C# Dictionary Increment Value
Introduction
C# is a versatile programming language that offers a wide range of data structures to efficiently manage and manipulate data. One such data structure is a dictionary, which is used to store key-value pairs. In this article, we will explore the concept of incrementing the value of a dictionary in C#. We will cover various aspects of working with dictionaries, including creating, adding, accessing, modifying, iterating, checking for key existence, removing key-value pairs, and, most importantly, incrementing the value of a dictionary.
What is a C# Dictionary?
A dictionary in C# is a data structure that stores a collection of key-value pairs. It is also known as an associative array or a hash map. The key is unique and serves as an identifier for the value associated with it. The value can be of any data type, including integers, strings, objects, or even other dictionaries. Dictionaries provide fast lookup times, making them ideal for scenarios that require efficient searching based on a key.
How to Create a Dictionary in C#?
Creating a dictionary in C# is straightforward. You can use the `Dictionary
“`csharp
Dictionary
“`
In the above code, we created an empty dictionary named `personAges` that can store string keys and integer values.
How to Add Key-Value Pairs to a Dictionary?
After creating a dictionary, you can add key-value pairs to it using the `Add()` method or by directly accessing the dictionary’s indexer. Here’s an example of adding key-value pairs to our `personAges` dictionary:
“`csharp
personAges.Add(“John”, 25);
personAges[“Alice”] = 32;
“`
In the first line, we used the `Add()` method to add the key-value pair “John” and 25 to the `personAges` dictionary. In the second line, we directly accessed the indexer of the dictionary and assigned 32 to the key “Alice”.
How to Access Values in a Dictionary?
To access the value associated with a specific key in a dictionary, you can use the indexer or the `TryGetValue()` method. Here are examples of both methods:
“`csharp
int johnsAge = personAges[“John”];
bool found = personAges.TryGetValue(“Alice”, out int alicesAge);
“`
In the first line, we accessed the value of the key “John” using the indexer and stored it in the `johnsAge` variable. In the second line, we used the `TryGetValue()` method to retrieve the value associated with the key “Alice”. The method returns a boolean indicating whether the key was found or not, and the associated value is stored in the `alicesAge` variable.
How to Modify Values in a Dictionary?
You can modify the value associated with a specific key in a dictionary by using the indexer. Here’s an example of changing the age of “John” in our `personAges` dictionary:
“`csharp
personAges[“John”] = 26;
“`
In the above code, we reassigned the value 26 to the key “John” using the indexer.
How to Iterate Over a Dictionary?
To iterate over a dictionary and access all its key-value pairs, you can use a foreach loop. Here’s an example:
“`csharp
foreach (KeyValuePair
{
Console.WriteLine($”Name: {person.Key}, Age: {person.Value}”);
}
“`
In the above code, we used a foreach loop to iterate over the `personAges` dictionary. Each iteration provides a `KeyValuePair
How to Check if a Key Exists in a Dictionary?
To check if a specific key exists in a dictionary, you can use the `ContainsKey()` method. Here’s an example:
“`csharp
bool johnExists = personAges.ContainsKey(“John”);
“`
The above code checks if the key “John” exists in the `personAges` dictionary and returns a boolean indicating the result.
How to Remove Key-Value Pairs from a Dictionary?
To remove a specific key-value pair from a dictionary, you can use the `Remove()` method or the `Clear()` method to remove all entries. Here are examples of both methods:
“`csharp
bool removed = personAges.Remove(“John”);
personAges.Clear();
“`
In the first line, we used the `Remove()` method to remove the key-value pair associated with the key “John”. The method returns a boolean indicating whether the removal was successful or not. In the second line, we used the `Clear()` method to remove all entries from the `personAges` dictionary.
How to Increment the Value of a Dictionary?
Incrementing the value of a dictionary requires a specific approach. Since dictionaries don’t directly expose the value for modification, we need to retrieve the value, modify it, and then set it back to the dictionary. Here’s an example of incrementing the age of “John” in our `personAges` dictionary by 1:
“`csharp
if (personAges.ContainsKey(“John”))
{
personAges[“John”] += 1;
}
“`
In the above code, we first check if the key “John” exists in the `personAges` dictionary. If it does, we access the value using the indexer and increment it by 1. We then set the modified value back to the dictionary.
FAQs
Q: Can a dictionary have duplicate keys?
A: No, a dictionary cannot have duplicate keys. Each key must be unique, and attempting to add a duplicate key will result in an exception.
Q: Can a dictionary store different data types for values?
A: Yes, a dictionary can store values of different data types, including integers, strings, objects, or even other dictionaries. The value type is determined when the dictionary is declared.
Q: How do I increment the value of a dictionary by a specific amount, not just by 1?
A: To increment the value of a dictionary by a specific amount, you can modify the increment statement accordingly. For example, to increment the age of “John” by 5, you can use `personAges[“John”] += 5`.
Q: How can I prevent exceptions when accessing non-existent keys in a dictionary?
A: To prevent exceptions when accessing non-existent keys, you can use the `TryGetValue()` method instead of the indexer. The method returns a boolean indicating whether the key was found or not, eliminating the need for exception handling.
In conclusion, dictionaries are powerful data structures in C# that allow efficient storage and retrieval of key-value pairs. By understanding how to create, add, access, modify, iterate, check for key existence, remove key-value pairs, and increment the value of a dictionary, you can effectively utilize this data structure in your C# projects.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# dictionary increment value
Categories: Top 90 C# Dictionary Increment Value
See more here: nhanvietluanvan.com
Images related to the topic c# dictionary increment value
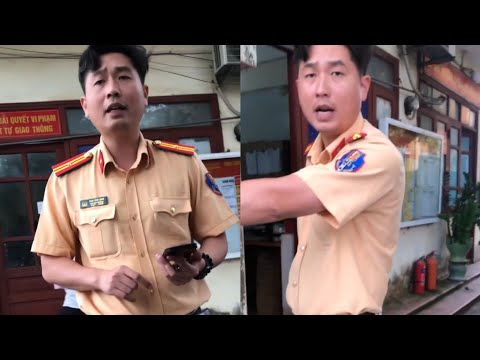
Article link: c# dictionary increment value.
Learn more about the topic c# dictionary increment value.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/