Go Check If Variable Is Empty
In programming, it is often necessary to check whether a variable holds a value or is empty. An empty variable can lead to unexpected behavior or errors in an application. In this article, we will delve into the concept of an empty variable, explore different types of variables that can be empty, understand the importance of checking for empty variables, and discuss various techniques and best practices to efficiently perform these checks. We will particularly focus on checking for empty variables in the Go programming language (Golang), and provide examples and insights for each topic.
Understanding the Concept of an Empty Variable
An empty variable refers to a situation where a variable does not hold any valid value. It could be uninitialized or explicitly set as empty. Depending on the programming language and variable type, an empty variable can be represented by various values, including null, undefined, zero, or an empty string. Identifying the specific type of empty variable is crucial for implementing the appropriate check.
Identifying the Different Types of Variables that can be Empty
Several types of variables can be empty, such as strings, numbers, arrays, objects, or even custom data structures defined in a programming language. Each type may require a different approach to determine if it is empty. For instance, an empty string can be defined as either an empty string literal (e.g., “”) or a string with only whitespace characters. Understanding the nuances of each variable type is essential when checking for emptiness.
Exploring the Importance of Checking for Empty Variables
Checking for empty variables is vital to ensure the correctness and reliability of a program. It helps prevent unexpected crashes, null pointer exceptions, or incorrect data manipulation. By verifying whether a variable is empty, developers can handle different scenarios appropriately, providing better error handling, improved user experiences, and overall robustness in their code.
Using Conditional Statements to Check if a Variable is Empty
One common technique to verify if a variable is empty is to use conditional statements. In Golang, a straightforward approach is to apply if-else conditions to examine the variable’s value. For strings, one can use the len() function to determine if it has a length of zero, indicating an empty string.
The Role of Null and Undefined Values in Empty Variables
Null and undefined values can represent emptiness in certain programming languages. In Golang, null is not a language-defined concept, but Go provides a built-in type called “nil” to indicate the absence of a value in certain types, such as pointers, interfaces, channels, and function types. Understanding how these special values apply to different variable types is crucial when checking for emptiness.
Employing Built-in Functions to Determine if a Variable is Empty
Golang offers various built-in functions that can assist in checking if a variable is empty or not. For example, the fmt.Sprintf() function can be used to format a string and check if it remains empty after processing. Additionally, the reflect.TypeOf() function from the reflect package can determine if a variable is nil or not.
Considerations when Checking for Empty Arrays or Objects
Checking for emptiness becomes slightly more complex when dealing with arrays or objects. In Golang, an empty array has a length of zero, and one can use the len() function to assess its emptiness. Similarly, for empty objects or structs, one can compare the value to its zero-value form to determine emptiness.
Handling Empty Variables in Different Programming Languages
Checking for emptiness varies across different programming languages. While Golang provides specific idiomatic ways to perform these checks, other languages may have different syntax or built-in functions. It is crucial to familiarize oneself with the conventions and tools available in the targeted programming language.
Best Practices for Efficiently Checking if a Variable is Empty
To ensure efficient and reliable code, following best practices when checking for empty variables is essential. Some tips include using the appropriate method based on the variable type, opting for idiomatic approaches provided by the programming language, and clearly documenting the purpose and behavior of empty variables in the codebase.
FAQs:
1. How can I check if a string is empty or whitespace in Golang?
To check if a string is empty or contains only whitespace characters (e.g., spaces, tabs, or line breaks), you can utilize the strings.TrimSpace() function along with the len() function. For example:
“`
func isStringEmpty(s string) bool {
return len(strings.TrimSpace(s)) == 0
}
“`
2. How do I check if a string is not empty in Golang?
The simplest way to check if a string is not empty is by comparing its length to zero using the len() function. If the length is greater than zero, it indicates a non-empty string. Here’s an example:
“`
func isStringNotEmpty(s string) bool {
return len(s) > 0
}
“`
3. How do I check if a string is nil or empty in Golang?
In Golang, string variables cannot be nil, as they are initialized as empty strings by default. Therefore, you only need to check if the string is empty using the len() function. Here’s an example:
“`
func isStringNilOrEmpty(s string) bool {
return len(s) == 0
}
“`
4. How can I check if a variable is initialized in Golang?
In Golang, variables are automatically initialized with their zero values. To check if a variable is initialized, you can compare it with its zero value using the equality operator. For example:
“`
func isVariableInitialized(v int) bool {
return v != 0
}
“`
5. How do I check if a string is null in Golang?
In Golang, the concept of null does not exist for most types, including strings. Instead, the “nil” value is used for certain types such as pointers, interfaces, channels, and function types. Therefore, you do not need to check for null specifically for strings in Golang.
6. How can I check if a struct is empty in Golang?
To check if a struct is empty in Golang, you can compare it with its zero-value form using the equality operator. If all the fields of the struct hold their zero values, it indicates an empty struct. Here’s an example:
“`
type Person struct {
Name string
Age int
}
func isStructEmpty(p Person) bool {
return p == Person{}
}
“`
7. How do I check if a variable is null in Golang?
In Golang, the concept of null applies to certain types such as pointers, interfaces, channels, and function types. To check if a variable of such types is null, you can compare it with the “nil” value using the equality operator. For example:
“`
func isVariableNull(p *Person) bool {
return p == nil
}
“`
8. How can I check if a struct is empty in Golang?
To check if a struct is empty in Golang, you can compare it with its zero-value form using the equality operator. If all the fields of the struct hold their zero values, it indicates an empty struct. Here’s an example:
“`
type Person struct {
Name string
Age int
}
func isStructEmpty(p Person) bool {
return p == Person{}
}
“`
In conclusion, checking if a variable is empty is a crucial aspect of programming that requires careful consideration. By understanding the concept of an empty variable, identifying types of variables that can be empty, and employing appropriate techniques and best practices, developers can ensure the reliability and effectiveness of their code. In the context of Golang, the provided examples and insights should assist programmers in efficiently performing these checks and handling empty variables.
41 Checking If Variable Is Empty
How To Check If Input Is Empty In Golang?
In software development, it is a common task to check if user input or a string variable is empty. Empty input can often cause errors or unexpected behavior in an application, so it is crucial to handle empty input appropriately. In this article, we will explore different methods to check if input is empty in the Go programming language (Golang) and discuss their pros and cons.
Method 1: Using the len() function
One of the simplest ways to check if a string is empty in Golang is to use the len() function. The len() function returns the number of characters in a string. If the length of the input is 0, it means the input is empty.
Here’s an example:
“`
input := “Hello, World!”
if len(input) == 0 {
fmt.Println(“Input is empty!”)
} else {
fmt.Println(“Input is not empty!”)
}
“`
Method 2: Using the strings.TrimSpace() function
The strings package in Golang provides a useful function called TrimSpace(). This function removes leading and trailing spaces from a string and returns a copy of the string. If the resulting string has a length of 0, it means the input was empty or contained only spaces.
Here’s an example:
“`
input := ” ”
if len(strings.TrimSpace(input)) == 0 {
fmt.Println(“Input is empty!”)
