Js Else If Shorthand
JavaScript is a versatile programming language that allows developers to create dynamic and interactive web pages. One important aspect of JavaScript is its ability to make decisions or perform specific actions based on different conditions. The “else if” statement is a key feature in JavaScript that allows you to handle multiple conditions in a more efficient way. In this article, we will explore the concept of else if shorthand in JavaScript, its syntax and usage, the benefits of using it, common mistakes to avoid, and examples of its applications.
Explanation of the Else If Statement
Before diving into else if shorthand, let’s first understand the concept of the else if statement in JavaScript. The else if statement is an extension of the if statement and is used when you want to test multiple conditions. It allows you to provide alternative code blocks to be executed when the first condition is not met.
Traditionally, the syntax of an else if statement looks like this:
“`javascript
if (condition1) {
// code block to be executed if condition1 is true
} else if (condition2) {
// code block to be executed if condition2 is true
} else {
// code block to be executed if none of the conditions are true
}
“`
Comparing Else If with If and Else Statements
To better understand the role of else if, let’s compare it with the if and else statements.
The if statement allows you to execute a code block if a certain condition is met. However, if you have multiple conditions to check, using multiple if statements can become repetitive and inefficient.
The else statement is used to define a code block that will be executed if the condition of the if statement is false. However, it does not allow you to check for additional conditions.
The else if statement comes in handy when you have multiple conditions to evaluate. It allows you to test each condition one by one until a true condition is found or execute the code block defined in the else statement if none of the conditions are true.
Syntax and Usage of Else If Shorthand
The else if shorthand in JavaScript provides a more concise way to write multiple else if statements. Instead of using the traditional else if syntax, you can achieve the same result using a shorter syntax.
“`javascript
condition1 ? (code block to be executed if condition1 is true)
: condition2 ? (code block to be executed if condition2 is true)
: condition3 ? (code block to be executed if condition3 is true)
: (code block to be executed if none of the conditions are true);
“`
Benefits of Using Else If Shorthand
Using the else if shorthand offers several benefits. Firstly, it simplifies your code by reducing the number of lines you need to write. This can lead to cleaner and more readable code. Secondly, it reduces the chances of making syntax errors by eliminating the need for nested if statements. Finally, it improves the performance of your code by reducing unnecessary evaluations.
Handling Multiple Conditions with Else If Shorthand
The else if shorthand comes in handy when you have multiple conditions to evaluate. You can chain multiple else if statements together to test each condition one by one until a true condition is found. If none of the conditions are true, the code block defined after the last else statement will be executed.
“`javascript
let grade = 75;
let result = (grade >= 90) ? ‘A’
: (grade >= 80) ? ‘B’
: (grade >= 70) ? ‘C’
: (grade >= 60) ? ‘D’
: ‘F’;
“`
In this example, the grade is evaluated against multiple conditions using the else if shorthand. The result will be assigned the corresponding grade value based on the condition that evaluates to true.
Nested Else If Statements in JavaScript
Nested else if statements can become complex and difficult to read. However, with else if shorthand, you can avoid the need for nested statements and simplify your code.
“`javascript
if (condition1) {
// code block to be executed if condition1 is true
} else if (condition2) {
// code block to be executed if condition2 is true
} else if (condition3) {
// code block to be executed if condition3 is true
} else {
// code block to be executed if none of the conditions are true
}
“`
By using the else if shorthand, you can achieve the same result without unnecessary indentation.
Common Mistakes and Pitfalls to Avoid with Else If Shorthand
While else if shorthand can be a powerful tool, it’s important to be aware of potential pitfalls. A common mistake is forgetting to include the final else statement, which can lead to unexpected results. Make sure to define a code block to be executed if none of the conditions are true.
Another mistake to avoid is using complex conditional expressions within the else if shorthand. This can make your code difficult to read and maintain. It’s recommended to use simple conditions or break down complex conditions into separate variables or functions for better readability.
Examples and Applications of Else If Shorthand
Now let’s look at some examples and applications of else if shorthand in different scenarios.
