Char Cannot Be Dereferenced
The char data type in Java is used to represent a single character. It is a 16-bit unsigned integer that can store values ranging from 0 to 65,535. It is important in handling characters and Unicode encoding, as it can represent various characters from different writing systems and languages.
In Java, characters are represented using the Unicode encoding scheme, which assigns a unique numeric value to each character. This allows for internationalization and support for different character sets.
Dereferencing in Java
Dereferencing is a concept in programming that allows access to the object or value that a reference variable points to. It is commonly used with objects and arrays to access their properties or elements.
When a reference variable is dereferenced, the dot operator (.) is used to access the methods and fields of the object or array. This allows for manipulation and retrieval of the data stored within the object or array.
Dereferencing is essential for accessing the behavior and data of objects, enabling programmers to work with complex data structures and perform operations on them.
Limitations of Dereferencing
In Java, not all types can be dereferenced. Only reference types, such as objects and arrays, can be dereferenced. Primitive types, like int, boolean, and char, cannot be dereferenced.
This limitation exists because primitive types do not have methods or fields associated with them. They are simple data types that hold values directly.
Primitive types are designed to be lightweight and efficient, without the need for complex data structures or behavior. This limitation ensures efficient memory usage and avoids unnecessary overhead associated with dereferencing.
Why char cannot be Dereferenced
The char data type in Java is a primitive type, which means it cannot be dereferenced. Unlike objects and arrays, char does not have any associated methods or fields that can be accessed through dereferencing.
Attempting to dereference a char variable will result in a compilation error, stating that “char cannot be dereferenced.” This error serves as a reminder that char is a simple value type and should not be treated as an object.
Comparison with other numeric primitive data types
Other numeric primitive data types in Java, such as int, double, and float, also cannot be dereferenced. They share the same limitations as char in terms of not having methods or fields associated with them.
These data types are designed to hold simple numeric values and perform arithmetic operations efficiently. They do not require the overhead of object-like behavior, making them more lightweight and suitable for basic numeric tasks.
Alternative Approaches
When char dereferencing is necessary, there are alternative approaches that can be used to achieve the expected results.
One approach is to convert the char value to a Character object, which can then be dereferenced. This can be done using the Character class’s static methods, such as valueOf(char) or new Character(char).
Another approach is to convert the char value to a String, which can be dereferenced as it is an object. This can be done using the String.valueOf(char) method or by concatenating the char with an empty string (“”) to implicitly convert it to a String.
Handling char Values in Java
When working with char values in Java, it is essential to follow best practices and strategies to ensure reliable, readable, and maintainable code.
Some techniques for handling char variables include performing operations such as concatenation, comparison, and transformation. These operations can be accomplished using the appropriate methods and operators available in Java.
For example, to check if a char is a number, the isDigit(char) method from the Character class can be used. To compare two char values while ignoring case, the toLowerCase(char) method can be applied before the comparison.
It is crucial to stay on topic and cover the subject of char dereferencing in-depth, addressing the common errors and providing practical examples that illustrate the proper handling of char values.
FAQs:
Q: What does the error “char cannot be dereferenced” mean?
A: This error occurs when attempting to access methods or fields of a char variable using the dot operator (.), which is used for dereferencing objects. Since char is a primitive type, it does not have any associated methods or fields to be accessed.
Q: Can I convert a char to a Character object to enable dereferencing?
A: Yes, converting a char to a Character object allows for dereferencing. This can be done using the Character class’s static methods, such as valueOf(char) or new Character(char).
Q: How can I check if a char is a number?
A: You can use the isDigit(char) method from the Character class to check if a char is a number. It returns true if the char is a digit, and false otherwise.
Q: How can I compare two char values while ignoring case?
A: To compare char values while ignoring case, you can convert them to lowercase using the toLowerCase(char) method before performing the comparison.
Q: Can I convert a char to a String to enable dereferencing?
A: Yes, you can convert a char to a String and then dereference the String object. This can be done using the String.valueOf(char) method or by concatenating the char with an empty string (“”).
Q: Are there any alternatives to char dereferencing in Java?
A: Yes, when char dereferencing is not possible, alternative approaches such as converting the char to a Character object or a String can be used to achieve similar results.
Error: Cannot Be Dereferenced (Java Tutorial)
How To Solve Char Cannot Be Dereferenced In Java?
Java is a popular programming language known for its simplicity and versatility. However, like any programming language, it has its quirks and challenges. One common issue that both novice and experienced Java programmers may encounter is the “char cannot be dereferenced” error. In this article, we will delve into the causes of this error and provide effective solutions to resolve it.
Understanding the Error: What does “char cannot be dereferenced” mean?
To grasp the meaning of the error message, it is crucial to understand what dereferencing means. In Java, variables can refer to objects, and when we dereference a variable, it means accessing the properties or methods of that object.
However, a “char” is a primitive data type in Java, which means it is not an object. Primitive data types, such as int, double, boolean, and char, do not have any methods or properties associated with them. Thus, attempting to dereference a char variable will result in this particular error message.
Common Causes of the Error
The “char cannot be dereferenced” error typically occurs due to one of these three scenarios:
1. Attempting to invoke a method on a char:
char letter = ‘A’;
int length = letter.length(); // This line will cause an error
Here, the “.length()” method, being an object method, cannot be used with primitive data types like char.
2. Comparing a char using the “==” operator:
char firstLetter = ‘A’;
char secondLetter = ‘B’;
if(firstLetter == secondLetter) {
// Some code here
}
In this scenario, the “==” operator can only be used to compare value equality between primitive data types, like char. However, it cannot be used to compare references of objects.
