Groupby To Dictionary C#
GroupBy is a powerful feature in C# that allows you to group elements in a collection based on a specified key. It is particularly useful when you need to analyze and manipulate data based on common characteristics. This article will provide a comprehensive overview of GroupBy in C#, including its definition, purpose, benefits, syntax, and implementation.
Definition of GroupBy
In C#, GroupBy is an extension method that is part of the System.Linq namespace. It is used to group elements in a collection based on a specified key. The result of a GroupBy operation is a sequence of groups, where each group consists of a key and a collection of elements that share the same key.
Purpose and Benefits of GroupBy
The main purpose of GroupBy is to simplify the process of grouping elements in a collection based on a specific criterion. This can be particularly useful in scenarios where you need to analyze data based on common attributes or perform aggregate operations on groups of elements.
Some of the benefits of using GroupBy in C# include:
1. Simplified code: GroupBy provides a concise and readable way to group elements in a collection without the need for complex loops or conditional statements.
2. Efficient data analysis: By grouping elements based on a key, you can easily analyze and manipulate data that share common characteristics.
3. Improved performance: GroupBy uses efficient algorithms to group elements, resulting in faster execution compared to manual grouping implementations.
4. Flexibility: GroupBy allows you to specify the key and value for the resulting groups, providing flexibility in how you organize and process your data.
Syntax and Implementation of GroupBy in C#
The syntax for using GroupBy in C# is as follows:
var result = collection.GroupBy(keySelector);
In this syntax, “collection” represents the source collection, and “keySelector” is a lambda expression or delegate that defines the key for grouping the elements.
Here’s an example that demonstrates the implementation of GroupBy in C#:
var numbers = new List
var result = numbers.GroupBy(x => x % 2 == 0 ? “Even” : “Odd”);
In this example, the “numbers” list is grouped based on whether each element is even or odd. The resulting groups will be “Even” and “Odd”.
Understanding the KeySelector and ElementSelector Parameters
The keySelector parameter in the GroupBy method is responsible for defining the key by which the elements will be grouped. It can be specified using a lambda expression or a delegate that takes an element as input and returns the key.
For example, if you have a collection of persons and you want to group them by their age, you can use the following keySelector lambda expression:
var result = persons.GroupBy(p => p.Age);
The elementSelector parameter is optional and allows you to specify a projection for each element in the resulting group. It can be used to extract certain properties or perform calculations on each element.
Using GroupBy with LINQ in C#
Before diving into the details of using GroupBy in C#, it’s important to have a basic understanding of LINQ (Language Integrated Query) in C#.
Introduction to LINQ in C#
LINQ is a powerful query language that allows you to perform operations on data sources such as collections, databases, and XML documents. It provides a consistent and intuitive way to query and manipulate data, making it easier to write code that is both concise and readable.
Utilizing GroupBy with LINQ Queries
In LINQ, you can use the GroupBy method within a query expression to group data based on a specified key. Here’s an example that demonstrates how to use GroupBy in a LINQ query:
var result = from person in persons
group person by person.Age into ageGroup
select new { Age = ageGroup.Key, Count = ageGroup.Count() };
In this example, the “persons” collection is grouped by the “Age” property, and the result is projected into an anonymous type containing the age and the count of persons for each age.
Applying GroupBy with Lambda Expressions
GroupBy can also be used with lambda expressions in LINQ. Here’s an example that demonstrates how to use GroupBy with lambda expressions:
var result = persons.GroupBy(p => p.Age)
.Select(ageGroup => new { Age = ageGroup.Key, Count = ageGroup.Count() });
This example achieves the same result as the previous LINQ query, but it uses lambda expressions instead of query syntax.
Comparing and Contrasting LINQ and Lambda Expressions in GroupBy
Both LINQ queries and lambda expressions can be used to apply GroupBy in C#. The choice between the two approaches depends on personal preference and the specific requirements of the application.
LINQ queries provide a declarative and expressive way to query and manipulate data. They are particularly useful when working with complex queries involving multiple operations such as filtering, sorting, and grouping.
Lambda expressions, on the other hand, provide a more concise and functional programming-oriented approach. They are often favored by developers who are more comfortable with functional programming or who prioritize code brevity.
