Bash For In Range
Bash, which stands for “Bourne Again SHell,” is a command-line interpreter widely used in Unix-based operating systems. It provides a robust and flexible scripting language for automating tasks and executing complex operations. One of the fundamental control structures in Bash is the for loop, which allows you to iterate over a range of numbers, a list of values, or elements in an array. In this article, we will delve into the various aspects of the “for” loop in Bash, including syntax, techniques, and troubleshooting tips.
Syntax of the for loop in Bash
To begin with, let’s explore the basic syntax of the for loop in Bash:
“`
for variable in range/list/array
do
# code to be executed
done
“`
Here, the “variable” represents a placeholder that takes different values from the specified range, list, or array. The code within the “do” and “done” block is executed for each iteration, with the “variable” holding the current value.
Unrolling a loop in Bash
Sometimes, you may need to unroll a loop, which means executing the loop a fixed number of times without any iterations. To achieve this, you can use the following approach:
“`
for ((i=0; i < 5; i++))
do
# code to be executed
done
```
In this example, the loop is unrolled five times, and you can perform repetitive tasks without any dependencies on the loop variable. It provides a useful way to execute code snippets multiple times without the overhead of iterating over a range or a list.
Executing a loop for a specific range of numbers in Bash
The for loop in Bash can be effectively used to iterate over a specific range of numbers. You can accomplish this using the `seq` command, which generates a sequence of numbers. Let's see an example:
```
for i in $(seq 1 5)
do
# code to be executed
done
```
In this case, the loop iterates from 1 to 5, with the "i" variable taking values 1, 2, 3, 4, and 5 in each iteration. You can customize the range by specifying the starting and ending numbers as arguments to the `seq` command.
Using variables in the for loop in Bash
Variables play a crucial role in Bash scripting, and they can be utilized within for loops as well. You can define a variable and assign it a range of numbers, list of values, or an array. Let's consider an example:
```
range="1 2 3 4 5"
for i in $range
do
# code to be executed
done
```
In this scenario, the variable "range" contains a list of numbers. The for loop then iterates over each element in the list, with the "i" variable representing the current value in each iteration. This method enables you to reuse and customize the list of values without modifying the loop structure.
Nested for loops in Bash
The flexibility of Bash allows you to nest loops within each other, known as nested for loops. This capability is particularly handy when you need to perform operations on multiple dimensions or iterate over combinations of variables. Let's explore an example:
```
for i in range1
do
for j in range2
do
# code to be executed
done
done
```
In this illustration, the outer loop iterates over "range1" and the inner loop iterates over "range2" for each iteration of the outer loop. You can modify the code within the inner loop to perform actions that involve both "i" and "j" variables.
Iterating over a list or array in Bash using the for loop
Besides iterating over ranges and numbers, the for loop in Bash can handle arrays and lists effectively. Let's explore both scenarios:
For iterating over an array:
```
my_array=("value1" "value2" "value3")
for value in "${my_array[@]}"
do
# code to be executed
done
```
Here, the for loop iterates over each element in "my_array," with the "value" variable holding the current array element.
For iterating over a list:
```
my_list="value1 value2 value3"
for value in $my_list
do
# code to be executed
done
```
In this case, the list of values is stored in a variable, and the for loop iterates over each element in the list to execute the desired code.
Common errors and troubleshooting tips for the for loop in Bash
While working with for loops in Bash, you may encounter some common errors and face troubleshooting challenges. Listed below are a few tips to overcome such issues:
1. Bash for in range step: By default, the for loop iterates through each element in the range or list incrementing by one. If you need to specify a custom step or increment value, you can achieve it by using the `seq` command with appropriate arguments.
2. If in shell: You can utilize an "if" condition within a for loop to check for specific conditions and execute certain code only if the condition is satisfied. This way, you can incorporate conditional execution within each iteration.
3. Modulo in bash: The modulo operator (%) can be used within a for loop to perform operations based on divisibility or to alternate between multiple actions after a specific number of iterations.
4. Variable in for loop bash: Variables can be used to define ranges, lists, or arrays in Bash. Ensure that the variable values are correctly assigned, and variables are enclosed within quotes to handle spaces or special characters.