} else {
fmt.Println(“Input is not empty!”)
}
“`
Method 3: Using regular expressions
Regular expressions can be a powerful tool for string manipulation and validation. In Golang, the built-in regex package can be used to check if a string is empty or contains only spaces.
Here’s an example:
“`
import “regexp”
input := ” ”
re := regexp.MustCompile(`^\s*$`)
if re.MatchString(input) {
fmt.Println(“Input is empty!”)
} else {
fmt.Println(“Input is not empty!”)
}
“`
Method 4: Using the strings.Compare() function
The strings package in Golang also provides a Compare() function that can be used to compare two strings. By comparing the input with an empty string, we can determine if the input is empty or not.
Here’s an example:
“`
input := “”
if strings.Compare(input, “”) == 0 {
fmt.Println(“Input is empty!”)
} else {
fmt.Println(“Input is not empty!”)
}
“`
FAQs:
Q1: Are there any performance differences between these methods?
A1: Yes, there can be slight performance differences. Method 1 (using len()) and Method 2 (using strings.TrimSpace()) are generally faster compared to Method 3 (using regular expressions) due to the overhead of regular expression matching. However, the performance difference might not be significant unless checking for empty input is done on a large scale.
Q2: Can these methods be used for other data types like integers or arrays?
A2: No, these methods are specifically designed to check empty strings. For other data types, you may need to use appropriate methods or functions.
Q3: When should I use one method over another?
A3: The choice of method depends on your specific use case and requirements. If you only need to check if the input is empty or contains only spaces, Method 2 (using strings.TrimSpace()) might be the simplest and most readable option. If you need more complex validation, regular expressions (Method 3) might be suitable. Consider the trade-offs between simplicity, performance, and accuracy when choosing a method.
Q4: Is there any difference between an empty string and a string with only spaces?
A4: Yes, there is a difference. An empty string is a string with no characters, whereas a string with only spaces has characters (spaces, in this case). The methods discussed in this article can differentiate between an empty string and a string with only spaces.
In conclusion, checking if input is empty is an important task in software development. By using techniques such as comparing lengths, trimming spaces, or using regular expressions, we can accurately determine if user input or a string variable is empty in Golang. Choosing the right method depends on the specific use case and requirements of your application. Remember to handle empty input appropriately to avoid errors and unexpected behavior in your programs.
How To Check Zero Value In Golang?
In the world of programming, understanding how to check for the zero value in a language is crucial. Golang, also known as Go, provides several ways to verify if a variable holds a zero value or not. In this article, we will explore methods to determine zero values in Golang and further discuss scenarios where this knowledge is particularly useful.
Checking Zero Value in Golang
Before diving into the various approaches, it is essential to understand what zero values are in Golang. In Go, each variable receives a default value during initialization. The default value usually depends on the variable’s data type. A zero value represents the default value for a particular variable type, indicating that no other value has been assigned to it.
Now let’s examine the different techniques for checking zero values in Golang:
1. Comparison with Zero:
In Golang, a variable can be directly compared with its zero value or its respective type’s zero value. For instance, if we have a variable named “age” of type int, we can simply check if age == 0. If the condition evaluates to true, the variable holds a zero value; otherwise, it does not.
2. Zero Value Function:
Golang also provides a built-in function called “zeroValue” to determine the zero value of any variable. This function takes the variable as its input and returns true if the variable holds a zero value, and false otherwise. This approach is particularly useful if you have a generic function that needs to handle different types of variables.
3. Reflect Package:
The Golang “reflect” package offers a more advanced method to check zero values. By utilizing reflection, we can inspect the type and value of variables dynamically. The “reflect” package includes the “Zero” function, which returns true if a variable holds a zero value and false otherwise. Although this approach might be more complex, it allows checking zero values across different types without explicitly comparing them.
4. Pointers:
In Golang, pointers play a vital role in memory management and can also be helpful in determining zero values. We can declare a pointer variable and assign it the memory address of the variable we want to check. By comparing the pointer variable against nil, we can infer if the original variable holds a zero value or not. However, this method is only suitable for pointers and not for all variable types.
When to Check Zero Value
Knowing how to check zero values in Golang can be particularly useful in various scenarios. Let’s explore some frequently asked questions to understand the practical significance of zero value checks:
FAQs
Q: When should I check for zero value in Golang?
A: Checking for zero values is essential when handling variables passed as function arguments or when initializing variables within your code. It helps in avoiding unexpected behaviors and making informed decisions based on the state of variables.
Q: Why is checking zero value important?
A: By determining if a variable holds a zero value, you can handle exceptional cases, provide default values, or identify uninitialized variables. It helps maintain program correctness and avoids potential errors or crashes.
Q: Can I use zero values for conditions directly in Golang?
A: Yes, Golang supports conditional checks using zero values within if statements or logical operators. For example, if a variable holds a zero value, it can be utilized to execute specific code paths or handle specific cases.
Q: How does checking zero value help with error handling?
A: When a function returns a value indicating an error, checking if the returned value is a zero value can help identify if the operation was successful or encountered an error. This approach is widely used in Go to handle errors gracefully.
Q: Are there any exceptions or special cases to consider while checking zero values?
A: Yes, it is crucial to understand the zero values for each data type in Golang. For example, an uninitialized boolean variable holds false as its zero value, while uninitialized integer variables would be assigned 0.
Q: Can I redefine zero values for a custom data type?
A: No, Golang defines zero values for each data type, and they cannot be modified. However, you can define struct types and use those to create custom zero value checks relevant to your application’s requirements.
Q: Are there performance implications when checking zero value using reflection?
A: Yes, using reflection for zero value checks can result in slower execution compared to direct comparisons. Reflection involves additional overhead, making it less performant. Therefore, it is recommended to use reflection only where necessary or with caution.
Conclusion
In this article, we explored various methods to check for zero values in Golang. We discussed comparison with zero, the zeroValue function, utilizing the reflect package, and pointers as approaches to verify zero values. Understanding zero values is crucial, as it helps in handling variables and conditions efficiently, avoiding potential errors, and ensuring program correctness. By grasping these concepts, Golang developers can write more reliable and robust code.
Keywords searched by users: go check if variable is empty golang check if string is empty or whitespace, golang check if string is not empty, golang check string nil or empty, golang check if variable is initialized, golang check if string is null, Golang struct check empty, Check null golang, golang check if struct is empty
Categories: Top 73 Go Check If Variable Is Empty
See more here: nhanvietluanvan.com
Golang Check If String Is Empty Or Whitespace
Checking if a string is empty or whitespace has different meanings based on the context. An empty string is when the string variable has no characters at all. On the other hand, whitespace refers to characters such as spaces, tabs, or line breaks.
Method 1: Checking the Length of the String
The simplest way to check if a string is empty or consists of whitespace is by using the string’s length. In Go, the `len()` function returns the length of a given string. By comparing the length with zero, we can determine if the string is empty.
“`go
func isEmptyOrWhitespace(s string) bool {
return len(s) == 0
}
“`
This method works well for empty strings since they have a length of zero. However, it doesn’t consider strings with whitespace. To tackle that, we need additional steps.
