in Python. Floats are numerical data types that represent real numbers and can include decimal points. They are commonly used for mathematical calculations that involve fractions or decimals.
Unlike strings or lists, float objects do not have the concept of indexing or slicing. This means that you cannot access individual elements or retrieve a range of elements from a float object. Instead, you can only perform mathematical operations or comparisons using float numbers.
Causes of the “TypeError: float object is not subscriptable” error
Now that we understand what float objects are and their limitations, let’s explore the causes of the “TypeError: float object is not subscriptable” error.
The most common cause of this error is attempting to access an index or slice a float object. For example:
“`python
my_float = 3.14
print(my_float[0]) # Causes TypeError: float object is not subscriptable
“`
In this case, we are trying to access the first element of `my_float` using index notation, which is not allowed since float objects are not subscriptable. The interpreter raises a TypeError indicating that float objects cannot be subscripted.
Common scenarios where the error may occur
The “TypeError: float object is not subscriptable” error can occur in various scenarios. Let’s discuss some common situations where this error may arise:
1. Accidental subscripting of a float object: It is easy to mistakenly apply indexing or slicing to a float object when you intended to use a different data type. For example:
“`python
result = 4.5 + 2.7
print(result[0]) # Causes TypeError: float object is not subscriptable
“`
Here, `result` is a float object, but we are trying to access its first element, which is not possible.
2. Incorrect usage of functions that expect subscriptable objects: Some functions or methods in Python expect subscriptable objects as input. Passing a float object instead of a subscriptable object can lead to this error. For instance:
“`python
my_float = 3.14
max_value = max(my_float) # Causes TypeError: float object is not subscriptable
“`
The `max()` function expects an iterable object like a list or tuple, but we mistakenly pass a float object, resulting in a TypeError.
Handling the “TypeError: float object is not subscriptable” error
When encountering the “TypeError: float object is not subscriptable” error, it is important to identify the root cause and resolve it accordingly. Here are some techniques for handling this error:
1. Check your code for accidental subscripting: Double-check your code to ensure that you are not applying subscripting operations (indexing or slicing) to float objects. If you intended to use a different data type, revise your code accordingly.
2. Verify the expected input for functions or methods: If the error occurs while using a specific function or method, review its documentation to understand the expected input. If subscriptable objects are required, ensure that you pass the correct data type.
3. Use appropriate data types: If you need to perform subscripting operations, use the correct data types such as lists or strings instead of float objects. Convert the float object to the appropriate type before applying subscripting operations.
“`python
my_float = 3.14
my_list = [my_float]
print(my_list[0]) # Output: 3.14
“`
Tips to prevent the occurrence of the error in Python programming
While handling the “TypeError: float object is not subscriptable” error is important, it is always better to prevent errors from occurring in the first place. Here are some tips to prevent this error in your Python programming:
1. Carefully review your code: Before running your code, thoroughly review it for any instances where indexing or slicing is applied to float objects.
2. Use proper variable naming conventions: Adopting meaningful variable names can help prevent confusion between float objects and subscriptable objects, reducing the chances of accidental subscripting.
3. Read documentation and error messages: Familiarize yourself with the functions and methods you are using and pay attention to error messages. Understanding the expected input can help you avoid inappropriate use of float objects.
4. Input validation and error handling: Implement input validation and error handling mechanisms to ensure that your code receives the correct data types and handles unexpected situations gracefully.
FAQs:
Q: What does the error message “TypeError: float object is not subscriptable” mean?
A: This error message suggests that you are trying to access an index or slice a float object, which is not allowed since float objects are not subscriptable.
Q: Can I access individual elements of a float object?
A: No, float objects do not support indexing or slicing. They can only be used for mathematical operations or comparisons.
Q: How can I handle the “TypeError: float object is not subscriptable” error?
A: To handle this error, review your code for accidental subscripting, ensure the proper input for functions or methods, and use appropriate data types.
Q: How can I prevent this error from occurring in my code?
A: Preventing this error involves careful code review, proper variable naming, understanding documentation, and implementing input validation and error handling mechanisms.
In conclusion, the “TypeError: float object is not subscriptable” error occurs when you try to access an index or slice a float object. Float objects are not subscriptable in Python, unlike subscriptable objects like lists or strings. By understanding the causes and common scenarios of this error, you can effectively handle and prevent it in your Python programming.
Fix Typeerror Int Or Float Object Is Not Subscriptable – Python
Keywords searched by users: typeerror float object is not subscriptable TypeError: ‘type’ object is not subscriptable, Int’ object is not subscriptable, Object is not subscriptable python3, Convert float list to int Python, TypeError property object is not subscriptable, List to float Python
Categories: Top 46 Typeerror Float Object Is Not Subscriptable
See more here: nhanvietluanvan.com
Typeerror: ‘Type’ Object Is Not Subscriptable
If you have encountered the error message “TypeError: ‘type’ object is not subscriptable” in your programming journey, you may have found yourself scratching your head in confusion. Don’t worry, you are not alone. This error is a common stumbling block that developers often come across when working with Python. In this article, we will delve into what this error means, why it occurs, and provide you with several solutions to help you overcome it.
What does the error message mean?
Before we dive into the specifics of this error, let’s first understand the concept of subscriptable objects. In Python, certain data types like lists, tuples, and dictionaries can be accessed using square brackets to retrieve individual elements. This process is called subscripting. However, not all objects in Python are subscriptable.
The error message “TypeError: ‘type’ object is not subscriptable” signifies that you are attempting to access an element of an object that is not subscriptable. In other words, you are trying to use square brackets on an object that does not support indexing.
Why does this error occur?
There could be several reasons for encountering this error. One common cause is mistakenly trying to subscript a type object instead of an instance of that object. For example:
“`python
class MyClass:
pass
element = MyClass[0] # Oops! MyClass is the type object, not an instance object.
“`
In the example above, we are trying to access an element of the class `MyClass`, but `MyClass` is not an instance; it is the type object itself. Therefore, subscripting it will result in the mentioned error.
Another reason for facing this error is when you are trying to access an item that does not exist within a non-subscriptable object. For instance:
“`python
my_variable = 123
element = my_variable[0] # Oops! Trying to access an index, but my_variable is not subscriptable.
“`
In this case, since `my_variable` is an integer, it is not subscriptable. Thus, trying to access its non-existent first element will raise a `TypeError`.
How to solve the ‘type’ object is not subscriptable error?
Now that we have a clearer understanding of what causes this error, let’s explore some potential solutions to overcome it.
1. Verify the object type: Make sure you are subscripting an object that is intended to be subscriptable. Double-check the type of object you are trying to subscript and verify that it supports indexing.
2. Verify the object instance: Ensure that you are subscripting an instance object rather than a type object. Instances of classes are subscriptable, while the class objects themselves are not. Be cautious of any unintended use of square brackets on class names.
3. Check the data type: If you are working with a particular data type, confirm that it is subscriptable. For example, lists, tuples, and dictionaries are subscriptable, while integers and floats are not.
4. Review the code logic: Inspect your code and ensure that the subscript operation is being applied in a valid and appropriate context. If necessary, consider revising your code logic to avoid unintended subscripting.
5. Debug with print statements: If you are still unable to identify the cause, consider placing print statements strategically in your code to trace the error. This can help you pinpoint the exact location where the problem arises.
Frequently Asked Questions (FAQs):
Q1: Is this error specific to Python?
A1: Yes, the “TypeError: ‘type’ object is not subscriptable” error is specific to Python. It occurs when you try to use square brackets to access an element of an object that doesn’t support indexing.
Q2: Can this error occur with any Python version?
A2: Yes, this error is not version-specific and can occur in any version of Python.
Q3: Are there any built-in Python functions that can trigger this error?
A3: No, this error typically arises due to a programmer’s mistake rather than a specific built-in function.
Q4: Is it possible to make non-subscriptable objects subscriptable?
A4: Yes, it is possible to make non-subscriptable objects subscriptable by implementing special methods such as `__getitem__` in custom classes. However, this requires modifying the object’s underlying code.
Q5: Are there any alternative data structures to achieve indexing in non-subscriptable objects?
A5: Yes, you can consider using alternative data structures like arrays or numpy arrays, which are built specifically for numerical computations and support indexing.
In conclusion, encountering the “TypeError: ‘type’ object is not subscriptable” error can be frustrating, but with a deeper understanding of the error message and the underlying causes, you can effectively resolve it. By employing the solutions we discussed and carefully inspecting your code, you will be well-equipped to tackle this error and continue developing your Python programs seamlessly.
Int’ Object Is Not Subscriptable
When working with Python programming language, you might come across an error message stating “TypeError: ‘int’ object is not subscriptable.” This error often occurs when you attempt to use indexing or slicing operations on an integer object, which is not allowed because integers are immutable and do not support item assignment. In this article, we will explore the meaning of this error message, understand why it occurs, and learn how to resolve it.
Understanding the Error:
The error message “TypeError: ‘int’ object is not subscriptable” indicates that you are trying to perform an operation, such as indexing or slicing, on an integer object that cannot be performed on this type of object. It essentially means that the object you are trying to access with square brackets [ ] is not subscriptable because it is an integer.
