Check If Key Exists In Dict Python
Overview:
Checking if a key exists in a dictionary is a common operation when working with Python dictionaries. In this article, we will explore various methods to accomplish this task. We will cover using the ‘in’ operator, the ‘get()’ method, the ‘keys()’ method, and the ‘has_key()’ method (in legacy versions). We will also discuss best practices for efficiently checking key existence, handling exceptions, and provide some key takeaways.
Using the ‘in’ operator to check for key existence:
The ‘in’ operator is a simple and straightforward way to check if a key exists in a dictionary. It returns a boolean value, ‘True’ if the key is present, and ‘False’ otherwise. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict:
print(“Key exists!”)
else:
print(“Key does not exist.”)
“`
Using the ‘get()’ method to check for key existence:
The ‘get()’ method in Python dictionaries allows us to retrieve the value associated with a given key. However, it can also be used to check for key existence. If the key is present, ‘get()’ returns the corresponding value. If the key is not found, it returns ‘None’ (by default) or a default value specified as the second argument. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
name = my_dict.get(‘name’)
if name is not None:
print(“Key exists!”)
else:
print(“Key does not exist.”)
“`
Using the ‘keys()’ method to check for key existence:
The ‘keys()’ method allows us to obtain a list of all the keys present in a dictionary. We can utilize this functionality to check if a particular key exists. By checking if the desired key is present in the returned list, we can determine its existence. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘name’ in my_dict.keys():
print(“Key exists!”)
else:
print(“Key does not exist.”)
“`
Using the ‘has_key()’ method to check for key existence (in legacy versions):
In legacy versions of Python (2.x), dictionaries had a ‘has_key()’ method. This method returns a boolean value indicating whether the specified key exists in the dictionary or not. However, this method has been removed in Python 3.x due to its redundancy with the ‘in’ operator. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.has_key(‘name’):
print(“Key exists!”)
else:
print(“Key does not exist.”)
“`
Best practices for efficiently checking key existence in dictionaries:
When dealing with large dictionaries, it is important to consider efficiency. Here are a few best practices to improve performance:
1. Use the ‘in’ operator or ‘get()’ method instead of ‘keys()’ for checking key existence, as it avoids unnecessary list creation.
2. Prefer the ‘in’ operator over the ‘get()’ method if you don’t need the corresponding value.
3. When repeatedly checking for key existence, store the dictionary keys in a separate set for faster lookup.
Handling exceptions when checking for key existence:
In certain cases, it may be appropriate to handle exceptions when checking for key existence. For example, you may want to perform a specific action if the key is present or absent. Here’s an example using a try-except block:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
value = my_dict[‘name’]
print(“Key exists: ” + value)
except KeyError:
print(“Key does not exist.”)
“`
Summary and Key Takeaways:
Checking if a key exists in a Python dictionary is a common task. In this article, we explored different methods to accomplish this, including using the ‘in’ operator, the ‘get()’ method, the ‘keys()’ method, and the ‘has_key()’ method (in legacy versions). We also discussed best practices for efficient key existence checking and handling exceptions. Remember to choose appropriate methods based on your specific requirements and consider efficiency when dealing with large dictionaries.
FAQs:
Q1: How can I check if a key does not exist in a dictionary in Python?
A1: You can check if a key does not exist in a dictionary by using the ‘not’ operator together with the ‘in’ operator. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘surname’ not in my_dict:
print(“Key does not exist!”)
“`
Q2: How can I iterate over key-value pairs in a Python dictionary?
A2: To iterate over key-value pairs in a Python dictionary, you can use a ‘for’ loop combined with the ‘items()’ method. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(key, value)
“`
Q3: How can I add a value to a key in a Python dictionary?
A3: You can add a value to a key in a Python dictionary by simply assigning the value to the key. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict[‘surname’] = ‘Doe’
print(my_dict)
“`
Q4: How can I change the value of a key in a Python dictionary?
A4: You can change the value of a key in a Python dictionary by assigning a new value to the key. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict[‘age’] = 30
print(my_dict)
“`
In conclusion, checking if a key exists in a dictionary is a fundamental operation in Python. By utilizing the ‘in’ operator, ‘get()’ method, ‘keys()’ method, or ‘has_key()’ method, you can easily determine the presence of a key. Additionally, following best practices and handling exceptions appropriately will help you write more efficient and reliable code.
How To Check If A Key Exists In A Python Dictionary?
How To Check If Dict Key Exists In Python?
In Python, dictionaries are used to store data in key-value pairs. Each key in a dictionary must be unique, and it is associated with a value. When working with dictionaries, it is common to check if a given key exists or not. In this article, we will explore different methods to determine the presence of a key in a dictionary.
Methods to Check if a Dict Key Exists:
1. Using the “in” Keyword:
The most common and straightforward method to check for the existence of a key in a dictionary is by using the “in” keyword. This keyword allows you to check if a specific key is present in the dictionary. For example:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
if “name” in my_dict:
print(“The key ‘name’ exists in the dictionary.”)
else:
print(“The key ‘name’ does not exist in the dictionary.”)
“`
Output:
The key ‘name’ exists in the dictionary.
2. Using the “get()” Method:
Another method to verify the presence of a key in a dictionary is by using the “get()” method. This method returns the value associated with the given key if it exists in the dictionary. If the key is not found, it returns a default value, which is None by default. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
key = “name”
value = my_dict.get(key)
if value is not None:
print(f”The key ‘{key}’ exists in the dictionary with value ‘{value}’.”)
else:
print(f”The key ‘{key}’ does not exist in the dictionary.”)
“`
Output:
The key ‘name’ exists in the dictionary with value ‘John’.
3. Using Exception Handling:
Python allows you to handle KeyError exceptions that occur when trying to access a non-existent key. By using the try-except block, you can catch the KeyError and conclude whether the key exists or not. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
key = “name”
try:
value = my_dict[key]
print(f”The key ‘{key}’ exists in the dictionary.”)
except KeyError:
print(f”The key ‘{key}’ does not exist in the dictionary.”)
“`
Output:
The key ‘name’ exists in the dictionary.
4. Using the “keys()” Method:
The “keys()” method in Python returns a list of all the keys present in a dictionary. By checking if a specific key is in the resulting list, you can determine if it exists or not. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
key = “name”
if key in my_dict.keys():
print(f”The key ‘{key}’ exists in the dictionary.”)
else:
print(f”The key ‘{key}’ does not exist in the dictionary.”)
“`
Output:
The key ‘name’ exists in the dictionary.
FAQs:
Q1: Can a dictionary have multiple keys with the same name?
A1: No, in Python, dictionary keys must be unique. If you try to add a duplicate key to a dictionary, the new value will overwrite the old value associated with that key.
Q2: What happens if I try to access a non-existent key using the “get()” method?
A2: If you use the “get()” method to access a non-existent key in a dictionary, it will return the default value specified (None by default).
Q3: Is it possible to check if a value exists in a dictionary?
A3: Yes, it is possible to check if a value exists in a dictionary using the “in” keyword or by iterating over the dictionary’s values.
Q4: What is the best method to check for the existence of a key in a dictionary?
A4: The “in” keyword method is the most commonly used and recommended method to check if a key exists in a dictionary due to its simplicity and readability.
Q5: Are there any performance differences among the different methods?
A5: While there may be minor performance differences between different methods, they are generally negligible for most use cases. The choice of method should primarily be based on code readability and clarity.
In conclusion, checking if a key exists in a dictionary in Python is a common task when working with data. By using the “in” keyword, the “get()” method, exception handling, or the “keys()” method, you can easily determine the presence of a key in a dictionary. Choose the method that best suits your needs and coding style to efficiently handle dictionaries in your Python programs.
How To Check If Key-Value Exists In List Of Dictionaries Python?
In Python, dictionaries are a powerful data structure used to present data in a key-value format. Sometimes, you may come across scenarios where you need to check if a specific key-value pair exists in a list of dictionaries. In this article, we will explore various methods to accomplish this task and provide in-depth explanations to help you understand the concepts.
Checking for key-value existence is a common requirement in many programming tasks. For instance, you might have a list of dictionaries that represent the details of multiple persons, and you want to check if a specific person with a specific name exists in the list. By learning how to carry out these checks efficiently, you can enhance your Python programming skills and make your code more robust.
Method 1: Using a for loop
The simplest approach to check the existence of a key-value pair in a list of dictionaries is by using a for loop. Let’s assume we have a list of dictionaries called “people” and we want to check if a dictionary with the key “name” and value “John” exists in the list. Below is an example of how this can be done:
“`python
people = [
{“name”: “John”, “age”: 28},
{“name”: “Alice”, “age”: 32},
{“name”: “Bob”, “age”: 25}
]
for person in people:
if person.get(“name”) == “John”:
print(“Key-value pair exists!”)
break
“`
In this code snippet, we iterate through each dictionary in the list “people” using a for loop. Within the loop, we use the `get()` method to retrieve the value associated with the key “name” for each dictionary. If the retrieved value matches our desired value “John”, we print a message indicating that the key-value pair exists and then break out of the loop.
