Count Unique Values Dataframe
Methods for Counting Unique Values in a DataFrame
1. Using the .nunique() method:
The easiest way to count unique values in a DataFrame is by using the .nunique() method. This method returns the number of unique values in each column.
“`python
df.nunique()
“`
The .nunique() method can also be applied to a specific column by passing it as an argument.
“`python
df[‘column_name’].nunique()
“`
This method is useful when you want to get a quick overview of the unique values in your DataFrame.
2. Exploring the unique() function:
The unique() function provides another way to obtain unique values in a DataFrame. It returns an array containing all the unique values in a column.
“`python
df[‘column_name’].unique()
“`
This method is often useful when you want to extract the unique values from a specific column and perform further analysis on them.
3. Applying value_counts() to count unique values:
The value_counts() function allows you to count the frequency of each unique value in a column. It returns a Series with the unique values as the index and their corresponding counts as the values.
“`python
df[‘column_name’].value_counts()
“`
This method is helpful when you want to identify the most common or rare values in a column.
4. Utilizing groupby() and nunique() together:
The groupby() function can be combined with the nunique() method to count unique values based on specific groupings. This is particularly useful when you want to understand the distribution of unique values within different categories.
“`python
df.groupby(‘grouping_column’)[‘column_name’].nunique()
“`
By specifying both the grouping column and the column you want to count the unique values from, you can generate a summary of unique values per group.
5. Counting unique values with pivot_table():
The pivot_table() function in pandas can also be used to count unique values in a DataFrame. By specifying the index and values parameters, you can create a table that displays the counts of unique values in a more structured format.
“`python
pd.pivot_table(df, index=’index_column’, values=’column_name’, aggfunc=pd.Series.nunique)
“`
This method is helpful when you want to have a comprehensive view of the unique values in a DataFrame.
6. Handling missing values while counting unique values:
Sometimes, your DataFrame may contain missing values, represented as NaN. Most of the methods mentioned above handle missing values automatically and exclude them from the count. However, if you want to count missing values as well, you can use the dropna parameter.
“`python
df[‘column_name’].nunique(dropna=False)
“`
This will include the count of missing values as a separate category.
7. Dealing with case sensitivity in unique value counting:
By default, pandas considers the case when counting unique values. So, ‘A’ and ‘a’ are treated as different values. If you want to disregard case sensitivity while counting unique values, you can convert the column to lowercase or uppercase before applying any of the counting methods.
“`python
df[‘column_name’] = df[‘column_name’].str.lower()
df[‘column_name’].nunique()
“`
FAQs
Q1: How can I count the unique values in a specific column?
A: You can use the .nunique() method by passing the column name as an argument. For example:
“`python
df[‘column_name’].nunique()
“`
Q2: How can I check the unique values in a column?
A: You can use the unique() function. It returns an array with all the unique values in a column. For example:
“`python
df[‘column_name’].unique()
“`
Q3: How can I count the frequency of each unique value in a column?
A: The value_counts() function can be used for this purpose. It returns a Series with the unique values as the index and their corresponding counts as the values. For example:
“`python
df[‘column_name’].value_counts()
“`
Q4: How can I count unique values based on specific groupings?
A: You can use the groupby() function in combination with the nunique() method. By specifying the grouping column and the column with the values to count, you can generate a summary of unique values per group. For example:
“`python
df.groupby(‘grouping_column’)[‘column_name’].nunique()
“`
Q5: How can I count unique values in a more structured format?
A: The pivot_table() function allows you to create a table that displays the counts of unique values. By specifying the index and values parameters, you can organize the data in a more structured way. For example:
“`python
pd.pivot_table(df, index=’index_column’, values=’column_name’, aggfunc=pd.Series.nunique)
“`
Q6: How can I include missing values when counting unique values?
A: Most of the counting methods automatically exclude missing values from the count. However, if you want to count missing values as well, you can use the dropna parameter and set it to False. For example:
“`python
df[‘column_name’].nunique(dropna=False)
“`
Q7: How can I disregard case sensitivity when counting unique values?
A: By default, pandas considers the case when counting unique values. To disregard case sensitivity, you can convert the column to lowercase or uppercase before applying any of the counting methods. For example:
“`python
df[‘column_name’] = df[‘column_name’].str.lower()
df[‘column_name’].nunique()
“`
In conclusion, pandas provides a variety of methods to count unique values in a DataFrame. Each method has its own strengths and limitations, allowing you to choose the most suitable approach based on your specific requirements. By understanding these techniques, you can efficiently analyze your data and gain valuable insights.
40- Pandas Dataframes: Counting And Getting Unique Values
How To Count Unique Values In Pandas Dataframe?
Pandas, one of the most popular data manipulation libraries in Python, provides powerful tools for working with structured data. One common task in data analysis is counting the number of unique values in a DataFrame. Whether you want to analyze a single column or the entire dataset, pandas offers several approaches to accomplish this task efficiently. In this article, we will explore different methods to count unique values in a pandas DataFrame.
Methods to Count Unique Values
1. Using the `nunique()` Function:
The `nunique()` function in pandas returns the number of unique elements in each column of a DataFrame. By calling this function on a specific column, we can obtain the count of unique values for that column. For example:
“`python
df[‘column_name’].nunique()
“`
By executing the above code snippet, pandas will return the count of unique values in “column_name”.
2. Using the `unique()` Function with `len()`:
Another approach is to use the `unique()` function to retrieve an array of all unique values in a column and then determine its length using the `len()` function. This method provides the count of unique values in a column. For instance:
“`python
len(df[‘column_name’].unique())
“`
Executing the above code will provide the count of unique values in the specified column.
3. Using `value_counts()` Function:
The `value_counts()` function in pandas returns a Series containing counts of unique values in a column. It sorts the values in descending order by default. By inspecting the length of this Series, we can obtain the count of unique values. Consider the following example:
“`python
df[‘column_name’].value_counts().count()
“`
By running this code, pandas will provide the count of unique values in “column_name”.
4. Applying `unique()` and `len()` Functions on an Entire DataFrame:
If you need to count the number of unique values across the entire DataFrame, you can merge all the columns together and use the `unique()` and `len()` functions. Here is an example:
“`python
len(pd.unique(df.values.ravel()))
“`
This code snippet will return the count of unique values across the DataFrame.
5. Using the `drop_duplicates()` Function:
The `drop_duplicates()` function in pandas eliminates duplicate rows from a DataFrame, leaving only the unique rows. By utilizing the `shape` attribute of the resulting DataFrame, we can obtain the count of unique values. Here is an example:
“`python
df.drop_duplicates().shape[0]
“`
By executing this code, pandas will provide the count of unique values in the DataFrame.
FAQs:
Q1. Can I count the number of unique values across multiple columns in a DataFrame?
Yes, you can count the number of unique values across multiple columns by appending the columns together using the `union()` function from the `sets` module. Here is an example:
“`python
df[[‘column1’, ‘column2’]].apply(lambda x: len(set(x[0]).union(set(x[1]))), axis=1)
“`
This code will return a Series containing the count of unique values across the specified columns.
Q2. How can I count unique values in a DataFrame and store the results in a new DataFrame?
To store the count of unique values in a new DataFrame, you can use the `to_frame()` function. Here is an example:
“`python
result = df[‘column_name’].value_counts().to_frame().reset_index()
result.columns = [‘Unique Values’, ‘Count’]
“`
Executing this code snippet will generate a new DataFrame containing the unique values and their respective counts.
