C# List Initialization With Values
In C#, lists provide a dynamic and flexible way to store and manipulate collections of data. One common scenario is initializing a list with values. This article will explore various ways to achieve this initialization, including object initializer syntax, literal values, existing arrays, collections, ranges of values, and query expressions. So let’s dive into the world of C# list initialization with values!
1. Using the Object Initializer Syntax
The object initializer syntax allows you to create and initialize an object in a single concise line of code. This syntax can also be used to initialize a list with values. Here’s an example:
List
In this case, we are creating a new List
2. Initializing a List with Literal Values
Another way to initialize a list with values is by using literal values directly within the list initialization syntax. Here’s an example:
List
In this example, we are creating a new List
3. Initializing a List with an Existing Array
If you have an existing array and want to initialize a list with its values, you can do so by using the List
string[] colors = { “red”, “green”, “blue” };
List
In this example, we are creating an array named “colors” with the values “red”, “green”, and “blue”. Then, we initialize a new List
4. Initializing a List with a Collection
If you have an existing collection, such as another list, you can initialize a new list with its values using the AddRange() method. Here’s an example:
List
List
List
mergedNumbers.AddRange(numbers1);
mergedNumbers.AddRange(numbers2);
In this example, we create two lists named “numbers1” and “numbers2” with their respective values. Then, we initialize a new list named “mergedNumbers” and use the AddRange() method to add the values from both “numbers1” and “numbers2” to the merged list.
5. Initializing a List with a Range of Values
Sometimes, you may need to initialize a list with a range of sequential values. This can be achieved using the Enumerable.Range() method combined with the ToList() method. Here’s an example:
List
In this example, we use the Enumerable.Range() method, which generates a sequence of integers within a specified range, and the ToList() method, which converts the sequence into a list. The resulting list will contain the values from 1 to 10.
6. Initializing a List with a Query Expression
Lastly, you can initialize a list with a query expression, which allows you to perform complex data manipulations and filter the values before initializing the list. Here’s an example:
List
from number in Enumerable.Range(1, 10)
where number % 2 == 0
select number
).ToList();
In this example, we use a query expression to generate a list of even numbers from 1 to 10. The result is a list containing the values 2, 4, 6, 8, and 10.
FAQs
Q: Can I modify the values of a list initialized with values?
A: Yes, you can modify the values of a list initialized with values by accessing them using their index and assigning new values.
Q: Can I use different data types when initializing a list with values?
A: No, the list needs to have a consistent data type. You cannot mix different data types when initializing a list, as it expects a single type.
Q: Can I initialize a list with duplicate values?
A: Yes, you can initialize a list with duplicate values. The list allows for duplicate entries and maintains the order of insertion.
Q: What are the advantages of using list initialization with values?
A: List initialization with values allows you to quickly and easily populate a list with the desired values. It is a concise and readable way to define the initial content of a list.
In conclusion, initializing a list with values in C# provides various ways to quickly populate lists with the desired values. Whether using object initializer syntax, literal values, existing arrays, collections, ranges of values, or query expressions, you have the flexibility to choose the approach that best suits your needs. By understanding these different methods, you can efficiently initialize and work with lists in your C# applications.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# list initialization with values
Categories: Top 28 C# List Initialization With Values
See more here: nhanvietluanvan.com
Images related to the topic c# list initialization with values
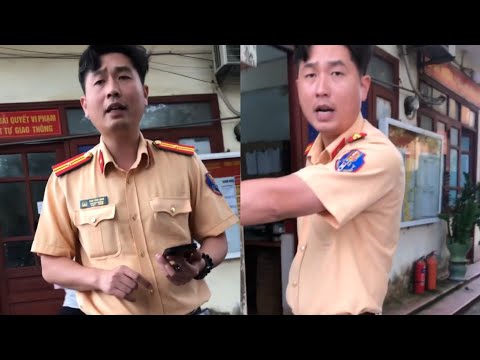
Article link: c# list initialization with values.
Learn more about the topic c# list initialization with values.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ C là gì? Code ví dụ Hello World – CodeCute
See more: https://nhanvietluanvan.com/luat-hoc/