In Python, subscriptable objects are those that can be accessed using indices, such as strings, lists, or dictionaries. These objects support indexing, which means you can access individual items or parts of the object by referring to their position within the object using brackets [ ].
Reasons for the Error:
The main reason for encountering this error is the misuse or misunderstanding of the data types involved in the operation. For example, consider the following code snippet:
“`python
num = 10
print(num[0])
“`
In this code, the variable `num` is initialized as an integer with the value 10. When we try to access the first element (index 0) of `num` using `num[0]`, we encounter the “TypeError: ‘int’ object is not subscriptable” error. This error occurs because integers are not subscriptable, and you cannot access their individual elements directly.
Furthermore, if a function or method returns an integer, and you mistakenly try to treat it as a subscriptable object, you will encounter the same error. Therefore, it is crucial to understand the type of object you are working with and the operations it supports.
Resolving the Error:
To resolve the “TypeError: ‘int’ object is not subscriptable” error, you need to carefully review your code and ensure that you are not attempting to perform subscript operations on integers. Here are some common scenarios where this error can occur and possible fixes:
1. Misuse of indexing: If you mistakenly try to access parts of an integer using indexing or slicing, you will encounter the error. To fix this, review your code and remove any attempts to use brackets [ ] on integer objects.
2. Incorrect return type: If a function or method is returning an integer instead of a subscriptable object, you should check the documentation or source code to determine the intended return type. If necessary, modify the function to return the desired object type.
3. Variable assignment: Ensure that variables are assigned values of the correct data type. If you mistakenly assign an integer to a variable that should hold a subscriptable object, update the assignment to the correct type.
Frequently Asked Questions:
Q1. What does “TypeError: ‘int’ object is not subscriptable” mean?
A1. This error message suggests that you are trying to perform subscript operations, such as indexing or slicing, on an integer object, which is not allowed because integers are not subscriptable.
Q2. When does this error occur?
A2. The error occurs when you attempt to access individual items or parts of an integer object using square brackets [ ] for indexing or slicing.
Q3. How can I fix this error?
A3. To resolve the error, make sure you are not trying to use subscript operations on an integer. Review your code, remove any improper indexing, and ensure that functions or methods return the expected object type.
Q4. Can I perform any operations on integers?
A4. Yes, integers support various mathematical operations, but they do not support indexing or slicing. For accessing individual digits or characters within an integer, you need to convert it to a string.
In conclusion, the “TypeError: ‘int’ object is not subscriptable” error occurs when attempting to perform subscript operations on an integer object. By understanding the nature of the error and carefully reviewing your code, you can resolve this issue and ensure proper usage of subscriptable objects in Python.
Object Is Not Subscriptable Python3
Concept of subscriptability:
Python objects can be classified into subscriptable and non-subscriptable types. Subscriptable objects are those which allow indexing, meaning you can use square brackets [] to access elements of these objects. On the other hand, non-subscriptable objects do not support indexing and hence you cannot access their elements using square brackets.
Generally, built-in data types such as lists, tuples, and strings are subscriptable in Python. These data types allow direct access to their elements using square brackets. However, not all objects support indexing. For example, dictionaries and sets do not support indexing, and therefore, using square brackets to access elements of these objects will result in the “Object is not subscriptable” error.
Why the error occurs:
The “Object is not subscriptable” error occurs when you try to use square brackets to access the elements of an object that does not support indexing. Let’s consider an example to understand this better:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25}
print(my_dict[‘name’]) # Output: John
print(my_dict[‘age’]) # Output: 25
print(my_dict[0]) # Error: Object is not subscriptable
“`
In the above example, we have a dictionary `my_dict` that stores a person’s name and age. We successfully accessed the elements ‘name’ and ‘age’ using square brackets. However, when we tried to access the element at index 0 (`my_dict[0]`), we encountered the “Object is not subscriptable” error, as dictionaries do not support indexing.
Solutions:
1. Check the object type: The first step to resolve this error is to check the type of object you are trying to access using square brackets. Ensure that it is a subscriptable type (list, tuple, string), and not a non-subscriptable type (dictionary, set). If it is a non-subscriptable type, consider using appropriate methods or attributes to access the desired data.
2. Use alternative methods: If you need to access elements of a non-subscriptable object, explore alternative methods provided by that object type. For example, dictionaries provide methods like `dict.get(key)` or `dict.keys()`, which allow you to retrieve values or keys respectively, without using indexing.
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25}
print(my_dict.get(‘name’)) # Output: John
print(my_dict.get(‘age’)) # Output: 25
“`
3. Convert the object: In some cases, you may need to convert your non-subscriptable object into a subscriptable one. For instance, if you have a string and want to access its characters, you can convert it to a list using the `list()` function.
“`python
my_string = “Hello, World!”
my_list = list(my_string)
print(my_list[7]) # Output: W
“`
4. Handle exceptions: If you are uncertain about the type of object you are dealing with, or if it could be dynamically changing, you can handle the “Object is not subscriptable” error using exception handling. Wrap the code that could raise this error in a try-except block and handle the exception gracefully.
“`python
my_data = {‘name’: ‘John’, ‘age’: 25}
try:
print(my_data[‘city’])
except TypeError:
print(‘Object is not subscriptable’)
“`
FAQs:
Q1. Can I use indexing with all built-in data types?
A1. No, not all built-in data types support indexing. Certain types like dictionaries and sets do not support indexing, while others like lists and strings do.
Q2. How can I determine whether an object is subscriptable or not?
A2. You can use the `isinstance()` function to check if an object belongs to a subscriptable type. For example:
“`python
my_list = [1, 2, 3]
print(isinstance(my_list, (list, tuple, str))) # Output: True
my_dict = {‘name’: ‘John’}
print(isinstance(my_dict, (list, tuple, str))) # Output: False
“`
Q3. I am sure that my object is subscriptable, but I still encounter the error. What could be the problem?
A3. In some cases, the object you are attempting to subscript may be empty or have fewer elements than you are trying to access. Make sure the object is not empty or has enough elements before attempting to use indexing.
Q4. Can I make my custom object subscriptable?
A4. Yes, you can make your custom objects subscriptable by implementing the special methods `__getitem__()` and `__setitem__()` in your class. These methods define how the object should behave when accessed using square brackets.
In conclusion, the “Object is not subscriptable” error occurs when you try to access elements of an object that does not support indexing. This article discussed the concept of subscriptability, explained why the error occurs, and provided solutions to handle this error. By understanding the nature of the object you are dealing with and utilizing appropriate methods or conversions, you can effectively overcome this error and write bug-free Python code.
