C# Remove First Item From List
When working with lists in C#, there may be instances where it becomes necessary to remove the first item from the list. Whether it’s for reordering, filtering, or any other specific requirement, C# provides several methods and techniques to achieve this. In this article, we will explore different approaches to remove the first item from a list in C#, including the usage of various methods and techniques.
Methods to Remove the First Item from a List in C#:
1. Using the RemoveAt() Method:
The RemoveAt() method is a built-in method in C# used to remove an item at a specified index from a list. To remove the first item from a list, we can simply call the RemoveAt(0) method, passing 0 as the index parameter. This will remove the item at index 0, which corresponds to the first item in the list.
Example:
“`csharp
List
numbers.RemoveAt(0);
“`
2. Using the RemoveRange() Method:
The RemoveRange() method allows us to remove a range of items from a list in C#. To remove the first item, we can use the RemoveRange(0, 1) method, specifying the starting index as 0 and the count as 1. This will remove the first item from the list.
Example:
“`csharp
List
names.RemoveRange(0, 1);
“`
3. Using the Shift Technique:
The shift technique involves copying the elements of the list starting from the second position to the first position, effectively removing the first item. This can be achieved using a simple loop.
Example:
“`csharp
List
for (int i = 1; i < letters.Count; i++)
{
letters[i - 1] = letters[i];
}
letters.RemoveAt(letters.Count - 1);
```
4. Using the Remove() Method with Index 0:
The Remove() method in C# removes the first occurrence of a specified object from a list. By passing the first item of the list as the parameter to Remove(), we can remove the first item from the list.
Example:
```csharp
List
fruits.Remove(fruits[0]);
“`
5. Using the Take() Method:
The Take() method returns a specified number of elements from the start of a list. By calling Take(1), we can retrieve the first item from the list and remove it using the ToList() method.
Example:
“`csharp
List
numbers = numbers.Take(1).ToList();
“`
6. Using the RemoveFirst() Method from LinkedList:
LinkedList is a data structure in C# that allows efficient insertion and removal of items. The LinkedList
Example:
“`csharp
LinkedList
linkedList.AddFirst(1);
linkedList.AddLast(2);
linkedList.RemoveFirst();
“`
7. Using the Dequeue() Method from Queue:
The Queue
Example:
“`csharp
Queue
queue.Enqueue(“Apple”);
queue.Enqueue(“Banana”);
string removedItem = queue.Dequeue();
“`
C# Remove First Item from List: FAQs
Q: Can I use the methods mentioned above with generic lists?
A: Yes, all the methods mentioned above can be used with generic lists like List
Q: Are these methods applicable to other collection types?
A: Some of the methods, such as RemoveFirst() and Dequeue(), are specific to LinkedList and Queue, respectively. However, the rest of the methods can be used with other collection types that implement the IList
Q: Is there any performance difference between these methods?
A: Yes, there can be performance differences depending on the collection type and the number of elements involved. Methods like RemoveAt() and RemoveRange() require shifting elements, which can be time-consuming for large lists. On the other hand, the LinkedList and Queue methods perform these operations more efficiently.
Q: How should I choose the right method for my use case?
A: The choice of method should depend on your specific requirements and the collection type you are working with. If you value performance and efficiency, LinkedList and Queue methods may be more suitable. However, for general-purpose lists, methods like RemoveAt() and RemoveRange() are commonly used.
Q: Are there any considerations while removing the first item from a list?
A: Yes, when removing the first item from a list, it’s essential to ensure that the list is not empty. Otherwise, an IndexOutOfRangeException or InvalidOperationException may occur. Therefore, you should always check the list’s count or use appropriate exception handling.
In conclusion, removing the first item from a list in C# is a common task with various methods and techniques available. From basic methods like RemoveAt() and RemoveRange() to specialized methods from LinkedList and Queue, there are multiple options to choose from based on your specific requirements. Consider the performance implications and the collection type you are working with to select the most appropriate method for your use case.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# remove first item from list
Categories: Top 38 C# Remove First Item From List
See more here: nhanvietluanvan.com
Images related to the topic c# remove first item from list
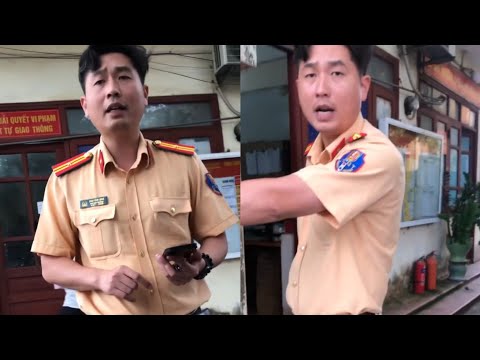
Article link: c# remove first item from list.
Learn more about the topic c# remove first item from list.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Trợ giúp > Mã của các nhãn – Cambridge Dictionary
See more: https://nhanvietluanvan.com/luat-hoc