3. Attempting to invoke a String method on a char:
char letter = ‘A’;
String strLetter = letter.toLowerCase(); // This line will cause an error
Here, attempting to call the “toLowerCase()” method on the char variable will result in an error because the method belongs to the String class, not the char data type.
Resolving the Error
Now that we understand the root causes of the “char cannot be dereferenced” error, let’s explore the solutions to fix it.
1. Convert the char to a String:
char letter = ‘A’;
String strLetter = Character.toString(letter);
int length = strLetter.length(); // No error here
By converting the char to a String using the “Character.toString()” method, we can bypass the error. Now, the “.length()” method can be used on the String variable.
2. Compare chars using the “equals()” method:
char firstLetter = ‘A’;
char secondLetter = ‘B’;
if(Character.valueOf(firstLetter).equals(secondLetter)) {
// Some code here
}
Since chars cannot be compared directly using the “==” operator, we can use the “Character.valueOf()” method to convert chars into Character objects. Then, the “equals()” method can be used to compare the two Character objects.
Frequently Asked Questions (FAQs)
Q1. Can I use the “char” data type with object methods in Java?
No, the “char” data type is a primitive data type and does not have any object methods associated with it. Thus, you cannot use methods like “.length()” directly on a char variable.
Q2. Can the “==” operator be used to compare two char variables in Java?
Yes, the “==” operator can be used to compare two char variables for value equality. However, it cannot be used to compare their references.
Q3. How can I convert a char to a String in Java?
You can convert a char to a String by using the “Character.toString()” method or by concatenating the char with an empty String.
In conclusion, the “char cannot be dereferenced” error is an issue that may occur when trying to invoke methods or operations that are not supported directly by the char data type. By understanding the causes and applying the appropriate solutions, you can overcome this error and continue with your Java programming tasks smoothly.
What Does It Mean Char Cannot Be Dereferenced?
In Java, a primitive data type called char represents a single character. It is used to store and manipulate characters such as alphabets, digits, symbols, or even escape sequences. However, when dealing with characters in Java, you may encounter an error stating, “char cannot be dereferenced.” This error occurs when you try to invoke a method or access a member on a char variable as if it were an object.
To fully understand why a char cannot be dereferenced, it is important to understand the difference between primitive types and objects in Java. A primitive type, such as char, is a simple data type that does not have any methods or attributes associated with it. On the other hand, objects are instances of classes and can have methods and attributes.
When you try to dereference a variable, it means you are trying to access the methods or attributes of an object. In Java, you can only dereference objects, not primitive types. So, when you attempt to dereference a char variable, the compiler throws an error since it is not an object.
To illustrate this, let’s consider an example:
“`java
char myChar = ‘A’;
System.out.println(myChar.toString());
“`
In this example, we have a char variable named myChar assigned the value ‘A’. However, when we try to invoke the toString() method on myChar, we get a “char cannot be dereferenced” error. This is because char does not have a toString() method as it is a primitive type.
To fix this error, we need to convert the char to its corresponding wrapper class, Character. Wrapper classes in Java provide a way to treat primitive types as objects by wrapping them around objects. To convert a char to a Character object, you can use the static method valueOf():
“`java
char myChar = ‘A’;
Character myCharacter = Character.valueOf(myChar);
System.out.println(myCharacter.toString());
“`
By using the Character.valueOf() method, we convert the primitive char to a Character object, allowing us to call the toString() method without any errors.
Frequently Asked Questions (FAQs):
Q1. Can I directly use methods on characters without converting them to objects?
No, you cannot directly use methods on characters since they are primitive types. To use methods such as toString(), you need to convert them to objects using their corresponding wrapper class.
Q2. Why are characters treated differently than other primitive types?
Characters are treated differently because they represent single symbols rather than numeric values. Therefore, Java provides additional methods and functionalities for working with characters by allowing them to be treated as objects.
Q3. Is it possible to dereference other primitive types in Java?
No, it is not possible to dereference other primitive types in Java. Only objects, which include wrapper classes for primitive types, can be dereferenced.
Q4. How can I check the properties of a character, such as its Unicode value?
To access properties like the Unicode value of a character, you can utilize the methods provided by the Character wrapper class. For example, the method Character.getNumericValue(char) returns the Unicode value of a character.
Q5. Are there any performance considerations when converting characters to objects?
Converting characters to objects incurs a memory and performance overhead since objects require additional memory compared to primitive types. Therefore, it is advised to only convert to objects when necessary and to avoid unnecessary conversions in performance-critical scenarios.
In conclusion, the error “char cannot be dereferenced” occurs when trying to invoke methods or access attributes on a char variable, as it is a primitive type. To overcome this error, you need to convert the char to a Character object using the corresponding wrapper class. Understanding the distinction between primitive types and objects is crucial to avoiding this error and correctly working with characters in Java.
Keywords searched by users: char cannot be dereferenced Char cannot be dereferenced, type `char` cannot be dereferenced rust, Int cannot be dereferenced, Check char is number java, Equal char Java, String to char Java, Convert char to Character Java, Compare char ignore case java
Categories: Top 21 Char Cannot Be Dereferenced
See more here: nhanvietluanvan.com
Char Cannot Be Dereferenced
In programming, the concept of dereferencing is crucial. It refers to accessing the value stored at a specific memory address, usually through a pointer. However, in Java, one common error faced by programmers is the message “char cannot be dereferenced.” This error is related to the attempt to invoke a method or retrieve a field from a char variable. In this article, we will explore char variables in Java, explain why they cannot be dereferenced, and provide answers to frequently asked questions regarding this issue.
