C++ Read File Line By Line
Reading files line by line is an essential aspect of C++ programming, particularly when dealing with large datasets or processing information sequentially. This method allows programmers to efficiently handle files that contain a significant amount of data, without overwhelming the memory or processing capabilities of the program. Additionally, reading files line by line enables developers to easily manipulate and extract specific information from the file, facilitating data analysis and processing tasks.
1. Opening a file for reading in C++
To read a file line by line in C++, the first step is to open the file using the `ifstream` class. This class provides input functionality and is defined in the `
“`cpp
#include
#include
int main() {
std::ifstream inputFile(“example.txt”);
if (!inputFile.is_open()) {
std::cout << "Error opening the file.";
return -1;
}
// File is successfully opened
inputFile.close(); // Close the file after processing
return 0;
}
```
In the code above, the `ifstream` instance `inputFile` is created, and the file "example.txt" is opened for reading. After opening the file, it is essential to check if the file was successfully opened or not. This can be done by invoking the `is_open()` method of the `ifstream` instance, which returns a Boolean value indicating the status of the file stream object. If opening the file fails, appropriate error handling can be implemented.
2. Checking if the file was successfully opened
Verifying whether a file was successfully opened is crucial to prevent potential errors in the subsequent reading and processing operations. Besides using the `is_open()` method, other approaches can be adopted for error handling:
a. Throwing exceptions: Instead of outputting an error message directly, an exception can be thrown to handle the error in a more controlled manner. For instance:
```cpp
if (!inputFile.is_open()) {
throw std::runtime_error("Error opening the file.");
}
```
b. Printing error messages: Alternatively, error messages can be printed to the console or a log file to provide details regarding the failure to open the file. For example:
```cpp
if (!inputFile.is_open()) {
std::cout << "Error opening the file.";
return -1;
}
```
By incorporating error handling, the program can gracefully handle situations where the file cannot be opened, preventing potential crashes or unpredictable behavior.
3. Reading and processing each line of the file
A common technique for reading a file line by line involves using a loop in conjunction with the `getline` function. This function retrieves one line at a time from the file and saves it in a string variable for further processing. Here's an example:
```cpp
std::string line;
while (getline(inputFile, line)) {
// Process each line
// Print the line to the console:
std::cout << line << std::endl;
// Tokenize the line, split by whitespace:
std::istringstream iss(line);
std::vector
std::string token;
while (iss >> token) {
tokens.push_back(token);
}
// Extract specific data or perform other processing tasks
}
“`
In the code snippet above, each line of the file is read and stored in the `line` variable using the `getline` function. This line can then be printed to the console or processed further, such as tokenizing the line into separate words.
4. Skipping empty lines and comments
Often, empty lines and comments in files are not relevant for processing and can be ignored. There are multiple techniques to skip these lines:
a. Conditional statements: By implementing conditional statements, empty lines or lines starting with a specific character (e.g., ‘#’) can be skipped. For instance:
“`cpp
std::string line;
while (getline(inputFile, line)) {
if (!line.empty() && line[0] != ‘#’) {
// Process non-empty non-comment lines
}
}
“`
b. Regular expressions: Regular expressions can be employed to identify and skip lines that match specific patterns, such as empty lines or comments. This approach requires including the `
“`cpp
#include
std::string line;
std::regex emptyLineRegex(“^\\s*$”);
std::regex commentLineRegex(“^\\s*#”);
while (getline(inputFile, line)) {
if (!regex_match(line, emptyLineRegex) && !regex_match(line, commentLineRegex)) {
// Process non-empty non-comment lines
}
}
“`
By intelligently skipping these unnecessary lines, the program’s efficiency and performance can be improved.
5. Handling different file formats
C++ provides several techniques to handle various file formats, such as CSV, TXT, or XML. Some common approaches include:
a. Parsing: Parsing involves extracting specific data or patterns from structured files, such as CSV or XML. To parse these files, one can utilize C++ libraries like `boost::spirit`, `TinyXML`, or `RapidXML` to simplify the extraction process. These libraries provide powerful parsing capabilities, making it easier to handle complex file formats.
b. Splitting: If the file contains data in a structured format, splitting the lines based on specific delimiters or separators can be used to extract relevant information. Strings can be split into substrings using delimiters like commas or spaces. Here’s an example of splitting a CSV line:
“`cpp
std::string line;
while (getline(inputFile, line)) {
std::string token;
std::istringstream iss(line);
while (getline(iss, token, ‘,’)) {
// Process each token
}
}
“`
c. Specific libraries: Depending on the file format, specific libraries exist to handle the extraction of data, such as the `csv-parser` library for reading CSV files. These libraries simplify the process by providing ready-made functions and structures to facilitate file handling.
6. Closing the file and error handling
Closing the file after processing is crucial to release system resources and ensure proper cleanup. The `close` method is used to close the file stream. Additionally, error handling can be implemented to handle any possible errors during the file closure:
“`cpp
inputFile.close();
if (inputFile.fail()) {
std::cout << "Error closing the file.";
return -1;
}
```
By utilizing proper error handling techniques during file closure, potential issues can be addressed, ensuring a robust and error-free program.
FAQs:
1. Q: How can I read a file line by line in C++?
A: To read a file line by line in C++, use the `getline` function in conjunction with a loop that checks the status of the file stream object.
2. Q: How do I skip empty lines and comments when reading a file?
A: Skimming empty lines and comments can be achieved by incorporating conditional statements or regular expressions to identify and ignore such lines.
3. Q: How can I handle different file formats, such as CSV or XML?
A: Handling various file formats often involves techniques like parsing, splitting, or utilizing specific libraries that provide functionalities for extracting relevant information from distinct formats.
4. Q: What should I do to ensure the file is closed correctly?
A: It is crucial to employ the `close` method to close the file stream after processing and warrant proper cleanup. Additionally, error handling techniques can be utilized during file closure to address any potential issues.
Read A Specific Line From A File | C Programming Example
How To Read A File Line Per Line In C?
Reading files is an essential task in any programming language, including C. Whether you are working with small text files or large data files, reading them line by line can be necessary to analyze or manipulate the data. In this article, we will guide you through the process of reading a file line per line in the C programming language. We will cover different methods, explain the necessary concepts, and provide example code to ensure a comprehensive understanding. So, let’s dive in!
## Table of Contents
1. Understanding File Handling in C
2. Opening a File in Read Mode
3. Reading a File Line by Line
4. Handling Exceptions
5. Closing the File
6. Example Code
7. FAQs
## 1. Understanding File Handling in C
File handling involves managing files within a program. The C programming language provides standard library functions and file pointers to handle files effectively. Before reading a file line by line, it is crucial to grasp the basic concepts of file handling in C.
A file pointer is a variable used to point to a file that is opened. It keeps track of the current position in a file, allowing us to read, write, or modify its contents. File pointers are declared using the `FILE` data type, which is defined in the `
## 2. Opening a File in Read Mode
Before you can read a file, you need to open it in read mode using the `fopen()` function. The syntax for opening a file is as follows:
“`
FILE *filePointer;
filePointer = fopen(“filename”, “mode”);
“`
In this case, `”filename”` represents the name of the file you wish to open, and `”mode”` specifies the purpose. To open a file for reading, pass the `”r”` mode.
## 3. Reading a File Line by Line
Once you have opened the file, you can start reading its contents line by line. The `fgets()` function allows us to read a specified number of characters from a file until it encounters a newline character (`’\n’`) or reaches the specified limit.
The syntax for using `fgets()` is as follows:
“`
char *fgets(char *str, int n, FILE *filePointer);
“`
Here, `str` represents the pointer to an array of characters where the read line will be stored, `n` is the maximum number of characters to be read, and `filePointer` is the file pointer associated with the opened file.
However, reading a line with `fgets()` includes the newline character at the end. To remove the newline character, we can use the `strcspn()` function that calculates the length of the string until the newline character is encountered.
The implementation for reading a file line by line can be achieved using a loop. Here’s an example code snippet:
“`c
char line[100]; // Assuming maximum line length is 100
while (fgets(line, sizeof(line), filePointer) != NULL) {
line[strcspn(line, “\n”)] = ‘\0’; // Removing newline character
// Process the line as desired
}
“`
In this example, we initialize a character array `line` to store each line of the file. We use `fgets()` within a `while` loop to read each line until `fgets()` returns `NULL`, indicating the end of the file. Then, using `strcspn()`, we remove the newline character from each line for further processing.
## 4. Handling Exceptions
While reading a file, it is necessary to handle exceptions that may occur. One common exception is when the file cannot be opened. In such cases, `fopen()` returns `NULL`, indicating the failure. Therefore, it is essential to check if the file was opened successfully before proceeding with the reading process. You can use an `if` statement to handle this exception.
“`c
FILE *filePointer;
filePointer = fopen(“filename”, “r”);
if (filePointer == NULL) {
printf(“Unable to open the file.\n”);
// Handle the exception, such as terminating the program
} else {
// Proceed with reading the file
// …
}
“`
## 5. Closing the File
After you have finished reading the file, it is crucial to close it properly using the `fclose()` function. Closing the file releases system resources and allows other programs to access the file. The syntax for closing the file is simple:
“`c
fclose(filePointer);
“`
Remember to close the file at the end of your program or when you no longer need further access to it.
