Non Default Argument Follows Default Argument
In programming, arguments are values that are passed into a function or method to perform a particular task. These arguments can either have default values or not, which affects how they are used within the program. However, when a non-default argument follows a default argument, it can result in an error known as “non-default argument follows default argument.” This article will delve into the intricacies of default and non-default arguments, explain the error message, give examples across different programming languages, provide best practices to avoid the error, discuss potential drawbacks of using default arguments, and present alternative solutions when designing functions.
Definition of Default and Non-Default Arguments in Programming:
Before diving into the error message, it is essential to understand the concepts of default and non-default arguments. A default argument is a value that is automatically assigned to a parameter in a function or method. When the function is called and an argument is not provided for that parameter, the default value is used.
A non-default argument, on the other hand, is a value that is explicitly passed into a function or method. It does not rely on a default value and is provided by the programmer when invoking the function.
How Default Arguments Work in Programming Languages:
Default arguments are a feature of many programming languages, including Python, C++, and JavaScript. They provide flexibility by allowing functions to be called with fewer arguments than defined, as the default values will be used.
For example, in Python, a function can be defined with a default argument as follows:
“`python
def greet(name=’Anonymous’):
print(‘Hello, ‘ + name + ‘!’)
greet() # Output: Hello, Anonymous!
greet(‘John’) # Output: Hello, John!
“`
The function `greet` has a default argument `name=’Anonymous’`. When the function is called without passing any arguments, the default value is used. However, when an argument is provided, it overrides the default value.
Explanation of the Error Message “Non-Default Argument Follows Default Argument”:
When a non-default argument is placed before a default argument in a function definition, it violates the syntax and results in the error message “non-default argument follows default argument.” This error serves as a reminder that default arguments should not be followed by non-default arguments.
“`python
def concatenate_strings(a, b=’ world!’, c):
return a + b + c
# Error: non-default argument follows default argument
“`
In this example, the argument `b=’ world!’` has a default value, but it is followed by the non-default argument `c`. To resolve the error, the function declaration should be modified, ensuring non-default arguments come before default arguments.
Reasons for Using Default Arguments in Functions:
Default arguments offer several advantages when designing functions. They provide increased flexibility by allowing functions to have optional parameters. This eliminates the need to define multiple versions of the same function to accommodate different argument requirements. Default arguments also enhance readability as they document the expected behavior of a function.
Common Mistakes Leading to the Non-Default Argument Follows Default Argument Error:
The error “non-default argument follows default argument” often occurs due to a few common mistakes made by programmers. One primary mistake is defining a function with a non-default argument after a default argument. Another related mistake is omitting a non-default argument unintentionally or providing it in the wrong order when the function is called.
Examples of the Error in Different Programming Languages:
The “non-default argument follows default argument” error is not exclusive to a specific programming language. Here are examples of the error in different languages:
1. Python:
“`python
def calculate_sum(a, b=5, c):
return a + b + c
# Error: non-default argument follows default argument
“`
2. C++:
“`cpp
int sum(int a, int b = 2, int c) {
return a + b + c;
}
// Error: non-default argument follows default argument
“`
3. JavaScript:
“`javascript
function multiply(a, b = 2, c) {
return a * b * c;
}
// Error: non-default argument follows default argument
“`
Best Practices to Avoid the Error in Programming:
To avoid the “non-default argument follows default argument” error, it is crucial to follow some best practices when defining functions:
1. Place non-default arguments before default arguments.
2. Ensure all required non-default arguments are included when calling the function.
3. Provide default arguments only at the end of the argument list.
Potential Drawbacks of Using Default Arguments in Functions:
While default arguments offer flexibility and ease of use, they may introduce certain drawbacks. One significant drawback is that default arguments can lead to confusion and ambiguity when misused or overused. They can also make a function’s behavior less predictable, especially when combined with mutable data types as default values. Additionally, default arguments can hinder code readability if their usage becomes excessive or arbitrary.
How to Fix the “Non-Default Argument Follows Default Argument” Error:
To fix the error, the non-default argument should be placed before the default argument in the function declaration.
For example, in Python:
“`python
def concatenate_strings(a, c, b=’ world!’):
return a + b + c
“`
Alternatively, if the order of the arguments is crucial, the default value can be removed, forcing the programmer to provide a value for that parameter.
Alternatives to Using Default Arguments When Designing Functions:
When designing functions, there are alternative approaches to default arguments:
1. Overloading: Create multiple versions of the function with different names or signatures to handle different argument requirements.
2. Use `None` as a sentinel value: Assign `None` to an argument and check for it within the function. If `None` is detected, then use a default value or perform a different behavior.
In conclusion, understanding the difference between default and non-default arguments is crucial for writing error-free code. The “non-default argument follows default argument” error can be easily avoided by following best practices and ensuring the correct order of arguments. While default arguments offer convenience in function design, it is essential to consider their potential drawbacks and explore alternative solutions when necessary. By adhering to these guidelines, programmers can develop robust and error-free software systems.
Python : Syntaxerror: Non-Default Argument Follows Default Argument
What Does Non-Default Argument Follows Default Argument Mean In Python?
In Python, a common error that programmers encounter is the “non-default argument follows default argument” error. This error occurs when defining a function with arguments, where a default argument is followed by a non-default argument. This issue occurs due to the way Python handles default arguments and can cause unexpected behavior or errors in the code. In this article, we will go in-depth to understand the reasons behind this error, its implications, and how to resolve it effectively.
The error message “non-default argument follows default argument” is generated by the Python interpreter to warn programmers about the improper arrangement of function arguments. To comprehend this error fully, we need to understand the concepts of default arguments and their limitations.
Default arguments in Python allow us to specify a default value for a function parameter if no argument value is given during a function call. This enables flexibility in function calls, as users can choose to omit certain arguments, and the function will use the default value instead. This simplifies code implementation and provides a seamless user experience.
Let’s consider an example to illustrate this error. Suppose we have a function named “calculate_area” that calculates the area of a rectangle and accepts two arguments, “length” and “width”. We decide to set the default value of “width” to 5. Here’s the code snippet:
“`python
def calculate_area(length, width=5):
area = length * width
return area
“`
This code will initially seem fine, as it compiles without any errors. However, problems arise when we attempt to define another argument after a default argument. For instance, let’s say we want to enhance our function to include a parameter called “color” to represent the color of the rectangle. Thus, we modify the code as follows:
“`python
def calculate_area(length, width=5, color):
area = length * width
return area
“`
Now, if we try to run this code, we will receive the “non-default argument follows default argument” error. The reason behind this error is the positional nature of arguments in Python. When defining a function, the parameters without any default values should be placed before the parameters with default values. So, in the above code, since “color” is a non-default argument following default arguments (“length” and “width”), Python raises an error.
To resolve this error, we need to rearrange the parameters in the function definition. In this case, we can solve the error by moving the “width” parameter after the “color” parameter:
“`python
def calculate_area(length, color, width=5):
area = length * width
return area
“`
By making this change, the error will disappear, and the function will work as expected.
FAQs:
1. Is it mandatory to provide default arguments in a function?
No, it is not mandatory to provide default arguments in a function. Default arguments are optional and can be omitted during a function call. However, all non-default arguments must be provided. Failure to do so results in a TypeError.
2. Can I have multiple default arguments in a function?
Yes, you can have multiple default arguments in a function. However, it is important to remember that when defining multiple default arguments, they should be placed at the end of the parameter list. Placing non-default arguments after default arguments will result in the “non-default argument follows default argument” error.
3. Can I override default arguments in a function call?
Yes, you can override default arguments in a function call by specifying a different value for the argument. When a function is called with an explicit argument value, it overrides the corresponding default value.
4. Are default arguments evaluated at runtime?
No, default arguments are evaluated only once, during function definition. The evaluated value is then used as the default value in subsequent function calls.
5. Can I use variables as default argument values?
Yes, variables can be used as default argument values. However, caution should be exercised when using mutable objects (e.g., lists, dictionaries) as default argument values, as they can retain their state throughout multiple function calls.
In conclusion, the “non-default argument follows default argument” error in Python arises when a non-default argument is defined after a default argument. This error can be resolved by rearranging the arguments in the function definition, placing non-default arguments before default arguments. Understanding how default arguments work and their limitations is crucial for writing efficient and error-free Python code. By following the guidelines discussed in this article, you can ensure smooth execution of your functions and avoid unexpected errors.
What Are The Disadvantages Of Default Arguments?
Default arguments are a powerful feature in many programming languages that allow you to assign a default value to a function parameter. This means that if the caller does not provide a value for that parameter, the default value will be used instead. While default arguments can be convenient, they also come with several disadvantages that developers should be aware of. In this article, we will explore these disadvantages and discuss why and when default arguments should be used cautiously.
1. Confusion and readability issues:
Default arguments can add complexity to the code, especially when multiple parameters have default values. It becomes difficult to understand the behavior of a function by just looking at its signature. Readers may need to dive into the function implementation to fully comprehend how it behaves. Overuse of default arguments can make the code less readable and harder to maintain, as the assumed values may not be immediately obvious to someone new to the codebase.
2. Hidden logic and unexpected behavior:
When default arguments are used, it adds a level of hidden logic to the function. While this can help reduce code verbosity, it also increases the chances of unexpected behavior. If developers are not aware of the default values assigned to parameters, they may inadvertently rely on them and assume certain values will always be used. However, if those default values change in future versions or in specific scenarios, it can lead to bugs that are difficult to trace and fix.
3. Issues with mutable default arguments:
In some programming languages, like Python, default arguments are evaluated only once during the function definition. This can lead to unexpected behavior when mutable objects, like lists or dictionaries, are used as default arguments. If the default argument is modified inside the function, the changes will persist across multiple invocations. This can create subtle bugs and make it harder to reason about the function’s behavior.
4. Dependency on implementation details:
Changing the default argument value can inadvertently affect other parts of the codebase that rely on that function. If multiple functions depend on the same default value, modifying the default value may break those functions unexpectedly. This can lead to cascading changes throughout the codebase, causing maintenance challenges and increasing the risk of introducing bugs.
5. Limited flexibility:
Default arguments limit the flexibility of the function as they restrict the values that can be passed to the parameter. If the default argument is not suitable for a particular use case, the caller needs to override it by explicitly passing a different value. This can be cumbersome and defeat the purpose of having default arguments in the first place. Moreover, when multiple parameters have default arguments, it becomes more challenging to remember the order in which the values should be passed, resulting in potential errors.
6. Testing and debugging complexities:
When functions have default arguments, testing all possible combinations of parameter values can become a challenging task. The number of test cases increases exponentially as each parameter can have multiple combinations of values with and without the default ones. Additionally, because default arguments introduce hidden logic, debugging issues arising from incorrect parameter values becomes more complex as it may not be apparent which combination of default and non-default values was used.
FAQs:
Q1. Should I never use default arguments?
While default arguments have disadvantages, they can still be valuable in certain cases. If the default value is carefully chosen and clearly documented, it can improve code readability and simplify function calls in many situations. However, it is important to use them judiciously and consider the potential drawbacks before employing them extensively.
Q2. Can I modify default arguments inside a function?
In some programming languages, it is possible to modify mutable default arguments within a function. However, doing so can lead to unexpected behavior and should generally be avoided. Instead, it is recommended to assign None as a default value and check for it explicitly within the function to handle cases where the parameter is not provided.
Q3. Are default arguments supported in all programming languages?
Default arguments are a language-specific feature, and not every programming language supports them. While popular languages like Python, C++, and JavaScript have default argument support, others may handle it differently or not support it at all. It is essential to consult the documentation or language specifications to determine if default arguments can be used in a specific language.
Q4. Can default arguments cause performance issues?
In most cases, default arguments do not significantly impact the performance of a program. However, if the default value involves complex calculations or resource-intensive operations, it can affect the program’s speed. This is especially true if the function is called frequently or in a performance-critical context. Therefore, it is important to consider the performance implications when choosing default argument values.