In conclusion, the for loop in Bash is a versatile and powerful control structure that allows you to iterate over ranges, lists, and arrays efficiently. It provides flexibility and customization options essential for automating tasks and performing repetitive operations. By understanding the syntax, techniques, and troubleshooting tips covered in this article, you can harness the full potential of the for loop in Bash and enhance your scripting skills. So, go ahead and explore the vast capabilities of this fundamental construct in the Bash scripting language.
Bash: For-Loop: Range And Read From File
Keywords searched by users: bash for in range Bash for in range step, Bash for loop range, Shell script for loop from 1 to n, If in shell, Modulo in bash, Variable in for loop bash, For in Bash, For shell script
Categories: Top 73 Bash For In Range
See more here: nhanvietluanvan.com
Bash For In Range Step
## Understanding the Bash for loop
The Bash for loop is a control structure that allows us to iterate over a set of values and execute a block of code for each iteration. The general syntax of a for loop in Bash is as follows:
“`bash
for variable in list
do
# code to be executed
done
“`
Here, `variable` is a user-defined name that represents each item in the `list` during each iteration. The list can be any sequence of elements, such as strings or numbers. The code block within the loop is executed for each value in the list.
## Utilizing the Bash for in range step
Bash does not provide a specific syntax for a for loop with a step, like some other languages do. However, we can achieve a similar functionality using the `seq` command and arithmetic expressions.
The `seq` command generates a sequence of numbers from a starting value to an ending value. By specifying the appropriate step size, we can effectively iterate through a range of values with a specific step in a for loop. The basic syntax for using `seq` is as follows:
“`bash
seq [OPTION]… FIRST [STEP]
“`
The `FIRST` argument specifies the starting value of the sequence, and the optional `STEP` argument defines the increment between each subsequent number. If `STEP` is not provided, the default value is 1.
To incorporate `seq` into a Bash script, we can use command substitution by enclosing the `seq` command within backticks or `$()`.
## Example usage
Let’s consider a practical example to demonstrate the usage of the Bash for in range step command. Suppose we want to print all the odd numbers between 1 and 10 using a step of 2.
“`bash
for i in $(seq 1 2 10)
do
echo $i
done
“`
In this example, the `seq` command generates a sequence starting from 1 with a step of 2 until 10. The for loop then iterates over each generated number and prints it using the `echo` command.
The output of this script would be:
“`
1
3
5
7
9
“`
As evident from the output, the script successfully prints all the odd numbers from 1 to 10, skipping every even number.
## FAQs about Bash for in range step
**Q: Can I use negative steps in a Bash for loop?**
A: Yes, you can use negative steps in Bash for loops. By specifying a negative step value, such as `-1`, you can iterate in reverse order or count down from a larger number to a smaller number.
**Q: How can I use a variable step value in a for loop?**
A: To use a variable step value, you can store the desired step size in a variable and then use that variable within the `seq` command. For example:
“`bash
step=3
for i in $(seq 1 $step 10)
do
echo $i
done
“`
**Q: Is there an alternative to using `seq` for generating a sequence in Bash?**
A: Yes, there are alternative ways to generate sequences in Bash without using `seq`. One common approach is to use a while loop along with an arithmetic expression to manually increment or decrement the loop variable until a specific condition is met.
**Q: Can I use non-integer values in a Bash for loop with a step?**
A: No, the `seq` command and the Bash for loop do not support non-integer values or decimal steps by default. However, you can achieve similar functionality by appropriately scaling the values and steps to work with integers.
In conclusion, understanding the usage and syntax of the Bash for in range step command can greatly enhance your scripting abilities. By utilizing the `seq` command, you can efficiently loop through a range of values with a specific step in your Bash scripts. So go ahead and experiment with different step sizes and values to automate your tasks and make your scripts more versatile.
Bash For Loop Range
The Bash scripting language provides an array of powerful features and functionalities, that make it an ideal choice for automating repetitive tasks. One such feature is the “for” loop range, which allows users to iterate through a sequence of elements. In this article, we will explore the Bash for loop range in depth, providing detailed explanations and examples of its usage.
