Convert String To Bytes Python
In Python, strings and bytes are two fundamental data types used to represent and manipulate text or binary data. The main difference between them is how they store and represent the data. Strings are sequences of Unicode characters, while bytes are sequences of raw binary data.
Strings in Python are immutable, meaning that once a string object is created, its contents cannot be modified. On the other hand, bytes objects are mutable and can be modified.
Converting a String to Bytes Using the encode() Method
To convert a string to bytes in Python, you can use the encode() method of strings. The encode() method converts a string to a bytes object using a specified encoding. Here’s an example:
“`python
string = “Hello, world!”
bytes_data = string.encode(‘utf-8’)
“`
The `encode()` method takes the encoding as an argument. In the example above, the string is encoded using the UTF-8 encoding, which is widely used and supports all Unicode characters.
Converting a String to Bytes Using the bytearray() Function
Another way to convert a string to bytes is by using the `bytearray()` function. The `bytearray()` function returns a mutable bytes object, and you can pass a string and the encoding as arguments. Here’s an example:
“`python
string = “Hello, world!”
bytes_data = bytearray(string, ‘utf-8′)
“`
In this example, the `bytearray()` function is used to create a bytearray object from the string. The second argument specifies the encoding to use.
Converting a String to Bytes Using the struct.pack() Function
If you need to convert a string to bytes with a specific format or structure, you can use the `struct.pack()` function from the `struct` module. The `struct.pack()` function formats the data according to a format string and returns a bytes object. Here’s an example:
“`python
import struct
string = “Hello, world!”
bytes_data = struct.pack(’10s’, string.encode(‘utf-8′))
“`
In the example above, the `struct.pack()` function formats the string as a fixed-length field of 10 bytes. The `s` format specifier indicates a string of bytes.
Handling Non-ASCII Characters: Encoding and Error Handling
Python supports various encodings for strings and bytes. When converting a string to bytes, it’s important to consider the encoding and how it handles non-ASCII characters. The most commonly used encoding is UTF-8, which can represent any Unicode character.
If a string contains non-ASCII characters and you try to encode it using an encoding that doesn’t support those characters, a `UnicodeEncodeError` will be raised. To handle this situation, you can use the `encode()` method’s `errors` parameter to specify an error handling policy. The common error handling policies are `strict`, `replace`, `ignore`, and `xmlcharrefreplace`.
Converting Bytes to a String Using the decode() Method
To convert bytes to a string in Python, you can use the `decode()` method of bytes objects. The `decode()` method decodes the bytes object using the specified encoding and returns a string. Here’s an example:
“`python
bytes_data = b’Hello, world!’
string = bytes_data.decode(‘utf-8′)
“`
The `decode()` method takes the encoding as an argument. In the example above, the bytes are decoded using the UTF-8 encoding.
Converting Bytes to a String Using the bytes.decode() Function
Another way to convert bytes to a string is by using the `bytes.decode()` function. The `bytes.decode()` function is a convenience function that calls the `decode()` method of bytes objects. Here’s an example:
“`python
bytes_data = b’Hello, world!’
string = bytes_data.decode(‘utf-8’)
“`
In this example, the `bytes.decode()` function is used to decode the bytes object using the UTF-8 encoding.
FAQs:
Q: How can I convert a string to bytes in Python?
A: There are several methods you can use to convert a string to bytes in Python. You can use the `encode()` method of strings, the `bytearray()` function, or the `struct.pack()` function.
Q: What is the difference between strings and bytes in Python?
A: Strings represent sequences of Unicode characters, while bytes represent sequences of raw binary data. Strings are immutable, while bytes are mutable.
Q: How can I handle non-ASCII characters when converting a string to bytes?
A: You can specify an error handling policy using the `errors` parameter of the `encode()` method. Common error handling policies are `strict`, `replace`, `ignore`, and `xmlcharrefreplace`.
Q: How can I convert bytes to a string in Python?
A: You can use the `decode()` method of bytes objects or the `bytes.decode()` function.
Q: What is the most commonly used encoding in Python?
A: The most commonly used encoding in Python is UTF-8, which can represent any Unicode character.
In conclusion, converting strings to bytes and vice versa is a common task when working with text or binary data in Python. Understanding the different methods and considerations for handling non-ASCII characters is crucial for correctly converting between strings and bytes. With the information provided in this article, you should now have a solid understanding of how to convert strings to bytes in Python.
Python 3 How To Convert String To Bytes
Keywords searched by users: convert string to bytes python String to bytes, Hex string to byte array Python, Base64 to bytes python, Int to byte Python, Convert String to byte array, Convert string to byte C#, Convert bytes to base64 Python, Bytearray() trong Python
Categories: Top 100 Convert String To Bytes Python
See more here: nhanvietluanvan.com
String To Bytes
In the world of programming, the conversion between different data types is a crucial task. One such conversion that is often encountered is converting a string into bytes. Understanding how this conversion works and exploring its usage can significantly enhance the capabilities of a programmer. In this article, we will delve deep into the concept of converting strings to bytes, walk through the process, and unravel its significance. So, let’s dive in!
What does it mean to convert a string to bytes?
In simple terms, converting a string to bytes refers to transforming a sequence of characters into a sequence of binary values. Since computers primarily operate on binary data, this conversion allows us to manipulate and process strings more efficiently. Converting strings to bytes also aids in the storage and transmission of string data.