In conclusion, default arguments can be a double-edged sword in programming. While they provide convenience and reduce code duplication, they introduce complexity and potential bugs. It is crucial for developers to weigh the advantages and disadvantages before deciding to use default arguments in their codebase. By using them judiciously and documenting their behavior clearly, developers can mitigate some of the drawbacks and leverage the benefits of default arguments effectively.
Keywords searched by users: non default argument follows default argument Positional argument follows keyword argument, Default parameter Python, Got multiple values for argument
Categories: Top 83 Non Default Argument Follows Default Argument
See more here: nhanvietluanvan.com
Positional Argument Follows Keyword Argument
In Python, there can be instances when you need to pass arguments to a function. Python allows you to pass these arguments in two different ways: positional arguments and keyword arguments. However, there is a specific rule to using these arguments to avoid any confusion or errors in your code. This article will dive into the topic of positional argument following keyword argument, explaining how it can be used and why it is important to follow this rule.
Firstly, let’s understand the difference between positional and keyword arguments. A positional argument is passed to a function based on its position in the function’s argument list. On the other hand, a keyword argument is passed to a function using its corresponding keyword. Consider the following example:
“`python
def greet(name, age):
print(f”Hello {name}, you are {age} years old.”)
greet(“John”, age=25)
“`
In this example, “John” is a positional argument passed to the `name` parameter of the `greet` function. The keyword argument `age=25` is explicitly assigned to the `age` parameter. The output of this code would be: “Hello John, you are 25 years old.”
Now, let’s discuss why it is important to follow the rule of not allowing positional arguments to follow keyword arguments. This rule ensures that there is no ambiguity in the way arguments are passed to a function. If this rule is violated, it can lead to unexpected behavior or errors, making your code difficult to debug.
Consider the following example:
“`python
def greet(name, age):
print(f”Hello {name}, you are {age} years old.”)
greet(age=25, “John”)
“`
In this code snippet, the positional argument `”John”` is placed after the keyword argument `age=25`. This violates the positional argument follows keyword argument rule. Running this code would result in a `SyntaxError`.
To avoid such errors, it is essential to follow the proper order of passing arguments. Any positional arguments must always come before any keyword arguments when calling a function.
Now let’s answer some frequently asked questions about positional argument following the keyword argument:
**Q: Why would I want to use positional arguments and keyword arguments together?**
A: Using both of these argument types gives you flexibility when calling a function. Positional arguments provide the basic functionality, while keyword arguments allow you to explicitly assign values to specific parameters. This combination can result in code that is more readable and easier to maintain.
**Q: How do I know the order of the parameters in a function that I want to call?**
A: The order of the parameters is typically defined in the function definition itself. You can check the parameter order in the function’s documentation or the source code. It’s always a best practice to refer to these resources before calling the function.
**Q: Can I mix positional and keyword arguments in any order?**
A: Yes, you can mix positional and keyword arguments, but remember that positional arguments must come before any keyword arguments.
**Q: What if I forget to pass a required argument as a keyword argument?**
A: If you forget to pass a required argument as a keyword argument, it would result in a `TypeError`. For example:
“`python
def greet(name, age):
print(f”Hello {name}, you are {age} years old.”)
greet(name=”John”) # This would raise a TypeError
“`
In this case, since the `age` argument is not provided, a `TypeError` would be raised.
**Q: Can I have positional arguments after keyword arguments within the function definition?**
A: Yes, you can have positional arguments after keyword arguments within the function definition. This rule only applies to the order in which arguments are passed when calling the function.
Remember, adhering to the positional argument follows keyword argument rule is crucial to avoid any errors or confusion in your Python code. Always place positional arguments before keyword arguments when calling a function. This practice will ensure that your code is more readable, maintainable, and less prone to errors.
In conclusion, by understanding the difference between positional and keyword arguments and following the rule of not allowing positional arguments to follow keyword arguments, you can write cleaner and more reliable Python code. Using positional and keyword arguments appropriately will enhance the readability and maintainability of your code, ultimately making your job as a programmer much more efficient.
Default Parameter Python
When defining a function in Python, we can assign default values to one or more of its arguments. This means that if the function is called without providing a value for these arguments, the default values will be used instead. To specify a default parameter, we simply include an equal sign followed by the default value after the argument name in the function definition.
For example, let’s consider a function called “greet” that takes two parameters, “name” and “message”. We want the “message” parameter to have a default value of “Hello”:
“`python
def greet(name, message=”Hello”):
print(message, name)
“`
In the above code, if we call the “greet” function without providing a value for the “message” parameter, it will default to “Hello”:
“`python
greet(“Alice”)
“`
Output:
“`
Hello Alice
“`
However, if we provide a value for the “message” parameter, it will override the default value:
“`python
greet(“Bob”, “Good morning”)
“`
Output:
“`
Good morning Bob
“`
Default parameters are especially useful when we have functions with many arguments, and we want to provide a sensible default value for some of them. By using default parameters, we can define flexible functions that can be called with a variable number of arguments, depending on the specific use case.
Python also allows us to use the default value of a parameter defined earlier in the parameter list for subsequent parameters. This allows us to set a parameter’s default value based on the value of a previous parameter.
For instance, let’s consider a function called “divide” that takes two parameters: “numerator” and “denominator”. We want the “denominator” parameter to have a default value equal to the default value of the “numerator” parameter:
“`python
def divide(numerator, denominator=numerator):
return numerator / denominator
“`
In this example, if we call the “divide” function without providing a value for the “denominator” parameter, it will default to the value of the “numerator” parameter:
“`python
result = divide(10)
“`
Output:
“`
1.0
“`
Here, since we didn’t provide a value for the “denominator” parameter, it defaulted to the value of the “numerator” parameter, resulting in a division of 10 by 10, giving us 1.0.
Now, let’s address some common questions about default parameters in Python:
**Q: Can I have a mix of parameters with and without default values in a function?**
A: Yes, you can. You can have any number of parameters with default values followed by parameters without default values. However, once you have a parameter without a default value, you cannot have any more parameters with a default value.
**Q: Can I change the default value of a parameter after defining the function?**
A: Yes, you can change the default value of a parameter by redefining the function with the updated default value. All function calls made after the redefinition will use the new default value.
**Q: What happens if I use a mutable object as a default parameter and modify it within the function?**
A: When a default parameter is a mutable object, such as a list or dictionary, and it is modified within the function, the modification persists across subsequent function calls that omit the corresponding argument. This can lead to unexpected outcomes, so it’s important to exercise caution when using mutable objects as default parameters.
**Q: Can I define default parameters in any order?**
A: No, the parameters with default values must follow the parameters without default values in the function definition.
Default parameters are a fundamental concept in Python that enables more flexibility in function design. By providing default values for function arguments, we can create functions that require fewer explicit arguments, allowing our code to be more concise and adaptable. However, it’s essential to understand the nuances and best practices associated with default parameters to avoid any unexpected behavior.
In conclusion, default parameters in Python allow us to specify default values for function arguments, reducing the need for repetitive code and enhancing the flexibility of our programs. By leveraging default parameters appropriately, we can create more concise and adaptable functions that cater to various use cases.
I hope this article has provided you with a comprehensive understanding of default parameters in Python!
Got Multiple Values For Argument
Understanding the “Got multiple values for argument” error:
The “Got multiple values for argument” error occurs when a function or method receives more arguments than it expects. In Python, every function or method defines a specific number of arguments in its signature, and calling that function with more arguments can lead to this error. Here’s an example of code that generates the “Got multiple values for argument” error:
“`
def greet(name):
print(f”Hello, {name}!”)
greet(“John”, “Doe”)
“`
In this example, the `greet()` function expects a single argument, `name`. However, when the function is called, two arguments, “John” and “Doe”, are provided. As a result, Python raises the “Got multiple values for argument ‘name'” error.
Causes of the “Got multiple values for argument” error:
1. Passing too many values: As shown in the example above, passing more arguments than expected can cause this error. It is crucial to match the number of arguments defined in the function’s signature.
2. Misusing unpacking syntax: When using the `*args` (variable-length arguments) syntax in function definitions, passing multiple arguments directly without unpacking can trigger the error. For instance:
“`
def multiply(a, b, c):
return a * b * c
numbers = [2, 3, 4]
multiply(*numbers) # Error: Got multiple values for argument ‘b’
“`
Here, when `numbers` is passed as an argument, its values try to populate the arguments of the function sequentially. Since the second value of `numbers` is assigned to the second argument (`b`), it leads to the error message.
Resolving the “Got multiple values for argument” error:
To fix this error, we need to ensure that the function or method is called with the correct number of arguments. Here are several solutions to resolve this issue:
1. Adjust the number of arguments: The simplest solution is to modify the function or method to accept the correct number of arguments. By altering the function’s signature, you can avoid this error.
2. Remove excess arguments: If you inadvertently passed extra arguments, remove them from the function call. Ensure that the number of arguments matches the function’s expected argument count.
3. Use keyword arguments: One efficient way to resolve this error is by using keyword arguments. Instead of relying on the positional order to pass arguments, you can specify the argument values with their corresponding keywords. This helps avoid confusion and ensures that the arguments are assigned correctly. Consider the following example:
“`
def calculate_total(price, quantity):
return price * quantity
calculate_total(price=10, quantity=5)
“`
Here, using keyword arguments guarantees that each argument is assigned to its respective parameter, regardless of their order. This bypasses the “Got multiple values for argument” error altogether.
FAQs:
Q: I receive the “Got multiple values for argument” error while using default parameter values. How can I fix it?
A: When using default parameter values in function signatures, ensure that arguments are provided in the correct order. If you want to skip an argument with a default value, pass it explicitly as `None`.
Q: Is it possible to have multiple values for a single argument without an error?
A: Yes, it is feasible to pass multiple values to a single argument in Python. To achieve this, the argument should be set as a variable-length argument (`*args`). However, each value needs to be packed into an iterable, such as a list or tuple, to avoid triggering the “Got multiple values for argument” error.
Q: Can I pass a variable number of arguments to a function?
A: Yes, you can pass a variable number of arguments using the `*args` syntax in the function’s signature. This allows the function to receive any number of arguments, which are then treated as a tuple within the function’s body.
In conclusion, the “Got multiple values for argument” error in Python occurs when more arguments are passed to a function or method than it expects. This error message is commonly encountered during development. However, by understanding the causes and implementing the suggested solutions, you can effectively resolve this error and ensure smooth execution of your code. Remember to carefully match the number of arguments and use keyword arguments where appropriate. Happy coding!
