An Object Reference Is Required For The Non Static Field
In Java, objects are the fundamental building blocks of programs. They are instances of classes, and each object has its own set of properties and behaviors defined by the class it belongs to. In order to access and manipulate these properties and behaviors, we need to create object references.
An object reference is essentially a variable that holds the memory address of an object. It allows us to access and manipulate the properties and behaviors of the object. However, it is important to understand the difference between static and non-static fields in order to use object references correctly.
The Difference Between Static and Non-Static Fields
In Java, fields are variables declared in a class that hold data encapsulated within an object. There are two types of fields: static and non-static.
Static fields are shared among all instances of a class. They belong to the class itself, rather than any specific instance. This means that all objects of that class share the same value for a static field. Static fields are declared with the keyword “static” and can be accessed through the class name.
On the other hand, non-static fields are specific to each instance of a class. They belong to the object and have different values for each individual object. Non-static fields are declared without the “static” keyword and can be accessed using the object reference.
Understanding the Error: “An Object Reference is Required for the Non-Static Field”
When working with non-static fields, it is common to encounter the error message “An object reference is required for the non-static field.” This error occurs when trying to access a non-static field without a valid object reference.
To understand this error, consider the following code:
public class MyClass {
private int myField;
public static void main(String[] args) {
myField = 5;
}
}
In this example, the main method is declared as static, which means it belongs to the class rather than an instance of the class. When trying to access the non-static field “myField” within a static context, the error occurs because there is no object reference to specify which instance of the class to access.
Causes of the Non-Static Field Error
There are several causes for the “An object reference is required for the non-static field” error:
1. Trying to access a non-static field from a static context: As mentioned earlier, non-static fields can only be accessed through an object reference. Attempting to access a non-static field within a static method or class will result in the error.
2. Not creating an instance of the class: If an object reference is not created and assigned to a non-static field, attempting to access that field will result in the error. It is necessary to create an instance of the class using the “new” keyword and assign it to a reference variable.
Resolving the Error through Instance Creation
To resolve the “An object reference is required for the non-static field” error, an instance of the class must be created and assigned to an object reference. This can be done using the “new” keyword followed by the class name and parentheses.
For example:
public class MyClass {
private int myField;
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.myField = 5;
}
}
In this example, an instance of the class MyClass is created using the new keyword, and assigned to the object reference “myObject”. Now, the non-static field “myField” can be accessed through the object reference without causing the error.
Passing an Object Reference to a Non-Static Field
Another way to resolve the error is by passing an object reference to a non-static field through a method. This can be done by creating a method that takes an object reference as a parameter and assigns it to the non-static field.
For example:
public class MyClass {
private int myField;
public void setField(int value) {
myField = value;
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.setField(5);
}
}
In this example, the method “setField” takes an integer value as a parameter and assigns it to the non-static field “myField”. By calling this method on the object reference, the value can be passed to the non-static field without causing the error.
Utilizing the ‘this’ Keyword
The ‘this’ keyword can also be used to reference the current object, allowing access to its non-static fields. ‘this’ refers to the current instance of the class, and can be used within instance methods or constructors.
For example:
public class MyClass {
private int myField;
public void setField(int value) {
this.myField = value;
}
public static void main(String[] args) {
MyClass myObject = new MyClass();
myObject.setField(5);
}
}
In this example, the ‘this’ keyword is used to reference the current object instance within the “setField” method. It allows access to the non-static field “myField” and assigns it the value passed as a parameter.
Dealing with Non-Static Fields in Static Methods or Classes
When working with static methods or classes, it is not possible to directly access non-static fields. However, it is still possible to access them by creating an instance of the class and using the object reference to access the fields.
For example:
public class MyClass {
private int myField;
public static void test() {
MyClass myObject = new MyClass();
myObject.myField = 5;
}
public static void main(String[] args) {
test();
}
}
In this example, the static method “test” creates an instance of the class MyClass using the “new” keyword, and assigns it to the object reference “myObject”. Now, the non-static field “myField” can be accessed and assigned a value within the static context.
Best Practices for Handling Object References in Java
To avoid errors related to object references, it is important to follow these best practices:
1. Always create an instance of a class using the “new” keyword before accessing its non-static fields.
2. Pass object references to non-static fields through methods or constructors whenever possible.
3. Use the ‘this’ keyword to reference the current object instance within instance methods.
4. When dealing with non-static fields in static methods or classes, create an instance of the class and use an object reference to access the fields.
By following these best practices, you can ensure the correct usage of object references and prevent errors related to non-static fields.
FAQs
Q: Can non-static fields be accessed directly within static methods or classes?
A: No, non-static fields cannot be directly accessed within static methods or classes. They require an object reference to access them.
Q: How can I pass an object reference to a non-static field?
A: You can pass an object reference to a non-static field by creating a method or constructor that takes the reference as a parameter and assigns it to the field.
Q: What does the ‘this’ keyword do?
A: The ‘this’ keyword is used to reference the current object instance within instance methods or constructors. It allows access to the object’s non-static fields.
Q: How can I access non-static fields in static methods or classes?
A: To access non-static fields in static methods or classes, you can create an instance of the class and use an object reference to access the fields.
Q: How can I prevent errors related to object references?
A: To prevent errors related to object references, always create an instance of a class before accessing its non-static fields, pass object references through methods or constructors, use the ‘this’ keyword when referencing non-static fields within instance methods, and create instances of classes when dealing with non-static fields in static methods or classes.
Cs0120: An Object Reference Is Required For The Non-Static Field, Method, Or Property… Easy Fix
Can Object Reference Is Required For A Non Static Field?
When working with object-oriented programming languages such as Java, C++, or Python, we often come across the terms “object reference” and “non-static field.” Understanding the relationship between these two concepts is crucial, as it directly impacts the behavior and functionality of our code.
In order to comprehend whether an object reference is required for a non-static field, we first need to comprehend what these terms mean individually.
What is an Object Reference?
An object reference is essentially a variable that holds the memory address of an object instance. In simpler terms, it acts like a pointer to a specific location in memory where an object is stored. By using this reference, we can access and manipulate the object’s properties and methods.
In programming languages, object references are extensively used to create and manage instances of objects dynamically. They enable us to allocate memory, initialize the object’s state, and perform various operations on it. Without an object reference, accessing or modifying the properties of an object would be virtually impossible.
What is a Non-Static Field?
A non-static or instance field, on the other hand, belongs to a specific instance of a class. It is a variable that holds unique data for each object created from that class. These fields are accessible only when an object reference is used to access them.
Unlike static fields, which are shared among all instances of a class, non-static fields have their own values specific to each individual instance. For example, consider a class representing a car. A non-static field in this context could be the car’s color, where each instance of the car class can have a different color value.
The Relationship Between Object References and Non-Static Fields
Now that we understand what an object reference and a non-static field are, let’s explore their relationship. When we declare a non-static field within a class, it means that each instance of that class will have a separate copy of that field. Therefore, to access or modify the value of a non-static field, we need to have an object reference pointing to a specific instance of that class.
In other words, an object reference is required to identify the object to which the non-static field belongs. Without such a reference, it wouldn’t be possible to determine which instance of the class’s non-static field we want to access or modify.
Frequently Asked Questions
Q: Can a non-static field be accessed without an object reference?
A: No, it is not possible to access a non-static field without an object reference. Since non-static fields belong to individual instances of a class, they require an object reference to identify which instance’s field we want to access or modify.
Q: What happens if we try to access a non-static field without an object reference?
A: If we attempt to access a non-static field without an object reference, the compiler will throw an error. This error message serves as a reminder that object references are necessary to access or modify non-static fields.
Q: What is the difference between static and non-static fields?
A: Static fields are shared among all instances of a class and can be accessed without an object reference. Non-static fields, however, belong to individual instances of the class and require an object reference to access or modify them. Static fields are commonly used for constants or properties that should be shared across all instances, whereas non-static fields are used for individual instance-specific data.
Q: Can non-static fields be accessed from static methods?
A: No, non-static fields cannot be accessed directly from static methods as static methods are not associated with a specific instance of a class. To access non-static fields within a static method, an object reference or an instance of the class must be passed as a parameter.
In conclusion, when working with non-static fields in object-oriented programming languages, an object reference is required to access or modify the values of these fields. Non-static fields belong to individual instances of a class, and the object reference helps identify the specific instance whose field we want to interact with. Understanding this fundamental relationship between object references and non-static fields is essential for writing efficient and error-free code.
How To Use Non Static Field In Static Method Java?
Java is an object-oriented programming language known for its great flexibility and powerful features. However, there are certain restrictions imposed on accessing non-static fields from within static methods. In this article, we will explore different approaches to overcome this limitation and learn how to use non-static fields in static methods effectively.
Understanding Static Methods and Non-Static Fields in Java
Before diving into the topic at hand, it is crucial to grasp the concepts of static methods and non-static fields in Java.
A static method belongs to a class rather than to the instances (objects) of that class. It can be accessed without creating an instance of the class. On the other hand, non-static fields (also called instance variables) are specific to each instance of a class. They can only be accessed within non-static methods or by creating an object instance.
The Problem with accessing non-static fields in static methods
As stated earlier, static methods cannot access non-static fields directly. When we try to do so, we encounter a compilation error. This limitation exists because static methods are not bound to any specific instance and can be invoked without creating an object of the class. Therefore, it would be ambiguous to refer to a non-static field that belongs to a specific instance when a static method is invoked.
Approaches to use Non-Static Fields in Static Methods
Despite the restriction placed on accessing non-static fields from static methods, there are several approaches to work around this limitation:
1. Pass an instance as a method parameter
In this approach, we pass an instance of the class that contains the non-static field as a parameter to the static method. By doing so, we can access the non-static fields through the provided instance. Let’s take a look at an example:
“`java
public class MyClass {
private int nonStaticField;
public static void staticMethod(MyClass instance) {
int value = instance.nonStaticField;
// perform operations on value
}
}
“`
2. Convert non-static fields to static fields
Sometimes, it is possible to modify the non-static fields into static fields. This approach is suitable when the field’s value is universal across instances or does not require specific instance-related information. However, this approach should be used with caution, as excessive usage of static fields may result in unexpected behavior and memory-related issues.
3. Initialize a local variable with the non-static field’s value
If the non-static field has a constant or unchanging value across instances, it can be stored in a local variable inside the static method. This way, we can use the local variable throughout the method without accessing the non-static field directly. Here’s an example:
“`java
public class MyClass {
private int nonStaticField;
public static void staticMethod() {
int value = MyClass.nonStaticField;
// perform operations on value
}
}
“`
FAQs
Q: Why can’t static methods access non-static fields directly?
A: Static methods are not associated with any specific instance of a class and can be accessed without creating an object. Therefore, it would be ambiguous to refer to non-static fields that belong to a specific instance.
Q: Can I change a non-static field to a static field?
A: Yes, you can convert a non-static field to a static field if its value is the same across instances or does not require instance-specific information. However, excessive usage of static fields should be avoided to prevent unexpected behavior and memory issues.
Q: How do I access non-static fields from a static method?
A: There are several approaches to access non-static fields from static methods in Java. These include passing an instance as a method parameter, converting non-static fields to static fields, and initializing a local variable with the non-static field’s value.
Q: What are the limitations of using non-static fields in static methods?
A: One limitation is the inability to access non-static fields directly due to the lack of a specific instance associated with static methods. Additionally, accessing non-static fields excessively through static methods may result in poorer code structure and potentially introduce bugs.
Q: When should I use non-static fields in static methods?
A: Non-static fields should be used in static methods when absolutely necessary, such as when the field’s value needs to be accessed or modified and cannot be made static. However, it is always recommended to minimize such usage to maintain code integrity and readability.
In conclusion, while accessing non-static fields from static methods in Java poses a challenge, there are multiple approaches to overcome this limitation. By applying appropriate techniques like passing instances as parameters or converting non-static fields to static fields, programmers can effectively utilize non-static fields within static methods. However, it is important to exercise caution and adhere to best practices in order to maintain code readability and avoid potential issues.
Keywords searched by users: an object reference is required for the non static field lỗi an object reference is required for the non-static field method or property, An object reference is required for the non static field method or property Unity, An object reference is required for the non static field method or property in asp net, Object reference not set to an instance of an object, Object reference not set to an instance of an object C#, A field initializer cannot reference the non-static field, method, or property, Not set to an instance of an object unity, Object reference not set to an instance of an object unity
Categories: Top 50 An Object Reference Is Required For The Non Static Field
See more here: nhanvietluanvan.com
Lỗi An Object Reference Is Required For The Non-Static Field Method Or Property
When working with object-oriented programming languages such as C#, Java, or Python, you may come across an error message saying “An object reference is required for the non-static field, method, or property.” This error is quite common among developers, especially beginners, and can be frustrating to troubleshoot. In this article, we will dive into the in-depth explanation of this error, its causes, and possible solutions.
Understanding the Error:
To grasp the meaning behind this error, it is crucial to understand the difference between static and non-static members within a class. In simple terms, a static member is shared among all instances (objects) of the class, while a non-static member is unique to each instance.