Method 2: Trimming Whitespace and Checking Length
To ignore whitespace characters, we can trim them from the string before checking its length. Go’s `strings` package provides a `TrimSpace()` function to remove leading and trailing whitespace from a string.
“`go
import “strings”
func isEmptyOrWhitespace(s string) bool {
trimmed := strings.TrimSpace(s)
return len(trimmed) == 0
}
“`
By applying `TrimSpace()` on the string, any leading or trailing whitespace is removed. The resulting trimmed string is then checked for emptiness using the `len()` function.
Method 3: Matching with Regular Expressions
Regular expressions provide a powerful way to check for various patterns in a string. We can utilize them to check if a string is empty or whitespace. The regular expression pattern `^\s*$` matches strings that consist of zero or more whitespace characters.
“`go
import “regexp”
func isEmptyOrWhitespace(s string) bool {
match, _ := regexp.MatchString(`^\s*$`, s)
return match
}
“`
Here, we use the `MatchString()` function from the `regexp` package, which returns a boolean indicating whether the string matches the given regular expression pattern. Since we are only interested in the boolean result, we discard the error value using `_`.
This method provides flexibility, allowing for more complex pattern matching if the need arises.
Method 4: Looping through Characters
An alternative approach to checking if a string contains only whitespace characters is by iterating through each character in the string and checking if it is a whitespace character. Go characters are represented as UTF-8 code points. We can use the `unicode` package to test each character’s properties.
“`go
import “unicode”
func isEmptyOrWhitespace(s string) bool {
for _, char := range s {
if !unicode.IsSpace(char) {
return false
}
}
return true
}
“`
In this method, we use a `for range` loop to iterate through each character in the string. The `unicode.IsSpace()` function returns true if the given character is a whitespace character.
The loop checks each character and returns `false` as soon as a non-whitespace character is encountered. If no non-whitespace character is found, the loop completes and we can conclude that the string consists solely of whitespace.
FAQs:
Q1: Why use Go for string operations?
A: Go offers several advantages for string operations, such as efficient memory usage, excellent performance, built-in concurrency support, and a rich standard library. Its simplicity and readability make string manipulations easier to implement and maintain.
Q2: Are there any performance differences between these methods?
A: Yes, there are performance differences in these methods. The first method, checking the length directly, is the simplest and generally the fastest. The regular expression method is slightly slower due to the overhead of regular expression matching. Looping through characters has similar performance to the regular expression method but can be optimized in certain scenarios by early termination.
Q3: Can I use these methods to check if a string contains characters other than whitespace?
A: Yes, these methods can be easily modified to check for other conditions. For example, you can modify the regular expression pattern to match a specific pattern or use the loop method to check for certain character properties using the functions provided by the `unicode` package.
In conclusion, checking if a string is empty or consists of only whitespace characters is a common task while working with strings in Go. We have discussed various methods to accomplish this, including using string length, trimming whitespace, regular expressions, and looping through characters. Each method offers a different approach and can be chosen depending on the specific requirements of your application.
Golang Check If String Is Not Empty
In the world of programming, dealing with strings is inevitable. Whether you are building a web application, writing a backend service, or developing an API, efficiently handling strings is a crucial part of the development process. In Go or Golang, a statically typed, compiled programming language, developers have several options when it comes to checking whether a string is empty or not. This article aims to explore different techniques and best practices to determine if a string is not empty in Go.
Understanding Empty Strings
Before diving into the methods of checking if a string is not empty, let’s first clarify what an empty string is. In Go, an empty string refers to a string that contains no characters or has a length of zero. It is represented by `””`.
Method 1: Comparing with Empty String
One of the most straightforward ways to check if a string is not empty in Go is by comparing it with an empty string. This can be done using the equality operator (`==`):
“`go
func isNotEmpty(str string) bool {
return str != “”
}
“`
In this method, the function `isNotEmpty` takes a string as an argument and checks whether it is equal to `””`. If the string is not empty, the expression `str != “”` will evaluate to `true`, indicating a non-empty string.
Method 2: Checking the Length
Another approach to determining if a string is not empty in Go is by checking its length. Go provides the `len()` function, which returns the length of a string. Using the `len()` function, we can easily check if a string has a length greater than zero:
“`go
func isNotEmpty(str string) bool {
return len(str) > 0
}
“`
Here, the function `isNotEmpty` receives a string as its argument and returns `true` if the length of the string is greater than zero.
Method 3: Regular Expressions
Regular expressions provide a powerful tool for pattern matching and string manipulation. Although they might be considered more advanced, they can be harnessed to check if a string is not empty. Here’s an example utilizing regular expressions in Go:
“`go
import “regexp”
func isNotEmpty(str string) bool {
regex := regexp.MustCompile(`\S`)
return regex.MatchString(str)
}
“`
In this method, we utilize the `MatchString()` function from the `regexp` package to check if the string `str` contains any non-whitespace character (represented by `\S` in the regular expression). If a match is found, the string is not empty, and the function returns `true`.
Method 4: Trimmed String
In some cases, strings may contain extra whitespace characters. If you want to consider a string not empty even if it only contains these characters, you can use the `strings.TrimSpace()` function to trim the string and then use one of the previous methods to check if the resulting trimmed string is not empty:
“`go
import “strings”
func isNotEmpty(str string) bool {
trimmedStr := strings.TrimSpace(str)
return len(trimmedStr) > 0
}
“`
Here, the `strings.TrimSpace()` function removes the leading and trailing whitespaces from the string `str`. The resulting trimmed string is then checked for non-emptyness using the length approach discussed earlier.
FAQs
Q: Can I use these methods for checking if a multiline string is not empty?
A: Yes, these methods can be used for both single-line and multiline strings. The methods determine emptiness based on the presence of any character, rather than considering line breaks or other formatting.
Q: Are there any performance differences between the methods?
A: While all the methods discussed above are efficient, there might be some minor performance differences. The equality operator (`==`) and the `len()` function are usually the fastest, while regular expressions can be slightly slower due to additional processing overhead.
Q: What happens if I pass a nil value to these methods?
A: If you pass a nil value as an argument, a runtime error will occur. It is your responsibility as a developer to ensure that the string is not nil before making the check.
Q: Can I modify these methods to check if a string is empty instead?
A: Absolutely! In all the methods mentioned, simply negate the condition (e.g., `return !isNotEmpty(str)` or `return len(str) == 0`) to achieve the opposite result, i.e., checking if a string is empty.
In conclusion, checking if a string is not empty in Go is a fundamental operation that programmers encounter on a regular basis. By leveraging these different approaches – comparing with an empty string, checking the length, using regular expressions, or considering trimmed strings – developers can ensure that their code accurately handles non-empty strings. Each method has its strengths and can be chosen based on specific use cases and requirements. Understanding these techniques will empower Go developers to write more robust and reliable applications while saving valuable development time.
Golang Check String Nil Or Empty
Introduction:
When working with strings in any programming language, one often encounters scenarios where it becomes necessary to check whether a string is nil (null) or empty. In this article, we will explore the various techniques to accomplish this task in the Go programming language, widely known as Golang.