How does the conversion process work?
The process of converting a string to bytes involves encoding the characters of the string into their corresponding byte values. The encoding scheme used can vary, but one of the most commonly used schemes is the UTF-8 encoding. UTF-8 can represent all possible characters in the Unicode standard, making it a widely adopted choice for string-to-bytes conversion.
To perform the conversion, the following steps are generally followed:
1. Choose an encoding scheme: Before converting a string to bytes, programmers need to select an appropriate encoding scheme based on the requirements of their project. Often, UTF-8 is the go-to choice due to its versatility.
2. Encode the string: Once the encoding scheme is determined, the string is encoded character by character. Each character is assigned a unique binary value according to the encoding scheme. These binary values collectively form the byte representation of the string.
3. Storage/transmission of bytes: The resulting byte sequence can then be stored in a file, database, or transmitted over a network, depending on the specific use case. The byte representation allows efficient handling and manipulation of string data across different platforms and systems.
Why is converting strings to bytes important?
1. Compatibility: Converting strings to bytes enables seamless integration between different platforms and systems. By converting a string to bytes using a widely accepted encoding scheme like UTF-8, you ensure that the string can be accurately interpreted and processed regardless of the underlying system’s architecture.
2. Security: In many scenarios, converting sensitive information, such as passwords or personal data, into bytes is crucial for secure storage or transmission. Byte representations are less susceptible to unintended data leaks or unauthorized access, making them integral to secure programming practices.
3. Network communication: Converting strings to bytes is essential when sending or receiving data over a network. Network protocols typically rely on bytes for efficient transmission. By converting strings to bytes, programmers can ensure smooth communication between different devices and systems.
FAQs:
Q1. Are all characters encoded into a single byte during the conversion?
No, not always. The number of bytes required to encode a character depends on the encoding scheme used. In UTF-8, for example, characters can be encoded using one to four bytes, depending on the character’s Unicode value.
Q2. Can converting a string to bytes cause data loss?
Not necessarily. As long as an appropriate encoding scheme is used, all characters in the string can be accurately represented as bytes. However, if an incompatible encoding scheme is chosen, data loss or incorrect interpretation of characters may occur.
Q3. How do I convert bytes back to a string?
To convert bytes back to a string, you need to perform the reverse process, known as decoding. The decoding process involves interpreting the byte values based on the chosen encoding scheme and converting them back into their original character representation.
Q4. Can I choose an encoding scheme other than UTF-8 for string-to-bytes conversion?
Yes, there are several encoding schemes available, such as ASCII, UTF-16, and UTF-32. The choice of encoding scheme depends on the specific requirements of your project and the range of characters you need to represent.
In conclusion, converting strings to bytes is a fundamental concept in programming. It allows seamless integration, enhances security, and ensures efficient data transmission. By understanding the conversion process and selecting an appropriate encoding scheme, programmers can leverage the power of string-to-bytes conversion to develop robust and interoperable applications.
Hex String To Byte Array Python
In programming, hexadecimal (hex) strings are often encountered when dealing with various data formats such as binary files, network protocols, and cryptography. Converting a hex string to a byte array is a common task required to manipulate and process such data. Fortunately, Python provides built-in functions that allow us to easily perform this conversion.
Understanding Hexadecimal Representation
Before diving into the conversion process, let’s briefly recap what hexadecimal representation means. In contrast to the decimal system which uses 10 digits (0-9), hexadecimal uses 16 digits (0-9 and A-F) to represent values. Each hexadecimal digit represents a four-bit segment, allowing for more compact representation of binary data. For example, the hex digit ‘A’ represents the binary value ‘1010’, and ‘FF’ represents ‘11111111’ in binary.
Conversion using int() and bytes.fromhex() functions
Python provides two primary functions for converting a hex string into a byte array: int() and bytes.fromhex(). The int() function is used to convert a string representation of a number to its integer value. By passing the hex string and the base (16 in this case) to int(), we can obtain the decimal representation of the hex string. However, this alone does not give us a byte array; we still need to convert the decimal value to bytes. This is where the bytes.fromhex() function comes into play.
Let’s take a look at a simple example to illustrate this process:
“`python
hex_string = “48656c6c6f20576f726c64” # Hex string representing “Hello World”
decimal_value = int(hex_string, 16)
byte_array = decimal_value.to_bytes((decimal_value.bit_length() + 7) // 8, ‘big’)
“`
In this example, we start with the hex string “48656c6c6f20576f726c64”, which represents the ASCII characters “Hello World”. By converting it to its decimal equivalent using int(), we obtain the value 5121047115466743815917483442943278437156. We then utilize the to_bytes() method to convert this decimal value into a byte array. The first argument specifies the number of bytes desired, calculated based on the bit length of the decimal value. By passing ‘big’ as the second argument, we define the byte order as big-endian, which places the most significant byte first.
Conversion using bytearray.fromhex() function
Python also provides the bytearray.fromhex() function as an alternative to bytes.fromhex(). The bytearray.fromhex() function directly converts the hex string to a bytearray object, eliminating the need for the intermediate step of converting to an integer first. However, note that the resulting bytearray is mutable, meaning its elements can be modified, while a bytes object is immutable.
