Add Minutes To Current Time Javascript
The JavaScript Date object is used to work with dates and times in JavaScript. It provides various methods to manipulate and extract information from dates. One common scenario is to add minutes to the current time using JavaScript.
Getting the Current Time in JavaScript
Before adding minutes to the current time, we need to obtain the current time using JavaScript. This can be achieved by creating a new instance of the Date object and calling the `getTime()` method on it. The `getTime()` method returns the number of milliseconds since January 1, 1970, which represents the current time.
Adding Minutes to the Current Time
To add minutes to the current time, we need to convert the number of minutes to milliseconds and then add it to the current time. JavaScript provides the `setTime()` method to set the time of a Date object based on the number of milliseconds since January 1, 1970. By using this method, we can add minutes to the current time.
Handling Edge Cases and Validations
When adding minutes to the current time, we should consider edge cases and perform validations to ensure the correct output. For example, we need to make sure that the added minutes do not cause the time to go beyond 23:59, as it would result in an invalid time. We can handle this by checking if the added minutes exceed 60 and adjust the hours accordingly.
Displaying the Updated Time to the User
Once we have the updated time, we can display it to the user in a readable format. We can use JavaScript techniques like concatenation or template literals to format the time string and present it to the user.
Implementing the Solution: Writing the JavaScript Code
Now let’s dive into the actual JavaScript code to add minutes to the current time. Here’s an example implementation:
“`
// Get the current time
const currentTime = new Date();
const currentMilliseconds = currentTime.getTime();
// Add minutes to the current time
const minutesToAdd = 2;
const millisecondsToAdd = minutesToAdd * 60 * 1000; // Convert minutes to milliseconds
const updatedMilliseconds = currentMilliseconds + millisecondsToAdd;
// Set the updated time
const updatedTime = new Date(updatedMilliseconds);
// Display the updated time
console.log(updatedTime);
“`
In this code, we first get the current time using the `getTime()` method. Then, we calculate the milliseconds to add based on the number of minutes provided by the user. After that, we add the milliseconds to the current time using the `setTime()` method. Finally, we create a new Date object from the updated milliseconds and display it using `console.log()`.
Testing and Troubleshooting
It’s important to test the code thoroughly to ensure its correctness and handle any potential issues. We can check if the output matches our expectations by manually verifying the displayed time. Additionally, we can perform further tests by adding different numbers of minutes and checking if the results are accurate.
FAQs
1. How do I add 2 minutes to the current time in JavaScript?
To add 2 minutes to the current time in JavaScript, you can follow the code example provided above. Simply set the `minutesToAdd` variable to 2 and run the code.
2. How do I add minutes to a specific date in JavaScript?
To add minutes to a specific date in JavaScript, you can create a new Date object with the desired date and then follow the same steps as adding minutes to the current time.
3. How can I add minutes to the current time using jQuery?
The jQuery library does not provide specific methods for manipulating dates and times like JavaScript’s Date object. However, you can still use jQuery to select HTML elements and display the updated time after adding minutes using JavaScript.
4. Can the Moment.js library be used to add minutes to the current time?
Yes, the Moment.js library provides powerful date and time manipulation capabilities. You can use its `add()` method to add minutes to the current time.
5. How do I add seconds to a date in JavaScript?
To add seconds to a date in JavaScript, you can follow similar steps as adding minutes. Instead of multiplying the minutes by 60, multiply the seconds by 1000 to convert them to milliseconds.
6. How do I convert time to a different format in JavaScript?
To convert time to a different format in JavaScript, you can use JavaScript’s `getHours()`, `getMinutes()`, and `getSeconds()` methods to extract the individual components of the time and format them as needed using string manipulation techniques.
7. How can I subtract date and time in JavaScript?
To subtract date and time in JavaScript, you can obtain the milliseconds of the two dates using `getTime()` and subtract them. Then, create a new Date object using the resulting milliseconds to get the difference.
In conclusion, adding minutes to the current time in JavaScript involves obtaining the current time, adding the desired number of minutes in milliseconds, setting the updated time, and displaying it to the user. It is important to handle edge cases and perform validations to ensure accurate results. By following the code examples and guidelines provided, you can easily add minutes to the current time in JavaScript and manipulate dates and times effectively.
How To Display Time On Website Using Html Css And Javascript | Current Time On Html Website
How To Add Minutes In Current Time In Javascript?
JavaScript is a powerful and widely used programming language that allows developers to build dynamic and interactive web applications. One common task developers face is manipulating dates and times. In this article, we will explore how to add minutes to the current time in JavaScript.
Working with Dates and Times in JavaScript
Before we dive into the details of adding minutes to the current time, let’s briefly discuss how JavaScript handles dates and times. JavaScript provides the Date object, which allows us to work with dates and times in various ways.
To create a new Date object that represents the current time, we can simply call the Date constructor without any arguments, as shown below:
“`javascript
const now = new Date();
“`
This will create a new Date object with the current date and time. We can then perform various operations on this object, such as getting or setting specific parts of the date and time.
Adding Minutes to the Current Time
To add minutes to the current time, we can make use of the `setMinutes` method provided by the Date object. This method allows us to set the minutes of a given date object.
Here’s an example of how we can add 10 minutes to the current time:
“`javascript
const now = new Date();
now.setMinutes(now.getMinutes() + 10);
“`
In the code above, we first create a new Date object representing the current time. Then, we call the `getMinutes` method on this object to retrieve the current minutes. We add 10 to the current minutes and use the `setMinutes` method to update the minutes of the date object.
By doing this, we have successfully added 10 minutes to the current time.
Handling Edge Cases
When adding minutes to a time, we need to consider edge cases such as exceeding 60 minutes or crossing into the next hour. JavaScript’s Date object takes care of these cases automatically.
For example, if the current time is 9:55 AM and we add 10 minutes, the resulting time will be correctly calculated as 10:05 AM. Similarly, if the current time is 11:50 PM and we add 20 minutes, the resulting time will be calculated as 12:10 AM (the following day).
Frequently Asked Questions (FAQs)
Q: Can I add negative minutes to go back in time?
A: Yes, you can add negative minutes to subtract time from the current time. For example, if you want to go back 5 minutes from the current time, you can do: `now.setMinutes(now.getMinutes() – 5);`.
Q: How can I display the updated time?
A: To display the updated time, you can use the various methods provided by the Date object, such as `getHours`, `getMinutes`, and `toLocaleString`. For example, to display the updated time in the format HH:MM, you can use: `console.log(now.getHours() + “:” + now.getMinutes());`.