Images related to the topic non default argument follows default argument
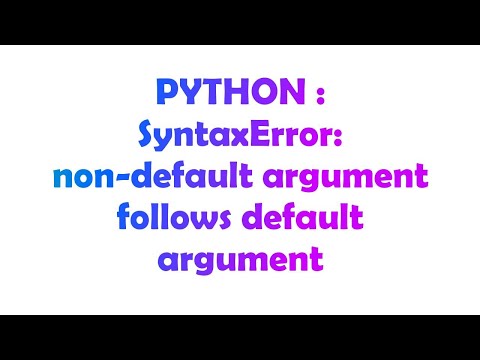
Found 34 images related to non default argument follows default argument theme
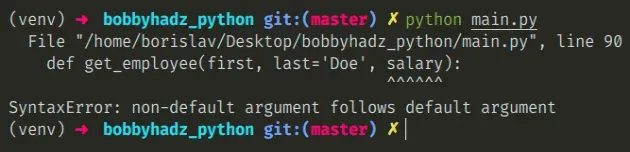
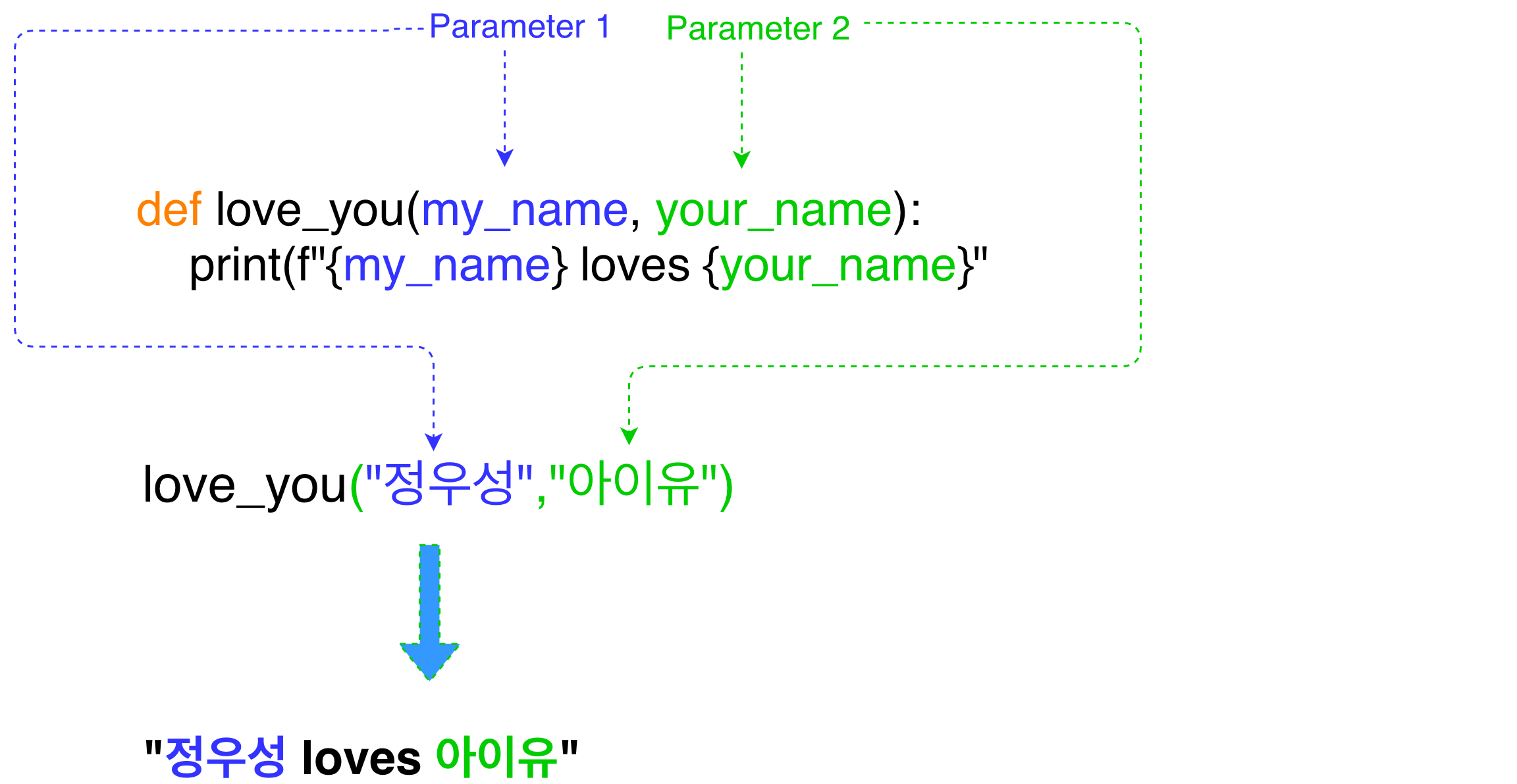
![Solved] SyntaxError: non-default argument follows default argument - Clay-Technology World Solved] Syntaxerror: Non-Default Argument Follows Default Argument - Clay-Technology World](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2020/06/image-40.png?ssl=1)