Understanding Char Variables:
In Java, each character is represented by the primitive data type called char. Char variables can store a single character and are declared using the keyword ‘char’. For example, ‘char myChar = ‘A” would declare a char variable named ‘myChar’ and assign it the value ‘A’.
Char Cannot Be Dereferenced:
The error message “char cannot be dereferenced” occurs when we try to treat a char variable as an object that can have methods or fields. In Java, primitive data types like char, int, boolean, etc., are not objects. They lack the behaviors and properties associated with objects in Java. Consequently, attempting to call methods or retrieve fields from a char variable results in a compile-time error.
The Reasoning Behind the Error:
Java provides a set of wrapper classes such as Character, Integer, Boolean, etc., which allow primitive data types to be treated as objects with methods and fields. These wrappers are automatically used when necessary, such as converting a char primitive to a Character object. However, directly calling methods on a char variable is not supported, as they are not wrapped by default.
Example of the Error:
To better understand the error, let’s consider an example:
“`java
char myChar = ‘A’;
int length = myChar.length(); // Produces an error: “char cannot be dereferenced.”
“`
Here, the attempt to invoke the `length()` method on a char variable results in the mentioned error. The char data type does not have the method `length()`, thus causing the program to fail at compile-time.
Workarounds:
To overcome the “char cannot be dereferenced” error, there are a few workarounds to consider:
1. Convert the char to a String: Since String objects have useful methods, converting the char to a String can provide the ability to perform operations that would otherwise be unavailable to char variables. For instance, in our previous example, we can solve the error by modifying the code as follows:
“`java
char myChar = ‘A’;
String myString = Character.toString(myChar);
int length = myString.length(); // Returns the length of the string, which is 1.
“`
By converting the char to a String using `Character.toString()`, we can now invoke the `length()` method on the resultant String object.
2. Use Character Wrapper class methods: Java provides several methods in the Character wrapper class that can operate on char primitive values. Some commonly used methods are `isDigit(char)`, `isLetter(char)`, and `toLowerCase(char)`. Leveraging these methods can allow us to perform various operations on char values without encountering the dereferencing error.
FAQs:
Q: What does the error message “char cannot be dereferenced” mean?
A: This error message typically occurs when trying to access fields or invoke methods on a char variable. It indicates that char values, being primitive data types, do not possess the characteristics of objects in Java and therefore lack associated methods.
Q: Can I change a char variable into an object?
A: Although char variables are not objects themselves, you can convert them into objects by using wrapper classes such as Character. This allows you to access methods and other functionalities that are only available for objects.
Q: Is there any difference between a char and a Character object?
A: Yes, there is. char is a primitive data type, while Character is a wrapper class that allows char to be treated as an object. The Character class provides various methods for working with char values, unlike the char primitive data type itself.
In summary, the error “char cannot be dereferenced” occurs when attempting to invoke methods or access fields from char variables. This error is due to the fact that char is a primitive data type in Java, lacking object-like behaviors. By understanding the limitations of char variables and employing workarounds such as converting them to String or using Character wrapper class methods, programmers can overcome this issue and achieve the desired results in their Java programs.
Type `Char` Cannot Be Dereferenced Rust
Introduction:
Rust is a modern systems programming language that guarantees memory safety without sacrificing performance. As a strongly typed language, it ensures that operations are done correctly and safely. However, one limitation to keep in mind is that the type `char` cannot be dereferenced. In this article, we will delve into the reasons behind this limitation, explore possible workarounds, and address common FAQs related to this topic.
Understanding the Limitation:
In Rust, when we want to access the data pointed to by a reference, we use the dereference operator `*`. However, Rust does not allow dereferencing `char` values and throws a compilation error on such attempts. This limitation arises from Rust’s philosophy of preventing any potentially unsafe operations.
The reason behind `char` being undereferencable is that a `char` in Rust represents a Unicode scalar value and can occupy multiple bytes in memory. Dereferencing a `char` value without considering this encoding complexity could lead to incorrect memory access and unsafe behavior. To ensure type safety and memory correctness, Rust forces programmers to handle `char` manipulations explicitly.
Workarounds and Alternative Approaches:
1. Iterating Over a String:
While direct dereferencing is not possible, Rust offers efficient alternatives to access and manipulate `char` values. For instance, when working with a string, you can use `chars()` method to iterate over each `char` value contained within. This allows you to access and process individual `char` elements safely.
Example:
“`rust
let my_string = String::from(“Hello, world!”);
for c in my_string.chars() {
// Access and process each char in the string
println!(“Character: {}”, c);
}
“`
2. Using `char` as an Integer:
In some cases, you may need to perform operations that require treating `char` values like integers. In such scenarios, you can cast them into their Unicode scalar values using the `as` keyword. This method allows you to work with the underlying integer representation of the `char`, enabling arithmetic operations.
Example:
“`rust
let my_char: char = ‘A’;
let my_char_value: u32 = my_char as u32;
println!(“Unicode value: {}”, my_char_value);
“`
3. Utilizing Unicode Methods:
Rust provides a rich set of built-in functions and methods to manipulate Unicode characters and strings through the `unicode` crate. These methods allow you to perform complex operations, such as string normalization or character categorization, while ensuring correct handling of Unicode encodings.
FAQs:
Q1: Why is `char` represented as four bytes in Rust?