Creating a Dictionary with GroupBy in C#
GroupBy in C# can also be used to create a dictionary where the key represents the grouping criteria, and the value represents the collection of elements that belong to that group.
Exploring the Dictionary Class in C#
In C#, a Dictionary is a collection that stores key-value pairs, where each key must be unique. It provides fast retrieval of values based on their associated keys, making it an efficient data structure for storing and accessing data.
How GroupBy Can Generate a Dictionary
By combining the GroupBy method with the Dictionary class, you can easily create a dictionary from a collection of elements based on a specified key. Here’s an example that demonstrates how to use GroupBy to generate a dictionary:
var personsByCountry = persons.GroupBy(p => p.Country)
.ToDictionary(g => g.Key, g => g.ToList());
In this example, the collection of persons is grouped by “Country”, and the result is converted into a dictionary where the key is the country and the value is a list of persons belonging to that country.
Specifying Key and Value for the Dictionary
In the previous example, the key for the dictionary is specified using the KeySelector lambda expression (p => p.Country). The value, which represents the collection of persons, is specified using the ElementSelector lambda expression (g => g.ToList()).
You can customize the key and value for the dictionary based on your specific requirements. For example, you can project additional properties or perform calculations on the grouped elements to derive the key and value.
Working with Multiple Levels of GroupBy in C#
In addition to grouping elements based on a single criterion, GroupBy in C# can also be used to perform multi-level grouping, where elements are grouped based on multiple keys.
Overview of Nested GroupBy
Nested GroupBy refers to the process of applying multiple GroupBy operations sequentially to achieve multi-level grouping. Each GroupBy operation is applied to the result of the previous operation, resulting in a hierarchical grouping structure.
Nesting GroupBy Statements
Here’s an example that demonstrates how to nest GroupBy statements to perform multi-level grouping in C#:
var result = persons.GroupBy(p => p.Country)
.Select(countryGroup => new
{
Country = countryGroup.Key,
Cities = countryGroup.GroupBy(p => p.City)
});
In this example, the persons’ collection is first grouped by “Country”, and then each country group is further grouped by “City”. The result is projected into an anonymous type that contains the country and a collection of cities for each country.
Analyzing the Composite Key in Multi-Level GroupBy
In multi-level GroupBy operations, the keys used for grouping are composite keys consisting of the keys from each level. For example, in the previous example, the key for the second-level grouping (cityGroup) is a combination of the country and city.
When working with composite keys, it’s important to consider the uniqueness of the keys to ensure that each group is correctly represented.
Performing Aggregate Operations on GroupBy Results in C#
GroupBy in C# not only allows you to group elements but also enables you to perform aggregate operations on the grouped elements. Aggregate operations involve applying an operation or function to a sequence of elements and returning a single result.
Introduction to Aggregate Operations in C#
Aggregate operations in C# involve functions or operations that are applied to a sequence of elements to calculate a summary value. Examples of aggregate operations include sum, count, average, minimum, and maximum.
Applying Aggregate Functions to GroupBy Results
You can easily apply aggregate functions to the groups generated by GroupBy in C#. Here’s an example that demonstrates how to use the Sum function on a grouped collection:
var result = persons.GroupBy(p => p.Country)
.Select(countryGroup => new
{
Country = countryGroup.Key,
TotalPopulation = countryGroup.Sum(p => p.Population)
});
In this example, the “Population” property of each person is summed within each country group, resulting in the total population for each country.
Understanding the Use of Aggregators in GroupBy
Aggregators in GroupBy allow you to perform custom aggregate operations on the grouped elements. Aggregators are implemented using the Aggregate method, which takes an accumulator function and an optional seed value.
Here’s an example that demonstrates how to use an aggregator in a GroupBy operation:
var result = persons.GroupBy(p => p.Country)
.Select(countryGroup => new
{
Country = countryGroup.Key,
LargestCity = countryGroup.Aggregate((max, next) => max.Population > next.Population ? max : next)
});
In this example, the aggregator function compares the population of each city within a country group and returns the city with the largest population.
Handling Null Values and Empty Collections in GroupBy in C#
When working with GroupBy in C#, it’s important to consider scenarios where the grouping criteria may result in null values or empty collections. Handling such scenarios effectively is crucial for producing accurate and error-free results.