If else if shorthand JavaScript:
“`javascript
let num = 10;
let result = (num > 0) ? ‘Positive’
: (num < 0) ? 'Negative'
: 'Zero';
console.log(result); // Output: Positive
```
JS shorthand if without else:
```javascript
let isRaining = true;
isRaining && console.log('Take an umbrella'); // Output: Take an umbrella
```
If...else one line JavaScript:
```javascript
let num = 5;
let result = (num % 2 === 0) ? 'Even' : 'Odd';
console.log(result); // Output: Odd
```
If...else JS:
```javascript
let age = 21;
(age < 18) ? console.log('Not eligible')
: console.log('Eligible'); // Output: Eligible
```
Shorthand if else Python:
```python
age = 21
status = 'Eligible' if age >= 18 else ‘Not eligible’
print(status) # Output: Eligible
“`
If JavaScript:
“`javascript
let x = 5;
if (x > 10) console.log(‘Greater than 10’);
else console.log(‘Less than or equal to 10’); // Output: Less than or equal to 10
“`
Switch case shorthand js:
“`javascript
let day = ‘Tuesday’;
switch(day) {
case ‘Monday’:
console.log(‘First day of the week’);
break;
case ‘Tuesday’:
console.log(‘Second day of the week’);
break;
default:
console.log(‘Invalid day’);
}
// Output: Second day of the week
“`
Short hand if else js else if shorthand:
“`javascript
let temperature = 25;
let weather = (temperature > 30) ? ‘Hot’
: (temperature > 20) ? ‘Warm’
: ‘Cool’;
console.log(weather); // Output: Warm
“`
In conclusion, the else if shorthand in JavaScript provides a more concise and efficient way to handle multiple conditions. It simplifies your code, reduces the chances of syntax errors, and improves its performance. By understanding the syntax and usage of else if shorthand, you can write cleaner and more readable code. Remember to avoid common mistakes and explore its various applications to make the most of this powerful feature.
FAQs
Q: Can I use multiple else if statements in JavaScript?
A: Yes, you can chain multiple else if statements together to handle multiple conditions.
Q: What is the benefit of using else if shorthand?
A: Using else if shorthand simplifies your code, reduces the chances of errors, and improves its performance.
Q: Is else if shorthand specific to JavaScript?
A: No, several programming languages provide shorthand syntax for else if statements.
Q: Can I nest else if statements in JavaScript?
A: Yes, you can nest else if statements, but it can make your code complex and harder to read.
Q: What are some common mistakes to avoid with else if shorthand?
A: Some common mistakes include forgetting to include the final else statement and using complex conditional expressions.
Javascript: Shorthand For If-Else Statement
Keywords searched by users: js else if shorthand If else if shorthand javascript, JS shorthand if without else, If…else one line JavaScript, If…else JS, Shorthand if else Python, If JavaScript, Switch case shorthand js, Short hand if else
Categories: Top 85 Js Else If Shorthand
See more here: nhanvietluanvan.com
If Else If Shorthand Javascript
In JavaScript, the if-else if-else shorthand is used when you have multiple conditions to evaluate. Instead of writing separate if-else statements for each condition, you can use the else if ladder to simplify your code. The shorthand syntax looks like this:
“`javascript
if (condition1) {
// code to execute if condition1 is true
} else if (condition2) {
// code to execute if condition2 is true
} else if (condition3) {
// code to execute if condition3 is true
} else {
// code to execute if none of the conditions are true
}
“`
Let’s understand how this shorthand works. The conditions are evaluated sequentially from top to bottom. If the first condition is true, the corresponding code block is executed, and the rest of the ladder is skipped. If the first condition is false, the next condition is evaluated, and so on until a true condition is found, or all conditions have been checked. If none of the conditions are true, the code inside the else block is executed.
Using the if else if shorthand can greatly simplify your code, especially when dealing with numerous conditions. It avoids the need to nest several if-else statements, which can make your code harder to read and debug. Instead, you can neatly organize your conditions in a ladder-like structure, making it easier to understand the logic flow at a glance.