Method 2: Using the any() function
The `any()` function can be a handy tool when checking the existence of a key-value pair in a list of dictionaries. This function takes an iterable (such as a list) as its argument and returns `True` if any of the elements in the iterable satisfy a specified condition. Here’s an example demonstrating its usage:
“`python
people = [
{“name”: “John”, “age”: 28},
{“name”: “Alice”, “age”: 32},
{“name”: “Bob”, “age”: 25}
]
check_exists = any(person.get(“name”) == “John” for person in people)
if check_exists:
print(“Key-value pair exists!”)
“`
In the above code, we use a generator expression inside the `any()` function. The generator expression iterates through each dictionary in the list and checks if the value associated with the key “name” is equal to “John”. If any dictionary satisfies this condition, the `any()` function returns `True`, indicating the existence of the key-value pair.
Method 3: Using list comprehension
List comprehension is another efficient way to check for the existence of a key-value pair in a list of dictionaries. Let’s modify the example we previously used to incorporate this method:
“`python
people = [
{“name”: “John”, “age”: 28},
{“name”: “Alice”, “age”: 32},
{“name”: “Bob”, “age”: 25}
]
check_exists = any(person.get(“name”) == “John” for person in people)
if check_exists:
print(“Key-value pair exists!”)
“`
In this code snippet, we utilize a similar generator expression as in the previous example. However, instead of passing it directly to the `any()` function, we enclose it within square brackets to transform it into a list. By doing so, we can apply additional list operations if needed, such as getting the index of the matching dictionary or extracting its properties.
FAQs
Q1. What if I want to check for the existence of multiple key-value pairs in the same dictionary?
If you want to check multiple key-value pairs within the same dictionary, you can extend the condition in the generator expression or for loop with logical operators like `and` or `or`. For example:
“`python
for person in people:
if person.get(“name”) == “John” and person.get(“age”) == 28:
print(“Key-value pair exists!”)
break
“`
In this code snippet, we use the `and` operator to combine two conditions: “name” equals “John” and “age” equals 28. The code will print the message if both conditions are satisfied.
Q2. Is there any built-in Python function to directly search for key-value pairs in dictionaries?
No, there is no exact built-in function that allows direct searching for key-value pairs in a list of dictionaries. However, you can use the methods we discussed earlier, such as using a for loop, the `any()` function, or list comprehension, to achieve similar results efficiently.
In conclusion, checking for the existence of a key-value pair in a list of dictionaries is a common task in Python programming. By utilizing the methods presented in this article, such as using a for loop, the `any()` function, or list comprehension, you can easily accomplish this task and make your code more efficient and robust. Remember to choose the method that suits your requirements best, and feel free to experiment with different techniques to further enhance your Python skills.
Keywords searched by users: check if key exists in dict python Check key in dict Python, Check if key not exists in dictionary Python, For key-value in dict Python, How to check if value exists in python, Python dict get value if key exists, Check value in dictionary Python, Add value to key dictionary Python, Change value in dictionary Python
Categories: Top 13 Check If Key Exists In Dict Python
See more here: nhanvietluanvan.com
Check Key In Dict Python
Python is a widely used programming language known for its ease of use and versatility. One of its key features is the ability to efficiently store and retrieve data using dictionaries. Dictionaries are unordered collections of key-value pairs, where each key is unique. The values can be of any data type, ranging from integers and strings to more complex objects. As a Python programmer, it is essential to understand various aspects of dictionary manipulation. In this article, we will focus on the concept of checking whether a key exists in a Python dictionary.
Understanding Dictionary Keys
Before we dive into the methods of checking for keys in a dictionary, it is vital to understand the nature of keys in Python dictionaries. As mentioned earlier, keys in dictionaries must be unique. Additionally, they must be immutable objects, meaning they cannot be changed once they are assigned. Common examples of immutable objects in Python include integers, strings, and tuples. On the other hand, mutable objects like lists, sets, and other dictionaries cannot be used as keys. Attempting to use mutable objects as keys will result in a TypeError.
Using the `in` Operator
The simplest way to check for a key in a dictionary is by using the `in` operator. The operator returns a boolean value indicating whether the specified key exists in the dictionary. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
print(‘name’ in my_dict) # Output: True
print(‘country’ in my_dict) # Output: False
“`
In the above code, we create a dictionary `my_dict` and check for the existence of the keys `’name’` and `’country’` using the `in` operator. The output is `True` for the existing key `’name’` and `False` for the non-existing key `’country’`.
Using the `get` method
Python provides us with another built-in method, `get()`, which allows us to check for keys in a dictionary. The `get()` method takes two arguments – the key we want to check and an optional default value. If the key exists in the dictionary, `get()` returns the corresponding value. Otherwise, it returns the default value, which is `None` if not specified. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
print(my_dict.get(‘name’)) # Output: John
print(my_dict.get(‘country’)) # Output: None
print(my_dict.get(‘country’, ‘Unknown’)) # Output: Unknown
“`
In the above code, we use the `get()` method to check for the keys `’name’` and `’country’` in the dictionary `my_dict`. We get the respective values `’John’` and `None`. In the third `get()` call, we provide a default value `’Unknown’`, which gets returned as the key `’country’` does not exist in the dictionary.
Using Exception Handling
Another approach to check for keys is by using exception handling. Python allows us to catch `KeyError` exceptions that occur when trying to access a non-existing key in a dictionary. By using a `try-except` block, we can handle the exception and execute alternative code if the key is not found. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
print(my_dict[‘name’]) # Output: John
print(my_dict[‘country’]) # KeyError: ‘country’
except KeyError:
print(‘Key not found’)
“`
In the above code, we attempt to access the keys `’name’` and `’country’` in the dictionary `my_dict`. Since `’name’` exists, it successfully prints `’John’`. However, trying to access the non-existing key `’country’` raises a `KeyError` exception, which is caught by the `except` block. In turn, the code within the `except` block is executed, which prints `’Key not found’`.
FAQs:
Q1. Can a dictionary have duplicate keys?
A1. No, a dictionary cannot have duplicate keys. Each key must be unique.
Q2. What happens if we try to add a duplicate key to a dictionary?
A2. When a duplicate key is added to a dictionary, the latter value associated with the key will overwrite the former value.
Q3. How can I remove a key-value pair from a dictionary?
A3. You can utilize the `del` statement or the `pop()` method to remove a specific key-value pair from a dictionary.
Q4. Can I use a variable as a key in a dictionary?
A4. Yes, you can use variables as keys in dictionaries as long as the variable’s value is an immutable data type.
In conclusion, checking for the existence of keys in Python dictionaries is a fundamental task in dictionary manipulation. Whether you use the `in` operator, the `get()` method, or exception handling, Python provides various mechanisms to perform key checks efficiently. By understanding these methods, you can unleash the full potential of dictionaries in your Python programs.
Check If Key Not Exists In Dictionary Python
In Python, dictionaries are widely used data structures that store key-value pairs. They provide a flexible and efficient way to access and manipulate data. When working with dictionaries, it is often necessary to determine if a specific key does not exist within the dictionary. In this article, we will explore various methods to check if a key does not exist in a Python dictionary.
Method 1: Using the ‘in’ operator
The ‘in’ operator can be used to check if a key is present or not in a dictionary. By placing the key in question to the left of the ‘in’ keyword and the dictionary to the right, we can determine if the key exists within the dictionary. If the key exists, the operator will return True; otherwise, it will return False. Here’s an example:
“`
my_dict = {‘A’: 1, ‘B’: 2, ‘C’: 3}
if ‘D’ not in my_dict:
print(“Key does not exist”)
“`
In this example, we define a dictionary called ‘my_dict’ with three key-value pairs. Using the ‘in’ operator with the key ‘D’, we check if it exists in the dictionary. Since ‘D’ is not a key in the dictionary, the condition evaluates to True, and the corresponding message is printed.
Method 2: Using the ‘get()’ method
The ‘get()’ method provides a convenient way to check if a key exists in a dictionary. If the key is found, it returns the associated value; otherwise, it returns None. By default, if the key is not found, the ‘get()’ method returns None. We can specify a default value as an optional parameter to be returned instead of None. Here’s an example:
“`
my_dict = {‘A’: 1, ‘B’: 2, ‘C’: 3}
if my_dict.get(‘D’) is None:
print(“Key does not exist”)
“`
In this example, we use the ‘get()’ method to retrieve the value associated with the key ‘D’. Since ‘D’ is not a key in the dictionary, the ‘get()’ method returns None, and the condition evaluates to True, resulting in the expected output.
Method 3: Using exception handling
Python provides an alternative approach to check if a key does not exist using exception handling. We can use a try-except block where the try block attempts to access the key and the except block handles the case when the key is not present. Here’s an example:
“`
my_dict = {‘A’: 1, ‘B’: 2, ‘C’: 3}
try:
value = my_dict[‘D’]
except KeyError:
print(“Key does not exist”)
“`
In this example, we try to access the value associated with the key ‘D’ directly using the square bracket notation. If the key is not present in the dictionary, a KeyError exception is raised, which we catch in the except block and print the corresponding message.
Method 4: Using the ‘not’ operator with ‘in’ operator
We can also use the ‘not’ operator along with the ‘in’ operator to check if a key does not exist in a dictionary. By negating the result of the ‘in’ operator, we can test whether a key does not exist. Here’s an example:
“`
my_dict = {‘A’: 1, ‘B’: 2, ‘C’: 3}
if not (‘D’ in my_dict):
print(“Key does not exist”)
“`
In this example, we negate the result of the ‘in’ operator by prepending the ‘not’ operator. If the key ‘D’ is not present in the dictionary, the condition evaluates to True, and the corresponding message is printed.
