Max Long Value Java
In Java, the long data type is used to represent 64-bit signed integral values. The maximum value that a long variable can hold is represented by the constant Long.MAX_VALUE, which is 9223372036854775807. This article explores various aspects of working with the max long value in Java, including representation, comparison operators, mathematical operations, casting, overflow and underflow issues, handling in loops and conditional statements, functions and methods, common errors and pitfalls, and best practices.
Representation of Max Long Value in Java
The max long value, as represented by Long.MAX_VALUE, is a constant that can be used to assign the maximum possible value to a long variable. It is often used when working with large numbers that cannot be accommodated by other data types such as int or double. The representation of the max long value is standardized in Java, ensuring consistency across different Java platforms and implementations.
Comparison Operators for Long Values in Java
When comparing long values in Java, the standard comparison operators such as ==, !=, <, >, <=, and >= can be used. The same principles apply when comparing the max long value with other long values. It is important to note that when using the equality operator (==) to compare a long value with Long.MAX_VALUE, care should be taken to compare it explicitly as a long value by appending “L” at the end of the constant, e.g., longValue == Long.MAX_VALUE.
Mathematical Operations with Max Long Value in Java
Mathematical operations involving the max long value should be implemented with caution due to the possibility of overflow or underflow. For example, adding 1 to Long.MAX_VALUE would result in a value of Long.MIN_VALUE, which is the minimum possible value for a long variable. It is crucial to consider these limitations and handle them appropriately in the code logic.
Casting Max Long Value to Other Data Types in Java
Casting the max long value to other data types in Java requires careful consideration of the range limitations of the destination data type. For example, casting Long.MAX_VALUE to an int would result in an overflow since the max int value, represented by Integer.MAX_VALUE, is smaller than Long.MAX_VALUE. In such cases, it is recommended to use data types such as BigInteger or BigDecimal to handle larger values without loss of precision.
Overflow and Underflow Issues with Max Long Value in Java
Working with the max long value poses the risk of overflow or underflow issues. Overflow occurs when a mathematical operation results in a value that exceeds the maximum value that can be represented. Underflow, on the other hand, occurs when a mathematical operation results in a value smaller than the minimum value that can be represented. It is important to handle these issues diligently to avoid incorrect results or unexpected behavior in the code.
Handling Max Long Value in Loops and Conditional Statements in Java
When dealing with loops and conditional statements involving the max long value, it is crucial to consider the specific conditions that may lead to overflow, underflow, or incorrect results. Proper range checks, conditionals, and error handling mechanisms should be implemented to ensure the correct behavior of the program.
Functions and Methods involving Max Long Value in Java
Functions and methods in Java that involve the max long value should have the necessary range checks and validation mechanisms to handle potential issues. It is advisable to provide clear documentation and comments within the code to assist other developers in understanding the limitations and special considerations when working with the max long value.
Common Errors and Pitfalls with Max Long Value in Java
Some common errors and pitfalls when working with the max long value in Java include:
1. Forgetting to append “L” at the end of Long.MAX_VALUE when comparing it with long values using the equality operator (==).
2. Neglecting to handle overflow or underflow situations in mathematical operations involving the max long value.
3. Casting the max long value to data types with smaller ranges without considering the potential loss of precision or overflow.
4. Misinterpreting the range limitations of long values and assuming they can hold larger values than they are capable of.
Best Practices for Working with Max Long Value in Java
To work effectively with the max long value in Java, the following best practices should be followed:
1. Always use Long.MAX_VALUE to assign or compare the maximum value for long variables.
2. Avoid using the equality operator (==) to compare long values, especially when comparing with Long.MAX_VALUE, and use explicit long comparison instead.
3. Consider using appropriate data types such as BigInteger or BigDecimal when working with values beyond the range of long.
4. Carefully validate and handle overflow or underflow situations in mathematical operations involving the max long value.
5. Provide clear documentation and comments within the code to guide developers on the limitations and considerations when working with the max long value.
In conclusion, the max long value in Java, represented by Long.MAX_VALUE, is a significant constant when working with large integral values. Understanding its representation, limitations, and best practices is crucial for developing reliable and accurate Java programs. By following the recommended practices and being aware of potential pitfalls, developers can effectively utilize the max long value in their code while avoiding common errors and ensuring the correctness of their applications.
FAQs:
Q1. What is the max value of an integer in Java?
In Java, the maximum value of an integer is represented by the constant Integer.MAX_VALUE, which is 2147483647.
Q2. What is the max value of a double in Java?
The maximum value of a double in Java is represented by the constant Double.MAX_VALUE, which is approximately 1.7976931348623157 x 10^308.
Q3. What is BigDecimal in Java?
BigDecimal is a Java class that provides arbitrary-precision decimal arithmetic. It can handle numbers with a larger range and precision compared to primitive data types like double or float.
Q4. What is BigInteger in Java?
BigInteger is a Java class that provides arbitrary-precision integer arithmetic. It can handle integers with no upper or lower bounds, making it suitable for working with very large or small values.
Q5. How to handle overflow and underflow with the max long value in Java?
To handle overflow and underflow with the max long value in Java, you should validate the range before performing mathematical operations. Additionally, consider using data types like BigDecimal or BigInteger to handle larger values without loss of precision.
Q6. Can the max long value be directly compared using the equality operator (==)?
When comparing the max long value with other long values using the equality operator (==), it is crucial to append “L” at the end of Long.MAX_VALUE to ensure explicit comparison as long values, e.g., longValue == Long.MAX_VALUE.
Q7. What are some common errors when working with the max long value in Java?
Common errors when working with the max long value in Java include forgetting to append “L” when comparing it using the equality operator (==), neglecting overflow or underflow situations in mathematical operations, improper casting to smaller data types, and misinterpreting the range limitations of long values.
Q8. What are the best practices for working with the max long value in Java?
Some best practices for working with the max long value in Java include always using Long.MAX_VALUE to assign or compare the maximum value, avoiding direct comparison using the equality operator (==), considering appropriate data types for larger values, handling overflow and underflow situations, and providing clear documentation and comments in the code.
How Do You Find The Max Value In An Array In Java | Find Min And Max Value Array In Java
What Is The Max Length Of Int In Java?
The Java programming language is widely used in software development due to its simplicity, flexibility, and robustness. One of the fundamental data types in Java is the “int,” which represents integer values. As with any data type, it is essential to understand its limitations and restrictions to ensure accurate and efficient programming. In this article, we will explore the maximum length of the “int” data type in Java, along with some frequently asked questions on the topic.
In Java, the “int” data type is a 32-bit signed two’s complement integer. This means that it can hold both positive and negative values within the range of -2^31 to 2^31 – 1. More precisely, the maximum value an “int” variable can hold is 2,147,483,647, while the minimum value is -2,147,483,648. These limits are determined by the number of bits allocated to store the value of an “int” in memory.
It is important to note that the maximum and minimum values of an “int” can be accessed through the constants “Integer.MAX_VALUE” and “Integer.MIN_VALUE” provided by the Java standard library. These constants can be useful when comparing or setting boundaries for “int” variables in your programs.
When attempting to assign a value to an “int” variable that exceeds its maximum or minimum limit, the program will encounter an integer overflow. An integer overflow occurs when the result of an arithmetic operation exceeds the range defined by the data type, leading to an unexpected behavior or incorrect calculation.
To prevent integer overflow, it is essential to validate user input and apply appropriate error handling mechanisms when necessary. Additionally, using larger data types with wider ranges, such as “long,” can accommodate larger integer values. The “long” data type in Java is a 64-bit signed two’s complement integer, which stores values within the range of -2^63 to 2^63 – 1.
FAQs:
Q: Can an “int” variable hold decimal values?
A: No, the “int” data type can only store integer values. If you need to work with decimal values, you should use the “float” or “double” data types.
Q: Why is the range of “int” limited?
A: The range of “int” is limited by the number of bits used to store its value in memory. By using 32 bits, Java ensures efficiency in memory usage while still providing a wide range suitable for most applications.
Q: Can I assign a value greater than “Integer.MAX_VALUE” to an “int” variable?
A: No, attempting to assign a value greater than “Integer.MAX_VALUE” will result in an integer overflow. To store larger values, you can use the “long” data type instead.
Q: Can I assign a value lower than “Integer.MIN_VALUE” to an “int” variable?
A: Similarly, attempting to assign a value lower than “Integer.MIN_VALUE” will also result in an integer overflow. It is important to ensure that assigned values fall within the valid range of the “int” data type.
Q: What happens if I perform arithmetic operations beyond the “int” range?
A: When arithmetic operations produce a result beyond the range of “int,” an integer overflow occurs, leading to unexpected behavior or incorrect calculations. It is crucial to handle such situations appropriately in your program.