Images related to the topic typeerror float object is not subscriptable
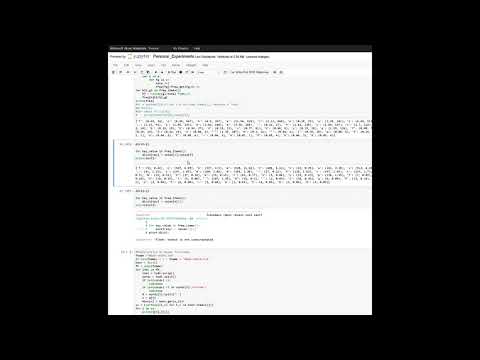
Found 28 images related to typeerror float object is not subscriptable theme
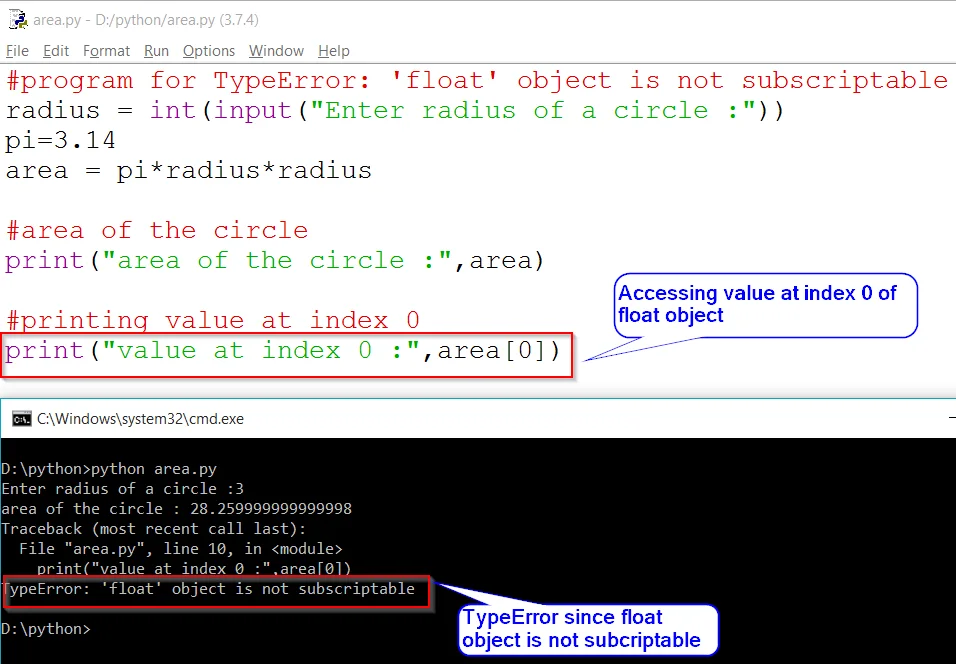


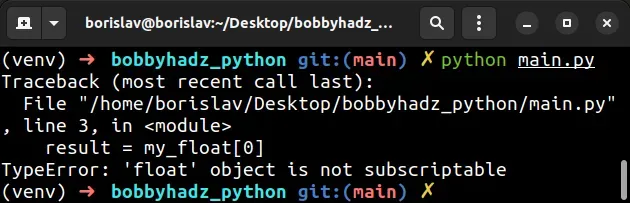
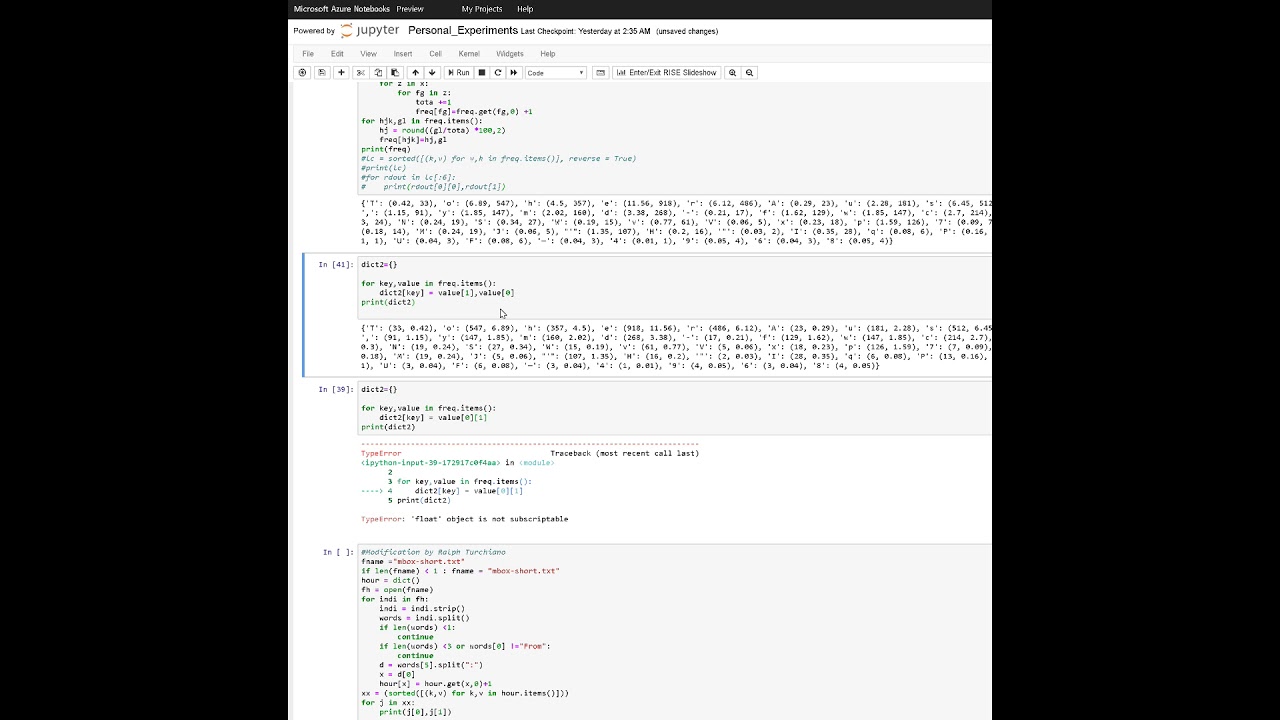


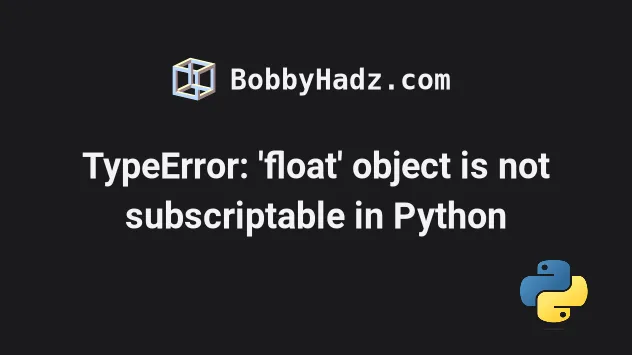
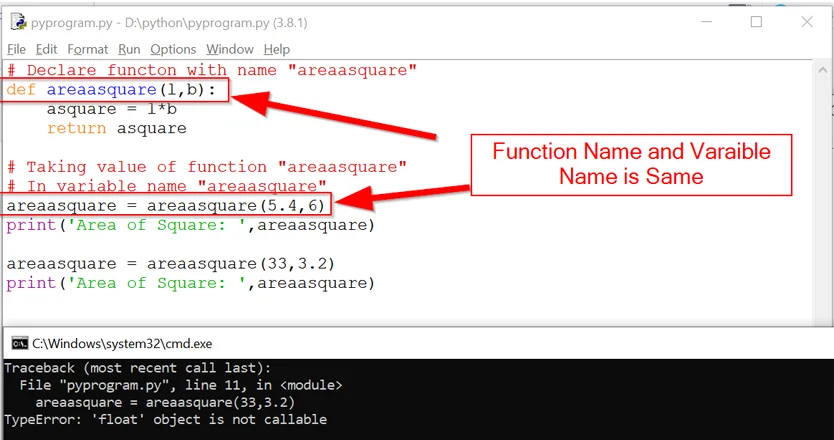
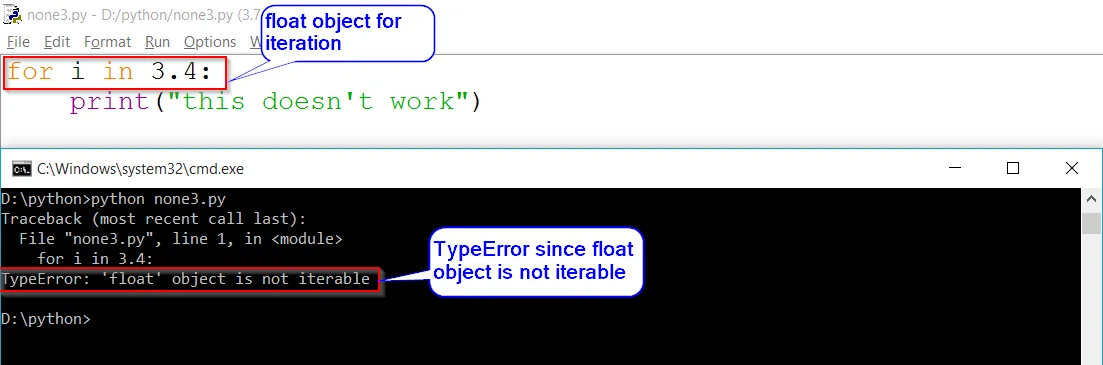
