C# How To Clone Object
Cloning an object refers to the process of creating an exact replica of the original object. This replica can be used independently, with any changes made to it not affecting the original object. In C#, cloning an object can be achieved using either shallow copy or deep copy techniques. These techniques provide different ways to duplicate objects, each with its own advantages and limitations.
Shallow Copy vs Deep Copy: Different Cloning Approaches in C#
Shallow copying involves creating a new object and copying the values of all fields from the original object to the new object. However, if the original object contains reference types, the references are copied, not the actual objects. This means that changes made to the referenced objects will be reflected in both the cloned and original objects.
On the other hand, deep copying creates a complete independent copy of the original object. This means that even if the original object contains reference types, the referenced objects are also cloned and any subsequent changes made to them will not affect the cloned object.
Implementing Shallow Copying: Cloning Objects in C# with MemberwiseClone()
C# provides a built-in method called `MemberwiseClone()` that performs a shallow copy of an object. This method creates a new object and copies all the fields from the original object to the new object. To use this method, the `ICloneable` interface needs to be implemented by the object being cloned.
Here’s an example of how to implement shallow copying using `MemberwiseClone()`:
“`csharp
public class Person : ICloneable
{
public string Name { get; set; }
public int Age { get; set; }
public object Clone()
{
return this.MemberwiseClone();
}
}
“`
In this example, the `Person` class implements the `ICloneable` interface and overrides the `Clone()` method to perform the shallow copy using `MemberwiseClone()`. Note that `MemberwiseClone()` only creates a shallow copy, so it is important to be cautious when dealing with reference types.
Implementing Deep Copying: Cloning Objects in C# with Serialization
To achieve deep copying, C# provides another approach – serialization. By serializing an object and then deserializing it, a deep copy can be created. This ensures that even reference types are duplicated, resulting in completely independent objects.
Here’s an example of how to implement deep copying using serialization:
“`csharp
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
public class Car : ICloneable
{
public string Make { get; set; }
public string Model { get; set; }
public object Clone()
{
using (MemoryStream stream = new MemoryStream())
{
BinaryFormatter formatter = new BinaryFormatter();
formatter.Serialize(stream, this);
stream.Seek(0, SeekOrigin.Begin);
return formatter.Deserialize(stream);
}
}
}
“`
In this example, the `Car` class implements the `ICloneable` interface and overrides the `Clone()` method to perform deep copying using serialization. The object is serialized into a memory stream, and then deserialized to obtain the cloned object.
Overcoming Challenges: Cloning Objects with Reference Types in C#
When cloning objects with reference types, it is crucial to ensure that the referenced objects are also cloned. This prevents any changes made to the referenced objects from affecting the cloned object.
One approach to overcome this challenge is to ensure that the referenced objects themselves implement the `ICloneable` interface and are cloned recursively. Alternatively, serialization can be used to achieve deep copying, as discussed earlier.
Additionally, it is important to consider the performance implications of cloning large objects or objects with complex object graphs. Deep copying can be a costly operation, so it is essential to evaluate whether cloning is necessary and if there are more efficient alternatives.
Best Practices and Considerations for Object Cloning in C#
– Avoid using object cloning as a default mechanism for creating copies. Consider whether a copy constructor or factory method may be more appropriate for creating object copies.
– Be cautious when cloning objects with reference types. Ensure that referenced objects are also cloned to prevent changes from affecting the cloned object.
– Consider using serialization for deep copying, especially when dealing with complex object graphs or objects with nested reference types.
– Use the `ICloneable` interface for consistent cloning implementations, but exercise caution when relying on it for deep copying, as it only provides support for shallow copying by default.
In conclusion, object cloning in C# can be achieved using either shallow copy or deep copy techniques. Shallow copying copies the values of fields, while deep copying creates a complete independent replica. C# provides built-in methods like `MemberwiseClone()` and serialization for implementing these techniques. However, it is important to consider challenges related to reference types and performance when cloning objects. By following best practices and considering the specific requirements of your application, you can effectively clone objects in C#.
FAQs
Q: Can I clone an object without implementing the ICloneable interface?
A: Yes, it is possible to clone an object without implementing the `ICloneable` interface. However, it is generally considered good practice to implement the interface to provide a standardized way of cloning objects.
Q: What is the main difference between shallow copy and deep copy?
A: Shallow copy only copies the values of fields, including any reference types. Deep copy, on the other hand, creates a complete independent copy of the original object, including any referenced objects.
Q: Are there any limitations to shallow copying or deep copying in C#?
A: Shallow copying can lead to unintended changes in the cloned object if reference types are not handled properly. Deep copying, on the other hand, may be more resource-intensive, especially for large objects or objects with complex object graphs.
Q: Can I use object cloning as a way to create object backups?
A: Object cloning can be a way to create object backups; however, it is important to consider the implications, such as memory usage and performance. Alternatives like copy constructors or Memento pattern may be more suitable for creating object backups.
Orange C-Section – 5 Babies 😱🍊 Leave A Like For The Little Ones❤️ #Fruitsurgery #Shorts #Animation
Keywords searched by users: c# how to clone object
Categories: Top 20 C# How To Clone Object
See more here: nhanvietluanvan.com
Images related to the topic c# how to clone object
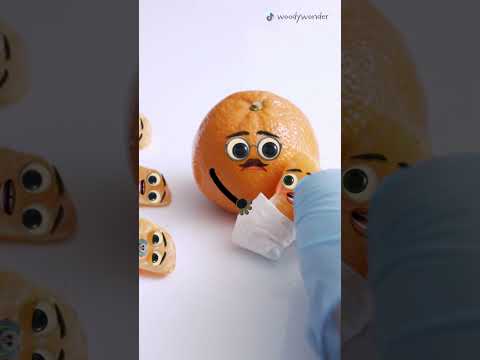
Article link: c# how to clone object.
Learn more about the topic c# how to clone object.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- C – Wiktionary tiếng Việt
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/