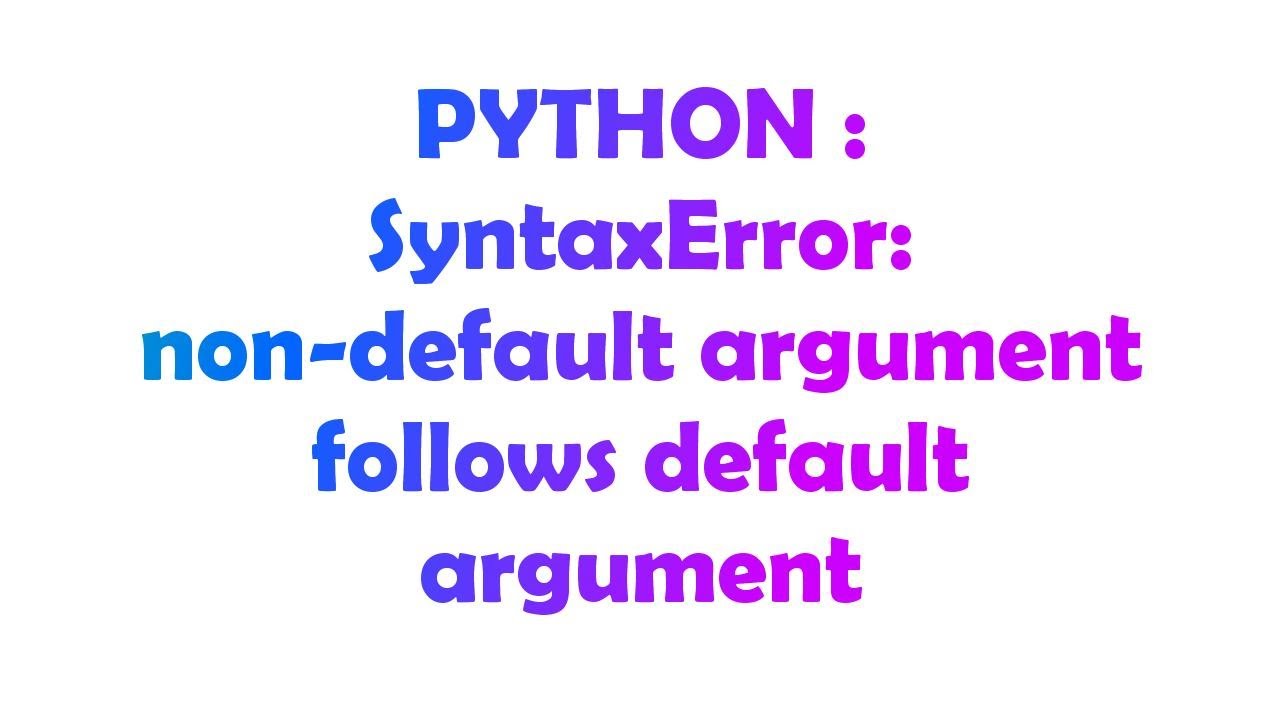

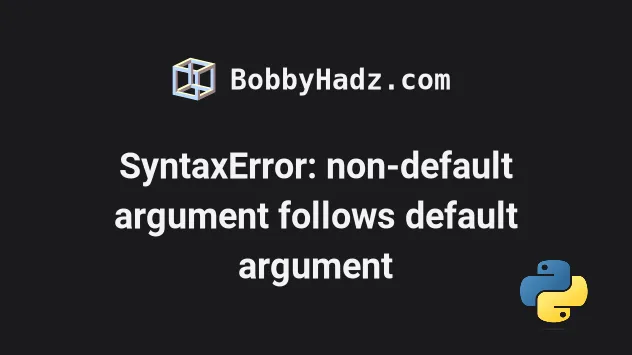
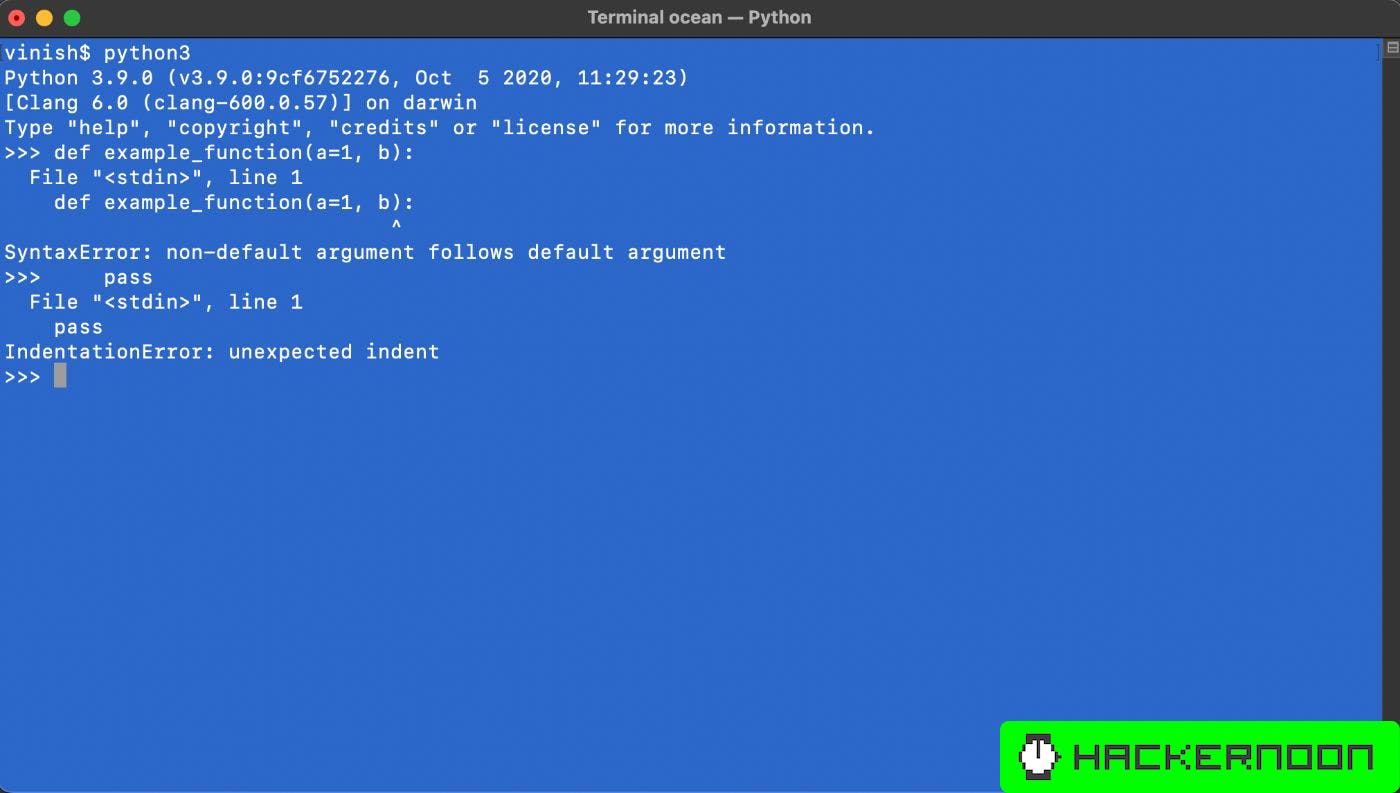

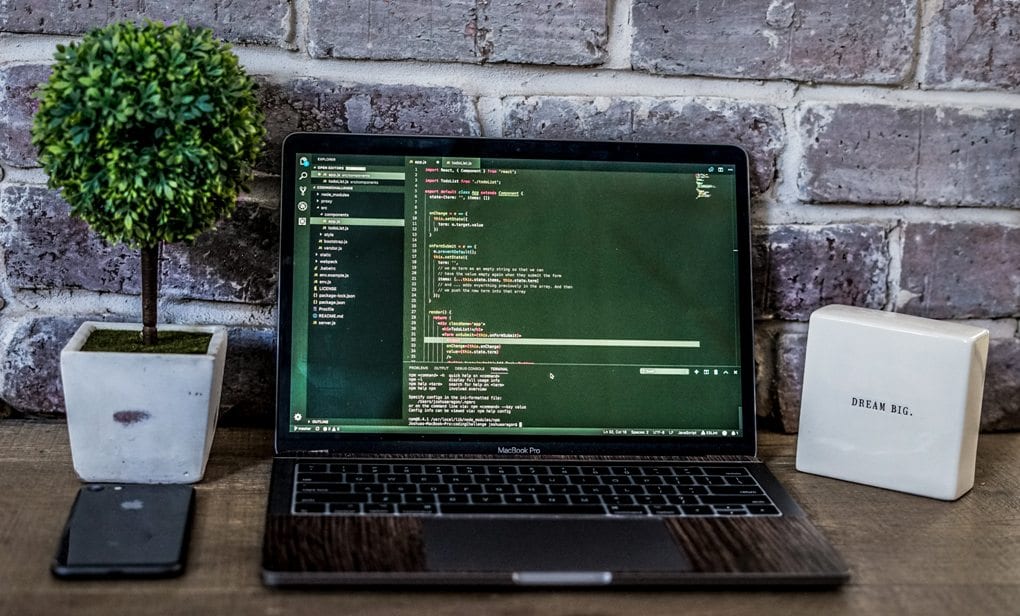
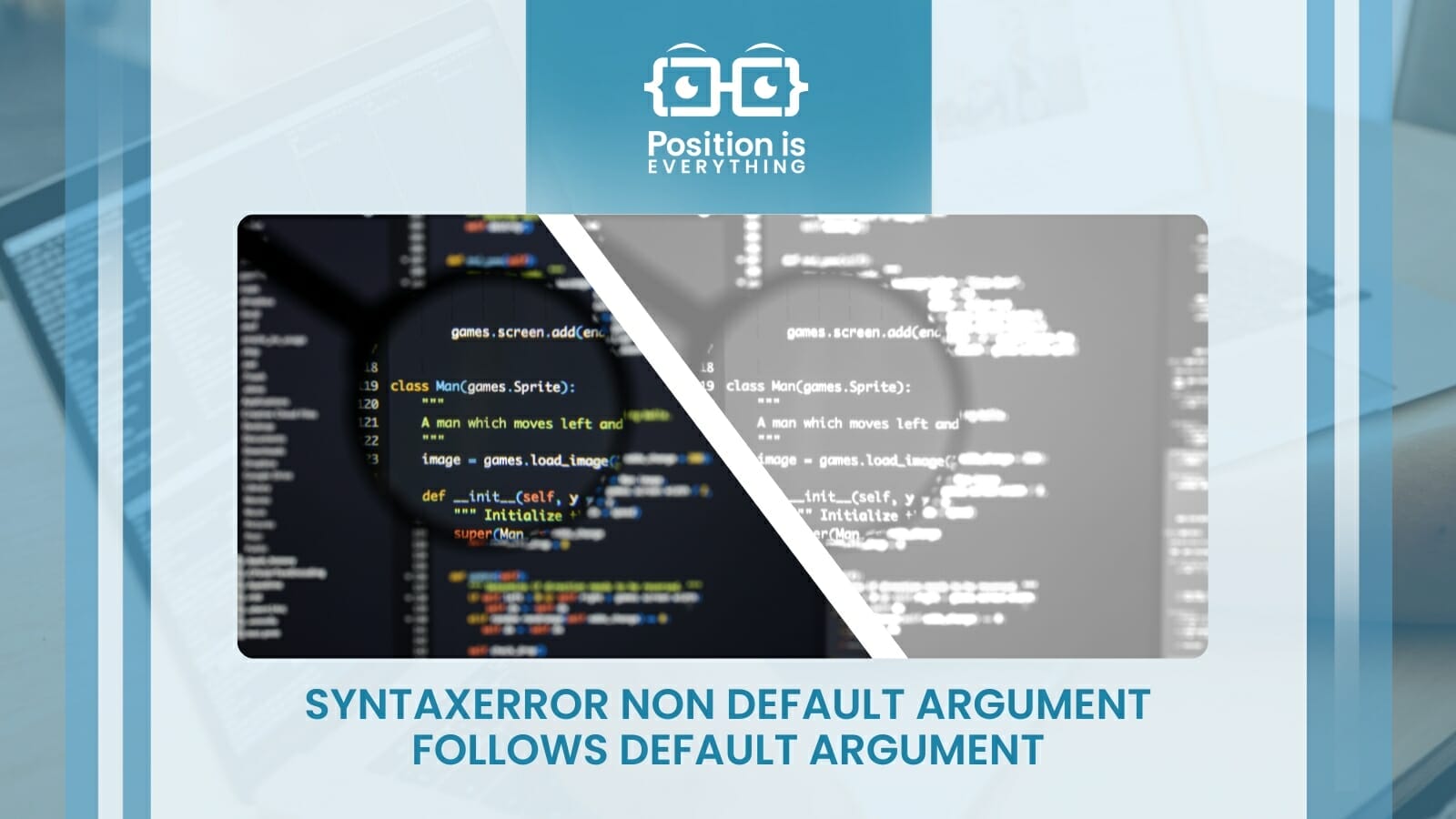
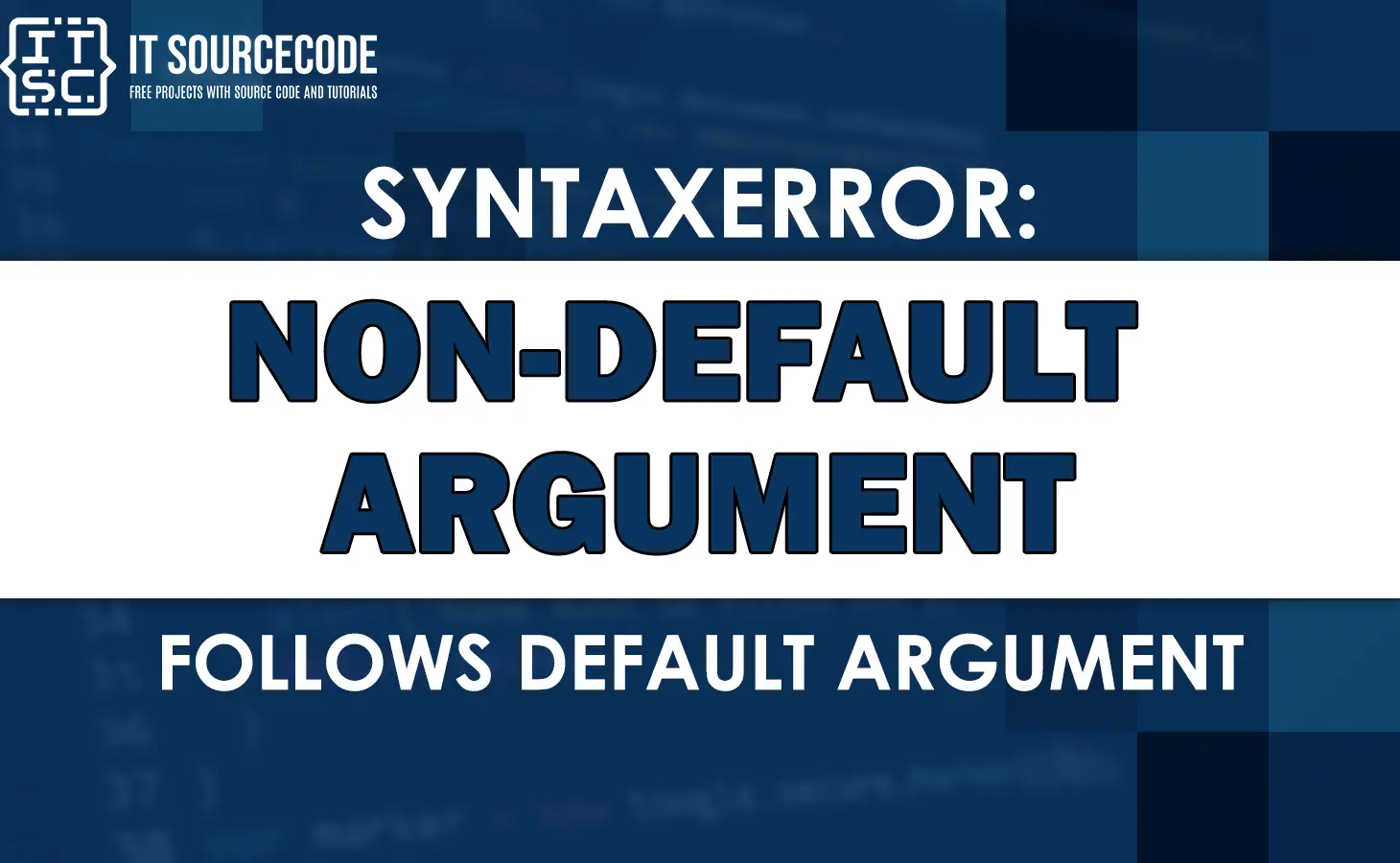
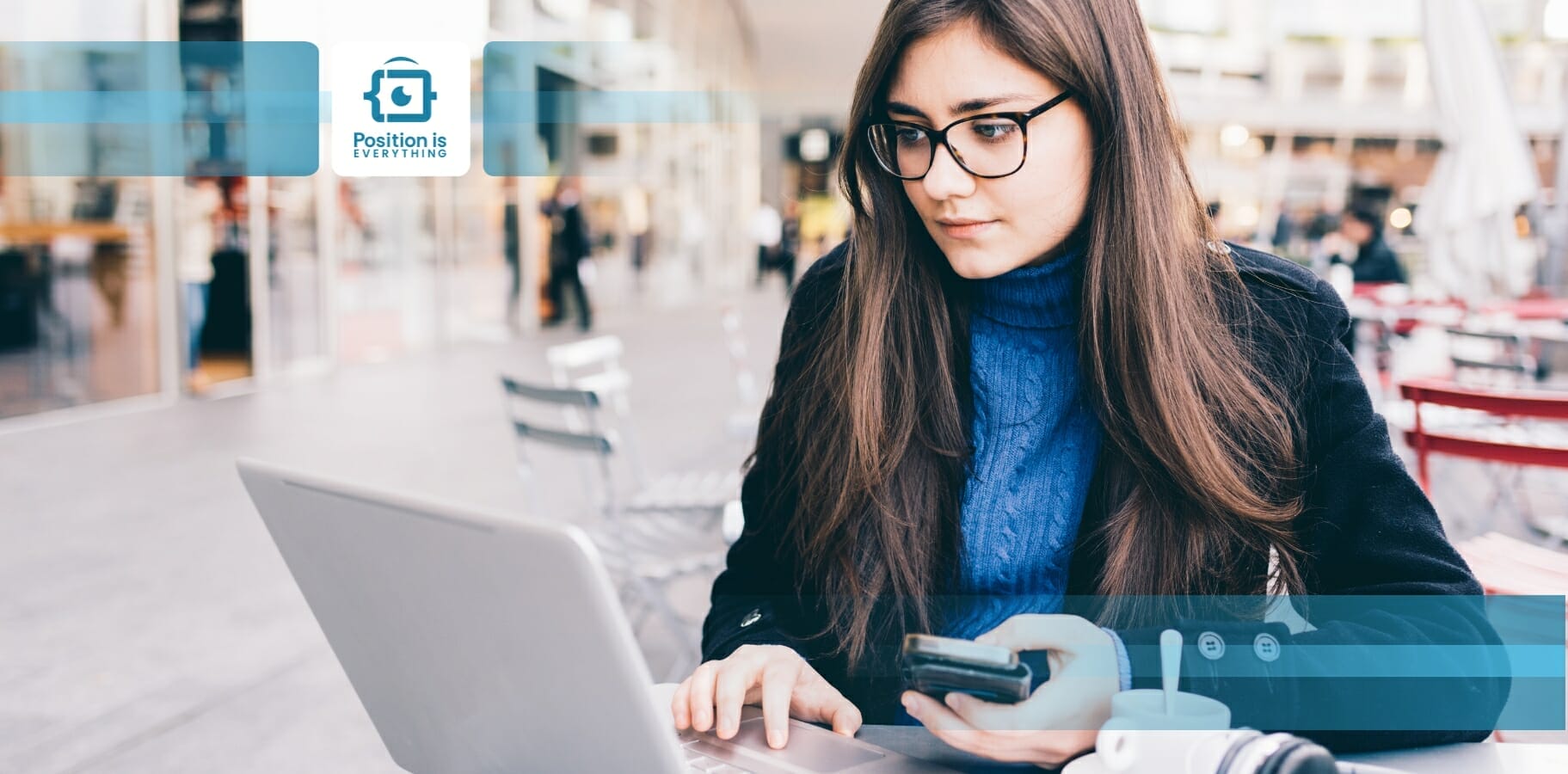
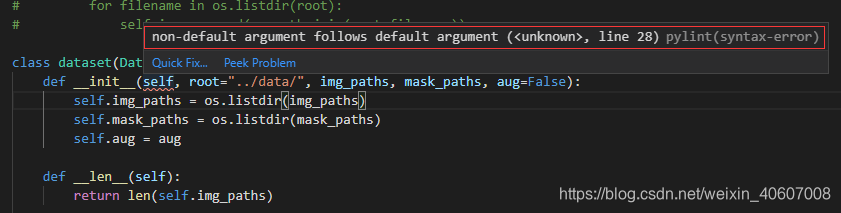
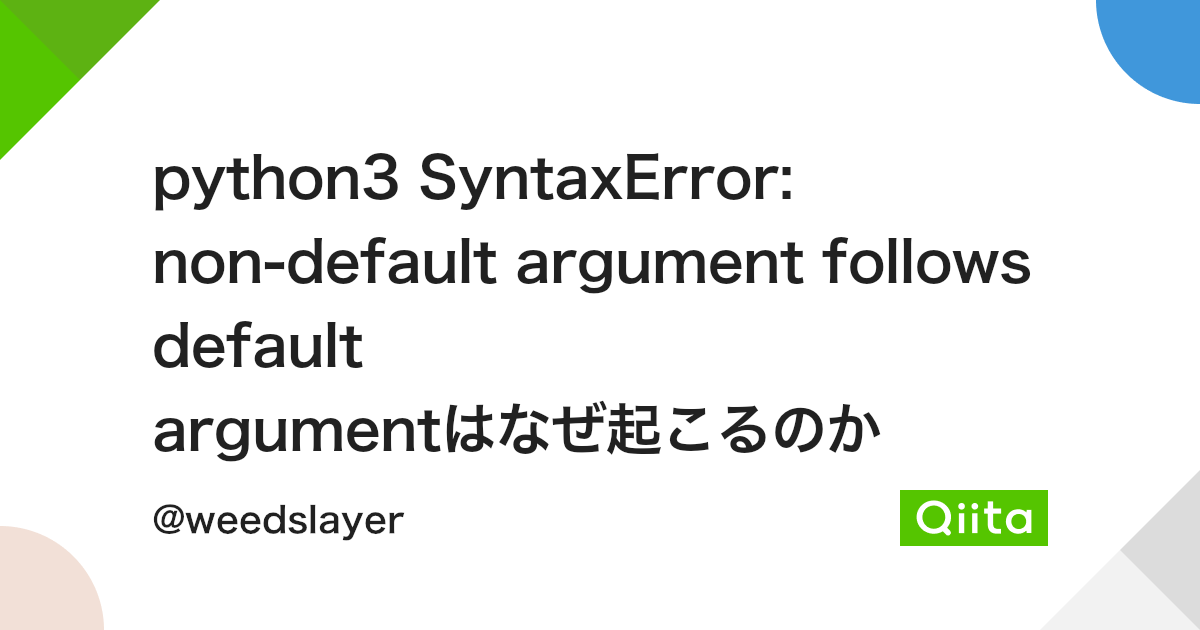
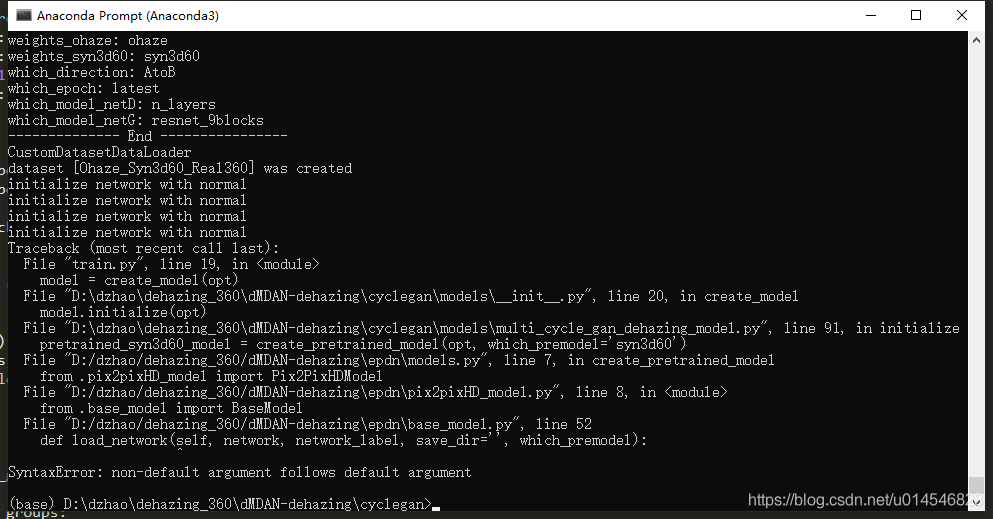

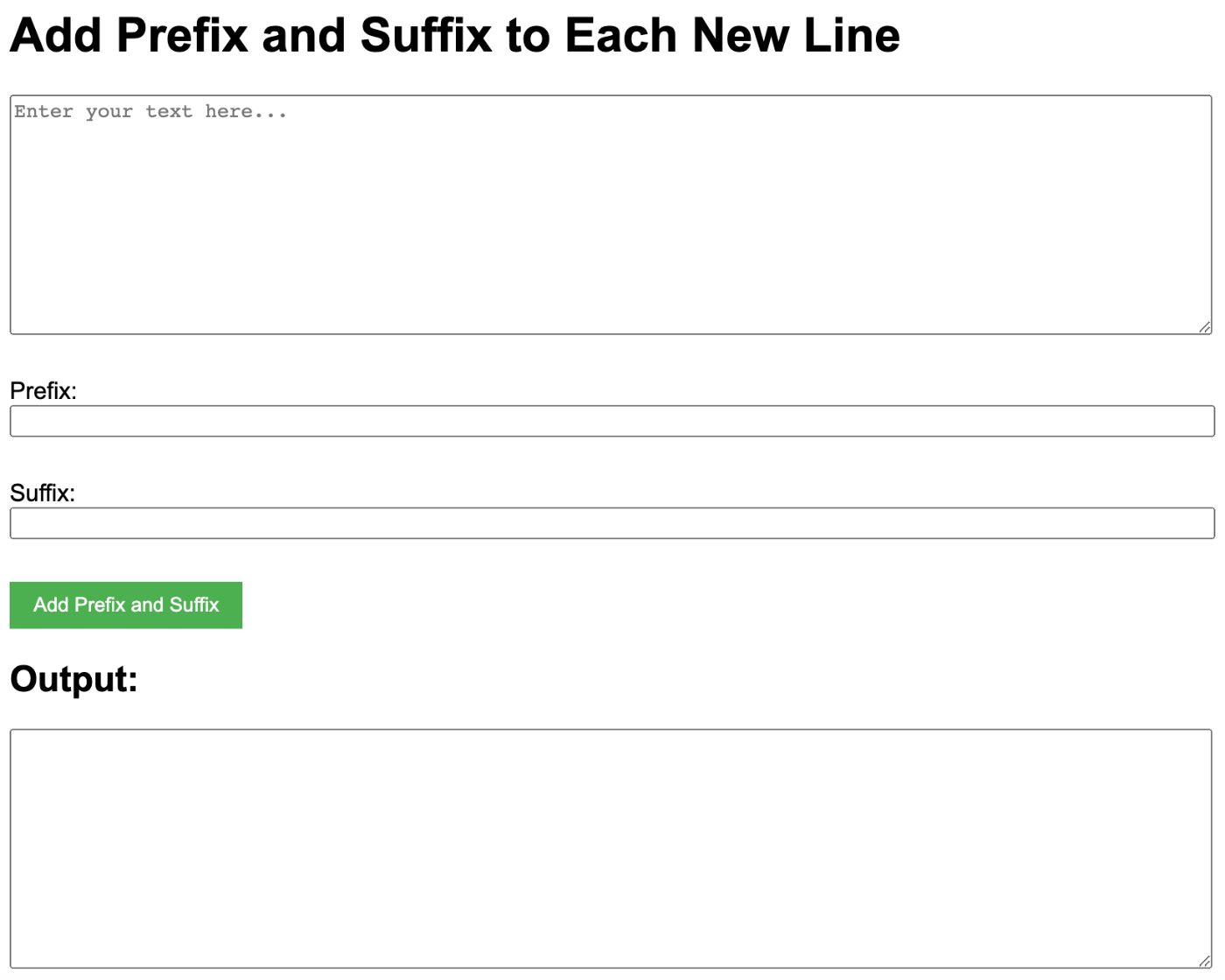
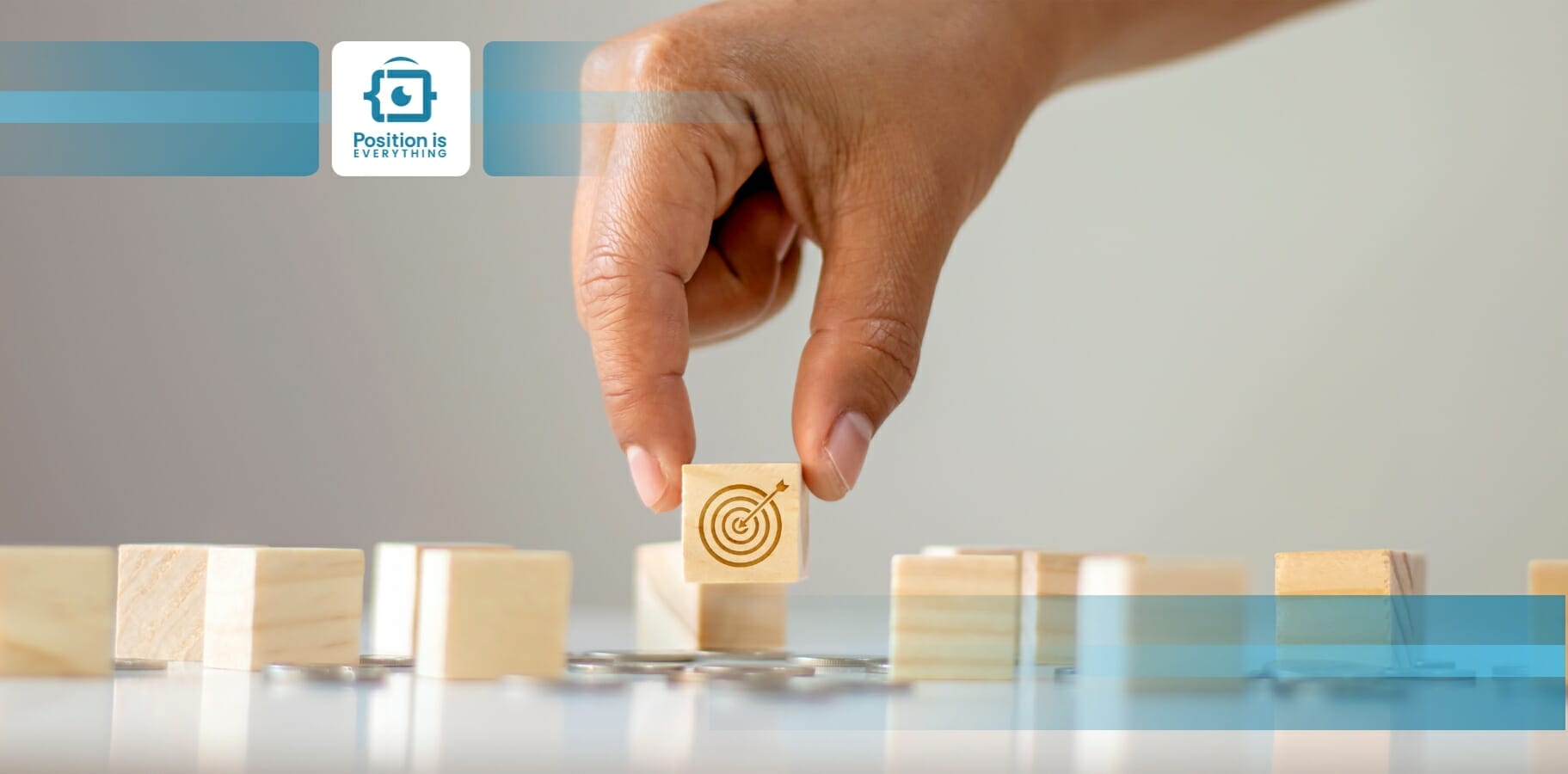
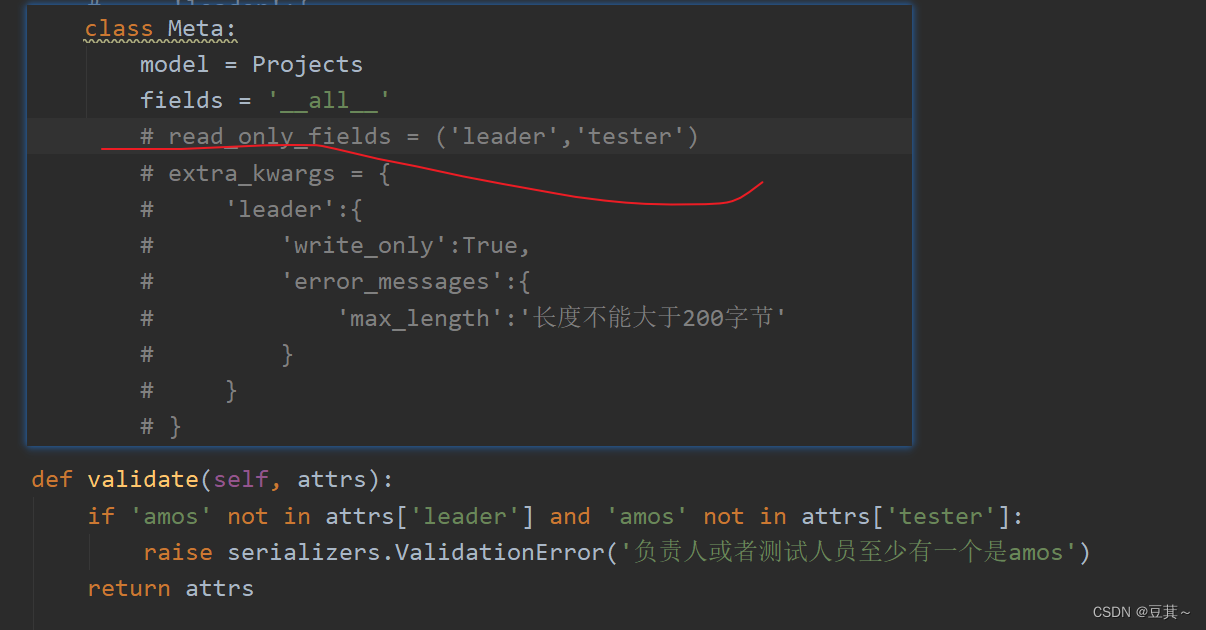