Q: Are there any alternative data types with larger ranges than “int”?
A: Yes, Java provides various data types with wider ranges, such as “long,” which can store values beyond the range of “int.” Additionally, for precise decimal calculations, the “BigDecimal” class can be used.
In conclusion, the “int” data type in Java represents 32-bit signed two’s complement integers, offering a range from -2^31 to 2^31 – 1. Understanding the limits of this data type is crucial to prevent integer overflow and ensure accurate calculations in your programs. By utilizing appropriate error handling mechanisms and considering larger data types when required, you can achieve efficient and reliable Java programming.
What Is The Max Value For Java?
In the world of Java programming, understanding the concept of maximum values is crucial, as it allows developers to work with data types effectively. Java, being a strongly typed language, requires explicit declaration and initialization of variables, making it vital to know the maximum values that can be assigned to them. In this article, we will explore the max value for Java and delve into the intricacies surrounding it.
Data Types and Maximum Values in Java:
Java supports a wide range of data types, each with its own maximum and minimum values. Let’s discuss some of the commonly used data types and their maximum values:
1. Integer Data Types:
– The data type ‘byte’ represents an 8-bit signed integer and has a maximum value of 127.
– The data type ‘short’ represents a 16-bit signed integer and has a maximum value of 32,767.
– The data type ‘int’ represents a 32-bit signed integer and has a maximum value of 2,147,483,647.
– The data type ‘long’ represents a 64-bit signed integer and has a maximum value of 9,223,372,036,854,775,807.
2. Floating-Point Data Types:
– The data type ‘float’ represents a single-precision 32-bit floating-point number. It has a maximum value of approximately 3.40282347E38 and can support up to 6-7 decimal digits of precision.
– The data type ‘double’ represents a double-precision 64-bit floating-point number and has a maximum value of approximately 1.7976931348623157E308. It can support up to 15 decimal digits of precision.
3. Character Data Type:
– The data type ‘char’ represents a single Unicode character. While it is primarily used to store alphanumeric characters, it can also store special symbols and has a maximum value of ‘\uFFFF’.
4. Boolean Data Type:
– The data type ‘boolean’ represents a boolean value, which can be either ‘true’ or ‘false’. It does not have a specific maximum value but rather operates on only two values.
Limitations and Overflow:
It is important to note that exceeding the maximum value of a data type leads to overflow issues. For instance, if a variable of type ‘int’ exceeds the maximum value, it would wrap around to the minimum value of that data type. This phenomenon is known as overflow.
For example, consider an ‘int’ variable with a maximum value of 2,147,483,647. If we increment this value by 1, it would wrap around and become the minimum value of -2,147,483,648. This behavior is a result of the way integers are stored in binary form using a fixed number of bits.
Frequently Asked Questions (FAQs):
Q: Can I assign a value greater than the max value of a data type?
A: Yes, you can assign a value greater than the max value of a data type, but it will result in overflow, which may produce unexpected results.
Q: Are there any data types with unlimited maximum values?
A: No, all data types in Java have specific maximum values or ranges. There is no data type that can represent unlimited values.
Q: What happens if I exceed the maximum value of a floating-point data type?
A: If you assign a value greater than the maximum value of a floating-point data type, it will be represented as “Infinity” or ‘-Infinity’. This is known as floating-point overflow.
Q: How can I handle potential overflow issues in my Java code?
A: To handle potential overflow issues, you can perform range checks before assigning values. Conditional statements or specialized methods can be used to ensure that the assigned value falls within the valid range.
Q: Is there a way to avoid overflow issues altogether?
A: Java provides a mechanism known as “BigInteger” and “BigDecimal” classes, which can handle arbitrarily large integers and decimal numbers, respectively. Using these classes, you can bypass the limitations of fixed-size data types.
Conclusion:
In Java, the maximum values of data types play a crucial role in programming, allowing developers to understand the limitations of their variables and prevent overflow issues. By adhering to these maximum values and utilizing appropriate techniques, one can ensure the accurate representation and manipulation of data. Being aware of the maximum values for Java’s data types allows programmers to write robust and reliable code.
Keywords searched by users: max long value java Integer max value Java, Double max value Java, BigDecimal max value, BigInteger max value, Double value in Java, Integer max_value trong Java, Int64 max value, BigInteger java docs
Categories: Top 75 Max Long Value Java
See more here: nhanvietluanvan.com
Integer Max Value Java
In Java, the Integer class is a wrapper class that provides methods and functionalities for working with integer values. One of the useful aspects of the Integer class is its ability to represent the maximum value that an integer can hold. In this article, we will delve into the concept of the Integer max value in Java, its significance, and how it can be utilized effectively in your Java programs.
Understanding the Integer Data Type
Before we delve into the Integer max value, it is important to have a clear understanding of the integer data type in Java. An integer is a whole number that can be either positive or negative, including zero. In Java, the int data type is the most commonly used data type for representing integers. However, the Integer class provides a wrapper around the int data type to use it as an object.
The Integer class provides various methods and constants to manipulate and work with integer values. One of these constants is the Integer.MAX_VALUE, which represents the maximum value that an integer can hold in Java. The Integer.MAX_VALUE is defined as 2^31 – 1, which is equal to 2,147,483,647.
Significance of the Integer.MAX_VALUE
The concept of the Integer.MAX_VALUE holds great significance in Java programming. It serves as a guideline and limit for programmers when dealing with large numbers or when they want to check if an integer value exceeds the maximum limit.
One common application of the Integer.MAX_VALUE is in input validation. For example, if a program receives user input for a variable that should be within a certain range, such as a person’s age, comparing the input with Integer.MAX_VALUE allows us to check if it is within the valid range without needing to hardcode the maximum value.
Moreover, the Integer.MAX_VALUE is crucial in scenarios where arithmetic operations might lead to overflow. Overflow occurs when a mathematical operation exceeds the maximum value that can be represented by an integer, resulting in unpredictable behavior. By checking an arithmetic operation against Integer.MAX_VALUE, we can prevent overflow and handle the situation gracefully.
Utilizing the Integer.MAX_VALUE
The Integer.MAX_VALUE can be utilized in various ways in your Java programs. Here are a few examples:
1. Raising Errors: In situations where the input is required to be within a particular range, you can compare the user input with Integer.MAX_VALUE. If the input exceeds this value, you can raise an error or display an appropriate message to the user, indicating that their input is invalid.
2. Checking Overflow: When performing arithmetic operations on integers, verifying if the result exceeds the Integer.MAX_VALUE helps in avoiding potential overflow. By comparing the result with Integer.MAX_VALUE before assigning it to a variable, you can detect when overflow occurs and handle it accordingly.
3. Setting Maximum Values: In cases where you need to initialize a variable with its maximum possible value, you can assign it to Integer.MAX_VALUE. This is particularly useful when you need a placeholder value that can later be replaced with an actual value during program execution.
FAQs about Integer Max Value in Java
Q1. Can an integer value exceed the Integer.MAX_VALUE?
No, an integer value in Java cannot exceed the Integer.MAX_VALUE. If an arithmetic operation or a user input results in a value larger than Integer.MAX_VALUE, it will overflow, resulting in unpredictable behavior or incorrect results.
Q2. Is the Integer.MAX_VALUE the same for all platforms and Java versions?
Yes, the value of Integer.MAX_VALUE remains the same across all Java platforms and versions since it is a predefined constant in the Integer class. It represents the maximum value that can be stored in an int data type.
Q3. Is there a minimum value for integers in Java?
Yes, just like the Integer.MAX_VALUE represents the maximum value, the Integer.MIN_VALUE represents the minimum value that an integer can hold in Java. The Integer.MIN_VALUE is defined as -2^31, which is equal to -2,147,483,648.
Q4. Can we use the Integer.MAX_VALUE to create an array of integers?
Yes, you can use Integer.MAX_VALUE as the size of an array since it represents a valid integer value. However, it is important to note that creating such a large array might have significant memory implications, so it should be done with caution.
Q5. How can we represent an integer value larger than Integer.MAX_VALUE?
For representing integer values larger than Integer.MAX_VALUE, you can make use of other data types like Long, BigInteger, or BigDecimal, which can handle larger numbers. These data types provide the flexibility to work with arbitrarily large integers.
In conclusion, understanding the Integer max value in Java is essential for Java developers as it helps in defining limits, preventing overflow, and handling large numbers effectively. By utilizing the Integer.MAX_VALUE constant in your Java programs, you can ensure that your code remains robust, error-free, and efficient.