Understanding Nil and Empty Strings:
Before we delve into the methods, it is crucial to understand the difference between nil and empty strings. A string is considered nil when it hasn’t been assigned any value or when it has been explicitly set to nil. On the other hand, an empty string is a string that contains no characters and is represented by “”.
Method 1: Using Comparison Operators:
One of the simplest ways to check if a string is nil or empty is by using the comparison operators. We can compare the string variable with nil or an empty string and take appropriate action accordingly. Here’s an example:
“`go
var str string
if str == “” {
fmt.Println(“String is empty.”)
} else {
fmt.Println(“String is not empty.”)
}
“`
Output:
String is empty.
Method 2: Utilizing len() Function:
The len() function returns the length of a string in Go. By utilizing this function, we can determine whether a string is empty or not. When len() returns 0, the string is empty, and any other value indicates a non-empty string. Here’s an example:
“`go
var str string
if len(str) == 0 {
fmt.Println(“String is empty.”)
} else {
fmt.Println(“String is not empty.”)
}
“`
Output:
String is empty.
Method 3: Using the strings.TrimSpace() Function:
The strings.TrimSpace() function is beneficial in scenarios where we want to check for strings that might contain whitespace characters. It not only checks if a string is empty but also trims leading and trailing whitespace. Here’s an example:
“`go
var str string
if strings.TrimSpace(str) == “” {
fmt.Println(“String is empty or contains only whitespace.”)
} else {
fmt.Println(“String is not empty.”)
}
“`
Output:
String is empty or contains only whitespace.
Method 4: Checking for Nil using Pointers:
In Golang, we can also check if a string is nil by using pointers. Pointers are variables that store the memory address of another variable. Here’s an example of how we can check for nil using pointers:
“`go
var str *string
if str == nil {
fmt.Println(“String is nil.”)
} else {
fmt.Println(“String is not nil.”)
}
“`
Output:
String is nil.
FAQs:
Q1. Can we check for nil or empty strings using regular expressions in Golang?
Yes, regular expressions can be used to check for empty strings, but they might not be the most efficient solution for this specific task. The simple techniques mentioned above are generally sufficient for checking empty or nil strings.