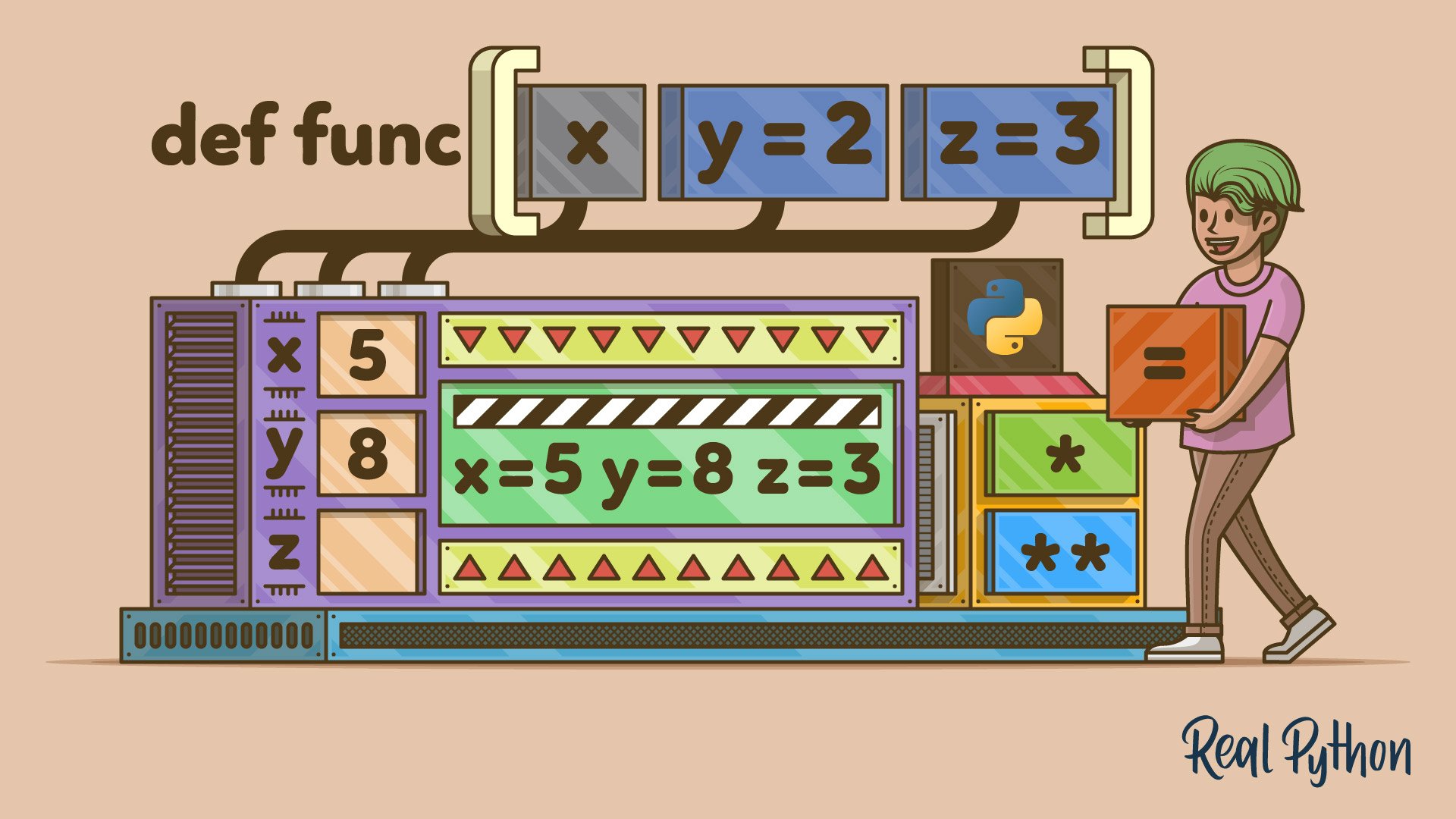
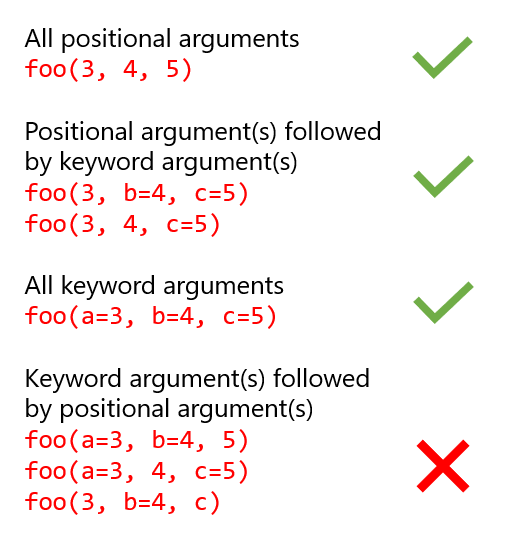

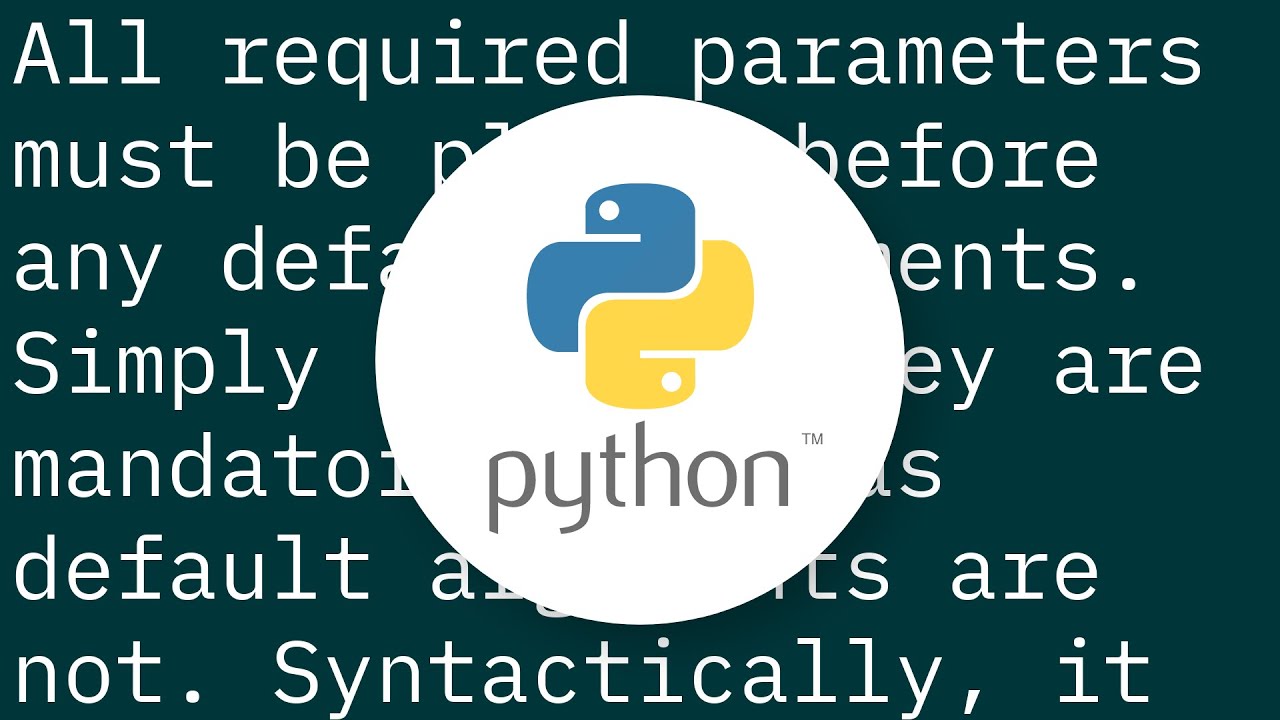

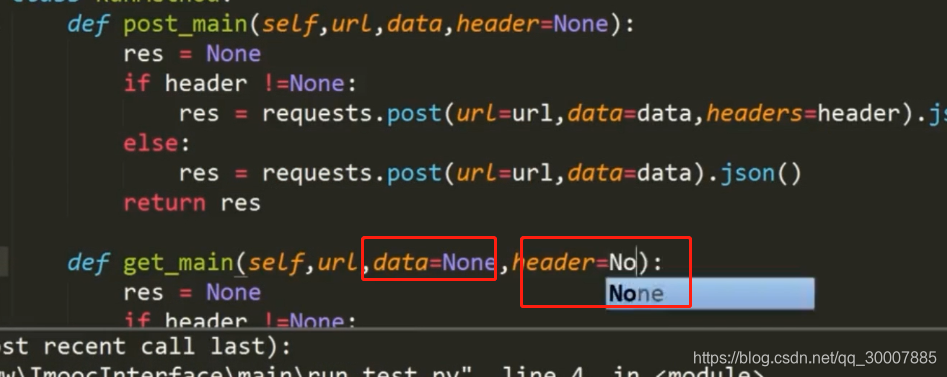
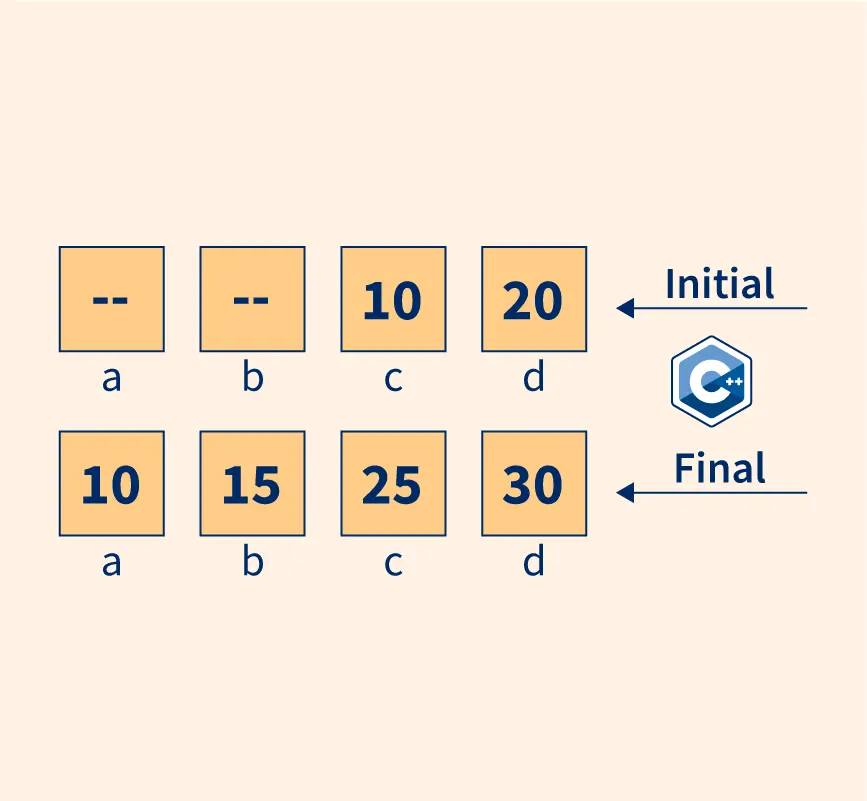
![Python Function Arguments [4 Types] – PYnative Python Function Arguments [4 Types] – Pynative](https://pynative.com/wp-content/uploads/2022/08/python_function_arguments_types.jpg)
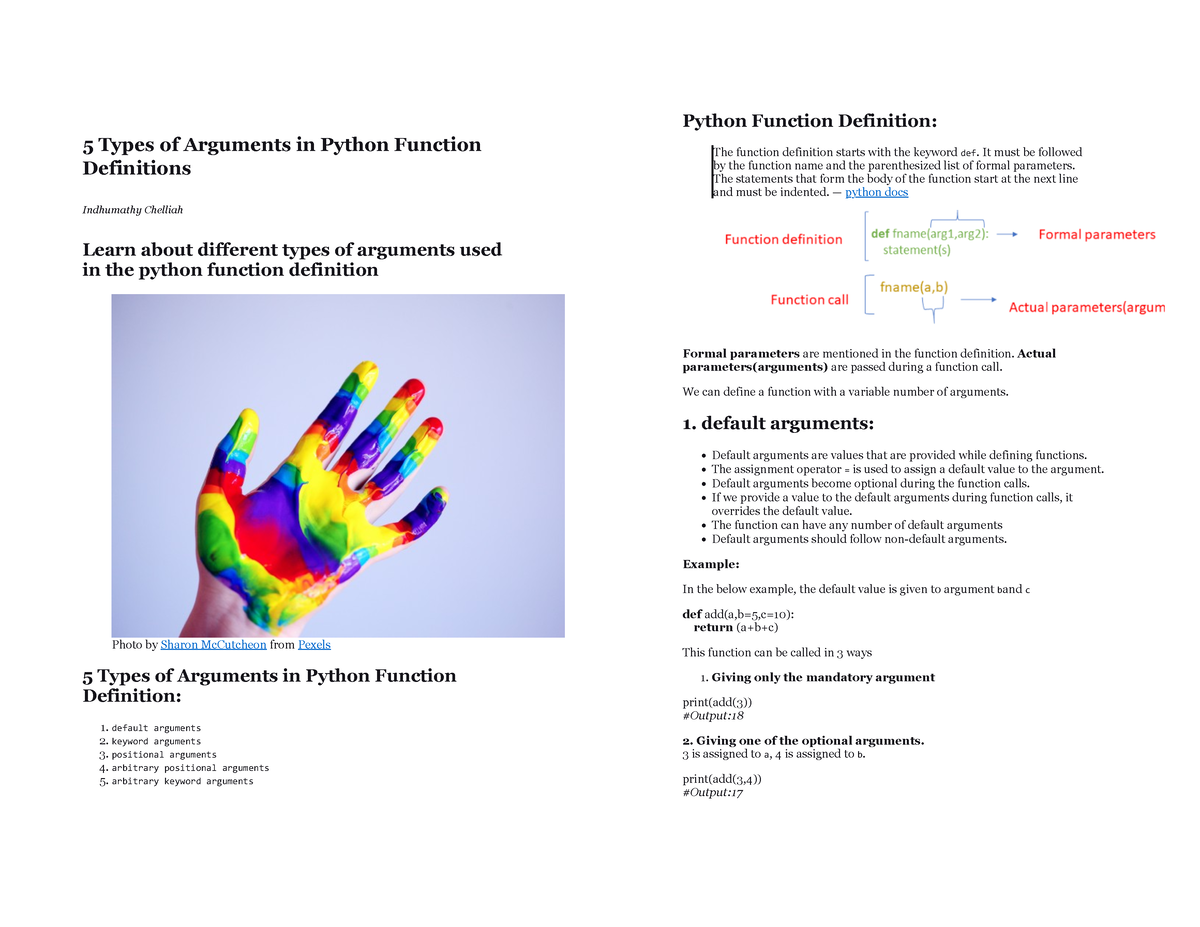
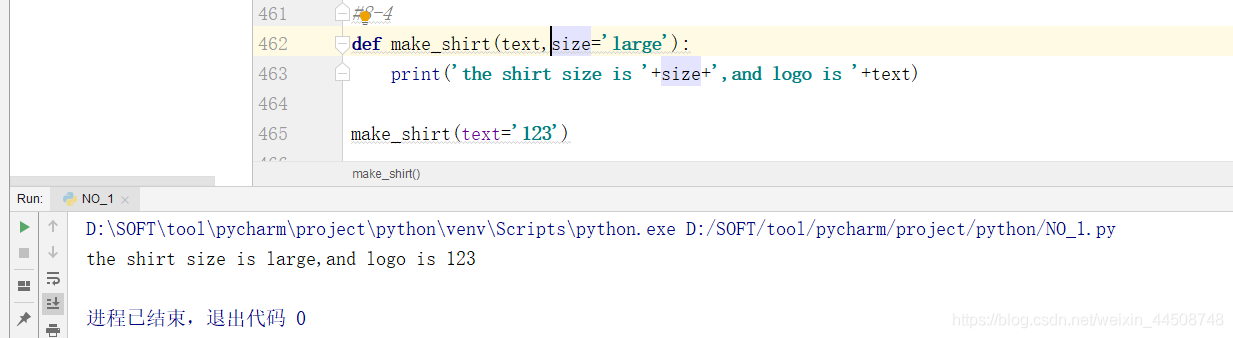
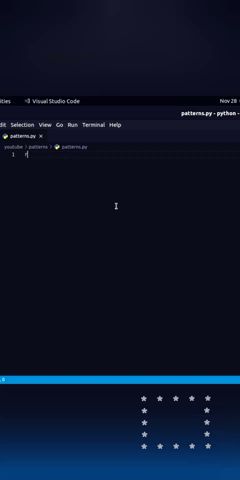
