C# Readonly Dictionary Example
In C#, a Dictionary is a collection of key-value pairs that allows for efficient lookup, insertion, and removal of elements based on their keys. Normally, a Dictionary can be modified after it is created, meaning its elements can be added, updated, or removed at any time.
However, there might be cases where you want to ensure that the contents of a dictionary cannot be altered once it is initialized. This is where a Readonly Dictionary comes into play. As the name suggests, a Readonly Dictionary is a dictionary where the key-value pairs cannot be modified after the dictionary is created.
Initializing a Readonly Dictionary in C#
To initialize a Readonly Dictionary in C#, you can make use of the System.Collections.Generic namespace. This namespace provides a class called “ReadOnlyDictionary” that allows you to create a dictionary that cannot be modified.
Here’s an example of how you can initialize a Readonly Dictionary:
“`csharp
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
class Program
{
static void Main(string[] args)
{
Dictionary
{
{ 1, “Apple” },
{ 2, “Banana” },
{ 3, “Cherry” }
};
ReadOnlyDictionary
// Now, readOnlyDictionary is a Dictionary that cannot be modified
}
}
“`
In the above example, we first create a regular Dictionary called “dictionary” and initialize it with some key-value pairs. Then, we pass this dictionary as an argument to the constructor of ReadOnlyDictionary to create a Readonly Dictionary called “readOnlyDictionary”.
Adding Elements to a Readonly Dictionary
As mentioned earlier, a Readonly Dictionary cannot be modified after it is created. Therefore, you cannot directly add elements to a Readonly Dictionary. If you attempt to do so, it will result in a runtime exception.
To add elements to a dictionary that needs to remain readonly, you should first create a regular dictionary, add elements to it, and then create a readonly dictionary from it.
Accessing Elements in a Readonly Dictionary
Accessing elements in a Readonly Dictionary is exactly the same as accessing elements in a regular Dictionary. You can retrieve the value associated with a key using the indexer notation or the TryGetValue method.
Here’s an example:
“`csharp
string value = readOnlyDictionary[1]; // Accessing the value associated with key 1
if (readOnlyDictionary.TryGetValue(2, out string value2))
{
// Accessing the value if key 2 exists in the dictionary
}
“`
Updating Elements in a Readonly Dictionary
Since a Readonly Dictionary cannot be modified, you cannot directly update elements in it. If you attempt to do so, it will result in a runtime exception.
To update elements in a dictionary that needs to remain readonly, you should first create a regular dictionary, modify the elements in it, and then create a readonly dictionary from it.
Removing Elements from a Readonly Dictionary
Similar to adding and updating elements, you cannot directly remove elements from a Readonly Dictionary. You will encounter a runtime exception if you try to do so.
If you need to remove elements from a readonly dictionary, you should follow the same approach mentioned earlier: create a regular dictionary, remove elements from it, and then create a readonly dictionary from it.
Iterating over Elements in a Readonly Dictionary
Iterating over the elements in a Readonly Dictionary is as straightforward as iterating over a regular Dictionary. You can use a foreach loop to iterate over the key-value pairs in the dictionary.
Here’s an example:
“`csharp
foreach (KeyValuePair
{
// Accessing the key-value pair
int key = kvp.Key;
string value = kvp.Value;
// Perform actions on the key-value pair
}
“`
Checking if an Element Exists in a Readonly Dictionary
To check if an element exists in a Readonly Dictionary, you can use the ContainsKey method. This method returns true if the specified key is found in the dictionary; otherwise, it returns false.
Here’s an example:
“`csharp
bool exists = readOnlyDictionary.ContainsKey(1); // Checking if key 1 exists in the dictionary
“`
Clearing a Readonly Dictionary
While you cannot directly clear a Readonly Dictionary since it cannot be modified, you can create a new Readonly Dictionary without any elements to achieve a similar effect.
“`csharp
readOnlyDictionary = new ReadOnlyDictionary
“`
This will essentially create a new empty Readonly Dictionary and assign it to the variable “readOnlyDictionary”, effectively clearing the dictionary.
FAQs about C# Readonly Dictionary
Q: Why should I use a Readonly Dictionary?
A: Readonly Dictionaries are useful in scenarios where you want to ensure that the contents of a dictionary cannot be modified once it is initialized. This can prevent accidental modifications and provide a level of immutability.
Q: Can I modify a Readonly Dictionary after it is created?
A: No, a Readonly Dictionary cannot be modified once it is created. Any attempt to add, update, or remove elements will result in a runtime exception.
Q: Can I use a Readonly Dictionary as a parameter in a method or as a return type?
A: Yes, you can use a Readonly Dictionary as a parameter in a method or as a return type. This can be useful when you want to pass or return a dictionary that should not be modified by the method or the caller.
Q: Are Readonly Dictionaries thread-safe?
A: No, Readonly Dictionaries are not inherently thread-safe. If you need to ensure thread-safety, you should consider using concurrent collections or apply appropriate synchronization mechanisms.
In conclusion, a Readonly Dictionary in C# provides a way to create a dictionary that cannot be modified once it is initialized. While it limits the flexibility of modification, it offers the benefits of immutability and prevents accidental changes. By understanding how to define, initialize, access, and iterate over a Readonly Dictionary, you can incorporate it into your code to ensure the integrity of your data.
Beach Song + More Nursery Rhymes \U0026 Kids Songs – Cocomelon
Keywords searched by users: c# readonly dictionary example
Categories: Top 87 C# Readonly Dictionary Example
See more here: nhanvietluanvan.com
Images related to the topic c# readonly dictionary example
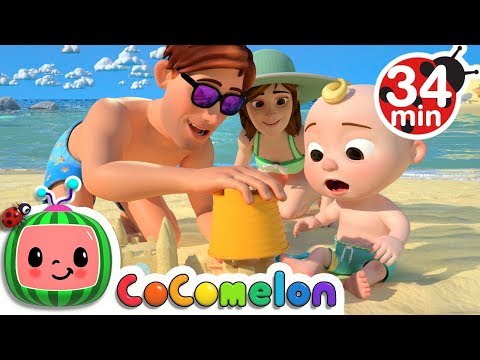
Article link: c# readonly dictionary example.
Learn more about the topic c# readonly dictionary example.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: https://nhanvietluanvan.com/luat-hoc