The error message “An object reference is required for the non-static field, method, or property” usually occurs when you try to access a non-static member from a static context, where no instance of the class has been created. Since static members are not tied to any specific object, they do not require an object reference to access them. However, non-static members can only be accessed using an object reference.
Common Causes of the Error:
1. Attempting to access a non-static member within a static method:
This is perhaps the most common cause of the error. When trying to access a non-static member, such as a method or property, from within a static method, the compiler generates an error. Since static methods are not associated with any particular instance, they cannot directly access non-static members.
2. Accessing a non-static member without instantiating the class:
If you try to access a non-static member without creating an instance of the class, the error will occur. Non-static members can only be accessed through an object reference, which can only be obtained by instantiating the class.
3. Misusing static and non-static members interchangeably:
If you mistakenly use static members as if they were non-static or vice versa, this error may arise. It is crucial to understand the distinction between static and non-static members and use them according to their designated purposes.
Solutions and Workarounds:
1. Instantiate the class:
If you are trying to access a non-static member, make sure you have instantiated the class first. By creating an instance of the class, you will have an object reference that can be used to access non-static members.
2. Declare the non-static member as static:
If the functionality of the non-static member does not rely on any instance-specific data, you can consider declaring it as static. This way, the member will be accessible without requiring an object reference. However, be cautious when making this change, as it may affect the overall behavior of your program.
3. Create a new instance of the class within the static method:
If you need to access a non-static member from within a static method, you can create a new instance of the class within that method. This will provide you with the required object reference. However, keep in mind that this approach should only be used when it aligns with the program’s logic and requirements.
FAQs:
Q1. Can I access static members from non-static methods?
Yes, you can access static members from non-static methods without any issues. Since static members are not tied to any particular instance, they can be accessed from both static and non-static contexts.
Q2. Why should I be cautious when converting a non-static member to static?
When converting a non-static member to static, its behavior changes, as it is no longer tied to any instance-specific data. This may lead to unforeseen consequences if the member was designed with instance-specific data in mind. Therefore, it’s important to carefully analyze the implications of such a change in order to maintain the intended functionality of your code.
Q3. Why can’t I directly access non-static members from static methods?
Static methods are not associated with any particular instance of a class and exist independently. Non-static (instance) members, however, require an object reference to be accessed. Since there is no object reference available within static methods, directly accessing non-static members is not permitted.
In conclusion, the “An object reference is required for the non-static field, method, or property” error can be resolved by understanding the fundamental difference between static and non-static members and their usage. By following the recommended solutions and best practices, developers can overcome this error and achieve smooth execution of their programs.
An Object Reference Is Required For The Non Static Field Method Or Property Unity
When encountering this error, it typically means that you are trying to access a non-static field, method, or property without an object reference. Let’s dive deeper into what this error means and explore potential solutions and best practices to avoid it.
Understanding the Error:
In object-oriented programming, object references are crucial for interacting with variables, methods, and properties defined within a class. Each instance of a class holds its own unique set of data, referred to as instance variables. These variables can be accessed and modified only through an object reference. Additionally, instance methods and properties are designed to operate on data specific to the object they belong to.
On the other hand, static fields, methods, or properties are shared among all instances of a class. They don’t require an object reference and can be accessed directly through the class.
When encountering the “An object reference is required…” error, it typically means you are trying to access a non-static element without providing an object reference. This can happen when trying to access an instance method, field, or property without an instantiated object or when mistakenly attempting to access it through the class.
Common Scenarios and Solutions:
1. Accessing an instance method without an object reference:
If you have defined an instance method within a class and you are attempting to access it directly without an object reference, this error will occur. To fix it, ensure that you have instantiated the class and are calling the method using the object reference.
2. Accessing a non-static field without an object reference:
Similar to instance methods, non-static fields cannot be accessed directly without an object reference. To resolve this issue, instantiate the class and access the field through the object reference.
3. Accessing a non-static property without an object reference:
Non-static properties, just like fields and methods, require an object reference for access. Make sure you have instantiated the object and then access the property using the object reference.
4. Accidentally accessing a non-static element through the class:
Sometimes, developers mistakenly try to access instance fields, methods, or properties through the class itself, without providing an object reference. This, however, is only possible with static elements. To avoid this error, check whether the element you are accessing is static or non-static and make sure you are using the appropriate syntax.
Best Practices to Avoid the Error:
1. Properly instantiate objects:
Always ensure that you have instantiated the object before accessing its non-static fields, methods, or properties. Instantiate the object using “new” keyword or through Unity methods like “Instantiate”.
2. Double-check references:
When passing objects between scripts or game objects in Unity, ensure that your references are properly set. Incorrectly referencing a script or game object can lead to the “object reference required” error.
3. Use serialized references in Unity Inspector:
Unity’s Inspector window allows you to assign object references directly. Utilize this feature by creating serialized fields and assigning the required object references in the Inspector, eliminating the need for direct object references in the code.
4. Understand the difference between static and non-static elements:
Familiarize yourself with the concept of static and non-static elements in object-oriented programming. Understanding the distinction will help you avoid mistakenly accessing non-static elements without object references.
FAQs:
Q: What does “An object reference is required for the non-static field, method, or property” error mean?
A: This error implies that you are trying to access a non-static field, method, or property without an object reference.
Q: How can I fix this error in Unity?
A: Ensure that you have instantiated the required object and use the appropriate object reference to access non-static fields, methods, or properties.
Q: What are some common scenarios that trigger this error?
A: Trying to access instance methods, fields, or properties without an instantiated object or mistakenly trying to access them through the class itself rather than an object reference can lead to this error.
Q: How can I prevent encountering this error in the future?
A: Follow best practices such as properly instantiating objects, using serialized references in the Unity Inspector, and understanding the distinction between static and non-static elements.
In conclusion, encountering the “An object reference is required for the non-static field, method, or property” error is quite common in Unity development. This error typically arises when attempting to access non-static elements without providing an object reference. Understanding the causes and implementing best practices will help you efficiently resolve this error and prevent it from occurring in the future.
An Object Reference Is Required For The Non Static Field Method Or Property In Asp Net
When working with ASP.NET, it is crucial to understand the difference between static and non-static members. Static members belong to a class, whereas non-static members belong to an instance of the class. Non-static members cannot be accessed directly using the class name; instead, they require an instance of the class to be accessed.
Causes of the “An object reference is required for the non-static field, method, or property” error
1. Accessing non-static members without creating an instance: This error mainly occurs when we try to access a non-static member directly through the class name, without creating an instance of the class. For example:
“`csharp
public class MyClass
{
public int MyProperty { get; set; }
public void MyMethod()
{
// Do something
}
}
// Accessing without instance – results in error
MyClass.MyProperty = 10;
MyClass.MyMethod();
“`
To resolve this issue, we should create an instance of the class and then access the non-static members:
“`csharp
MyClass myObject = new MyClass();
myObject.MyProperty = 10;
myObject.MyMethod();
“`
2. Inconsistent usage of static and non-static members: Another reason for encountering this error is when there is a mix of static and non-static members being accessed inappropriately. If we have a combination of static and non-static methods or properties within a class, it is important to use them correctly.
