C++ Lambda To Function Pointer
C++ lambda expressions, introduced in C++11, provide a way to define and use anonymous functions. They are particularly useful when we need a small piece of code that we only intend to use once and do not want to write a separate named function for it. Lambdas can be used as function arguments or can be assigned to variables. One interesting use of lambdas is converting them to function pointers.
Lambda Expressions in C++
A C++ lambda expression is a compact way to define an anonymous function. It consists of the lambda introducer `[ ]`, followed by a pair of parentheses `( )`, and then the body of the lambda enclosed in curly braces `{ }`. The body can contain any valid C++ code, including statements and return statements.
Lambda expressions can capture variables, allowing them to access local variables from their enclosing scopes. The capture list, specified in the square brackets `[ ]` before the introducer, defines which variables the lambda will capture. By default, variables are captured by value, but they can also be captured by reference or using a mixture of both.
Syntax of Lambda Expressions
The basic syntax of a lambda expression is as follows:
“`cpp
[ capture list ] ( [ parameter list ] ) -> return_type
{
// code to be executed
}
“`
The capture list is optional. If we do not need to capture any variables, we can omit it. The parameter list is also optional. If the lambda does not take any arguments, we can leave it empty. Finally, the return type can be deduced by the compiler using `auto`, or it can be explicitly specified.
Lambda Expressions vs Function Pointers
Both lambda expressions and function pointers allow us to define and use functions in C++. However, they have some differences. Lambda expressions are more flexible and convenient, as they can capture variables and have a concise syntax. On the other hand, function pointers are more traditional and can only point to named functions or static member functions.
Converting Lambda Expressions to Function Pointers
Converting a lambda expression to a function pointer involves assigning the lambda to a function pointer variable. Here’s an example that demonstrates the process:
“`cpp
int (*add)(int, int) = [](int a, int b) {
return a + b;
};
“`
In this example, we define a function pointer variable `add` that points to a lambda expression. The lambda takes two `int` parameters and returns their sum.
Use Cases and Benefits of Using Lambda Expressions as Function Pointers
Using lambda expressions as function pointers provides several benefits.
Assigning a Lambda to a Function Pointer:
Assigning a lambda to a function pointer allows us to store the lambda in a variable and use it as a regular function pointer. This is particularly useful when dealing with legacy code that expects a function pointer as an argument.
Converting a Lambda to a Function Pointer:
Converting a lambda to a function pointer provides a way to pass the lambda as an argument to functions that only accept function pointers. This allows us to use lambda expressions in scenarios where only function pointers are supported.
Lambda vs Function Pointer:
Lambda expressions are more flexible and can capture variables, while function pointers can only point to named functions or static member functions. The choice between lambda expressions and function pointers depends on the specific requirements of the code and the desired level of flexibility.
Std::function to Function Pointer:
The `std::function` type can be used to wrap lambda expressions and provide a more flexible alternative to function pointers. However, if function pointers are required, the `std::function` can be converted to a function pointer using the `std::function::target` function and then assigning it to a function pointer variable.
Passing a Lambda to a Function in C++:
To pass a lambda as an argument to a function in C++, the function parameter should be declared as accepting a function pointer, such as `void (*func)(int)`. Then, the lambda can be passed directly as an argument when calling the function.
Casting Void to Function Pointer:
To cast a lambda to a function pointer with `void` return type, we can use a `reinterpret_cast`. Here’s an example:
“`cpp
void (*func)() = reinterpret_cast
“`
This allows us to convert a lambda without a return statement to a function pointer with a `void` return type.
Function Pointer in C++:
Function pointers in C++ allow us to store and call functions indirectly, providing a level of flexibility. They can be useful in scenarios where we need to pass a function as an argument, store it in a variable, or dynamically invoke different functions based on certain conditions.
Function Pointer in C++ vs C:
Function pointers in C++ are similar to those in C. However, C++ lambda expressions introduce a more convenient and flexible way to define anonymous functions compared to the traditional function pointer syntax in C.
In conclusion, C++ lambda expressions provide a powerful way to define and use anonymous functions. Converting lambdas to function pointers allows us to use them in scenarios where only function pointers are supported, such as legacy code or functions that expect a function pointer as an argument. By leveraging these features, developers can write more concise and expressive code in C++ while maintaining compatibility with existing codebases.
C++ Weekly – Ep 97 – Lambda To Function Pointer Conversion
Is A Lambda Function A Function Pointer?
Lambda functions have gained popularity in modern programming languages, especially in languages like Python. They are often used as anonymous functions, which means they can be defined and used without a name. While lambda functions share some similarities with function pointers, they are not the same thing.
To understand the difference between a lambda function and a function pointer, it is important to first understand what each of them is and how they work.
What is a lambda function?
A lambda function, also known as an anonymous function, is a function that does not have a name. It is defined using a specific syntax that allows for the creation of small, one-line functions. Lambda functions are commonly used in scenarios where a function is only needed once and does not require a separate definition.
In languages like Python, lambda functions are created using the keyword “lambda” followed by a list of input parameters and a colon, “:”. The body of the function is then specified after the colon. Here is an example of a simple lambda function in Python:
“`python
sum = lambda a, b: a + b
print(sum(5, 10)) # Output: 15
“`
In this example, the lambda function takes two input parameters “a” and “b” and returns their sum. The lambda function is then assigned to the variable “sum” and can be used like a regular function.
What is a function pointer?
A function pointer is a variable that stores the address of a function. It allows for the indirect invocation of a function through the function pointer. Function pointers are commonly used in languages like C and C++ to achieve dynamic function invocations.
In C and C++, function pointers are declared using the function’s declaration syntax with an asterisk (*) preceding the name of the variable. Here is an example of a function pointer declaration in C:
“`c
int (*sum)(int, int);
“`
In this example, the function pointer “sum” is declared to point to a function that takes two integers as parameters and returns an integer. This allows for the dynamic invocation of different functions using the same function pointer variable.
Differences between a lambda function and a function pointer
Despite sharing some similarities, lambda functions and function pointers have fundamental differences:
1. Syntax: Lambda functions use a specific syntax to define anonymous functions, while function pointers use a declaration syntax to store the address of a function.
