C# Length Of List
In C#, a List is a generic type that provides a dynamic, resizable collection of elements. It can hold elements of any type, making it a versatile container for storing and manipulating data. Lists are part of the System.Collections.Generic namespace, and they offer a variety of built-in methods and properties to work with the data they contain.
Declaration and instantiation of a List
To use a List in C#, you need to declare and instantiate it. Here’s how you can do it:
“`csharp
List
“`
The `T` in `List
“`csharp
List
“`
Adding elements to a List
Once you have created a List, you can add elements to it using the `Add` method. Here’s an example:
“`csharp
List
fruits.Add(“apple”);
fruits.Add(“banana”);
fruits.Add(“orange”);
“`
In this example, we create a List of strings called `fruits` and add three different fruits to it.
Accessing elements in a List
To access elements in a List, you can use the index of the element you want to retrieve. Lists use zero-based indexing, which means the first element has an index of 0. Here’s an example:
“`csharp
List
fruits.Add(“apple”);
fruits.Add(“banana”);
fruits.Add(“orange”);
string firstFruit = fruits[0]; // Retrieves the first fruit (apple)
“`
In this example, we retrieve the first fruit from the `fruits` List using the index 0.
Removing elements from a List
Lists provide several methods for removing elements. The commonly used ones are `Remove`, `RemoveAt`, and `RemoveRange`.
– The `Remove` method removes the first occurrence of a specific element from the list:
“`csharp
List
fruits.Remove(“banana”); // Removes the first occurrence of “banana”
“`
– The `RemoveAt` method removes an element at a specified index:
“`csharp
List
fruits.RemoveAt(1); // Removes the element at index 1 (“banana”)
“`
– The `RemoveRange` method removes a range of elements starting from a specified index and count:
“`csharp
List
fruits.RemoveRange(1, 2); // Removes two elements starting from index 1 (“banana”, “orange”)
“`
Checking the length of a List
To determine the length (number of elements) of a List in C#, you can use the `Count` property. Here’s an example:
“`csharp
List
int length = numbers.Count; // Returns the length of the list (5)
“`
In this example, we calculate the length of the `numbers` List using the `Count` property.
Methods to determine the length of a List
In addition to the `Count` property, there are a few other methods you can use to determine the length of a List in C#.
– The `Any` method returns `true` if the List contains at least one element; otherwise, it returns `false`:
“`csharp
List
bool hasElements = fruits.Any(); // Returns true
“`
– The `IsEmpty` method checks if a List is empty (contains no elements):
“`csharp
List
bool isEmpty = emptyList.IsEmpty(); // Returns true
“`
Performance considerations for large Lists
When dealing with large Lists, it’s important to consider the performance implications. Some key points to keep in mind are:
1. Adding elements to the end of a List is generally fast, as the List automatically expands its capacity when necessary.
2. Inserting or removing elements at the beginning or in the middle of a List can be slower, as it requires shifting the subsequent elements.
3. If you frequently need to remove elements from a List based on their index, using a `LinkedList` instead may provide better performance.
4. Accessing elements by index in a List has O(1) complexity, meaning it’s a constant-time operation regardless of the List size.
Common mistakes when dealing with List lengths
When working with List lengths in C#, it’s important to avoid some common mistakes:
1. Forgetting to initialize the List before adding elements: Always instantiate a List using `new List
2. Not checking if a List is empty before accessing its elements: To prevent exceptions, ensure that a List is not empty before trying to access its elements.
3. Failing to update the List length after removing elements: If you remove elements from a List, don’t forget to update its length using the `Count` property.
FAQs
Q: Can a List in C# contain elements of different types?
A: No, a List in C# can only hold elements of a single specified type.
Q: What is the difference between `Remove` and `RemoveAt` methods?
A: The `Remove` method removes the first occurrence of a specific element, while the `RemoveAt` method removes an element at a specific index.
Q: How can I check if a List is empty?
A: You can use the `Any` method, which returns `true` if the List contains at least one element, or the `IsEmpty` method, which checks if the List is empty.
Q: Is it possible to change the length of a List in C#?
A: Yes, Lists in C# are dynamic and resizable, meaning their length can change as you add or remove elements.
In conclusion, understanding how to work with the length of a List in C# is crucial for efficient data manipulation. By correctly instantiating, adding, accessing, and removing elements, and considering performance considerations for large Lists, you can harness the full potential of Lists in your C# applications.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# length of list
Categories: Top 11 C# Length Of List
See more here: nhanvietluanvan.com
Images related to the topic c# length of list
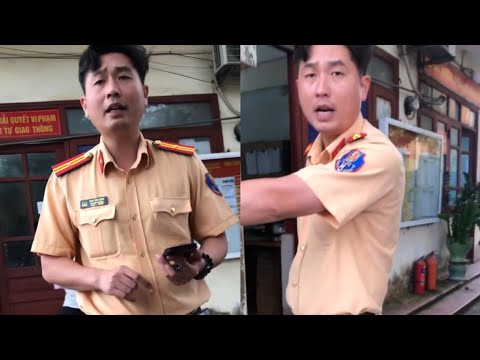
Article link: c# length of list.
Learn more about the topic c# length of list.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- C for Beginners – CodeLearn
See more: https://nhanvietluanvan.com/luat-hoc/