“`csharp
public class MyClass
{
public static int MyStaticProperty { get; set; } // Static property
public int MyNonStaticProperty { get; set; } // Non-static property
public static void MyStaticMethod()
{
// Do something
}
public void MyNonStaticMethod()
{
// Do something
}
}
// Accessing static members
MyClass.MyStaticProperty = 10;
MyClass.MyStaticMethod();
// Accessing non-static members incorrectly – results in error
MyClass.MyNonStaticProperty = 10;
MyClass.MyNonStaticMethod();
“`
To resolve this issue, ensure that static members are accessed using the class name, while non-static members are accessed through an instance of the class.
“`csharp
// Accessing static members
MyClass.MyStaticProperty = 10;
MyClass.MyStaticMethod();
// Accessing non-static members correctly
MyClass myObject = new MyClass();
myObject.MyNonStaticProperty = 10;
myObject.MyNonStaticMethod();
“`
Implications of the “An object reference is required for the non-static field, method, or property” error:
This error can lead to unexpected behavior or application failures if not handled properly. It indicates a logical flaw in the code, where non-static members are being accessed incorrectly. Ignoring or suppressing this error might result in data inconsistency, incorrect outputs, or even application crashes.
Frequently Asked Questions (FAQs):
Q1. What is the difference between static and non-static members in ASP.NET?
A1. Static members belong to a class and can be accessed using the class name directly. Non-static members, on the other hand, belong to an instance of the class and require an object reference to access them.
Q2. How can I resolve the “An object reference is required for the non-static field, method, or property” error?
A2. To resolve this error, create an instance of the class and then access the non-static members through that instance. Ensure correct usage of static and non-static members within the class.