A1: In Rust, a `char` is internally represented as a Unicode Scalar Value, which can range up to U+10FFFF. Since this exceeds the range of a single byte, `char` is represented using four bytes (32 bits) to accommodate the full Unicode spectrum.
Q2: Can I dereference a `char` pointer in unsafe Rust code?
A2: No, even in unsafe Rust code, you cannot dereference a `char` pointer directly. The safety limitations remain the same, and to prevent undefined behavior, explicit handling of `char` values is required.
Q3: Are there any performance implications when working with `char` in Rust?
A3: While there might be some performance overhead associated with manipulating `char` values due to handling multibyte Unicode characters, Rust’s Unicode handling methods and iterators are designed to efficiently work with such scenarios, minimizing any potential performance impact.
Conclusion:
Rust’s restriction on dereferencing `char` types ensures memory safety by preventing unsafe memory access and data corruption. By explicitly handling `char` manipulations, Rust programmers can ensure code correctness and avoid potential security vulnerabilities. Utilizing Rust’s built-in methods and iterators, developers can work effectively with Unicode characters and strings, ensuring proper encoding handling and efficient processing.
Images related to the topic char cannot be dereferenced
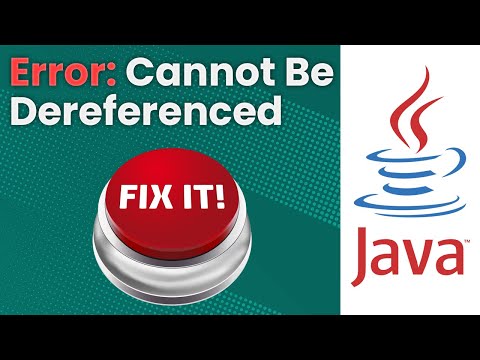
Found 8 images related to char cannot be dereferenced theme
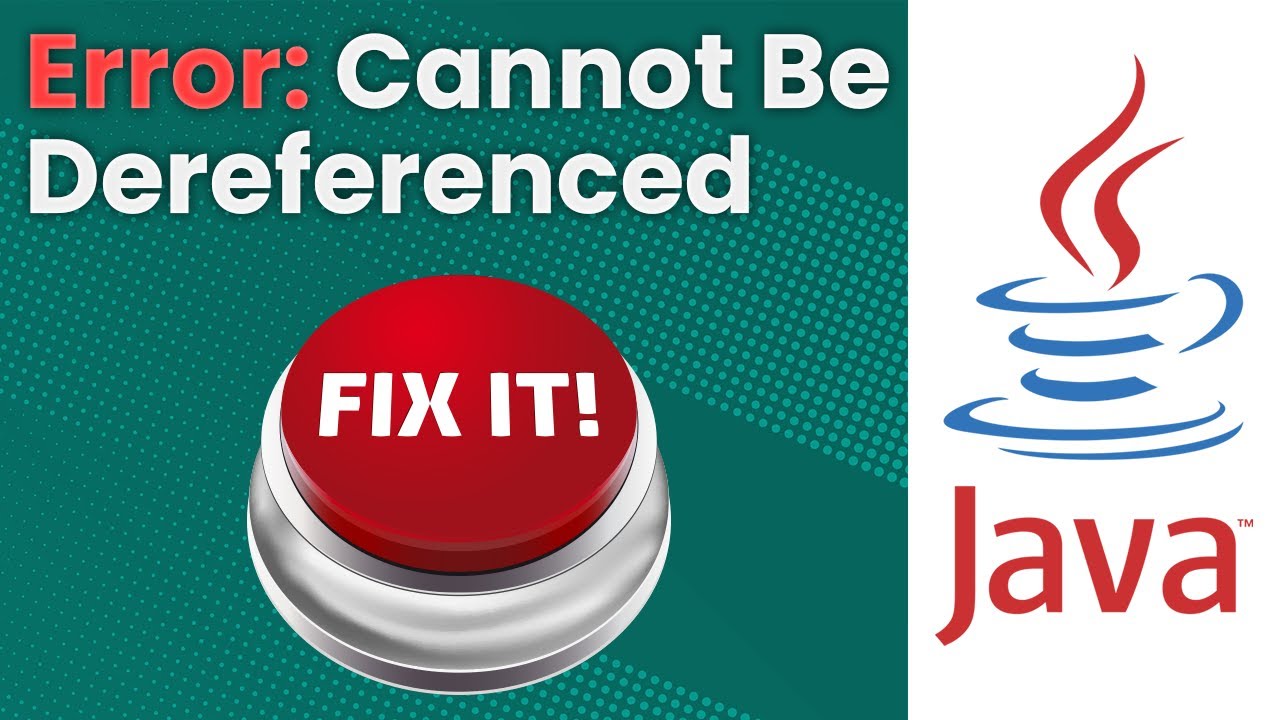
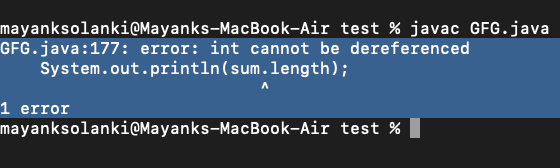

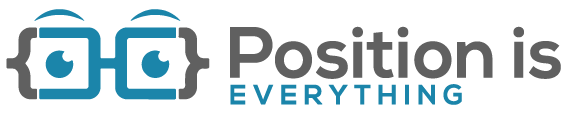
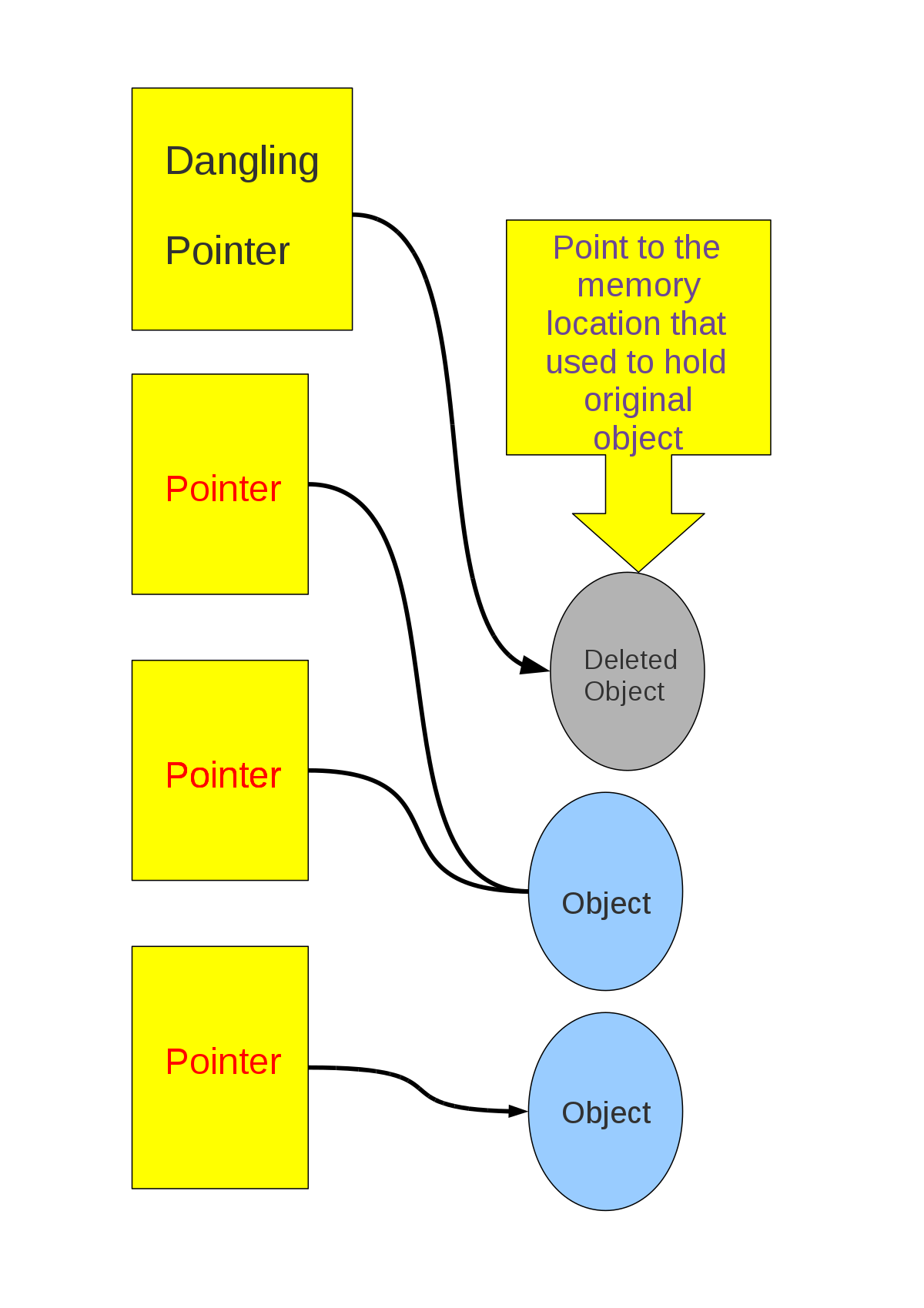
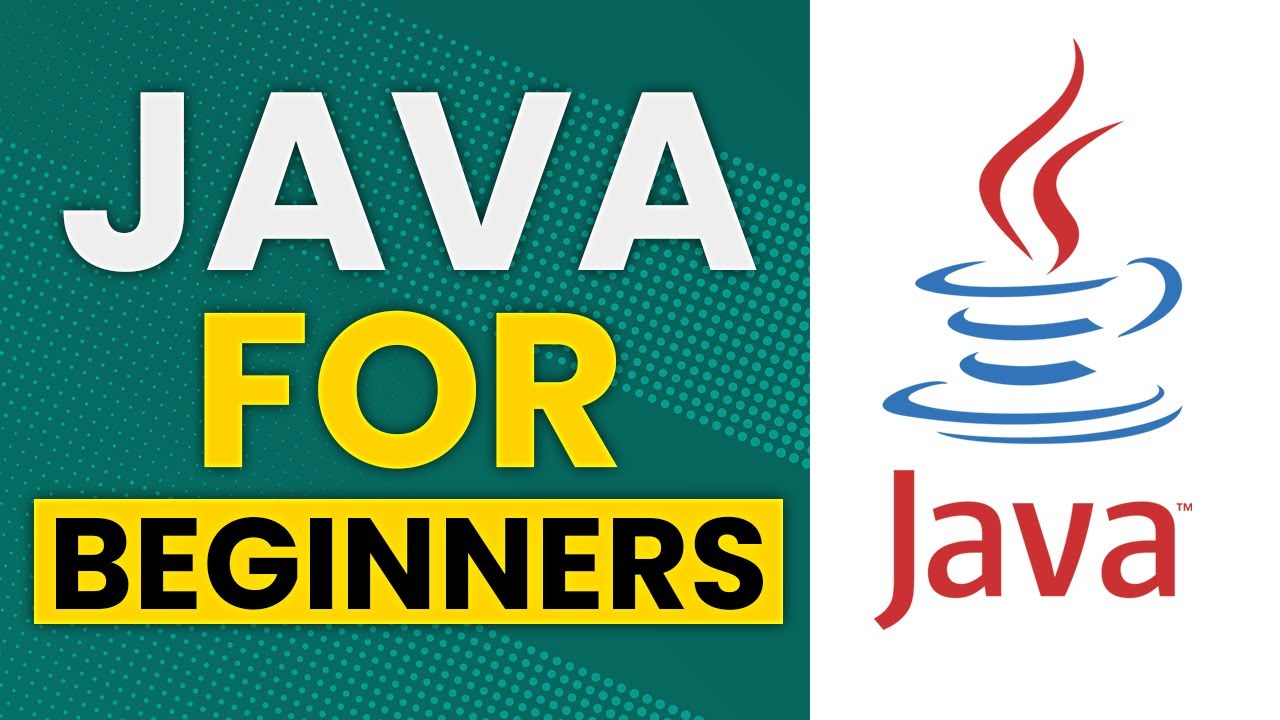


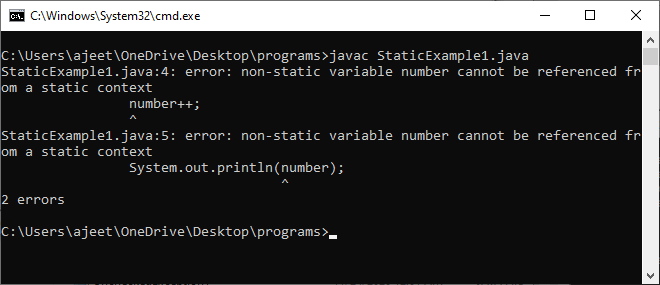
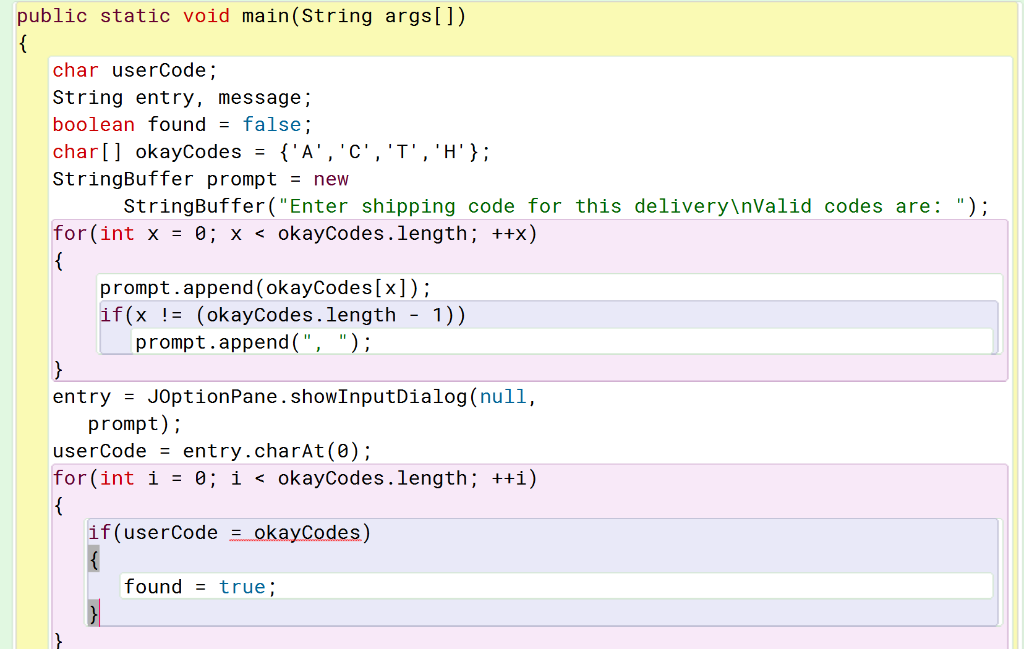
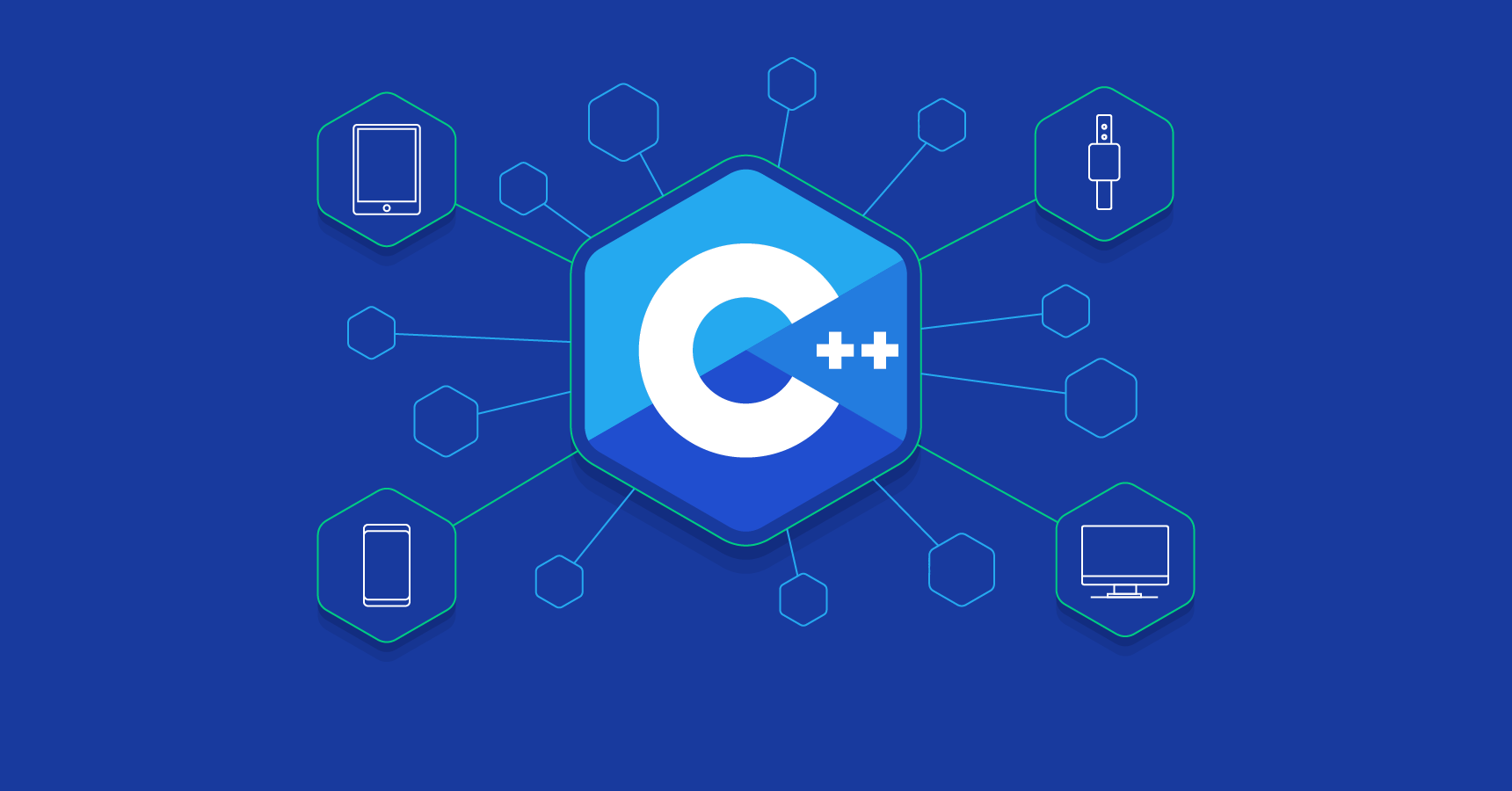
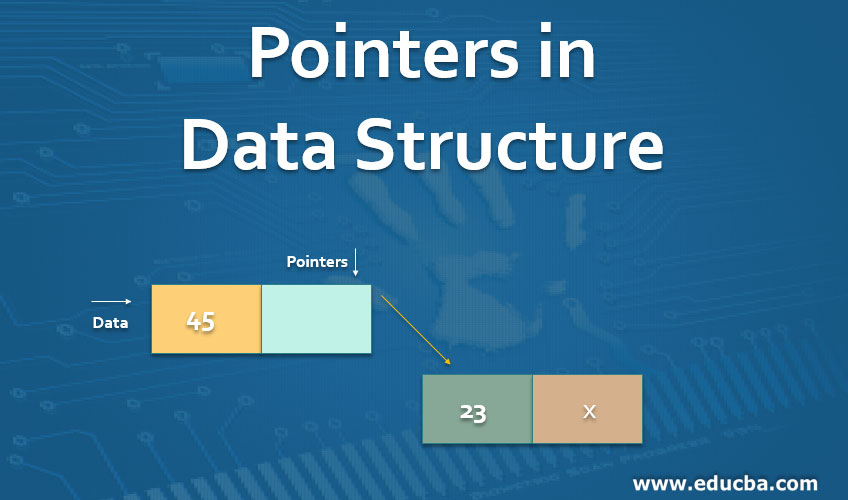
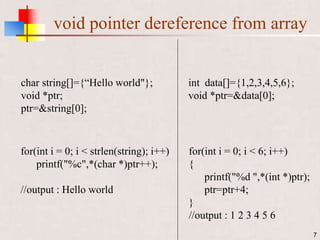


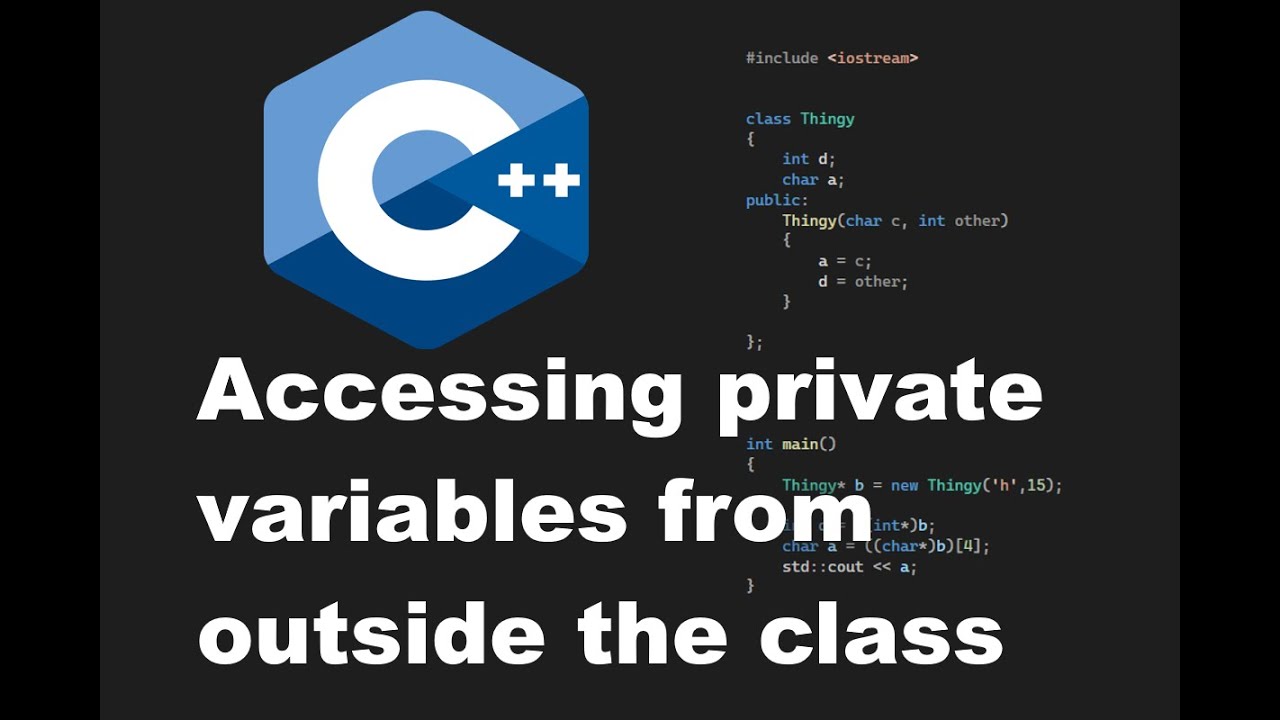

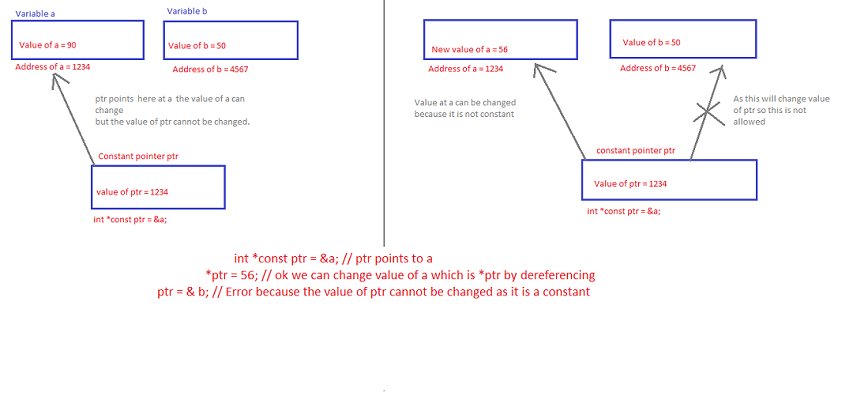