Double Max Value Java
In the world of programming, Java is widely recognized as one of the most popular and powerful programming languages. It offers a wide range of features and functionalities that allow developers to create robust and efficient applications. One such feature is the double data type, which allows for the representation of floating-point numbers in Java. In this article, we will explore the concept of the double max value in Java, its significance, and how it can be utilized effectively in programming.
Understanding the Double Data Type in Java
Java provides several data types to store and manipulate different types of values. The double data type is one of them, primarily used to store decimal numbers or floating-point values. Unlike integers, which can only store whole numbers, doubles can store fractional values with great precision.
The double data type in Java is represented by a 64-bit IEEE 754 floating-point number. This means that it can have a maximum range and precision. The range of the double data type can be divided into positive and negative values, with zero in between. In most cases, the positive range is identical to the negative range, resulting in a symmetric range centered around zero.
Understanding Double Max Value in Java
The double data type in Java has a predefined maximum value, known as Double.MAX_VALUE. This variable represents the largest finite positive value that can be stored in a double. It is a constant value defined within the Double class and can be accessed by any part of a Java program.
The Double.MAX_VALUE is approximately 1.7976931348623157 * 10^308. It is an extremely large number and represents the upper limit of the double data type’s range. Anything above this value is considered infinity or non-finite, as it surpasses the maximum limit of representable finite values. It is important to note that the negative counterpart of Double.MAX_VALUE is -Double.MAX_VALUE.
Significance of Double Max Value
The Double.MAX_VALUE plays a crucial role in various mathematical calculations and algorithms. It allows developers to work with extremely large or small numbers without losing precision. For instance, when dealing with financial calculations, scientific calculations, or any other scenario requiring high precision, the double data type with its max value comes in handy.
Moreover, the Double.MAX_VALUE constant can be used to check if a calculation or a result has exceeded the limit of a double data type. Since the double data type cannot represent numbers larger than Double.MAX_VALUE, it can be used as a threshold for error detection or to handle exceptional scenarios in the program.
FAQs:
Q: Can I assign a value greater than Double.MAX_VALUE to a double variable?
A: No, attempting to assign a value greater than Double.MAX_VALUE to a double variable will result in an overflow or infinity. The double data type has a limited range, and Double.MAX_VALUE signifies its upper limit.
Q: How does the Double.MAX_VALUE differ from Float.MAX_VALUE?
A: The Float.MAX_VALUE is the maximum value of the float data type in Java, while the Double.MAX_VALUE represents the maximum value of the double data type. The double data type provides higher precision and a larger range than the float data type.
Q: Can I perform mathematical operations with Double.MAX_VALUE?
A: Yes, you can perform mathematical operations, such as addition, subtraction, multiplication, and division, with Double.MAX_VALUE. However, when the result exceeds Double.MAX_VALUE, the value becomes non-finite, as it exceeds the representable range of the double data type.
Q: Are there any specific considerations while comparing values with Double.MAX_VALUE?
A: Comparing values with Double.MAX_VALUE should be done with caution. Due to the limited precision of floating-point values, an exact comparison may not yield accurate results. It is recommended to use an epsilon value or utilize the BigDecimal class for precise comparisons.
In conclusion, understanding the concept of Double.MAX_VALUE in Java is crucial for developers working with floating-point numbers and requiring high precision calculations. It provides a means to handle extremely large or small values within the range of the double data type. By utilizing Double.MAX_VALUE effectively, programmers can ensure accurate results and smooth functioning of their Java applications.
Bigdecimal Max Value
Introduction:
In the world of programming and computer science, numbers play a fundamental role. Whether it’s a simple addition or complex mathematical computations, the precision and accuracy of numbers are imperative. However, with limited storage capacity and computational resources, there are limitations to representing and manipulating numbers. One such limitation is encountered with the BigDecimal max value, which determines the upper bound for the range of numbers that can be represented using the BigDecimal data type.
Understanding BigDecimal:
BigDecimal is a Java class that offers a high level of precision and accuracy for representing and performing arithmetic operations on decimal numbers. Unlike other numeric types like int or float, BigDecimal does not suffer from inherent rounding and precision errors. It can handle large numbers with a large number of decimal places, making it suitable for financial applications and any scenario where precise calculations are required.
The BigDecimal class supports a variety of methods for performing arithmetic operations, such as addition, subtraction, multiplication, division, and exponentiation. It also provides rounding, comparison, and formatting capabilities. However, it is worth noting that the computational cost of using BigDecimal is generally higher than using other numeric types, due to the increased precision and extended range it offers.
Understanding the concept of max value:
Every data type in Java has an upper limit, known as the maximum value, beyond which it cannot represent any higher number. BigDecimal is no exception to this rule. As a high precision numeric data type, the maximum value of BigDecimal is determined by the underlying hardware resources and limitations of the programming language.
The max value of a BigDecimal is represented as a constant in the class BigDecimal called MAX_VALUE. This constant determines the largest number that can be represented using a BigDecimal object. It is important to be aware of this limit to avoid situations where computations or assignments exceed the range of BigDecimal.
BigDecimal MAX_VALUE limitation:
The BigDecimal.MAX_VALUE constant represents the largest finite positive value that can be represented using the BigDecimal class. The value of BigDecimal.MAX_VALUE is equivalent to 10^2147483647 – 1, which is a gigantic number and far exceeds the capacity of most practical computing scenarios.
BigDecimal.MAX_VALUE is constrained by the available resources and is determined by the architecture of the computer and the language implementation. The value is primarily limited by the amount of memory allocated for storing the number and the maximum range allowed by the language specification. Therefore, different programming languages or platforms may have different maximum values for BigDecimal.
FAQs:
Q1. What happens if I exceed the maximum value of BigDecimal?
If you attempt to assign or compute a number greater than the maximum value of BigDecimal, you will encounter an arithmetic overflow exception. This exception indicates that the result of the operation exceeds the limit that can be represented using the BigDecimal class. It’s essential to handle such exceptions gracefully in your application to prevent unexpected errors or crashes.
Q2. Can I modify the maximum value of BigDecimal?
No, the maximum value of BigDecimal is determined by the language specification and the underlying hardware resources. It cannot be modified or extended beyond the predefined limit set by the programming language. However, you can use alternative approaches, such as breaking down large numbers into smaller segments or using scientific notation, to handle calculations involving extremely large values.
Q3. Are there any alternatives to BigDecimal for handling large numbers?
Yes, if you need to work with extremely large numbers that exceed the maximum value of BigDecimal, you can consider using libraries or frameworks specifically designed for arbitrary precision arithmetic, such as BigInteger. BigInteger offers an unlimited range of integers, but doesn’t support decimal places. There are also specialized libraries available for handling specific use cases, such as financial calculations or cryptography.
Q4. Is there a minimum value for BigDecimal as well?
Yes, similar to the maximum value, BigDecimal also has a minimum value represented by the constant MIN_VALUE. The value of BigDecimal.MIN_VALUE is equivalent to -10^2147483647, indicating the smallest finite positive value that can be represented using BigDecimal.
Conclusion:
BigDecimal max value serves as a crucial guide to understanding the limitations of representing large decimal numbers in programming. Being aware of these limitations is essential to prevent unexpected errors or overflows in applications requiring high precision arithmetic. While BigDecimal.MAX_VALUE places a generous limit on the range of numbers that can be handled, alternative approaches or specialized libraries can be explored for exceptional cases. Understanding the constraints and utilizing appropriate techniques help developers work with precision and accuracy in their programming endeavors.