2. Usage: Lambda functions are often used for small, one-line functions that do not require a separate definition. Function pointers, on the other hand, are used to achieve dynamic function invocations and are commonly used in low-level languages like C and C++.
3. Flexibility: Lambda functions are limited in their capabilities compared to function pointers. Lambda functions are typically used within the context they are defined, while function pointers can be used to invoke different functions at runtime.
FAQs
Q: Can a lambda function be assigned to a function pointer?
A: No, a lambda function cannot be directly assigned to a function pointer. Lambda functions are distinct entities that do not have the same type as a function pointer.
Q: Can lambda functions and function pointers be used interchangeably?
A: No, lambda functions and function pointers have different use cases and cannot be used interchangeably. Lambda functions are used for anonymous functions, while function pointers are used to achieve dynamic function invocations.
Q: Which languages support lambda functions?
A: Many programming languages, such as Python, JavaScript, and Ruby, support lambda functions. However, the syntax for defining and using lambda functions may vary between languages.
Q: Are lambda functions more efficient than regular functions?
A: Lambda functions and regular functions have similar performance characteristics. The efficiency of a lambda function depends on the underlying implementation of the programming language.
In conclusion, while lambda functions and function pointers may seem similar at first glance, they are distinct entities with different purposes. Lambda functions are used for anonymous functions, whereas function pointers are used for dynamic function invocations. Understanding the differences between these concepts is crucial for effectively using them in programming languages that support them.
Is Lambda A Function Pointer In C++?
Lambda expressions are a powerful feature introduced in the C++11 standard that allow for the creation of unnamed functions inline within the code. While lambda expressions may seem similar to function pointers at first glance, they are, in fact, quite different.
What are Lambda Expressions?
Lambda expressions are a concise way to define functions in-place, without the need to write a separate named function. They are often used in situations where a small, one-time function is required, and creating a separate function would be unnecessary and add additional clutter to the code.
Syntax and Benefits of Lambda Expressions
The syntax for lambda expressions in C++ is as follows:
“`
[ capture clause ] (parameters) -> return_type { body }
“`
The capture clause defines which variables from the current scope the lambda expression can access. The parameters section defines the input arguments for the lambda function, just like in regular function declarations. The return_type specifies the type of value that the lambda function returns, and the body contains the actual code to be executed.
One of the key benefits of lambda expressions is their ability to capture variables from the surrounding scope. This allows lambda functions to access and operate on variables that are not explicitly passed as parameters, making them highly adaptable and flexible.
Lambda Expressions vs. Function Pointers
Although lambda expressions and function pointers can be used to achieve similar functionalities, they differ in their implementation and usage. Here are some key differences between the two:
1. Syntax:
Lambda expressions have a unique syntax that clearly differentiates them from function pointers. They use square brackets to specify the capture clause, whereas function pointers do not require any special syntax. This distinction helps improve code readability and maintainability.
2. Type Inference:
Lambda expressions automatically deduce the return type and parameter types based on their usage, whereas function pointers need to have their return type and parameter types explicitly specified.
3. Object-Oriented Nature:
Lambda expressions are objects of a unique, unnamed type generated by the compiler, which allows them to have their own functions and constructors. On the other hand, function pointers are just pointers to named functions and lack these features.
4. Flexibility:
Lambda expressions can capture variables from their enclosing scope, which provides them with greater flexibility compared to function pointers. This means that lambda functions can easily use and modify variables without explicitly passing them as parameters.
5. Inline Definition:
Lambda expressions are defined inline within the code, making them more compact and easier to read compared to function pointers, which require separate function definitions.
FAQs:
Q: Can lambda expressions be assigned to function pointers?
A: No, lambda expressions cannot be directly assigned to function pointers. Lambda expressions have their own unique type generated by the compiler, and they do not have a compatible type with regular function pointers. However, lambda expressions can be converted to function pointers if the lambda does not capture any variables from the enclosing scope.
Q: Can lambda expressions be used as template parameters?
A: Yes, lambda expressions can be used as template parameters in C++. However, since lambda types are unique and unnamed, using them as template parameters can be more complex compared to using regular function pointers.
Q: Are lambda expressions more efficient than function pointers?
A: The efficiency of lambda expressions and function pointers depends on the specific use case. In general, lambda expressions have some additional overhead due to their ability to capture variables and their object-oriented nature. However, modern compilers often optimize lambda expressions effectively, making their performance comparable to that of function pointers in many cases. It is best to measure performance in specific scenarios before drawing conclusions.
Q: Can lambda expressions be used in multi-threaded programming?
A: Yes, lambda expressions can be used effectively in multi-threaded programming. Their ability to capture variables from the enclosing scope makes them particularly useful in implementing parallel algorithms and thread-safe operations.
In conclusion, while lambda expressions in C++ may share some similarities with function pointers, they are distinct entities with unique characteristics. Lambda expressions provide a more flexible, inline way to define functions, allowing for greater adaptability and readability in code. Understanding the differences between lambda expressions and function pointers is essential for effectively utilizing them in C++ programming.
Keywords searched by users: c++ lambda to function pointer Assign lambda to function pointer, Convert lambda to function pointer, Lambda vs function pointer, Std::function to function pointer, Pass lambda to function C++, Cast void to function pointer c++, Function pointer in C, Function pointer C++
Categories: Top 84 C++ Lambda To Function Pointer
See more here: nhanvietluanvan.com
Assign Lambda To Function Pointer
In the world of programming, the C++ language has been a formidable force since its inception. With each iteration, C++ has consistently introduced new features and improvements to make development more efficient and expressive. One particular area where C++ shines is its support for a functional programming paradigm, allowing developers to write code in a declarative and concise way. Among the many features that leverage this paradigm is the ability to assign a lambda expression to a function pointer. This article aims to explore the concept of assigning a lambda expression to a function pointer, delve into the benefits this brings, and provide answers to frequently asked questions that may arise.
Understanding Lambda Expressions in C++
First, let’s establish a foundation by understanding what lambda expressions are in the context of the C++ language. A lambda expression is an unnamed function object that can be used inline. It enables developers to write code that resembles a normal function, but without explicitly defining a separate function. This feature is particularly useful in situations where a function is only needed once or when a concise and straightforward solution is desired.