Q2. What happens if we try to access a nil string?
Attempting to access or use a nil string will result in a runtime panic. It is essential to check for nil strings before performing any operations on them to prevent such errors.
Q3. How can I handle nil or empty strings in function parameters?
When working with function parameters, it is recommended to use pointers. By passing a pointer to the string as an argument, you can easily detect if the string is nil or empty within the function.
Conclusion:
Checking whether a string is nil or empty is a common task in programming, including Golang. In this article, we explored several methods to accomplish this task, including comparison operators, len() function, strings.TrimSpace() function, and pointers. By using these techniques, you can effectively handle null or empty strings in your Golang code and prevent potential errors.
Images related to the topic go check if variable is empty
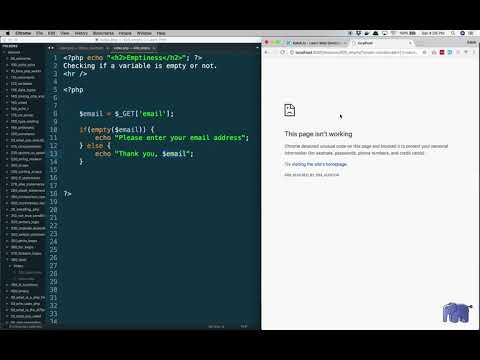
Found 33 images related to go check if variable is empty theme