Q3. Is there any method to count the number of unique values based on condition?
Yes, you can count the number of unique values based on a condition using the `groupby()` function in pandas. By grouping the DataFrame based on a specific column and applying the `nunique()` function, you can get the count of unique values satisfying a given condition. For example:
“`python
df.groupby(‘column_name’)[‘another_column’].nunique()
“`
Executing this code snippet will provide the count of unique values in “another_column” based on the groups formed by “column_name”.
In conclusion, pandas offers various ways to count unique values in a DataFrame. By leveraging functions such as `nunique()`, `unique()`, `value_counts()`, `drop_duplicates()`, and the combination of `len()` and `unique()`, you can efficiently obtain the desired count whether for a single column or the entire dataset. Additionally, pandas provides methods for counting unique values based on conditions and storing the results in a new DataFrame.
Keywords searched by users: count unique values dataframe Pandas count unique values in column, Dataframe unique values, Check unique values in column pandas, Count value in column pandas, Count unique Python, Count value in Pandas, Pandas count unique values in multiple columns, Print the total number of unique categories
Categories: Top 22 Count Unique Values Dataframe
See more here: nhanvietluanvan.com
Pandas Count Unique Values In Column
Pandas provides the `value_counts()` method, which enables us to count the unique values in a column and display them in descending order. This method is typically used when we want to observe the frequency distribution of different values in a column. Let’s consider a dataset containing information about students’ grades:
“`python
import pandas as pd
data = {‘Name’: [‘John’, ‘Emily’, ‘Michael’, ‘John’, ‘Michael’],
‘Grade’: [‘A’, ‘B’, ‘C’, ‘A’, ‘B’]}
df = pd.DataFrame(data)
“`
To count unique values in the “Grade” column, we can simply use the `value_counts()` method:
“`python
grade_counts = df[‘Grade’].value_counts()
print(grade_counts)
“`
The output will display the unique values alongside their respective counts:
“`
A 2
B 2
C 1
Name: Grade, dtype: int64
“`
This shows that the “A” grade appears twice, the “B” grade appears twice, and the “C” grade appears once in the “Grade” column.
If we want to obtain the count of unique values without sorting them, Pandas provides the `unique()` method. This method returns an array of the unique values in the column. Combining it with the `len()` function enables us to count the unique values:
“`python
unique_grades = df[‘Grade’].unique()
grade_count = len(unique_grades)
print(grade_count)
“`
The output will display the count of unique grades, which in this case is 3:
“`
3
“`
Another approach to counting unique values in a column involves the `nunique()` method. This method returns the number of distinct elements in the column. Let’s count the number of unique names in the “Name” column of our dataset:
“`python
name_count = df[‘Name’].nunique()
print(name_count)
“`
The output will present the count of unique names:
“`
3
“`
Now that we have explored different methods to count unique values, it’s time to address some frequently asked questions to further enhance our understanding:
**FAQs**
**Q1: Can we count unique values in multiple columns simultaneously?**
A1: Yes, Pandas allows us to count unique values in multiple columns simultaneously. To achieve this, we can use the `select_dtypes()` method to filter the dataset based on specific data types, such as object or numeric. For instance, to count unique values in all object columns, we can use the following code:
“`python
object_columns = df.select_dtypes(include=[‘object’]).columns
unique_counts = df[object_columns].nunique()
print(unique_counts)
“`
This will provide the count of unique values in each object column.
**Q2: How can we count missing values in a column?**
A2: Pandas provides the `isna()` method, which returns a boolean mask indicating missing values in the column. By summing the `True` values, we can obtain the count of missing values. Consider the following code:
“`python
missing_count = df[‘Column_Name’].isna().sum()
print(missing_count)
“`
`Column_Name` should be replaced with the desired column name. The output will display the count of missing values in that column.
**Q3: What if we want to count unique values in a specific row range?**
A3: To count unique values in a specific row range, we can use the `iloc` indexer provided by Pandas. The `iloc` indexer allows us to select rows and columns based on their integer positions. Let’s say we want to count unique values in the “Grade” column for rows 1 to 3. We can achieve this using the following code:
“`python
grade_counts = df.iloc[1:4][‘Grade’].value_counts()
print(grade_counts)
“`
The output will display the unique values and their respective counts within the specified row range.
**Q4: Can we count unique values in a DataFrame column while ignoring NaN values?**
A4: Yes, Pandas provides the `dropna()` method to remove missing values from the column before counting unique values. Consider this code:
“`python
unique_values = df[‘Column_Name’].dropna().nunique()
print(unique_values)
“`
This will count the unique values in the column while excluding NaN values, thereby providing an accurate count.
Pandas offers several methods to count unique values in a column, allowing data analysts and scientists to efficiently explore and analyze their datasets. By leveraging the `value_counts()`, `nunique()`, and `unique()` methods, users gain flexibility in obtaining insights from their data. Additionally, Pandas’ intuitive functionality enables data exploration in a streamlined manner, catering to various scenarios and preferences.
Dataframe Unique Values
When working with data, one of the essential tasks is to identify and understand the unique values within a dataset. A popular tool for data manipulation and analysis is the pandas library in Python. Within pandas, a powerful data structure known as a DataFrame allows us to efficiently handle, process, and analyze data. In this article, we will delve into the concept of unique values within a DataFrame, explore various methods to extract these values, and discuss their significance in data analysis.
Understanding DataFrames:
Before diving into the specifics of unique values, let’s briefly touch upon the DataFrame structure. A DataFrame is a two-dimensional labeled data structure with labeled axes (rows and columns). It can be thought of as a table, where each column represents a different feature or attribute, and each row corresponds to a particular observation or record. DataFrames are commonly used to represent structured data, such as data from spreadsheets, CSV files, SQL tables, or even scraped from the web.
Extracting Unique Values in DataFrame:
The process of extracting unique values from a DataFrame can be crucial in several scenarios. It helps in identifying distinct categories within a column, eliminating duplicate records, or understanding the overall uniqueness of the dataset. Thankfully, pandas provides multiple methods that we can utilize for this purpose. Let’s explore some popular techniques:
1. unique():
The unique() method is used to obtain an array of unique values within a column of a DataFrame. It returns the values in the order they appear. For instance, if we have a DataFrame ‘df’ and want to extract unique values from the ‘city’ column, we can use the command: df[‘city’].unique()
2. nunique():
The nunique() method is handy when we want to know the count of unique values in a column. For example, if we want to find the number of unique names in a ‘name’ column of a DataFrame, we can use df[‘name’].nunique(). It returns an integer representing the count of distinct values.
3. drop_duplicates():
The drop_duplicates() method is useful when we want to remove duplicate records from a DataFrame. It returns a DataFrame with all duplicate rows removed, leaving only one instance of each unique combination of values. We can specify the subset of columns to consider while detecting duplicates. For example, df.drop_duplicates(subset=[‘name’, ‘age’]) would remove duplicates based on the ‘name’ and ‘age’ columns.
4. value_counts():
The value_counts() method is another powerful tool that provides the count of unique values in a particular column. It returns a Series object that lists unique values as indices and their respective frequencies as values. This method is most valuable when we want a quick look at the distribution of various categories within a column.