Q: Can I add minutes to a specific date and time, rather than the current time?
A: Yes, you can add minutes to any specific date and time by creating a new Date object with the desired date and time, and then using the `setMinutes` method as shown previously.
Q: Is it possible to add hours or seconds instead of minutes?
A: Yes, in addition to `setMinutes`, the Date object provides methods like `setHours` and `setSeconds` to manipulate hours and seconds, respectively. You can use these methods to add hours or seconds to the current time.
Q: Do I need to worry about cross-browser compatibility?
A: For the most part, JavaScript’s Date object and its methods are well-supported across all modern browsers. However, it is always a good practice to test your code in different browsers to ensure compatibility.
Conclusion
In this comprehensive article, we explored how to add minutes to the current time in JavaScript. We learned about the Date object and its various methods, focusing on `setMinutes` to manipulate the minutes of a given date object. We also discussed handling edge cases and provided answers to common questions regarding adding minutes to the current time in JavaScript. With this knowledge, you can now confidently perform time calculations in your JavaScript applications.
How To Add 1 Hour To Current Time In Javascript?
JavaScript, being a versatile and powerful scripting language, allows developers to manipulate time effortlessly. Adding or subtracting time from the current time can be quite useful in various applications such as scheduling tasks, calculating durations, or implementing countdown timers. In this article, we will explore different approaches to add 1 hour to the current time in JavaScript and discuss some frequently asked questions.
Method 1: Using the Date Object
One of the easiest and most common ways to add time in JavaScript is by using the built-in Date object. The following code snippet demonstrates how to add 1 hour to the current time:
“`javascript
const currentDateTime = new Date();
currentDateTime.setHours(currentDateTime.getHours() + 1);
console.log(currentDateTime);
“`
In this method, we first create a new Date object representing the current date and time. Then, we use the `setHours()` method to modify the hours component of the datetime object by adding 1 to the existing value. The resulting updated datetime object is then displayed in the console.
Method 2: Using the UTC Methods
Another approach is to use the UTC methods provided by the Date object. These methods handle time in a consistent manner across different time zones. Here’s an example of how to add 1 hour to the current time using the UTC methods:
“`javascript
const currentDateTime = new Date();
currentDateTime.setUTCHours(currentDateTime.getUTCHours() + 1);
console.log(currentDateTime);
“`
Here, we utilize the `setUTCHours()` method instead of `setHours()` to modify the UTC hours component of the datetime object. This ensures that the time manipulation is performed based on the UTC time zone, which can be helpful when dealing with international applications.
Method 3: Using the Timestamp
Alternatively, we can add 1 hour to the current time by converting it into a timestamp, performing the addition, and then converting it back to a readable format. Here’s an example implementation:
“`javascript
const currentDateTime = new Date();
const timestamp = currentDateTime.getTime();
const updatedTimestamp = timestamp + 1 * 60 * 60 * 1000; // 1 hour in milliseconds
const updatedDateTime = new Date(updatedTimestamp);
console.log(updatedDateTime);
“`
In this method, we obtain the current time as a timestamp using the `getTime()` method. Then, we add the desired time increment in milliseconds (1 hour is equal to 60 minutes * 60 seconds * 1000 milliseconds) to the timestamp. Finally, we create a new Date object using the updated timestamp, resulting in the datetime with the added hour.
FAQs
Q1: Can I add a different time increment, such as minutes or seconds, using these methods?
Yes, you can easily modify the examples provided to add any desired time increment. The key is to convert the desired interval into milliseconds. For example, to add 30 minutes, multiply `30 * 60 * 1000`, where 60 represents minutes and 1000 represents milliseconds.
Q2: How can I display the updated time in a specific format?
JavaScript provides several methods to format date and time according to different requirements. The `toLocaleString()` method is particularly useful for obtaining a localized string representation of the datetime. You can customize the format further by using methods such as `toLocaleTimeString()` and `toLocaleDateString()`.
Q3: How can I handle time across different time zones?
By default, the Date object uses the local time zone of the user’s browser. To handle time in specific time zones or to convert between different time zones, you can use external libraries like Moment.js or JavaScript’s built-in `toLocaleString()` method, which accepts locale-specific options as arguments.
In conclusion, JavaScript offers multiple approaches to add 1 hour to the current time. Whether you choose to use the Date object’s built-in methods, UTC methods, or timestamps, you can easily manipulate time to fulfill your specific requirements. Remember to consider time zones and formatting options for handling time accurately and displaying it in a way that meets your application’s needs.
Keywords searched by users: add minutes to current time javascript Add 2 minutes to current time in javascript, Add minutes to Date JavaScript, Jquery add minutes to current time, Moment add minutes, Javascript add minutes to time string, Add second to date JavaScript, Convert time JavaScript, How to subtract date time in javascript
Categories: Top 95 Add Minutes To Current Time Javascript
See more here: nhanvietluanvan.com
Add 2 Minutes To Current Time In Javascript
JavaScript is a versatile programming language that allows developers to add interactivity to web pages. One common task that developers often face is manipulating dates and times. In this article, we will explore how to add 2 minutes to the current time using JavaScript.
Getting the current time in JavaScript
To add minutes to the current time, we first need to obtain the current time value. JavaScript provides the `Date` object for working with dates and times. By creating a new instance of the `Date` object without any arguments, we can get the current date and time.
“`javascript
let currentTime = new Date();
“`
This will create a `Date` object representing the current date and time. Once we have the current time, we can proceed to add minutes to it.
Adding 2 minutes to the current time
To add minutes to the current time, we can use the `setMinutes()` method available in the `Date` object. This method accepts two arguments: the minutes to be added and an optional second argument for specifying additional seconds.
“`javascript
currentTime.setMinutes(currentTime.getMinutes() + 2);
“`
The `getMinutes()` method is used to obtain the current value of minutes, and then we add 2 to it. The `setMinutes()` method updates the `Date` object with the new time value.
Converting the new time to a human-readable format
By default, the value returned by the `setMinutes()` method is in milliseconds since January 1, 1970. To display the updated time in a readable format, we can make use of various `Date` object methods such as `getHours()`, `getMinutes()`, and `getSeconds()`.
“`javascript
let newTime = currentTime.getHours() + ‘:’ + currentTime.getMinutes() + ‘:’ + currentTime.getSeconds();
“`
In this example, we extract the hour, minute, and second values from the `currentTime` object and concatenate them into a single string. The resulting `newTime` variable will contain the updated time in the format “hh:mm:ss”.