Understanding the Syntax
The syntax of the Bash for loop range follows a specific pattern. The basic structure is as follows:
“`bash
for variable in range
do
# commands to be executed
done
“`
Here, the “variable” represents the placeholder that will store each element of the range as the loop iterates. The “range” specifies the sequence of elements to be iterated over. It can be defined in multiple ways, as we will discuss further.
Specifying a Range with Literal Values
The simplest way to define a range in a Bash for loop is by explicitly listing the values. For example, to iterate through the elements 1, 2, and 3, we can use the following syntax:
“`bash
for i in 1 2 3
do
echo $i
done
“`
The loop will iterate three times, with the variable “i” taking the values 1, 2, and 3 in each iteration. In this case, the “range” is defined by the literal values provided.
Using a Range Operator
Bash also allows the usage of range operators to define a sequence of values. The two primary range operators are “..” and “…”.
The “..” operator generates a range up to, and including, the specified value. For instance, to iterate through the elements 1, 2, 3, we could define the range as:
“`bash
for i in {1..3}
do
echo $i
done
“`
Similarly, the “…” operator generates a range up to, but excluding, the specified value. Using the previous example, the new syntax would be:
“`bash
for i in {1…3}
do
echo $i
done
“`
Both these operators enhance the convenience of defining ranges, resulting in concise and readable code.
Specifying a Range with Variables
In addition to literal values, we can also utilize variables to define the range in a Bash for loop. This flexibility allows for dynamic programming and handling of larger datasets.
Consider the following example, where we want to iterate through a range specified by two variables, “start” and “end”:
“`bash
start=1
end=5
for i in $(seq $start $end)
do
echo $i
done
“`
Here, the “seq” command generates a sequence of numbers between the “start” and “end” values, as specified by the variables. The loop will iterate through the elements 1, 2, 3, 4, and 5.
The Importance of the Bash For Loop Range
The Bash for loop range is a powerful tool that enables automation and streamlines repetitive tasks. It allows users to perform operations on a set of elements without manual iterations, thereby saving time and effort. With the ability to define ranges using literal values, range operators, and variables, the Bash for loop becomes even more versatile.
Frequently Asked Questions (FAQs):
Q: Can the Bash for loop iterate over non-numeric elements?
A: Yes, the Bash for loop is not restricted to numeric ranges. It can iterate over any sequence of elements, whether alphanumeric or textual.
Q: How can I iterate through a range in reverse order?
A: To iterate through a range in reverse order, you can specify the range in descending order or utilize the “seq” command with the “step” argument. For example:
“`bash
for i in {5..1}
do
echo $i
done
“`
or
“`bash
for i in $(seq 5 -1 1)
do
echo $i
done
“`
Both these methods will iterate through the range 5, 4, 3, 2, 1.
Q: Is it possible to nest multiple for loops?
A: Yes, it is possible to nest multiple for loops within each other. This allows for complex iterations and handling of multidimensional datasets.
In conclusion, the Bash for loop range is a fundamental feature of the Bash scripting language that greatly enhances the automation capabilities. By understanding its syntax and various ways to define a range, users can leverage this powerful tool to efficiently process data, automate tasks, and perform repetitive operations.