Significance of Unique Values in Data Analysis:
Extracting and understanding unique values within a DataFrame is crucial for various data analysis tasks. They help in identifying distinct categories, spotting anomalies, examining data quality, or preparing data for machine learning algorithms. Some use cases where unique values play a significant role include:
1. Data Cleaning:
Identifying and removing duplicate records is a vital part of data cleaning. Unique value extraction functions assist in detecting and eliminating duplication. Dropping duplicate records ensures the integrity and accuracy of the data, particularly when dealing with large datasets.
2. Categorical Analysis:
Unique values allow us to categorize data and perform categorical analysis. By understanding the distinct categories within a column, we can study the distribution and patterns to gain valuable insights. It helps analyze market segments, customer behaviors, or demographic distributions.
3. Aggregation and Grouping:
Unique values play a crucial role when performing aggregations or grouping operations on datasets. For example, when calculating the total sales for each product category, we need to identify the unique categories available. Aggregating data based on these unique categories provides us with meaningful summaries and insights.
4. Data Preprocessing:
In machine learning tasks, unique values guide data preprocessing steps like encoding categorical variables, imputing missing values, or scaling features. Each unique value represents a distinct group that may require specific handling to produce accurate models.
FAQs:
1. Can we find unique values across multiple columns in a DataFrame?