## 6. Example Code
Let’s put everything together by providing an example code to read a file line by line in C:
“`c
#include
#include
#include
int main() {
FILE *filePointer;
char line[100]; // Assuming maximum line length is 100
filePointer = fopen(“example.txt”, “r”);
if (filePointer == NULL) {
printf(“Unable to open the file.\n”);
return 1; // Terminate the program
}
while (fgets(line, sizeof(line), filePointer) != NULL) {
line[strcspn(line, “\n”)] = ‘\0’; // Removing newline character
printf(“%s\n”, line); // Printing the line for demonstration
}
fclose(filePointer);
return 0;
}
“`
In this example, we open a file named `”example.txt”` in read mode. If the file cannot be opened, we display an error message and terminate the program. Otherwise, we read the file line by line using the `fgets()` function and print each line for demonstration purposes. Finally, we close the file.
## 7. FAQs
**Q1: Can I read a binary file line by line using the methods explained above?**
Yes, you can read a binary file line by line using the same methods mentioned in this article. However, note that the content of each line might not be meaningful, as binary files can contain characters that do not represent text.
**Q2: How can I read a file in reverse order, starting from the last line?**
To read a file in reverse order, starting from the last line, you can read the file line by line into an array or a linked list and then traverse the array or linked list in reverse order. Alternatively, you can use file seek operations (`fseek()` and `ftell()`) to navigate through the file in reverse order. This approach is more complex but allows direct access to specific lines without loading the entire file into memory.
**Q3: Is there a limit to the size of the file that can be read using the methods described above?**
The limit on the file size depends on the system’s memory constraints and available resources. Reading large files may require loading chunks of data at a time rather than the whole file into memory. This allows processing the file in a memory-efficient manner.
In conclusion, reading a file line by line in C involves opening the file in read mode, using the `fgets()` function within a loop to read each line, handling exceptions, and closing the file properly. By following this method, you can efficiently process text files or binary files line by line, making it convenient for various programming tasks.
How To Read Next Line In C?
Reading input from a file or user input is a common requirement for many C programs. In order to process textual data, it is essential to know how to read each line of a file or user input, character by character, until the end of the line is reached. In this article, we will explore various methods and functions in the C programming language to accomplish this task.
1. Reading a Single Line Using scanf()
One of the simplest ways to read a line of input in C is by using the scanf() function. However, scanf() has limitations when it comes to reading lines with spaces or special characters. Nevertheless, it can be used if your input does not contain these constraints. The code snippet below demonstrates reading a line using scanf():
“`c
char line[256]; // Maximum line length is assumed to be 255 characters
scanf(“%255[^\n]”, line); // Read characters until a newline is encountered
“`
In this code, we declare a character array named “line” which will store the input line. The “%255[^\n]” format specifier inside scanf() instructs it to read characters until a newline character is encountered.
2. Using fgets() to Read a Line
The fgets() function is a safer and versatile alternative to scanf() for reading lines. It reads characters from a file or user input stream, up to a specified limit, including the newline character. The following code demonstrates its usage:
“`c
char line[256];
fgets(line, sizeof(line), stdin);
“`
In this example, fgets() reads the line from the standard input stream (stdin) into the “line” character array, limiting the read to the size of the array passed as the second argument.
3. Reading Multiple Lines Using fgets()
To read multiple lines from a file or user input continuously, we can combine fgets() with a loop. Each time fgets() is called, it reads the next line until the end of the file or user input stream is reached. Here’s an example that demonstrates reading multiple lines:
“`c
char line[256];
while (fgets(line, sizeof(line), stdin) != NULL) {
// Process the line here
}
“`
This code reads lines from the standard input stream (stdin) until NULL is encountered, indicating the end of the stream. You can perform processing operations inside the while loop for each line read.
4. Reading Lines Using getline()
The getline() function is another useful method for reading lines of input. It has some advantages over fgets() since it can handle lines of any size without requiring a predefined buffer. Here’s an example that demonstrates its usage:
“`c
#include
#include
int main() {
char* line = NULL;
size_t len = 0;
ssize_t nread;
while ((nread = getline(&line, &len, stdin)) != -1) {
// Process the line here
}
free(line);
return 0;
}
“`
In this example, we declare a pointer “line” as NULL and the variable “len” to store the length of each read line. After each call to getline(), it checks if any characters were read, indicated by the return value of getline() not equal to -1.
FAQs
Q1. Which method is better for reading lines in C, scanf(), or fgets()?
Both methods have their pros and cons. Generally, fgets() is preferred as it is safer for reading lines, especially if the input contains spaces or special characters. However, if you are sure about the input format and do not have such constraints, scanf() can be used. It ultimately depends on the specific requirements of your program.
Q2. Are there any limitations to reading lines using fgets()?
fgets() has a limitation on the line size as it requires a pre-defined character array to store the line. If the input line exceeds the array size, fgets() will read the maximum number of characters equal to the size limit and append a null character at the end. If the line is larger, you may need to handle it differently, such as reading the line in chunks.
Q3. How can I handle errors while reading lines in C?
When using scanf() or fgets(), you should always check the return value to ensure that the reading operation was successful. If the return value is NULL or -1, it indicates an error or the end of the stream/file. You can then handle the error appropriately, such as printing an error message or terminating the program.
Q4. Can I read lines of a file other than the standard input in C?
Absolutely! You can open a file using functions like fopen() and then pass the resulting file pointer to fgets() or getline() instead of stdin. This way, you can read lines from the specified file instead of the standard input.
Conclusion
Reading lines in C is a fundamental operation for many programs. In this article, we explored various methods to read lines, such as scanf(), fgets(), and getline(). By understanding these methods and their differences, you can confidently read input lines character by character, process the data, and handle different scenarios as necessary. Remember to choose the appropriate method based on the requirements and constraints of your program.
Keywords searched by users: c++ read file line by line Read file C++ line by line, Read text file C, Fscanf C line by line, Read line by line from file in C, Read char file in c, Getline in C, Read first line in C, Read file in C
Categories: Top 29 C++ Read File Line By Line
See more here: nhanvietluanvan.com
Read File C++ Line By Line
Reading file line by line is a common requirement in many programming tasks. In C++, there are several methods and approaches to accomplish this. In this article, we will explore the different techniques and provide a comprehensive guide on how to read a file line by line in C++. Before diving into the coding aspects, let’s understand the basics.
Understanding file streams in C++
In C++, working with files involves streams that handle the input and output operations. The most common streams are ifstream (input file stream) and ofstream (output file stream). To read a file, we need to create an ifstream object and open the file using the file path. Once the file is opened, we can start reading its contents.
Method 1: Using getline()
One straightforward approach to read a file line by line is by utilizing the getline() function. This function reads a line from the input stream until it encounters a newline character (‘\n’) or reaches the end of the file. Here’s an example demonstrating its usage:
“`cpp
#include
#include
using namespace std;
int main() {
ifstream inputFile(“file.txt”);
string line;
if (inputFile.is_open()) {
while (getline(inputFile, line)) {
cout << line << endl;
}
inputFile.close();
}
return 0;
}
```
In this code, we open the file "file.txt" using the ifstream object inputFile. We then loop through the file using getline() to read each line, which is stored in the variable line. We can perform any required operations on the line inside the loop. Finally, we close the file using inputFile.close().
Method 2: Using >> operator
The extraction operator (>>) can also be used to read a file line by line, but it requires a bit more effort compared to getline(). This method reads data separated by a space or tab character, and each consecutive extraction reads the next word on the line. Here’s an example:
“`cpp
#include
#include
using namespace std;
int main() {
ifstream inputFile(“file.txt”);
string word;
if (inputFile.is_open()) {
while (inputFile >> word) {
cout << word << " ";
}
inputFile.close();
}
return 0;
}
```
Here, we use the ifstream object inputFile to read words from the file, storing them in the variable word. We continue reading until the end of the file is reached. Again, any required operations can be performed within the loop.
FAQs
Q: What happens if the file cannot be opened?
A: If the file cannot be opened, ifstream's is_open() function will return false, indicating a failure to open the file. Make sure to handle such cases appropriately, for example, by displaying an error message or terminating the program gracefully.
Q: How can I pass the filename as a command-line argument?
A: When running the program, you can provide the filename as command-line arguments. The main function signature can be modified to include parameters for the command-line arguments:
```cpp
int main(int argc, char *argv[])
```
Then, you can access the filename using argv, such as:
```cpp
ifstream inputFile(argv[1]);
```
Q: How can I check if the end of the file has been reached?
A: In both methods discussed above, we can check if the end of the file has been reached by evaluating the file stream while performing the input operation inside the loop. The loop will terminate when reaching the end of the file.
Q: Can I read files from remote locations or URLs?
A: The methods described in this article are suitable for reading local files only. To read files from remote locations or URLs, additional libraries or frameworks may be required.
Q: How can I handle large files efficiently?
A: If dealing with large files, it's advisable to read the file in chunks rather than reading the entire file at once. This can be accomplished by specifying a maximum line length or using a buffer to read lines in smaller portions.
In conclusion, reading a file line by line in C++ can be achieved using various methods, such as getline() and the extraction operator (>>). Both approaches are effective and can be chosen based on the specific requirements of the task at hand. By leveraging these techniques, programmers can efficiently process file contents and manipulate data accordingly.