Images related to the topic max long value java
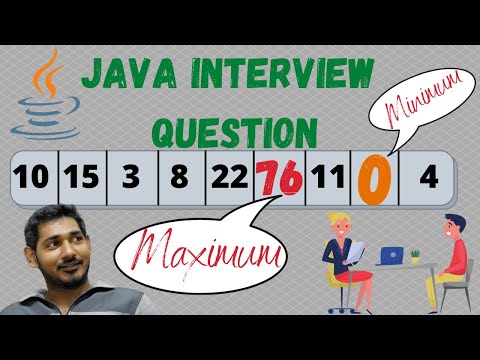
Found 7 images related to max long value java theme
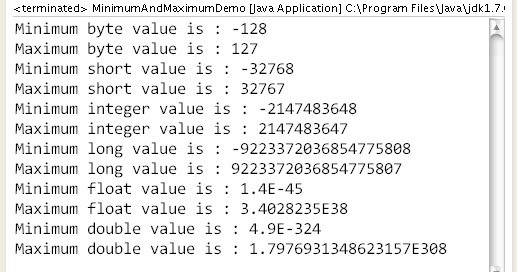
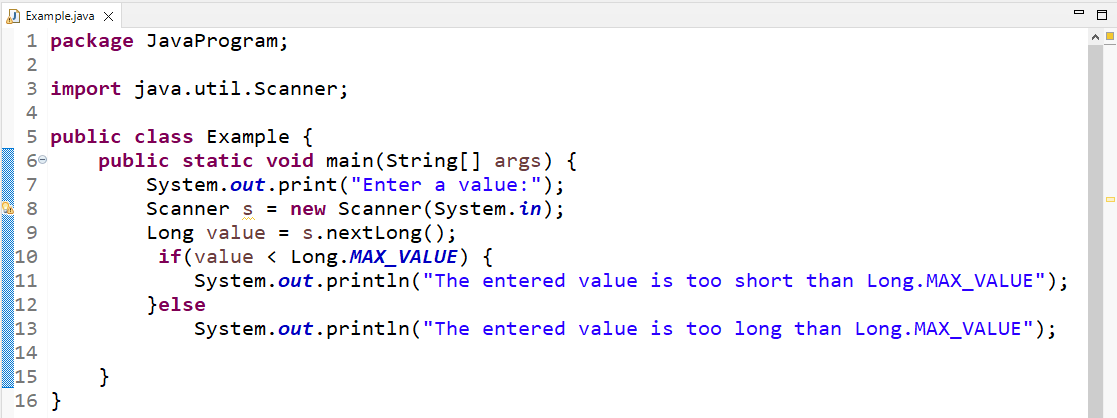

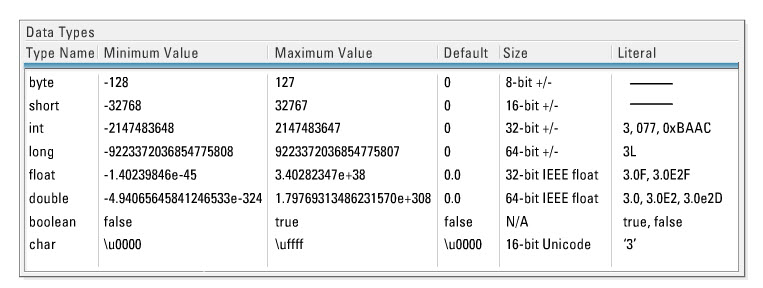
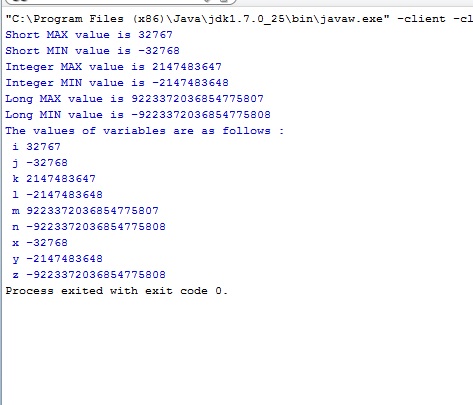
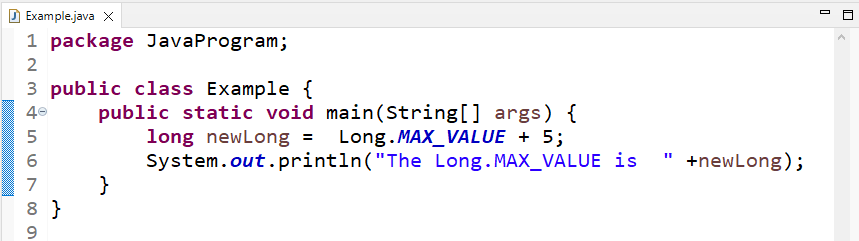
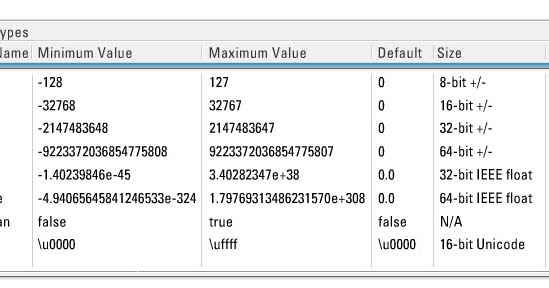
![SOLVED: Java- Array Question Why is the max value 3.5? Explain like you're explaining to a 5 yr old Test.java X 1 public class Test 20 public static void