The syntax for a lambda expression is as follows:
“`cpp
[capture clause] (parameters) -> return_type {
// implementation goes here
}
“`
The capture clause allows the lambda expression to access variables from its surrounding context, either by value or by reference, depending on the developer’s requirements. Parameters, if any, define the inputs the lambda expression expects. The return type specifies the type of value that the lambda expression will produce upon evaluation. Finally, the implementation block contains the code that will be executed when the lambda expression is called.
Assigning a Lambda to a Function Pointer
Now that we have an understanding of lambda expressions, let’s dive into the main topic at hand – assigning a lambda expression to a function pointer in C++.
A function pointer is essentially a variable that points to a function. By assigning a lambda expression to a function pointer, we can treat the lambda expression as if it were a regular function. To assign a lambda to a function pointer, the function pointer should have the same signature as the lambda expression.
Take the following example:
“`cpp
void (*functionPtr)() = []() {
std::cout << "Hello, Lambda!" << std::endl;
};
```
In this case, `functionPtr` is a function pointer that points to the lambda expression. The lambda expression takes no parameters (`()`), and returns void. The body of the lambda expression prints "Hello, Lambda!" to the console.
Benefits of Assigning Lambdas to Function Pointers
Assigning lambdas to function pointers offers several advantages for C++ developers. Here are a few key benefits:
1. Flexibility: By assigning lambdas to function pointers, developers can pass the function pointer as an argument to other functions, making it much easier to pass behavior around.
2. Code Reusability: Since lambdas can be assigned to function pointers, they can be reused in multiple places, reducing code duplication. This promotes the DRY (Don't Repeat Yourself) principle, leading to cleaner and more maintainable code.
3. Simplified Callbacks: Callbacks, a common design pattern in software development, can be seamlessly implemented using a function pointer assigned to a lambda. This allows for dynamic behavior, where the code can execute different functions based on certain conditions or user interactions.
4. Expressive Syntax: Function pointers can have a somewhat archaic syntax, which can make code less readable. By assigning lambdas to function pointers, developers can leverage the more modern and expressive syntax of lambda expressions, resulting in cleaner and more understandable code.
FAQs (Frequently Asked Questions)
Q: Can lambdas be used with function pointers that have parameters?
A: Absolutely! Lambdas can have parameters, just like regular functions. Make sure to match the parameter types and number when assigning a lambda to a function pointer.
Q: Can lambdas be used with function pointers that have a return type?
A: Yes, lambdas can have return types. Ensure that the return type of the lambda expression matches the return type of the function pointer.
Q: Can I modify the lambda expression assigned to a function pointer after assignment?
A: Yes, function pointers can be reassigned to different lambda expressions at runtime, allowing flexibility in changing behavior dynamically.
Q: What happens if I assign a lambda expression with a different signature to a function pointer?
A: Assigning a lambda expression with a different signature to a function pointer will result in a compilation error, as the types do not match.
Q: Are there any performance implications when using lambdas with function pointers compared to regular functions?
A: In general, there shouldn't be significant performance differences between lambdas assigned to function pointers and regular functions. However, like any code, profiling and benchmarking specific scenarios is always recommended.
In conclusion, C++'s support for assigning lambdas to function pointers offers a powerful tool for developers to write more expressive and flexible code. By leveraging lambda expressions, developers can simplify callbacks, reuse code, and benefit from the concise syntax provided by lambda expressions. Understanding how to assign a lambda to a function pointer opens up a world of possibilities in leveraging C++'s functional programming paradigm and pushing the boundaries of code elegance and maintainability.
Convert Lambda To Function Pointer
Lambda functions, a powerful feature introduced in C++11, allow programmers to define anonymous functions in a concise and intuitive manner. They provide a convenient way to write functional programming style code, especially when dealing with algorithms, predicates, or callbacks. However, there may be situations where we need to convert a lambda function into a function pointer, either due to compatibility requirements or to interface with legacy code. In this article, we will explore the mechanism of converting lambdas to function pointers in C++ and delve into the intricacies involved in this process.
Understanding Lambda Functions
Before diving into the conversion, let’s briefly recap the fundamentals of lambda functions. A lambda function is essentially a shortcut for defining small, anonymous functions in C++. They consist of a lambda introducer, parameter list, mutable specification, exception specification, and a lambda body.
The following is a basic example of a lambda function:
“`cpp
auto addition = [](int a, int b) { return a + b; };
“`
Here, `addition` is a lambda function that takes two integers as parameters and returns their sum. The `[]` inside the square brackets, known as the capture clause, captures external variables for use within the lambda function.
Converting Lambda to Function Pointer
Now let’s move on to the main focus of this article: converting a lambda function into a function pointer. Although this process might seem straightforward, it requires some careful consideration due to the different nature of these two entities.
To convert a lambda function to a function pointer, we need to follow a few steps. First, we need to explicitly specify the signature of the lambda function. The signature comprises the return type and the parameter types. This is crucial because function pointers rely on knowing the exact types of the functions they are pointing to.
Once the signature is determined, we can define a function pointer with the matching signature. In C++, function pointers have a specific syntax, where the name of the pointer is followed by the parameters within parentheses. For example:
“`cpp
int (*fp)(int, int);
“`
The `fp` in the above example is a function pointer that points to a function taking two integers as parameters and returning an integer.
To convert our lambda function `addition` to a function pointer, we simply assign it to the function pointer variable `fp` with the same signature:
“`cpp
int (*fp)(int, int) = addition;
“`
Now we have successfully converted the lambda function `addition` into a function pointer!
FAQs:
Q1: Can all lambda functions be converted to function pointers?
A: Unfortunately, no. Only stateless lambda functions, i.e., those that do not capture any external variables, can be converted to function pointers. Lambdas that capture variables have an implicit closure object associated with them, making them incompatible with function pointers.
Q2: What about lambda functions with captures? Is there any workaround for converting them to function pointers?