Read Text File C
How to Use the Read Text File C Command:
To utilize the Read text file C command, you need to follow a specific syntax that varies slightly between programming languages. Let’s delve into the syntax of this command for a few popular languages.
1. Using Read Text File C in C++:
To read a text file named “C” in C++, you need to follow the steps outlined below:
Step 1: Start by including the necessary header file, `
“`cpp
#include
“`
Step 2: Declare an object of the `ifstream` class (input file stream) by specifying the file name as a parameter and open the file.
“`cpp
std::ifstream file(“C”);
“`
Step 3: Check if the file has been successfully opened.
“`cpp
if (!file) {
// File opening failed
}
“`
Step 4: Read the contents of the file using the file object.
“`cpp
std::string line;
while (std::getline(file, line)) {
// Manipulate each line of the file as required
// For example, print the line
std::cout << line << std::endl;
}
```
2. Using Read Text File C in Python:
Python provides a simple way to read a text file named "C" using the following steps:
Step 1: Open the file using the `open()` function.
```python
file = open("C", "r")
```
Step 2: Read the contents of the file using the `readlines()` method, which returns a list of lines.
```python
lines = file.readlines()
```
Step 3: Manipulate the lines as required.
```python
for line in lines:
# Manipulate each line of the file as needed
# For instance, print the line
print(line)
```
3. Using Read Text File C in Java:
In Java, file reading is accomplished by creating an instance of the `FileReader` class and using a `BufferedReader` for efficient reading. Follow the steps below to read a text file named "C" in Java:
Step 1: Import the necessary classes.
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
```
Step 2: Create an instance of the `FileReader` and `BufferedReader` objects to read the file.
```java
try {
FileReader fileReader = new FileReader("C");