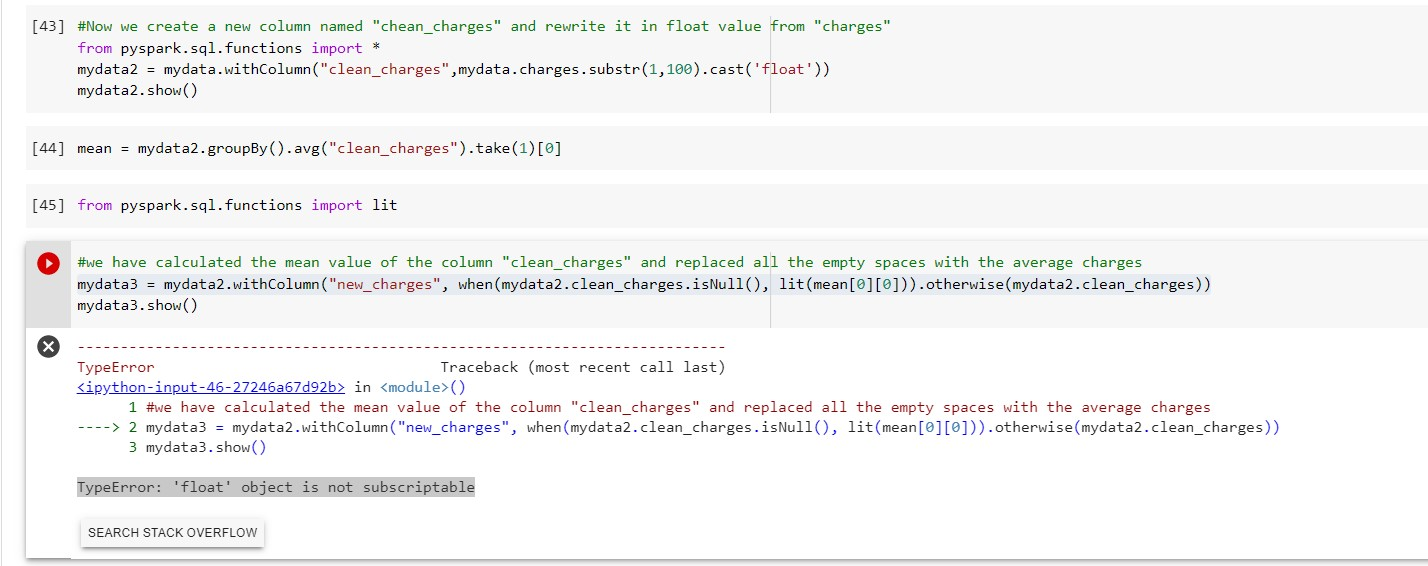
![Typeerror: 'float' object is not subscriptable [SOLVED] Typeerror: 'Float' Object Is Not Subscriptable [Solved]](https://itsourcecode.com/wp-content/uploads/2023/04/typeerror-float-object-is-not-subscriptable.png)
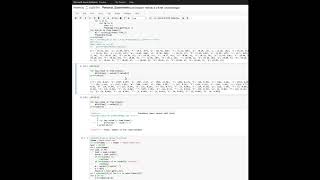
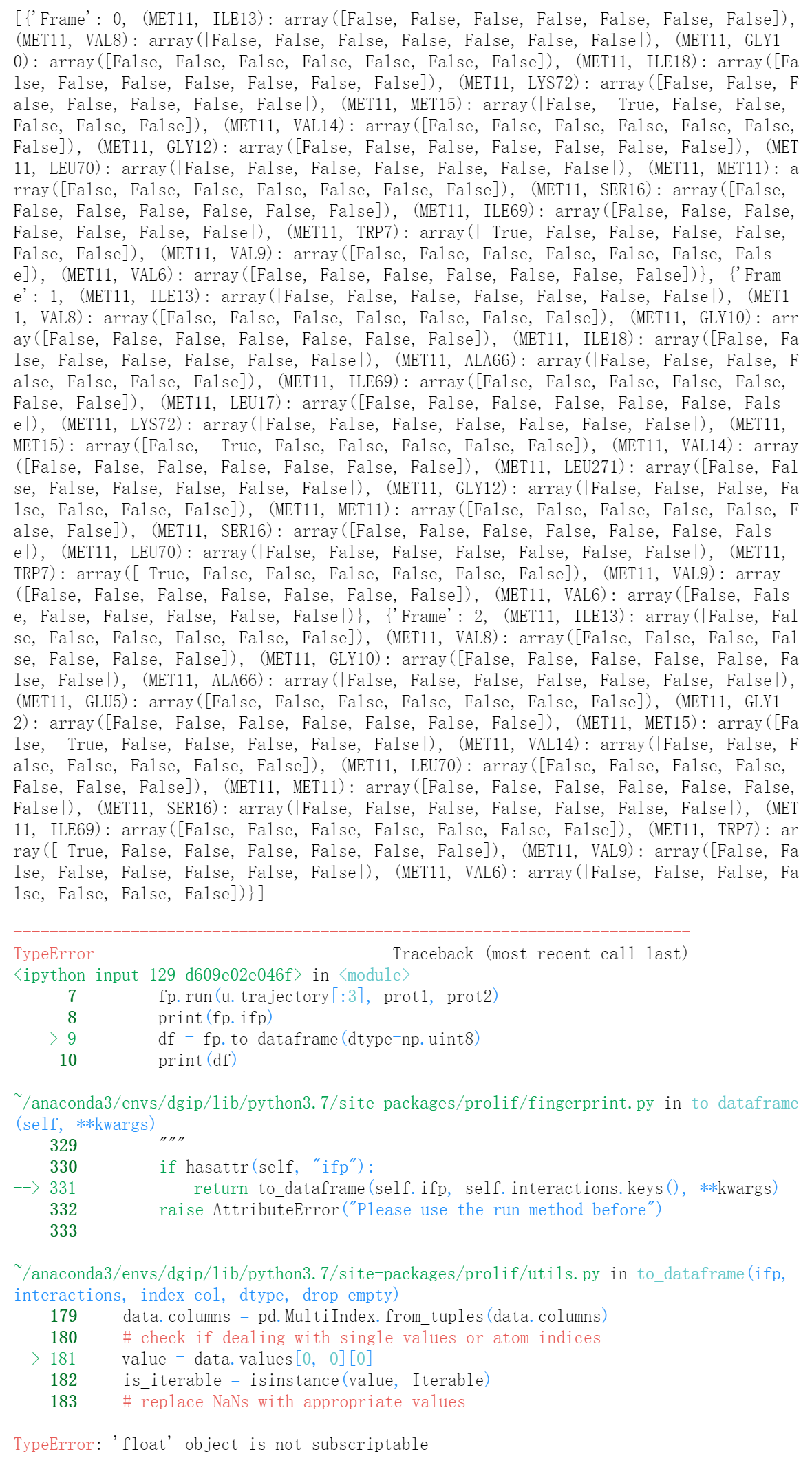

![TypeError: 'int' object is not subscriptable [Solved Python Error] Typeerror: 'Int' Object Is Not Subscriptable [Solved Python Error]](https://www.freecodecamp.org/news/content/images/2022/10/in_not_subable.png)
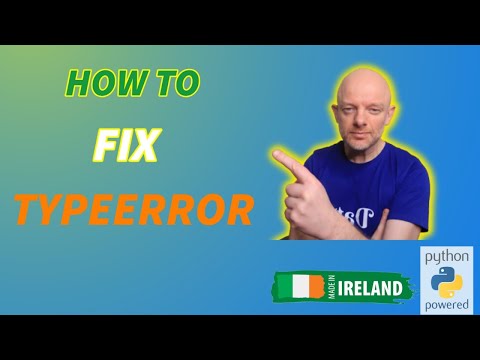
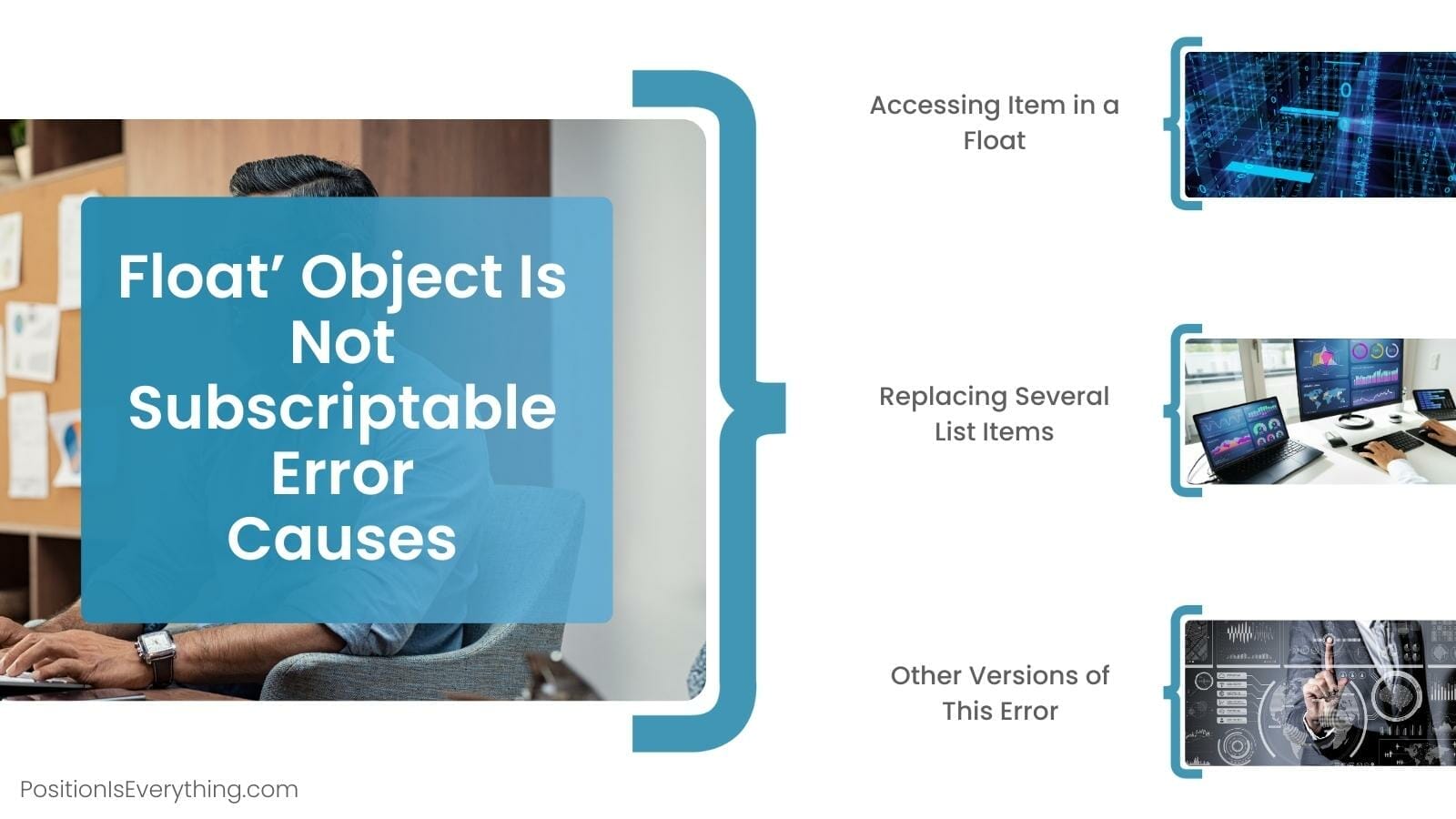
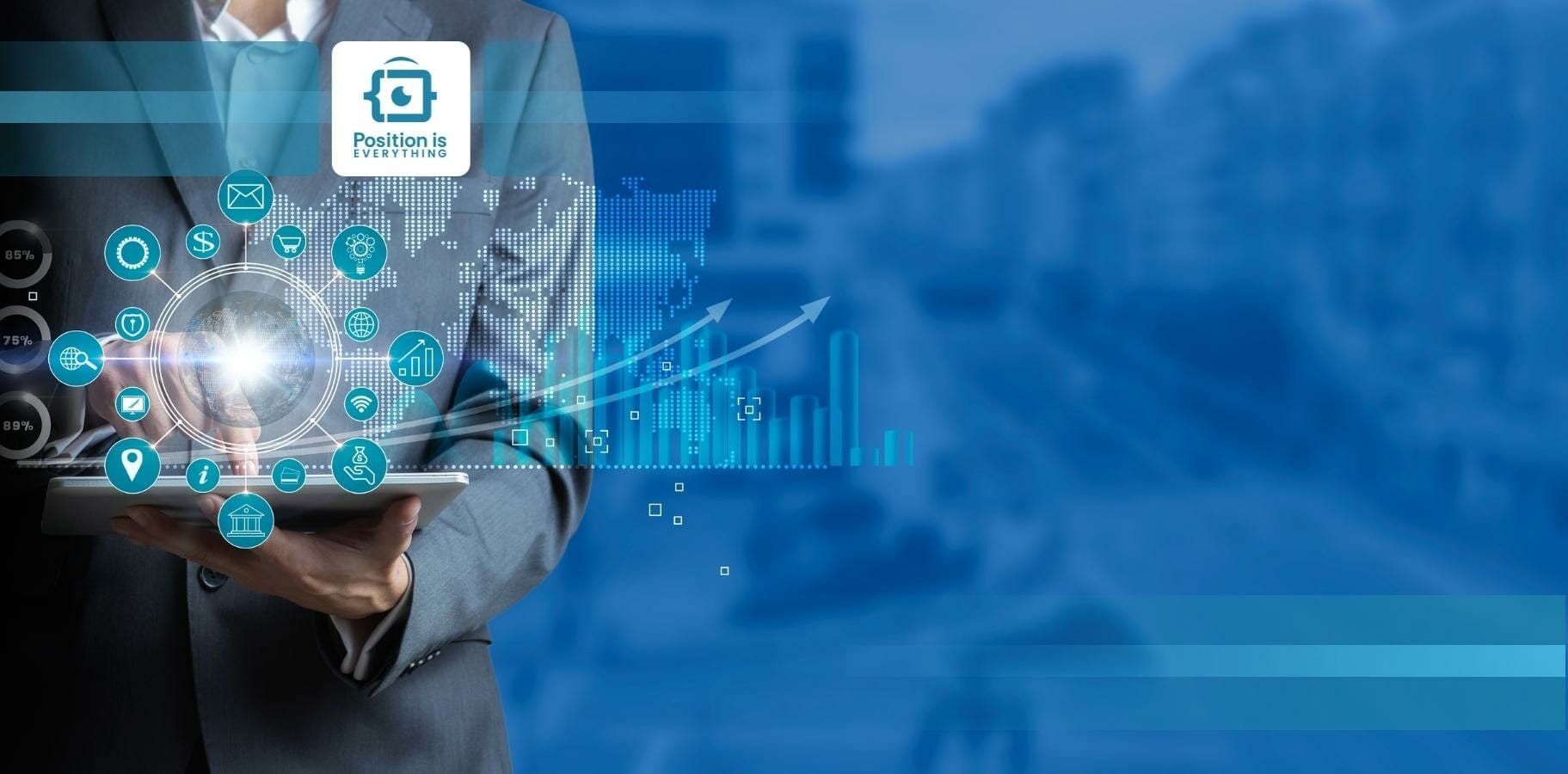
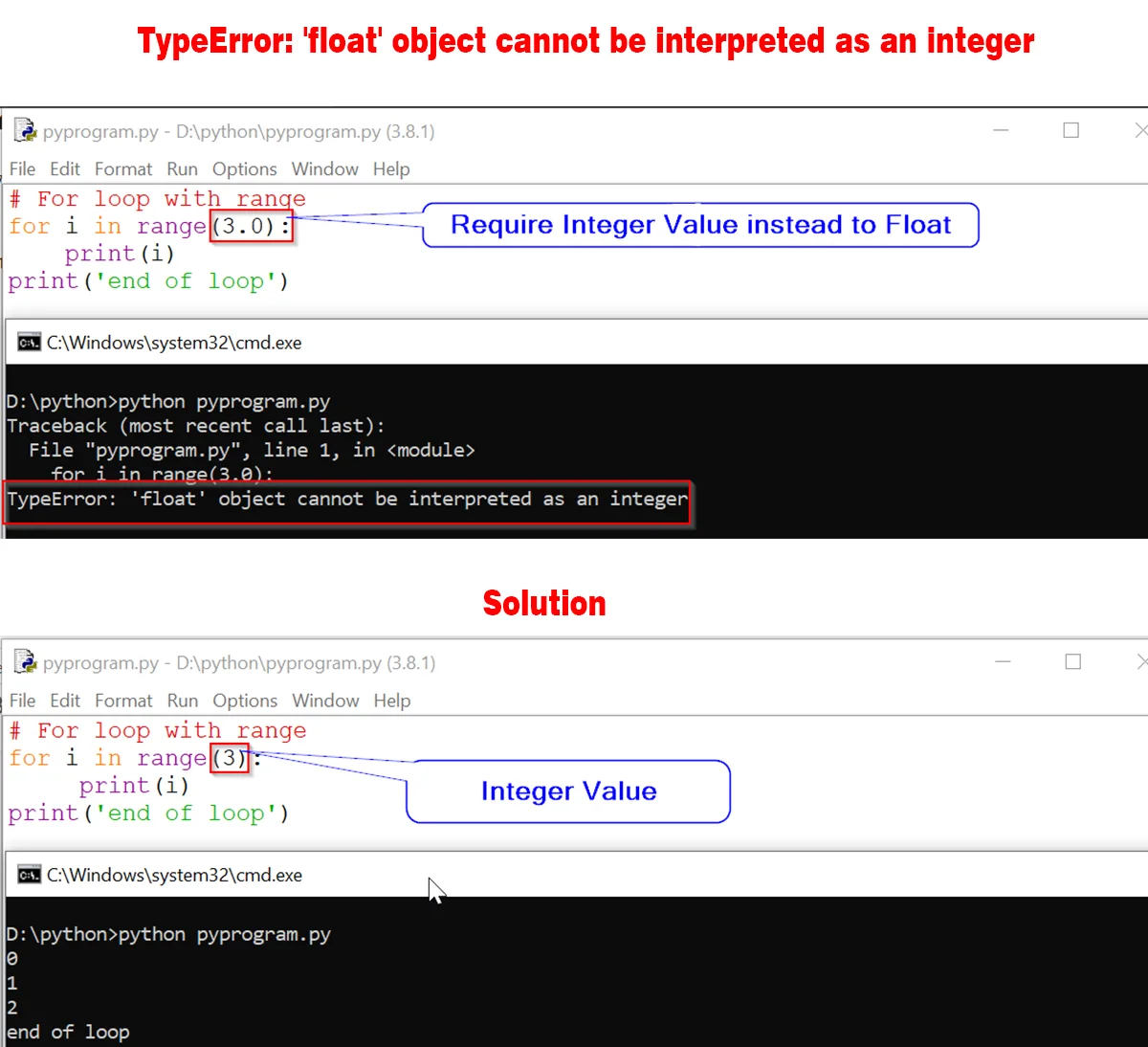
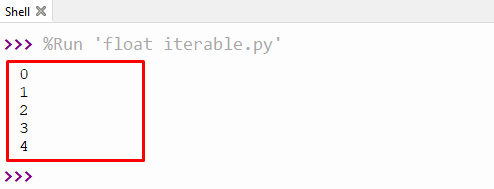
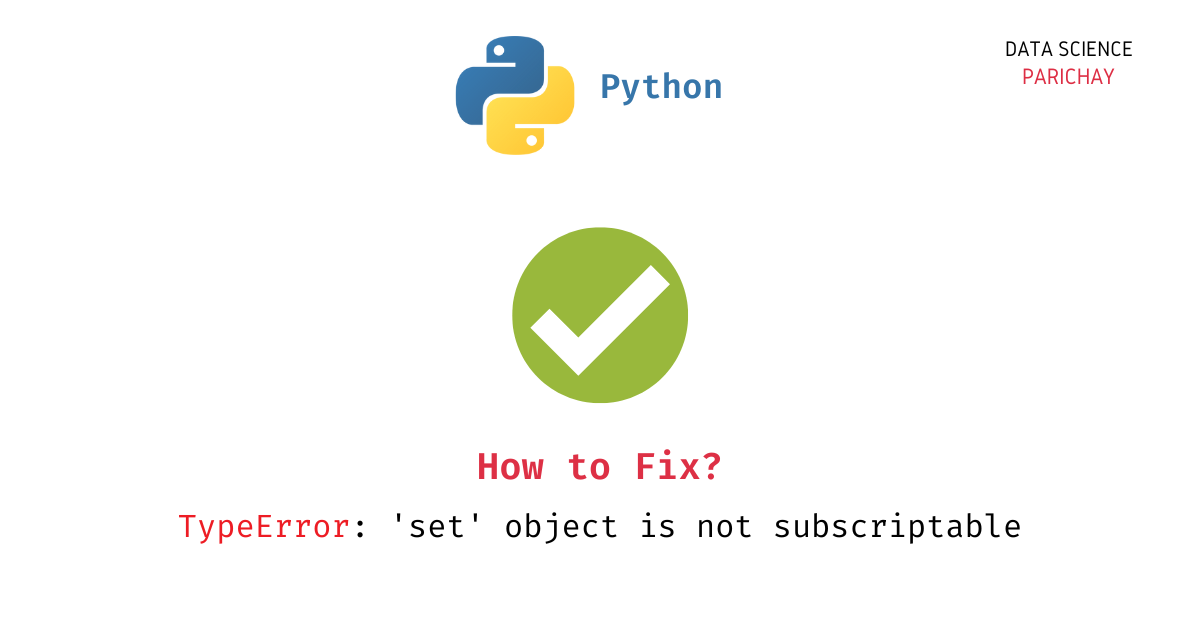
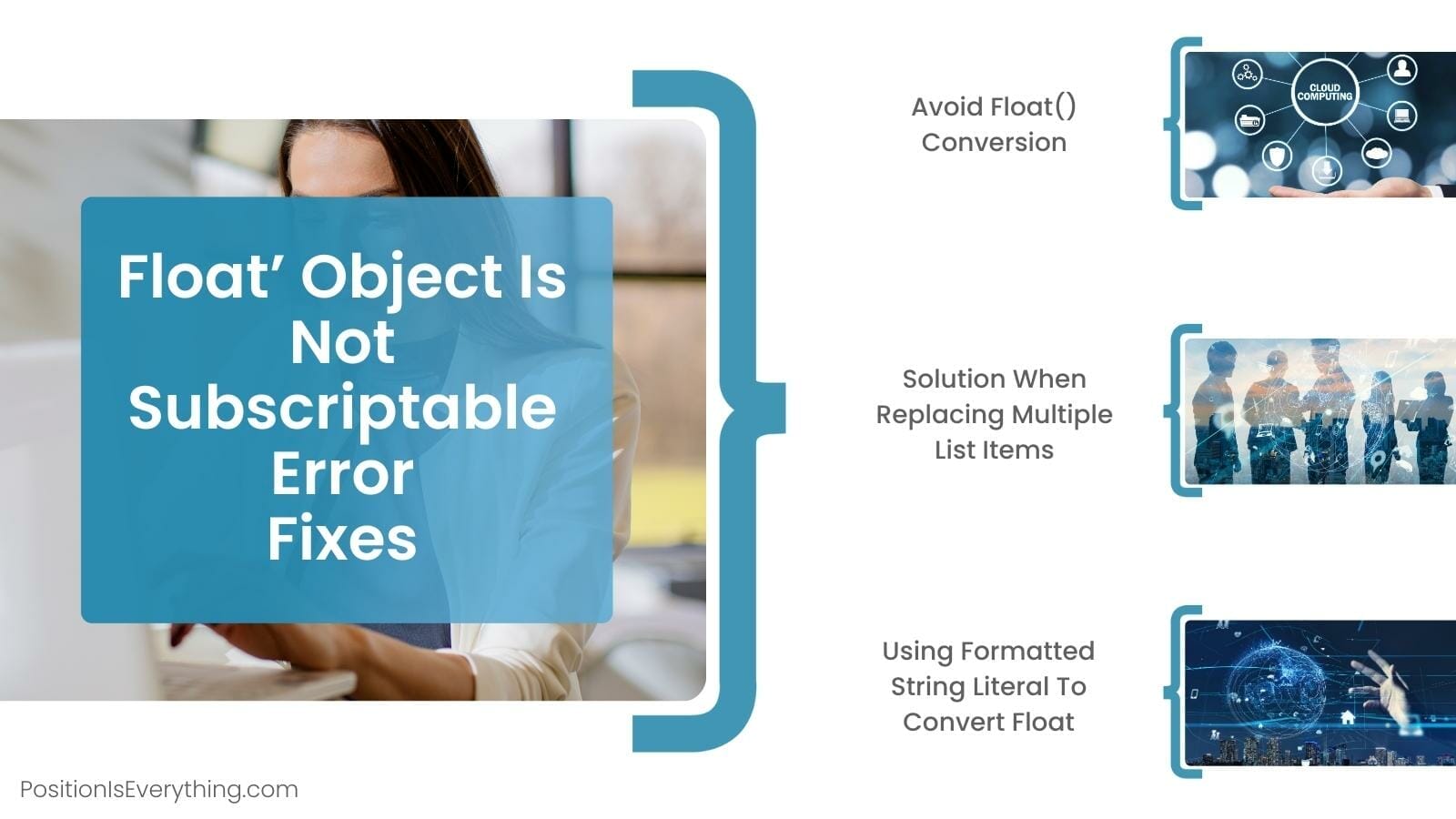


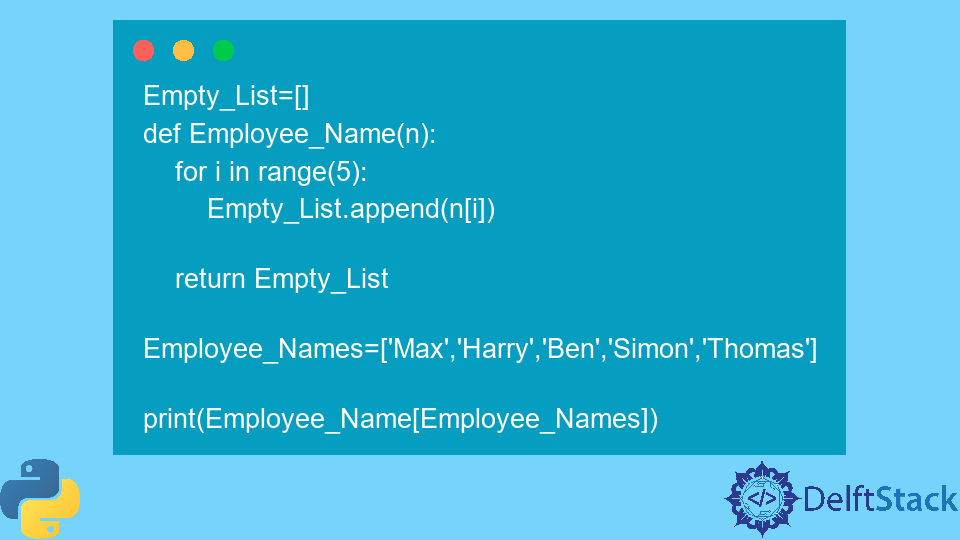
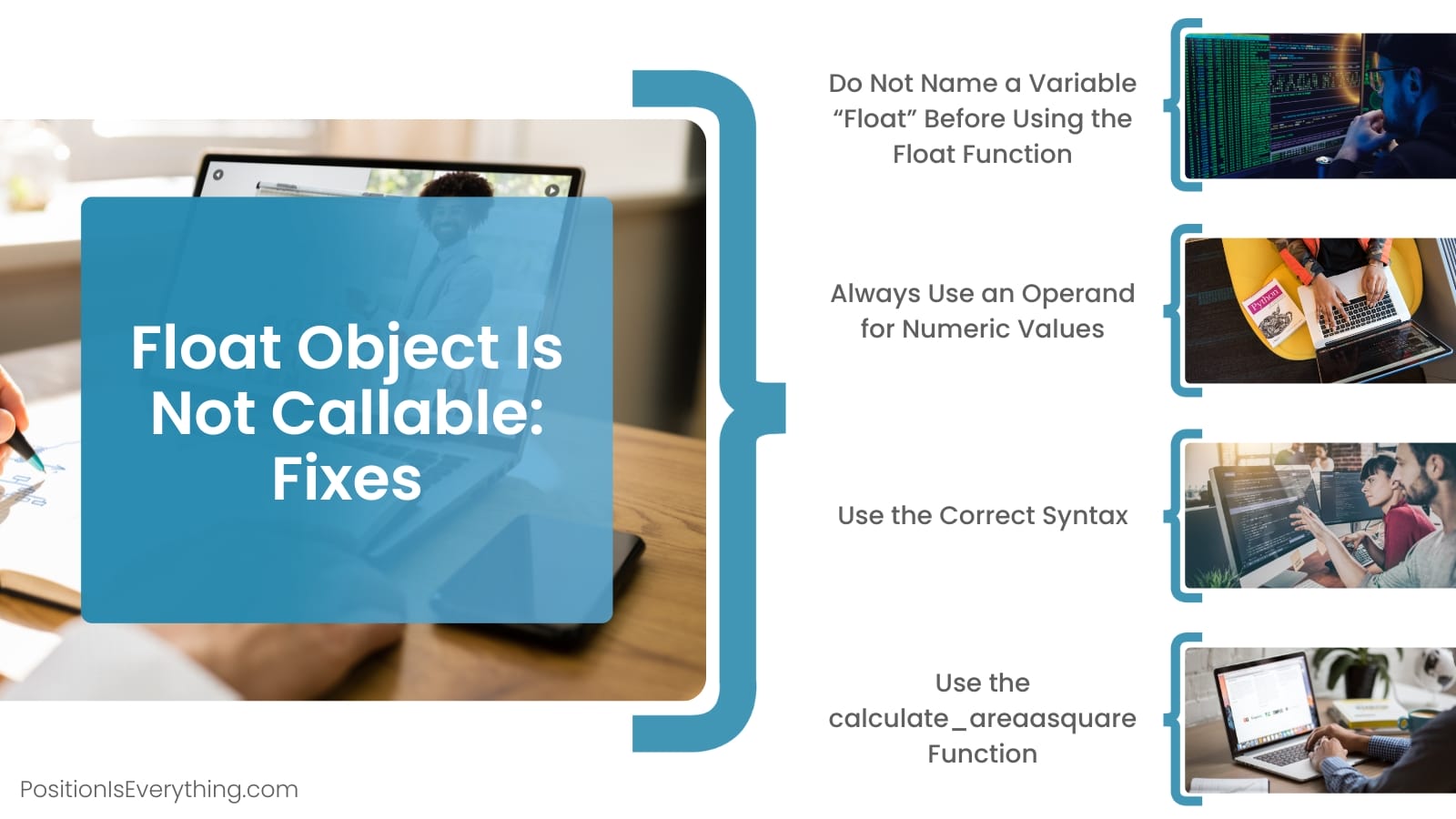