Images related to the topic bash for in range
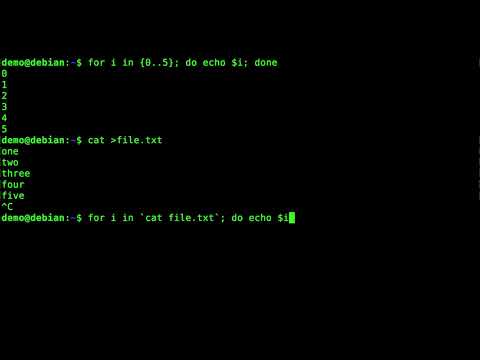
Found 18 images related to bash for in range theme
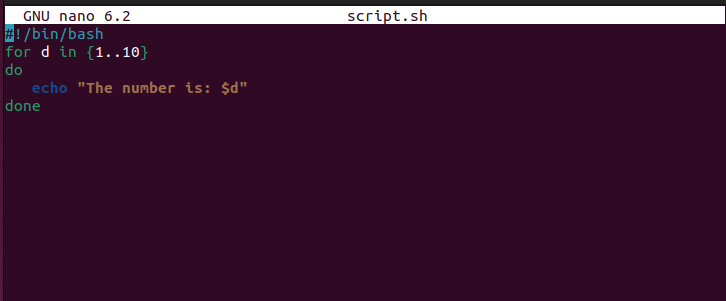
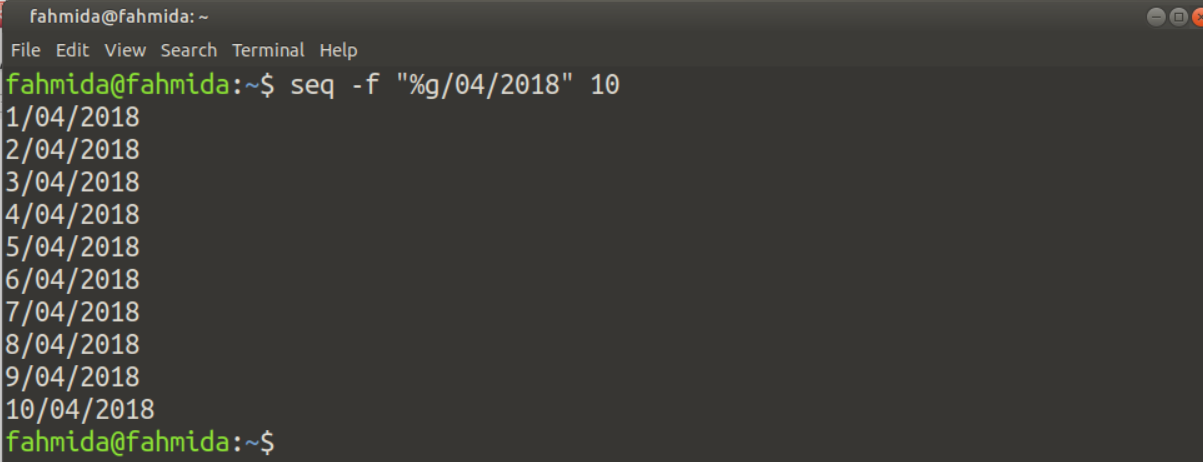

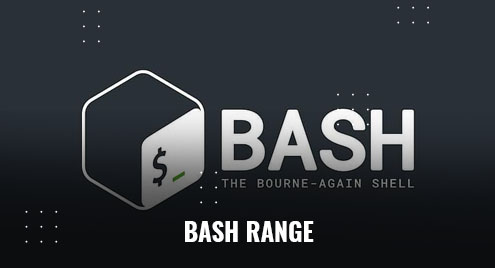
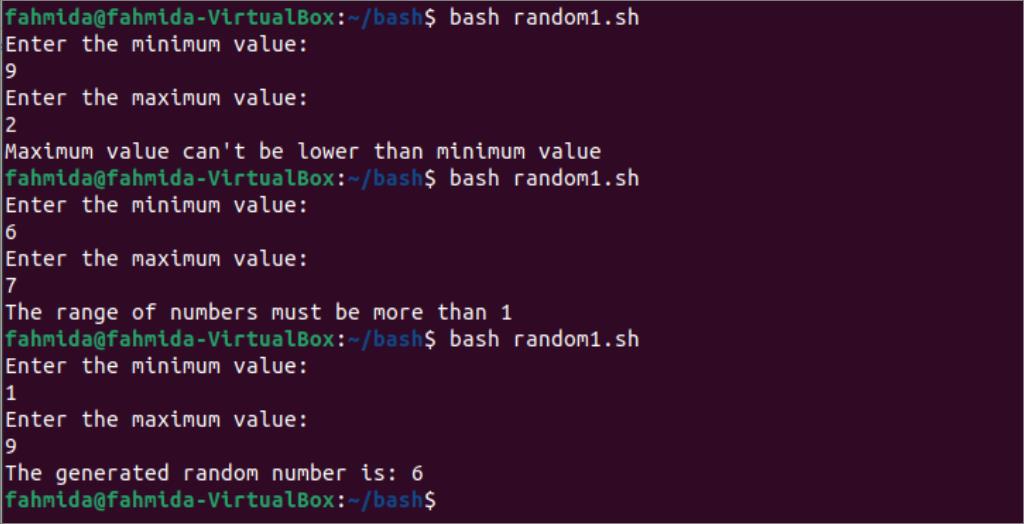