FAQs:
Q1. Why is it important to check if a key does not exist in a dictionary?
A1. Checking if a key does not exist allows for error handling and prevents potential issues when accessing nonexistent keys. It ensures that the program does not raise exceptions or produce unexpected results.
Q2. How can I check if a key not exists in a nested dictionary in Python?
A2. To check if a key exists in a nested dictionary, you can use the ‘in’ operator or the ‘get()’ method with each level of nesting until you reach the desired key. For example:
“`
nested_dict = {‘A’: {‘B’: {‘C’: 1}}}
if ‘C’ not in nested_dict[‘A’][‘B’]:
print(“Key does not exist”)
“`
Q3. Which is the most efficient method to check if a key does not exist in a dictionary?
A3. The ‘in’ operator is generally considered to be the most efficient method to check if a key does not exist in a dictionary. It has a time complexity of O(1) on average, as it uses hash tables for fast key lookup.
Q4. Does the ‘not in’ operator also work for values in a dictionary?
A4. No, the ‘not in’ operator is specifically used to check if a key does not exist in a dictionary. To check for the absence of a value, you can iterate over the dictionary’s values using a loop or use the built-in ‘values()’ method and apply the ‘not in’ operator accordingly.
In conclusion, checking if a key does not exist in a dictionary is a common operation in Python. This article has provided various methods using the ‘in’ operator, ‘get()’ method, exception handling, and the ‘not’ operator to accomplish this task efficiently. By understanding and utilizing these methods, you can ensure proper handling of dictionaries and avoid potential errors when interacting with data stored as key-value pairs.
Images related to the topic check if key exists in dict python
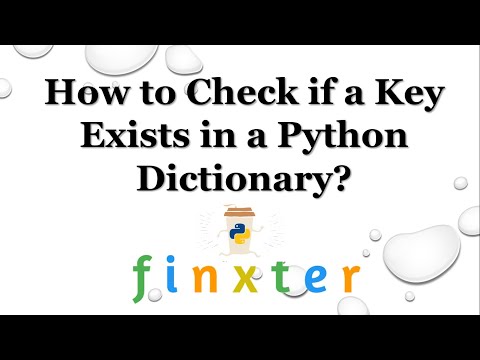
Found 25 images related to check if key exists in dict python theme
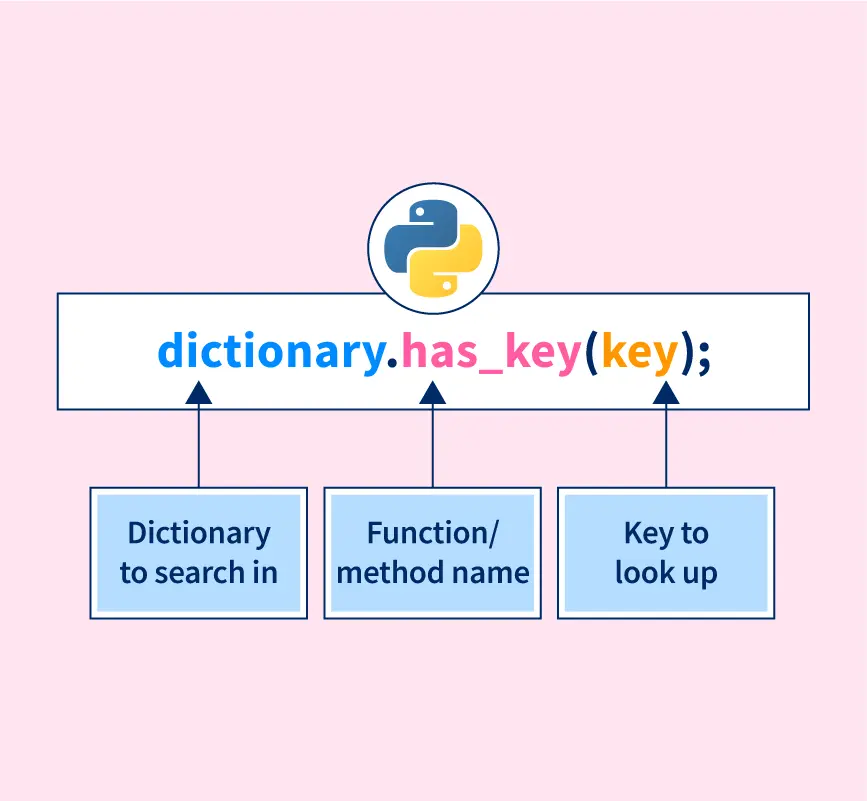
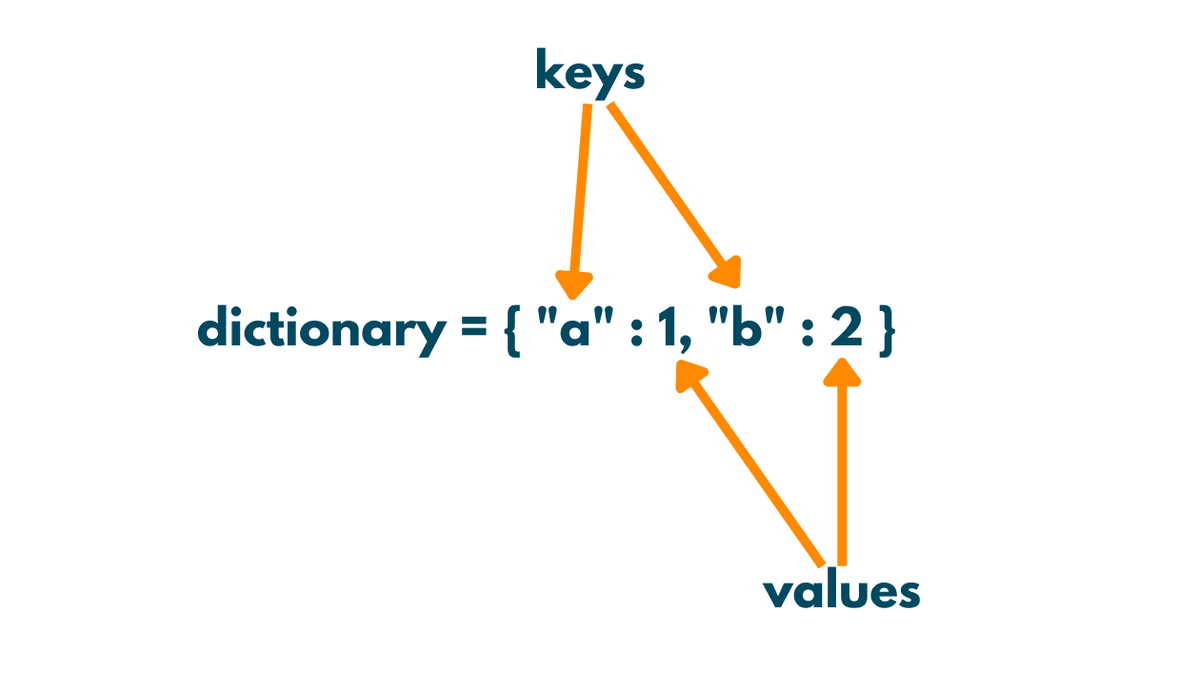

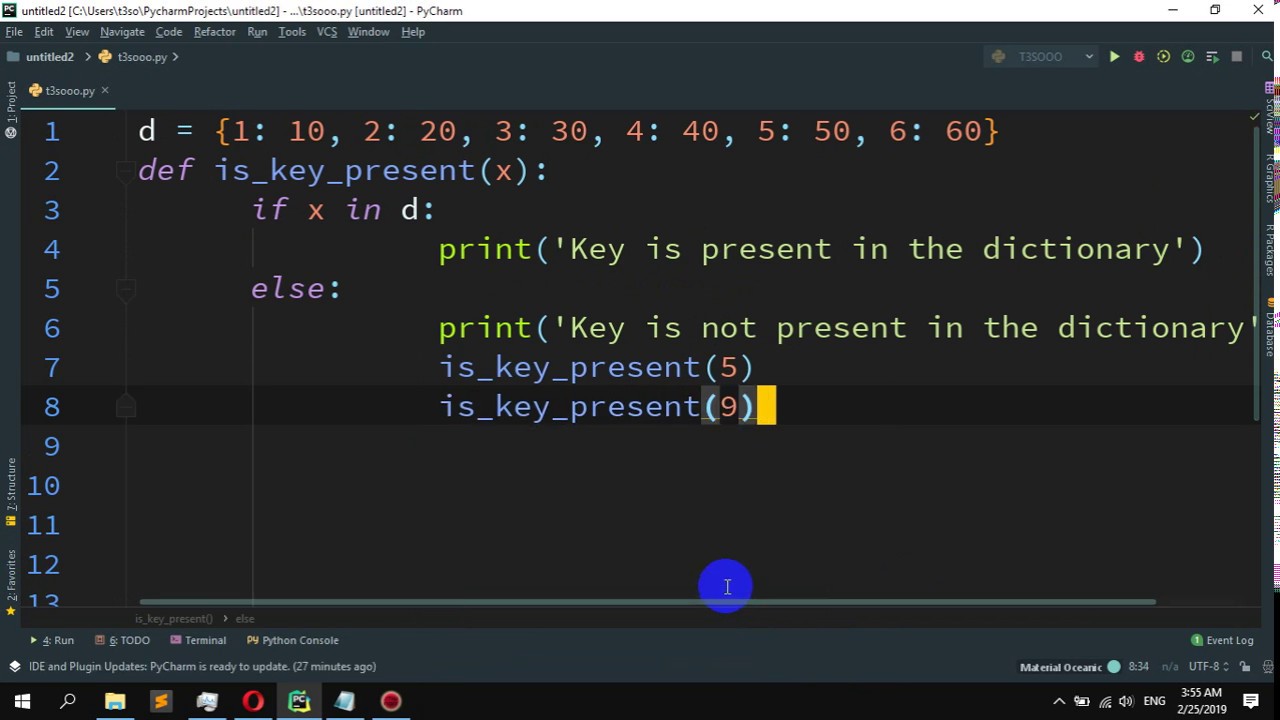
