C# Dictionary Get Value By Key
– Overview of C# Dictionary:
A Dictionary is a generic class in C# that is part of the System.Collections.Generic namespace. It works by associating a key with a value, creating a key-value pair. The key serves as an identifier for the value, allowing for efficient lookup and retrieval.
In C#, a Dictionary can store any type of data as both the key and the value. This flexibility makes it a versatile tool in the hands of developers, as they can easily adapt it to their specific needs.
– Understanding Key-Value Pairs in C# Dictionary:
A key-value pair is the fundamental building block of a C# Dictionary. It consists of a unique key that identifies a particular value. The key must be unique within the Dictionary, meaning that you cannot have two key-value pairs with the same key.
When you add a new key-value pair to a Dictionary, the key is used to determine the position of the value in memory. This allows for fast and efficient retrieval of values using their associated keys.
– Retrieving a Value from a C# Dictionary using the Key:
To retrieve a value from a C# Dictionary, you need to provide the key associated with that value. You can do this by directly accessing the value using the square bracket notation [].
For example, if you have a Dictionary called myDictionary with a key-value pair “name” – “John Smith”, you can retrieve the value “John Smith” by using the following code:
string name = myDictionary[“name”];
If the specified key is not present in the Dictionary, an exception of type System.Collections.Generic.KeyNotFoundException will be thrown. To prevent this exception, you can use the TryGetValue method.
– Using the TryGetValue Method in C# Dictionary:
The TryGetValue method in C# Dictionary provides a safer way to retrieve values from a Dictionary. It allows you to check if a key exists in the Dictionary and retrieve its associated value in a single operation.
The TryGetValue method takes in two parameters: the key you want to search for and an output parameter that will hold the retrieved value. If the key is found, the method returns true and assigns the value to the output parameter. If the key is not found, the method returns false, and the output parameter is set to the default value of the value type.
Here’s an example of using the TryGetValue method:
string name;
if (myDictionary.TryGetValue(“name”, out name))
{
// Key found and value retrieved successfully
Console.WriteLine(“Name: ” + name);
}
else
{
// Key not found
Console.WriteLine(“Key not found”);
}
Using the TryGetValue method helps avoid unnecessary exceptions and allows for smoother error handling when a key is not found in the Dictionary.
– Handling Cases of Missing Keys in C# Dictionary:
As mentioned earlier, if you try to retrieve a value using a key that does not exist in the Dictionary, a KeyNotFoundException will be thrown. To handle this case, you can use the ContainsKey method to check if a key exists in the Dictionary before retrieving its value.
Here’s an example:
if (myDictionary.ContainsKey(“age”))
{
int age = myDictionary[“age”];
Console.WriteLine(“Age: ” + age);
}
else
{
Console.WriteLine(“Key not found”);
}
By using the ContainsKey method, you can conditionally retrieve values from a Dictionary based on the presence of the specified key.
– Improving Performance when Getting Values from a C# Dictionary:
While C# Dictionary provides efficient lookup and retrieval of values by key, there are a few considerations to keep in mind when working with large dictionaries or needing to access values frequently.
One approach to improving performance is by using the TryGetValue method, as mentioned earlier. This method avoids exceptions and provides a more optimized way to retrieve values, especially if the key might not exist in the Dictionary.
Another technique is to use the indexer property of the Dictionary class. By accessing values through the indexer property, you avoid method calls and potentially improve performance.
Finally, if you need to perform multiple key lookups, it might be beneficial to cache the retrieved values in local variables. This can prevent redundant lookups and further enhance performance.
FAQs:
Q: Can I store custom objects as values in a C# Dictionary?
A: Yes, you can store any type of data, including custom objects, as values in a C# Dictionary.
Q: Can I use non-unique keys in a C# Dictionary?
A: No, the keys in a C# Dictionary must be unique. Trying to add a duplicate key will result in an exception.
Q: What happens if I try to retrieve a value using a key that doesn’t exist in the Dictionary?
A: If the key doesn’t exist, a KeyNotFoundException will be thrown, unless you use the TryGetValue method or check for key existence using ContainsKey.
Q: How can I iterate over all the key-value pairs in a C# Dictionary?
A: You can use a foreach loop to iterate over the KeyValuePair collection returned by the Dictionary’s GetEnumerator method.
In conclusion, the C# Dictionary provides an efficient and flexible way to store and retrieve key-value pairs. Retrieving values using keys is straightforward, and developers have options like direct access or using the TryGetValue method for safer retrieval. By considering performance optimizations and handling possible exceptions, developers can fully leverage the power of C# Dictionary in their applications.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# dictionary get value by key
Categories: Top 96 C# Dictionary Get Value By Key
See more here: nhanvietluanvan.com
Images related to the topic c# dictionary get value by key
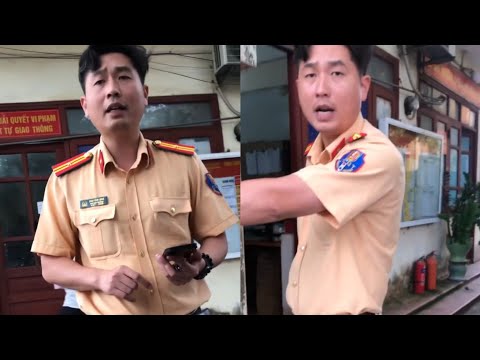
Article link: c# dictionary get value by key.
Learn more about the topic c# dictionary get value by key.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: https://nhanvietluanvan.com/luat-hoc/