Handling Null Values in GroupBy
If the keySelector or elementSelector lambda expression in the GroupBy operation returns null for any element, it can lead to unexpected behavior or exceptions. To handle null values, consider using null coalescing operators or conditional statements within the lambda expressions.
Managing Empty Collections in GroupBy
If the GroupBy operation results in an empty collection, it may indicate that there are no elements that satisfy the grouping criteria. It’s important to handle this scenario gracefully in your code by checking for empty collections and applying appropriate logic.
Best Practices for Dealing with Null and Empty Values in GroupBy
Here are some best practices to follow when dealing with null and empty values in GroupBy:
1. Ensure the keySelector or elementSelector lambda expressions handle null values appropriately, either by returning a default value or skipping the null elements.
2. Handle empty collections by checking for them explicitly and applying the necessary logic to prevent errors or unexpected behavior.
3. Use defensive programming techniques, such as null checks and exception handling, to handle unexpected scenarios and provide informative error messages.
By following these best practices, you can ensure that your code is robust and resilient when dealing with null values or empty collections in GroupBy operations.
FAQs
1. Can GroupBy be applied to any type of collection in C#?
Yes, GroupBy can be applied to any collection that implements the IEnumerable interface, such as arrays, lists, or dictionaries.
2. Can I use GroupBy with custom objects?
Yes, you can use GroupBy with custom objects. However, you need to ensure that the keySelector lambda expression or delegate is defined properly to specify the grouping criteria for the custom objects.
3. How can I perform aggregation on specific properties of each element within a group?
You can use aggregate functions such as Sum, Count, Average, Min, or Max to perform calculations on specific properties of the elements within a group. This can be done by specifying the property to be aggregated within the lambda expression used in the aggregate function.
4. What is the difference between ToLookup and GroupBy in C#?
ToLookup and GroupBy are similar in that they both allow you to group elements based on a key. The main difference is that ToLookup returns a lookup table that supports multiple values for each key, whereas GroupBy returns a sequence of groups that each contains a key and a collection of elements.
Conclusion
GroupBy in C# is a powerful feature that allows you to easily group elements in a collection based on a specified key. It provides a concise and efficient way to analyze and manipulate data, allowing you to perform aggregate operations or create dictionaries based on grouping criteria. By understanding the syntax, implementation, and best practices for using GroupBy in C#, you can enhance the efficiency and readability of your code.
Part 18 Groupby In Linq
Keywords searched by users: groupby to dictionary c# List group by to dictionary C#, Linq group by count to dictionary, LINQ group by, IGrouping to dictionary, Tolookup vs groupby, List to Dictionary C#, Dictionary group by multiple values c#, C# group by multiple values
Categories: Top 33 Groupby To Dictionary C#
See more here: nhanvietluanvan.com
List Group By To Dictionary C#
Introduction:
In C#, working with data often requires grouping and aggregating information based on specific attributes or criteria. This task can be quite cumbersome and time-consuming, especially when dealing with large amounts of data. However, C# provides a powerful and efficient method called “List Group By to Dictionary” that simplifies this process. This article will explore the usage and benefits of List Group By to Dictionary in C#, along with examples and frequently asked questions.
Understanding List Group By to Dictionary:
The List Group By to Dictionary method in C# allows us to group data in a list based on a specified key and convert it into a dictionary. This dictionary then provides easy access to the grouped data, making it convenient for subsequent operations, analysis, or retrieval.
The Syntax:
The basic syntax of List Group By to Dictionary in C# is as follows:
“`csharp
var dictionary = list.GroupBy(item => item.KeyProperty)
.ToDictionary(group => group.Key, group => group.ToList());
“`
In the above syntax, `list` represents the original list of data, `item.KeyProperty` is the property used for grouping, and `group` gives access to the grouped items. Finally, the ToDictionary method is used to convert the grouped data into a dictionary, with `group.Key` as the dictionary key and `group.ToList()` as the corresponding value.
Example Usage:
To clarify the usage of List Group By to Dictionary, let’s consider an example where we have a list of employees and we want to group them by their departments.
“`csharp
public class Employee
{
public string Name { get; set; }
public string Department { get; set; }
}
List
{
new Employee { Name = “John”, Department = “HR” },
new Employee { Name = “Alice”, Department = “IT” },
new Employee { Name = “Mark”, Department = “IT” },
new Employee { Name = “Sarah”, Department = “HR” }
};
var employeesByDepartment = employees.GroupBy(emp => emp.Department)
.ToDictionary(group => group.Key, group => group.ToList());
“`
In the above example, the `employees` list is grouped by the `Department` property using List Group By to Dictionary. The resulting dictionary, `employeesByDepartment`, will have keys as the department names (“HR” and “IT”) and corresponding values as lists of employees belonging to each department.
Benefits and Use Cases:
List Group By to Dictionary offers several benefits that simplify data manipulation and analysis in C#. Some of these include:
1. Easy access: By converting a grouped list into a dictionary, we can access grouped data conveniently using keys, allowing faster retrieval and operations.
2. Improved efficiency: Grouping data with List Group By to Dictionary reduces the complexity of subsequent data operations as it eliminates the need for iteration or manual grouping.
3. Enhanced data analysis: List Group By to Dictionary is particularly useful when performing data analysis, allowing for efficient aggregation, filtering, or calculation of grouped data.
4. Simplified data retrieval: Using a grouped dictionary makes it simpler to fetch specific groups of data without unnecessary iterations, resulting in faster and more optimized code.
Frequently Asked Questions (FAQs):
Q1: Can the List Group By to Dictionary method handle custom objects as keys?
A1: Yes, List Group By to Dictionary can handle custom objects as keys. The keys used for grouping can be any type, including custom objects, provided they properly implement equality and hash code comparison methods.
Q2: How does List Group By to Dictionary handle duplicate keys?
A2: When duplicate keys are encountered during grouping, an exception is thrown. To handle duplicate keys, you can use the `ToLookup` method instead, which returns an `ILookup` object that can hold multiple values under a single key.
Q3: Is it possible to perform additional operations on grouped data after using List Group By to Dictionary?
A3: Yes, it is possible to further manipulate grouped data after converting it into a dictionary. The resulting dictionary provides a collection of Key-Value pairs, allowing easy access to grouped data for subsequent operations such as filtering, aggregation, or even nested groupings.
Q4: Can List Group By to Dictionary be used on LINQ query results?
A4: Yes, List Group By to Dictionary can be used on the results of LINQ queries. It allows efficient grouping and conversion of query results into a dictionary, enabling further analysis or data operations.
Conclusion:
The List Group By to Dictionary method in C# is a powerful tool for simplifying data manipulation and analysis. By grouping data in a list based on specific criteria and converting it into a dictionary, this method improves efficiency, provides easy access to grouped data, and simplifies subsequent operations. Whether you are working on data analysis, filtering, or need to organize data for retrieval, List Group By to Dictionary proves to be a valuable technique in C# programming.
Linq Group By Count To Dictionary
Introduction:
In the world of computer programming, there are various tools and techniques that streamline the process of data manipulation. Among these, Language Integrated Query (LINQ) stands out for its simplicity and versatility. One powerful feature of LINQ is the ability to group data based on certain criteria. In this article, we will delve into the concept of grouping data using LINQ’s Group By clause and utilize a Dictionary to store the results. By the end, you will have a solid understanding of how to utilize LINQ Group By Count to Dictionary in your C# projects.
Understanding LINQ Group By Count:
Before we dive into the details, let’s clarify the purpose of the Group By clause in LINQ. It allows us to partition a collection based on specified criteria and create groups of elements that share common attributes. This grouping operation is extremely helpful when dealing with large datasets and wanting to perform operations on subsets of data.
The Group By Count operation is particularly useful when we want to count the occurrences of a specific condition within each group. For instance, let’s assume we have a collection of students, and we want to know how many students belong to each grade level. By using the Group By Count operation, we can easily determine the frequency of students in each grade.
Implementation of LINQ Group By Count to Dictionary in C#:
To employ the Group By Count to Dictionary technique, we first need a collection of data to work with. Let’s say we have a List of Student objects as follows:
“`csharp
List
{
new Student { Name = “John”, Grade = 10 },
new Student { Name = “Emily”, Grade = 9 },
new Student { Name = “Michael”, Grade = 10 },
new Student { Name = “Samantha”, Grade = 11 },
new Student { Name = “David”, Grade = 9 },
new Student { Name = “Olivia”, Grade = 11 },
};
“`
Here, the Student class has two properties: Name and Grade. Our goal is to count the occurrences of each grade level. To achieve this, we can use the GroupBy() method in LINQ along with the Count() method. Here’s how:
“`csharp
var gradeCounts = students
.GroupBy(s => s.Grade)
.ToDictionary(g => g.Key, g => g.Count());
“`
In this code snippet, we first use the GroupBy() method to group the students based on their grade. Then, we convert the resulting grouped data into a Dictionary using the ToDictionary() method. The Dictionary’s key is the Grade, while the value is the count of students in that grade.
Now, if we iterate through the gradeCounts dictionary, we can access the grade levels and their respective counts:
“`csharp
foreach (var gradeCount in gradeCounts)
{
Console.WriteLine($”Grade {gradeCount.Key}: {gradeCount.Value} students”);
}
“`
This will produce the following output:
“`
Grade 10: 2 students
Grade 9: 2 students
Grade 11: 2 students
“`
As you can see, we have successfully grouped the students by grade and obtained the count of students in each grade level using LINQ’s powerful Group By Count to Dictionary technique.
FAQs:
Q1. What is the advantage of using LINQ Group By Count to Dictionary over traditional loops?
A1. One major advantage is the concise and readable syntax that LINQ offers. It simplifies complex grouping and counting operations that would otherwise require multiple lines of code. Additionally, LINQ’s performance optimizations make it more efficient for handling large datasets.
Q2. Can we group data based on multiple criteria?
A2. Absolutely! LINQ allows you to chain multiple Group By operations to achieve multi-level grouping. For instance, you can group students by grade level first and then by gender or any other criteria you desire.
Q3. Can I use Group By Count to Dictionary with any type of collection?
A3. Yes, as long as the collection implements the IEnumerable
Q4. Are there any performance considerations when using Group By Count to Dictionary?
A4. While LINQ offers performance optimizations under the hood, it is important to remember that extensive use of LINQ operations can impact performance. In scenarios where performance is critical, it is advisable to analyze the specific requirements and evaluate whether LINQ offers the most efficient solution.
Conclusion:
LINQ Group By Count to Dictionary is a powerful technique that allows us to effectively group data and obtain count results in a concise and readable manner. By utilizing the capabilities of LINQ and the flexibility of the Dictionary data structure, we can perform complex grouping and counting operations with minimal code. Remember to always analyze the specific requirements of your project and use LINQ’s Group By Count to Dictionary when it best suits your needs. Happy coding!
Linq Group By
Introduction:
In the world of data processing and analysis, grouping data based on certain criteria is a common task. LINQ, short for Language-Integrated Query, is a powerful querying language integrated into .NET programming languages such as C#. One of LINQ’s essential features is the “group by” clause, which allows developers to group and aggregate data with ease. In this article, we will explore the concept of grouping data using LINQ’s “group by” clause, its syntax, and some practical examples. Let’s dive in!
Understanding the “Group By” Clause:
The “group by” clause in LINQ enables us to group elements of a collection based on common characteristics or properties. It is similar to the SQL GROUP BY clause but with more flexibility and extensibility. The “group by” clause transforms a flat collection into a hierarchical structure, allowing us to work with grouped data more efficiently.
Syntax of the “Group By” Clause:
The basic syntax of the “group by” clause is as follows:
var result = from element in collection
group element by element.Property into groupedElements
select groupedElements;
In the above syntax, “element” refers to each element of the collection, while “Property” represents the property based on which we want to group the elements. “groupedElements” is a range variable that contains the grouped elements.
Example 1: Grouping Integers based on Parity
Let’s start with a simple example to grasp the concept. Consider a collection of integers, and we want to group them based on their parity (either even or odd). Here’s the LINQ query for accomplishing this task:
var numbers = new List
var groupedNumbers = from number in numbers
group number by number % 2 into grouped
select grouped;
In the above example, we define a collection of integers and apply the “group by” clause to group them based on the remainder when divided by 2. The resulting “groupedNumbers” will be a hierarchical structure with two groups, one for even numbers and one for odd numbers.
Example 2: Grouping Employees by Department
Let’s move on to a more practical example. Suppose we have a collection of employee objects, each having properties like Name, Department, and Salary. We want to group employees by their respective departments. Here’s the LINQ query to achieve this:
var employees = new List
var groupedEmployees = from employee in employees
group employee by employee.Department into grouped
select grouped;
In this example, we have a collection of Employee objects and group them based on their Department property. The resulting “groupedEmployees” will provide a hierarchical structure with each group representing employees from a specific department.
Aggregation with Group By:
The “group by” clause not only allows us to group data but also facilitates aggregation within each group. We can use aggregation functions like Count, Sum, Average, Min, Max, etc., to perform calculations on grouped data. Here’s an example demonstrating the aggregation capability:
var salaries = new List
var groupedSalaries = from salary in salaries
group salary by salary > 2000 into grouped
select new
{
Category = grouped.Key ? “High” : “Low”,
AverageSalary = grouped.Average(),
TotalEmployees = grouped.Count()
};
In this example, we group salaries based on whether they are greater than 2000. The resulting “groupedSalaries” object will contain two groups labeled as “High” and “Low,” along with their average salaries and the count of employees in each group.
FAQs (Frequently Asked Questions):
Q: Can we group elements based on multiple properties simultaneously?
A: Yes, we can use anonymous types or custom classes to combine multiple properties and use them for grouping.
Q: What happens if a group has no elements?
A: If a group has no elements, it still appears in the result with an empty sequence.
Q: Can we use LINQ’s “group by” clause with databases?
A: Absolutely! LINQ’s “group by” clause works seamlessly with databases too. You can group data fetched from a database table using LINQ to SQL or LINQ to Entities.
Q: Is the order of groups determined?
A: The order of groups in the result is not guaranteed unless specifically mentioned using an additional sorting clause.
Q: Can we nest “group by” clauses?
A: Yes, we can nest “group by” clauses to achieve hierarchical grouping based on multiple properties.
In conclusion, LINQ’s “group by” clause is a powerful tool for grouping and aggregating data efficiently. It simplifies the process of data analysis and enables developers to work with grouped data effortlessly. Understanding the syntax and various applications of the “group by” clause allows programmers to write elegant and concise code for complex data grouping tasks. So, unleash the power of LINQ’s “group by” clause and simplify your data grouping and aggregation workflow!
Images related to the topic groupby to dictionary c#
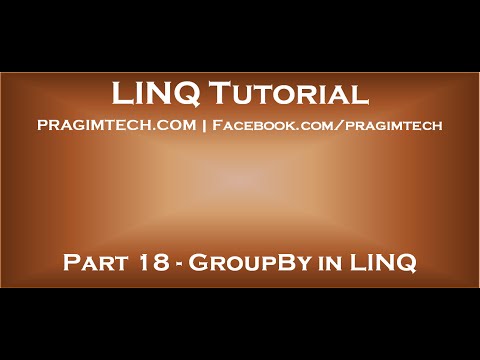
Article link: groupby to dictionary c#.
Learn more about the topic groupby to dictionary c#.
- LINQ Group By into a Dictionary Object – Stack Overflow
- LINQ Group By to Dictionary Object | End Your If
- Using Linq to create a Dictionary of sub-Lists by grouping from …
- Dictionary.GroupBy, Uiml.net C# (CSharp) Code Examples
- ToDictionary() – for enumerations of groupings – C#
- Group query results (LINQ in C#) – Microsoft Learn
- LINQ Group By to Dictionary Object | End Your If
- LINQ ToDictionary() Method – Javatpoint
- C# | Dictionary.Add() Method – GeeksforGeeks
- Add key-value pair in C# Dictionary – Tutorialspoint
- [Solved]-How to get list of keys in a dictionary with group by …
- C# GroupBy Method – Dot Net Perls
- Python | Grouping dictionary keys by value – GeeksforGeeks
- c# – Need help finding an efficient way to group the keys in a …
See more: nhanvietluanvan.com/luat-hoc