![Solved] SyntaxError: non-default argument follows default argument - Clay-Technology World Solved] Syntaxerror: Non-Default Argument Follows Default Argument - Clay-Technology World](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2021/03/cropped-output.png?fit=350%2C350&ssl=1)
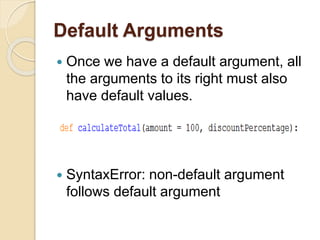

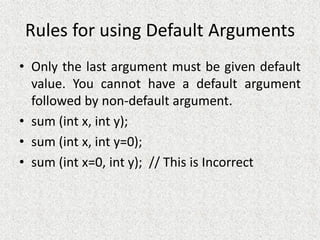
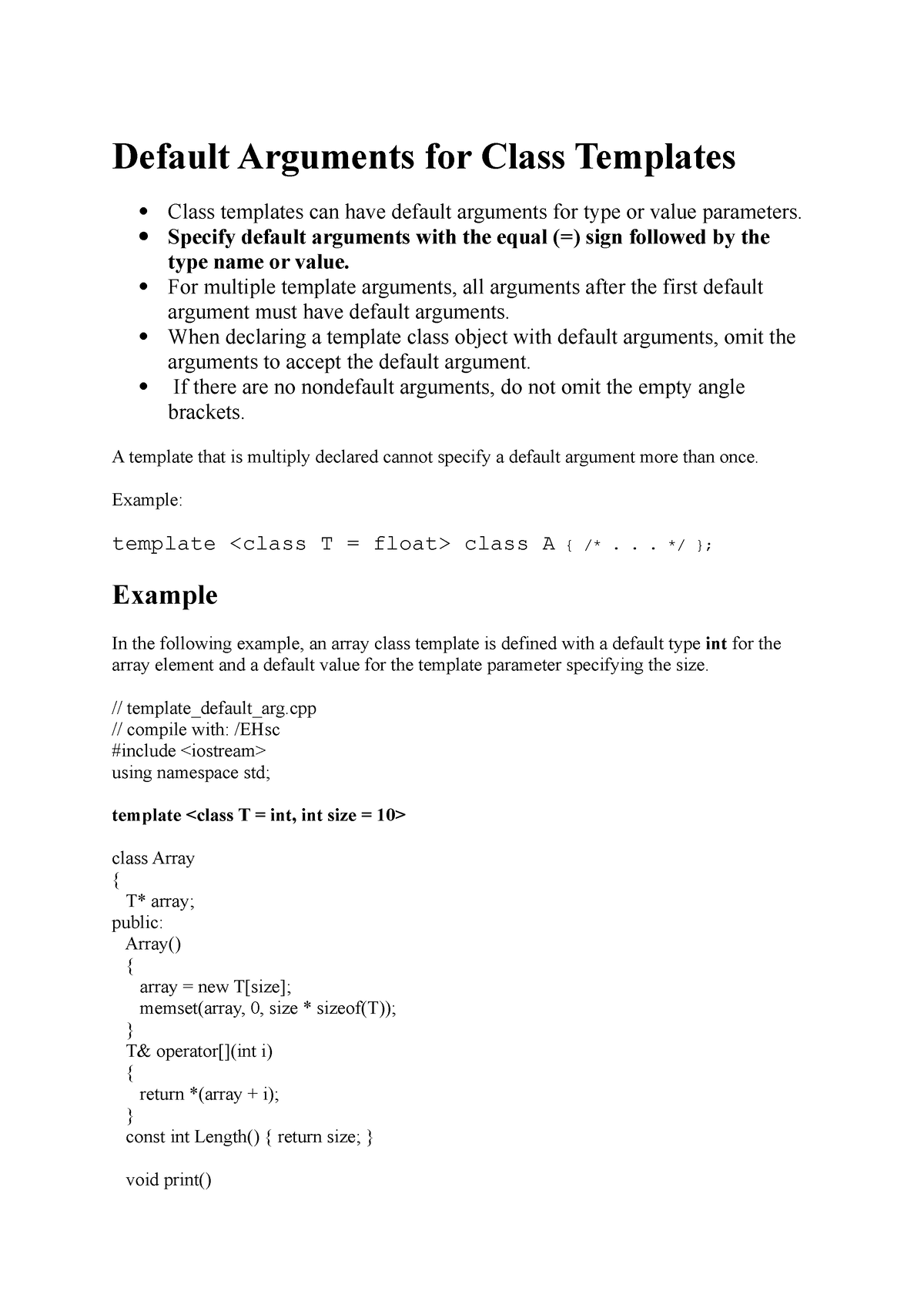

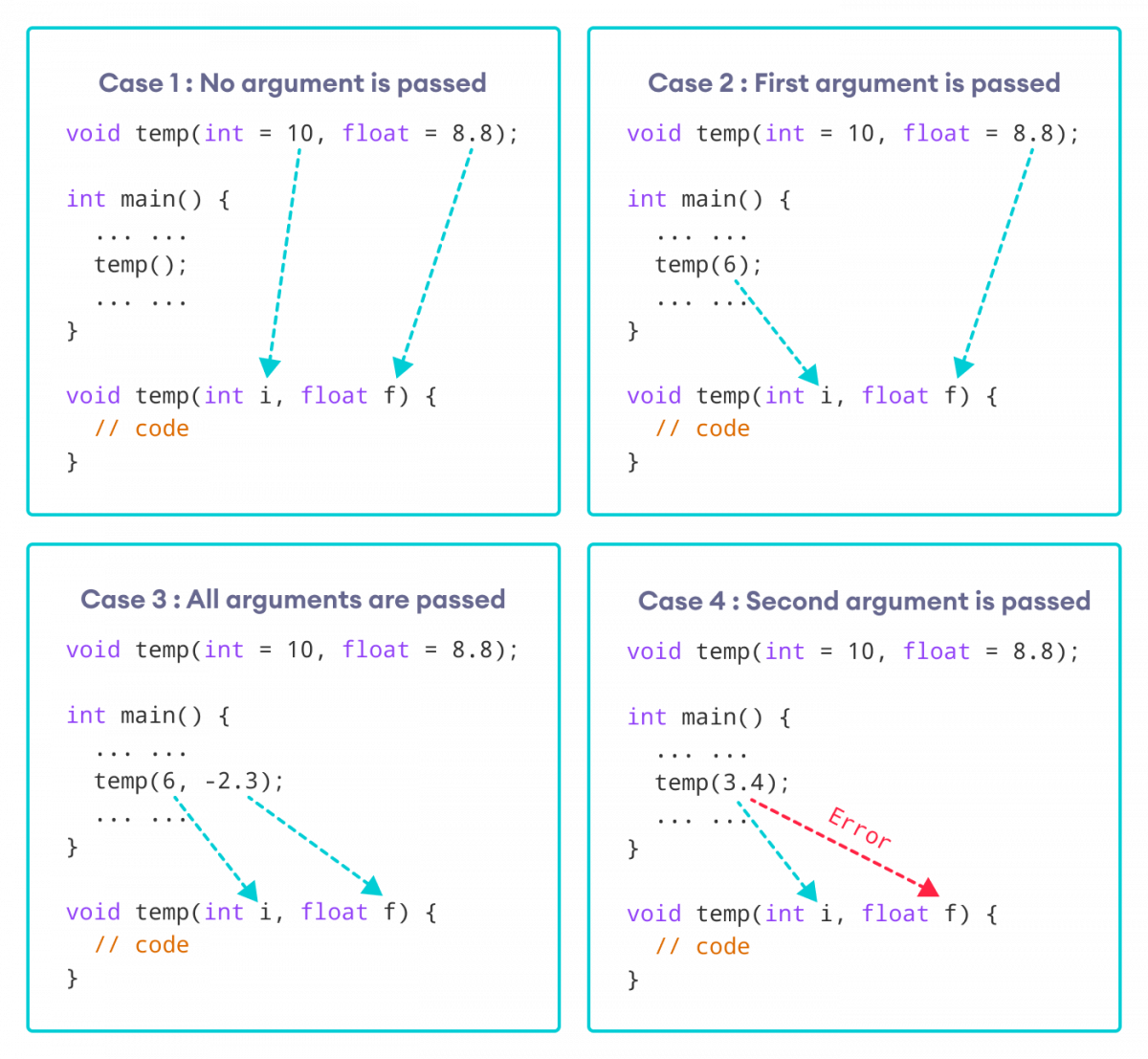
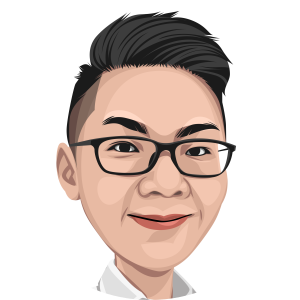
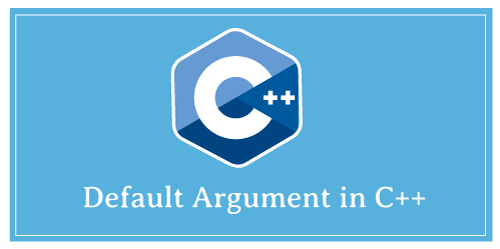