Implementing the code in a real-life scenario
Now that we know how to add 2 minutes to the current time using JavaScript, let’s imagine a scenario where we need to display the updated time on a web page. To achieve this, we can use the Document Object Model (DOM) to manipulate the HTML and display the updated time.
Here’s an example of how the JavaScript code can be integrated into an HTML page:
“`html
Updated Time:
“`
In this example, we have an empty `
` element with the id ‘updated-time’ to represent where the updated time will be displayed. The JavaScript code retrieves this element using the `getElementById()` method and updates its content with the new time using the `textContent` property.
FAQs:
Q: Can I add more than 2 minutes to the current time using this method?
A: Yes, you can easily modify the code to add any desired number of minutes. Simply change the value being added in the `setMinutes()` method to the desired number.
Q: Does this method take daylight savings time into account?
A: No, the code provided does not account for daylight savings time. If you need to consider daylight savings time, you may need to implement additional logic and utilize other JavaScript date and time functions.
Q: Can this method be used for subtracting minutes from the current time?
A: Absolutely! Instead of adding minutes, you can subtract minutes from the current time by changing the sign of the value being added in the `setMinutes()` method.
In conclusion, JavaScript provides an easy way to add minutes to the current time using the `Date` object. By applying the `setMinutes()` method and formatting the result, you can manipulate and display time-related information in a web application.
Add Minutes To Date Javascript
JavaScript offers powerful functions to manipulate dates and times, allowing developers to perform various operations with ease. One common requirement is to add or subtract minutes to a given date. In this article, we will explore different approaches for achieving this task in JavaScript, providing practical examples and covering potential pitfalls along the way.
### Getting Started
Before diving into the specifics, let’s first understand how JavaScript represents dates. In JavaScript, dates are represented using the `Date` object. The `Date` object allows you to work with dates, times, and perform various operations on them.
To create a new `Date` object, you can use the `new Date()` constructor. By default, it creates a `Date` object with the current date and time.
“`javascript
let currentDate = new Date();
“`
This will create a `Date` object representing the current date and time. Now, let’s explore different approaches to add minutes to this date.
### Approach 1: Using setTime()
The `Date` object provides a method called `setTime()`, which allows you to set the milliseconds since January 1, 1970. By utilizing this method, we can add minutes to a date by converting minutes to milliseconds and adding them to the current date.
“`javascript
let currentDate = new Date();
let minutesToAdd = 30;
currentDate.setTime(currentDate.getTime() + (minutesToAdd * 60000));
“`
In the above code snippet, we first create a new `Date` object representing the current date. We then calculate the milliseconds equivalent to the minutes we want to add (30 minutes in this case) by multiplying the minutes with 60000 (1 minute = 60,000 milliseconds). Finally, we pass this duration to the `setTime()` function, which adds the specified number of milliseconds to the current date.
### Approach 2: Using the built-in methods
Another approach to add minutes to a date in JavaScript is by utilizing the built-in methods provided by the `Date` object. These methods allow you to manipulate the individual components of a date, such as hours, minutes, seconds, etc.
“`javascript
let currentDate = new Date();
let minutesToAdd = 30;
currentDate.setMinutes(currentDate.getMinutes() + minutesToAdd);
“`
In this approach, we use the `setMinutes()` method to add minutes directly to the current date. The `getMinutes()` method retrieves the current minutes, and we add the desired minutes to it. The `setMinutes()` method updates the `Date` object with the new minutes value.
This approach is more straightforward and doesn’t involve any calculations with milliseconds. However, it is important to note that it only adds minutes and may not work as expected when dealing with hours or other time units.
### Pitfalls and Considerations
When adding minutes to a date, it’s important to consider the potential pitfalls and edge cases. Here are a few considerations to keep in mind:
1. **Overlapping Hours**: Adding a large number of minutes may result in overlapping hours. For example, adding 90 minutes to a date that is initially 23:30 will result in a date with a time of 01:00 on the next day. Be aware of these edge cases and handle them accordingly.
2. **Daylight Saving Time**: In regions that observe daylight saving time, adding minutes to a date can have unexpected results during the transitions. It is crucial to account for such time changes and ensure accurate calculations.
3. **Immutable Dates**: The `Date` object in JavaScript is mutable, meaning that modifying it directly can have unintended side effects. To avoid such issues, consider creating a new `Date` object rather than modifying an existing one.
4. **Handling Invalid Dates**: Adding minutes may occasionally result in an invalid date, for example, a date with February 30th. Make sure to handle such invalid cases and validate the resulting dates accordingly.
### FAQs
#### How can I subtract minutes from a date in JavaScript?
To subtract minutes from a date in JavaScript, you can use the same approaches mentioned above, but with a negative value for the minutes to subtract. For example, to subtract 10 minutes from a given date, you can use either `setTime()` or the built-in methods with `-10` for `minutesToAdd`.
#### How can I format the resulting date in a specific format?
JavaScript’s `Date` object provides various methods to extract individual components of a date, such as year, month, day, hours, minutes, etc. By utilizing these methods, you can format the resulting date in any desired format. Alternatively, you can explore external libraries like Moment.js, which provide more advanced date formatting capabilities.
#### Can I add minutes to a specific date and time, not the current date?
Yes, by modifying the initialization of the `Date` object, you can add minutes to a specific date and time. For example, to add 15 minutes to March 10, 2022, at 14:30, you can create a `Date` object with the desired date and time and then add the desired minutes using any of the mentioned approaches.
### Conclusion
In this article, we explored different ways to add minutes to a date in JavaScript. We discussed the use of `setTime()` to add minutes by converting them into milliseconds, as well as using the built-in methods to manipulate the date components directly. We also highlighted the potential pitfalls and considerations, such as overlapping hours and daylight saving time. Ultimately, understanding the various approaches will empower you to handle date manipulations effectively in JavaScript projects.
Jquery Add Minutes To Current Time
Adding minutes to the current time can be useful in various scenarios. For instance, you may want to display an updated time on a web page, schedule an event by incrementing the current time, or calculate a future timestamp for a specific task. Whatever the requirement, jQuery makes it easy to perform such time manipulations in a user-friendly manner.