Let’s see an example of using bytearray.fromhex():
“`python
hex_string = “48656c6c6f20576f726c64” # Hex string representing “Hello World”
byte_array = bytearray.fromhex(hex_string)
“`
In this example, we directly convert the hex string to a bytearray by using bytearray.fromhex(). The resulting byte array will be the same as in the previous example.
FAQs
Q1. Can I convert a byte array back to a hex string in Python?
A1. Yes, you can convert a byte array back to a hex string using the built-in hexadecimal representation with the hex() or the binascii.hexlify() functions. The hex() function returns a string of hexadecimal digits representing the integer values of the elements in the byte array. On the other hand, the binascii.hexlify() function returns the hexadecimal representation of the byte array as a binary data string.
Q2. Can I convert a hex string with odd length to a byte array?
A2. Yes, you can convert a hex string with an odd length to a byte array. However, an odd-length hex string will result in a byte array with an additional zero byte at the beginning. This zero byte is added to preserve the byte alignment required for processing the data.
Q3. How can I handle hex strings with leading zeros in Python?
A3. Python automatically handles hex strings with leading zeros. The bytes.fromhex() and bytearray.fromhex() functions both recognize and process hex strings with any number of leading zeros. The resulting byte array will maintain the correct byte order and size, regardless of the number of leading zeros.