main(string[] args) 3 Solved: Java- Array Question Why Is The Max Value 3.5? Explain Like You'Re Explaining To A 5 Yr Old Test.Java X 1 Public Class Test 20 Public Static Void Main(String[] Args) 3](https://cdn.numerade.com/ask_images/85c9807cb6134504aef2e9f1293150e1.jpg)

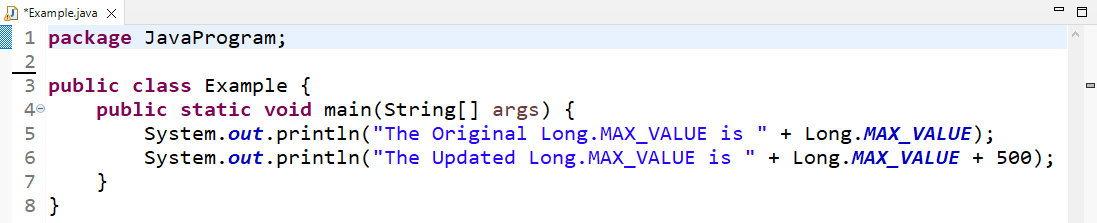
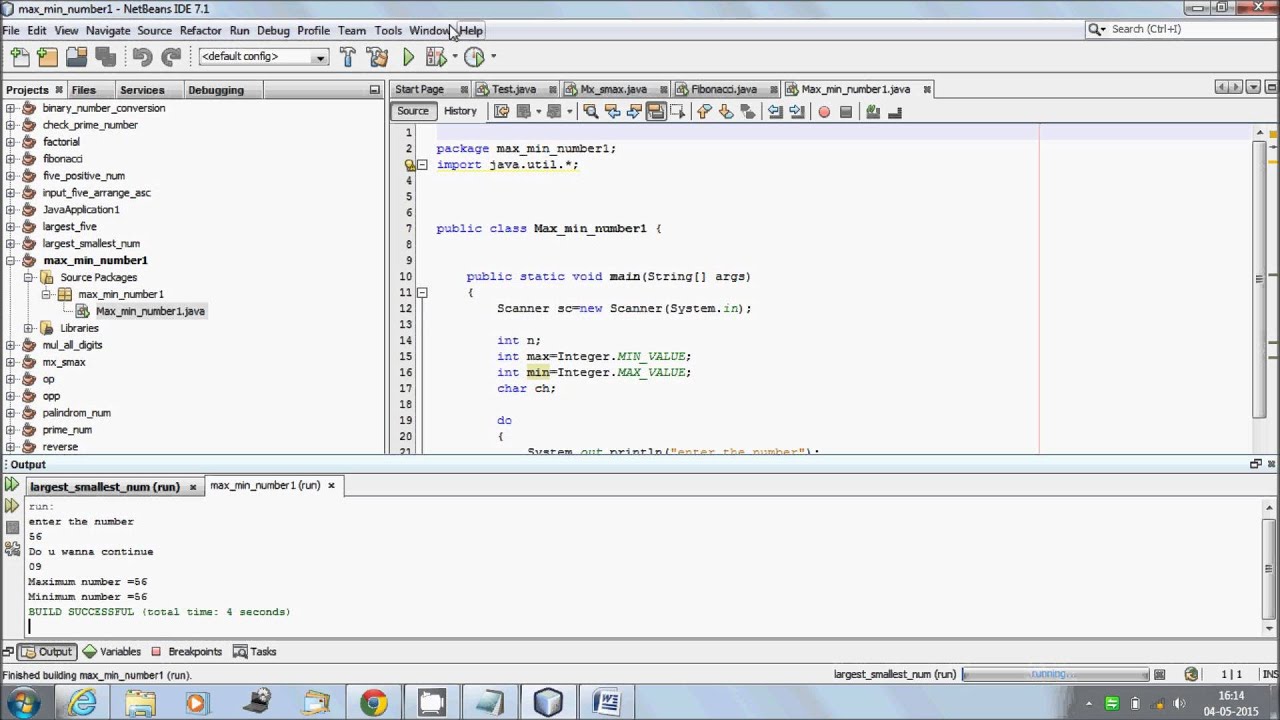
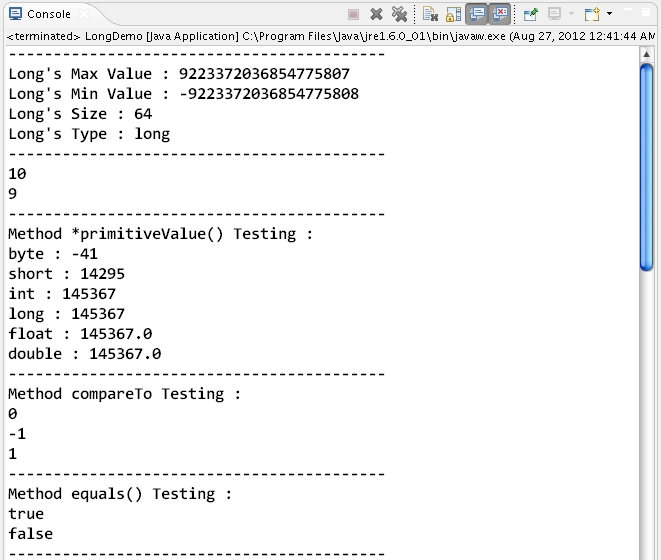
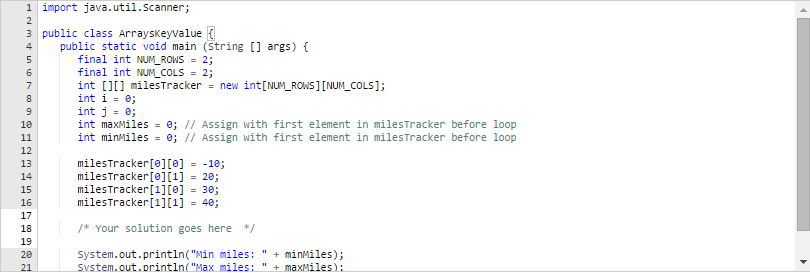

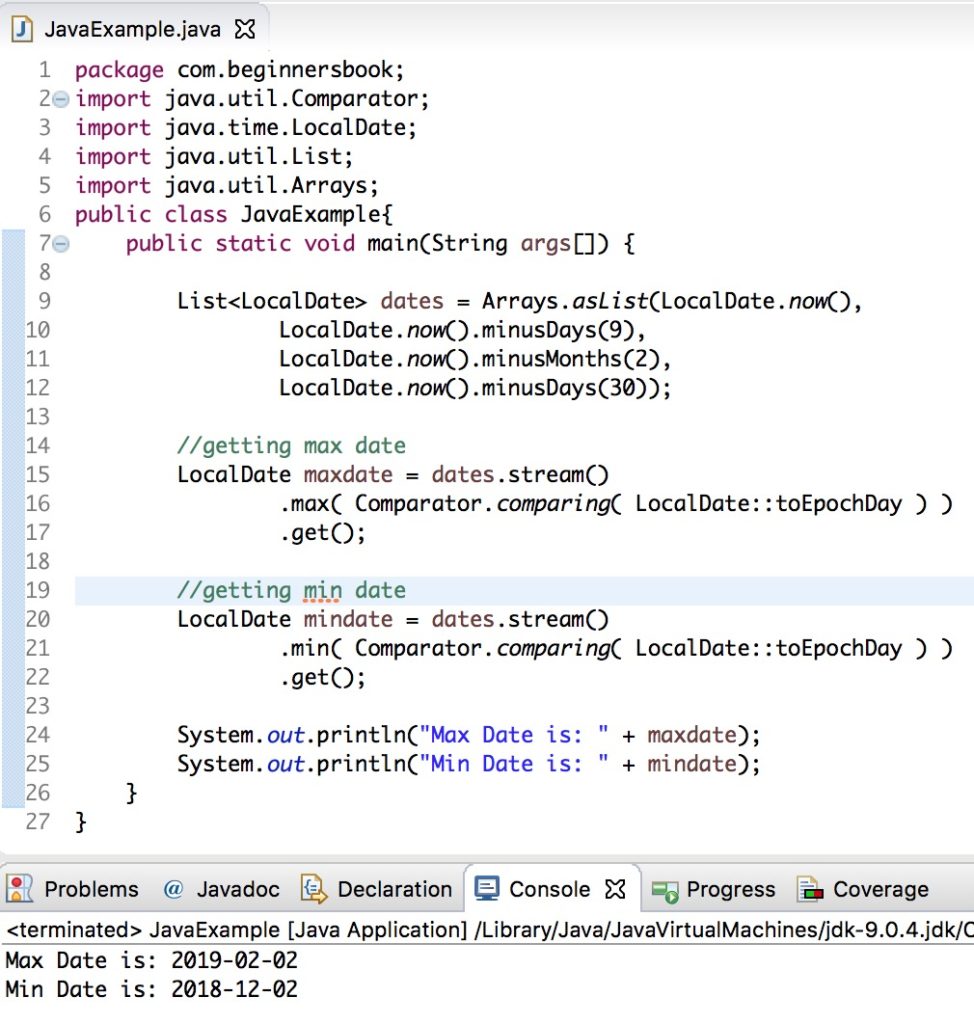

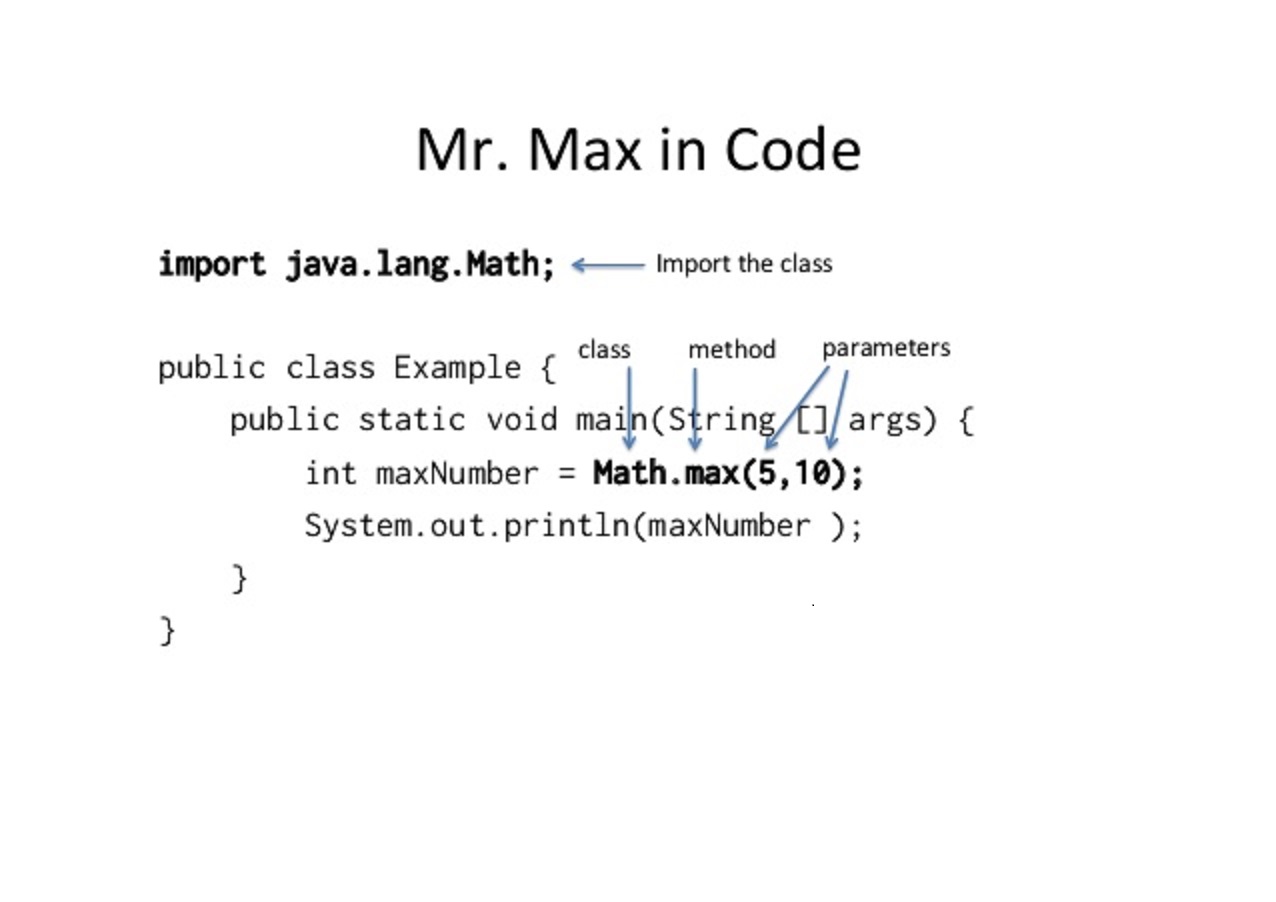