To begin, let’s take a look at the basic structure of a jQuery script that adds minutes to the current time:
“`javascript
$(document).ready(function() {
var currentTime = new Date();
var minutesToAdd = 30; // Change this value to add different minutes
currentTime.setMinutes(currentTime.getMinutes() + minutesToAdd);
var formattedTime = currentTime.getHours() + ‘:’ + currentTime.getMinutes();
// Display the updated time
$(‘#time’).text(formattedTime);
});
“`
In the snippet above, we start by wrapping our code in the `$(document).ready()` function to ensure it executes when the DOM is ready. We then create a `Date` object, representing the current time, using `new Date()`. The `minutesToAdd` variable allows you to specify the number of minutes you want to add to the current time; you can modify this value as needed.
To perform the actual addition, we utilize the built-in `setMinutes()` function of our `Date` object. It allows us to add minutes to the current time by passing the sum of the current minutes (`currentTime.getMinutes()`) and the desired minutes to add (`minutesToAdd`).
Next, we format the updated time by extracting the hours (`currentTime.getHours()`) and minutes (`currentTime.getMinutes()`) from our `Date` object. Here, you can apply any desired formatting, such as adding leading zeroes or displaying in a 12-hour format rather than the default 24-hour format.
Finally, we display the updated time on the webpage by targeting an HTML element with an `id` attribute of `time` and changing its text using the `text()` function of jQuery.
With this basic structure in place, you can easily adapt the code to fit your specific requirements. For instance, you could integrate it with user inputs to allow for dynamic addition of minutes or apply further time manipulations such as subtracting hours or days.
Now, let’s address some common questions that arise when working with jQuery to add minutes to the current time:
—
### FAQs
**Q1. How can I add minutes to the current system time rather than the user’s local time?**
By default, the `Date` object in JavaScript returns the current local time, which is based on the user’s system time settings. If you want to add minutes to the current system time, you can use techniques like server-side scripting or AJAX to fetch the system time from the server and then perform the necessary calculations.