Here’s an example to illustrate its usage:
“`javascript
let age = 25;
if (age < 18) { console.log("You are underage."); } else if (age >= 18 && age < 65) { console.log("You are of working age."); } else { console.log("You are a senior citizen."); } ``` In this example, the code checks the value of the variable `age` and prints a corresponding message based on the condition that matches. If the age is less than 18, it logs "You are underage." If the age is between 18 and 65 (inclusive), it logs "You are of working age." Otherwise, it logs "You are a senior citizen." FAQs: 1. Can I have multiple else if statements in the ladder? Yes, you can have as many else if statements as needed. Each condition will be evaluated in order until a true condition is found, or none of the conditions match. 2. Do I need to include an else block at the end? No, the else block is optional. If you don't include it, nothing will be executed if none of the conditions match. 3. Can I mix if-else statements with the if else if shorthand? Yes, you can mix both forms of statements in your code. However, be cautious about the logic flow and ensure that you maintain a clear and concise structure. 4. Can I use other logical operators and expressions within the conditions? Certainly! You can use various logical operators (e.g., &&, ||) and complex expressions within each condition. This allows you to perform more advanced checks and make your conditions more flexible. In conclusion, the if else if shorthand in JavaScript is a useful tool for handling multiple conditions in a concise and readable manner. By organizing your code into a ladder-like structure, you can easily manage different scenarios without the need for complicated nested if-else statements. Embrace this shorthand technique to simplify your code and improve its maintainability.
Js Shorthand If Without Else
In JavaScript, the `if` statement is a commonly used conditional statement that allows you to execute a block of code based on a specified condition. Often, when using `if`, we also include an `else` statement to define what happens if the condition evaluates to `false`. However, there are scenarios where you may want to write more concise code without explicitly including the `else` statement. In such cases, you can utilize the shorthand `if` syntax in JavaScript. In this article, we will explore the concept of using the shorthand `if` without `else`, its advantages, potential use cases, and some FAQs related to this topic.
## Shorthand `if` Syntax
The shorthand `if` syntax in JavaScript is achieved by using a ternary operator (`?`) along with a colon (`:`). The basic structure of the shorthand `if` statement is as follows:
“`javascript
(condition) ? expression1 : expression2;
“`
Here, `condition` represents the condition you want to evaluate, `expression1` is the value or statement that is executed if the condition is `true`, and `expression2` is the value or statement executed if the condition is `false`. When you omit the `expression2` (i.e., the `else` part), the shorthand `if` statement behaves similar to an `if` statement without an `else` clause.
## Advantages of Shorthand `if`
Using the shorthand `if` without `else` provides a compact and concise way to write conditional statements. It can not only make your code shorter but also improve its readability. By excluding the `else` part, you are explicitly conveying that you do not require any specific action for the `false` case. This can help reduce cognitive load and make the code more focused.
## Use Cases for Shorthand `if` Without `else`
The shorthand `if` without `else` is particularly useful in scenarios where you want to assign a value to a variable based on a condition. Consider the following example:
“`javascript
let price;
const isElectric = true;
if (isElectric) {
price = 1000;
} else {
price = 500;
}
“`
The above code can be rewritten using shorthand `if` as:
“`javascript
const price = (isElectric) ? 1000 : 500;
“`
This concise form is not only easier to read but also ensures fewer lines of code. However, it is important to note that using shorthand `if` without `else` is not always appropriate. It is recommended to use the shorthand only when the `false` action is unnecessary or implicit.
## FAQs
1. Can I nest the shorthand `if` without `else` within another shorthand `if`?
Yes, the shorthand `if` without `else` can be nested within another shorthand `if` to accommodate multiple conditions. For example:
“`javascript
const category = (rating > 4) ? ‘Excellent’ : (rating > 3) ? ‘Good’ : ‘Average’;
“`
2. Is it mandatory to use the shorthand `if` when there is no `else`?
No, it is not mandatory to use the shorthand `if` when there is no `else`. You can still use the traditional `if` statement without `else` for such cases. However, the shorthand `if` offers a more elegant and concise approach.
3. Are there any performance implications with shorthand `if` without `else`?
No, there are no significant performance implications with shorthand `if` without `else`. The JavaScript engine handles the shorthand syntax efficiently, and any potential performance differences are negligible.
4. Can I use shorthand `if` without `else` for complex conditions?
Yes, the shorthand `if` without `else` is flexible enough to handle complex conditions when used in conjunction with logical operators (`&&`, `||`, etc.). However, it is crucial to ensure code maintainability and readability by not overcomplicating the expressions.
## Conclusion
Using the shorthand `if` syntax without an `else` statement provides a concise and elegant way to write conditional expressions in JavaScript. It offers improved readability and can be particularly useful when working with variable assignments based on conditions. However, it should be used judiciously, considering the specific requirements of each scenario. By understanding the concept of shorthand `if` without `else` and its appropriate use cases, you can enhance your JavaScript coding skills and write more succinct code.
Images related to the topic js else if shorthand
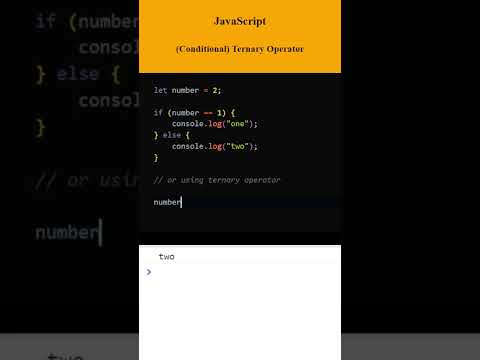
Found 49 images related to js else if shorthand theme


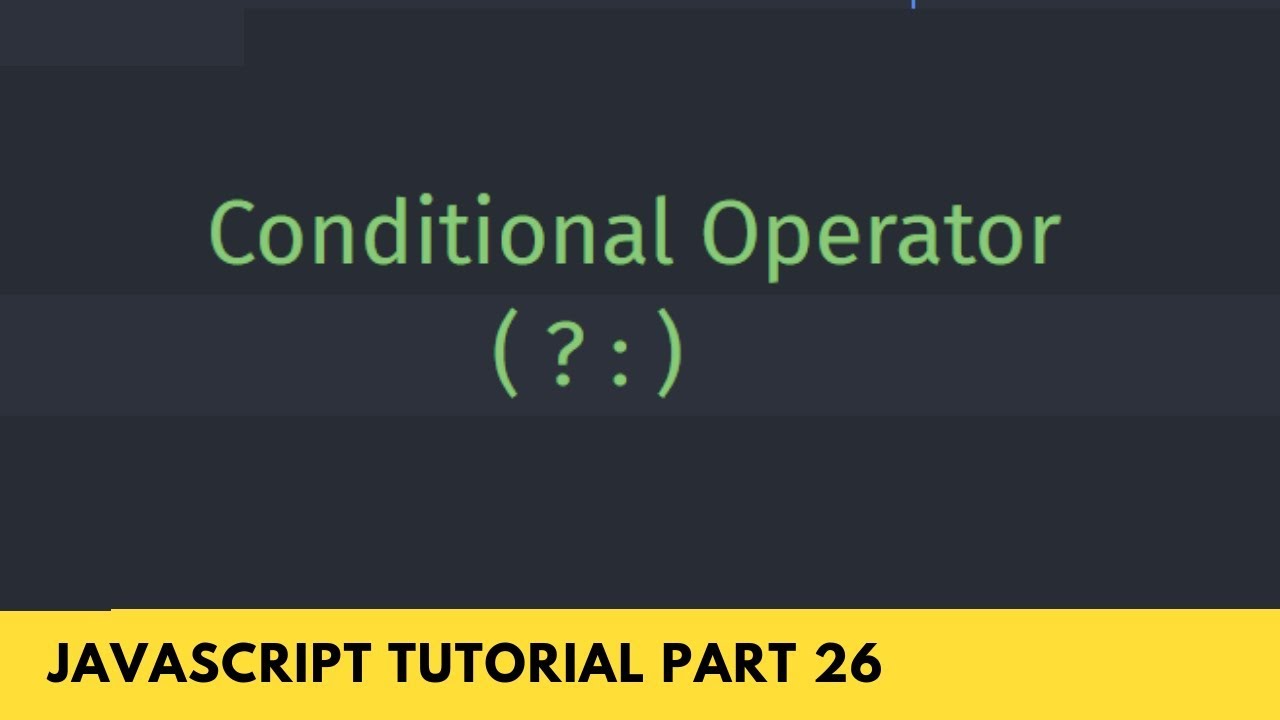

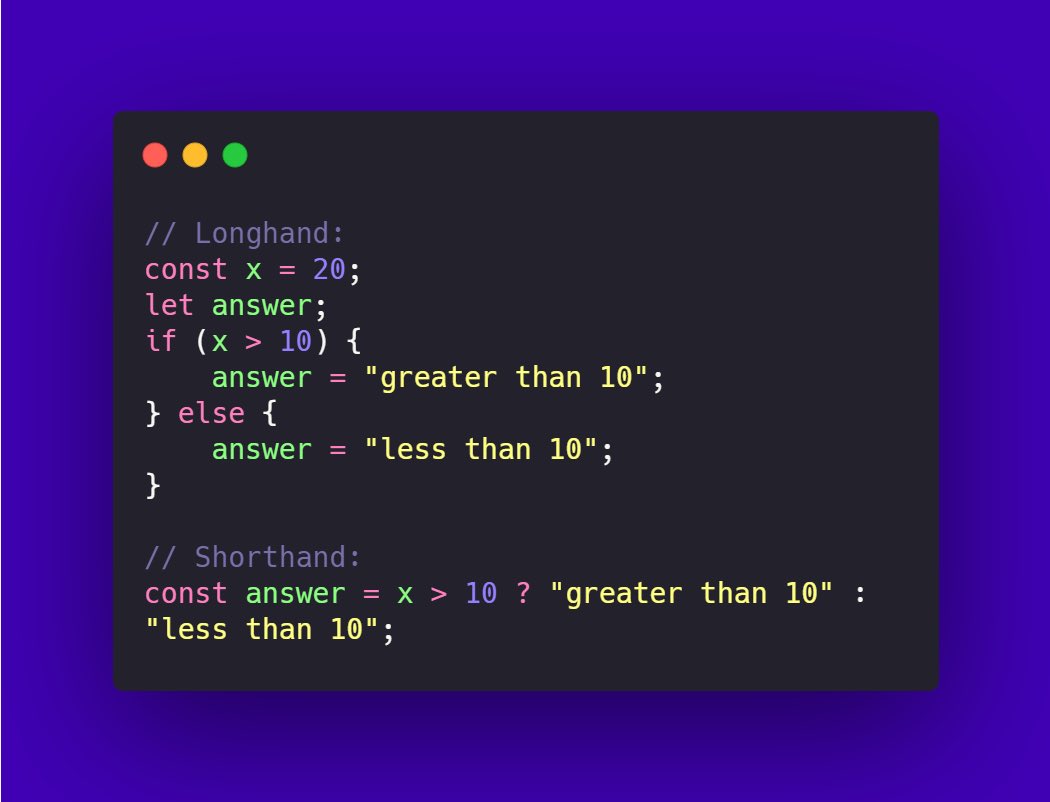
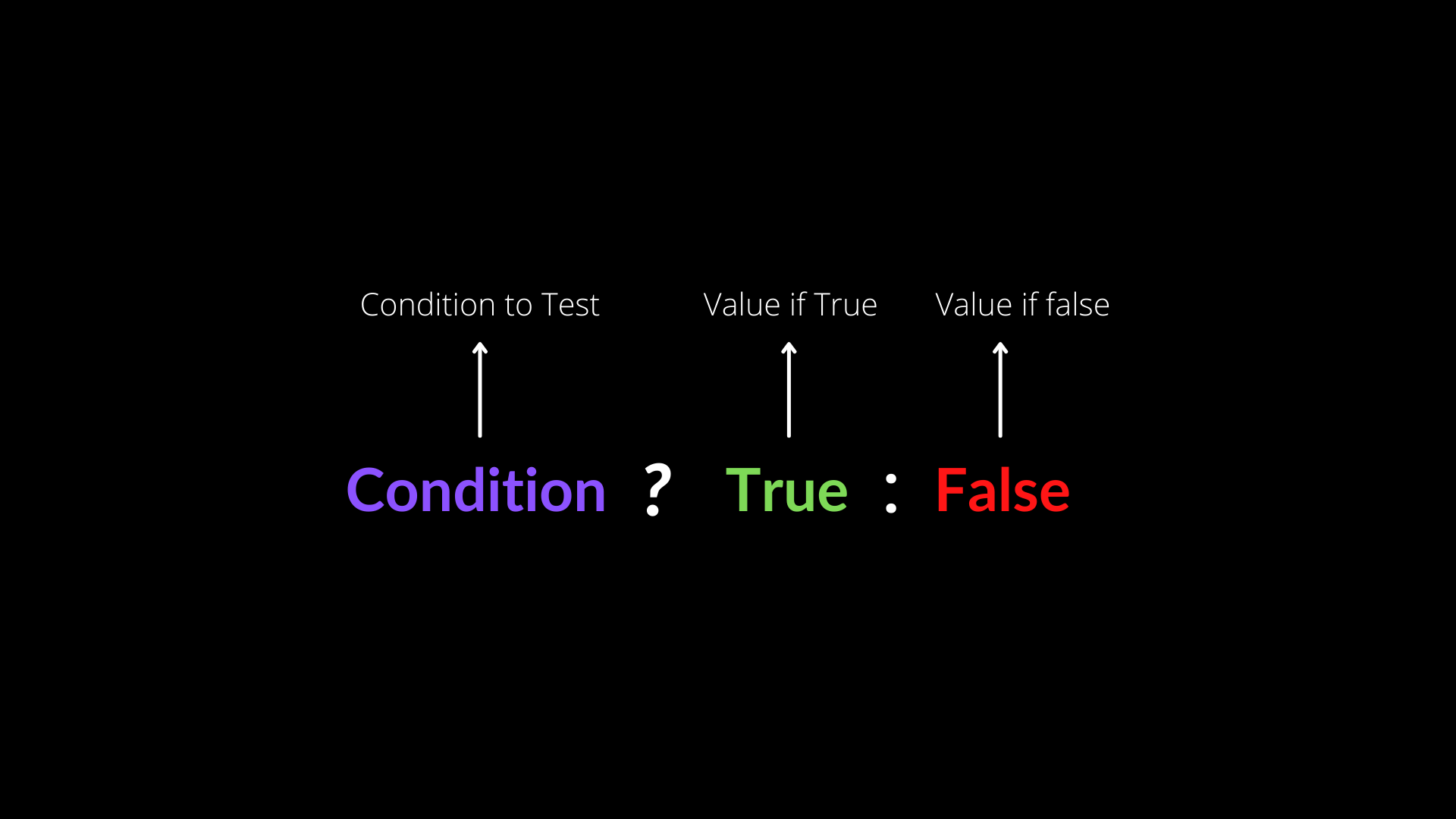
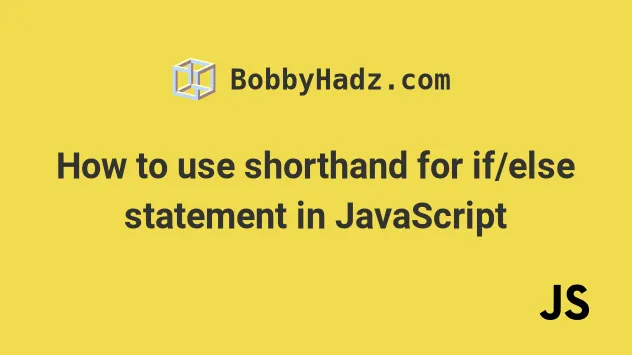