![Long.MAX_VALUE & Long.MIN_VALUE in Java [Practical Examples] | GoLinuxCloud Long.Max_Value & Long.Min_Value In Java [Practical Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/java_long_mx_min.jpg)
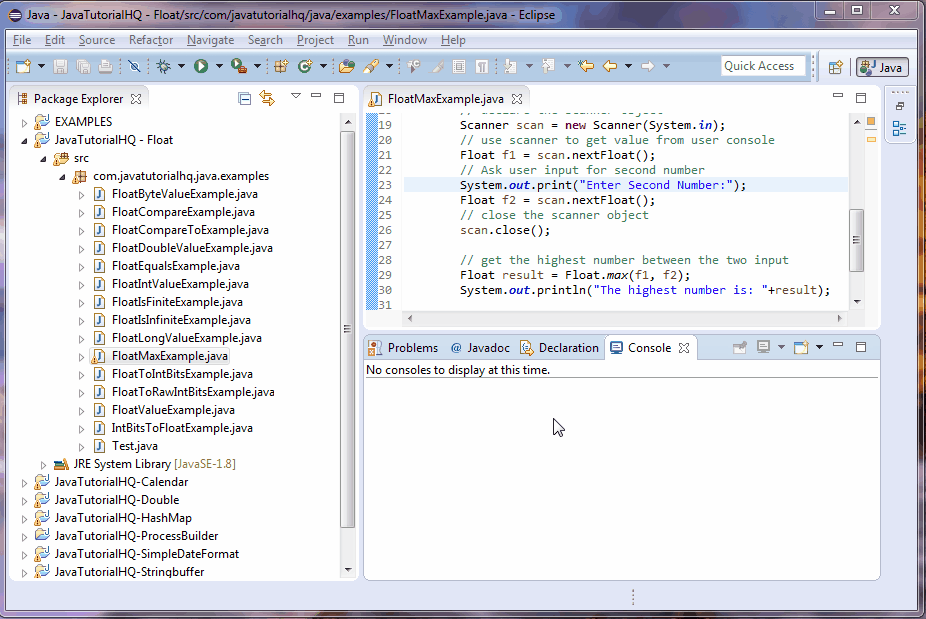
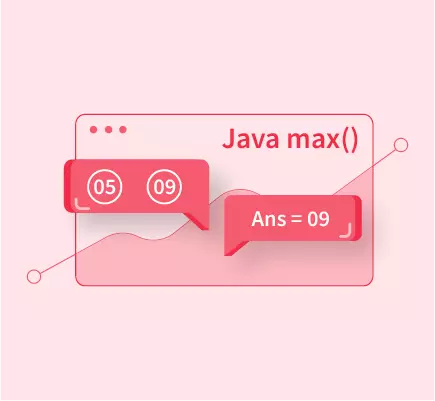
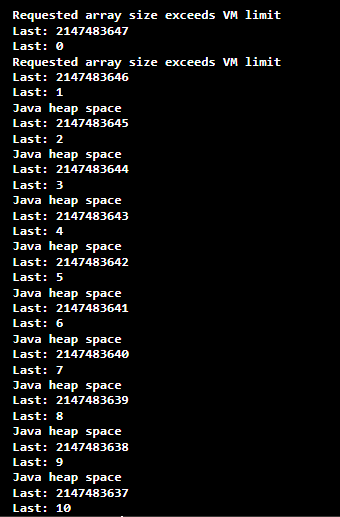
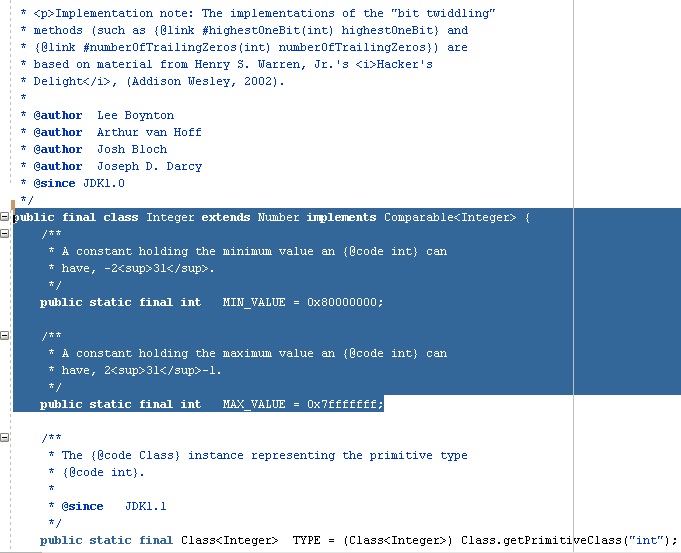
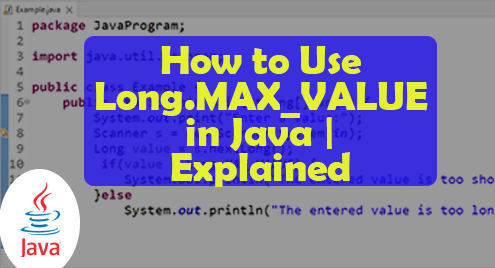
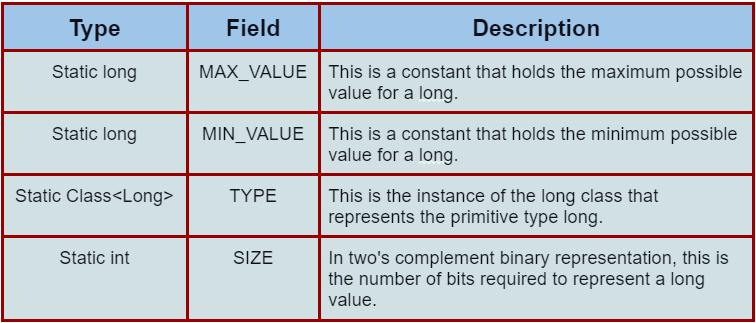
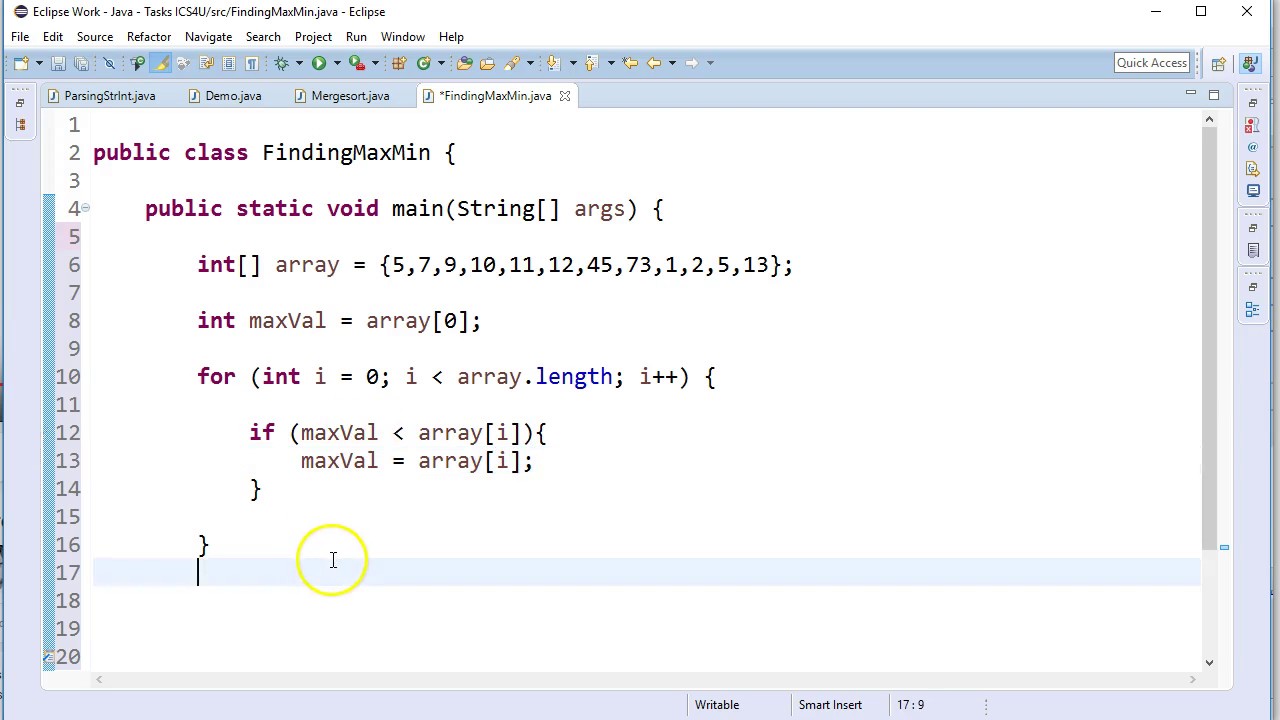

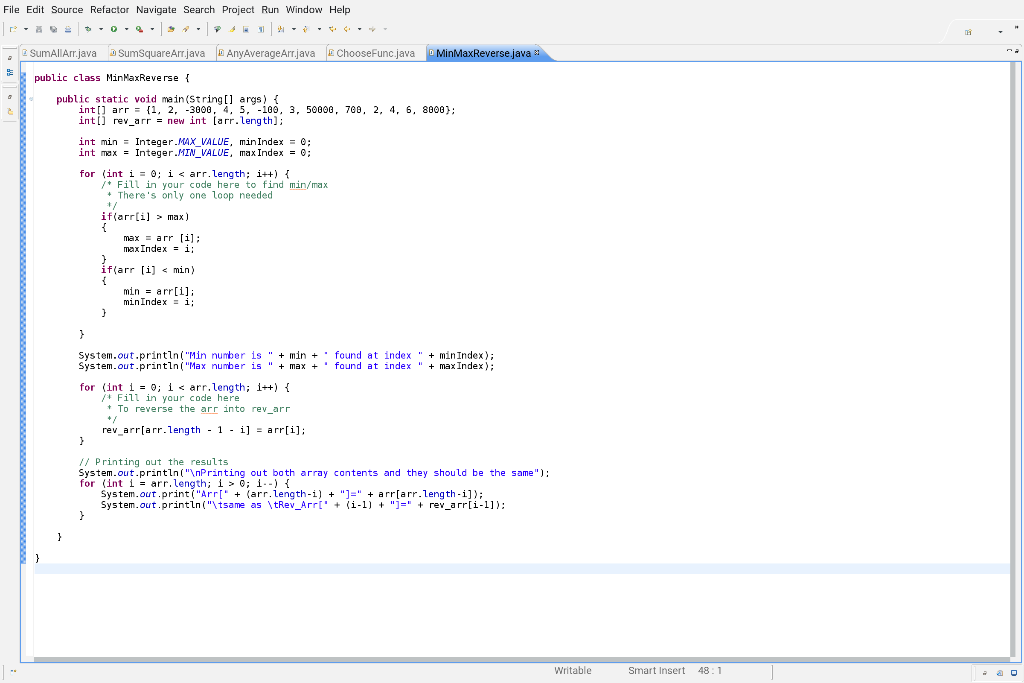

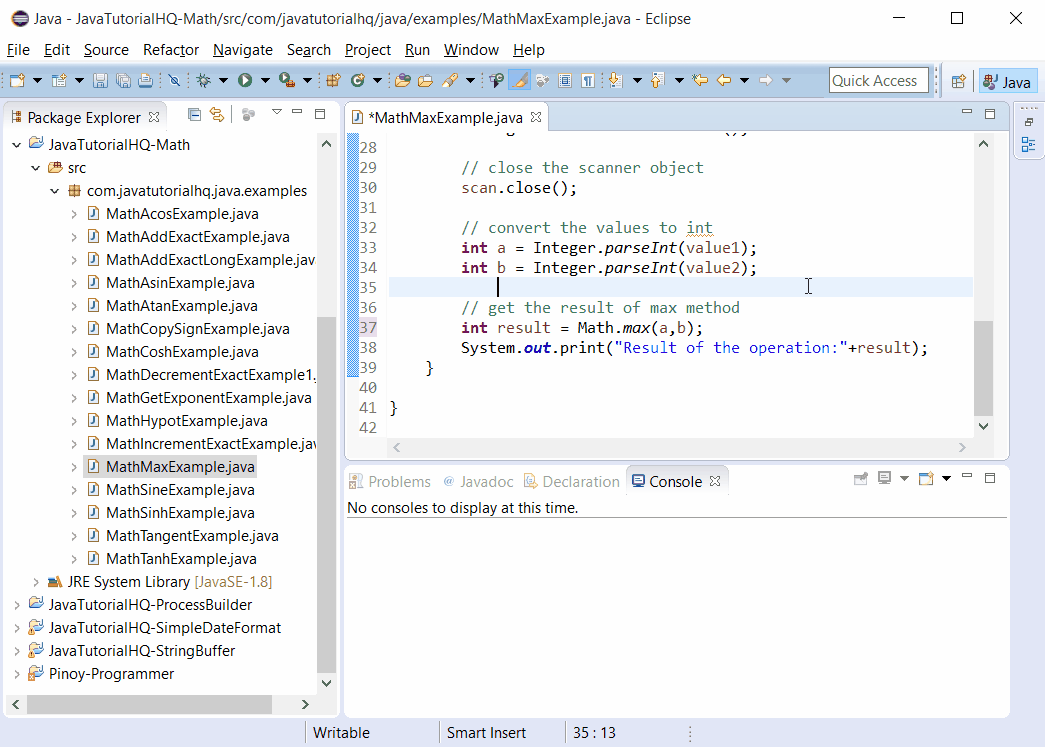