A: Yes, there is a workaround. By encapsulating the lambda into a regular function template, we can define the lambda function’s signature explicitly within the template parameters. This enables us to convert the lambda function to a function pointer. However, this approach is less flexible and less concise compared to capturing-free lambdas.
Q3: What are some practical use cases for converting lambda functions to function pointers?
A: One common use case is when interfacing with legacy code that expects function pointers as arguments. By converting the lambda function to a function pointer, we can seamlessly integrate our modern code with older systems or libraries. Additionally, some C libraries or APIs may have support for function pointers only, necessitating the conversion for compatibility reasons.
Q4: Are there any performance implications in converting lambdas to function pointers?
A: Converting lambdas to function pointers does not introduce any significant performance overhead. The conversion process itself is usually optimized by the compiler, resulting in similar execution times for equivalent lambda and function pointer versions.
In conclusion, converting lambda functions to function pointers provides a way to bridge the gap between modern C++ and legacy code or APIs that rely on function pointers. While the process is relatively straightforward, it is important to recognize the limitations imposed by captures in lambda functions. By understanding the mechanism behind this conversion, developers can successfully leverage the strengths of lambdas while ensuring seamless integration with existing systems or libraries.
Lambda Vs Function Pointer
In the realm of programming, developers often encounter various constructs that allow the manipulation of functions. Two widely used constructs are lambdas and function pointers. While they share similarities in some respects, they differ significantly in others. In this article, we will delve into the differences between these two constructs, exploring their characteristics, use cases, and benefits. So, let’s get started!
Understanding Lambda Functions
A lambda function, also known as an anonymous function, is a feature introduced in modern programming languages to encapsulate and pass around pieces of code as objects. Lambda functions can be defined inline and can capture variables from their surrounding environment.
One of the primary advantages of lambda functions is their conciseness. They eliminate the need for named function declarations for simple operations, making code more streamlined and readable. By creating functions inline, developers can efficiently define functions where they are needed, reducing clutter in the overall codebase.
Lambda functions can be assigned to variables, stored in data structures, or passed as parameters to other functions. Their ability to capture variables from the surrounding scope makes them versatile and powerful. They can access not only local variables but also global variables and parameters from the enclosing function.
Understanding Function Pointers
Function pointers, on the other hand, are a mechanism that allows functions to be treated as first-class citizens, just like variables or objects. A function pointer holds the memory address of a function, enabling the program to call the referenced function at runtime.
Unlike lambdas, function pointers are not anonymous. They require explicit declaration and assignment to a particular function. This adds a layer of indirection, making the syntax slightly more elaborate than that of lambdas. However, this also allows function pointers to be more flexible in their usage.
Function pointers are commonly used in lower-level languages and systems programming. They enable dynamic dispatching, allowing functions to be selected and invoked dynamically based on runtime conditions. This capability proves especially beneficial in scenarios where the choice of function needs to be determined programmatically.
Comparing Lambda Functions and Function Pointers
Now that we have a good understanding of the basics, let us compare the characteristics of lambda functions and function pointers:
1. Flexibility: Lambda functions shine in scenarios requiring concise and straightforward operations. They excel at providing a lightweight way to create and manipulate functions inline. Function pointers, on the other hand, offer more flexibility because they can point to any function with compatible signature, providing opportunities for dynamic dispatching and polymorphic behavior.
2. Capture Mechanism: Lambdas have the unique ability to capture variables from their enclosing scope. This feature enables them to carry state and makes them suitable for scenarios requiring callbacks with data context. Function pointers, on the other hand, do not have a built-in capture mechanism. They only allow the passing of a function reference without any additional context.
3. Syntax Complexity: Lambda functions have a simpler and more concise syntax compared to function pointers. Their inline nature eliminates the need for explicit declaration and assignment, resulting in more readable code. While function pointers require more verbose syntax, they offer finer control by allowing explicit assignment and declaration.
4. Portability and Language Support: The availability of lambda functions and function pointers varies among programming languages. While lambda functions are supported in languages like Python, C++, and Java (starting from Java 8), function pointers are commonly found in lower-level languages like C and C++. When developing code that requires cross-language compatibility, it is crucial to carefully consider which construct to use.
Use Cases for Lambda Functions and Function Pointers
Lambda functions and function pointers find their utility in different scenarios within programming:
Lambda Function Use Cases:
– Callbacks: Lambdas are ideal for defining callback functions that encapsulate simple operations. They can be used in event-driven programming to handle events or asynchronous operations.
– List and Stream Manipulation: Lambda functions offer an expressive way to manipulate lists and streams. They can be used to filter, transform, or reduce collections of data, enhancing code readability and maintainability.
Function Pointer Use Cases:
– Polymorphism: Function pointers are commonly used in object-oriented programming to implement polymorphism. They enable selecting and invoking different methods based on the dynamic type of an object.
– Dynamic Library Loading: Function pointers allow dynamic library loading and binding at runtime, enabling systems to load and use external libraries on-demand.
FAQs
Q1. Can lambda functions be used as function pointers?
A1. Conceptually, lambda functions capture variables from their enclosing scope and are not directly compatible with function pointers. However, some programming languages provide ways to convert lambda functions to function pointers, allowing them to be used in function pointer-based APIs.
Q2. How are lambdas and function pointers implemented at the bytecode level?
A2. The implementation details vary across programming languages and compilers. However, in many cases, lambdas are transformed into function objects or closures capturing their context, while function pointers are represented as raw memory addresses or pointers to executable code.
Q3. Which construct is more performant: lambdas or function pointers?
A3. The performance characteristics depend on the programming language, compiler optimizations, and the specific use case. In general, function pointers may have a slight edge in performance due to their direct invocation mechanism. However, the actual impact on performance is highly influenced by the surrounding code and the efficiency of the underlying compiler or runtime system.
In conclusion, both lambda functions and function pointers offer unique advantages and serve different purposes in programming. Lambda functions excel at providing concise and inline function definitions, enabling straightforward operations and callbacks. Function pointers, on the other hand, offer flexibility and fine control, allowing dynamic dispatching and polymorphism. Choosing between them depends on the specific requirements of your programming task, the language you are using, and the level of control and dynamism needed.