Q4. Is there a way to handle invalid hex strings or exceptions during conversion?
A4. Yes, you can use exception handling mechanisms, such as try/except blocks, to handle invalid hex strings or exceptions during conversion. By wrapping the conversion code within a try block and implementing appropriate error handling in the except block, you can gracefully handle cases where the hex string is not valid or unexpected errors occur.
Conclusion
Converting a hex string to a byte array is a fundamental operation when working with data formats that involve hexadecimal representation. Python provides intuitive and efficient methods, including int(), bytes.fromhex(), and bytearray.fromhex(), to perform this conversion seamlessly. By leveraging these built-in functions, you can effortlessly manipulate and process hex string data in your Python programs.
Images related to the topic convert string to bytes python
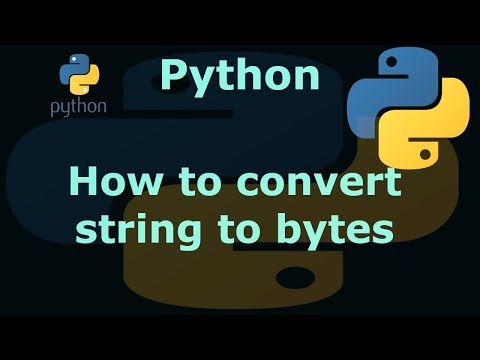
Found 20 images related to convert string to bytes python theme
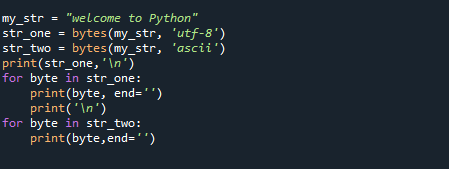







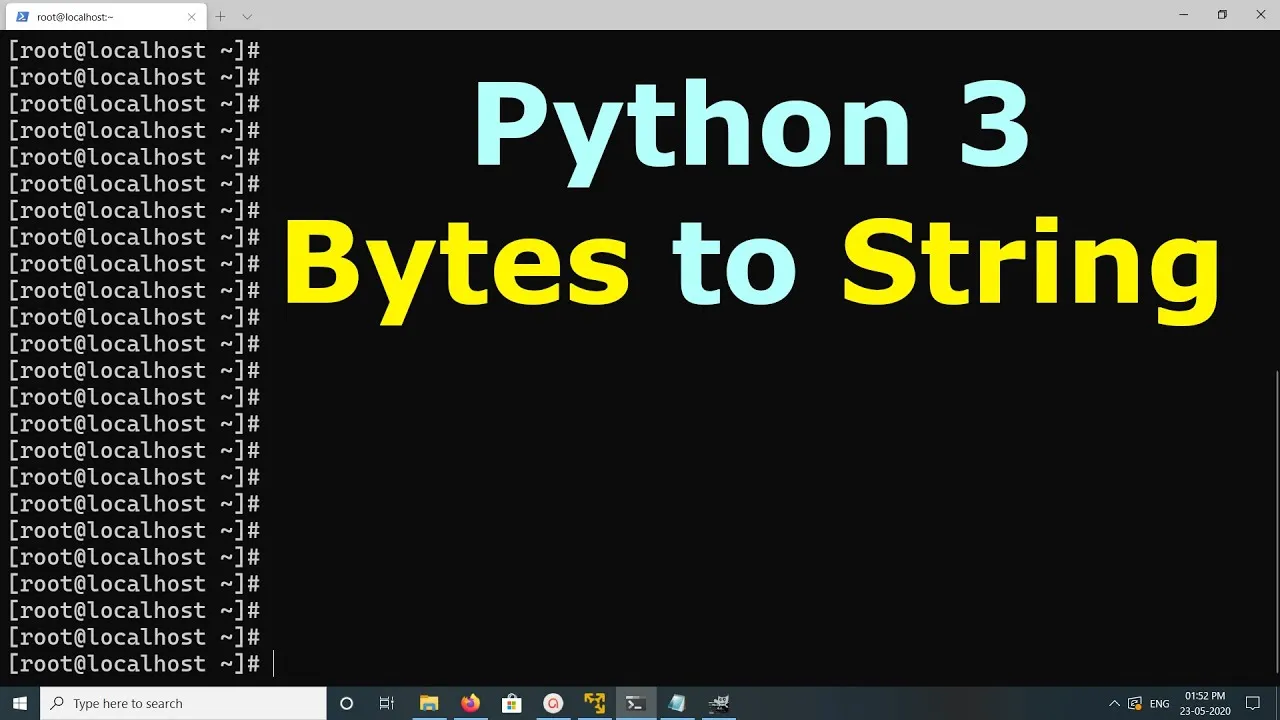


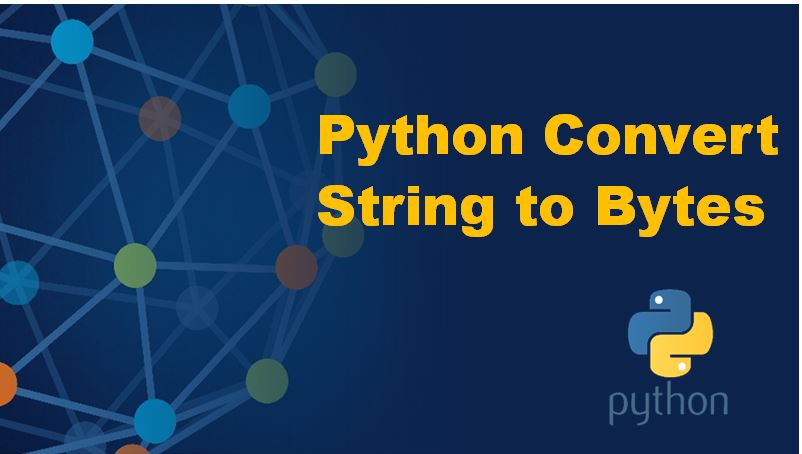

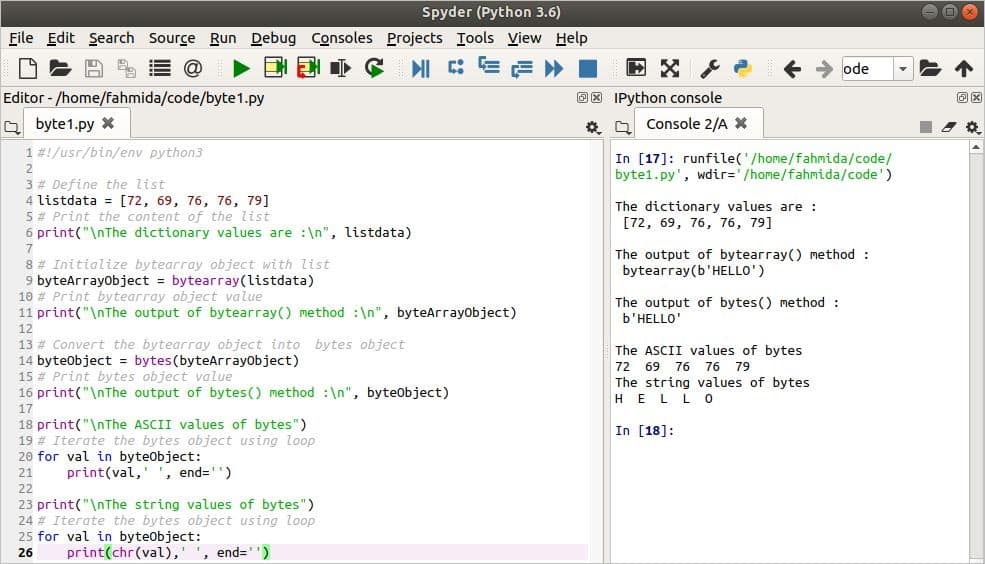
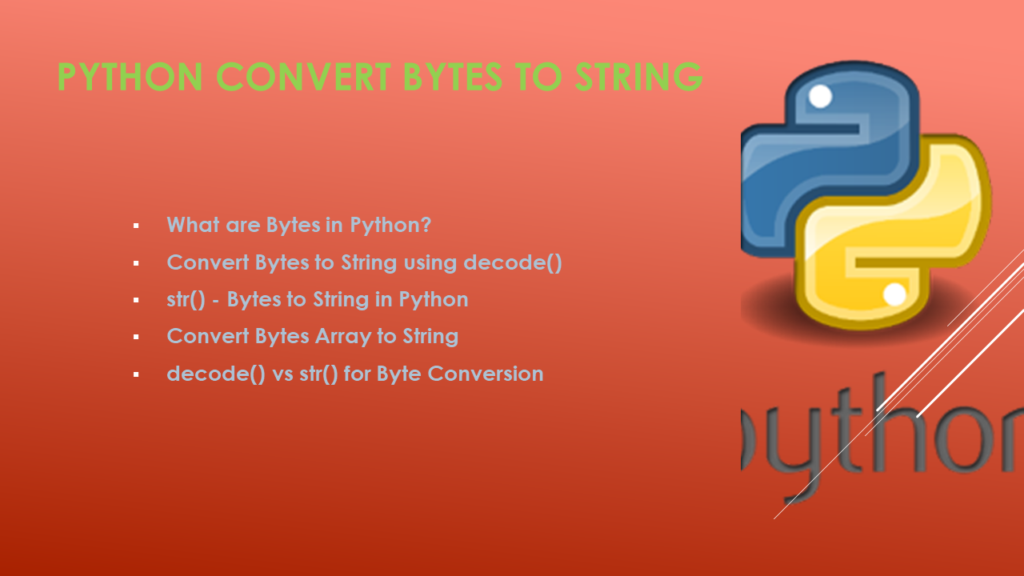
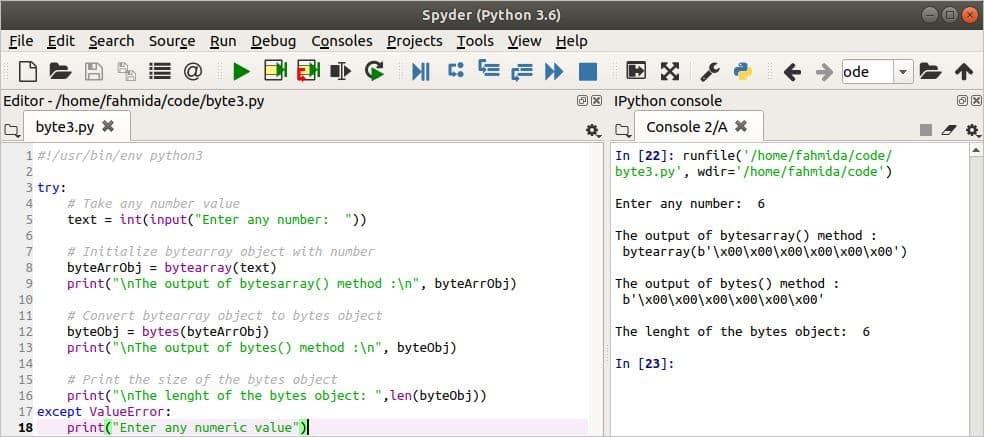
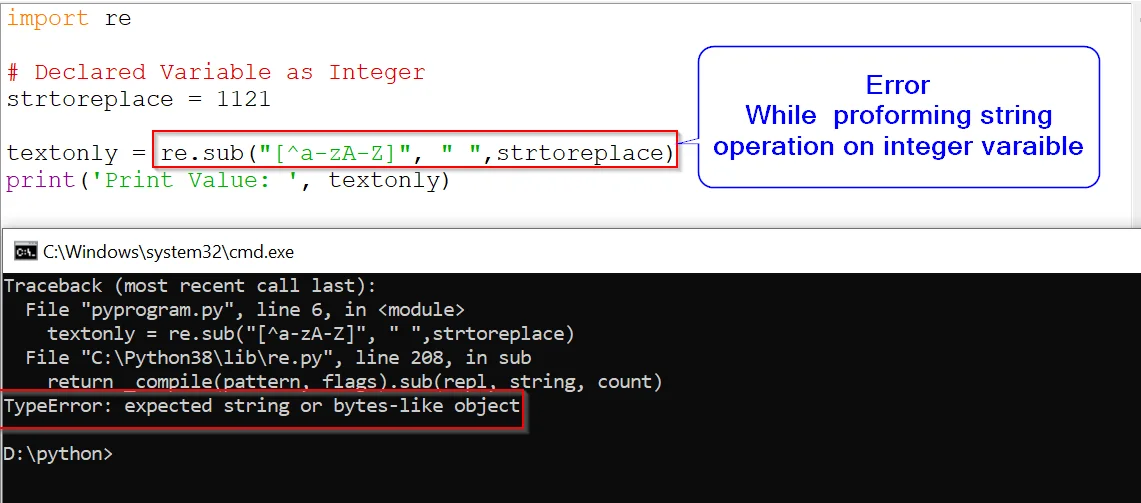

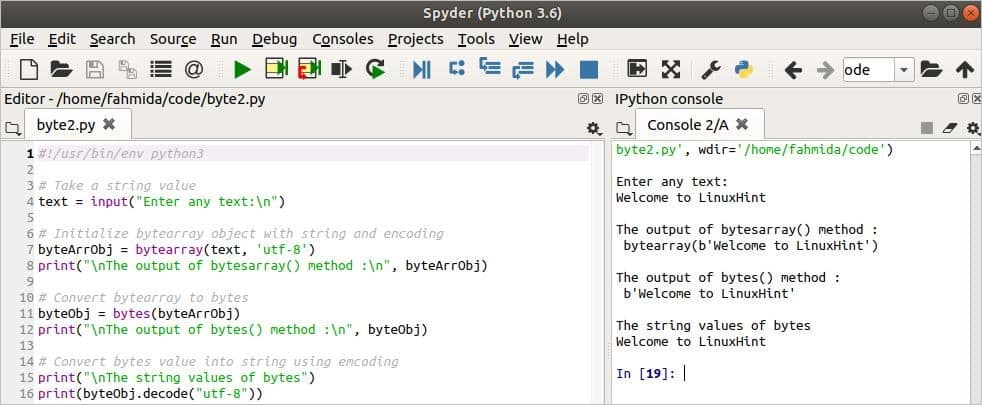


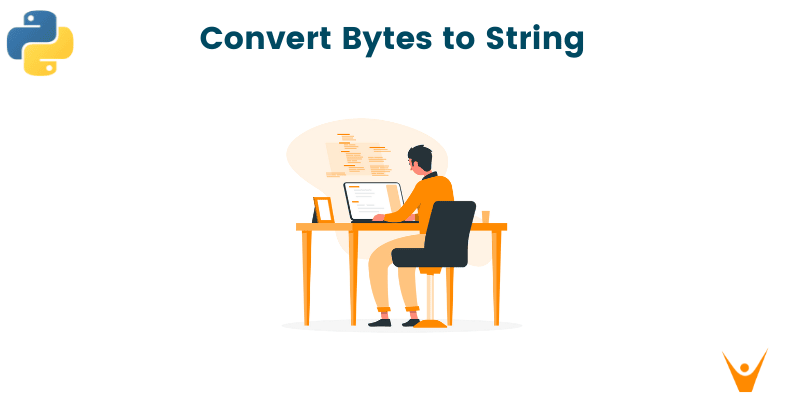

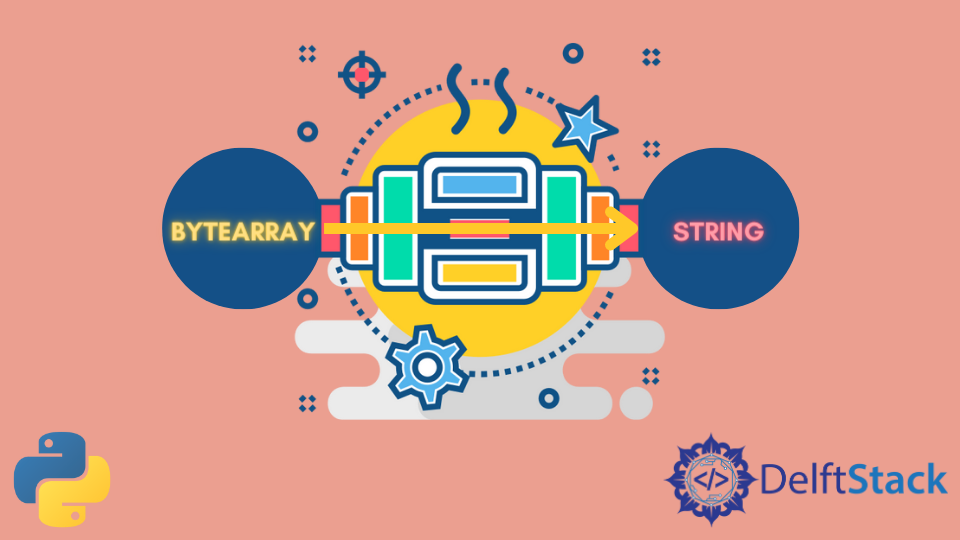