Q3. Can I convert a non-static member to a static member to avoid this error?
A3. Converting a non-static member to a static member can resolve the error, but it completely changes the essence and behavior of the code. It should only be done if it aligns with the design and requirements of the application.
Q4. Are there any tools or IDE features to detect such errors?
A4. Modern Integrated Development Environments (IDEs) often provide static code analysis tools that can help detect and highlight such errors at build time. Utilizing these tools can assist in identifying and resolving the error.
In conclusion, the “An object reference is required for the non-static field, method, or property” error is commonly encountered in ASP.NET development when non-static members are accessed incorrectly. It occurs when non-static members are accessed without creating an instance of the class. It is important to ensure correct usage of static and non-static members and to access non-static members through an instance of the class. Resolving this error effectively will result in bug-free and reliable ASP.NET applications.
Images related to the topic an object reference is required for the non static field
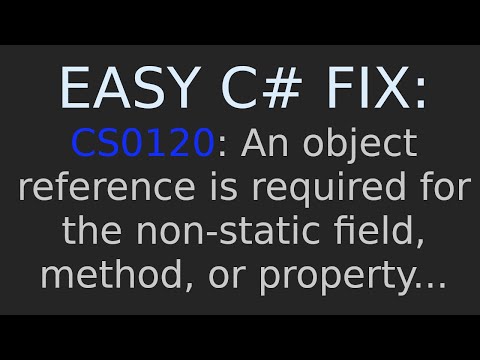
Found 10 images related to an object reference is required for the non static field theme
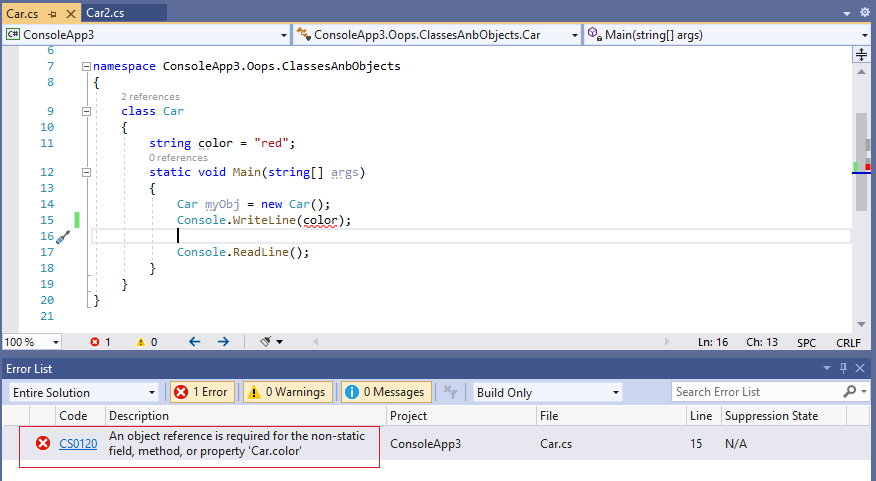
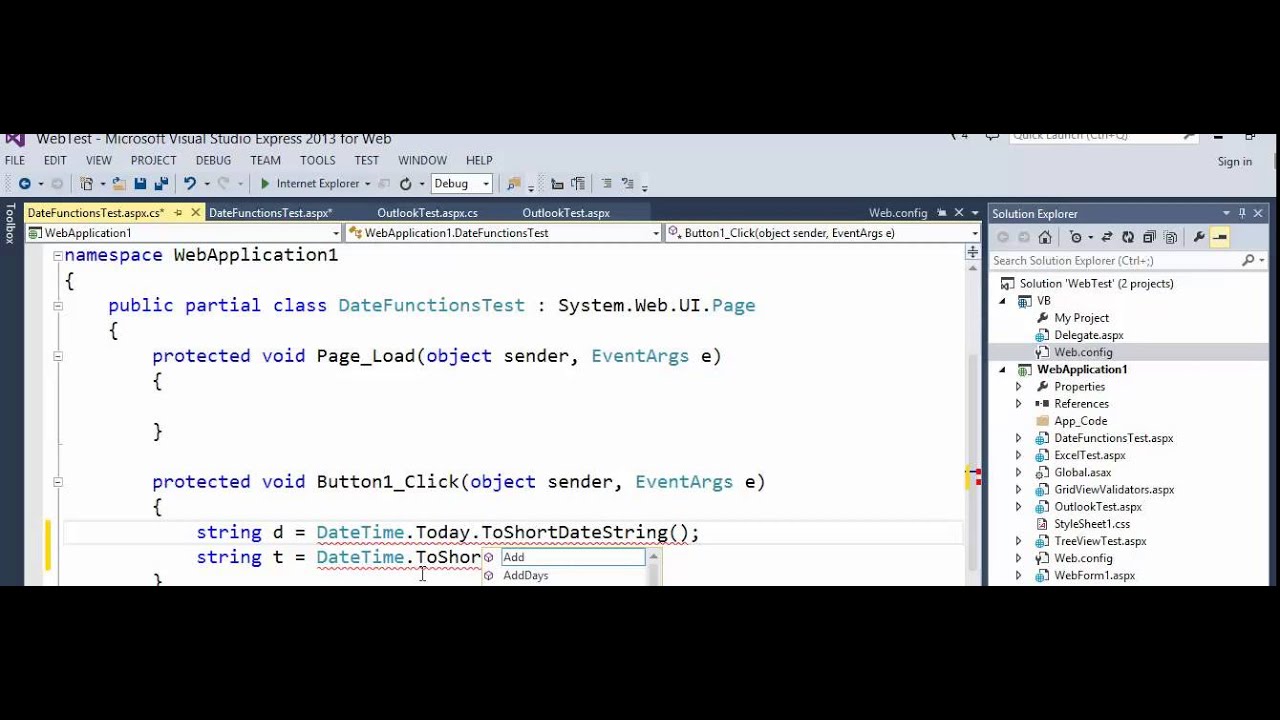





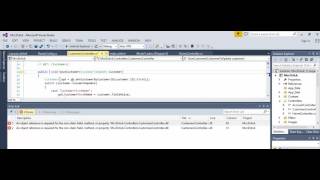
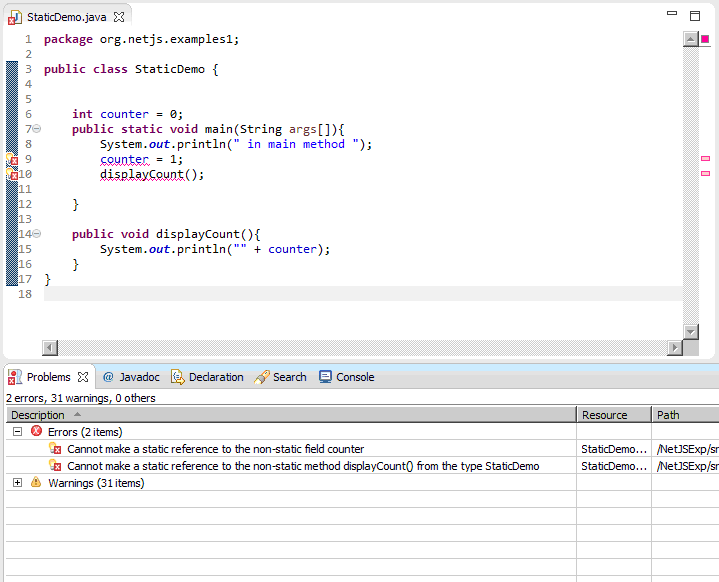






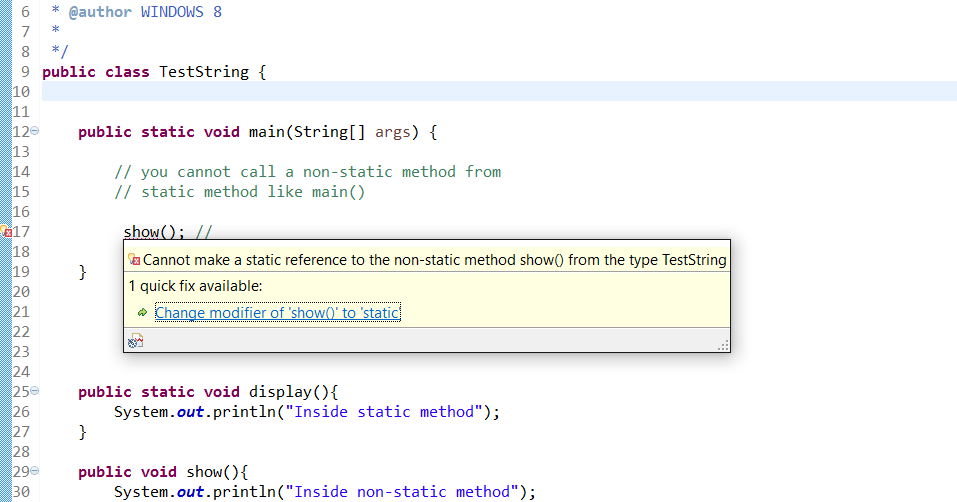
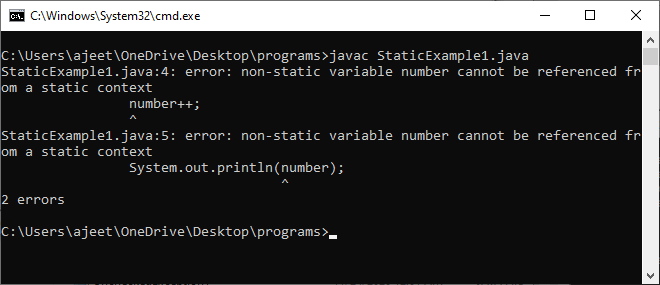
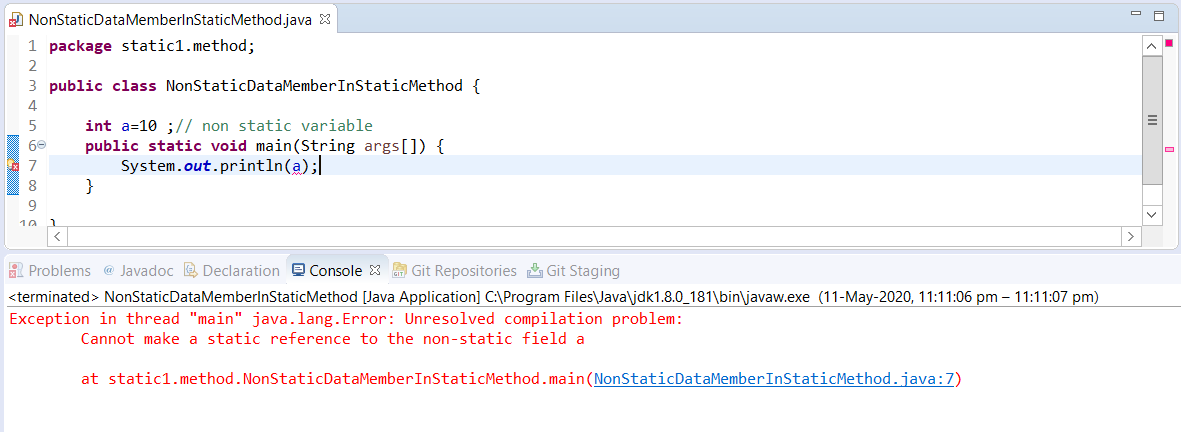




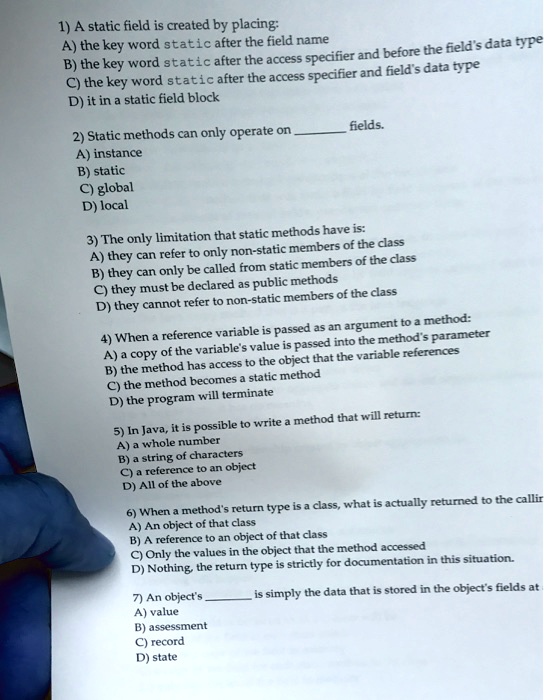


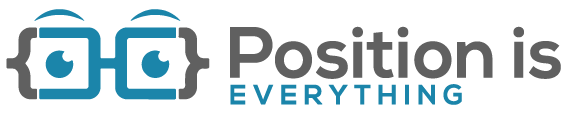
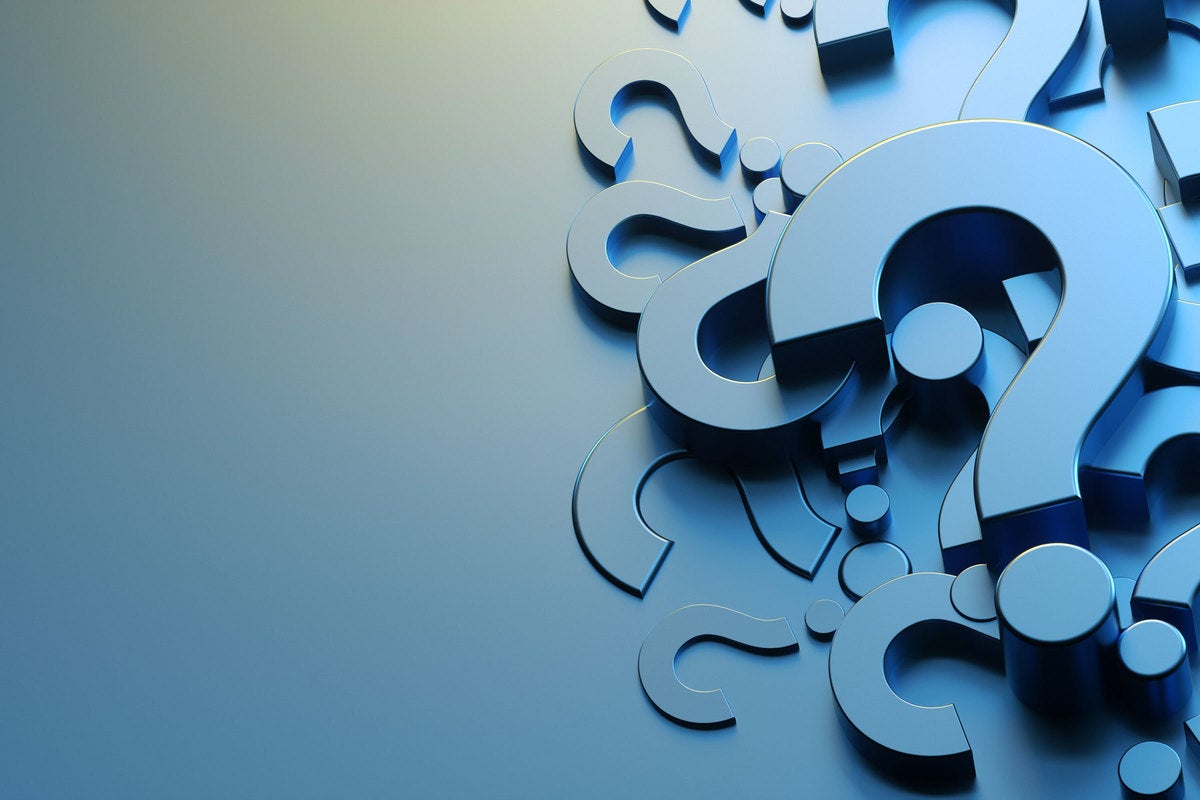
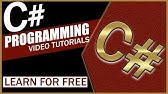
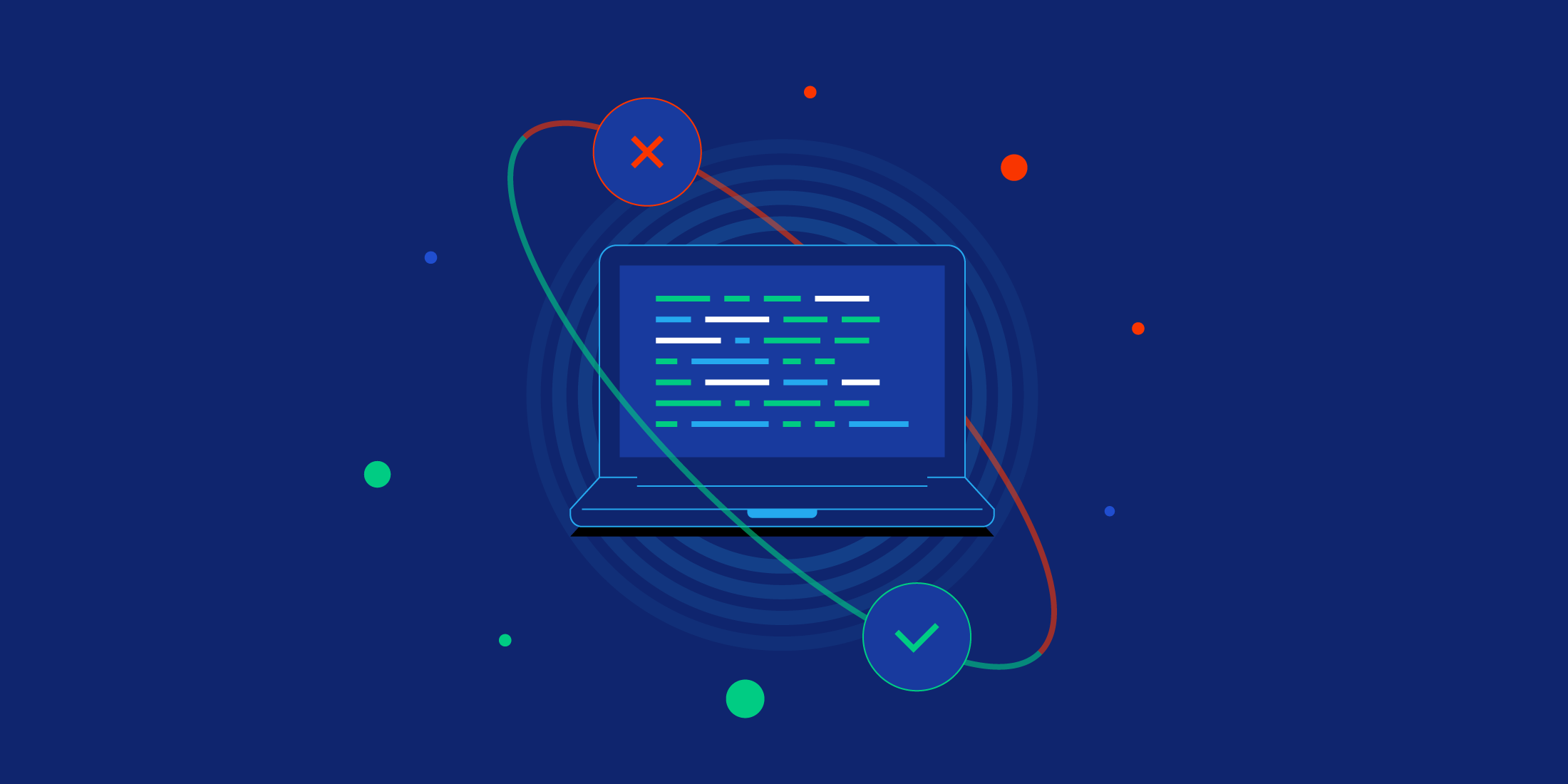
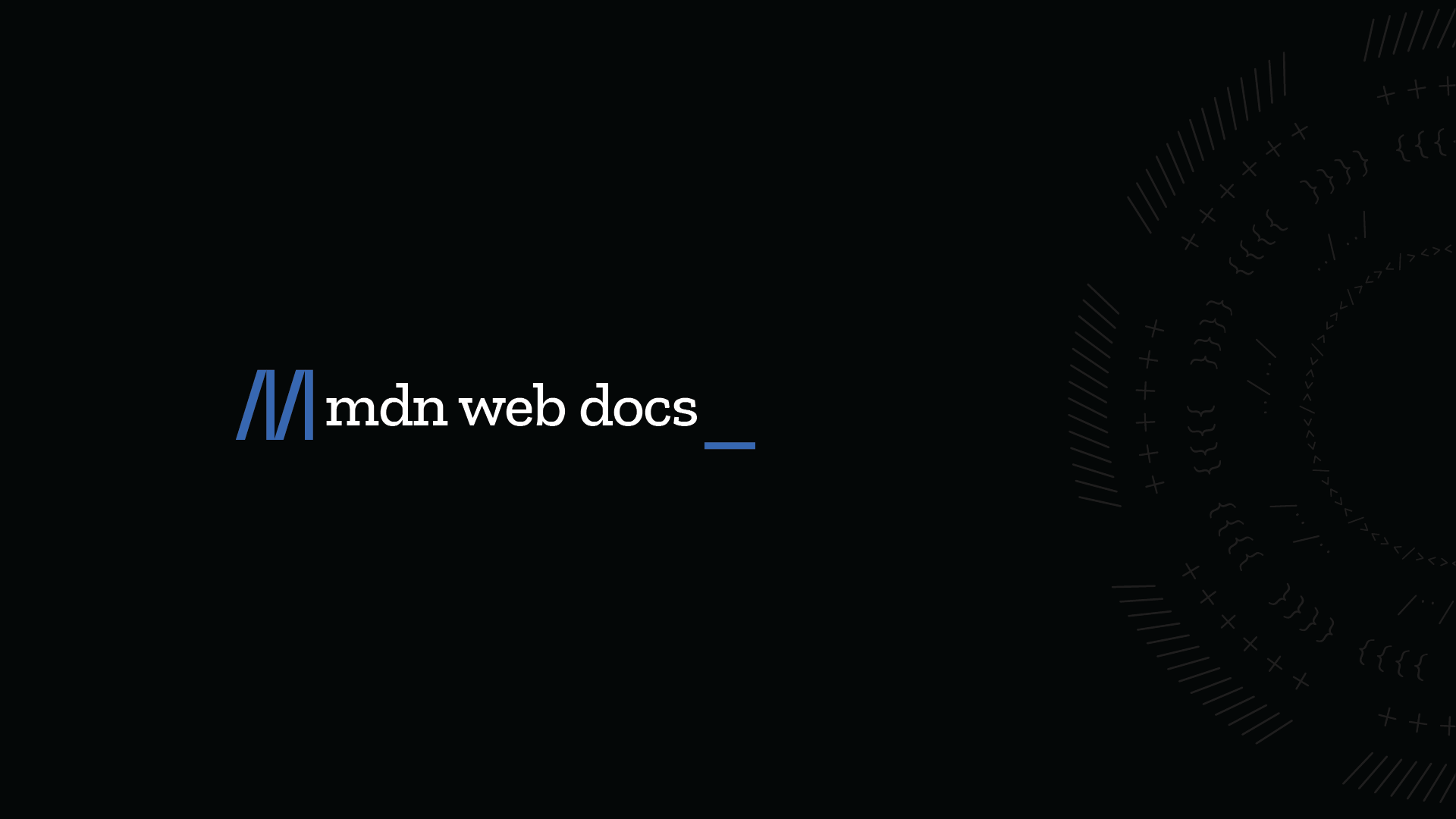
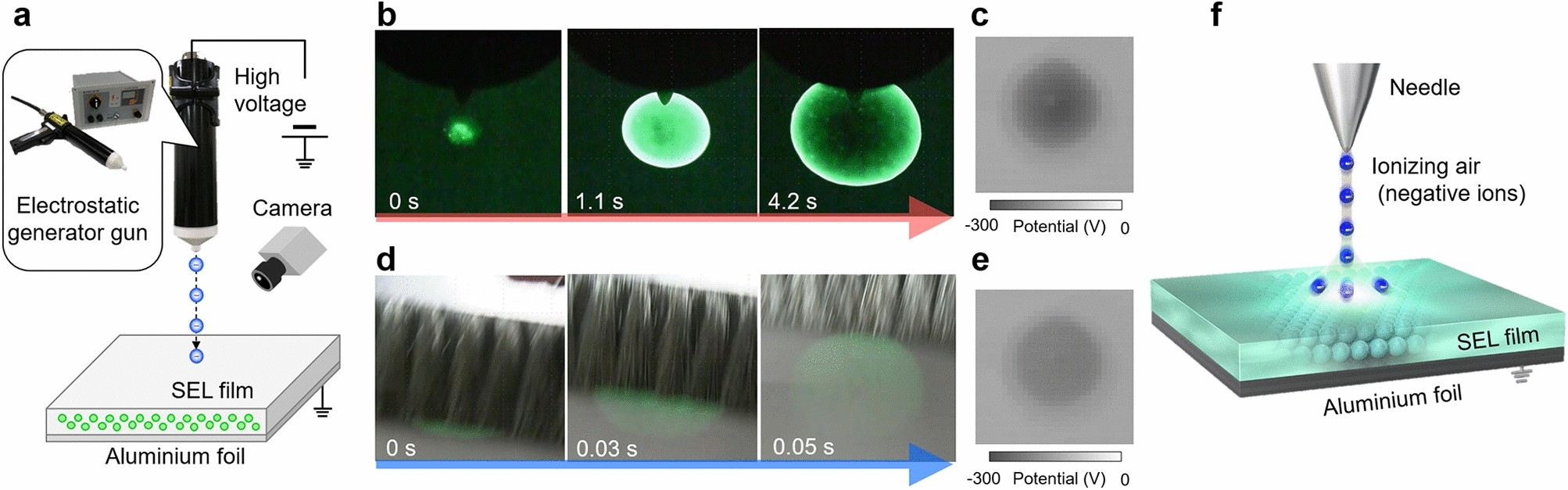