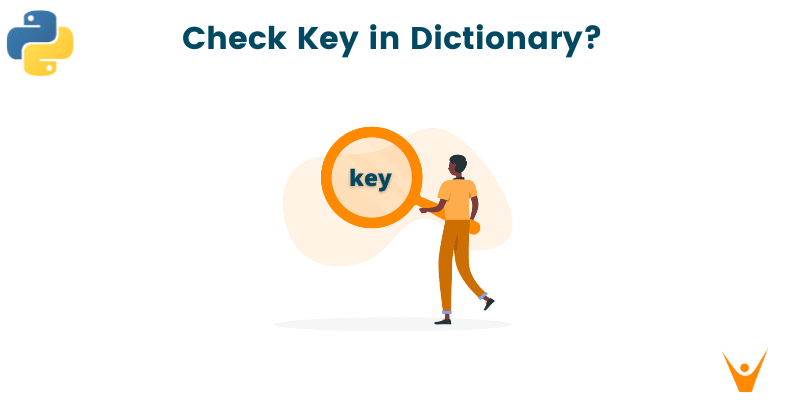
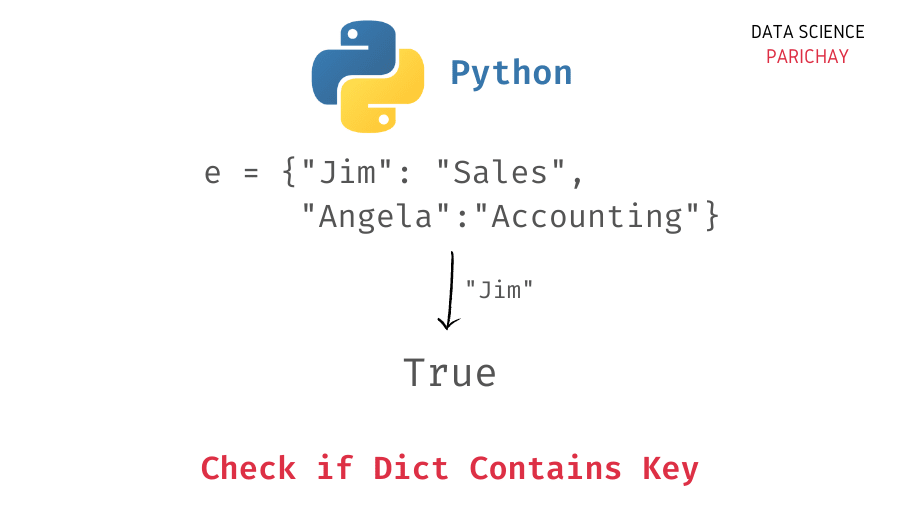

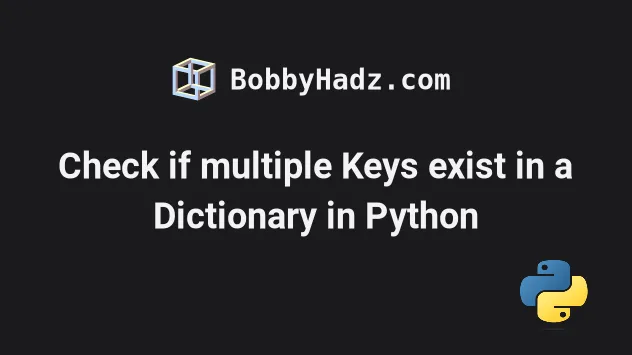
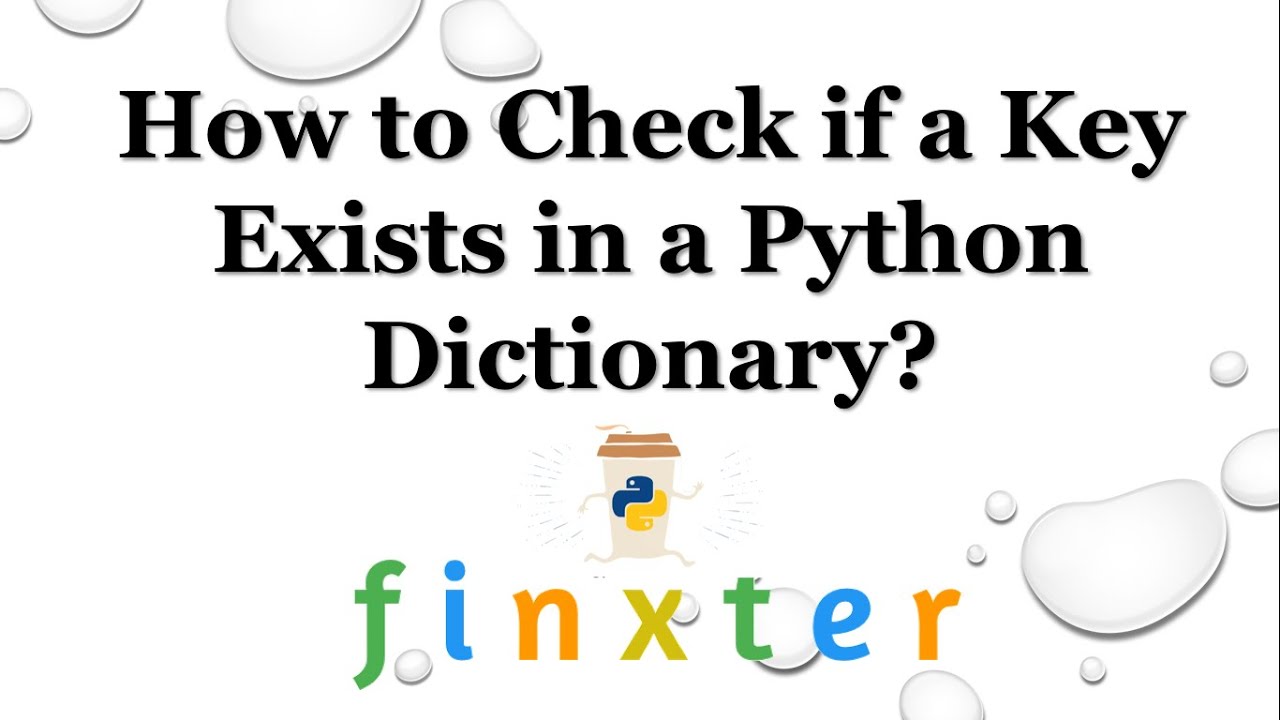

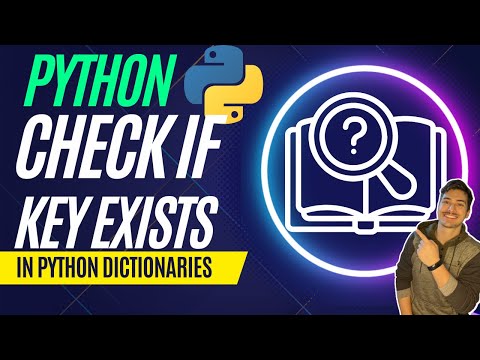

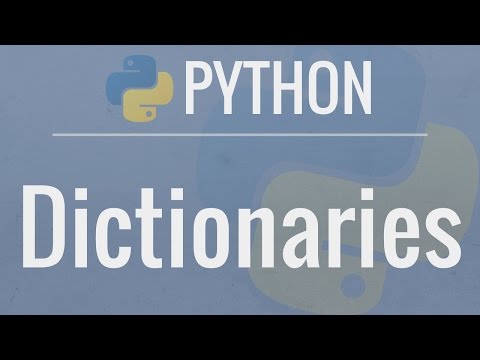

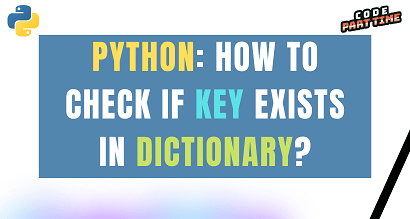
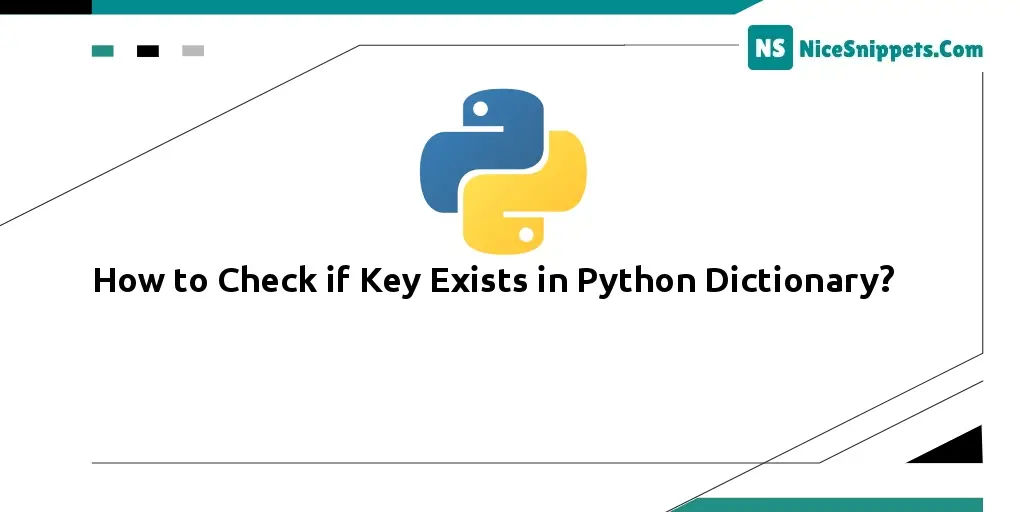

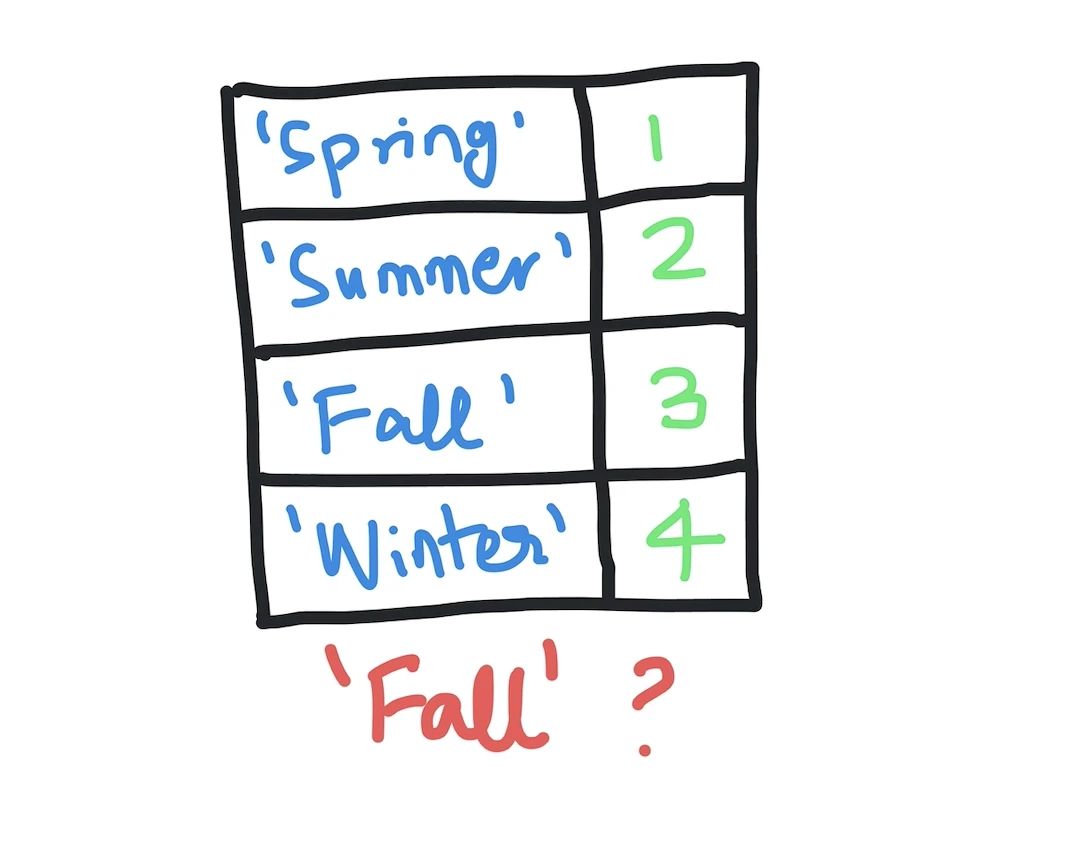
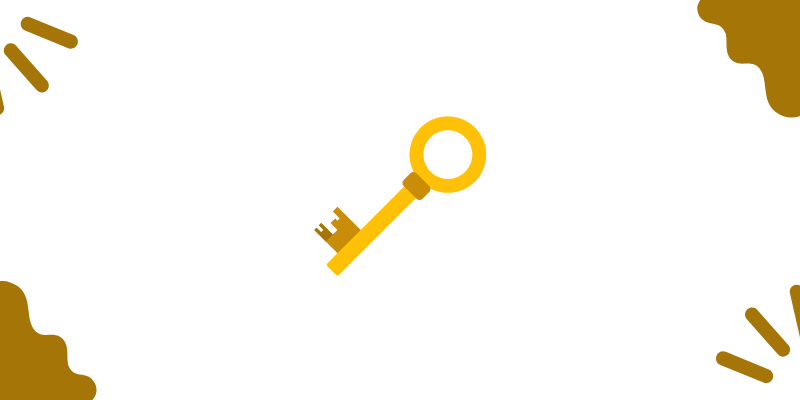
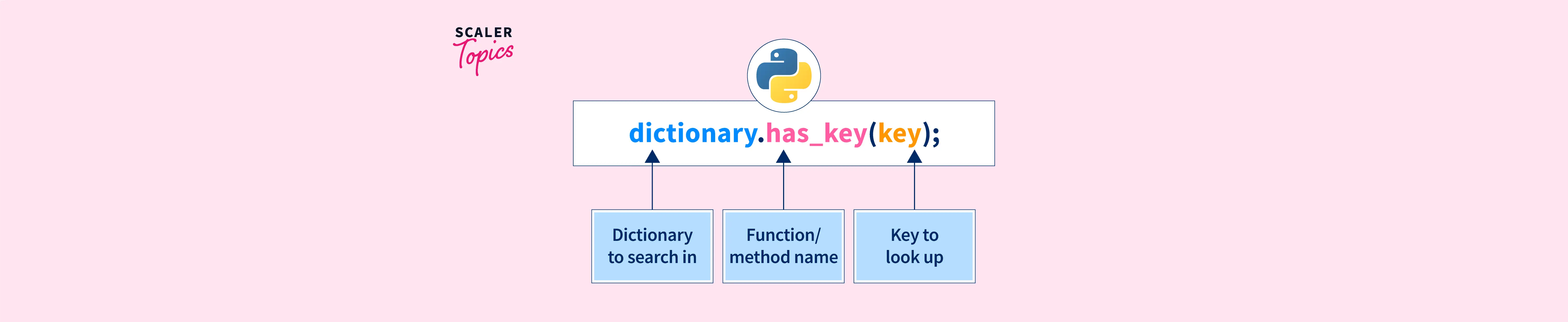
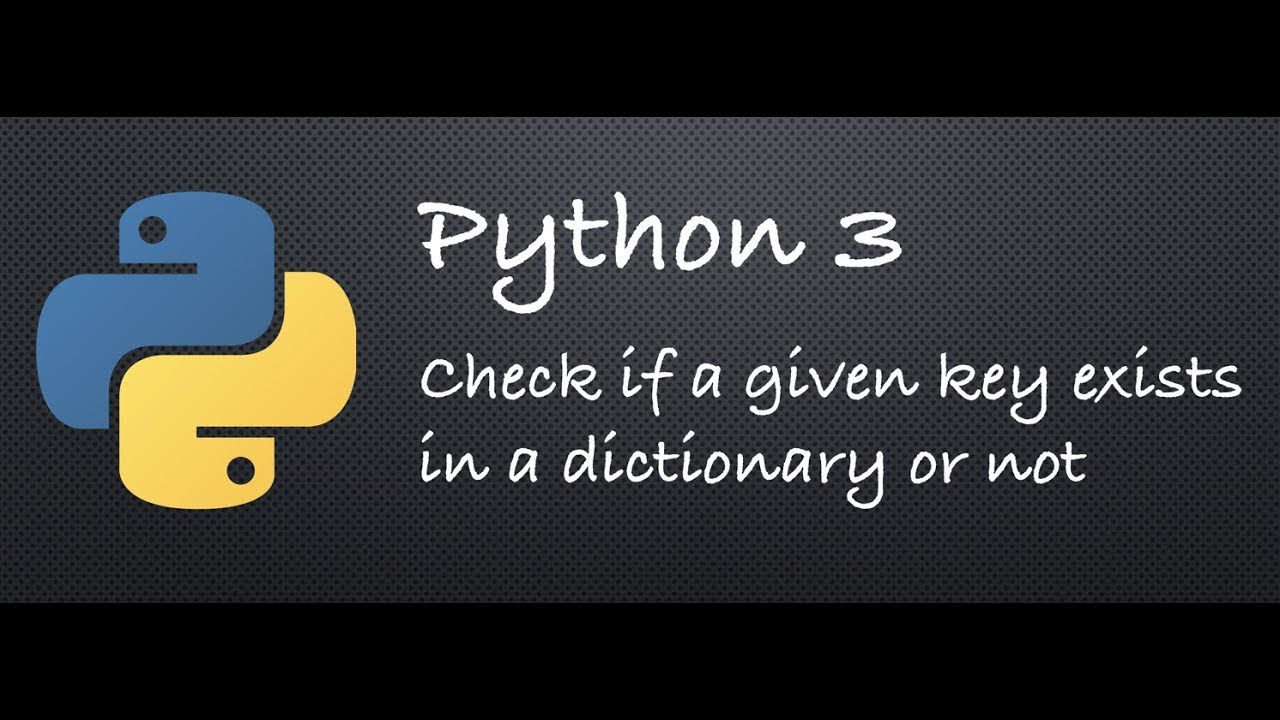
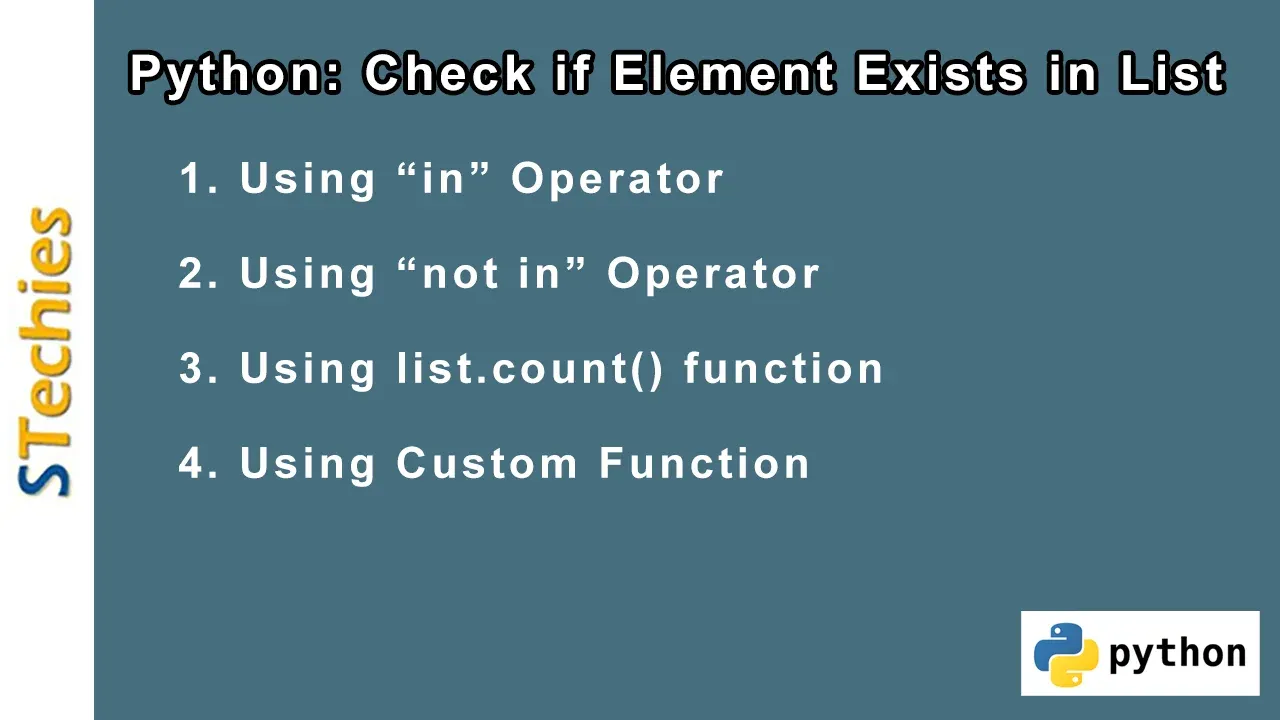
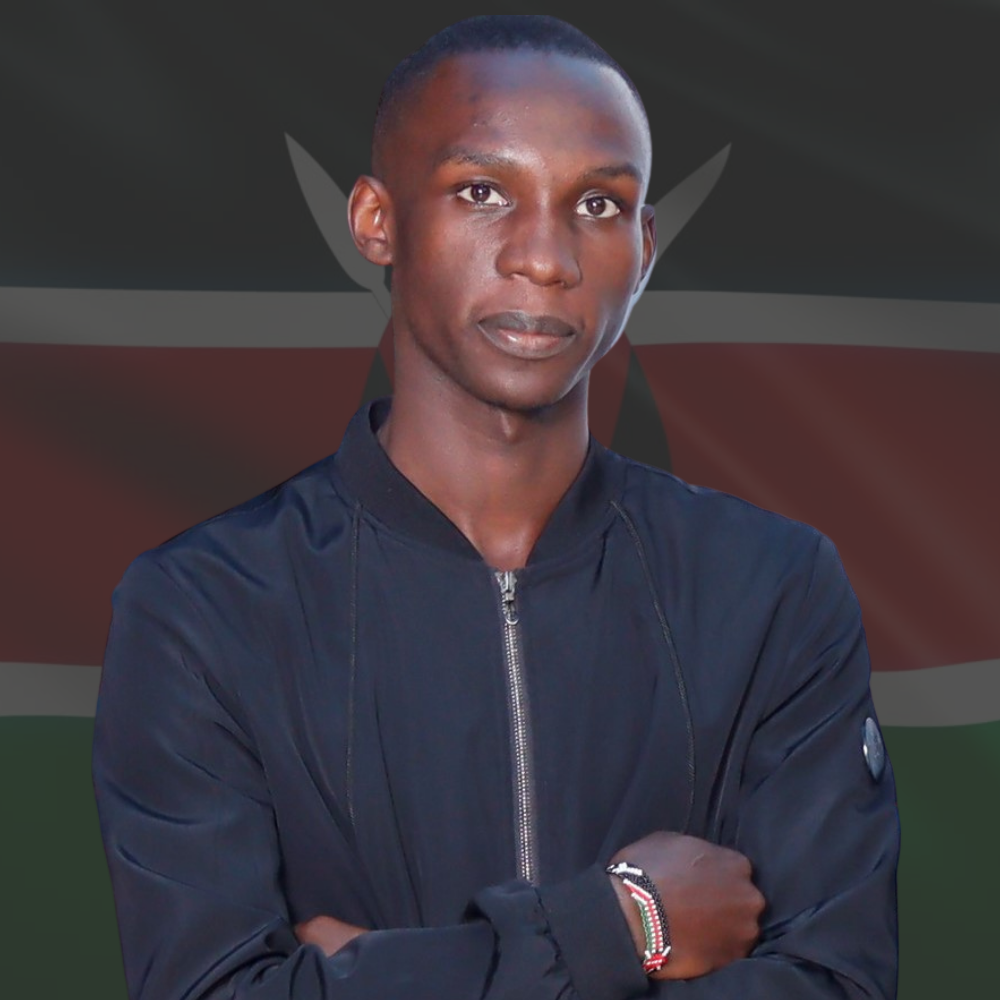
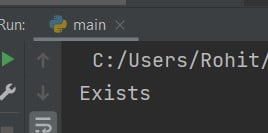
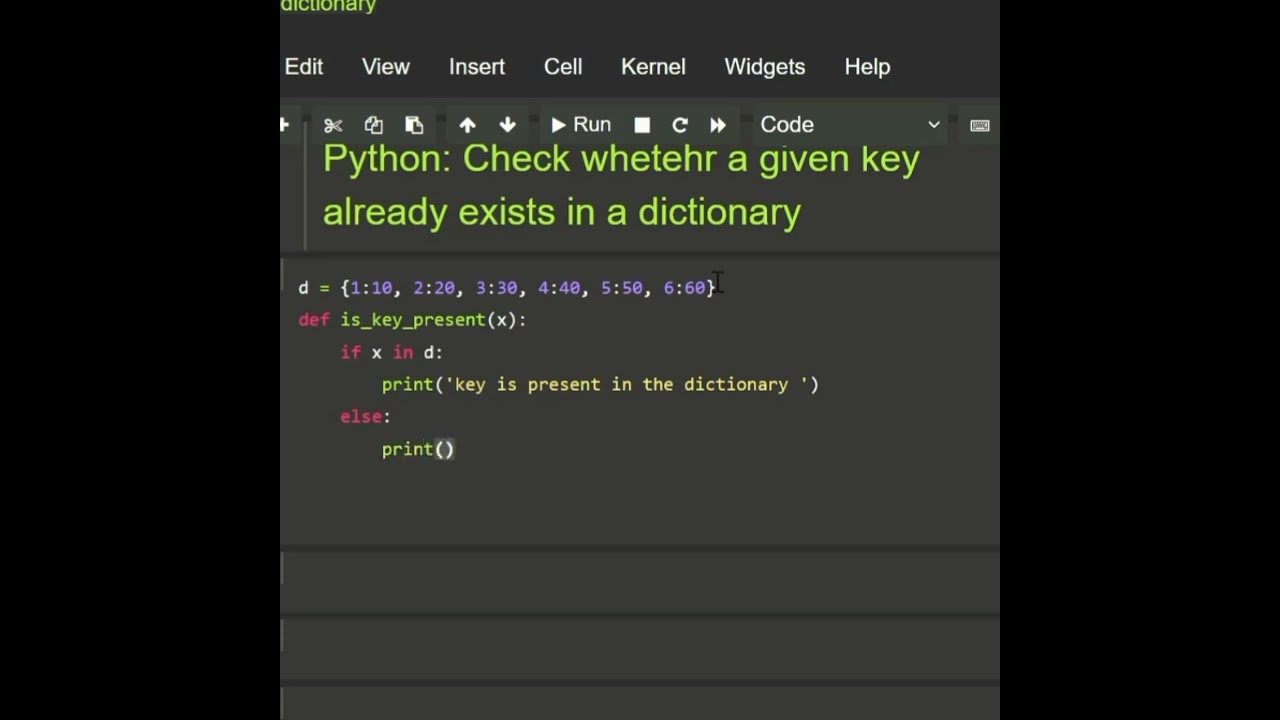