![Converting Bytes To String In Python [Guide] Converting Bytes To String In Python [Guide]](https://cd.linuxscrew.com/wp-content/uploads/2021/03/Converting-Bytes-To-String.png)

![Convert Bytes to String [Python] - YouTube Convert Bytes To String [Python] - Youtube](https://i.ytimg.com/vi/BV5FZtQHsaI/maxresdefault.jpg)


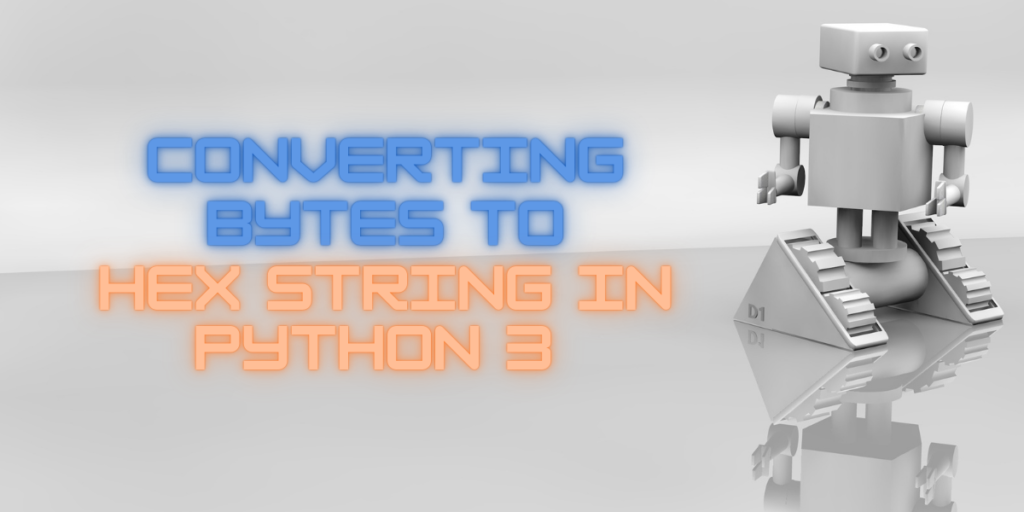

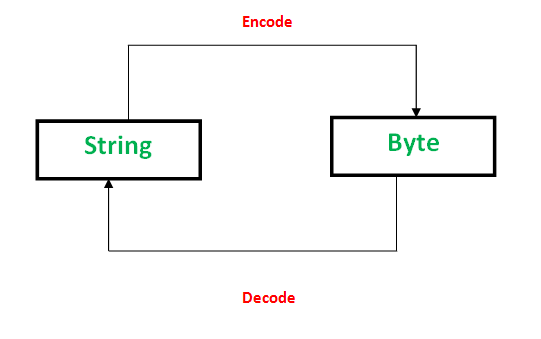
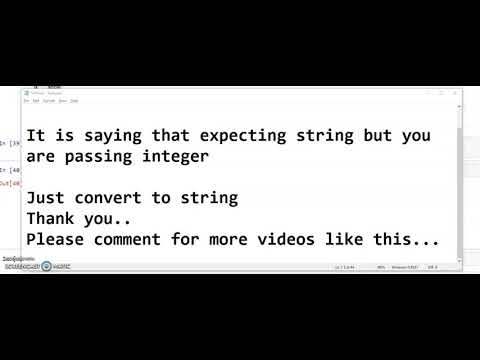

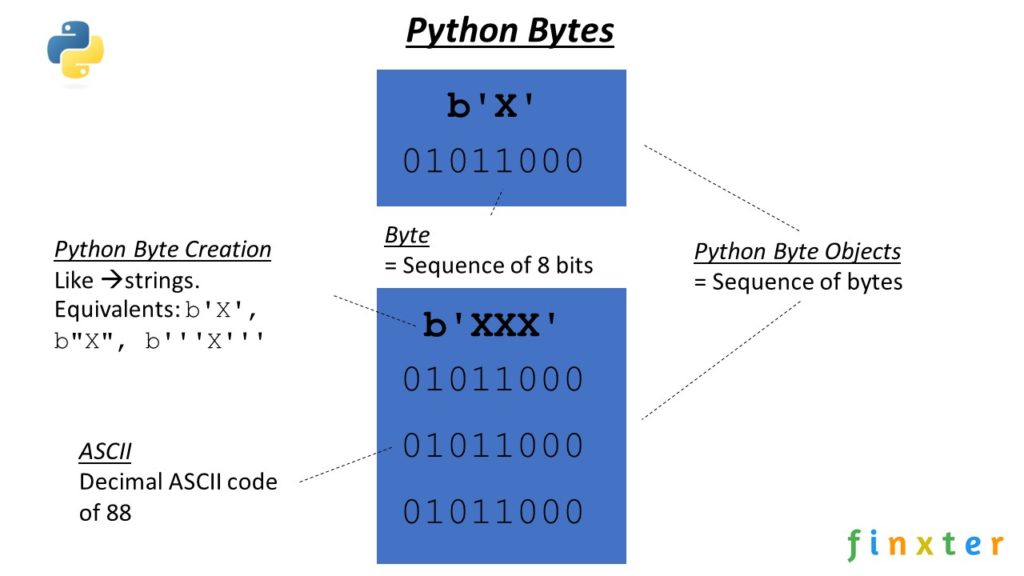
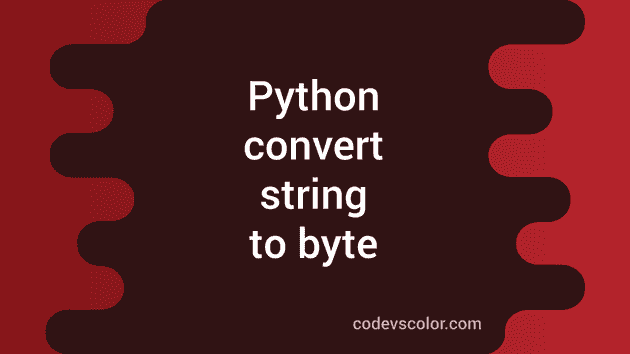


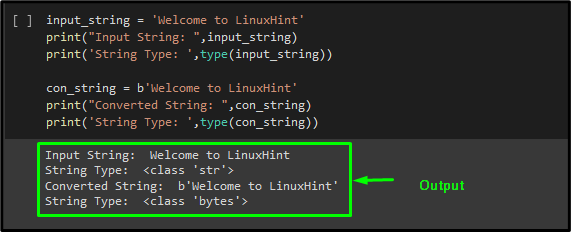




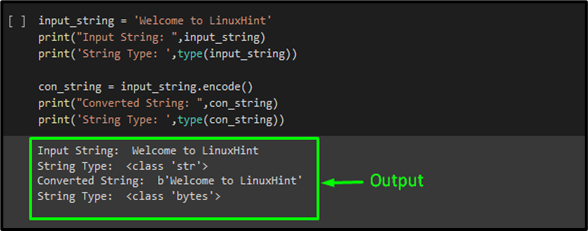
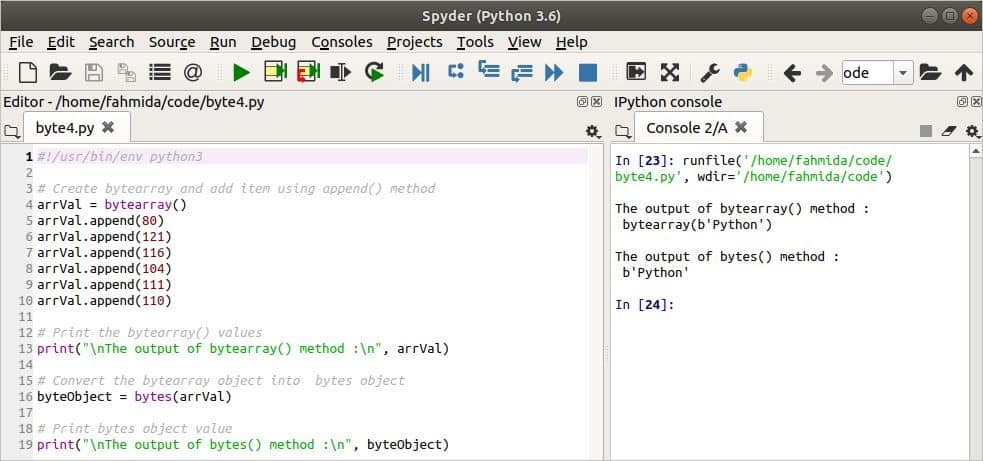
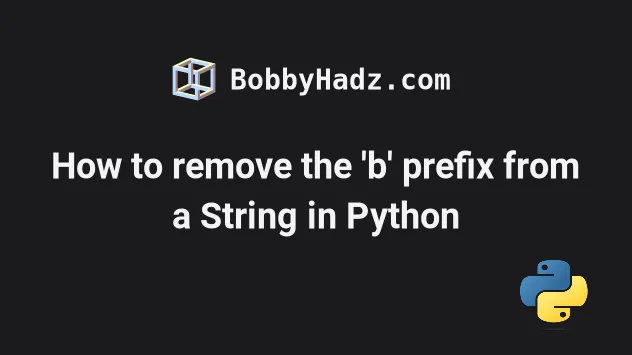
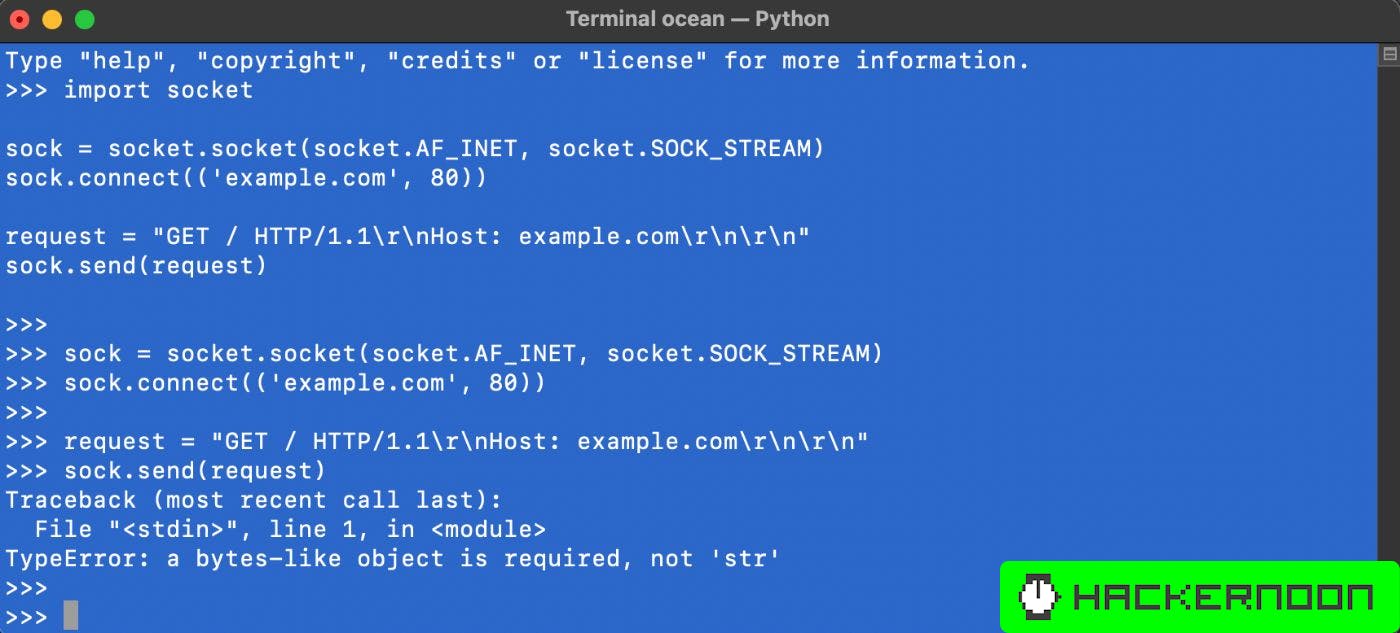
Article link: convert string to bytes python.
Learn more about the topic convert string to bytes python.
- How to convert strings to bytes in Python – Educative.io
- Python | Convert String to bytes – GeeksforGeeks
- Best way to convert string to bytes in Python 3? – Stack Overflow
- Best way to convert string to bytes in Python – Edureka
- How To Convert Python String To Byte Array With Examples
- Python Convert String to Bytes – Linux Hint
- Python Convert Bytes to String – Spark By {Examples}
- How to Convert a String to Bytes Using Python – Pierian Training
- How to convert a string to bytes in Python? – thisPointer
- Python Bytes to String – How to Convert a Bytestring
See more: https://nhanvietluanvan.com/luat-hoc/