Yes, we can apply the unique() method on a subset of columns by passing them as a list, e.g., df[[‘col1’, ‘col2’]].unique()
2. How can we ignore NaN or missing values while extracting unique values?
By default, unique value methods ignore NaN values. However, if required, we can explicitly handle NaN values beforehand using pandas functions like dropna() or fillna().
3. Can we determine the unique values in a DataFrame based on multiple column combinations?
Yes, we can use the drop_duplicates() method and specify multiple columns as parameters to detect uniqueness based on their combinations.
4. Are unique values preserved when applying data transformations or filtering operations on a DataFrame?
Yes, unique values remain unchanged, even after performing various operations like filtering, sorting, or grouping on a DataFrame.
In conclusion, understanding unique values within a DataFrame is a fundamental aspect of data analysis. Pandas provides several efficient methods to extract these values, allowing us to explore categorical distributions, eliminate duplication, and preprocess data accurately. By leveraging the power of unique values, analysts and data scientists can gain valuable insights and make informed decisions.
Images related to the topic count unique values dataframe
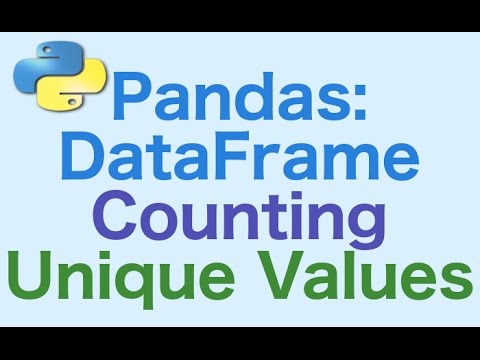
Found 42 images related to count unique values dataframe theme
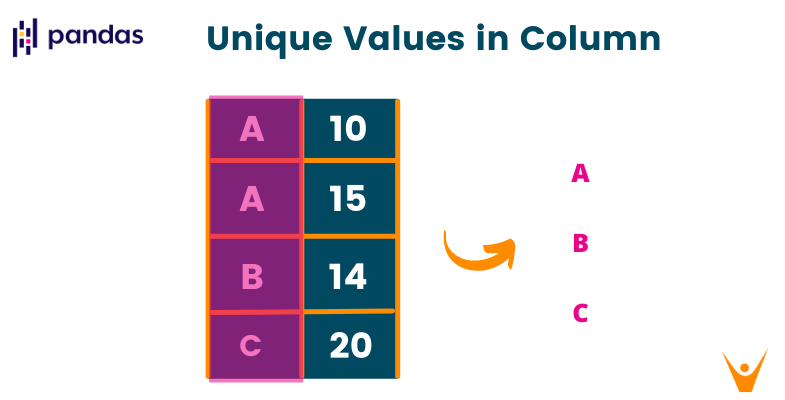
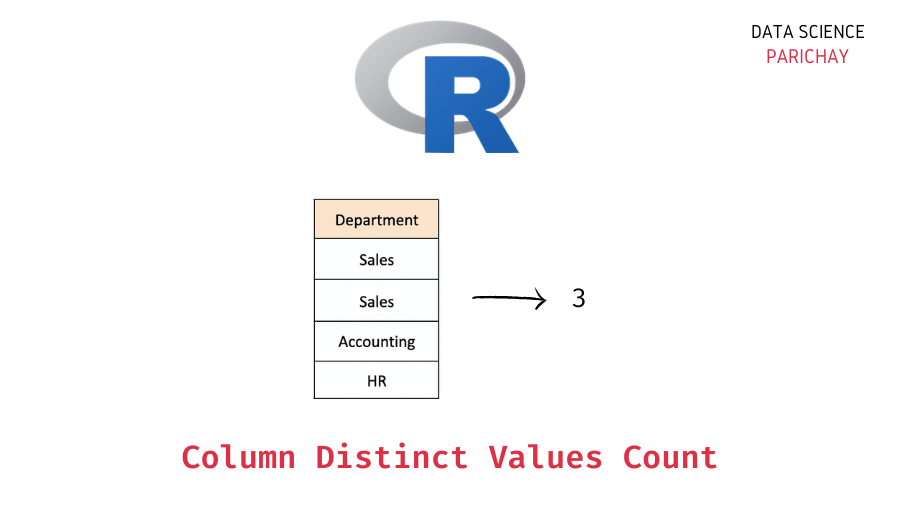
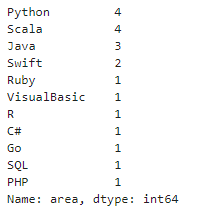

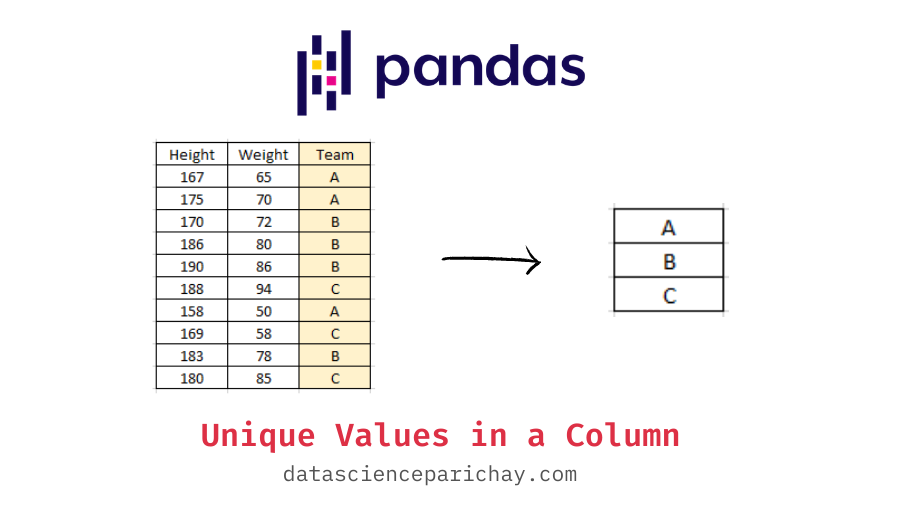
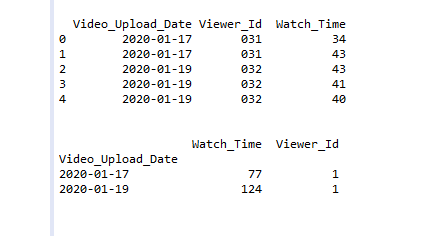
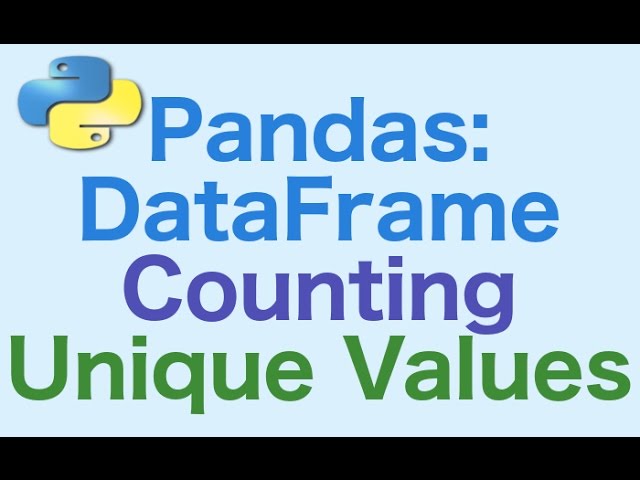