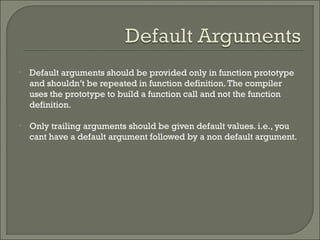
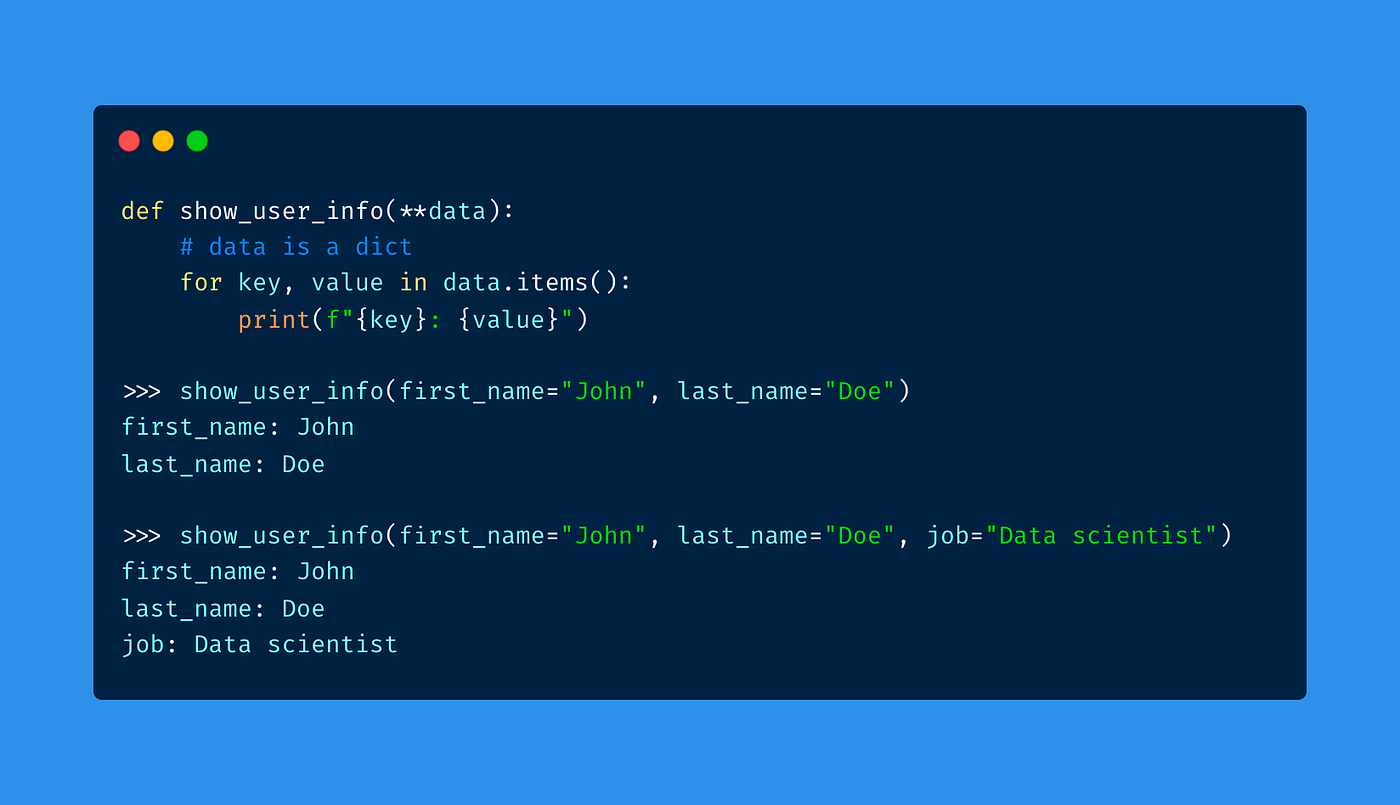
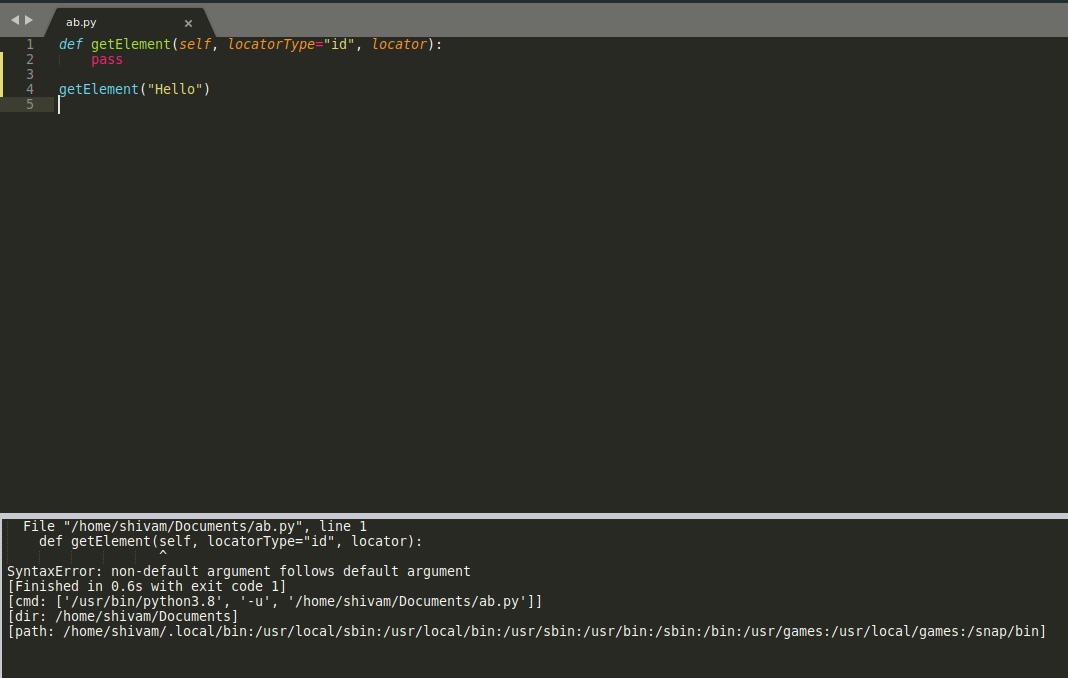
Article link: non default argument follows default argument.
Learn more about the topic non default argument follows default argument.
- How to Resolve the “SyntaxError: Non-Default Argument …
- SyntaxError: non-default argument follows default argument
- SyntaxError: non-default argument follows … – bobbyhadz
- Python SyntaxError: non-default argument follows default
- 4 Ways to Fix Python SyntaxError: Non-Default Argument …
- What is Default Argument in C++? | Scaler Topics
- Default Arguments in C++ – GeeksforGeeks
- Python Function Arguments (Default, Keyword and Arbitrary) – Toppr
- 4 Ways to Fix Python SyntaxError: Non-Default Argument …
- [Solved]: non-default argument follows default argument
- [Solved] SyntaxError: non-default argument follows default …
- “SyntaxError: non-default argument follows default … – GitHub
- How do you handle non-default and default arguments here?
See more: https://nhanvietluanvan.com/luat-hoc/