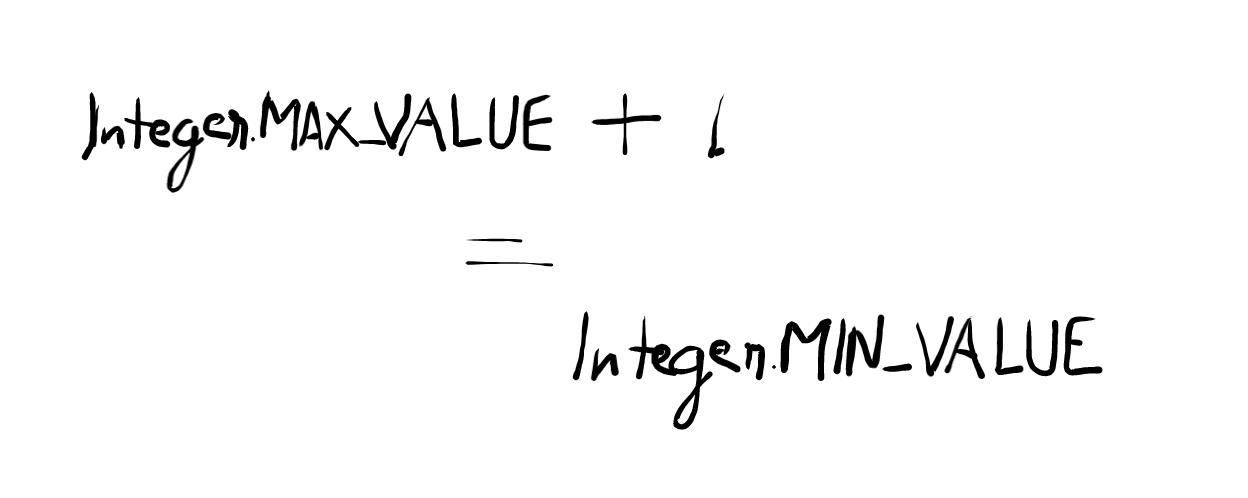

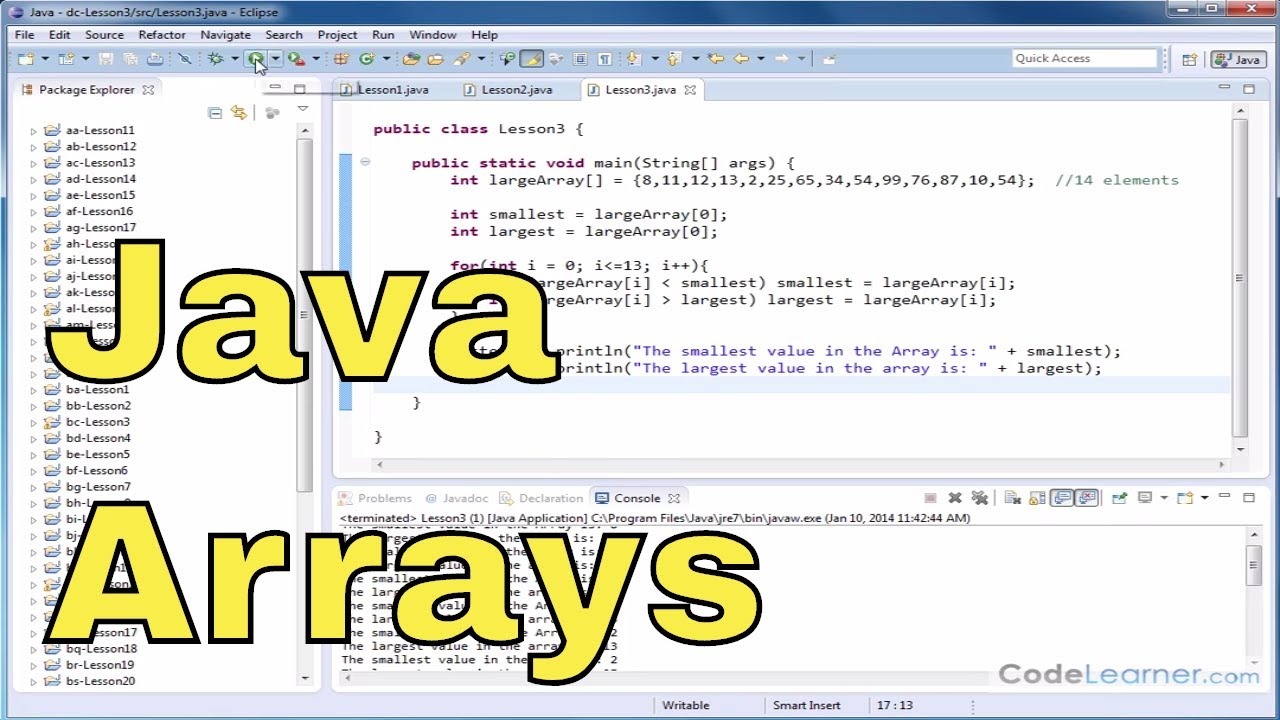
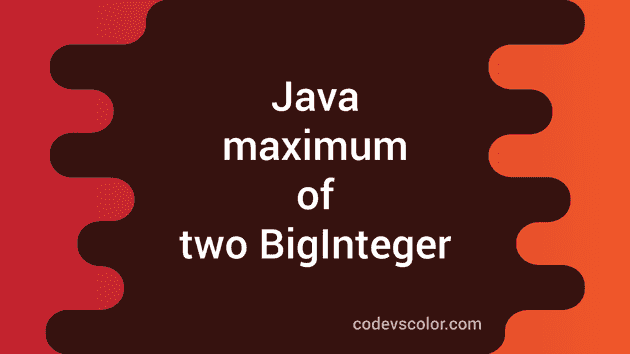
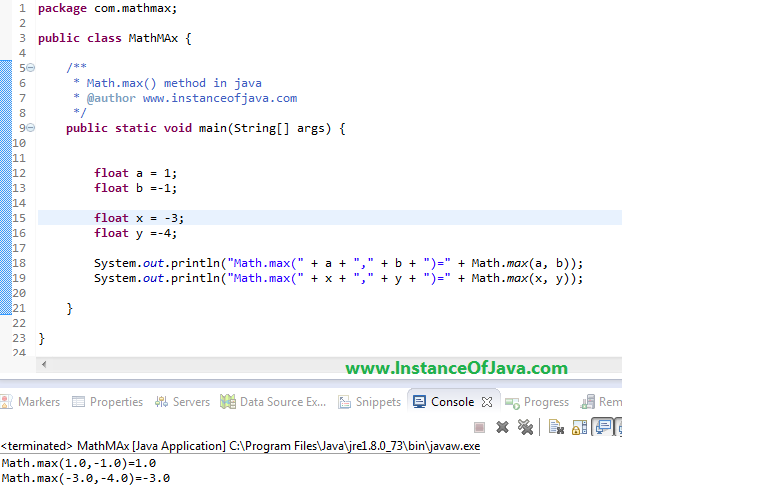



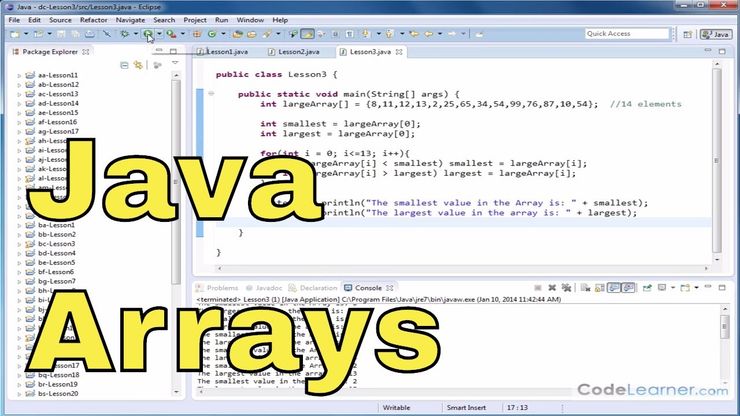
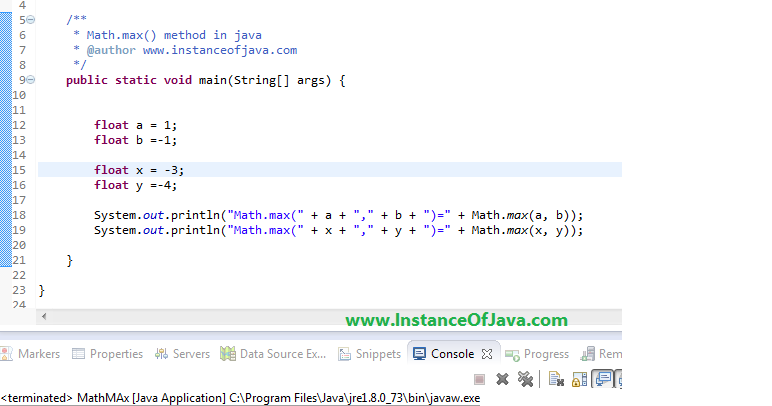



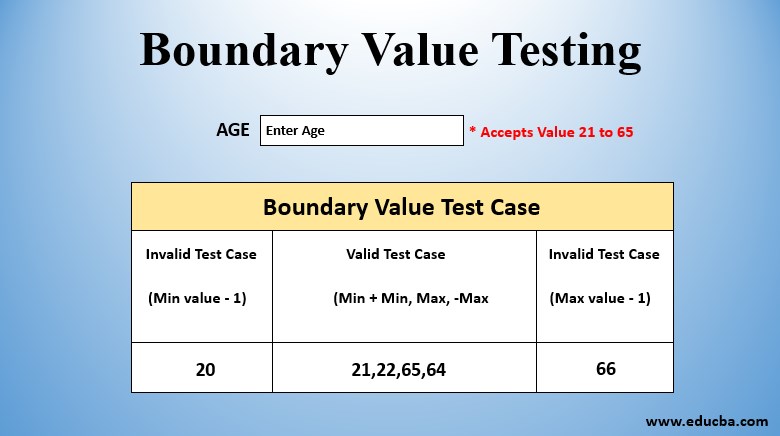
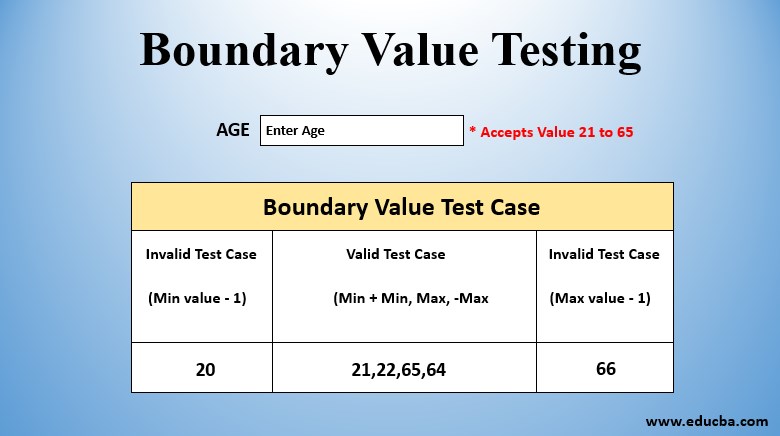
Article link: max long value java.
Learn more about the topic max long value java.
- How to Use Long.MAX_VALUE in Java | Explained – Linux Hint
- Long.MAX_VALUE in Java | Delft Stack
- A long bigger than Long.MAX_VALUE – Stack Overflow
- 3.7. Integer Min and Max — AP CSA Java Review – Obsolete
- Integer.MAX_VALUE in Java with Examples – CodeGym
- Java Long.MAX_VALUE and Long.MIN_VALUE – GoLinuxCloud
- Primitive Data Types – Java Tutorials – Oracle Help Center
- Integer.MAX_VALUE in Java with Examples – CodeGym
- 3.7. Integer Min and Max — AP CSA Java Review – Obsolete
- Java.lang.Math.max() Method – Tutorialspoint
- Java – get long max value – Dirask
- max value of integer – W3docs
See more: https://nhanvietluanvan.com/luat-hoc