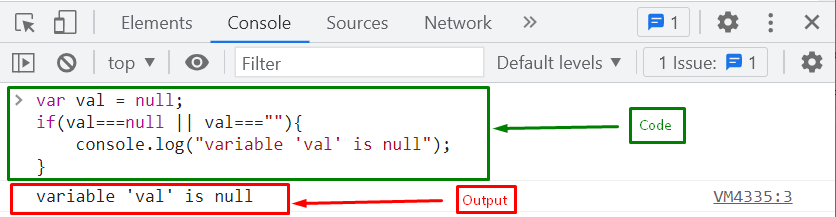
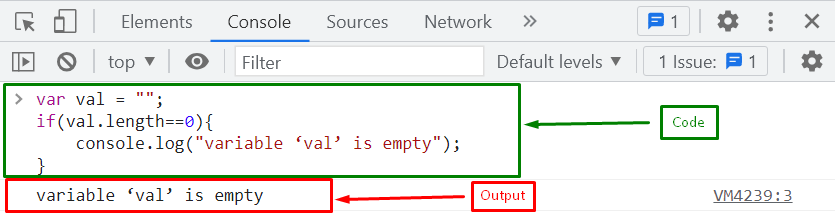
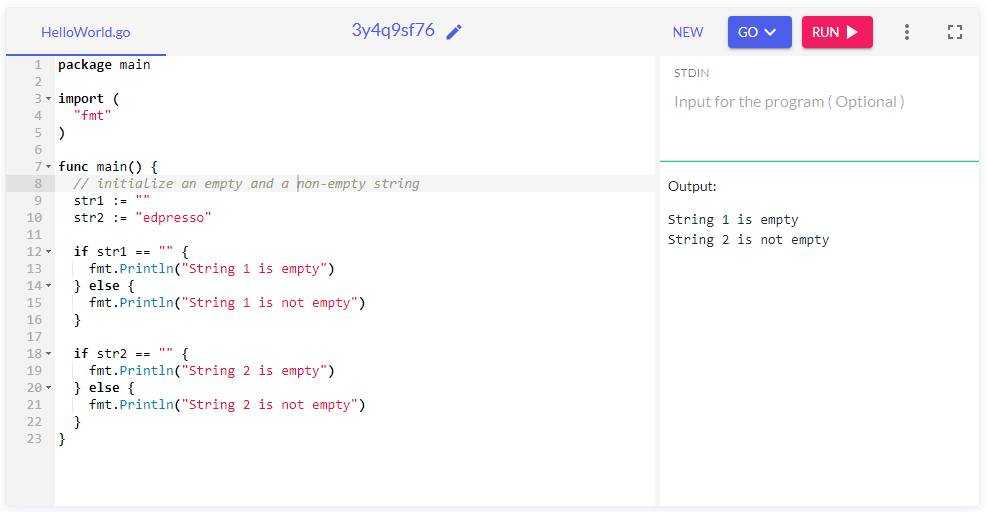
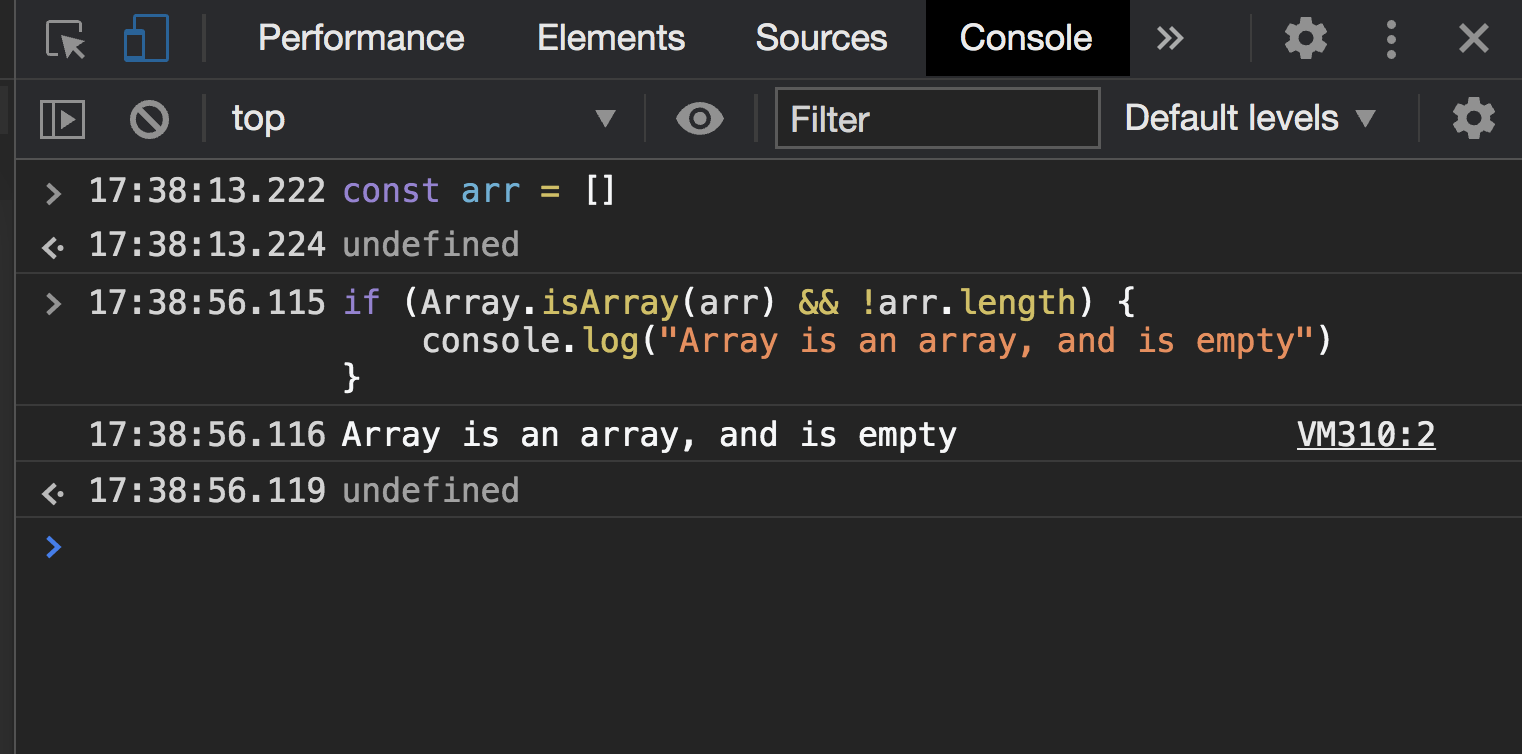


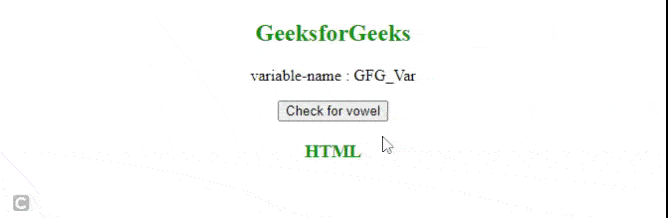

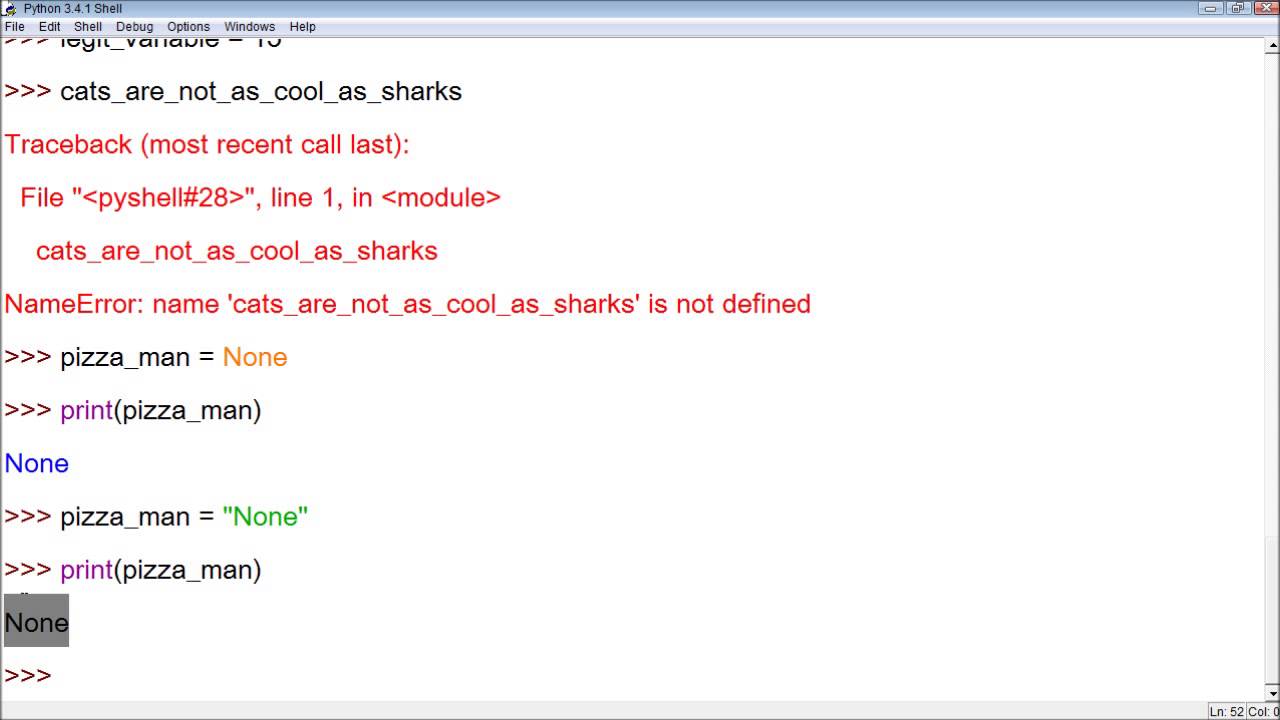
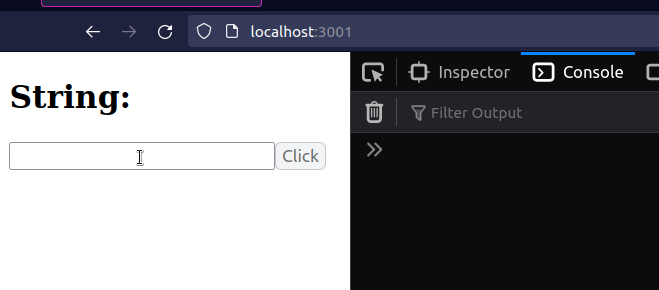
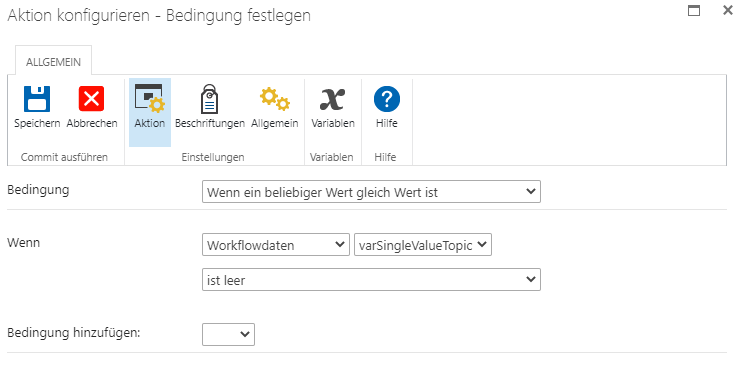
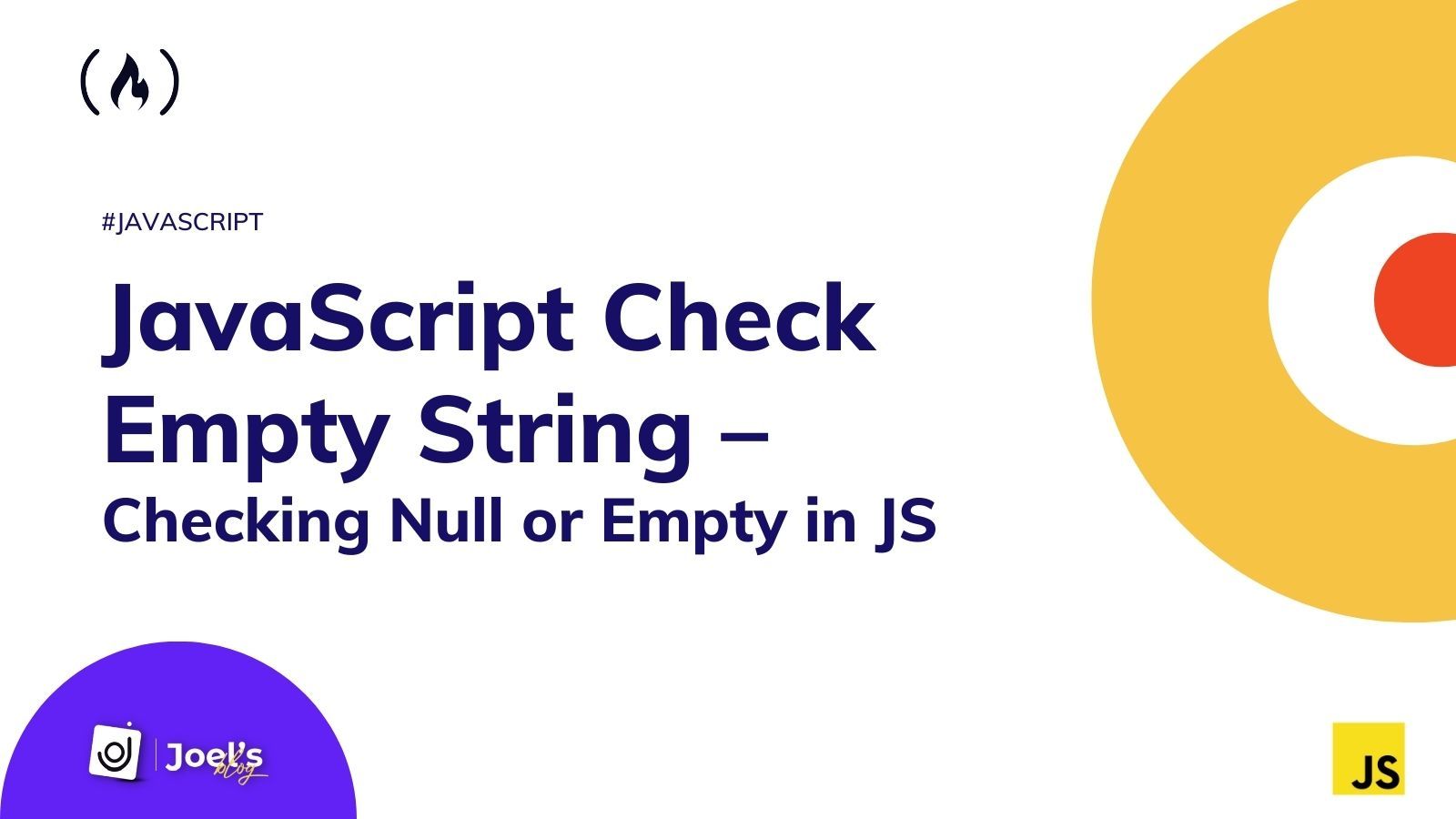
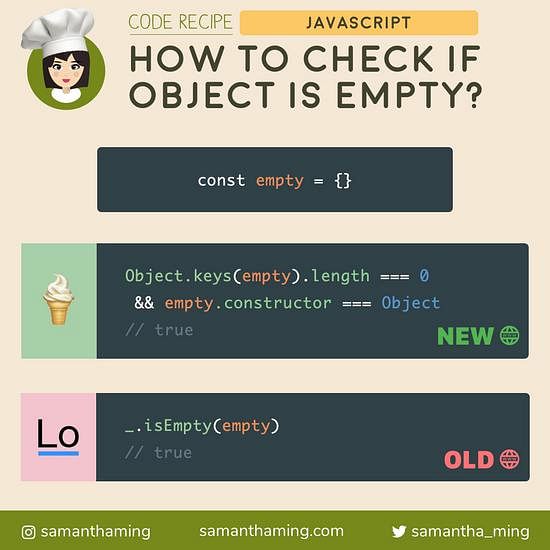
![How to check if struct is empty in GO? [SOLVED] | GoLinuxCloud How To Check If Struct Is Empty In Go? [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/error2.jpg)


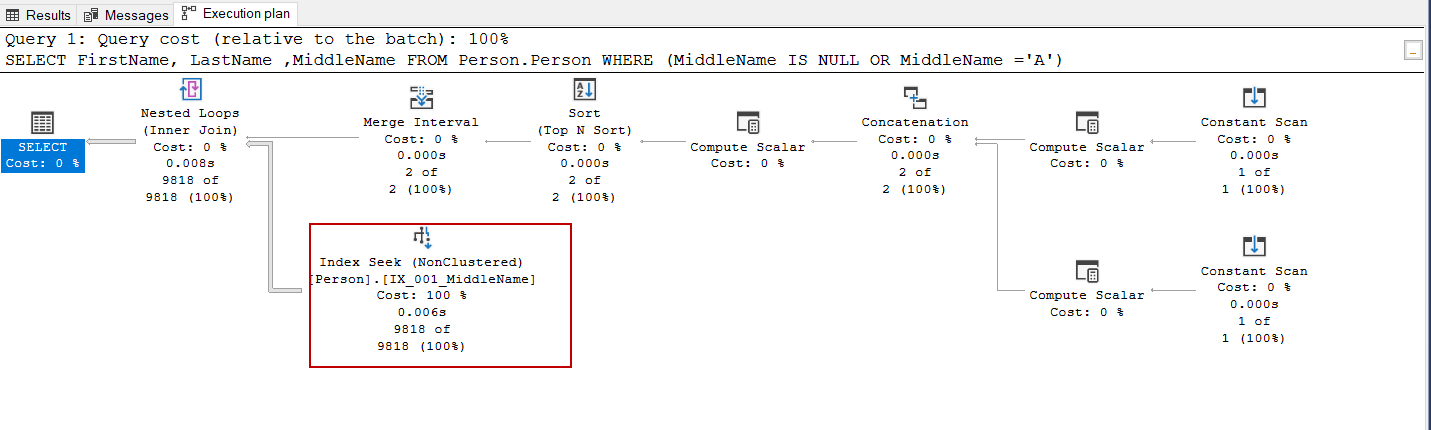
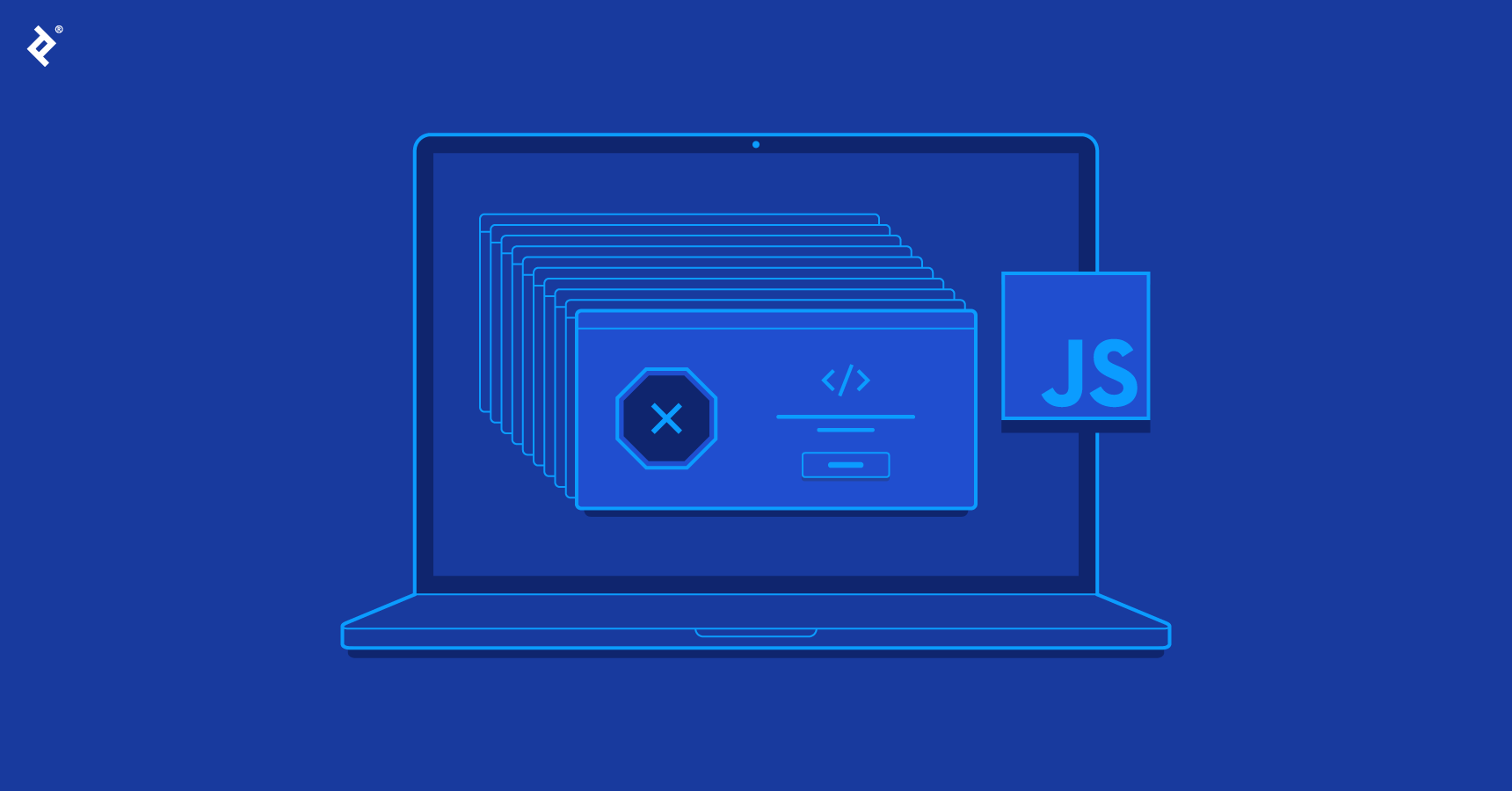
![Power Automate IF Expression [With 51 Examples] - SPGuides Power Automate If Expression [With 51 Examples] - Spguides](https://i0.wp.com/www.spguides.com/wp-content/uploads/2021/11/expression-to-check-for-blank-values-in-power-automate.png)
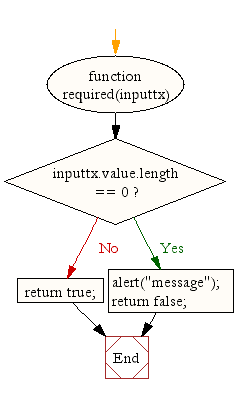

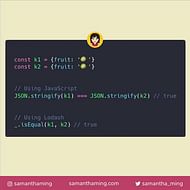
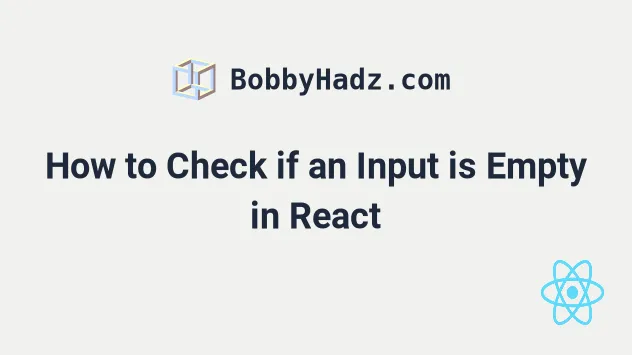
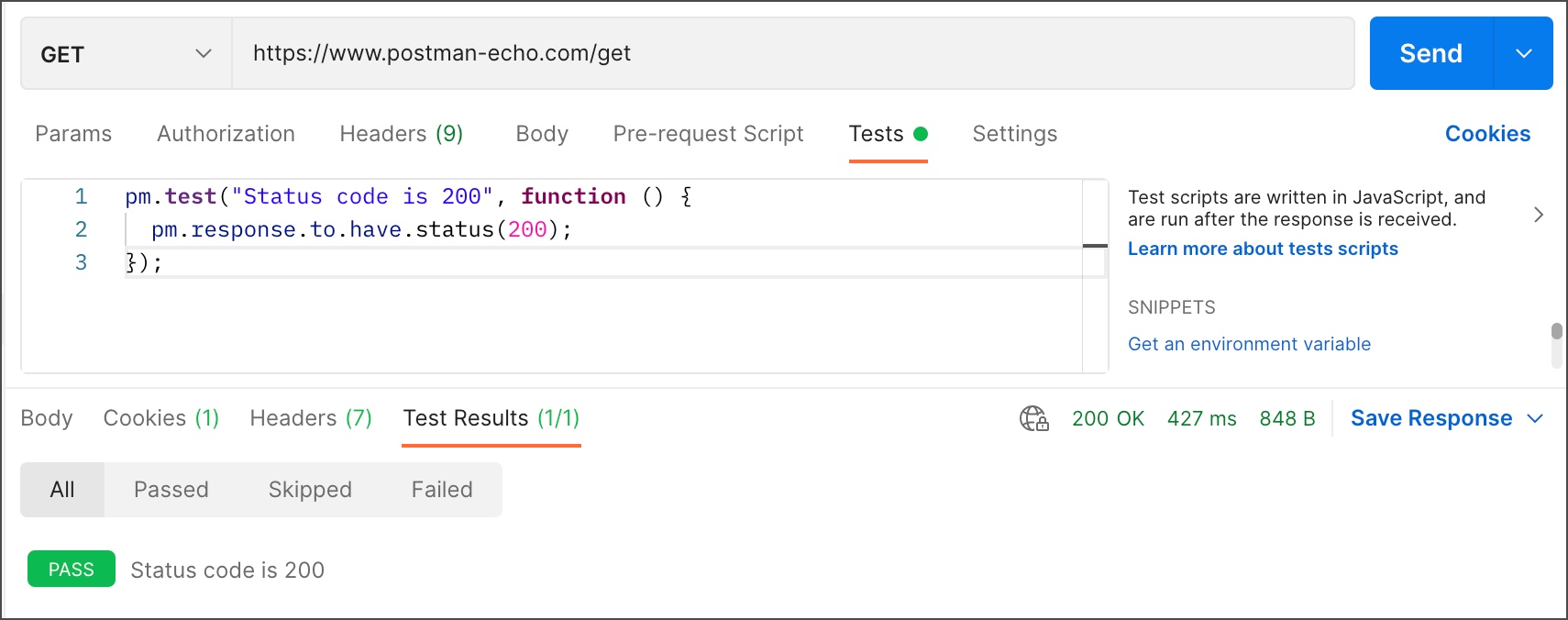
