C# Read A Text File
When it comes to programming, reading text files is a common requirement for various tasks. Whether you’re working with data extraction, data processing, or even logging, being able to read text files efficiently and effectively is crucial. In this article, we will discuss how to read a text file in C# and explore the different methods and techniques available for this task.
File Handling in C#
Before diving into the specifics of reading a text file, let’s first understand the concept of file handling in C#. File handling refers to the processes involved in creating, opening, reading, writing, and closing files. It is an essential aspect of programming, as it enables us to work with files and perform various operations on them.
In the context of reading a text file, file handling plays a crucial role in managing the file and accessing its content. It provides the necessary tools and methods to interact with the text file and retrieve the desired information.
Opening a Text File in C#
To read a text file in C#, the first step is to open the file. There are multiple ways to achieve this, depending on the requirements of your program.
One common approach is to use the File class, provided by the System.IO namespace in C#. This class contains static methods that simplify file-related operations, including opening and reading text files.
The File class provides a method called OpenText, which opens a text file for reading and returns a StreamReader object that allows us to read the file’s content. Here’s an example of how you can use this method:
“`csharp
string filePath = “path/to/textfile.txt”;
StreamReader reader = File.OpenText(filePath);
“`
Reading a Text File Line by Line
Once you have opened the text file, you can start reading its content. One of the common methods for reading a text file is to read it line by line. This approach is useful when you need to process each line individually or perform line-based operations.
To read a text file line by line in C#, you can use the StreamReader class, which is also provided by the System.IO namespace. The StreamReader class provides methods like ReadLine, which allows you to read a single line from the text file.
Here’s an example of how you can read a text file line by line using StreamReader:
“`csharp
string line;
while ((line = reader.ReadLine()) != null)
{
// Process the line here
Console.WriteLine(line);
}
“`
Reading a Text File as a Whole
While reading a text file line by line is a common method, there are cases where you may want to read the entire content of the file at once. This approach can be beneficial when you need to perform operations on the entire content as a whole, such as parsing or searching.
To read a text file as a whole in C#, you can use the ReadToEnd method provided by the StreamReader class. This method reads the entire content of the text file and returns it as a string.
Here’s an example of how you can read a text file as a whole using the ReadToEnd method:
“`csharp
string content = reader.ReadToEnd();
Console.WriteLine(content);
“`
Handling File Exceptions
During the process of reading a text file, various exceptions may occur. These exceptions can result from issues like file not found, permission denied, or improper file format. To ensure robust and error-free code, it’s essential to handle these exceptions properly.
In C#, you can use try-catch blocks to handle file exceptions. By wrapping the file reading code in a try block and catching specific exceptions in the catch block, you can implement the necessary error-handling logic.
Here’s an example of how you can handle file exceptions while reading a text file in C#:
“`csharp
try
{
// File reading code here
}
catch (FileNotFoundException ex)
{
Console.WriteLine(“File not found: ” + ex.FileName);
}
catch (IOException ex)
{
Console.WriteLine(“An error occurred while reading the file: ” + ex.Message);
}
“`
Manipulating the Read Data
Once you have successfully read the content of a text file, you may need to manipulate the data according to your program’s requirements. C# provides a range of tools and methods to help you perform various data manipulations efficiently.
Some common data manipulations include splitting the content into separate elements, filtering out specific data points, or parsing the data into meaningful structures. Be sure to choose the appropriate data manipulation technique based on your specific needs and the structure of the text file.
Closing the Text File
After completing the required operations on a text file, it’s crucial to close the file properly. Failing to do so can result in resource leaks and potential issues with file availability for other processes or programs.
In C#, you can use the Close method to close a text file that you have opened using StreamReader. Additionally, best practices recommend using the using statement, which automatically closes the file when the code block is exited, even in the case of exceptions.
Here’s an example of how you can properly close a text file using the using statement:
“`csharp
using (StreamReader reader = File.OpenText(filePath))
{
// Perform file reading operations
} // The file will automatically be closed at this point
“`
Best Practices for Reading Text Files in C#
To ensure efficient and clean code when reading text files in C#, consider the following best practices:
1. Use the appropriate method based on the requirements of your program. If you need to process each line independently, read the file line by line. If you require the entire content at once, read the file as a whole.
2. Implement proper exception handling to handle file-related exceptions gracefully. Consider the specific exceptions that may occur and provide appropriate error messages or fallback mechanisms.
3. Take advantage of available tools and methods to manipulate the data read from the text file efficiently. Choose the appropriate data manipulation technique based on your requirements and the structure of the text file.
4. Always close the text file properly after completing your operations. Use the Close method or the using statement to ensure resource management and prevent potential issues with the file.
5. When working with large text files, consider optimizing your code for performance and memory usage. Use techniques like buffering, parallel processing, or memory mapping to handle large text files efficiently and reliably.
Conclusion
Reading a text file in C# is an essential skill for many programming tasks. By understanding the file handling concepts and utilizing the available tools and techniques, you can effectively read and manipulate the content of text files. Remember to follow best practices for efficient and clean code and consider the specific requirements and challenges posed by large text files. With these techniques and practices, you’ll be well-equipped to handle text file operations in your C# programs.
FAQs
Q1. How do I read a text file in C#?
To read a text file in C#, you can use the File class from the System.IO namespace. The OpenText method provided by the File class opens the text file and returns a StreamReader object that allows you to read the file’s content. Here’s an example:
“`csharp
string filePath = “path/to/textfile.txt”;
StreamReader reader = File.OpenText(filePath);
“`
Q2. What is the difference between reading a text file line by line and reading it as a whole?
Reading a text file line by line involves processing each line individually, which is useful for operations that depend on specific line-based logic. Reading a text file as a whole involves reading the entire content of the file at once, which is beneficial for operations that require the entire content as a whole, such as parsing or searching.
Q3. How can I handle file exceptions while reading a text file in C#?
You can handle file exceptions by using try-catch blocks in your code. By wrapping the file reading operations in a try block and catching specific exceptions in the catch blocks, you can implement the necessary error-handling logic. For example:
“`csharp
try
{
// File reading code here
}
catch (FileNotFoundException ex)
{
// Handle file not found exception
}
catch (IOException ex)
{
// Handle IO exception
}
“`
Q4. Can I manipulate the data read from a text file in C#?
Yes, you can manipulate the data read from a text file in C#. C# provides a range of tools and methods to help you perform various data manipulations efficiently. For example, you can split the content into separate elements, filter out specific data points, or parse the data into meaningful structures.
Q5. Is it important to close a text file after reading operations?
Yes, it is important to close a text file after completing your reading operations. Failing to do so can result in resource leaks and potential issues with the file’s availability for other processes or programs. Closing the file properly helps with resource management and ensures the file is released correctly.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# read a text file
Categories: Top 25 C# Read A Text File
See more here: nhanvietluanvan.com
Images related to the topic c# read a text file
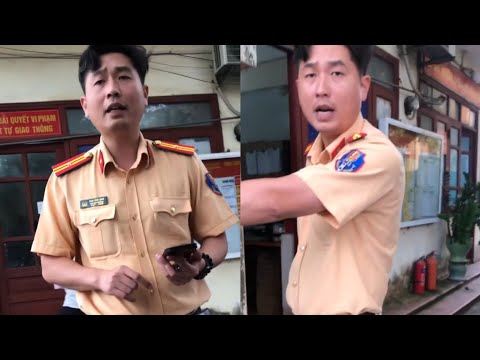
Article link: c# read a text file.
Learn more about the topic c# read a text file.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc