Mkdir If Not Exists
Introduction: Why the “mkdir if not exists” Command is Useful
In the world of shell scripting and command line operations, it is often necessary to create directories dynamically as part of a script or program. However, sometimes you may encounter a situation where you need to create a directory only if it does not already exist. This is where the “mkdir if not exists” command becomes extremely useful.
Checking for the Existence of a Directory
Before creating a directory, it is essential to check if it already exists. There are several ways to perform this check, each with its own advantages and limitations.
1. Ways to Check if a Directory Exists
1.1. Using the “test” Command
The “test” command, also known as the “[ ]” command, is a powerful tool for performing various checks in shell scripts. To check the existence of a directory, you can use the “-d” flag with the “test” command. For example:
“`bash
if [ -d /path/to/directory ]; then
echo “Directory exists!”
else
echo “Directory does not exist!”
fi
“`
1.2. Using the “stat” Command
The “stat” command provides detailed information about files and directories. By using the “-c %F” option, followed by the path to the directory, you can check if it is a directory. If it returns “directory,” it means the directory exists. Here’s an example:
“`bash
if [ “$(stat -c %F /path/to/directory)” == “directory” ]; then
echo “Directory exists!”
else
echo “Directory does not exist!”
fi
“`
1.3. Using the “find” Command
The “find” command can be utilized to search for files and directories based on various criteria. By specifying the appropriate search parameters, such as the name of the directory, you can determine if it exists. Here’s an example:
“`bash
if find /path -type d -name “directory” >/dev/null 2>&1; then
echo “Directory exists!”
else
echo “Directory does not exist!”
fi
“`
1.4. Using the “ls” Command
The “ls” command is typically used for listing files and directories. By redirecting the output to “null” and using the “-d” flag, you can suppress any output and determine if the directory exists. Here’s an example:
“`bash
if ls -d /path/to/directory >/dev/null 2>&1; then
echo “Directory exists!”
else
echo “Directory does not exist!”
fi
“`
1.5. Using the “if [ -d ]” Command
Another way to check if a directory exists is by using the “if [ -d ]” command. This command allows you to directly check if a directory exists without any additional conditions. For example:
“`bash
if [ -d /path/to/directory ]; then
echo “Directory exists!”
else
echo “Directory does not exist!”
fi
“`
Creating a Directory If It Does Not Exist
2. The “mkdir” Command and Its Functionality
The “mkdir” command is used to create directories in shell scripting. By combining it with the appropriate conditional statements, you can create a directory only if it does not already exist.
2.1. Syntax of the “mkdir” Command
The basic syntax for creating a directory using the “mkdir” command is as follows:
“`bash
mkdir [OPTIONS] DIRECTORY
“`
Where “OPTIONS” can be various flags and options that enhance the functionality of the “mkdir” command, and “DIRECTORY” is the path or name of the directory to be created.
2.2. Handling Errors and Warnings
When using the “mkdir” command, it is essential to handle any errors or warnings that may occur. For example, if a directory with the same name already exists, the “mkdir” command might display an error. To handle such cases, you can use the “-p” option.
“`bash
mkdir -p /path/to/directory
“`
The “-p” option ensures that if the directory already exists, the command will continue without displaying an error.
2.3. Options and Flags to Enhance Functionality
The “mkdir” command offers various options and flags that can enhance its functionality. Some commonly used options are:
– “-m”: Specifies the permissions (mode) to set for the created directory.
– “-v”: Displays a message for each directory created.
– “-p”: Creates parent directories if they do not already exist.
By utilizing these options, you can customize the behavior of the “mkdir” command as per your requirements.
Using Conditional Statements in Shell Scripts
3. Introduction to Conditional Statements
Conditional statements are a crucial aspect of shell scripting, allowing you to make decisions based on specific conditions. In the case of creating a directory if it does not exist, conditional statements play a vital role in checking the existence of the directory and executing the “mkdir” command accordingly.
3.1. The Basics of Conditionals in Shell Scripts
In shell scripting, conditional statements are typically written using the “if” keyword, followed by the condition in square brackets “[ ]” and concluded with the “then” keyword. Here’s a basic syntax of a conditional statement:
“`bash
if [ condition ]; then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
“`
The condition can be any valid command or expression that evaluates to either true or false.
3.2. The “if-else” Statement
The “if-else” statement expands the functionality of the basic conditional statement by allowing you to execute different blocks of code based on the condition’s result. Here’s an example:
“`bash
if [ condition ]; then
# code to be executed if the condition is true
else
# code to be executed if the condition is false
fi
“`
3.3. The “if-elif-else” Statement
The “if-elif-else” statement further extends the capabilities of conditional statements by allowing multiple conditions to be checked sequentially. If none of the conditions evaluates to true, the “else” block is executed. Here’s an example:
“`bash
if [ condition1 ]; then
# code to be executed if condition1 is true
elif [ condition2 ]; then
# code to be executed if condition2 is true
else
# code to be executed if neither condition1 nor condition2 is true
fi
“`
3.4. Nesting Conditional Statements
Conditional statements can also be nested within one another to create more complex logic. By nesting conditional statements, you can check for multiple conditions simultaneously and execute the corresponding code blocks accordingly. Here’s an example:
“`bash
if [ condition1 ]; then
if [ condition2 ]; then
# code to be executed if both condition1 and condition2 are true
else
# code to be executed if condition1 is true and condition2 is false
fi
else
# code to be executed if condition1 is false
fi
“`
Implementing “mkdir if not exists” in Shell Scripts
4. Writing a Shell Script to Automate the Process
Now that we understand how to check for the existence of a directory and create one if needed, let’s dive into implementing the “mkdir if not exists” functionality in a shell script.
4.1. Combining the “if” Statement with “mkdir”
To create a directory if it does not exist, you can combine the “if” statement with the “mkdir” command. Here’s an example:
“`bash
if [ ! -d /path/to/directory ]; then
mkdir /path/to/directory
echo “Directory created!”
else
echo “Directory already exists!”
fi
“`
In this script, we first check if the directory does not exist using the “![ -d ]” conditional statement. If the condition is true, we execute the “mkdir” command to create the directory. Otherwise, we display a message stating that the directory already exists.
4.2. Checking for the Existence of a Directory
To make the script more dynamic and flexible, you can use user prompts or variables to specify the directory path. Here’s an example:
“`bash
read -p “Enter the path to the directory: ” directory_path
if [ ! -d “$directory_path” ]; then
mkdir “$directory_path”
echo “Directory created!”
else
echo “Directory already exists!”
fi
“`
In this modified script, the user is prompted to enter the path to the directory. The value is stored in the variable “directory_path,” which is then used in the conditional statement and the “mkdir” command.
4.3. Utilizing the Conditional Operator
The conditional operator “&&” allows you to execute a command or code block only if the previous command was successful (exited with a status of 0). By combining the “if” statement with the “mkdir” command using the conditional operator, you can create a directory in a single line. Here’s an example:
“`bash
[ ! -d /path/to/directory ] && mkdir /path/to/directory && echo “Directory created!”
“`
In this example, the “mkdir” command is executed only if the directory does not exist, and the subsequent echo statement is only executed if the “mkdir” command succeeds.
Examples of “mkdir if not exists” in Practice
5. Example 1: Creating a Directory For Logs
Consider a scenario where you want to create a directory to store log files. If the directory already exists, the script should proceed without creating a new directory. Here’s an example script:
“`bash
log_directory=”/path/to/logs”
if [ ! -d “$log_directory” ]; then
mkdir “$log_directory”
echo “Log directory created!”
else
echo “Log directory already exists!”
fi
“`
5.1. Checking If the Directory Exists
Before creating the log directory, the script checks if it already exists using the “![ -d ]” conditional statement.
5.2. Creating the Directory If It Does Not Exist
If the directory does not exist, the script creates it using the “mkdir” command. After successful execution, a message is displayed informing the user that the log directory has been created.
6. Example 2: Organizing Files by Date
In some cases, you might want to organize files into subdirectories based on the current date. If the date folder does not already exist, it should be created before moving or organizing the files. Here’s an example script:
“`bash
current_date=$(date +%Y-%m-%d)
date_folder=”/path/to/files/$current_date”
if [ ! -d “$date_folder” ]; then
mkdir “$date_folder”
echo “Date folder created!”
else
echo “Date folder already exists!”
fi
“`
6.1. Checking If the Date Folder Exists
To check if the date folder exists, the script constructs the path using the current date obtained from the “date” command. It then checks for the existence of the folder using the “![ -d ]” conditional statement.
6.2. Creating the Date Folder If It Does Not Exist
If the date folder does not exist, the script creates it using the “mkdir” command. A corresponding message is displayed to inform the user.
7. Example 3: Creating Multiple Directories
In some scenarios, you may need to create multiple directories simultaneously and check if they already exist. If any of the directories do not exist, they should be created. Here’s an example script:
“`bash
directories=(“/path/to/directory1” “/path/to/directory2” “/path/to/directory3”)
for directory in “${directories[@]}”; do
if [ ! -d “$directory” ]; then
mkdir “$directory”
echo “Directory created: $directory”
else
echo “Directory already exists: $directory”
fi
done
“`
7.1. Checking If All Directories Exist
The script uses a “for” loop to iterate through an array of directories. For each directory, it checks if it already exists using the “![ -d ]” conditional statement.
7.2. Creating the Missing Directories If They Do Not Exist
If a directory does not exist, the script creates it using the “mkdir” command. A corresponding message is displayed for each directory, indicating whether it was created or already existed.
Conclusion: Harnessing the Power of “mkdir if not exists” in Shell Scripts
The “mkdir if not exists” command, when combined with conditional statements and the “mkdir” command, provides a powerful tool for automating the creation of directories in shell scripts. By checking for the existence of a directory before attempting to create it, you can ensure that your scripts function smoothly without encountering errors or overwriting existing directories.
In this article, we explored various methods to check if a directory exists, discussed the syntax and options of the “mkdir” command, and delved into implementing the “mkdir if not exists” functionality in shell scripts. We also provided examples to demonstrate its practical usage in scenarios such as creating log directories, organizing files by date, and creating multiple directories at once.
By utilizing the concepts and techniques presented in this article, you can streamline your shell scripting workflows, automate directory creation tasks, and enhance the efficiency of your scripts. So go ahead and start harnessing the power of “mkdir if not exists” in your shell scripts!
How To Mkdir Only If A Directory Does Not Already Exist?
Keywords searched by users: mkdir if not exists Create folder java if not exists, Touch file if not exist, Os mkdir exist ok, Mkdir -p option, Create folder if it doesn t exist Python, Rm if exists, Bash check folder exist, Mkdir bash script
Categories: Top 78 Mkdir If Not Exists
See more here: nhanvietluanvan.com
Create Folder Java If Not Exists
Java, being one of the most popular programming languages, offers a vast array of features and functionalities. In this article, we will dive deep into the concept of creating a folder in Java, and how we can handle situations where the folder may already exist. We will explore various methods and provide explanations to empower you in carrying out this task effectively. Additionally, we will conclude the article with a frequently asked questions (FAQs) section to address commonly encountered issues related to this topic.
**Creating a Folder in Java**
In Java, creating a folder or directory is made possible through the `File` class, which provides numerous methods and functionalities to interact with files and directories. To create a folder, we need to follow these steps:
1. Importing the required classes:
“`java
import java.io.File;
“`
2. Defining the path for the folder:
“`java
String folderPath = “C:\\myFolder”;
“`
Note that the path can be adjusted to suit your specific needs.
3. Creating the directory using the `mkdir()` method:
“`java
File folder = new File(folderPath);
boolean folderCreated = folder.mkdir();
“`
Here, the folder is created by calling the `mkdir()` method on the `File` object. This method returns a boolean value that signifies whether the folder was successfully created or not. It returns `true` if the folder was created, and `false` otherwise.
4. Checking if the folder was created:
“`java
if (folderCreated) {
// Folder created successfully
} else {
// Folder creation failed”
}
“`
By executing the code inside the appropriate block, you can handle the outcome of the folder creation accordingly.
**Handling Existing Folders**
Occasionally, you may encounter scenarios where the folder you are attempting to create already exists. In such cases, Java provides different methods to handle this situation effectively.
1. Using the `mkdir()` method:
“`java
File folder = new File(folderPath);
boolean folderCreated = folder.mkdir();
if (folderCreated) {
// Folder created successfully
} else {
// Folder already exists
}
“`
Here, even if the folder already exists, the `mkdir()` method will return `false` and the corresponding block will be executed, notifying you that the folder already exists.
2. Using the `mkdirs()` method:
“`java
File folder = new File(folderPath);
boolean folderCreated = folder.mkdirs();
if (folderCreated) {
// Folder created successfully
} else {
// Folder already exists or creation failed
}
“`
The `mkdirs()` method, in addition to creating the specified folder, will also create all necessary parent directories if they do not already exist. If the folder already exists, the method will not throw an exception and will simply return `false`.
In both cases, you can choose to implement additional logic, such as renaming the existing folder or performing a specific action based on the existence of the folder.
**FAQs**
Q1. Can I create multiple folders at once?
Yes, you can create multiple folders simultaneously by providing a path that includes multiple folder names separated by the appropriate delimiter. For example:
“`java
String multiFolder = “C:\\parentFolder\\childFolder”;
File folder = new File(multiFolder);
boolean folderCreated = folder.mkdirs();
“`
Q2. Can I specify a location other than the local file system?
Absolutely! Java allows you to create folders not only on the local file system but also on network drives and even remote locations. You simply need to provide the correct path or URL to the desired location.
Q3. How can I check if a folder exists before creating it?
Before creating a folder, you can use the `exists()` method of the `File` class to check if the folder already exists. It returns `true` if the folder exists, and `false` otherwise. Here’s an example:
“`java
File folder = new File(folderPath);
boolean folderExists = folder.exists();
if (folderExists) {
// Folder already exists
} else {
// Create the folder
}
“`
Q4. Do I need special permissions to create a folder?
Creating a folder does not typically require special permissions, assuming you have the necessary access rights to write to the target directory. However, when operating within restricted environments, such as certain server configurations or shared networks, you may need elevated privileges or specific permissions to create or write to folders.
Q5. What happens if I create a folder with an invalid name or path?
If you create a folder with an invalid name or path, an exception of type `SecurityException` or `InvalidPathException` may be thrown, respectively. It is always recommended to handle such exceptions to ensure graceful error handling and prevent unexpected application crashes.
In conclusion, creating folders in Java is a straightforward process facilitated by the `File` class. By using the appropriate methods, such as `mkdir()` or `mkdirs()`, we can effortlessly create folders and efficiently handle situations where the folders may already exist. By becoming familiar with these concepts and addressing common FAQs, you can confidently work with file operations in Java and enhance your programming skills.
Touch File If Not Exist
Introduction (78 words):
In the world of computer programming, the ability to create or modify files is an essential skill for developers. Among the plethora of commands available, “touch” holds a unique place due to its capability to create files if they don’t already exist. In this article, we will delve into the touch command, exploring its features, potential use cases, and address common queries in a comprehensive FAQ section.
Understanding the Touch Command (191 words):
The touch command, commonly used in Unix-like operating systems, serves the dual purpose of updating the timestamp on an existing file and creating a file that doesn’t already exist. Designed to handle both use cases, touch simplifies file management by providing an efficient and concise solution.