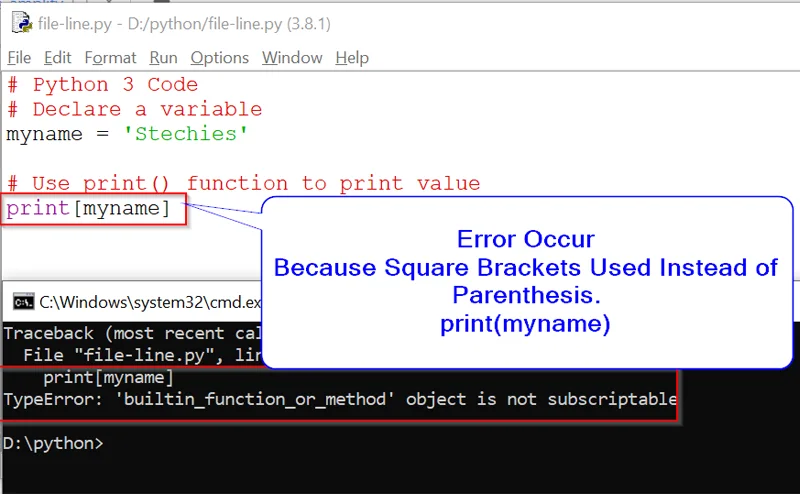
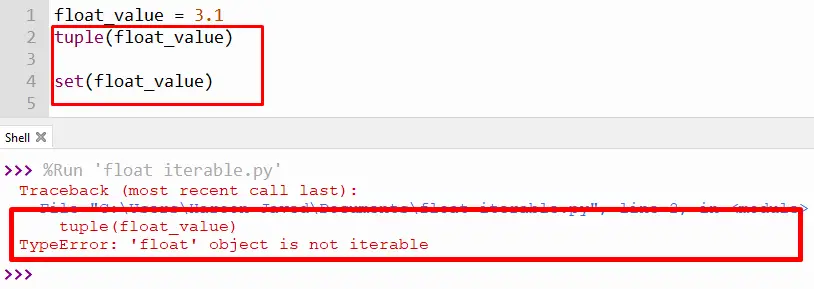
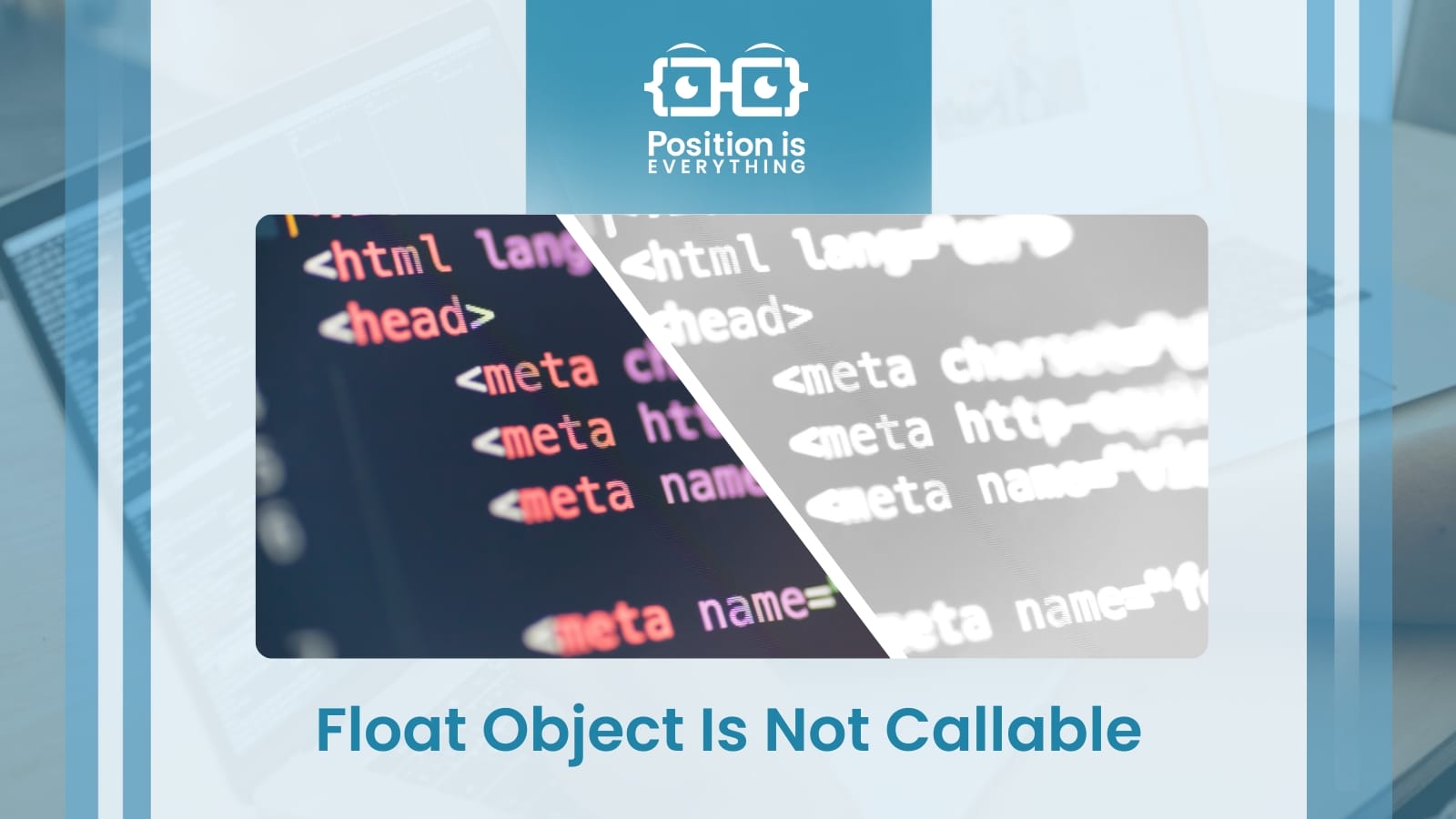

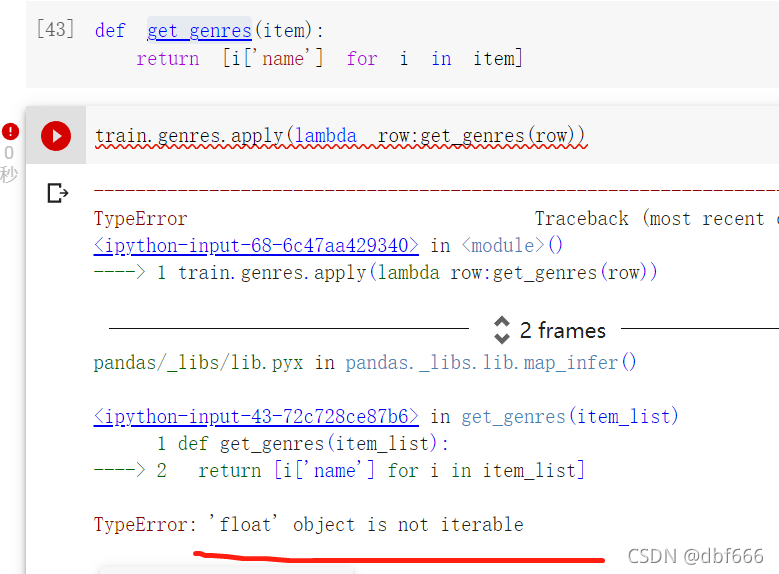


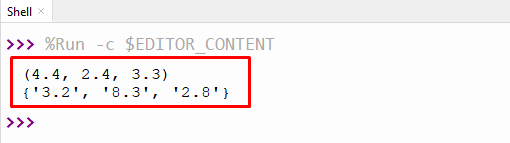
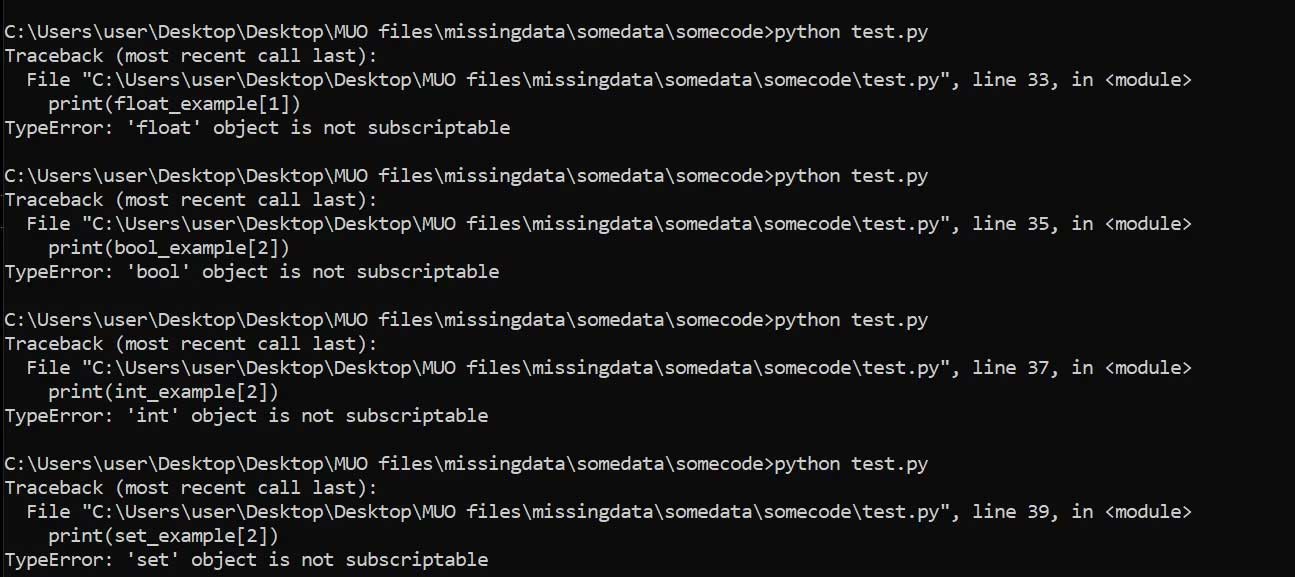

![Solved] TypeError: 'int' Object Is Not Subscriptable in Python – Be on the Right Side of Change Solved] Typeerror: 'Int' Object Is Not Subscriptable In Python – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2021/03/typeError.png)