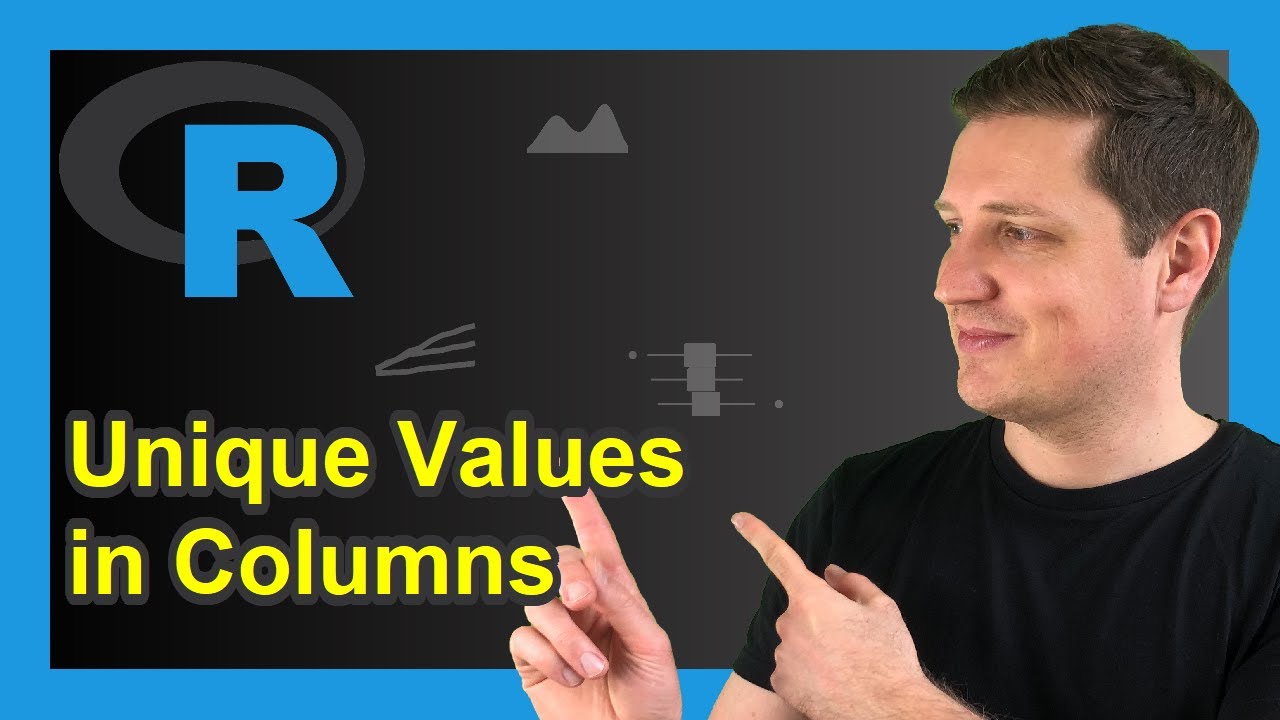

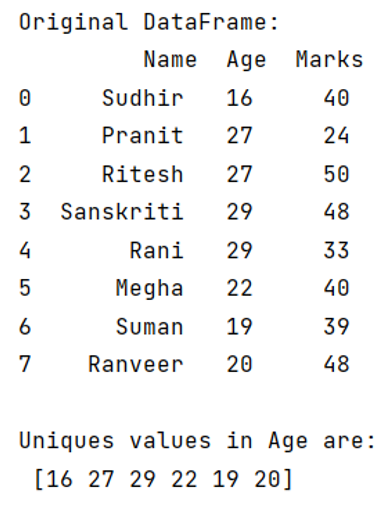
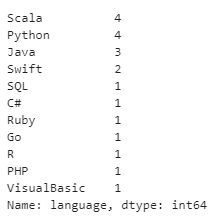
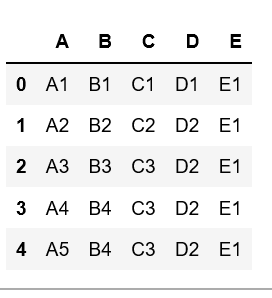
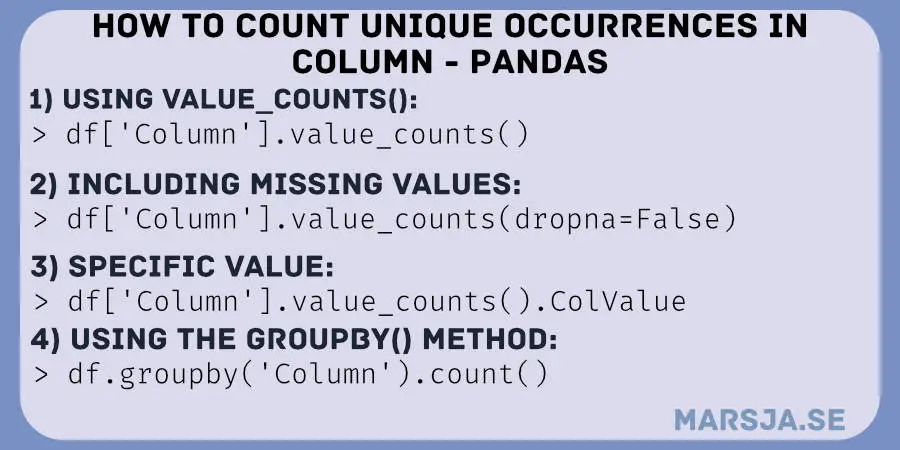
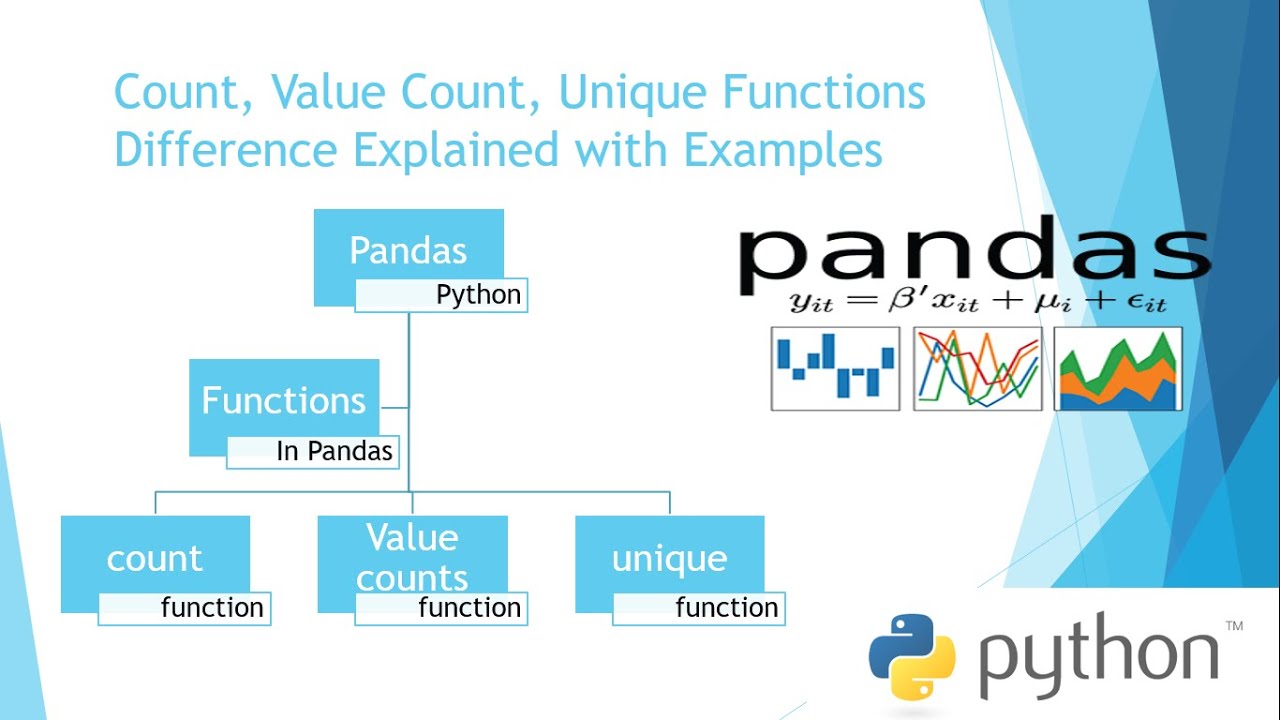



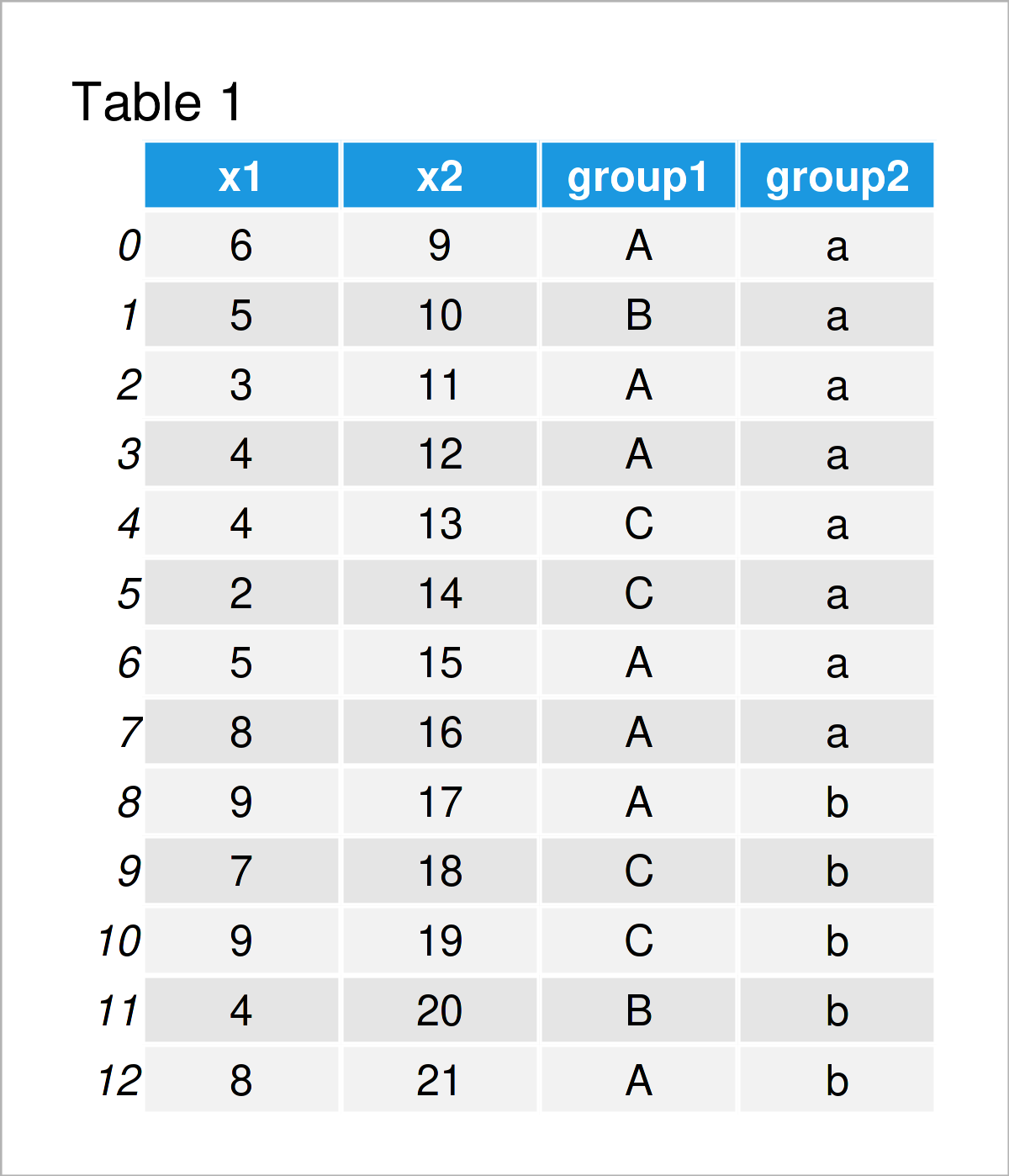
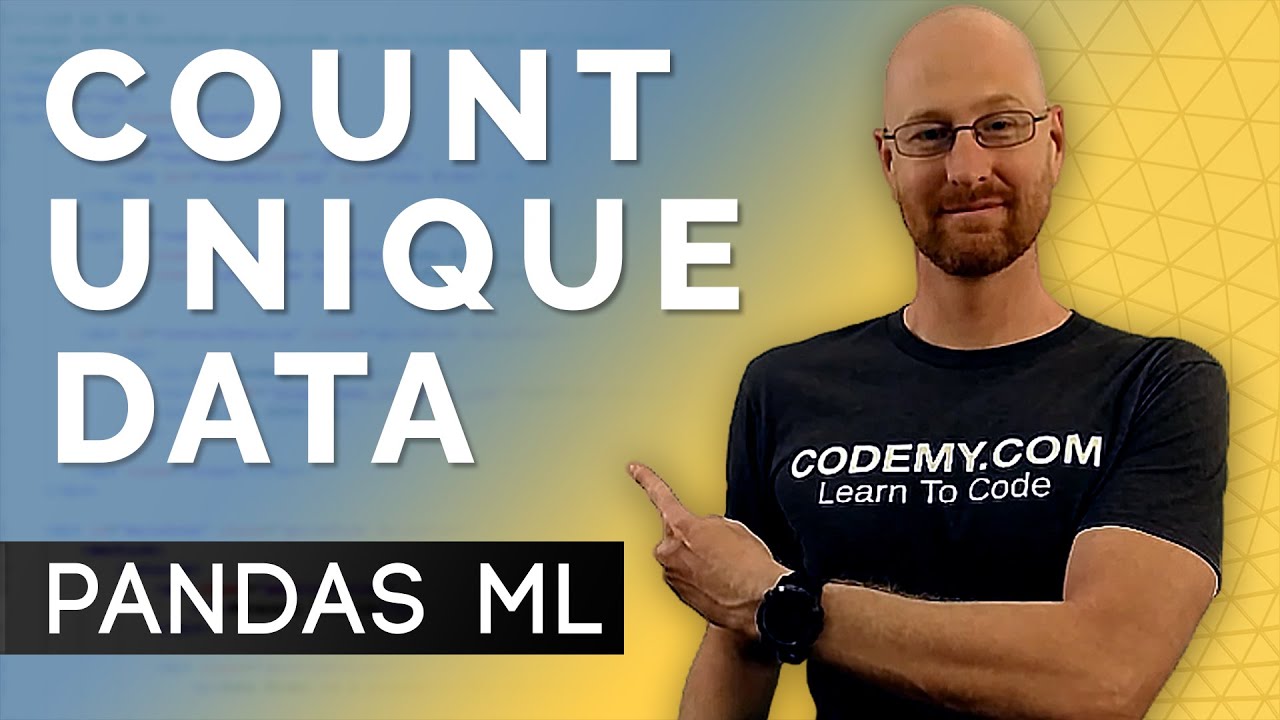
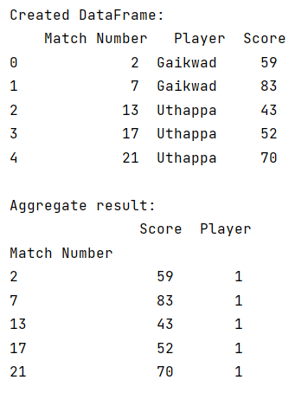

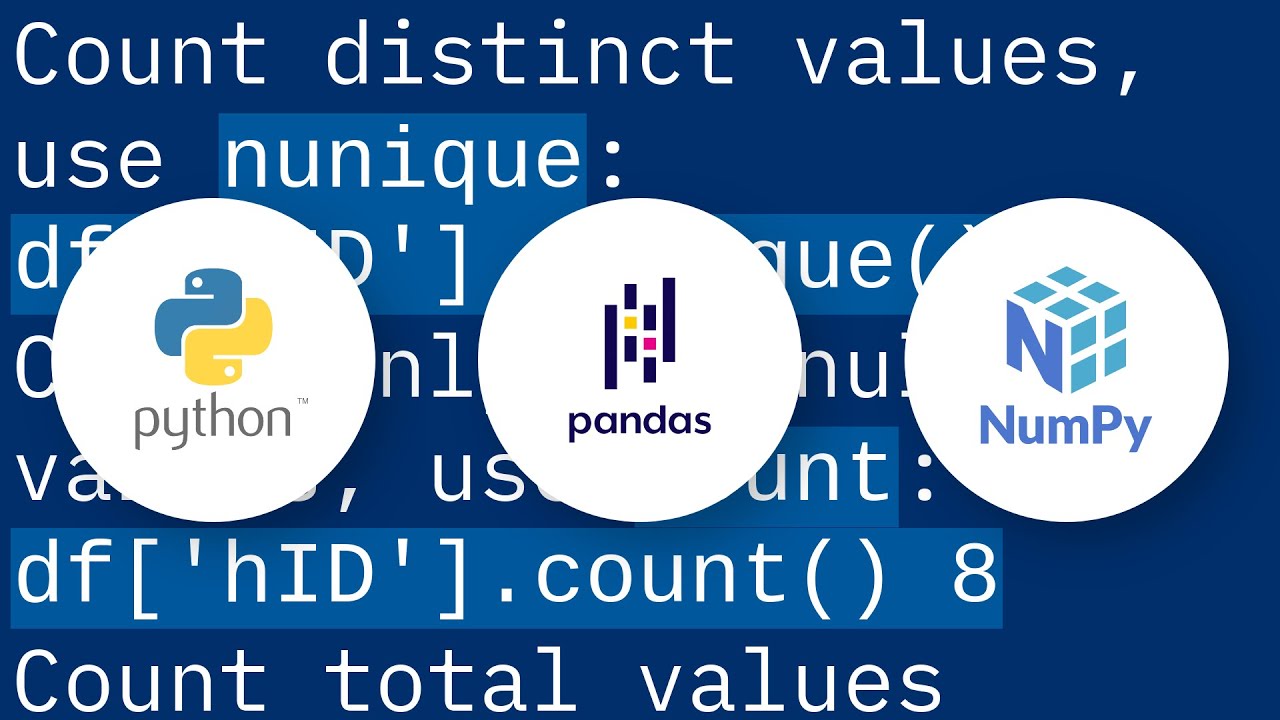


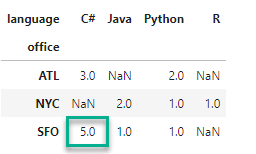
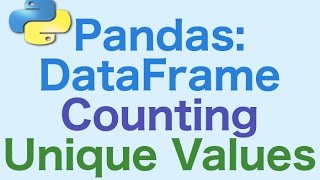

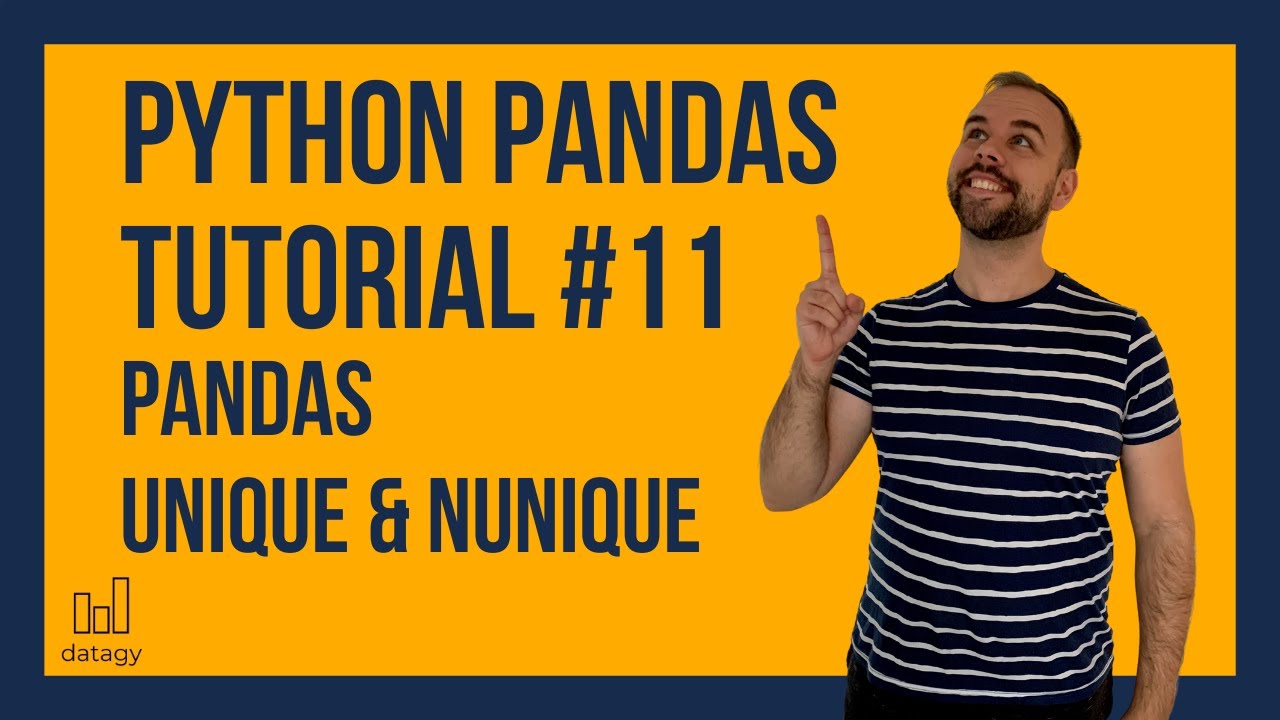

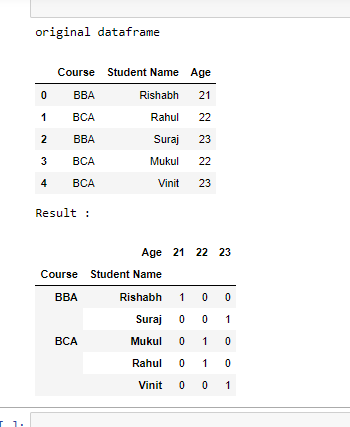


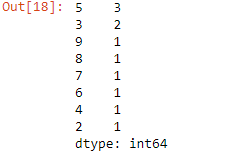

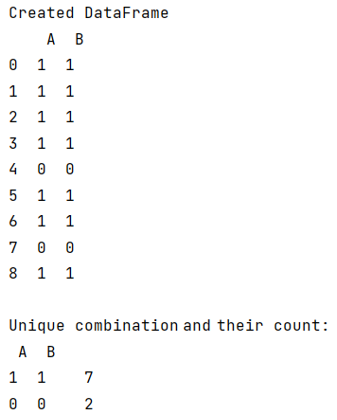
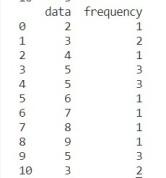

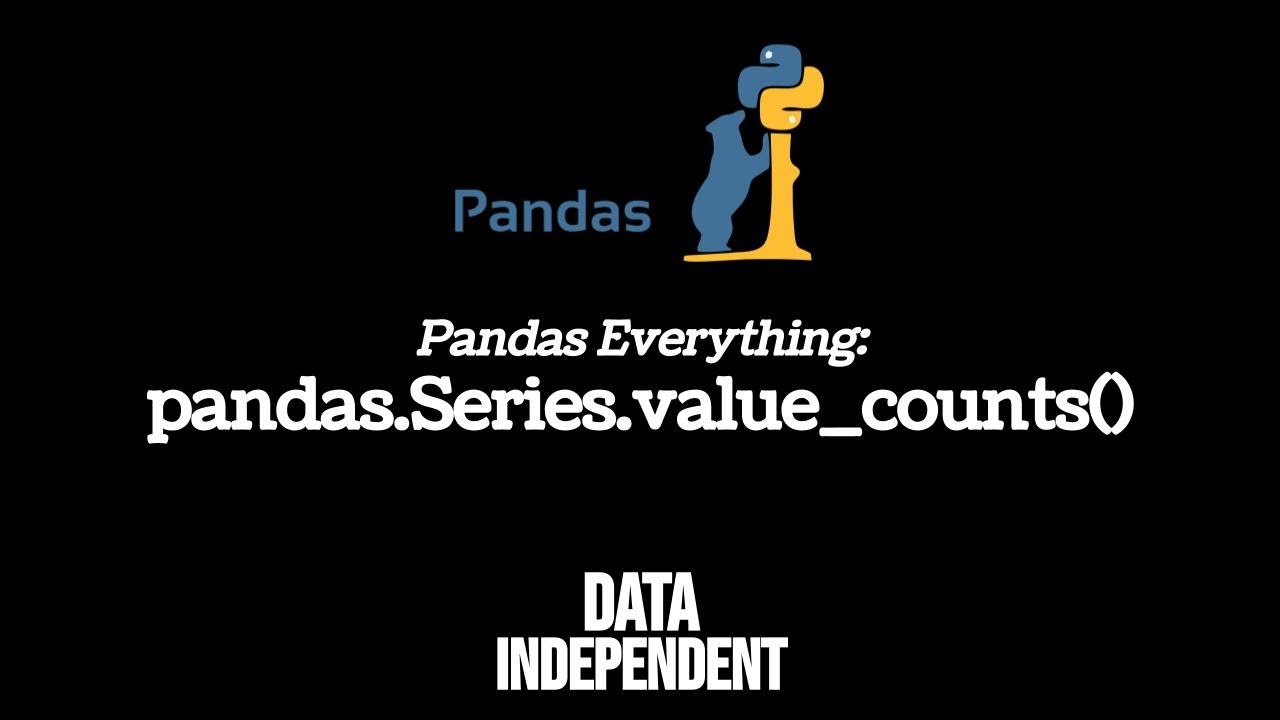
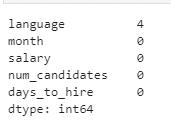
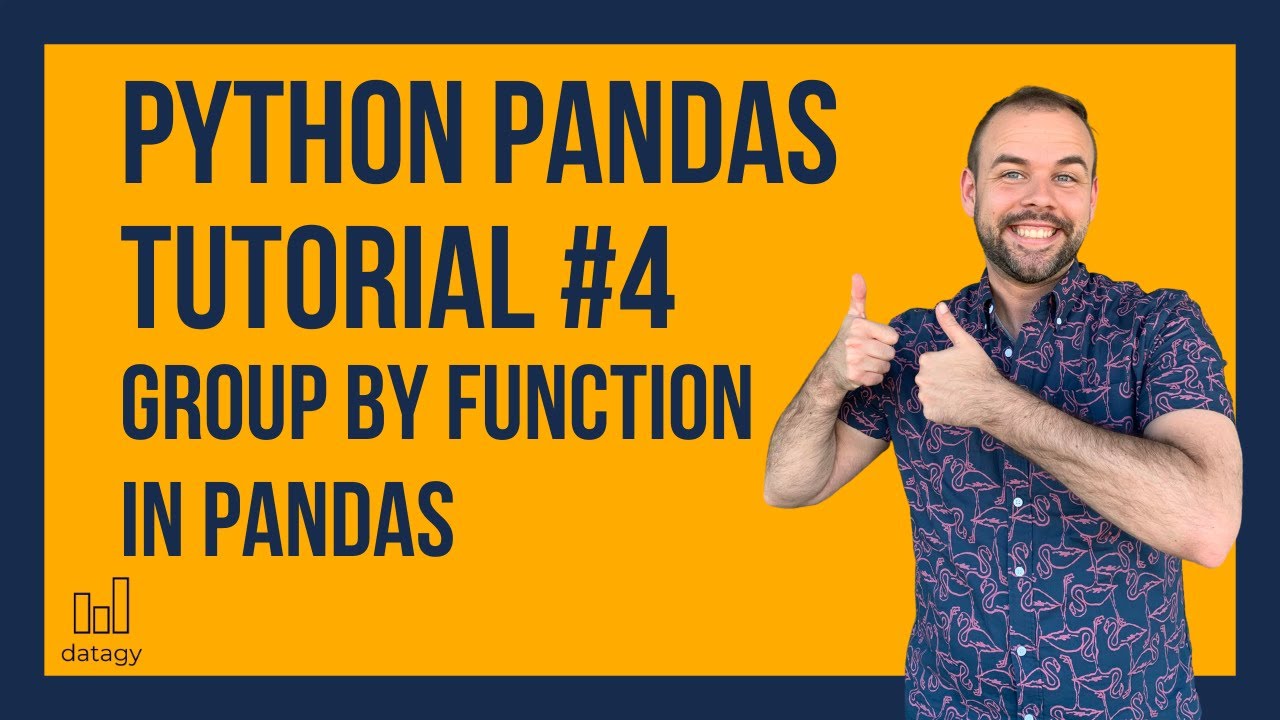
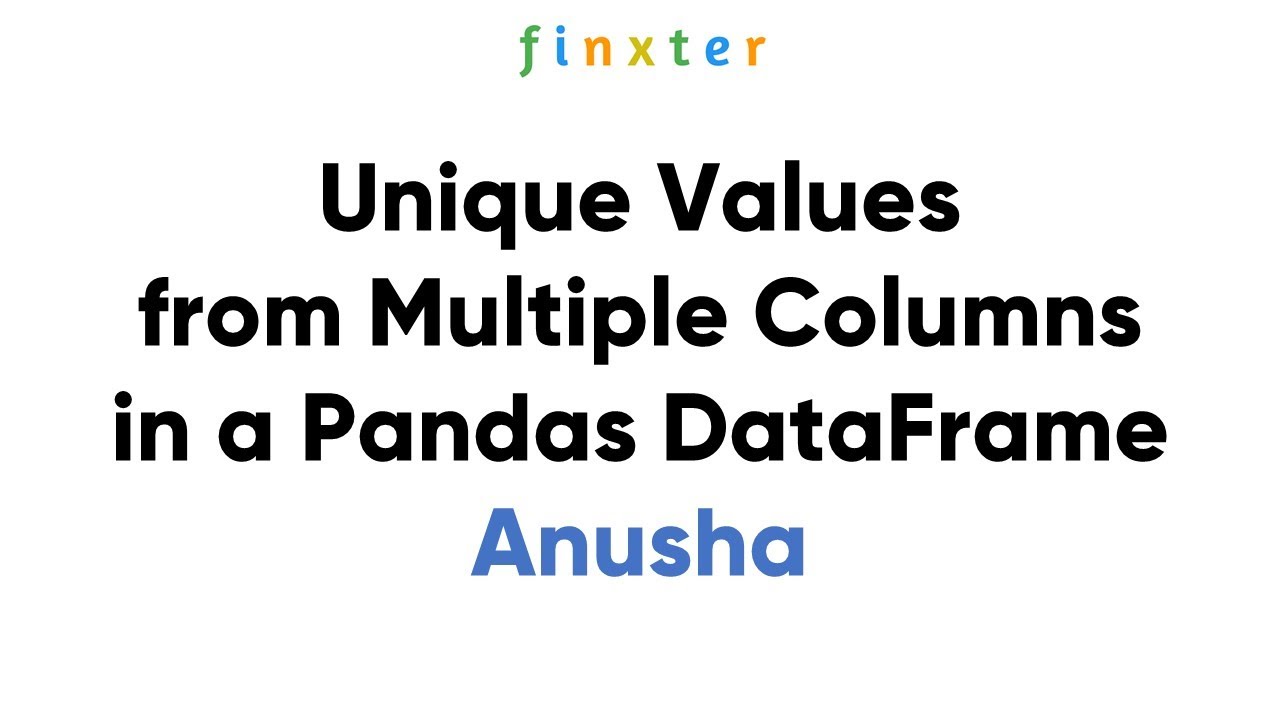