BufferedReader bufferedReader = new BufferedReader(fileReader);
```
Step 3: Read the contents of the file using the `readLine()` method and manipulate the lines as needed.
```java
String line;
while ((line = bufferedReader.readLine()) != null) {
// Manipulate each line of the file as required
// For example, print the line
System.out.println(line);
}
```
Step 4: Close the `BufferedReader` to release system resources.
```java
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
```
FAQs (Frequently Asked Questions):
Q1. What if the file doesn't exist or the path is incorrect?
If the file doesn't exist or the specified path is incorrect, an error will occur while opening the file. It is essential to ensure that the file name and path are accurate to avoid such issues.
Q2. Is it possible to read a file from a remote location?
Yes, it is possible to read a file from a remote location by specifying the absolute path or URL to the file instead of a local file name.
Q3. Can I read binary files using the Read text file C command?
The Read text file C command is primarily designed for reading text files. To read binary files, you may need to use different commands or methods specific to handling binary data within your programming language.
Q4. How can I read only a specific number of lines from a file?
To read a specific number of lines from a file, you can use a counter variable to keep track of the lines read and break the loop when the desired number is reached or add additional conditions to limit the number of iterations.
In conclusion, the Read text file C command provides a convenient way to access and process the content of a text file in a programming language. By following the syntax specific to your chosen language, you can read the contents of a file line by line, enabling various data manipulation tasks. Remember to ensure the correct file path and handle any errors that may occur during file opening.
Images related to the topic c++ read file line by line