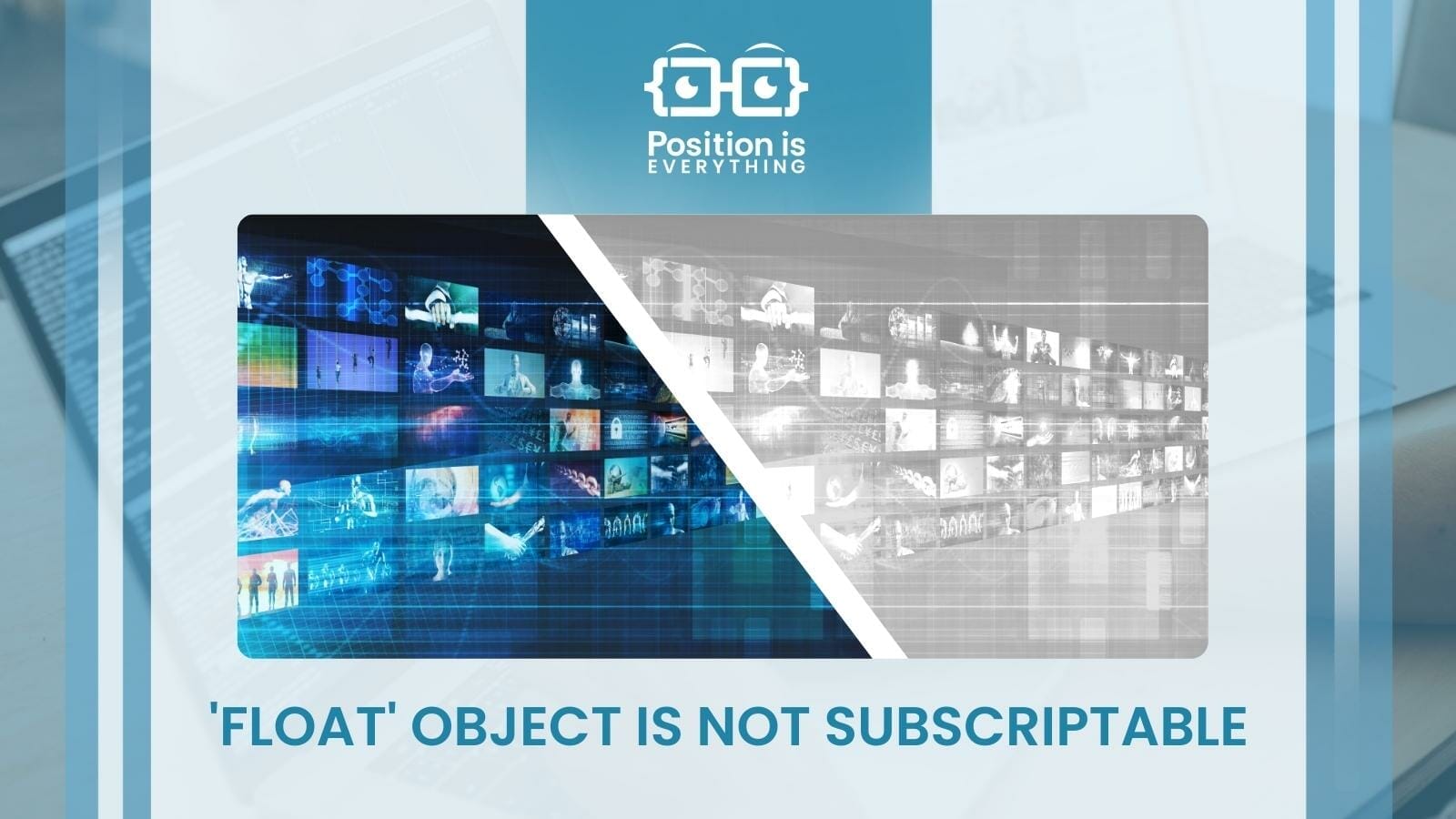

Article link: typeerror float object is not subscriptable.
Learn more about the topic typeerror float object is not subscriptable.
- TypeError: ‘float’ object is not subscriptable in Python
- TypeError: ‘float’ object is not subscriptable – Stack Overflow
- (Solved) Python TypeError: ‘float’ object is not subscriptable
- TypeError: ‘float’ object is not subscriptable – STechies
- Python typeerror: ‘float’ object is not subscriptable Solution
- Solve Python TypeError: ‘float’ object is not subscriptable
- Typeerror: ‘float’ object is not subscriptable [SOLVED]
- ‘float’ object is not subscriptable: How to fix it in Python
- [Solved] TypeError: ‘float’ object is not subscriptable
- ‘float’ object is not subscriptable python – AI Search Based Chat
See more: nhanvietluanvan.com/luat-hoc