Images related to the topic c++ lambda to function pointer
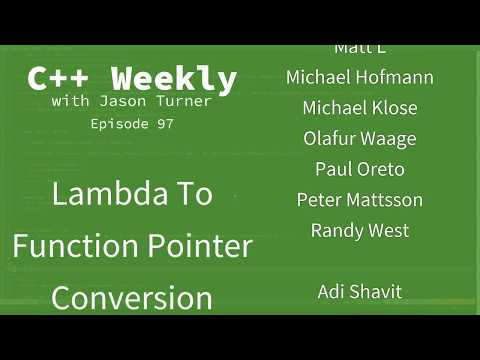
Found 50 images related to c++ lambda to function pointer theme
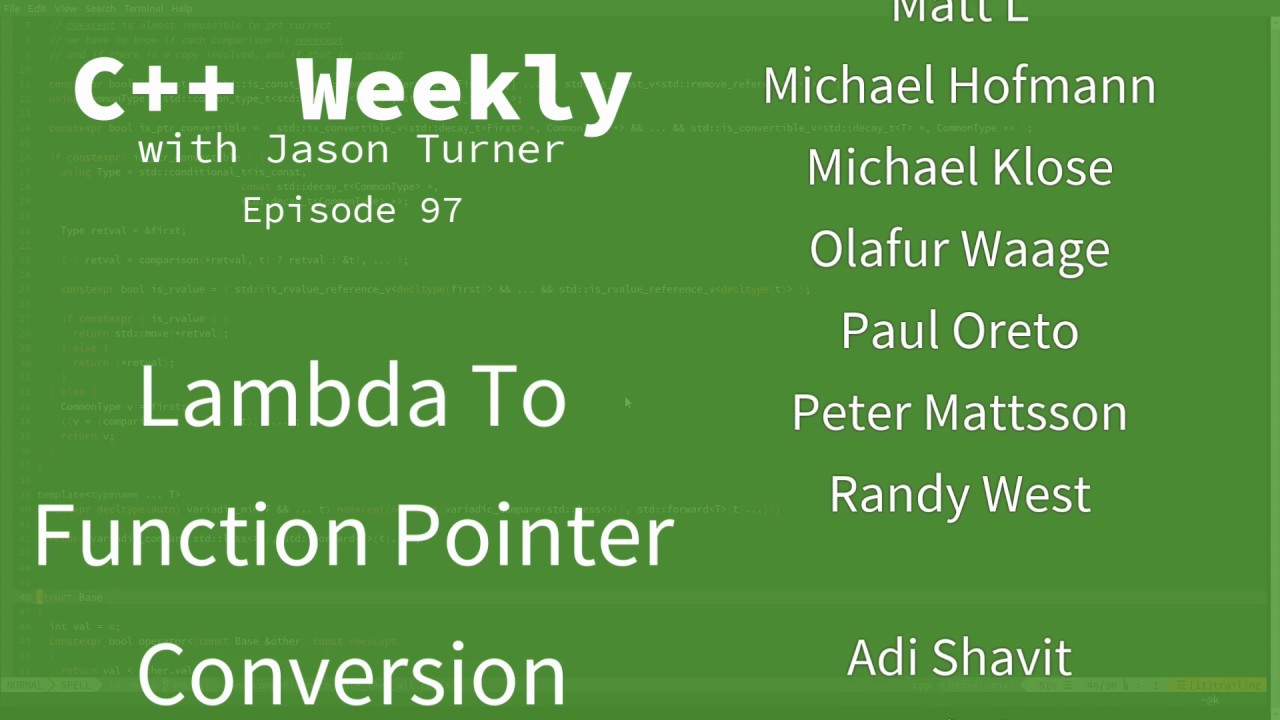



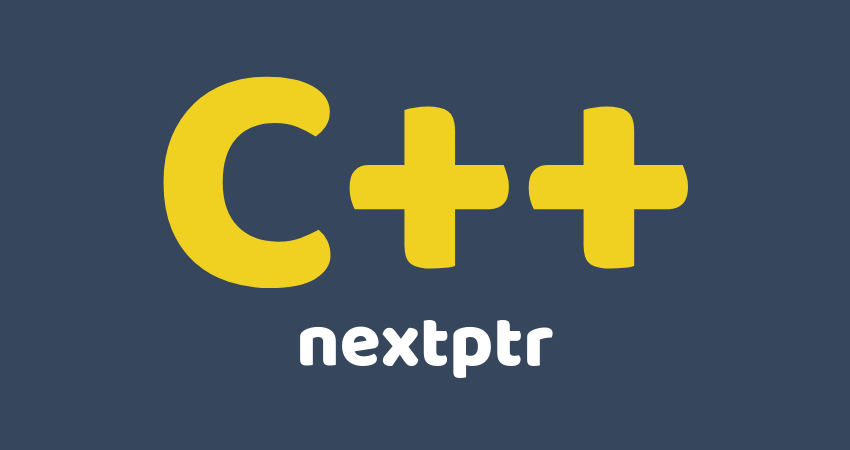




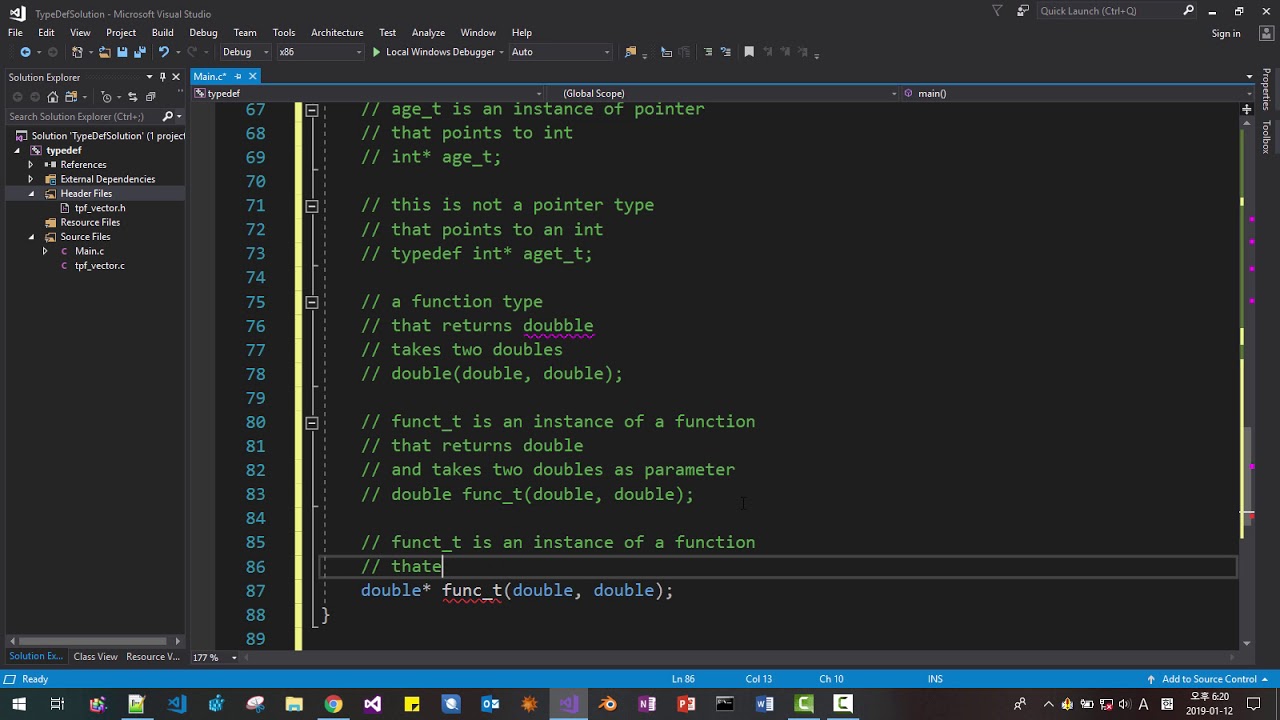



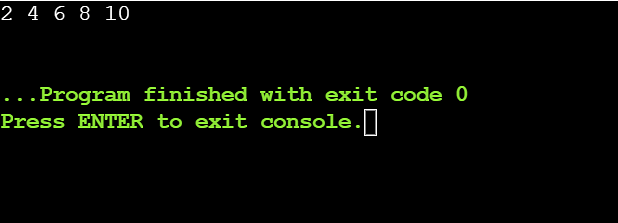




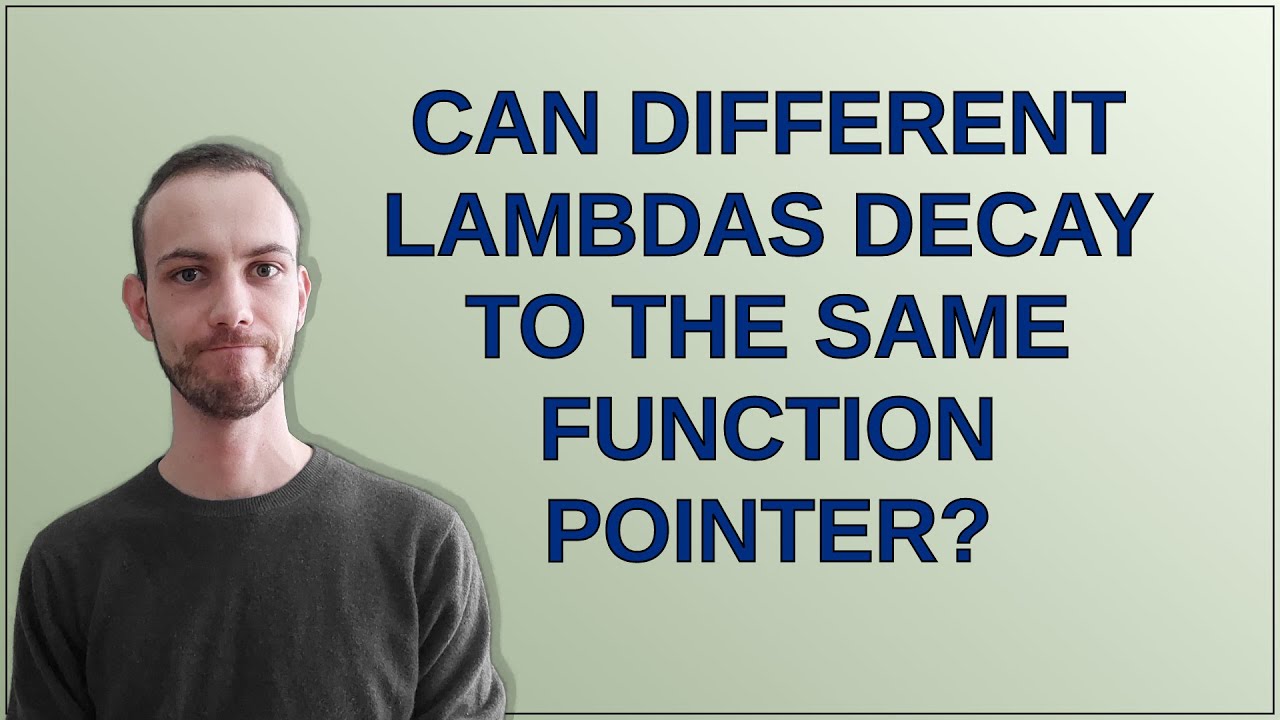
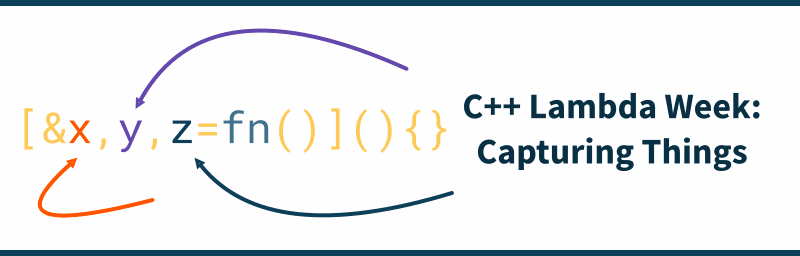

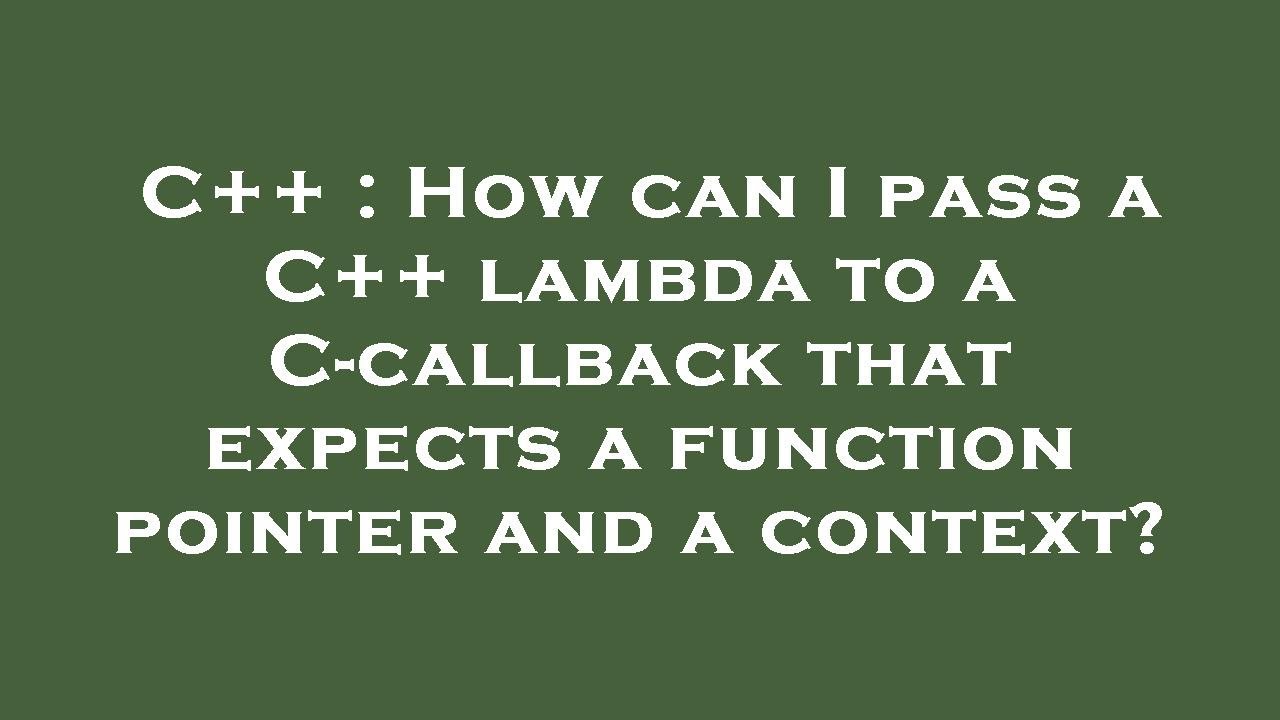