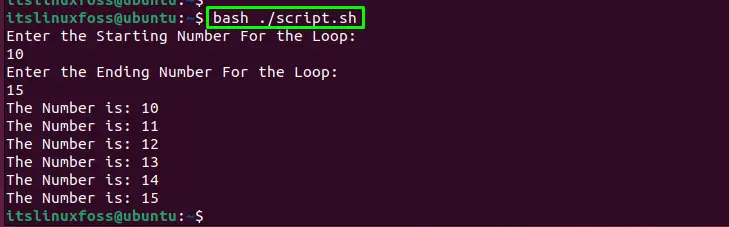
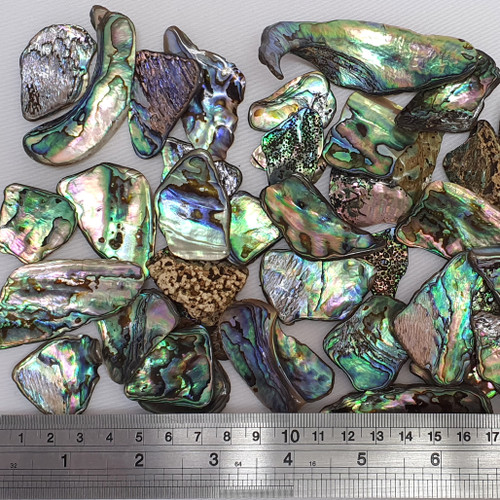

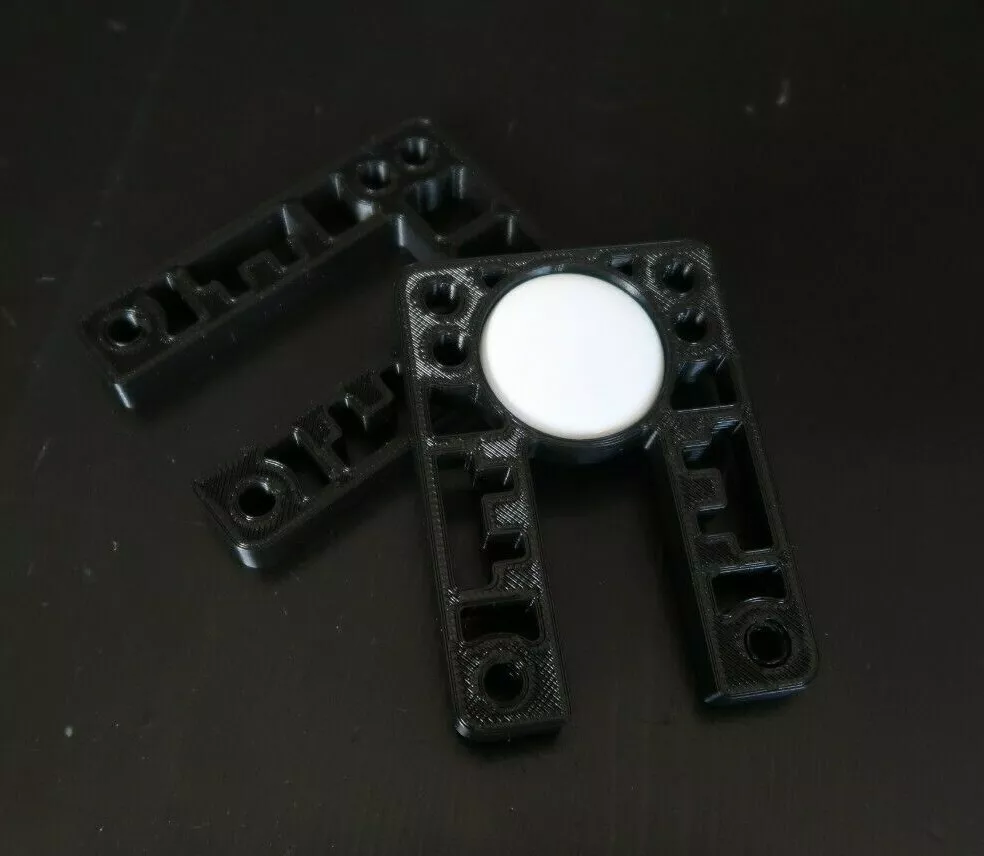
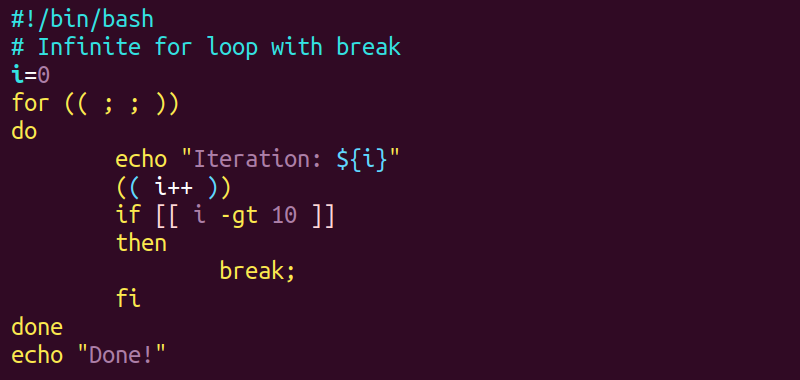
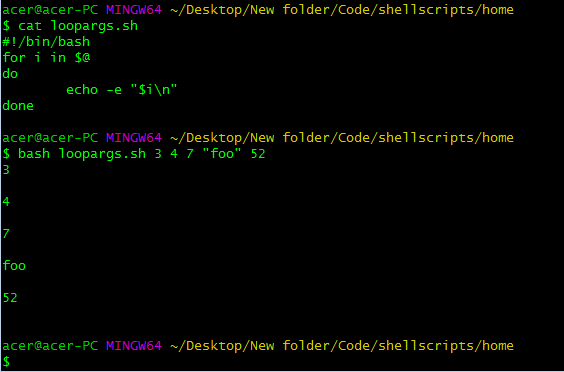
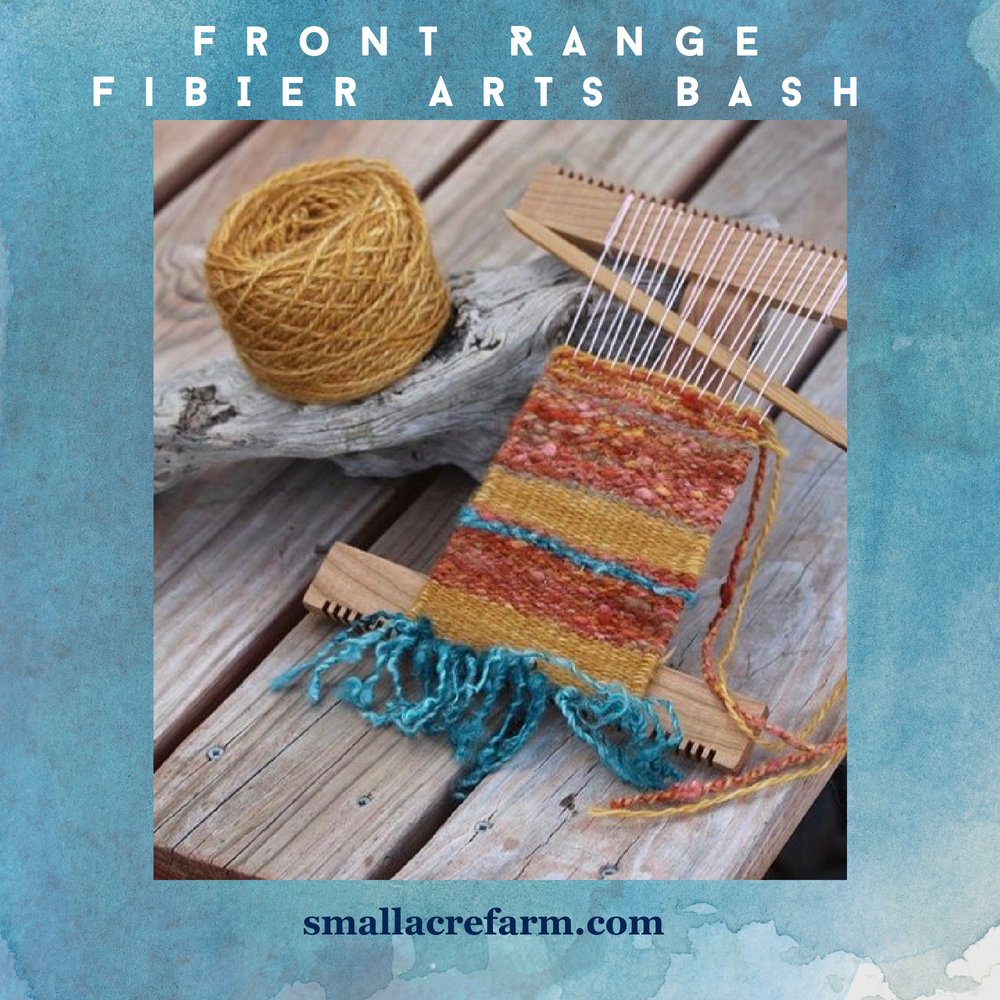

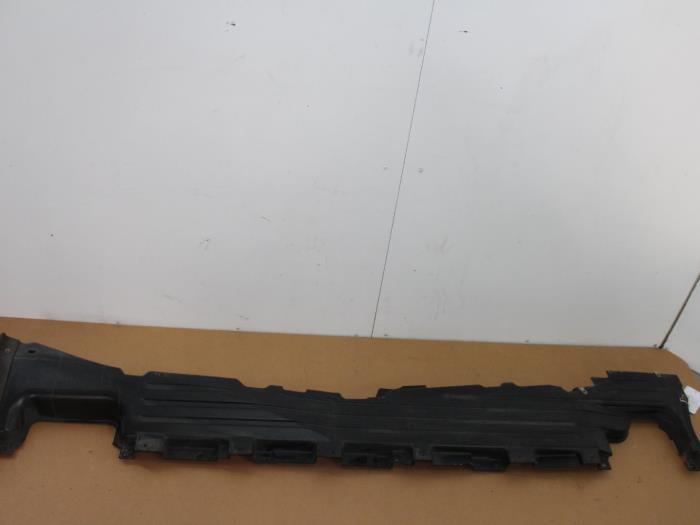
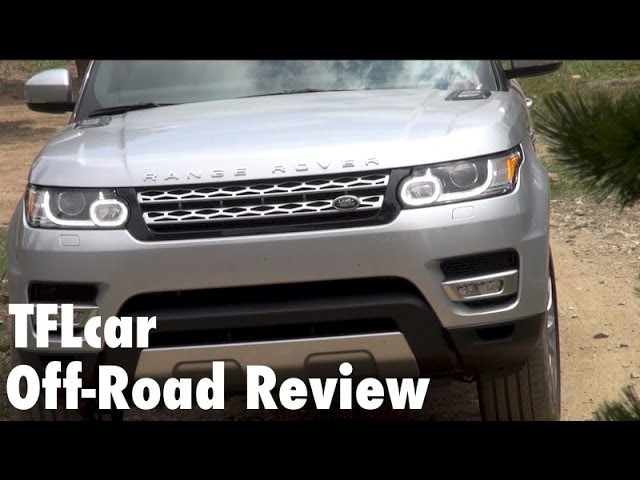
Article link: bash for in range.
Learn more about the topic bash for in range.
- How to iterate Bash For Loop variable range Under Unix / Linux
- How do I iterate over a range of numbers defined by variables …
- Bash Range – Linux Hint
- Iterate Over a Range of Numbers Defined by Variables in Bash
- Bash for Loop With Range {#..#} – Its Linux FOSS
- Bash for loop with range {#..#} – command line – Ask Ubuntu
- Bash for Loop Range Variable – LinuxOPsys
- How to use range and sequence expression in bash – Xmodulo
- bash random number in range – SoftHints
- Bash Ranges | Delft Stack
See more: https://nhanvietluanvan.com/luat-hoc