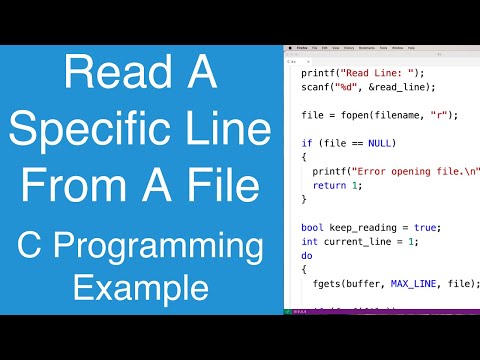
Found 7 images related to c++ read file line by line theme
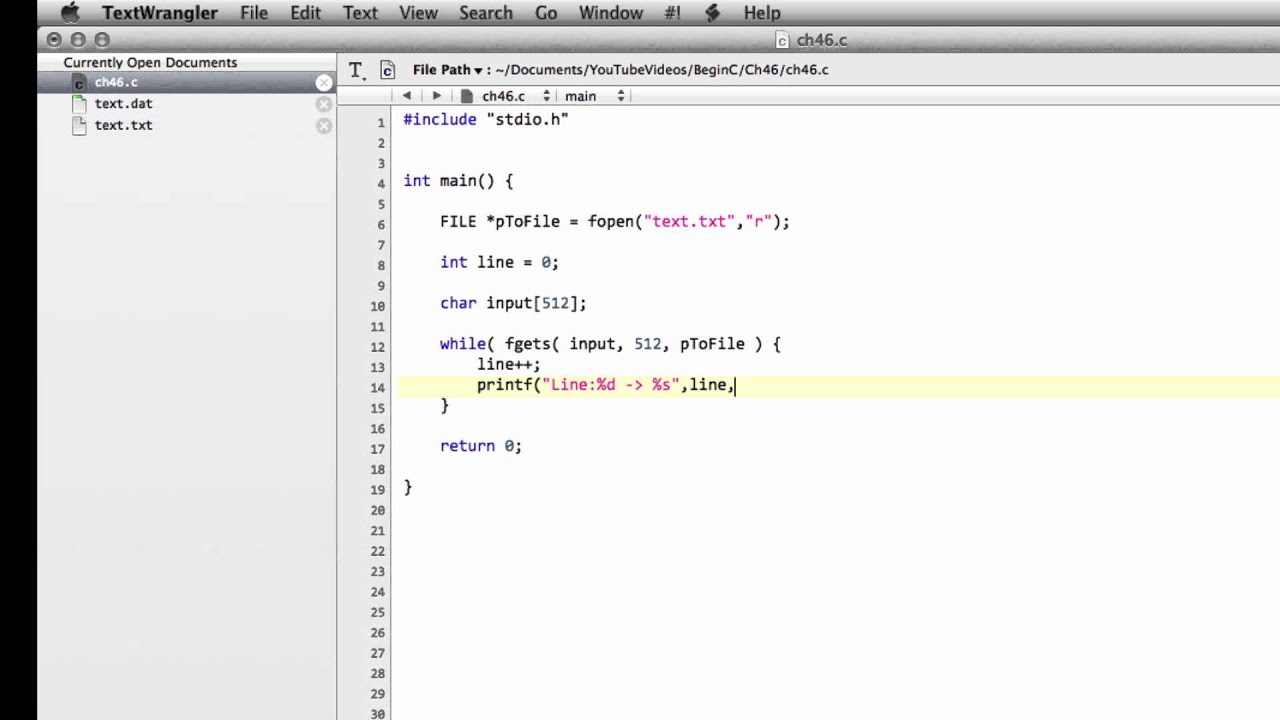
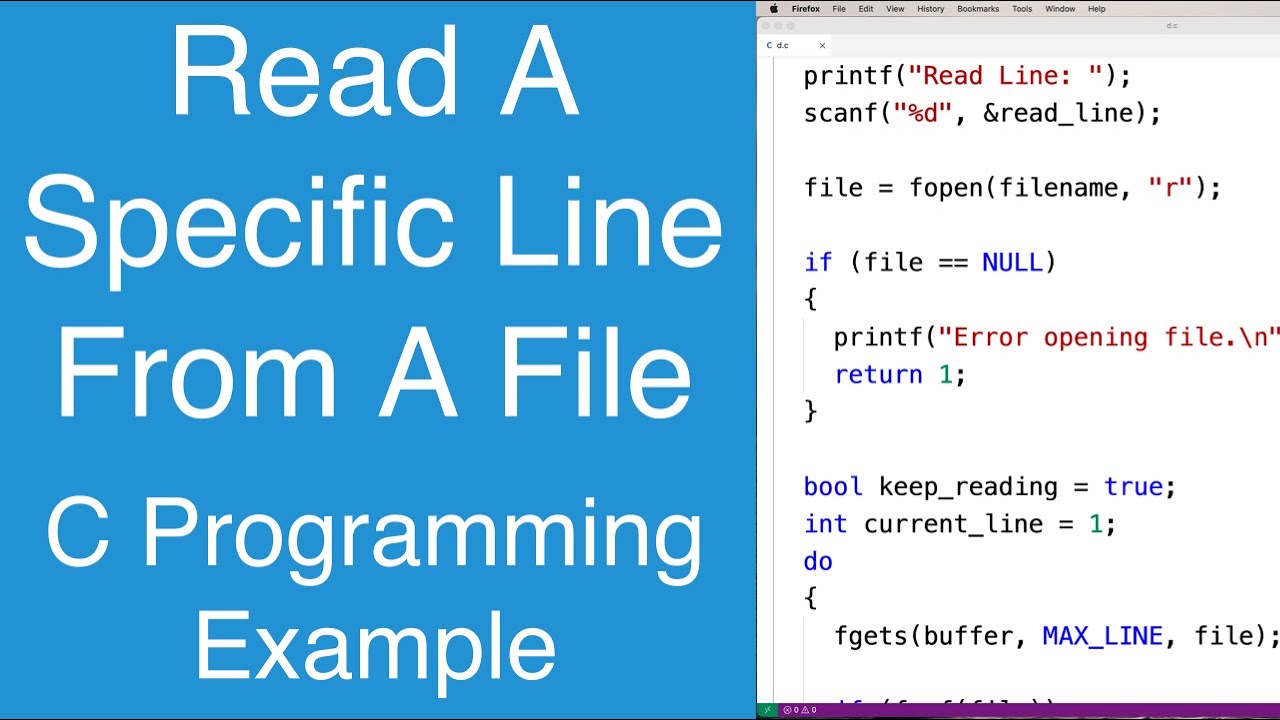
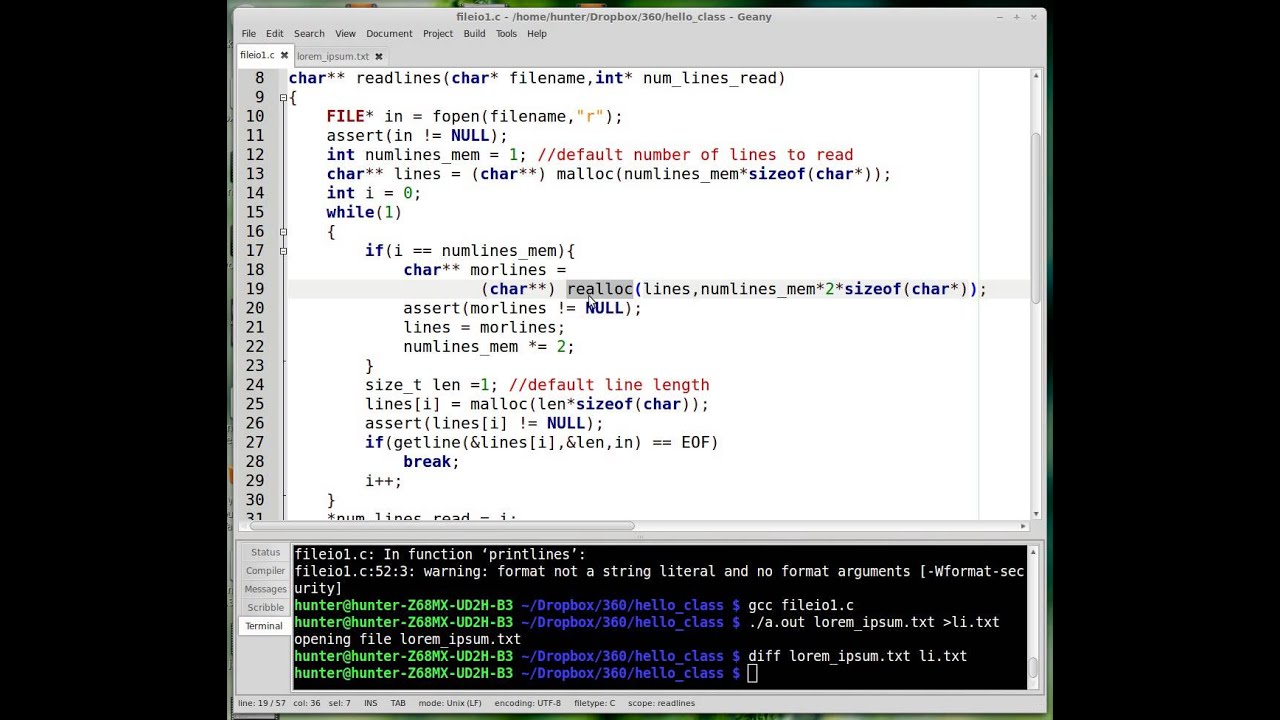
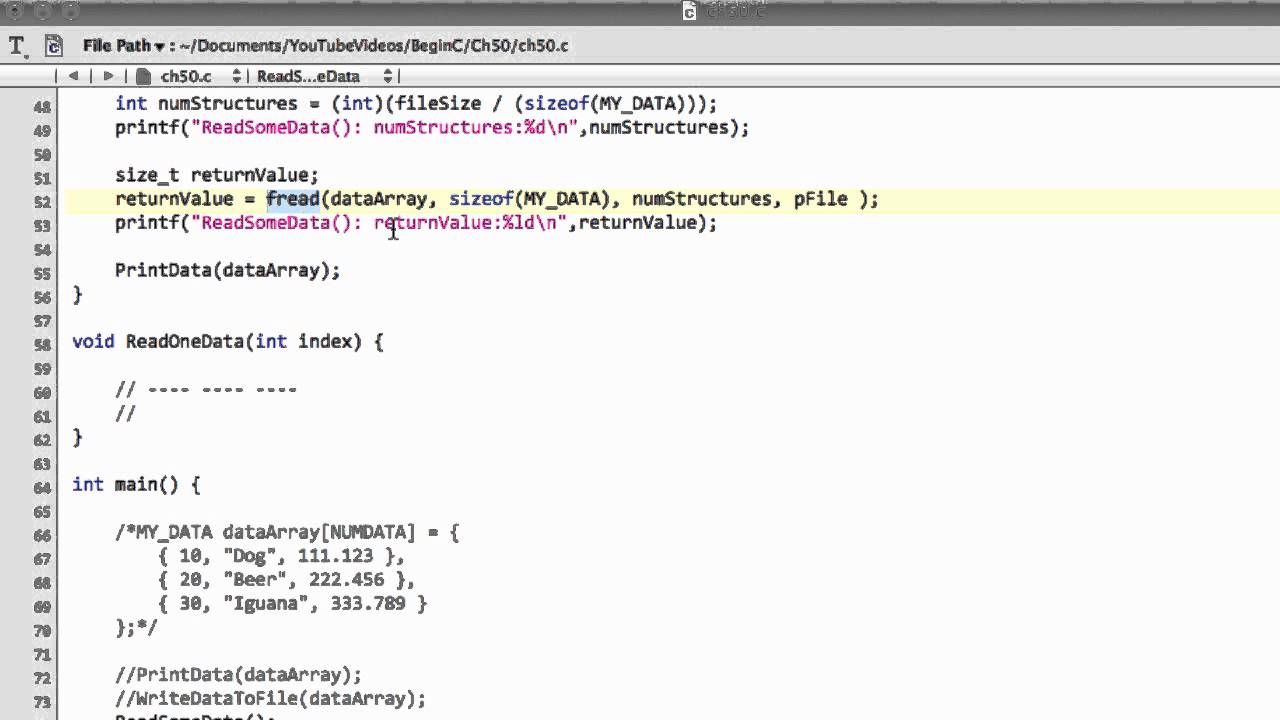
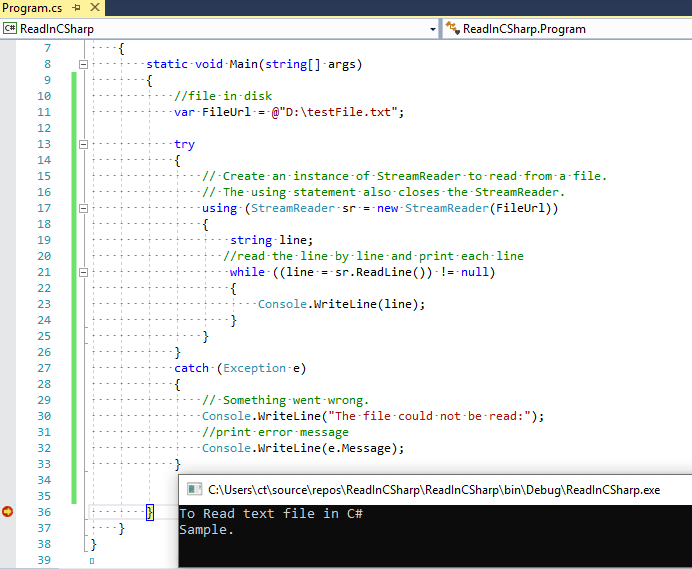
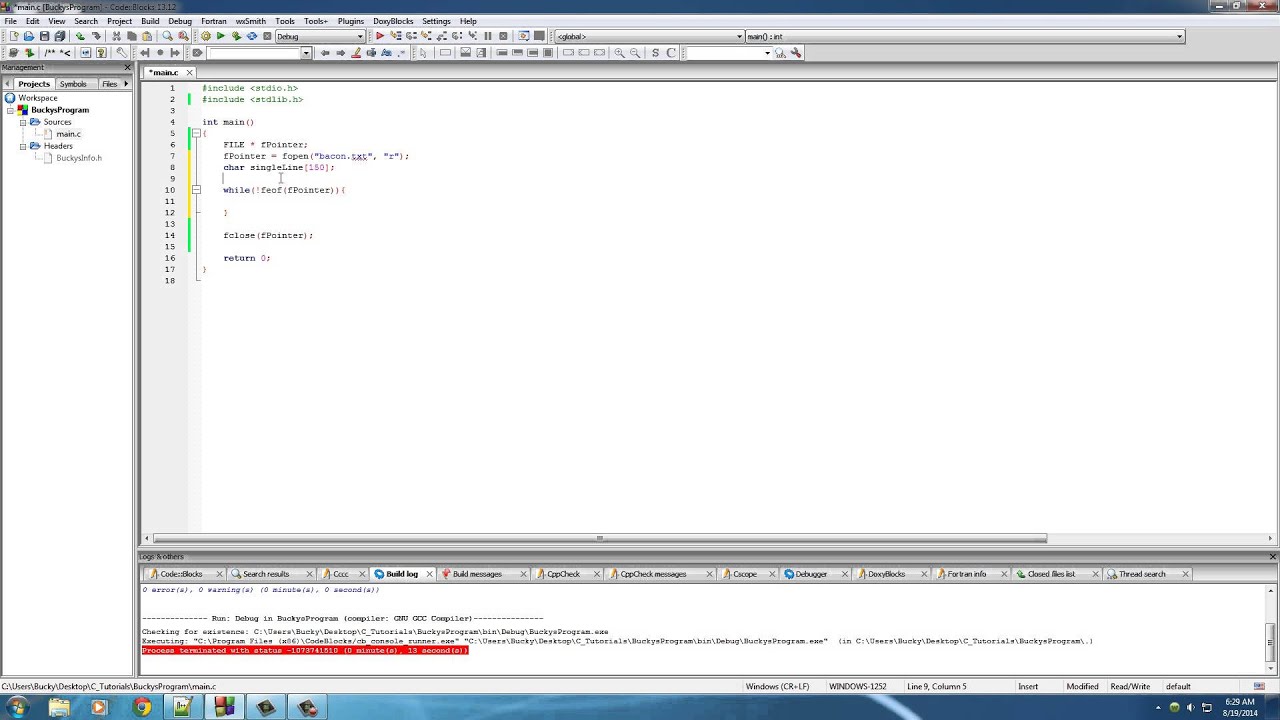

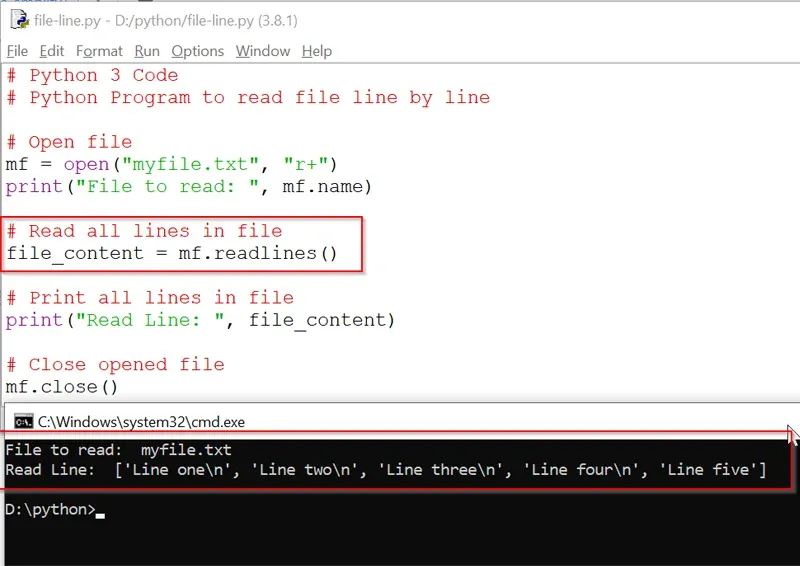
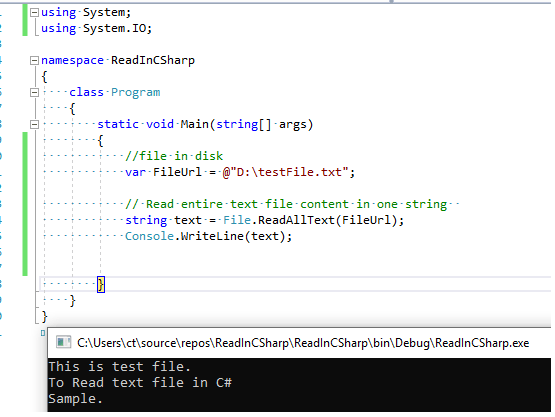
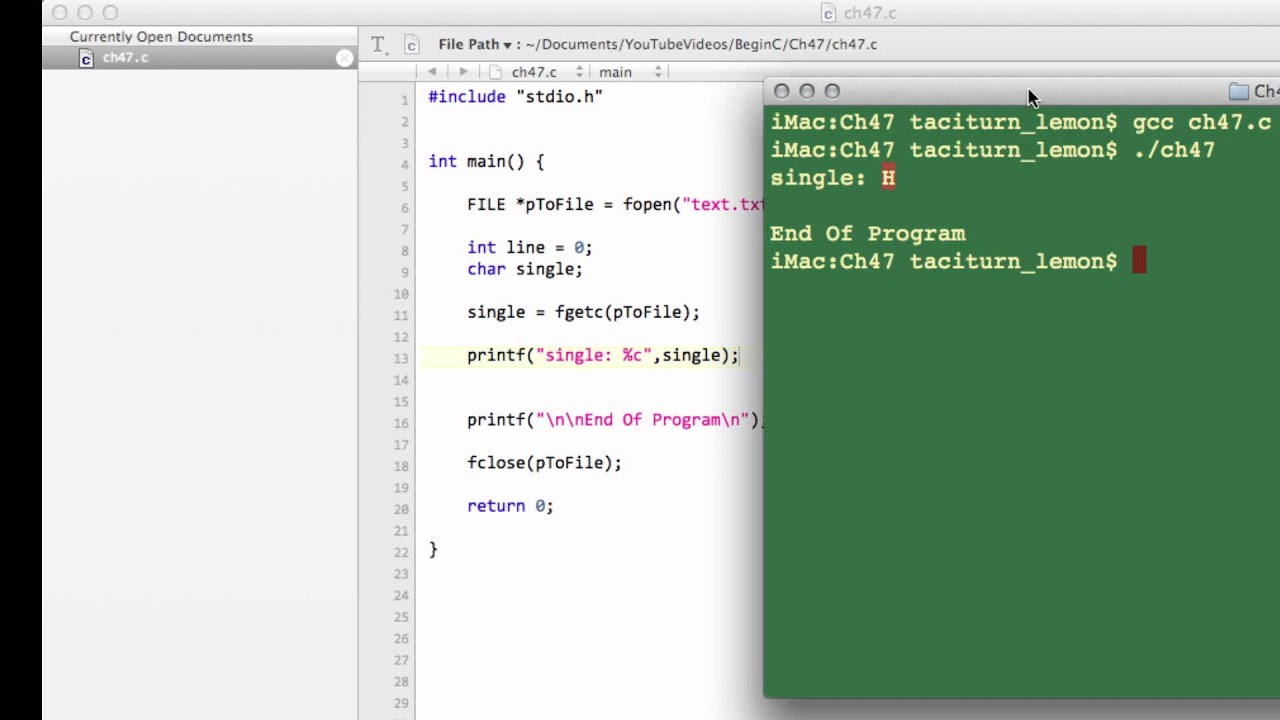
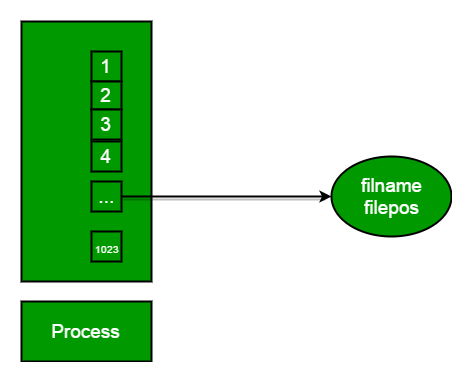
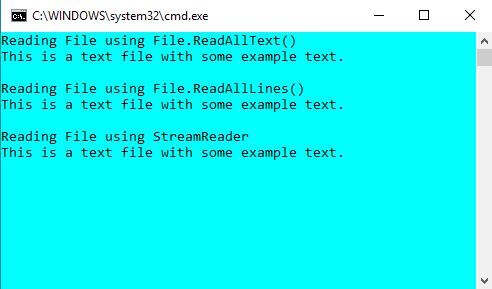




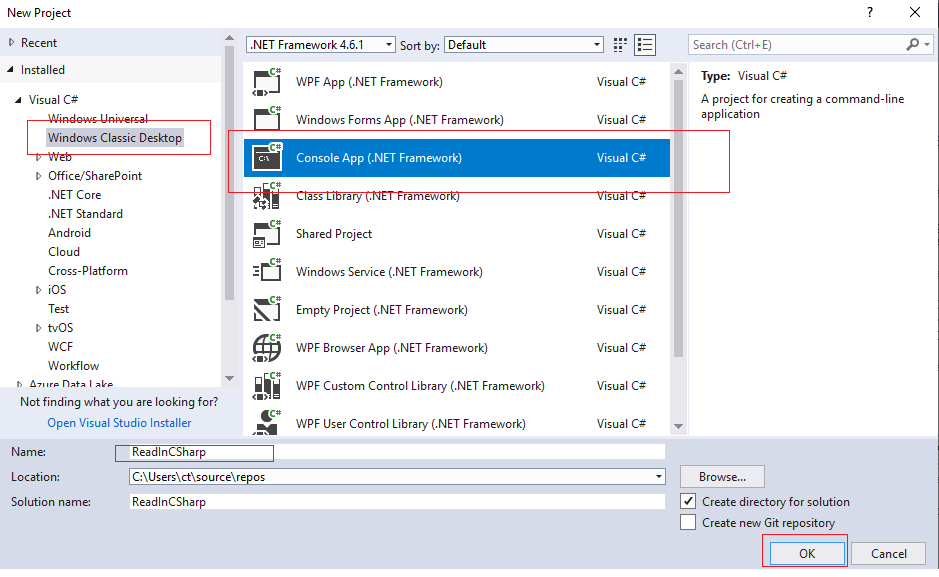
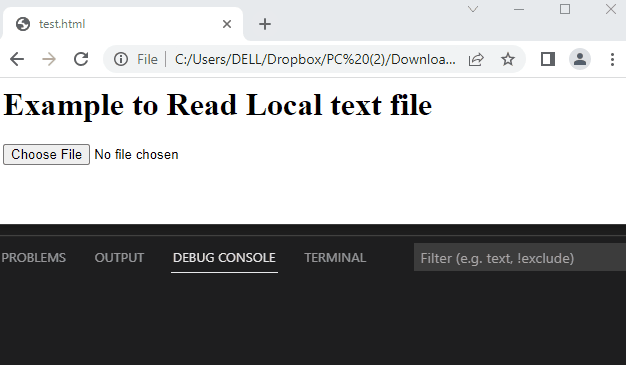
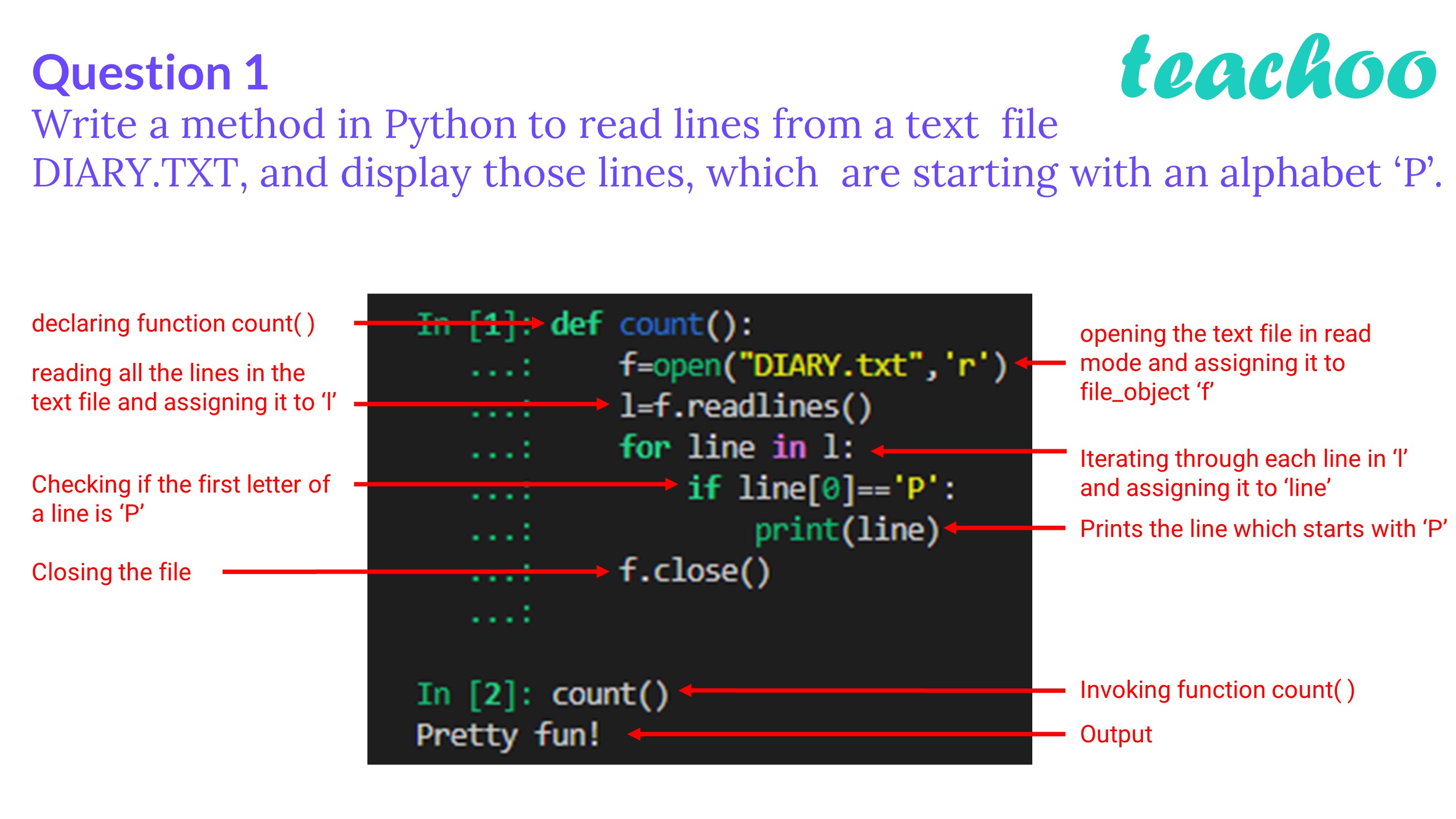
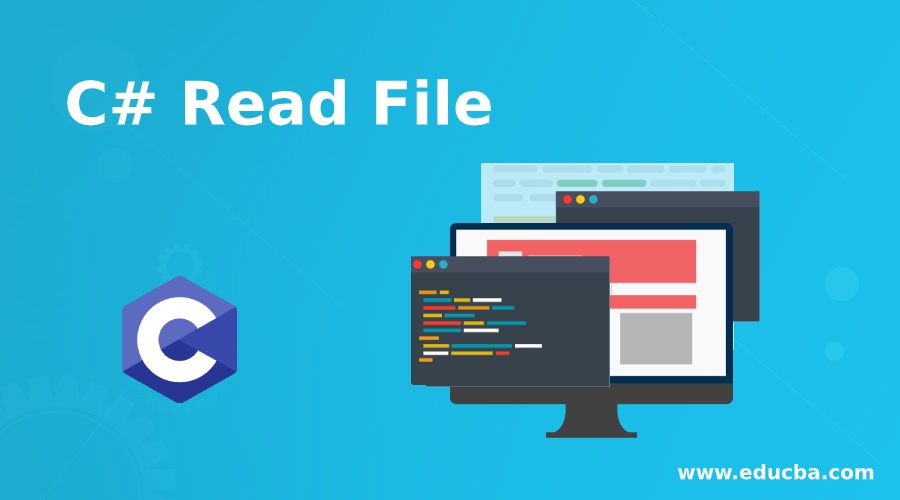


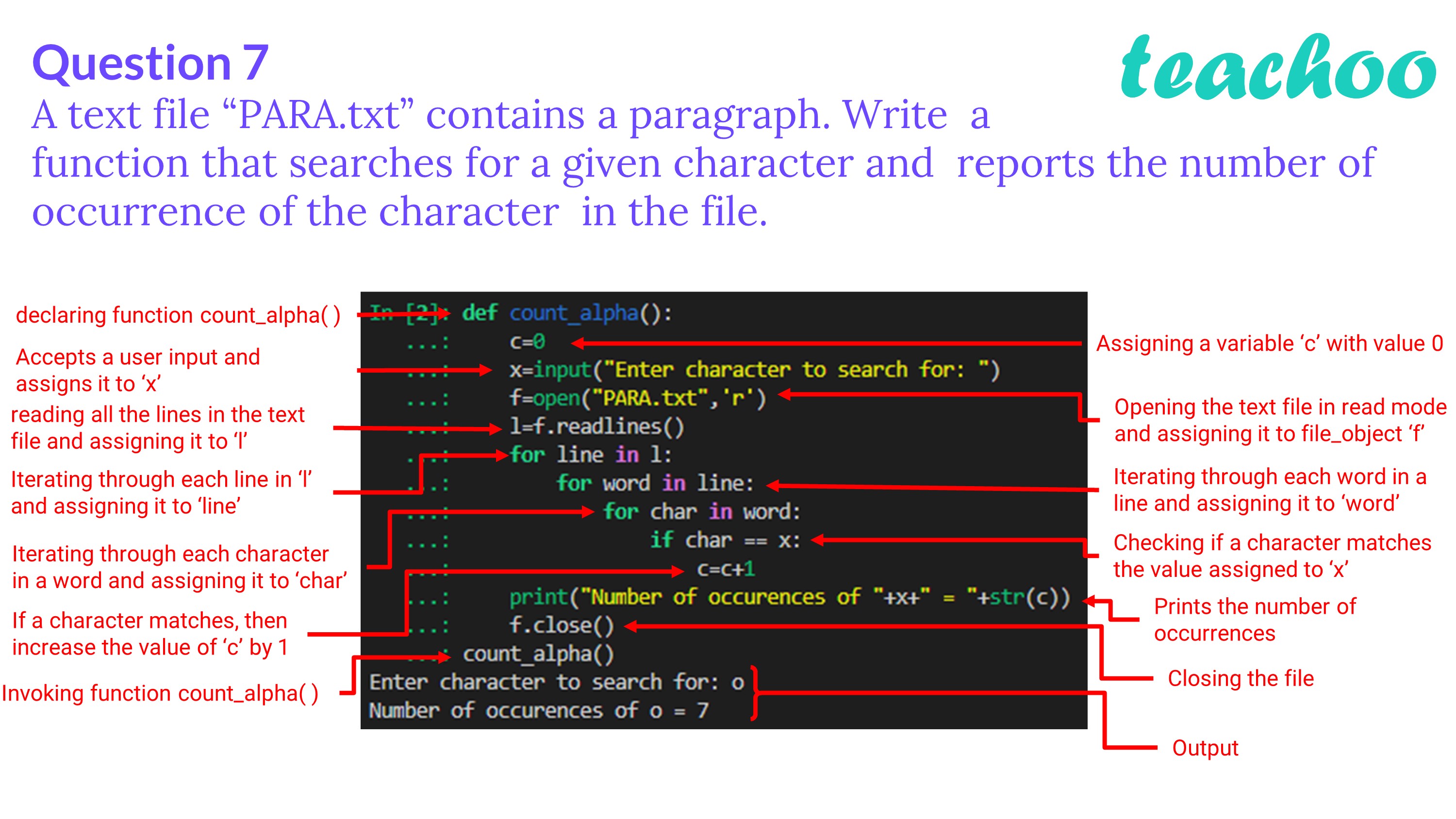
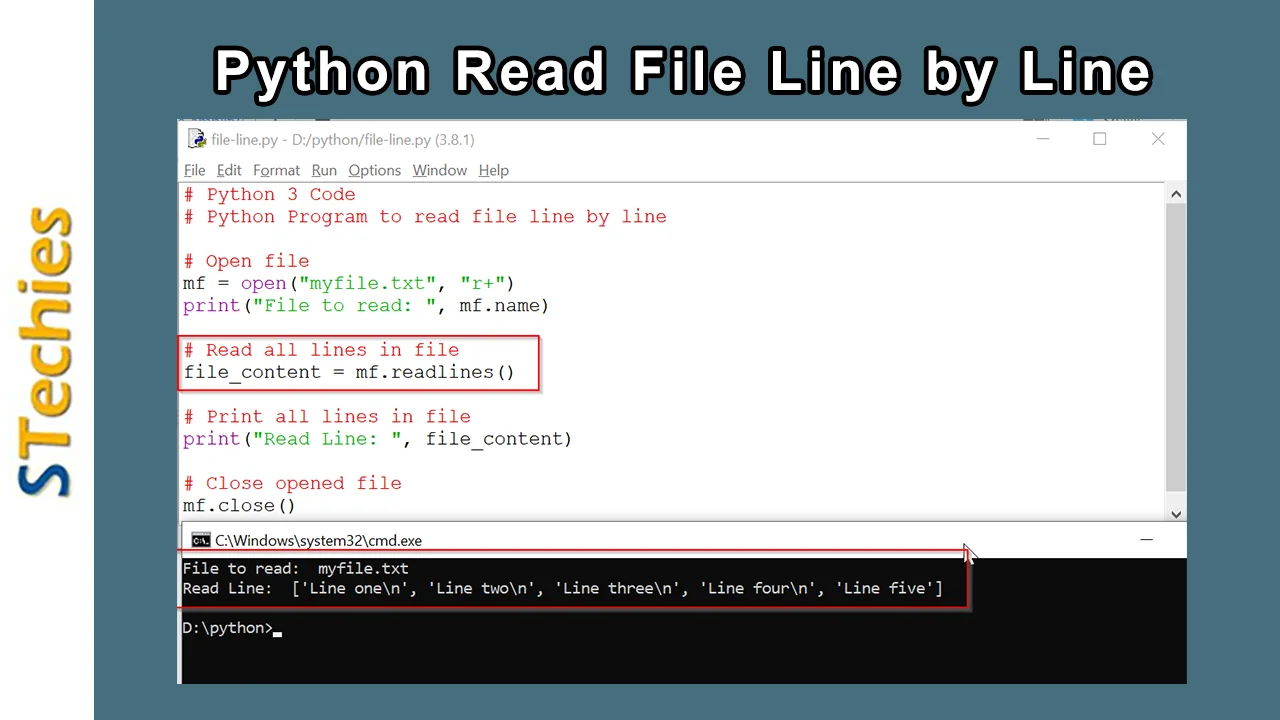
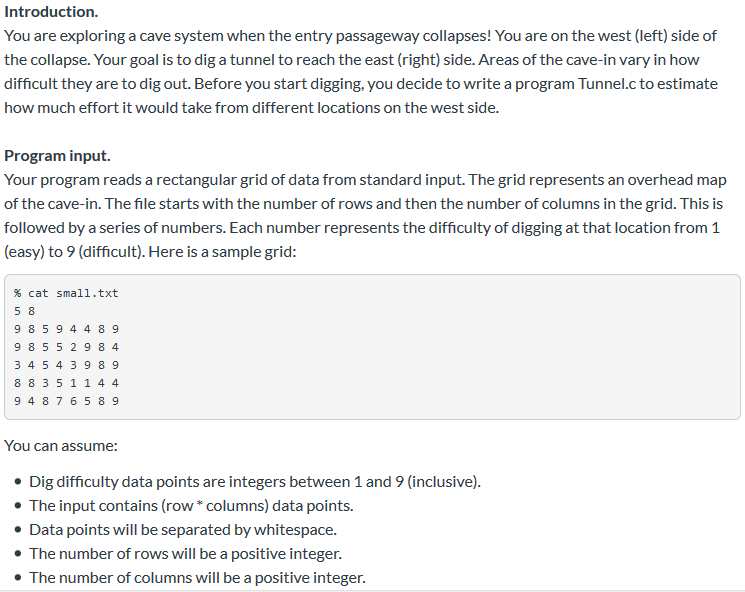

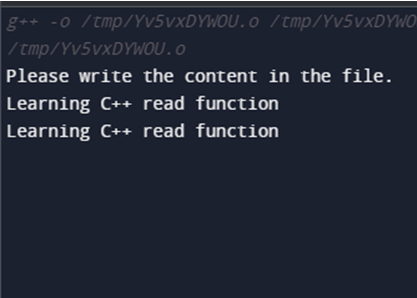

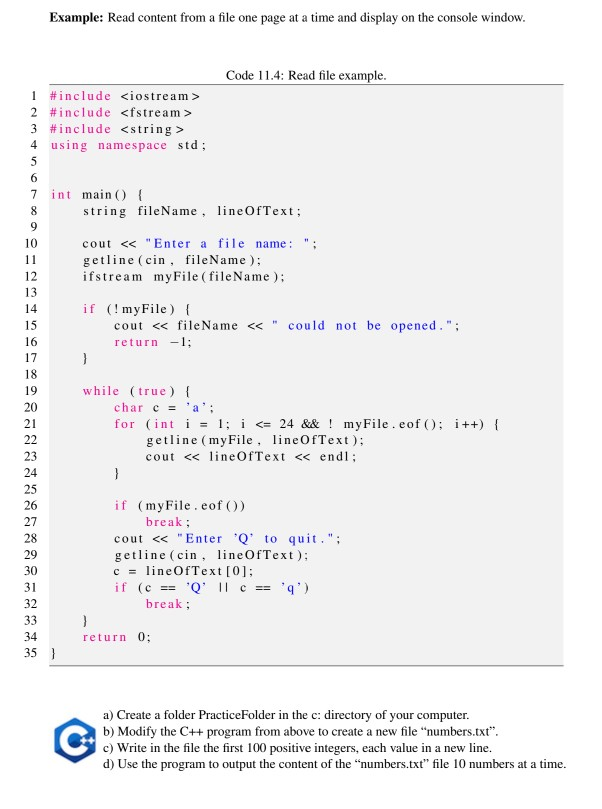


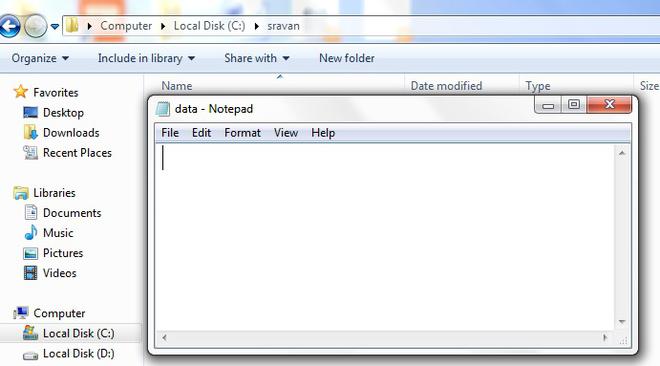