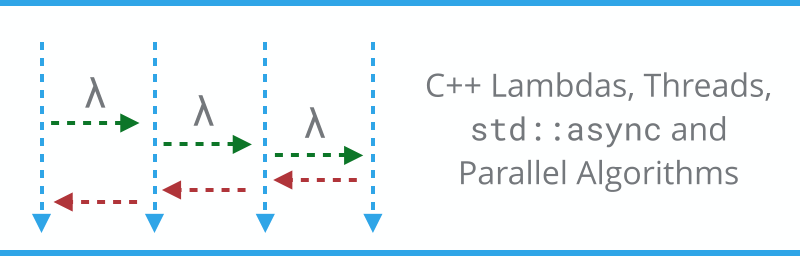
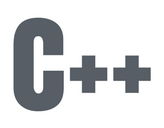



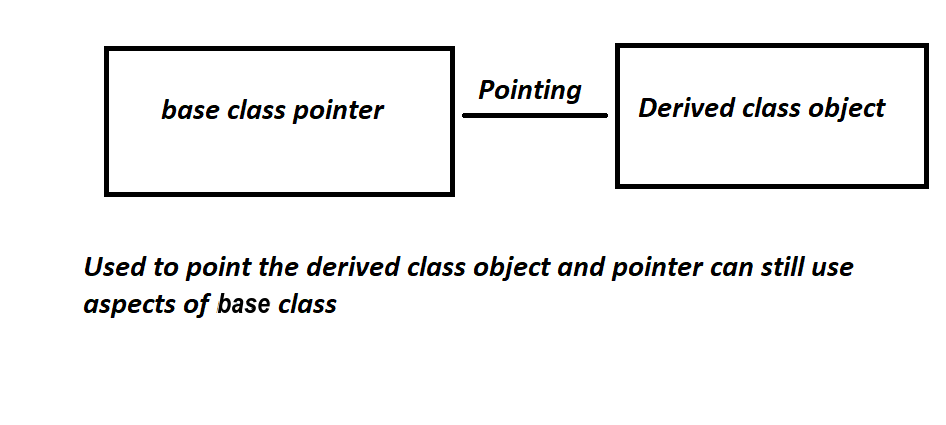
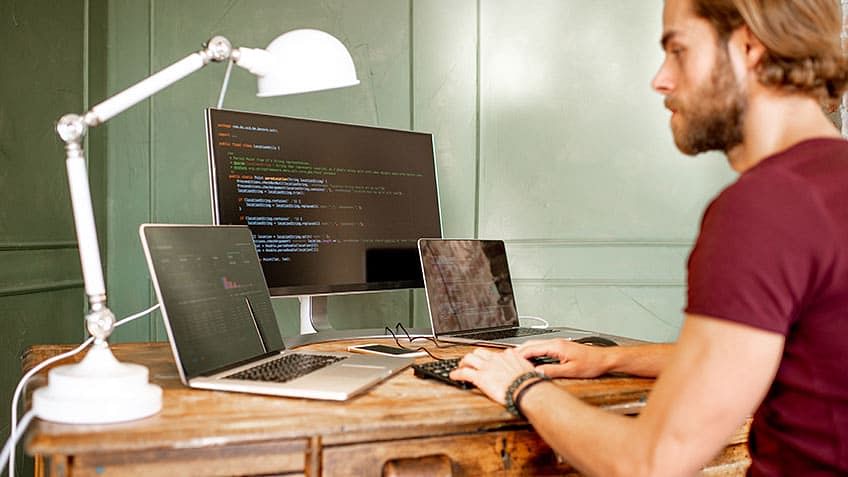

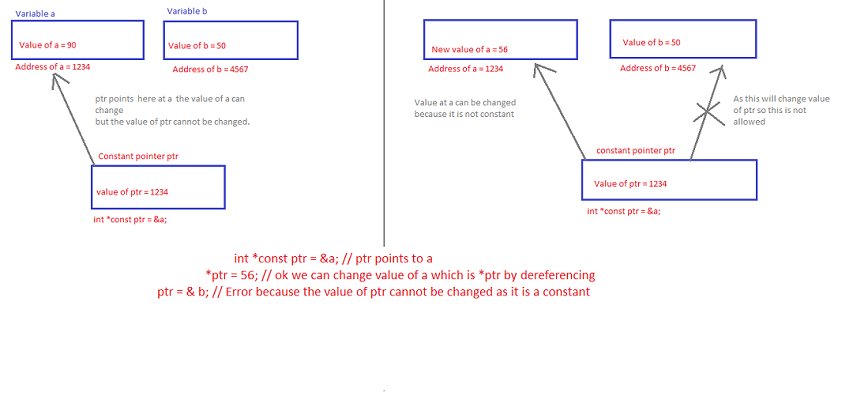
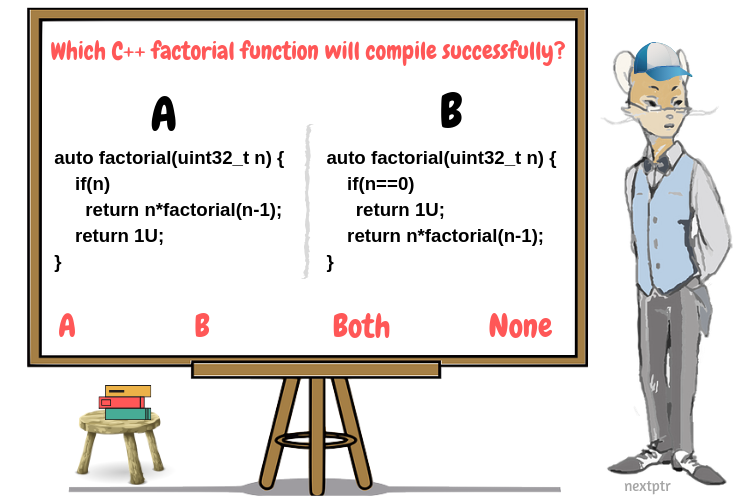


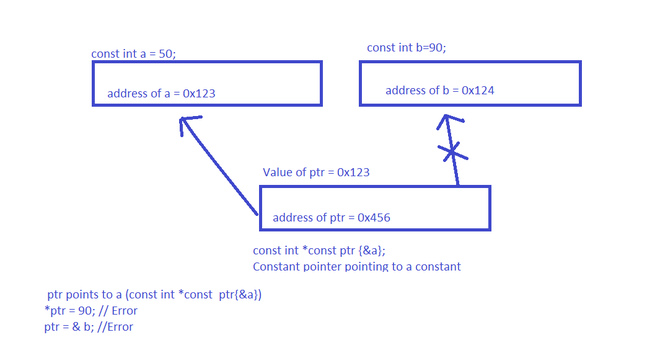


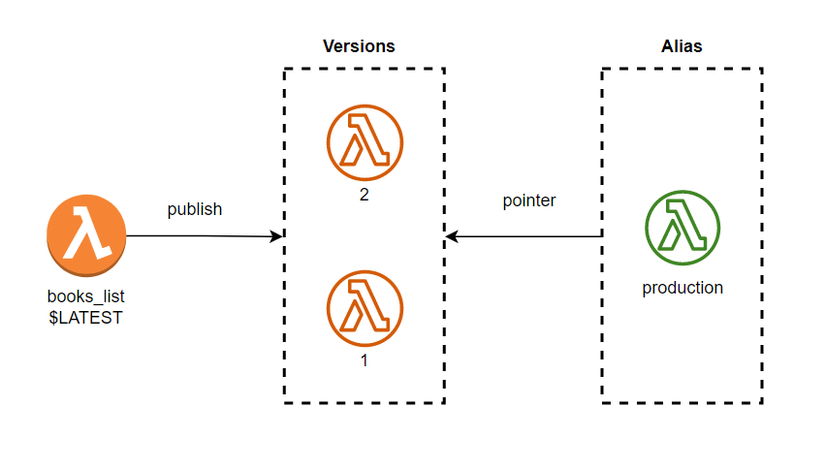
![Difference between int (*p)[3] and int* p[3]? - GeeksforGeeks Difference Between Int (*P)[3] And Int* P[3]? - Geeksforgeeks](https://media.geeksforgeeks.org/wp-content/uploads/20201123001107/gfg2.jpg)
Article link: c++ lambda to function pointer.
Learn more about the topic c++ lambda to function pointer.
- Passing capturing lambda as function pointer – Stack Overflow
- Passing C++ captureless lambda as function pointer to C API
- Passing C++ lambdas to C-function pointer callbacks
- Lambda Expressions vs Function Pointers – GeeksforGeeks
- C++ Tutorial => Conversion to function pointer
- Function Pointers, Functors, and Lamda Functions
- How To Bind Lambda To Function Pointer – Adroit Things
- convert lambda to member function pointer – Google Groups
- Passing capturing lambda as function pointer – Stack Overflow
- Passing C++ captureless lambda as function pointer to C API
- How to declare a pointer to a function in C – Tutorialspoint
- Significance of Lambda Function in C/C++ – Tutorialspoint
- Closure, Lambda, Functor trong C++ [Part 2] – function – Viblo
See more: https://nhanvietluanvan.com/luat-hoc