C# Remove Duplicates From A List
Introduction
In C#, a list is a fundamental data structure that allows you to store and manage collections of objects. A list can contain duplicate elements, meaning that it can store multiple objects with the same value. However, there are situations where it becomes necessary to remove these duplicates. This article will explore various methods to efficiently remove duplicates from a list in C#, providing you with the knowledge and tools to optimize your code.
What is a List in C# and Why Does it Allow Duplicates?
A list in C# is a generic collection class that provides the ability to store and manage a dynamic number of objects of the same type. It is commonly used when you need to work with a set of elements that can grow or shrink in size. The List
The reason a list allows duplicates is to provide flexibility. In many scenarios, having the ability to store multiple objects with the same value can be useful. For example, when dealing with sales data, you may have a list of products where the same product can appear multiple times. However, there are situations where removing duplicates becomes necessary, such as when you want to perform unique operations, improve performance, or ensure data integrity.
The Importance of Removing Duplicates from a List in C#
There are several reasons why removing duplicates from a list in C# is important:
1. Improved Efficiency: By eliminating duplicates, you reduce the amount of memory required to store the list, leading to improved performance and efficiency.
2. Data Integrity: In certain scenarios, having duplicate entries in a list can cause data integrity issues. For instance, consider a list of user emails, where duplicate emails compromise the accuracy and reliability of the data.
3. Enhanced Accuracy: Removing duplicates ensures that operations performed on the list are more accurate and reliable. For example, calculating the average of a list of values becomes more precise when duplicates are eliminated.
Using the Distinct() Method to Remove Duplicates from a List in C#
C# provides an easy and efficient way to remove duplicates from a list using the Distinct() method. The Distinct() method is a LINQ extension method that returns a new list containing only the distinct elements from the original list.
Here’s an example of how you can use the Distinct() method to remove duplicates:
“`csharp
List
List
“`
In this example, the distinctNumbers list will only contain the unique elements from the numbers list, resulting in [1, 2, 3, 4, 5].
Implementing IEqualityComparer to Define Custom Equality for Objects in a List
In some cases, you may need to remove duplicates based on specific properties of the objects in the list. To achieve this, you can implement the IEqualityComparer
Here’s an example of how you can implement IEqualityComparer
“`csharp
class Employee
{
public int Id { get; set; }
public string Name { get; set; }
}
class EmployeeEqualityComparer : IEqualityComparer
{
public bool Equals(Employee x, Employee y)
{
return x.Id == y.Id;
}
public int GetHashCode(Employee obj)
{
return obj.Id.GetHashCode();
}
}
List
{
new Employee { Id = 1, Name = “John” },
new Employee { Id = 2, Name = “Jane” },
new Employee { Id = 1, Name = “Jack” } // Duplicate
};
List
“`
In this example, the distinctEmployees list will only contain unique Employee objects, comparing them based on their Id property.
Removing Duplicates Based on Multiple Properties Using LINQ in C#
In scenarios where you want to remove duplicates based on multiple properties, you can leverage the power of LINQ to achieve this efficiently.
Here’s an example of how you can use LINQ to remove duplicates based on multiple properties:
“`csharp
class Student
{
public int RollNumber { get; set; }
public string Name { get; set; }
}
List
{
new Student { RollNumber = 1, Name = “John” },
new Student { RollNumber = 2, Name = “Jane” },
new Student { RollNumber = 2, Name = “Jack” } // Duplicate based on RollNumber
};
List
.Select(g => g.First())
.ToList();
“`
In this example, the distinctStudents list will only contain unique Student objects, considering both the RollNumber and Name properties.
Using HashSet to Efficiently Remove Duplicates from a List in C#
Another efficient way to remove duplicates from a list in C# is by utilizing the HashSet class. A HashSet is a collection that does not allow duplicate elements. By converting a list to a HashSet and then back to a list, you can eliminate the duplicate entries.
Here’s an example of how you can use HashSet to remove duplicates:
“`csharp
List
HashSet
List
“`
In this example, the distinctList will only contain unique elements, resulting in [1, 2, 3, 4, 5].
Using a For Loop and a Separate List to Remove Duplicates from a List in C#
If you prefer a more traditional approach, you can use a for loop and a separate list to iterate through the original list and build a new list, excluding duplicates.
Here’s an example of how you can use a for loop and a separate list to remove duplicates:
“`csharp
List
List
for (int i = 0; i < numbers.Count; i++)
{
if (!distinctNumbers.Contains(numbers[i]))
{
distinctNumbers.Add(numbers[i]);
}
}
```
In this example, the distinctNumbers list will only contain unique elements, resulting in [1, 2, 3, 4, 5].
Performance Considerations When Removing Duplicates from Large Lists in C#
When dealing with large lists, removing duplicates can have a significant impact on performance. Here are a few considerations to keep in mind:
1. Complexity: Be mindful of the time complexity of each method. The Distinct() method has a complexity of O(n), while iterating through a list using a for loop has a complexity of O(n^2) as it compares each element with all the previous elements.
2. Custom Equality: Using IEqualityComparer or LINQ to compare object properties can result in increased execution time, especially if the properties are complex or involve expensive operations. Consider the trade-off between flexibility and performance.
3. HashSet Efficiency: Utilizing the HashSet method can provide better performance when dealing with large lists. However, keep in mind that HashSet uses additional memory.
FAQs
Q: How do I remove duplicates from a list in C# without changing the original order?
A: If preserving the original order is a requirement, you can use an additional list to keep track of the unique elements. When iterating through the original list, check if the element already exists in the new list before adding it.
Q: Can I remove duplicates from a list based on specific conditions?
A: Yes, you can remove duplicates based on specific conditions. You can implement IEqualityComparer
Q: Which method should I use to remove duplicates from a list in C#?
A: The best method depends on your specific requirements. If performance is a priority, HashSet or Distinct() methods are recommended. If you need flexibility in defining custom equality, IEqualityComparer or LINQ can be used.
Conclusion
Removing duplicates from a list in C# plays a crucial role in optimizing performance, ensuring data integrity, and improving accuracy. This article explored various methods to efficiently accomplish this task, including using the Distinct() method, implementing IEqualityComparer, leveraging LINQ, utilizing HashSet, and using a for loop. By applying these methods effectively, you can enhance the efficiency and effectiveness of your C# programs while maintaining accurate and reliable results.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# remove duplicates from a list
Categories: Top 48 C# Remove Duplicates From A List
See more here: nhanvietluanvan.com
Images related to the topic c# remove duplicates from a list
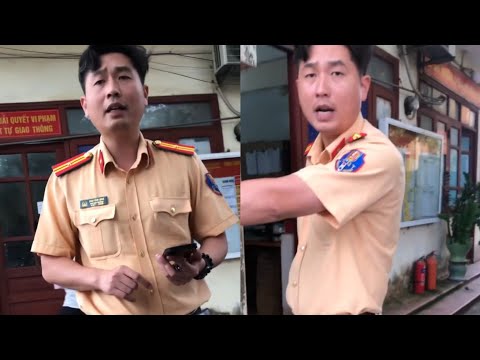
Article link: c# remove duplicates from a list.
Learn more about the topic c# remove duplicates from a list.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Trợ giúp > Mã của các nhãn – Cambridge Dictionary
See more: https://nhanvietluanvan.com/luat-hoc