**Q2. Can I add minutes to a specific time rather than the current time?**
Yes, you can add minutes to any specific time by creating a `Date` object representing that time instead of using `new Date()` to get the current time. You can then follow the same procedure described earlier to add the desired minutes.
**Q3. How can I display the updated time continuously without refreshing the page?**
To display the updated time continuously without refreshing the entire page, you can use JavaScript’s `setInterval()` function. This function allows you to execute a piece of code at specific time intervals. By placing the time manipulation code inside the `setInterval()` function, you can continually update the time without any manual interaction.
**Q4. Are there any limitations when adding minutes to the current time using jQuery?**
There are no inherent limitations when adding minutes to the current time using jQuery. However, keep in mind that all time calculations are ultimately performed based on the user’s system time settings. Any discrepancies or variations in the system time may affect the results. Additionally, be cautious when handling time zones, especially when working with international audiences or server-side logic.
—
In conclusion, jQuery provides a convenient and efficient way to add minutes to the current time in web development. By leveraging the `Date` object and jQuery functions, you can easily manipulate time and display it on your web pages. Whether you need to update a clock, schedule events, or calculate future timestamps, this technique empowers you with the ability to work with time dynamically within your web applications.
Images related to the topic add minutes to current time javascript
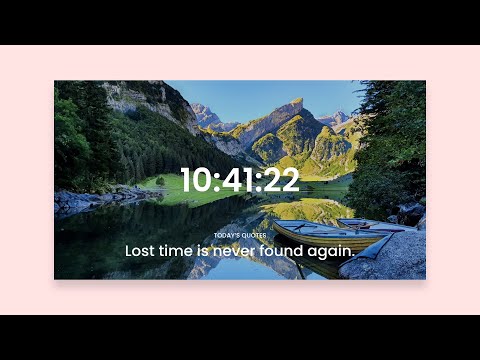
Found 47 images related to add minutes to current time javascript theme



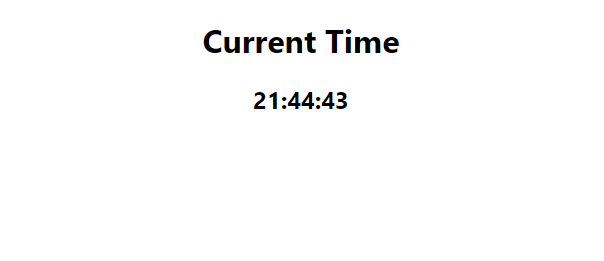
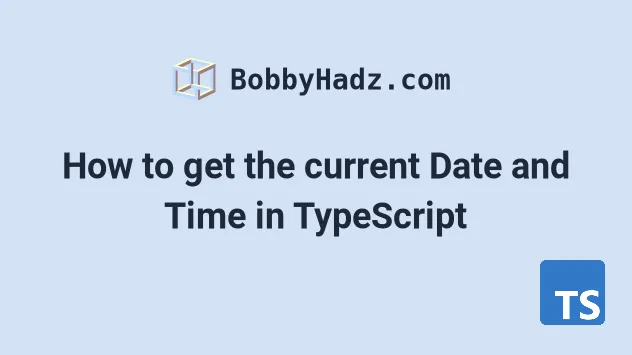
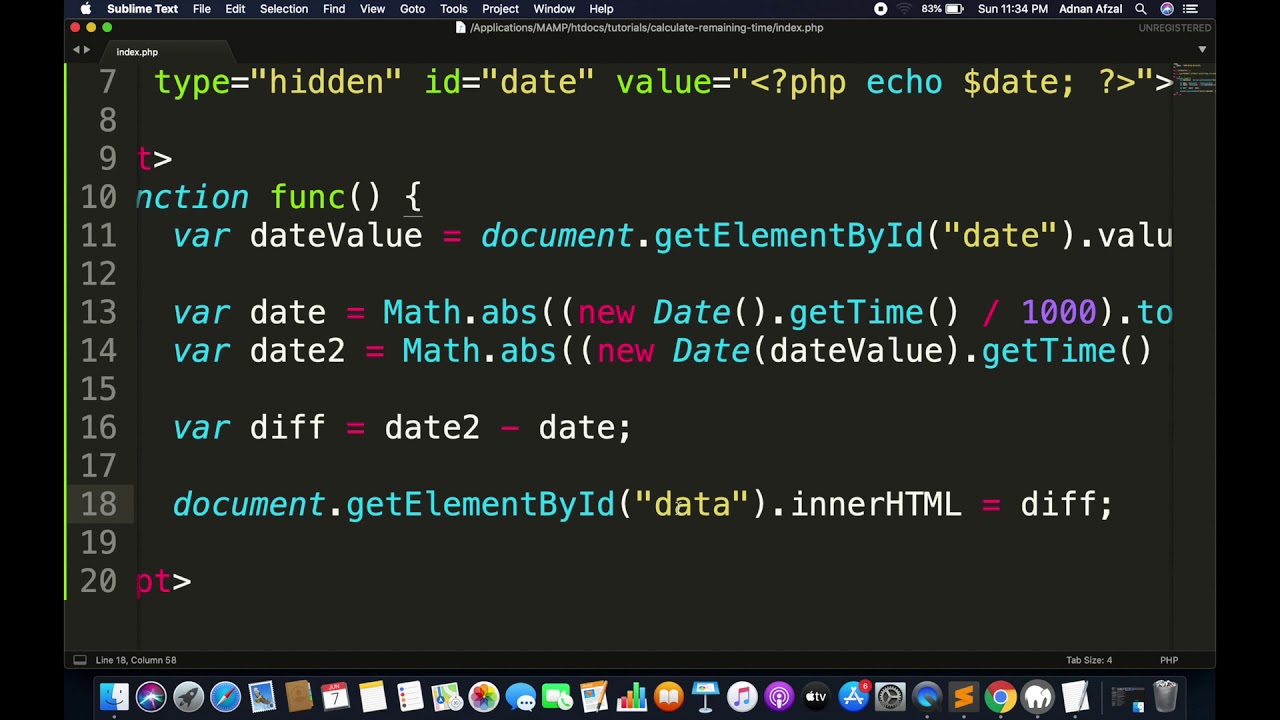
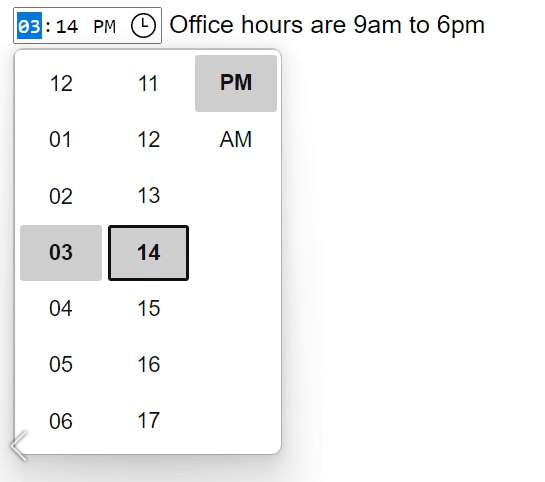


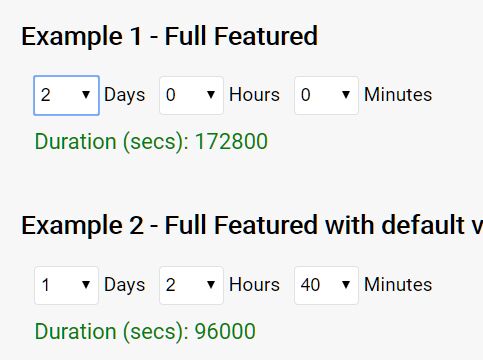

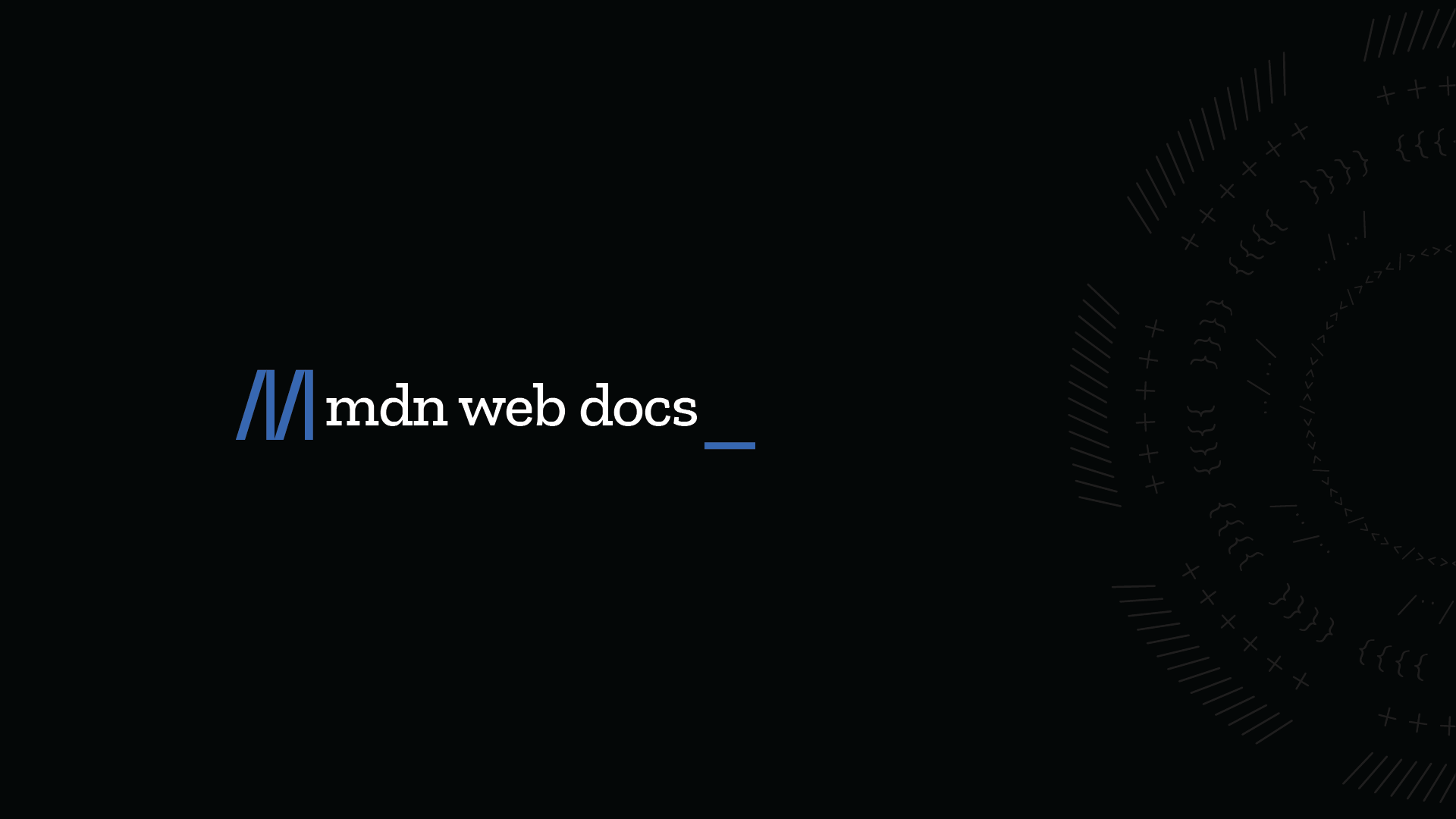
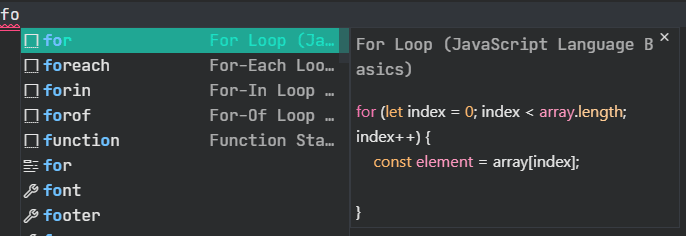
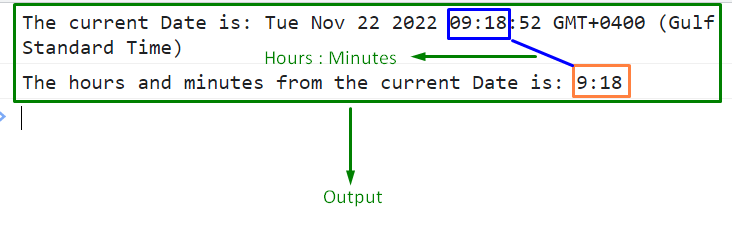

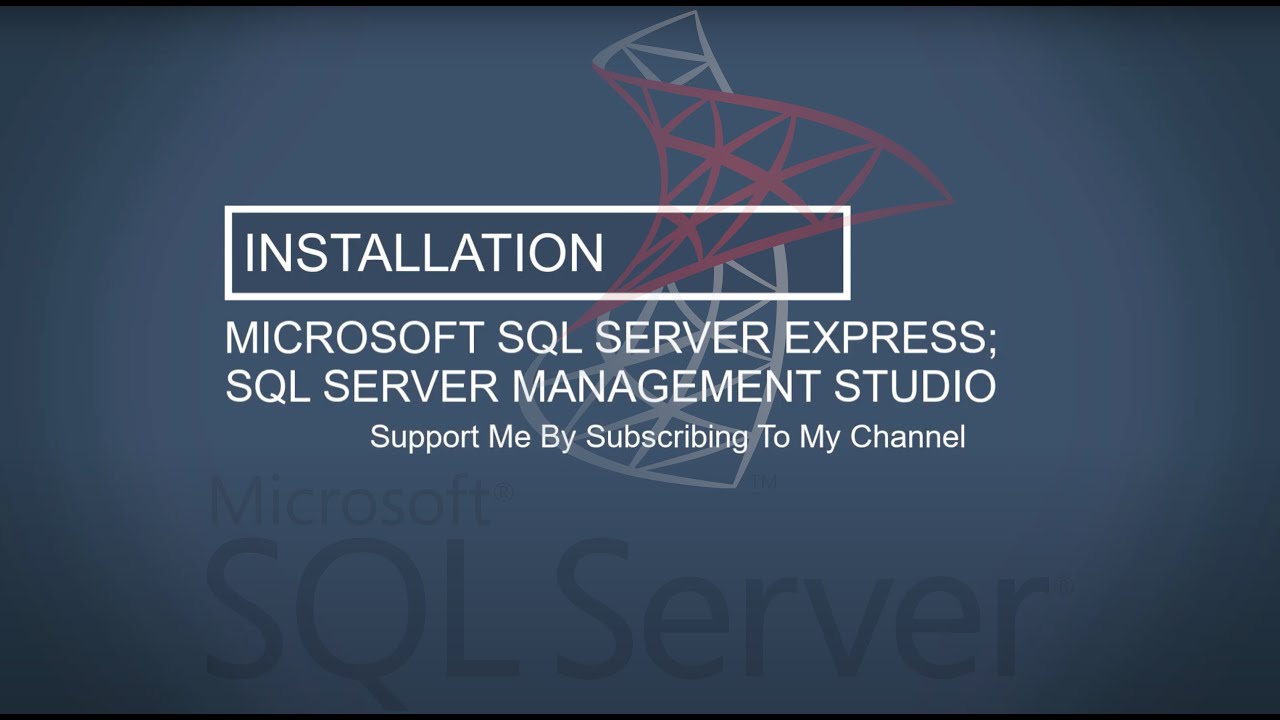
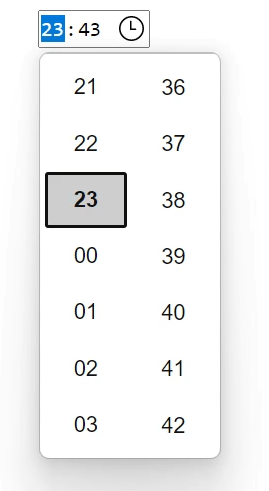
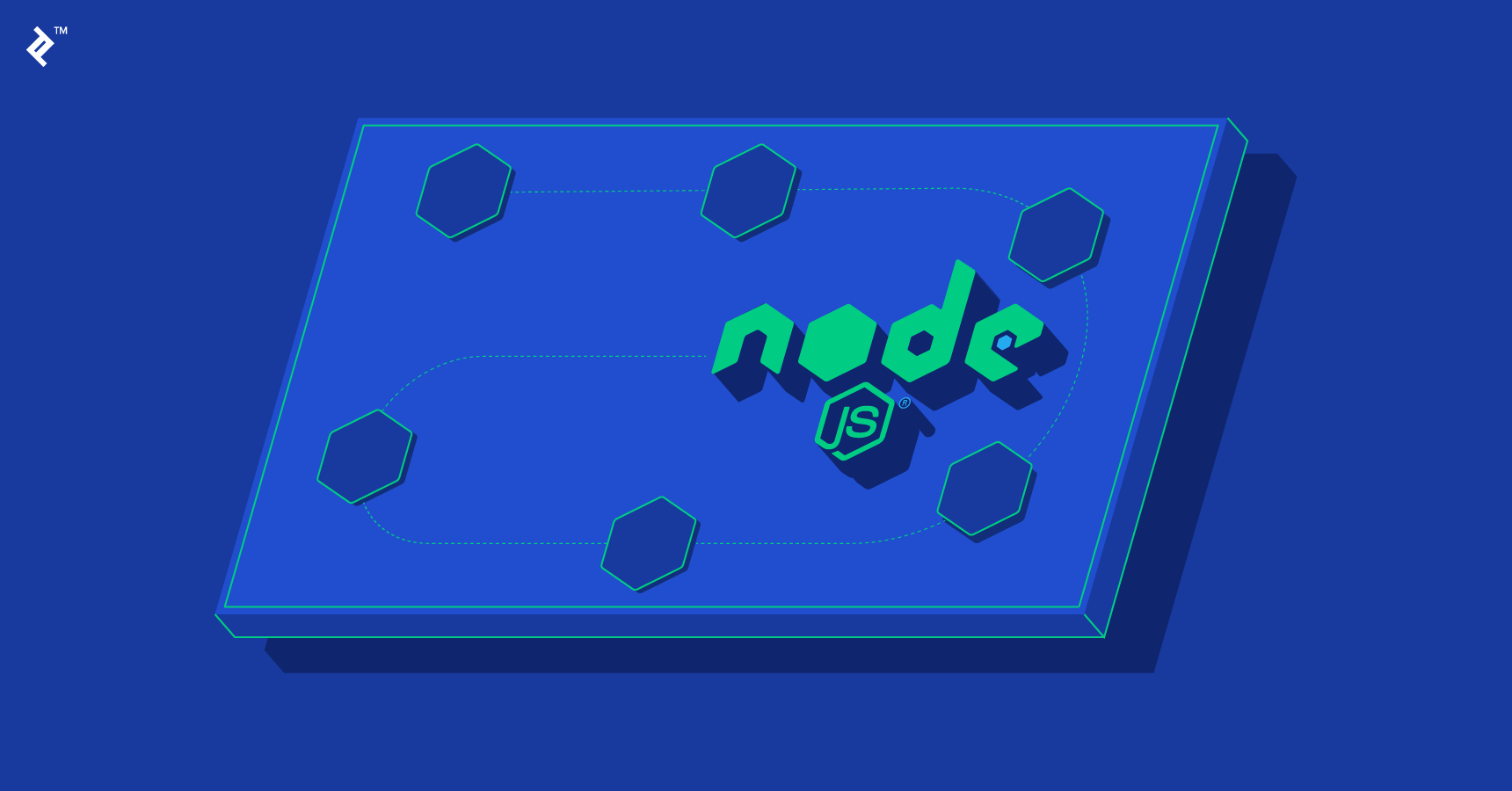
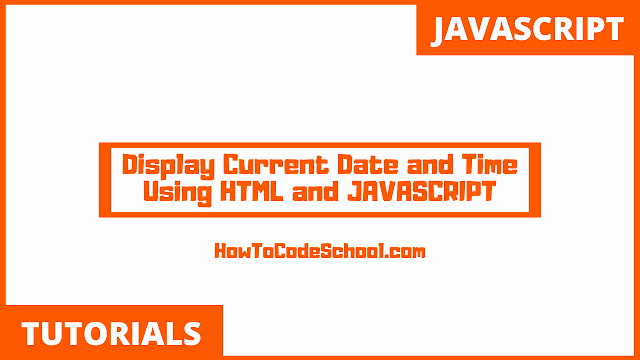

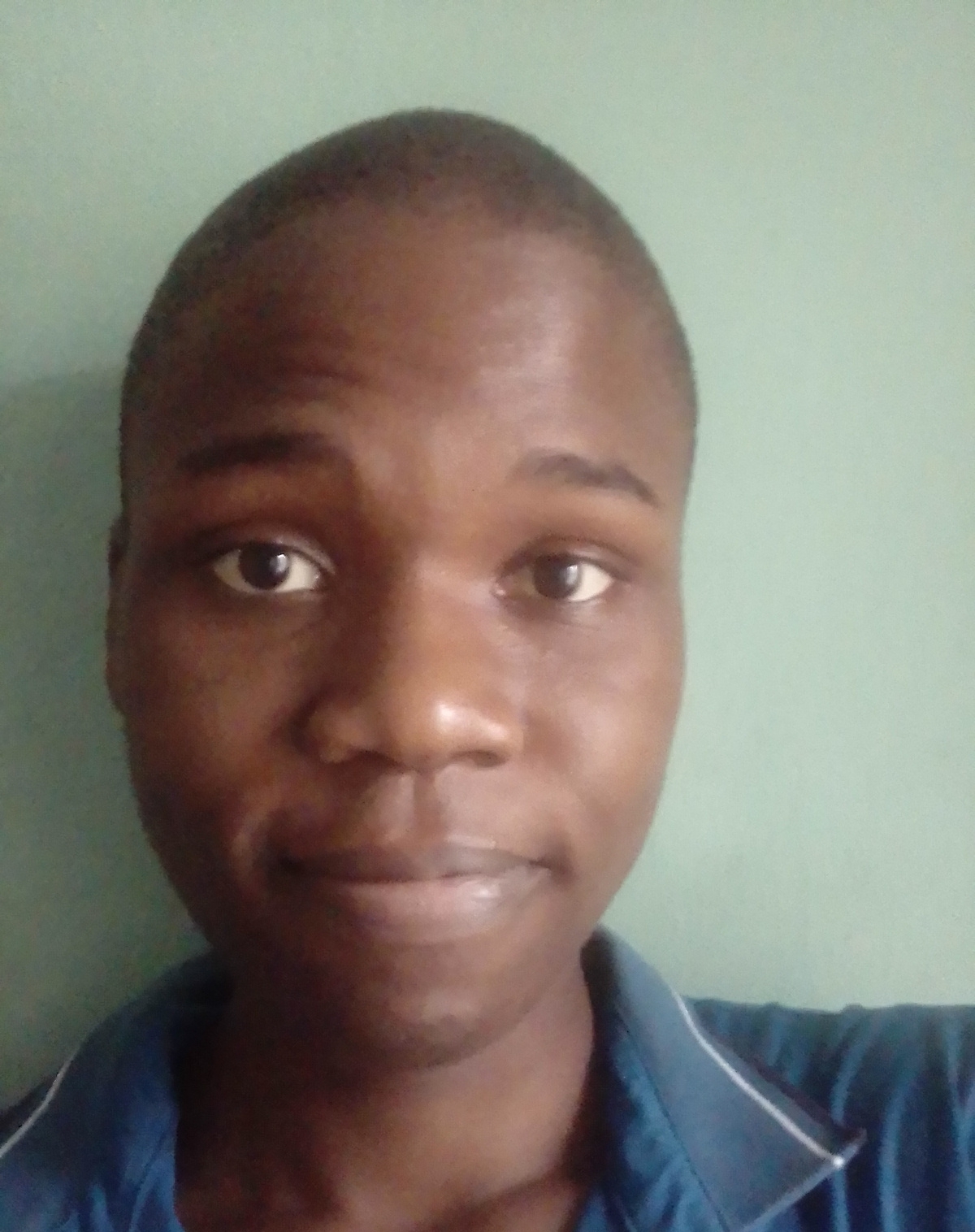

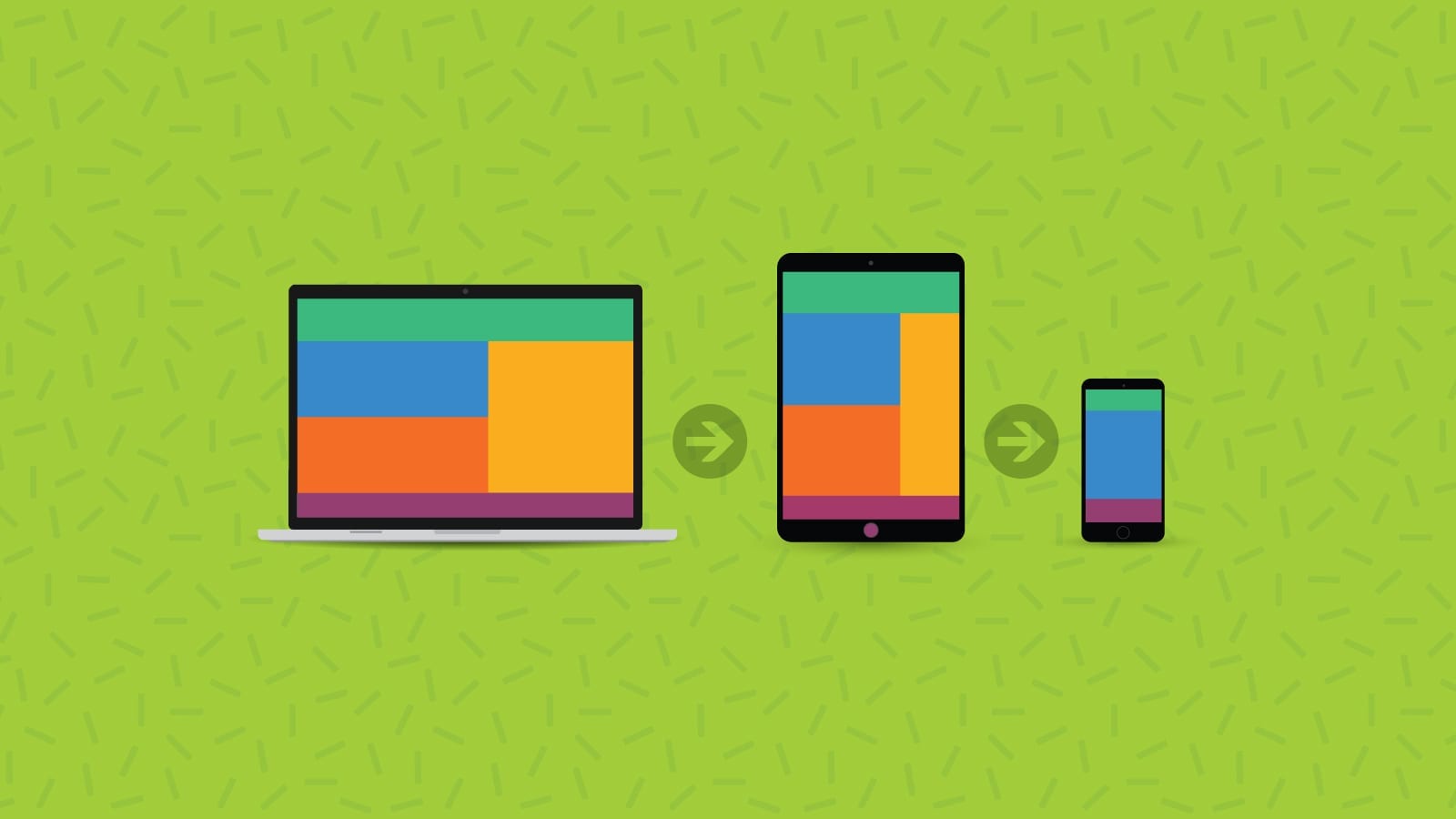
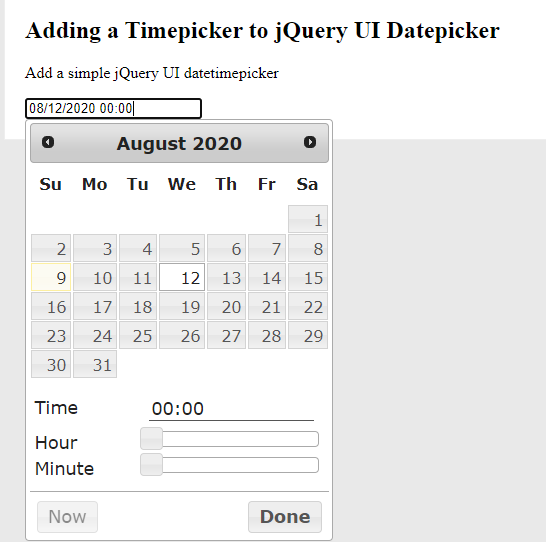
Article link: add minutes to current time javascript.
Learn more about the topic add minutes to current time javascript.
- How to add 30 minutes to a JavaScript Date object?
- How to Add Minutes to a Date in JavaScript | bobbyhadz
- How to Add Minutes to a Date in JavaScript – Coding Beauty
- JavaScript: Add minutes to a Date object – w3resource
- How to add 30 minutes to a JavaScript Date object
- How to Add Hours to a Date in JavaScript – Coding Beauty
- Add 10 seconds to a Date – javascript – Stack Overflow
- How to add 30 minutes to a JavaScript Date object
- Add Minutes to a Date in JavaScript or Node.js – Future Studio
- Add Minutes to a Date in JavaScript – Linux Hint
- JavaScript Date setMinutes() Method – W3Schools
- JavaScript Adding minutes to Date object – GeeksforGeeks
- How to add minutes to a date in JavaScript
See more: blog https://nhanvietluanvan.com/luat-hoc