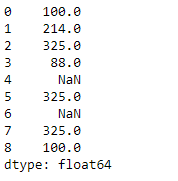
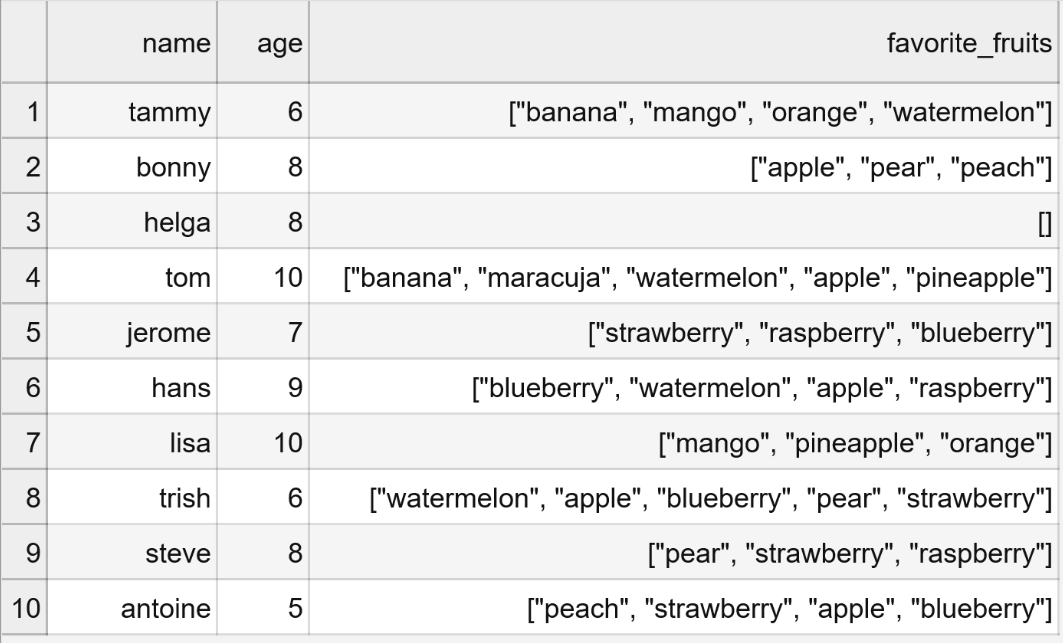
Article link: count unique values dataframe.
Learn more about the topic count unique values dataframe.
- How to identify and count unique values in Pandas
- How to Count Distinct Values of a Pandas Dataframe Column?
- How to Count Unique Values in Pandas (With Examples)
- Pandas Count Unique Values in Column – Spark By {Examples}
- Counting unique values in a column in pandas dataframe like …
- How to identify and count unique values in Pandas
- Pandas – Count of Unique Values in Each Column
- How to count unique items in pandas – Educative.io
- Count Unique Values in Pandas – Datagy
- Pandas Count Distinct – Linux Hint
- How to count unique values in a pandas DataFrame group in …
See more: blog https://nhanvietluanvan.com/luat-hoc