![Power Automate IF Expression [With 51 Examples] - SPGuides Power Automate If Expression [With 51 Examples] - Spguides](https://i0.wp.com/www.spguides.com/wp-content/uploads/2022/05/Checking-if-field-is-empty-or-null-in-MS-Flow-1024x648.png)


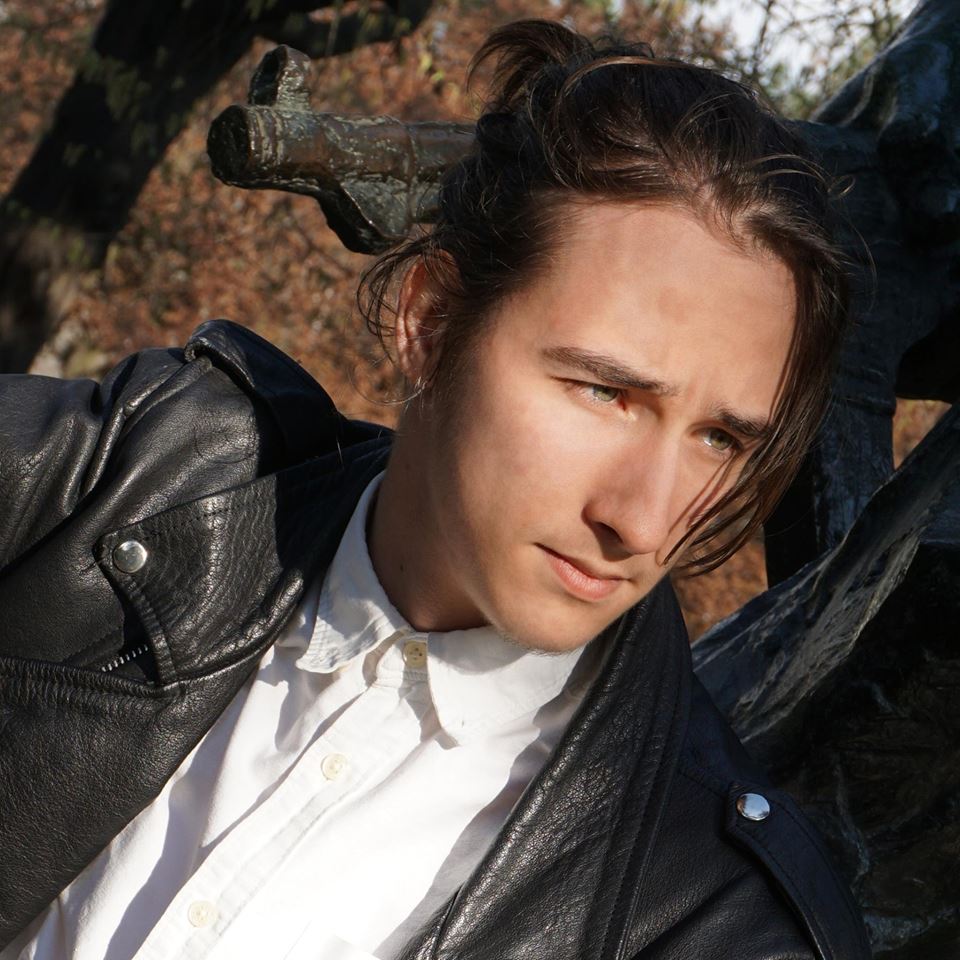
Article link: go check if variable is empty.
Learn more about the topic go check if variable is empty.
- What is the best way to test for an empty string in Go?
- 3 Easy Ways to Check If String is Empty in Golang – AskGolang
- How to check if a string is empty in Golang – Educative.io
- Golang program to check if a string is empty or null
- How to Check for an Empty String in Go – Go language Tutorial
- How to check if a string is empty in Go – Reactgo
- How to check for an empty struct in Go – Freshman.tech
- Checking if structure is empty or not in Golang – GeeksforGeeks
- 3 Easy Ways to Check If String is Empty in Golang – AskGolang
- reflect.IsZero() Function in Golang with Examples – GeeksforGeeks
- Zero values – A Tour of Go
- How to find the type of a variable in Golang – Educative.io
- Solved: How to test if string is empty in GoLang? – GoLinuxCloud
- Golang Check If Time Is Empty Example
See more: nhanvietluanvan.com/luat-hoc