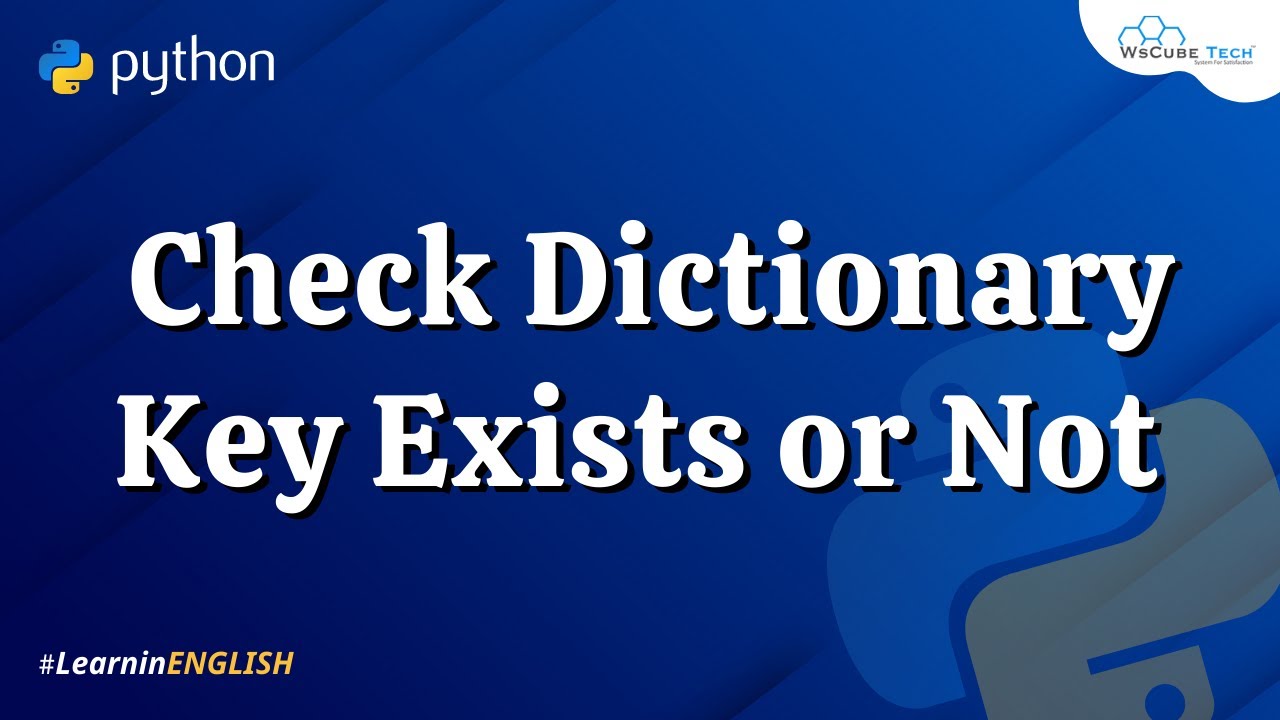

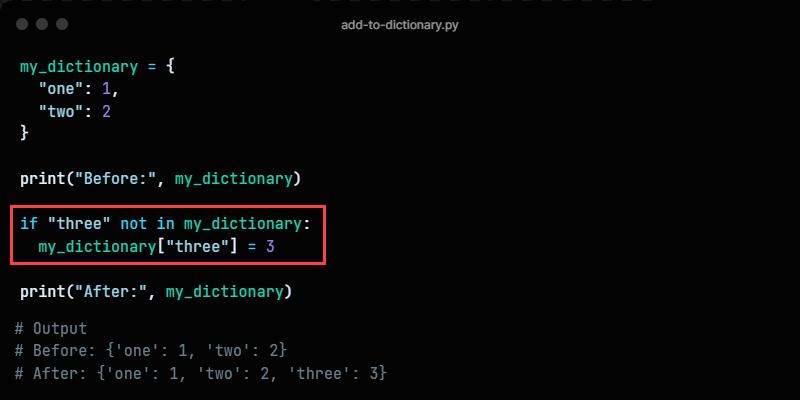

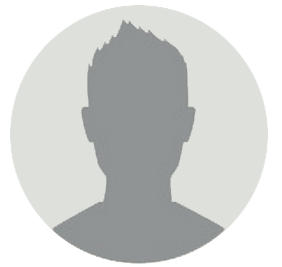
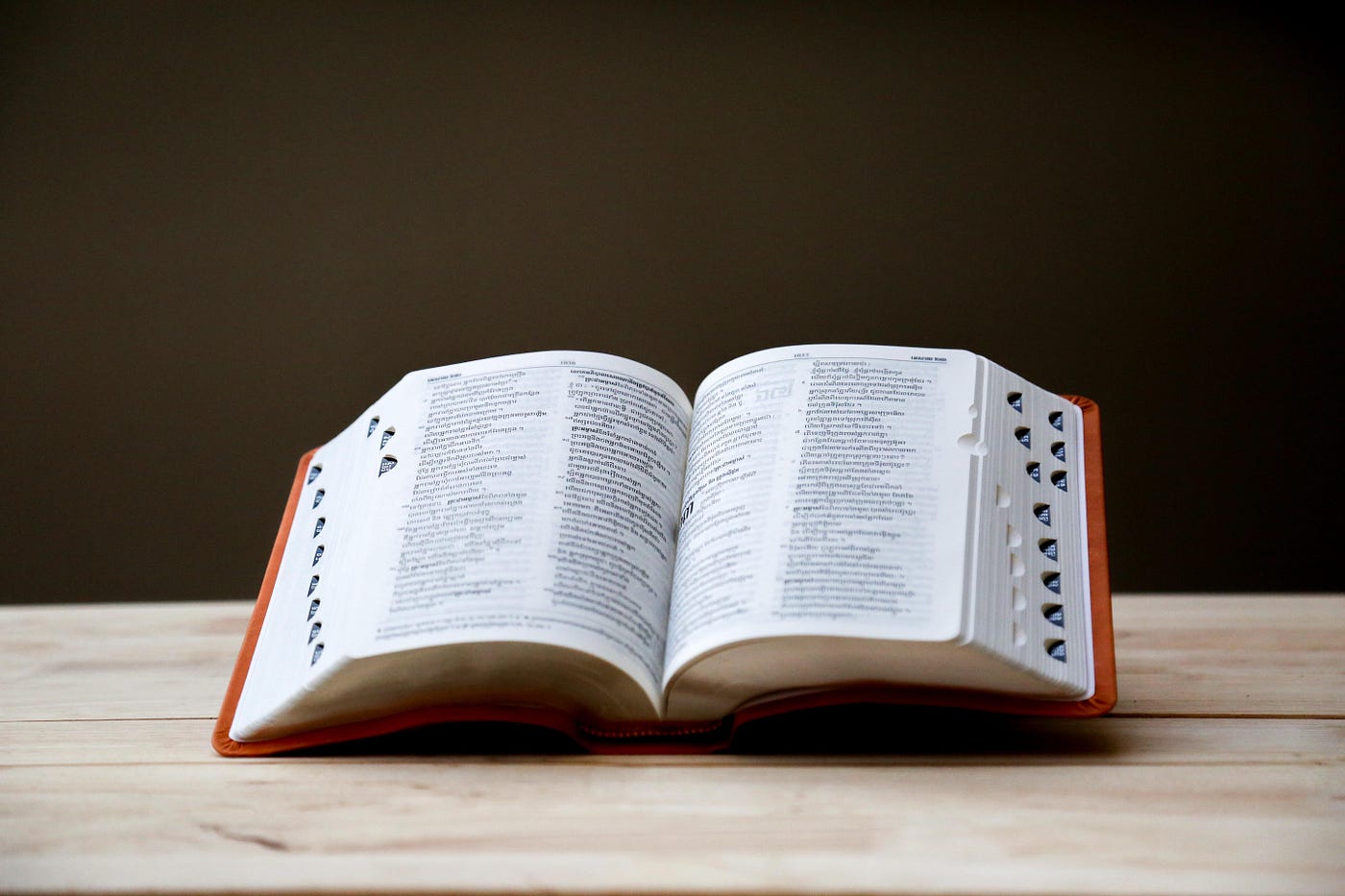
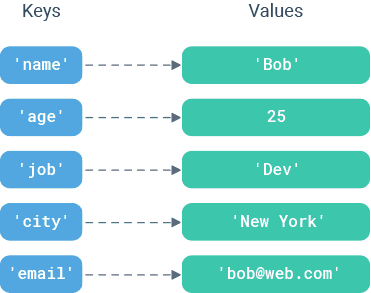
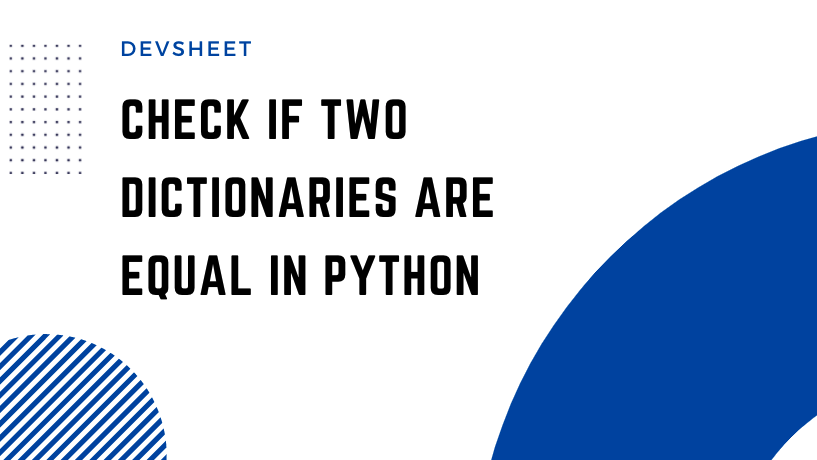
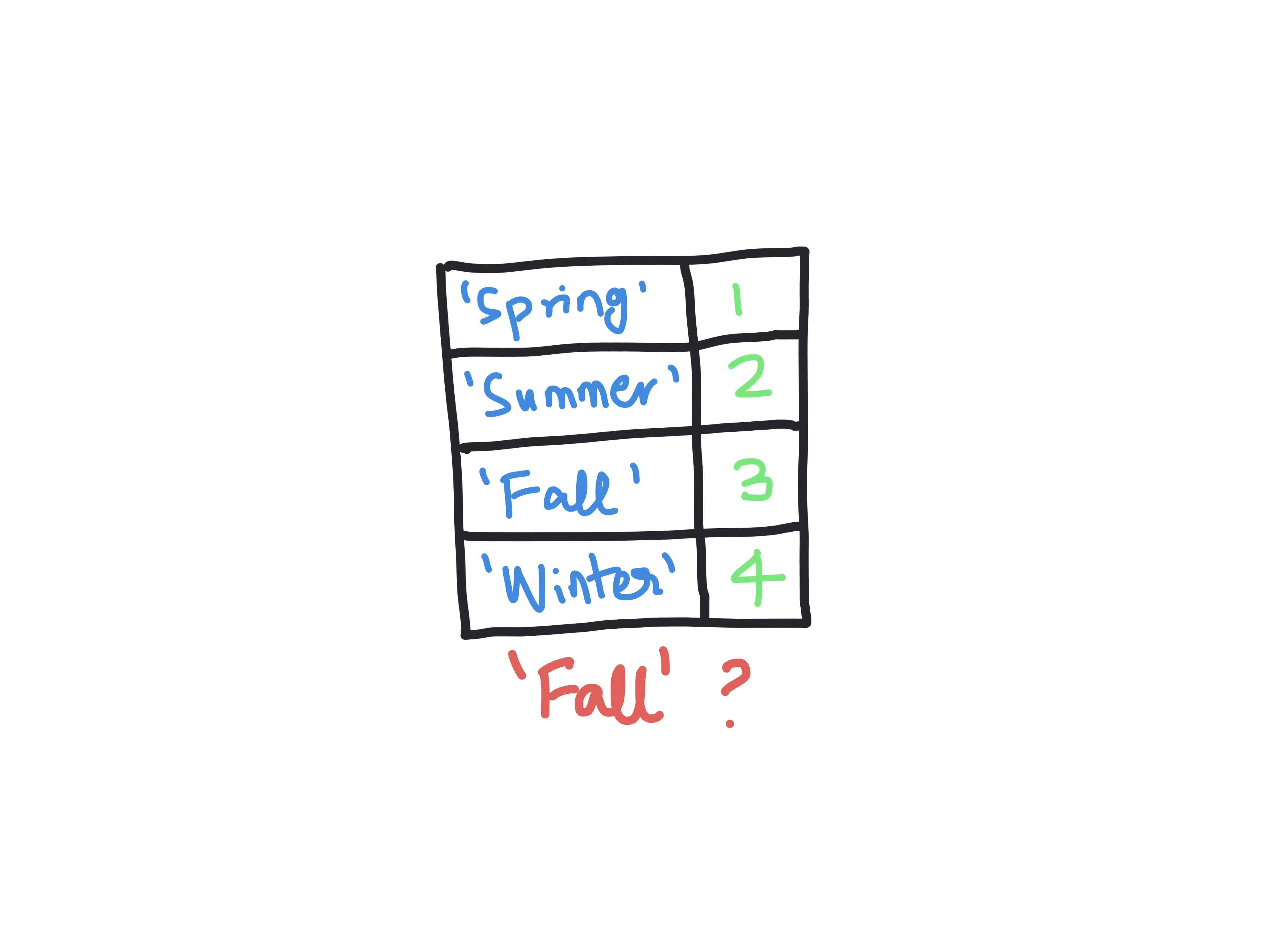
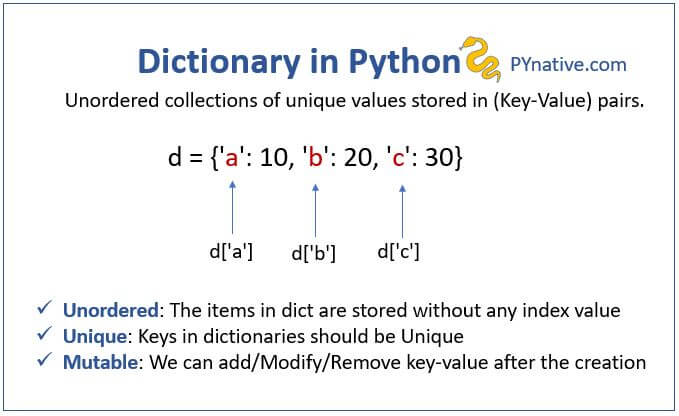

![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example3.jpg)
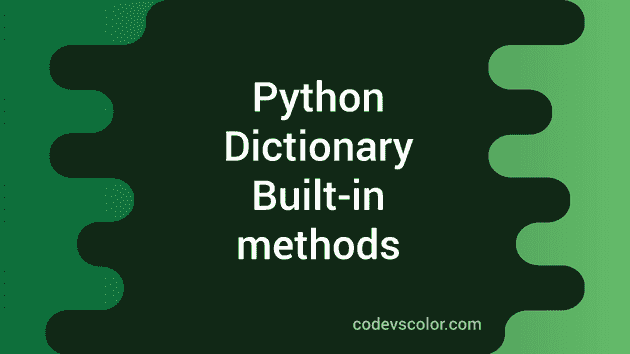



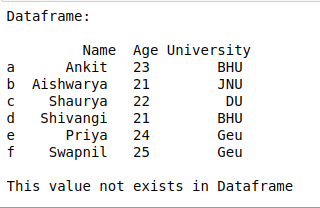

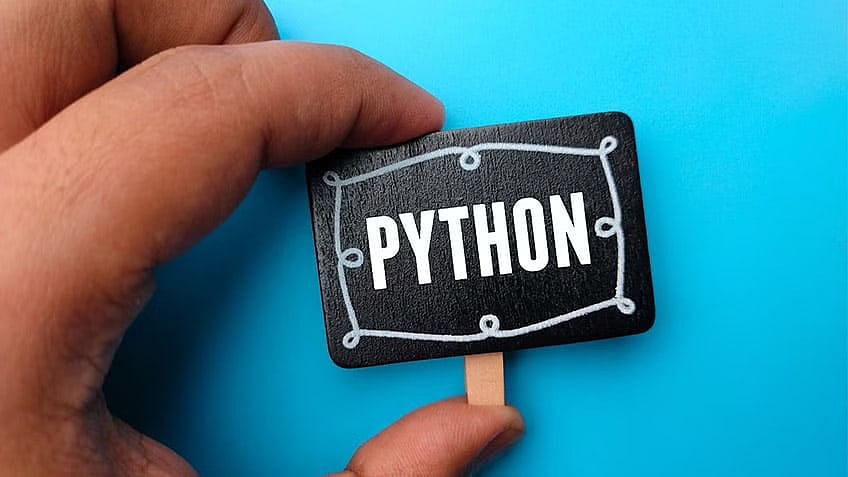
Article link: check if key exists in dict python.
Learn more about the topic check if key exists in dict python.
- How to check if key exists in a python dictionary? – Flexiple
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if a given key already exists in a dictionary
- Check whether given Key already exists in a Python Dictionary
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Check if Key Exists in List of Dictionaries in Python (3 Examples)
- Add an item if the key does not exist in dict with setdefault in Python
- Check whether given Key already exists in a Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- Check if Key exists in Dictionary (or Value) with Python code
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- How to Check if a Key Exists in a Python Dictionary – Javatpoint