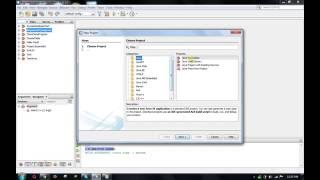
![Solved] The System Can Not Find The Path Specified Error in Command Prompt | Java Hungry Solved] The System Can Not Find The Path Specified Error In Command Prompt | Java Hungry](https://1.bp.blogspot.com/-VHJbBNHsknk/YOnEm7bm92I/AAAAAAAAByM/gyrV4OHjxtwi7TJG9FzdfCkk7mSVIO_NQCLcBGAsYHQ/s635/change%2Bthe%2Bcurrent%2Bworking%2Bdirectory%2Bto%2Ba%2Bnon-existing%2Bdirectory.png)

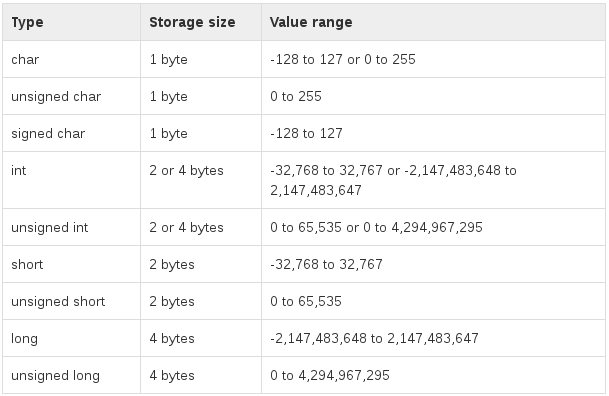
Article link: char cannot be dereferenced.
Learn more about the topic char cannot be dereferenced.
- “Char cannot be dereferenced” error – java – Stack Overflow
- [Solved] char cannot be dereferenced in java
- [Fixed] char cannot be dereferenced in java – Java2Blog
- Java “int/char Cannot Be Dereferenced” Error | Baeldung
- charAt error “char cannot be converted to string” – java – Stack Overflow
- [Fixed] char cannot be dereferenced in java – Java2Blog
- Java “int/char Cannot Be Dereferenced” Error | Baeldung
- char cannot be dereferenced – Tech Stack Journal –
- Java Char Cannot Be Dereferenced – Delft Stack
- [Solved] char cannot be dereferenced in java
- Solve Error “char cannot be dereferenced” in Java
- HELP! error: char cannot be dereferenced – Treehouse
- Char cannot be dereferenced – JAVA – Codecademy Forums