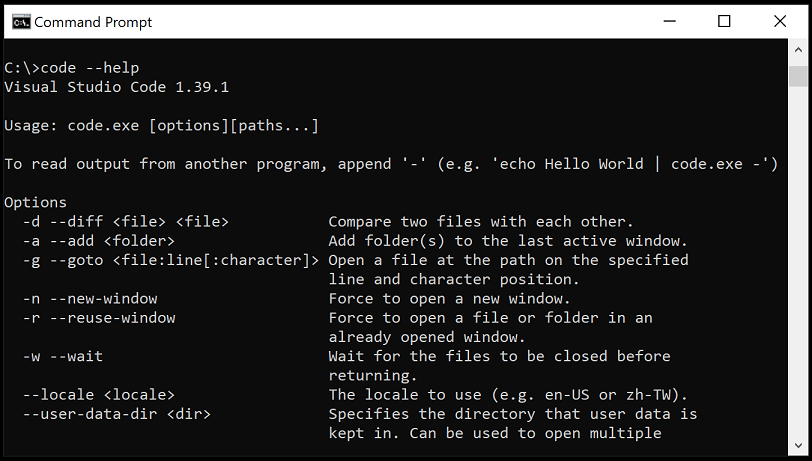

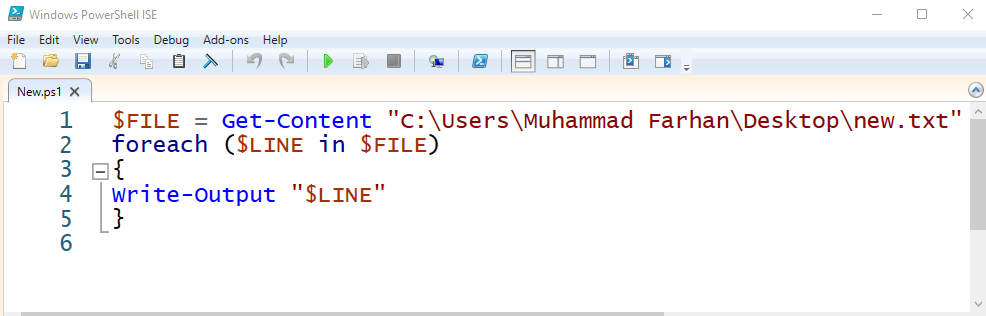
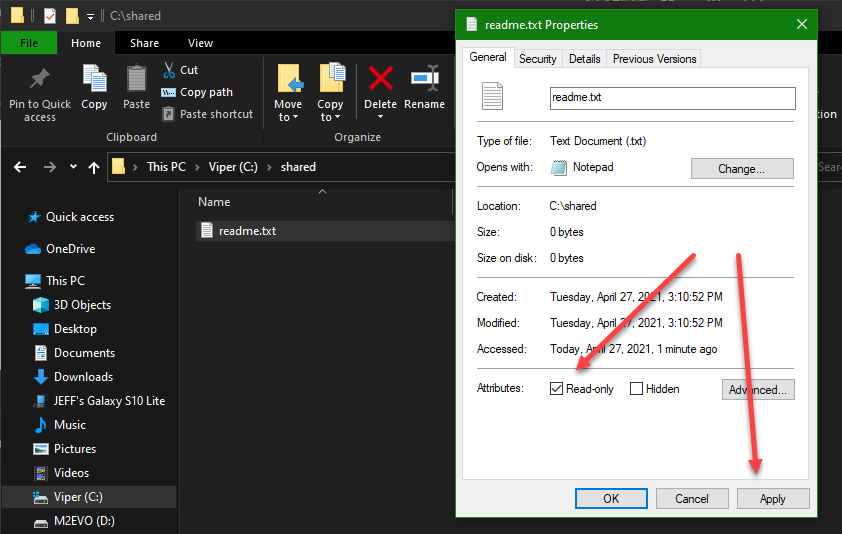
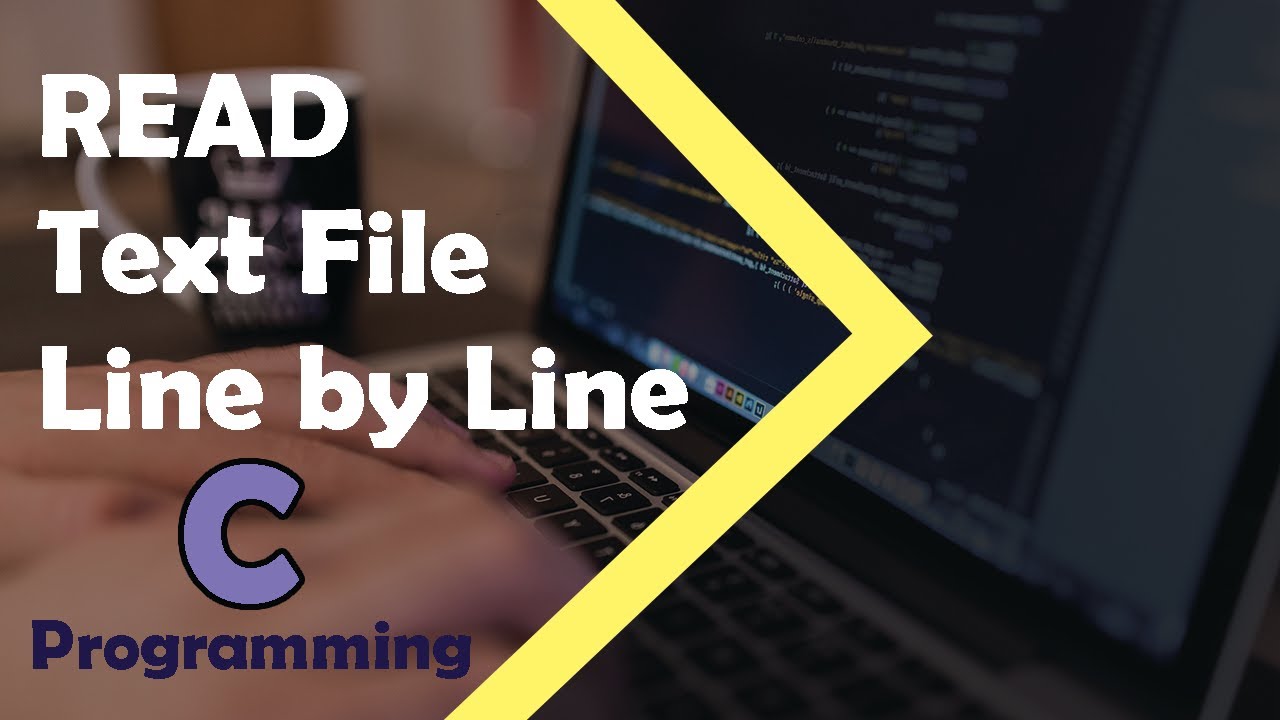





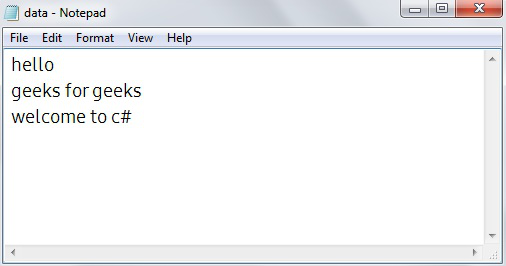
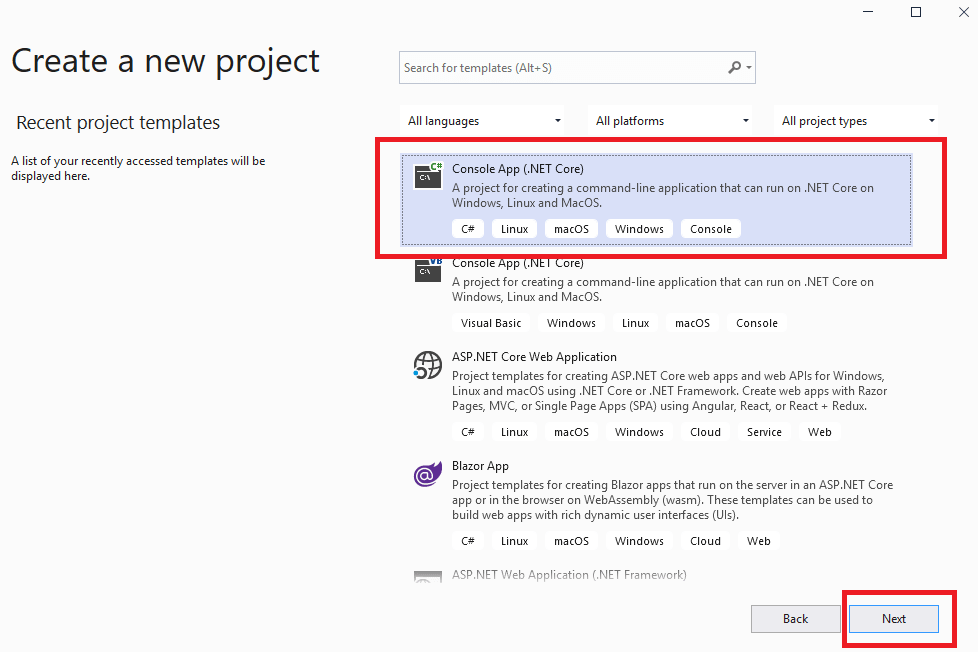
Article link: c++ read file line by line.
Learn more about the topic c++ read file line by line.
- C read file line by line – Stack Overflow
- C Programming – read a file line by line with fgets and getline …
- C Language Tutorial => Read lines from a file
- C program to read a text file line by line – CppBuzz
- C – Read Text File – Tutorial Kart
- C Programming – read a file line by line with fgets and getline …
- C New Lines – W3Schools
- C Strings Input/Output functions – Scaler Topics
- C – File Handling – Read and Write Integers – C Programming
- C Program to read contents of Whole File – GeeksforGeeks
- C Program to Read the First Line From a File – Programiz
See more: https://nhanvietluanvan.com/luat-hoc