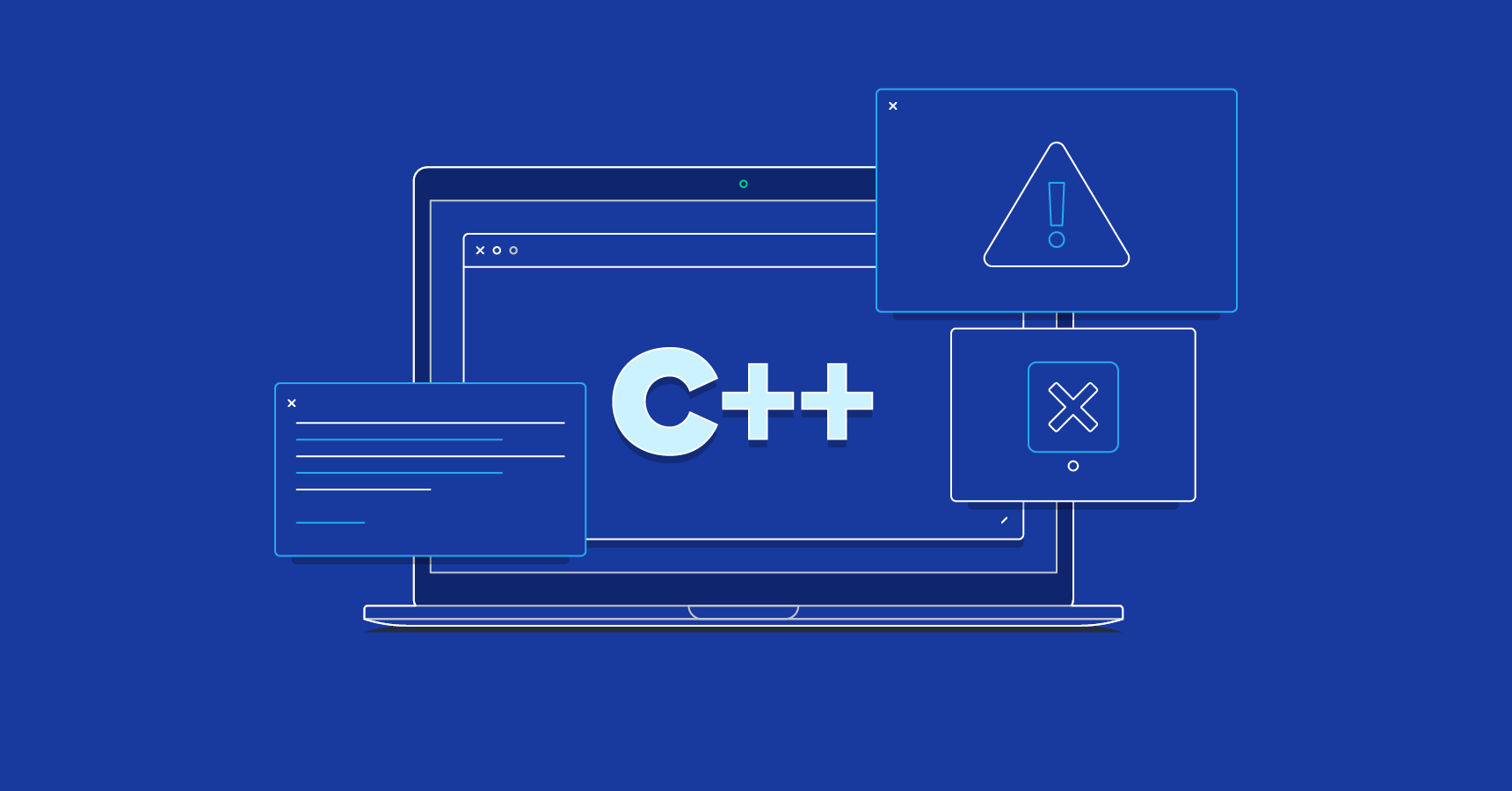
Article link: an object reference is required for the non static field.
Learn more about the topic an object reference is required for the non static field.
- An object reference is required for the nonstatic field, method …
- C# Error: How to Resolve an object reference is required for …
- C# Error: How to Resolve an object reference is required for …
- Static Variable in Java with Examples – Scaler Topics
- Static vs Non-Static Members in C# with Examples – Dot Net Tutorials
- Difference between Static and Non-Static fields of a class.
- An Object Reference Is Required for the Non-static Field: Fix It …
- Compiler Error CS0120 | Microsoft Learn
- error An object reference is required for the non-static field …
- Understanding The Importance Of Object Reference For Non …
- An object reference is required for the non-static … – Unity Forum
- Error CS0120 An object reference is required for the non-static …