When executed with a filename as an argument, touch checks if the file exists. If the file is not found, touch instantly creates a new empty file with the given name. In case the file already exists, it updates the file’s timestamp to the current time. This feature makes touch a valuable tool for checking and modifying timestamps during file management tasks.
Use Cases and Applications (233 words):
The touch command finds applications in various scenarios, particularly within the software development domain. Some notable use cases and applications include:
1. File creation during automation: In automation scripts or batch processes, touch ensures that specific files exist before further operations are performed, maintaining a stable workflow.
2. Timestamp modifications: Touch enables developers to modify file timestamps for debugging, updating modified dates, or resetting access times, among other purposes.
3. Dependency tracking: Developers often use touch to mark dependencies, triggering an update when any dependent file’s timestamp changes. This ensures efficient compilation, code generation, or building processes.
Frequently Asked Questions (FAQs):
Q1. Can touch be used with multiple filenames? (87 words)
Yes, touch can accept multiple filenames as arguments. For example, executing the command `touch file1.txt file2.txt file3.txt` creates three new files simultaneously.
Q2. Can touch modify a file’s content as well? (94 words)
No, touch does not directly modify file content. Its primary purpose is to create empty files or update timestamps. Modifying file content requires other commands or text editors available on the respective operating system.
Q3. Can touch be used to create nested directories? (104 words)
The touch command alone cannot create directories; it can only create files. For creating nested directories, developers should employ utilities like mkdir (make directory) to ensure appropriate file hierarchy.
Q4. Can touch be used for remote files as well? (88 words)
Yes, touch can be used for remote files as long as you have the necessary permissions. By connecting to a remote server using SSH or a similar protocol, you can execute touch commands on files hosted on remote machines.
Conclusion (82 words):
The touch command proves to be an invaluable tool for file management, allowing programmers to create files on the fly and update timestamps effortlessly. By understanding its functionalities and exploring various use cases, developers can harness the power of touch command to enhance their productivity and streamline file operations. With its simplicity and versatility, touch continues to remain a vital component in every programmer’s toolkit.
Images related to the topic mkdir if not exists
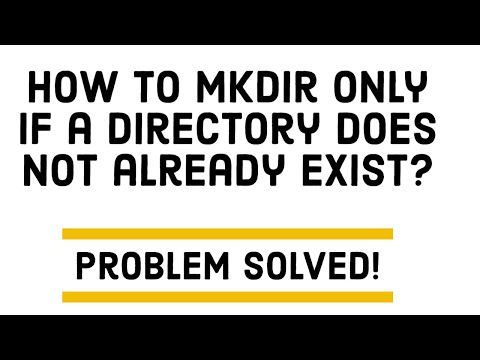
Found 28 images related to mkdir if not exists theme
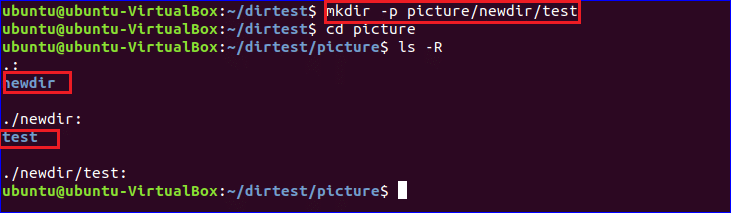
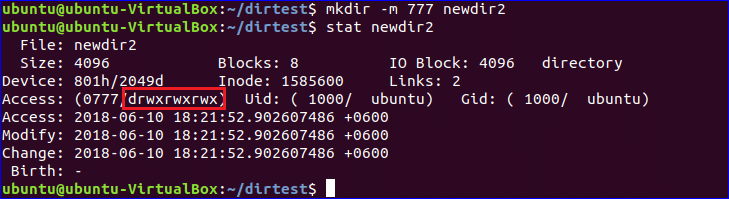
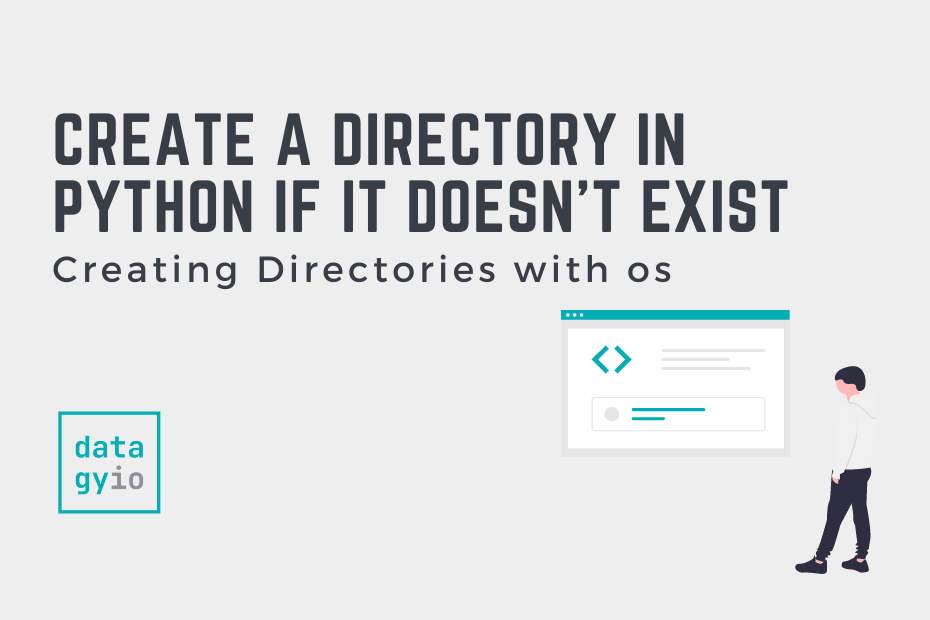
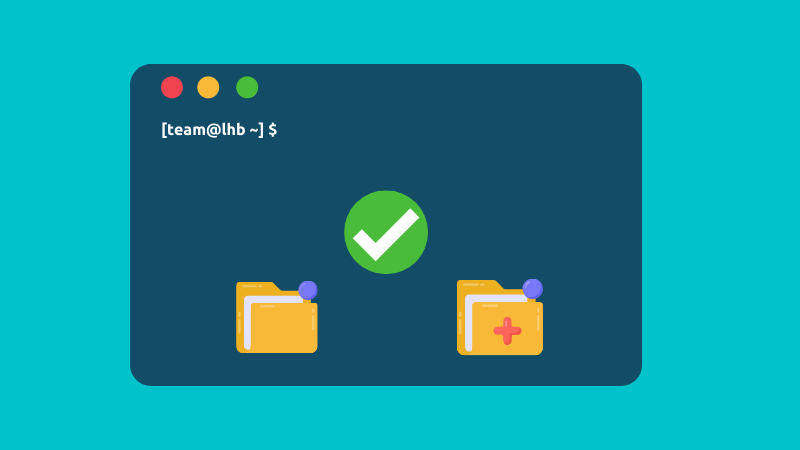
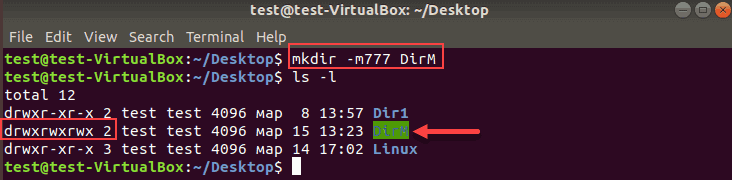
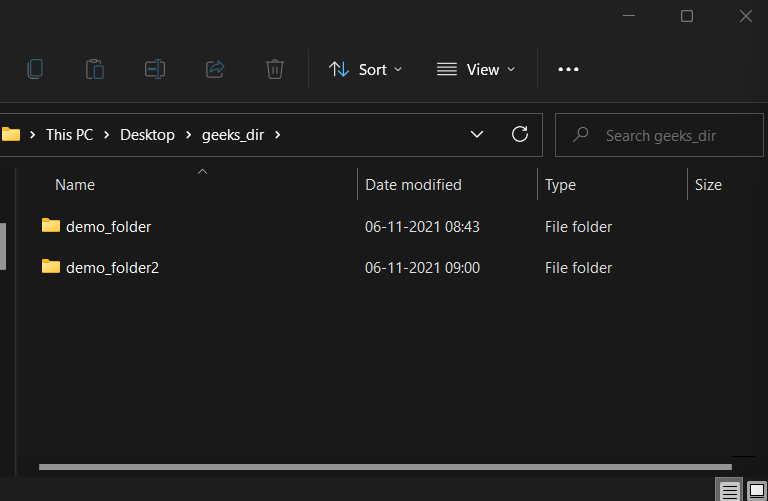
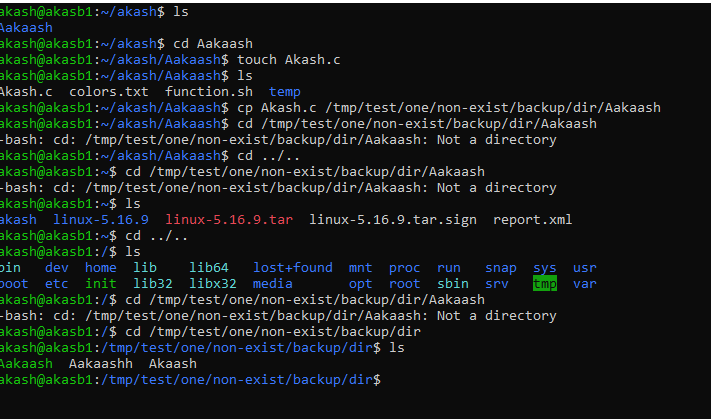
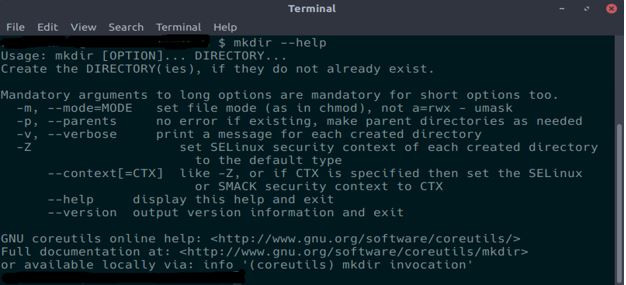

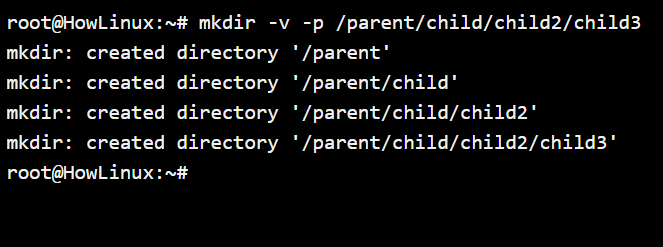
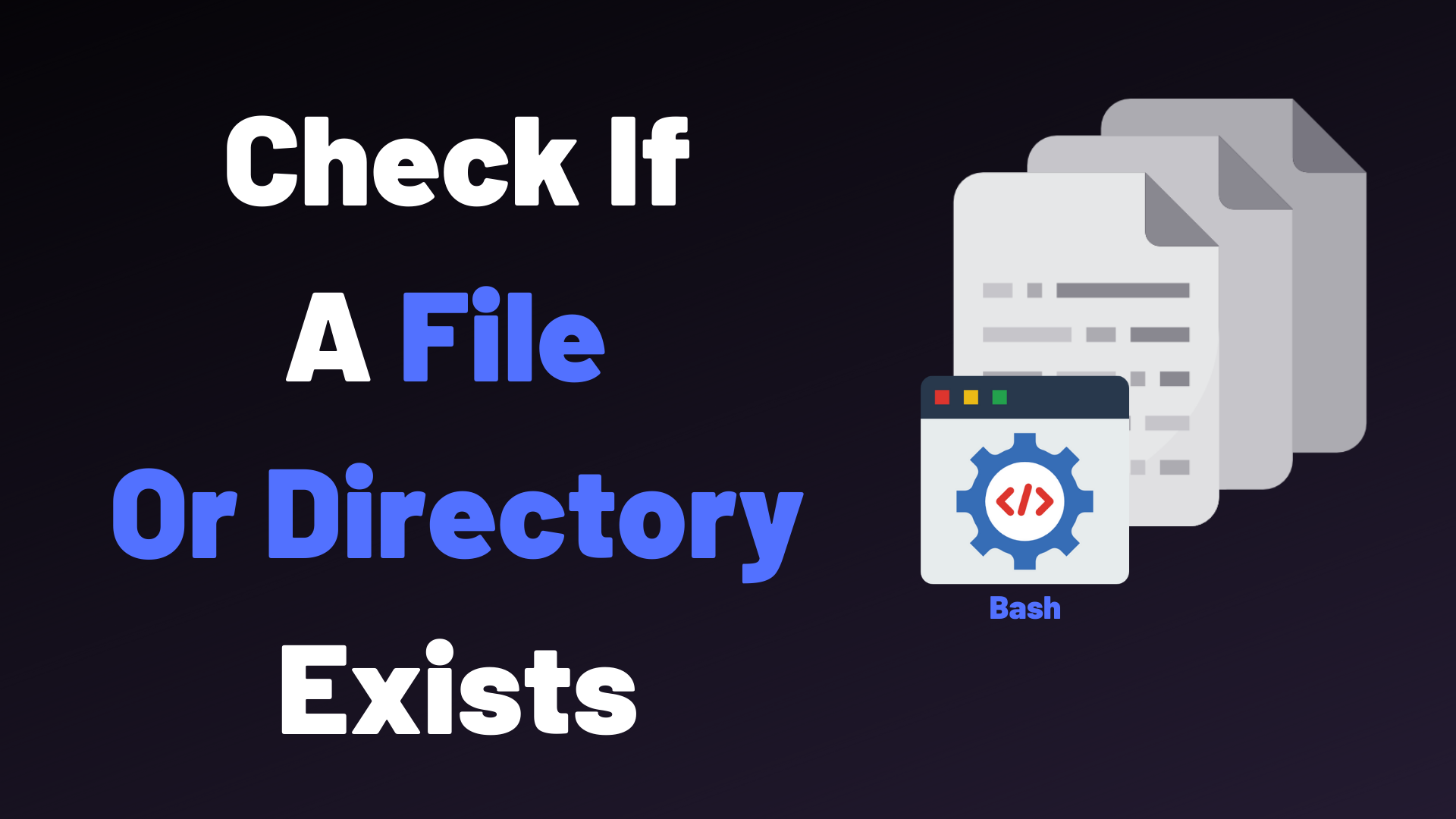
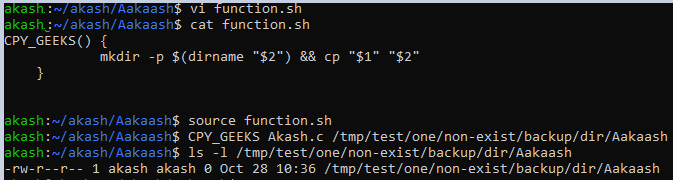
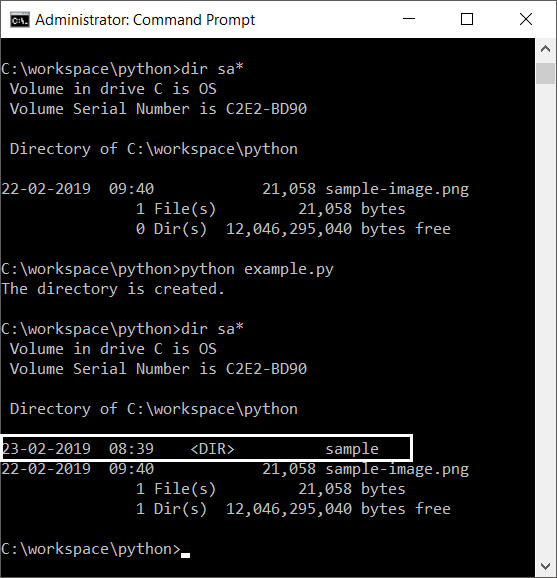

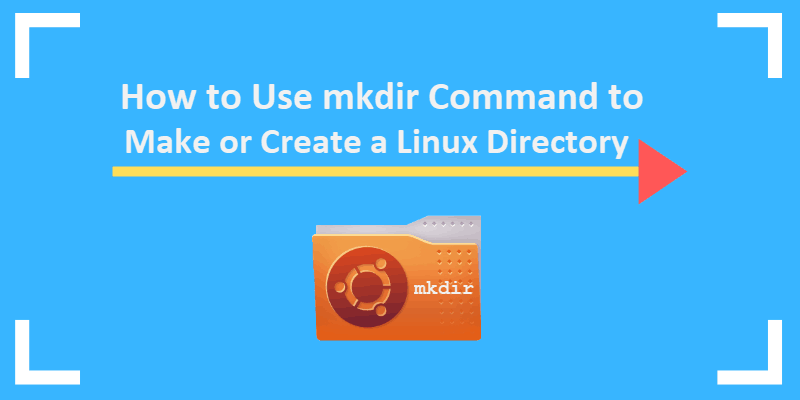
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/all-fs-methods-1.png)
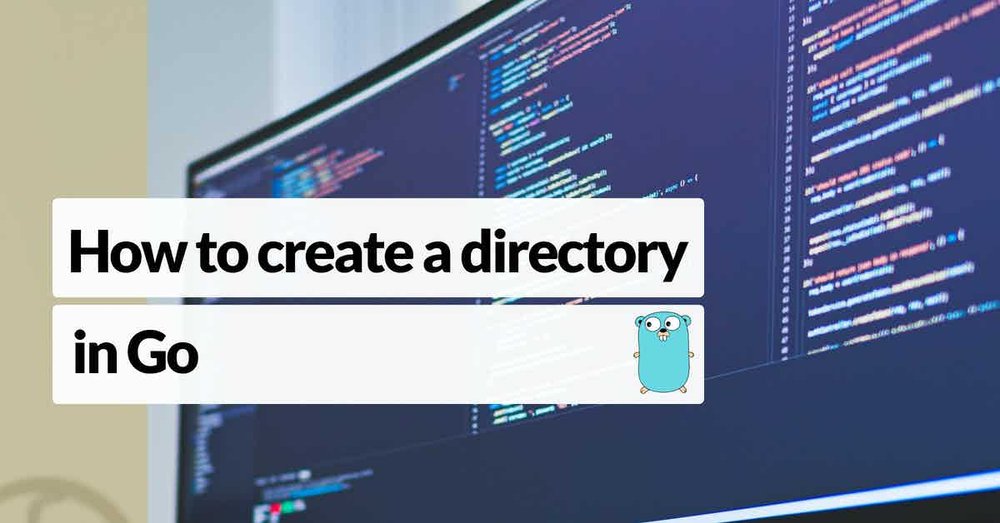

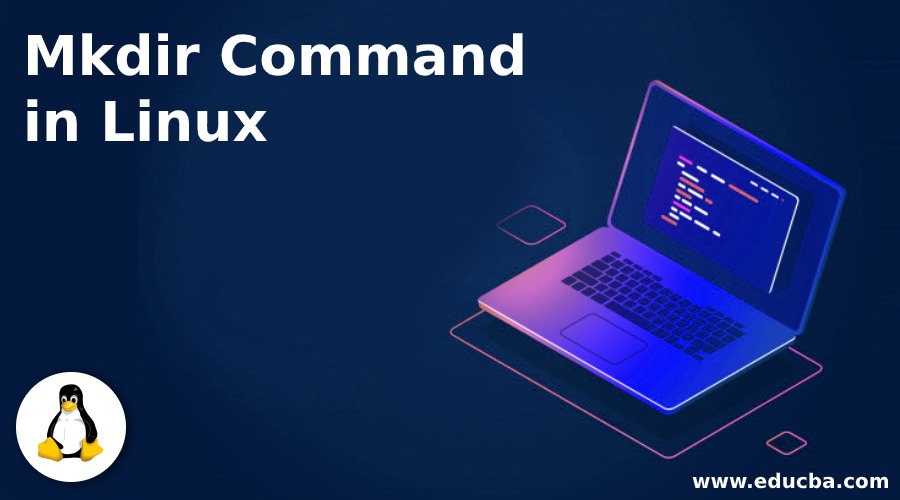


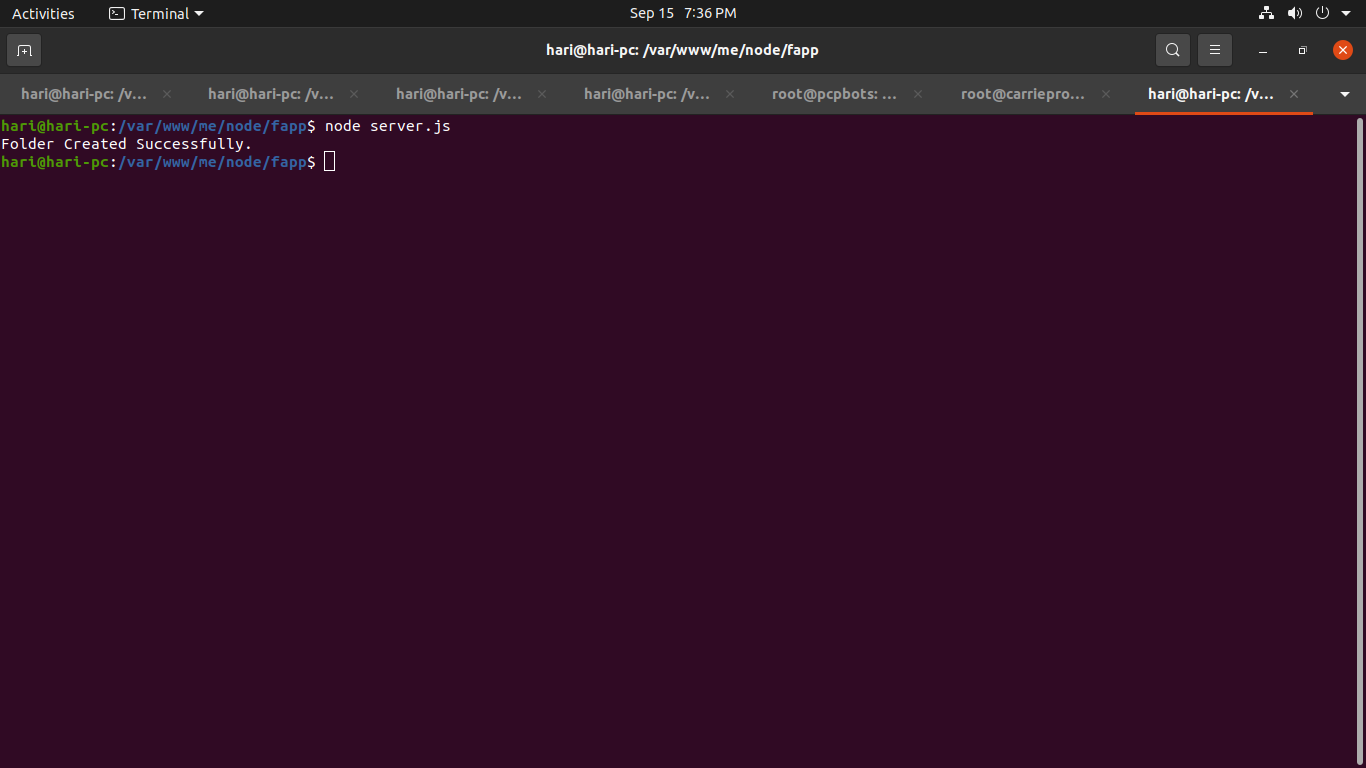


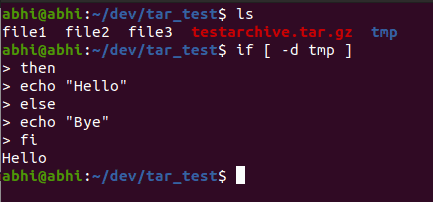
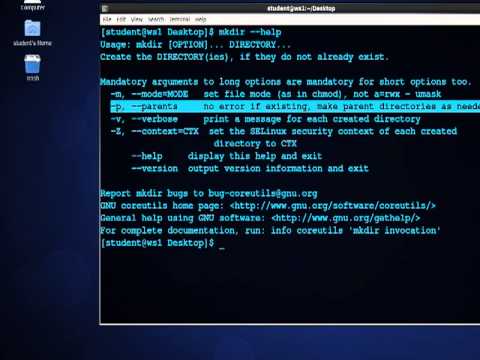
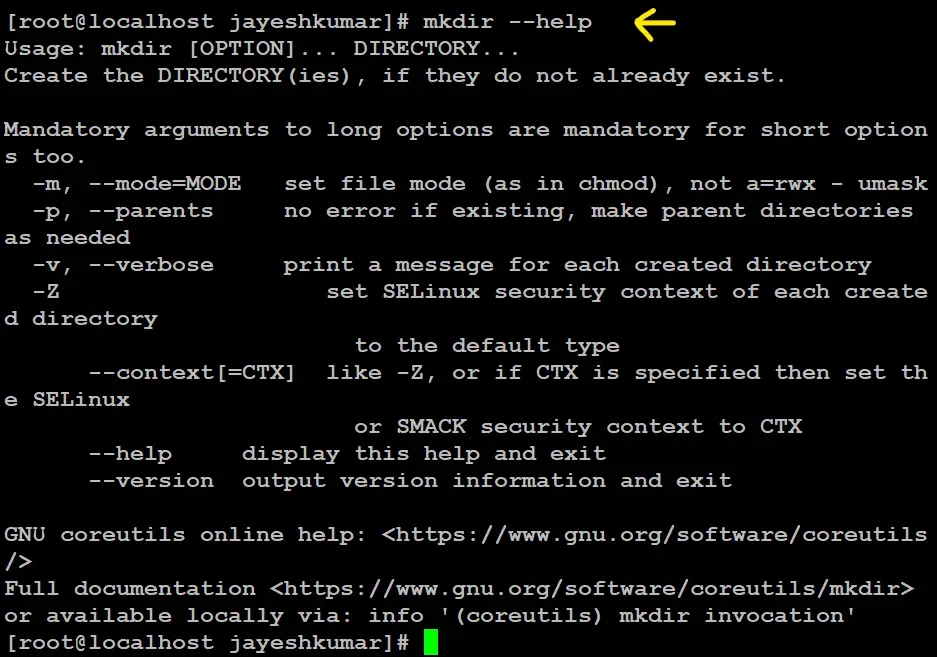
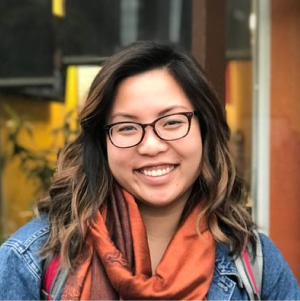
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/recursive-directory-creation.png)

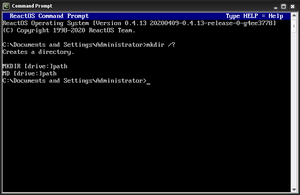
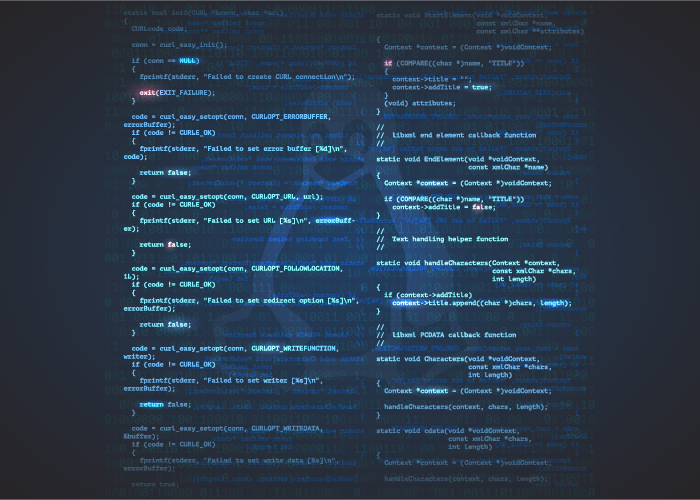
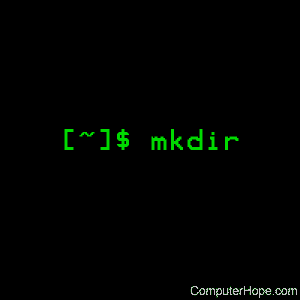
![Create Folder Linux | mkdir command in Linux [Create Directory] Create Folder Linux | Mkdir Command In Linux [Create Directory]](https://monovm.com/wp-content/uploads/2022/12/create-directory-linux107-main.webp)
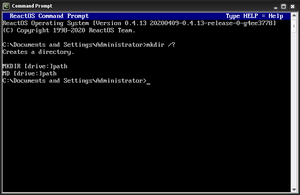

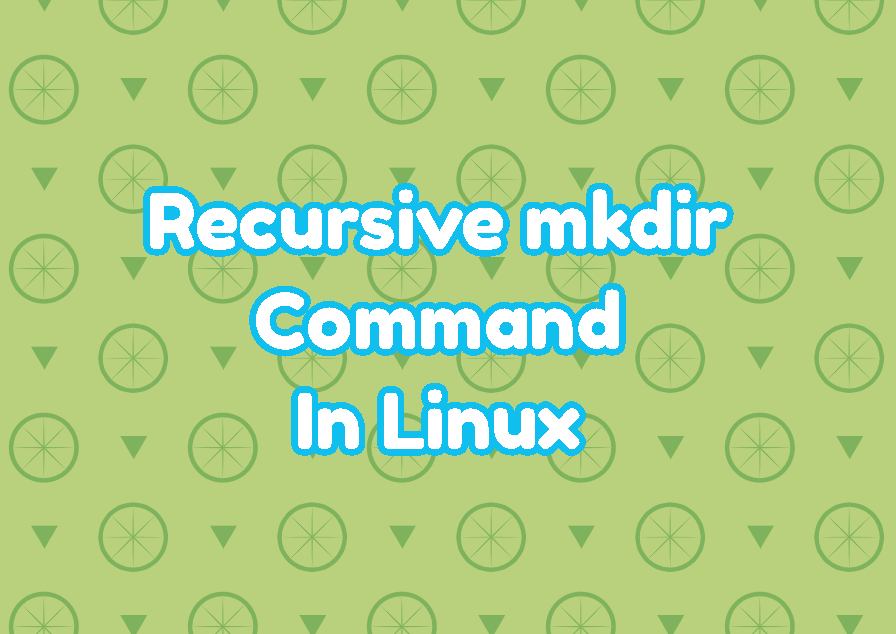
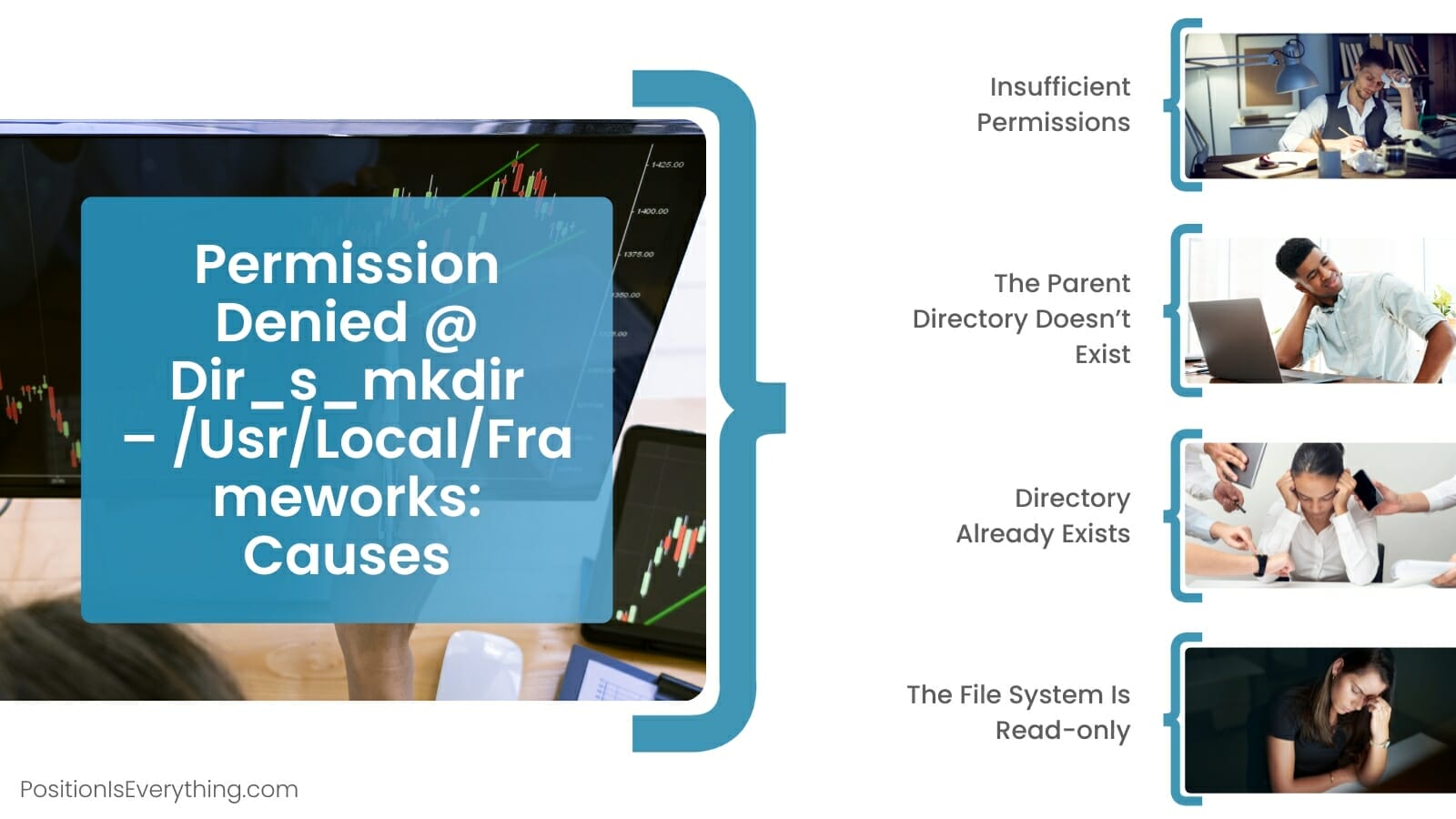
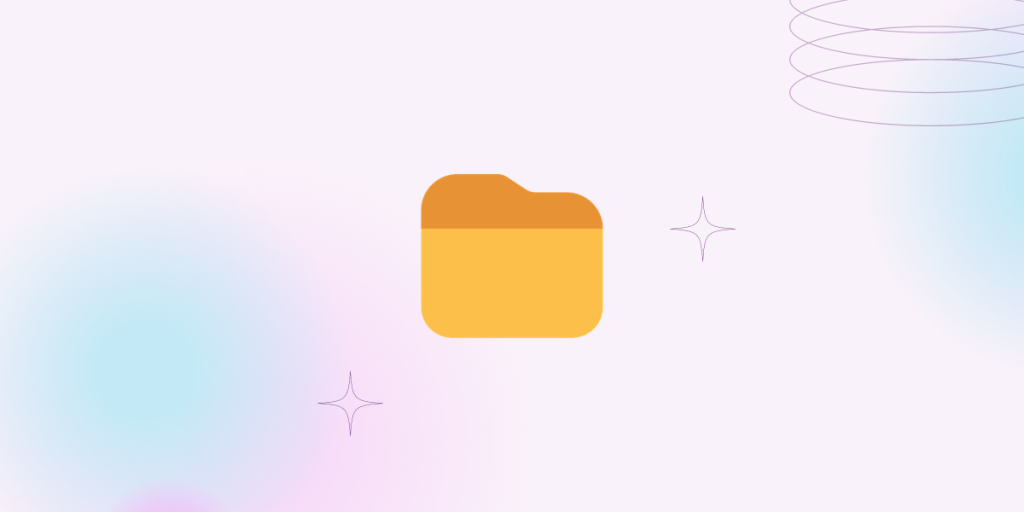
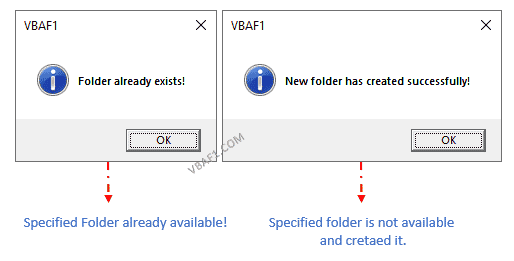
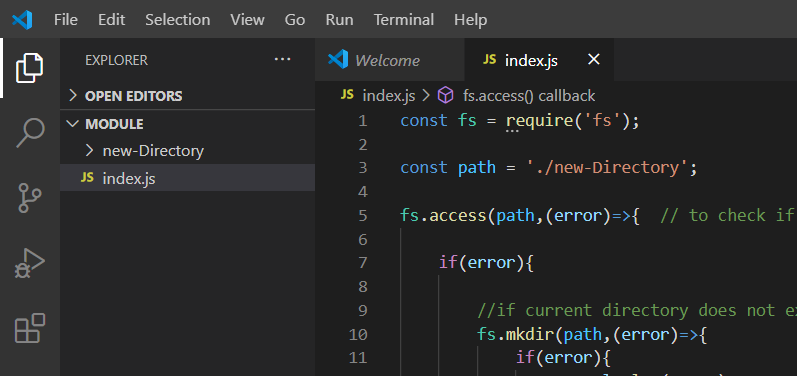


![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/create_dir_nodejs.jpg)
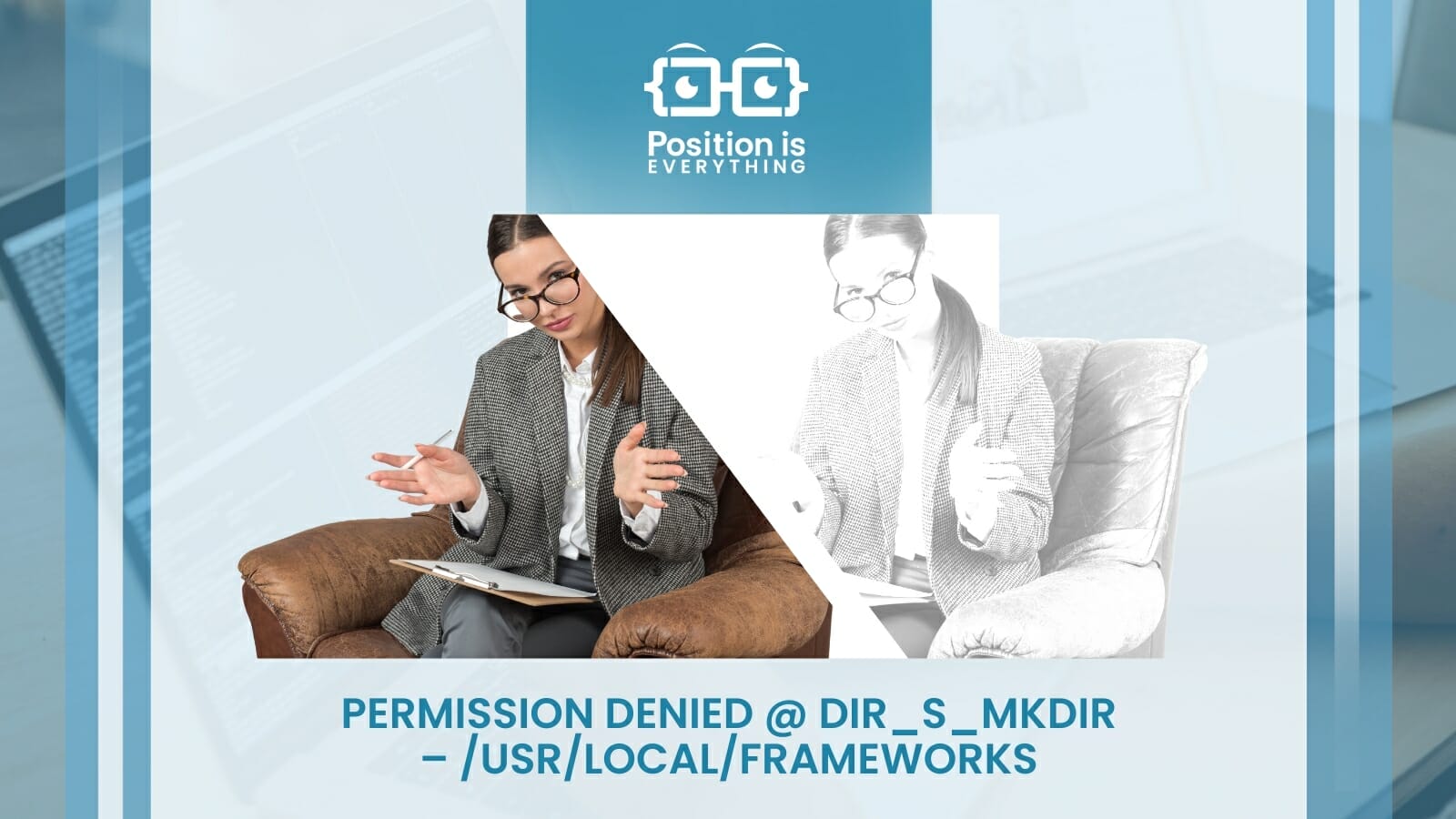
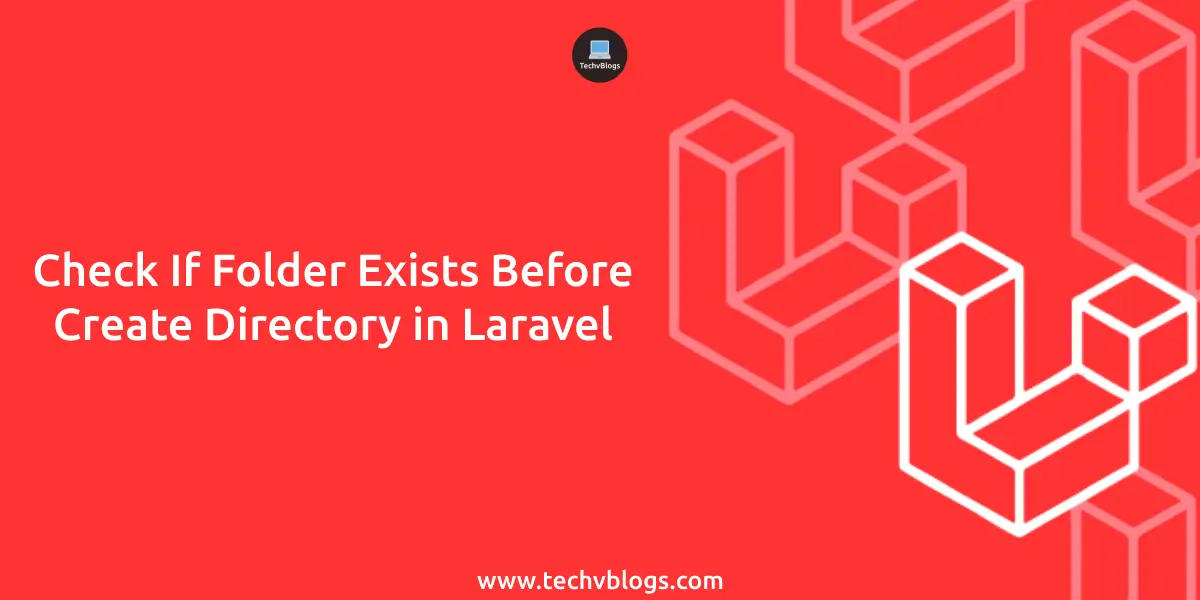
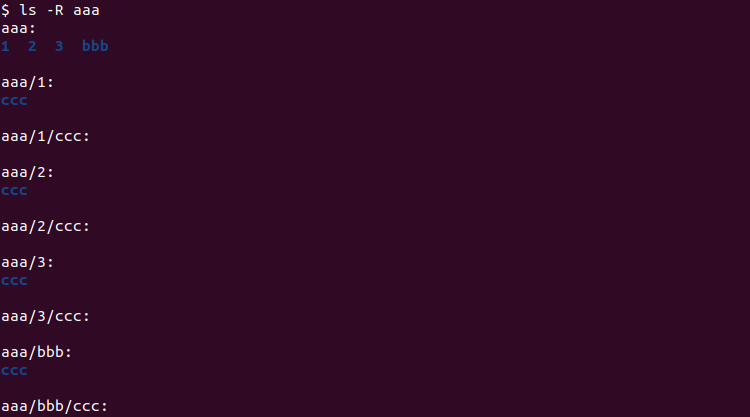
Article link: mkdir if not exists.
Learn more about the topic mkdir if not exists.
- How to mkdir only if a directory does not already exist?
- mkdir if not exists bash / linux – Warp terminal
- Make Directory Only if it Doesn’t Exist Already in Linux
- How to Create a Directory If It Does Not Exist in Linux
- How To “mkdir” Only If Directory Not Exist In Linux? – LinuxTect
- Bash ‘mkdir’ not existent path – Linux Hint
- How can I create a directory in Python using ‘mkdir if not exists’?
- How to create a directory if it does not exist in Go
- Python: Create a Directory if it Doesn’t Exist – Datagy
- How can I create a directory if it does not exist using Python
See more: https://nhanvietluanvan.com/luat-hoc