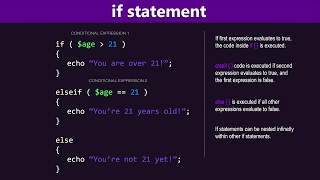
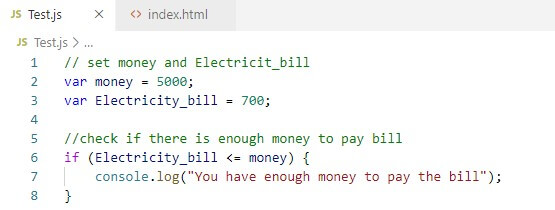
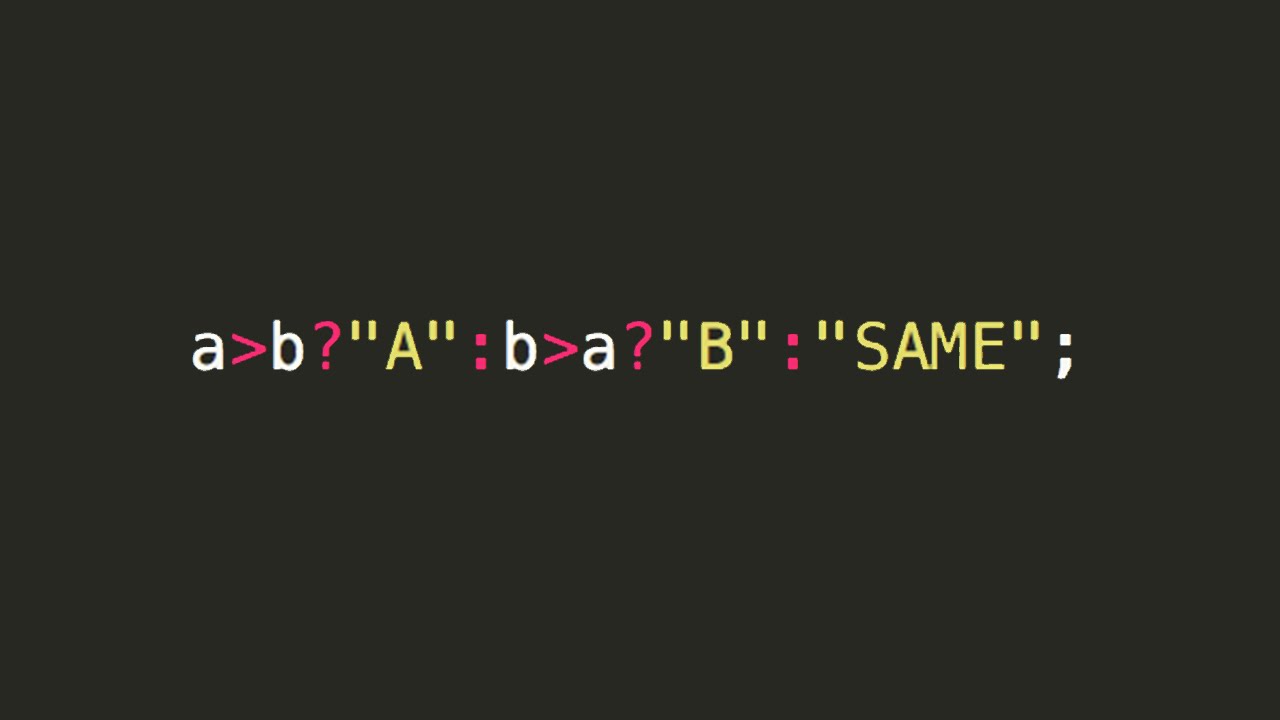
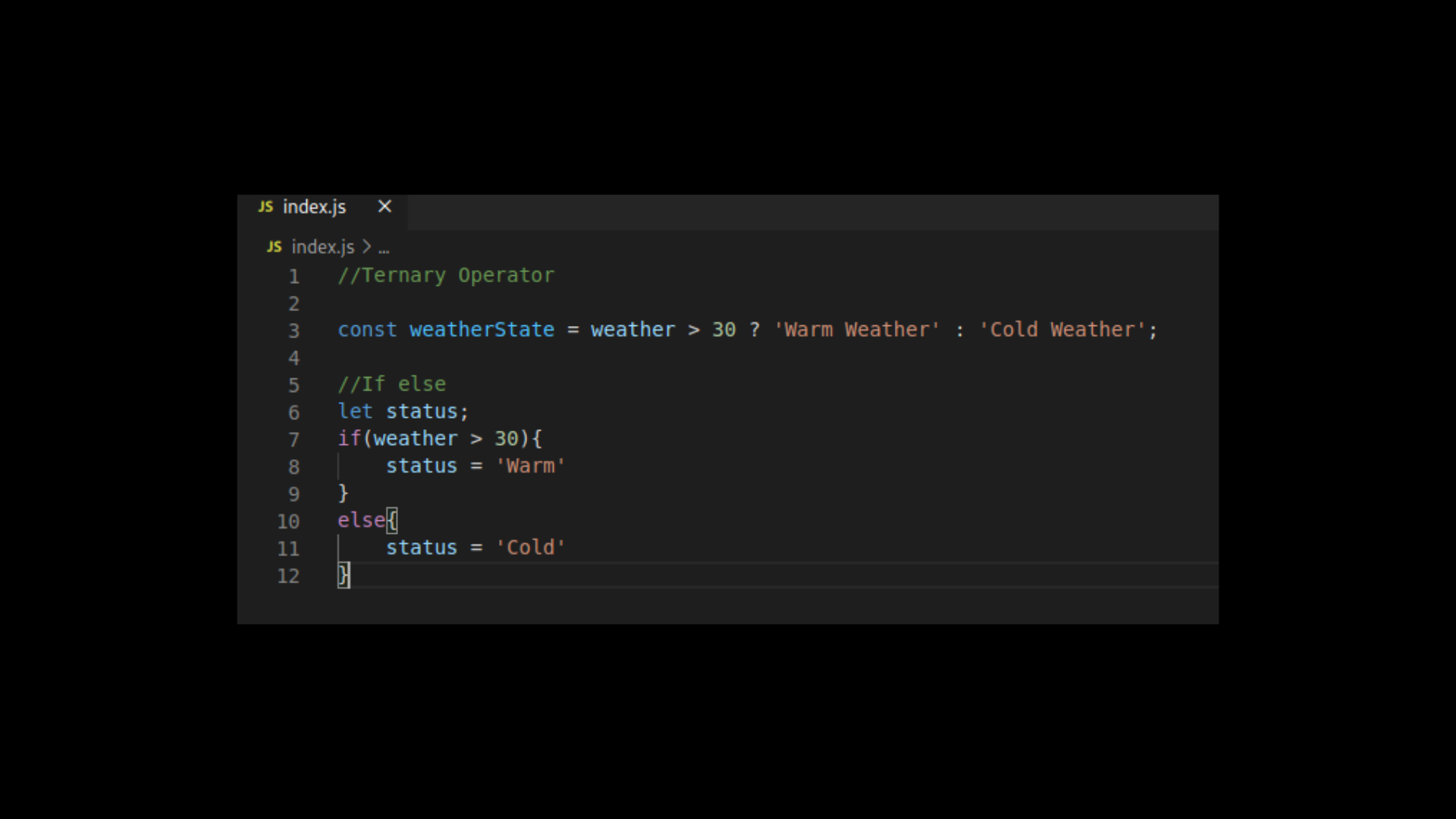

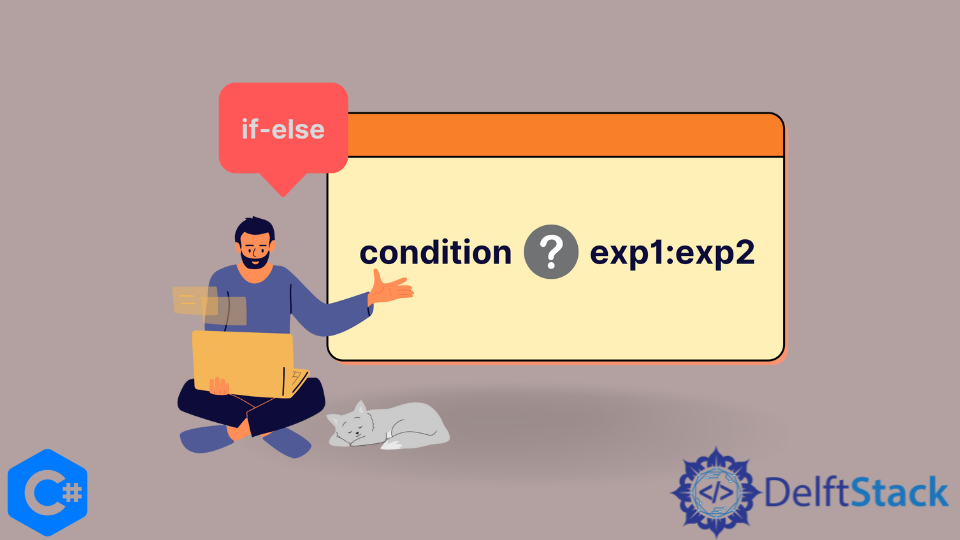


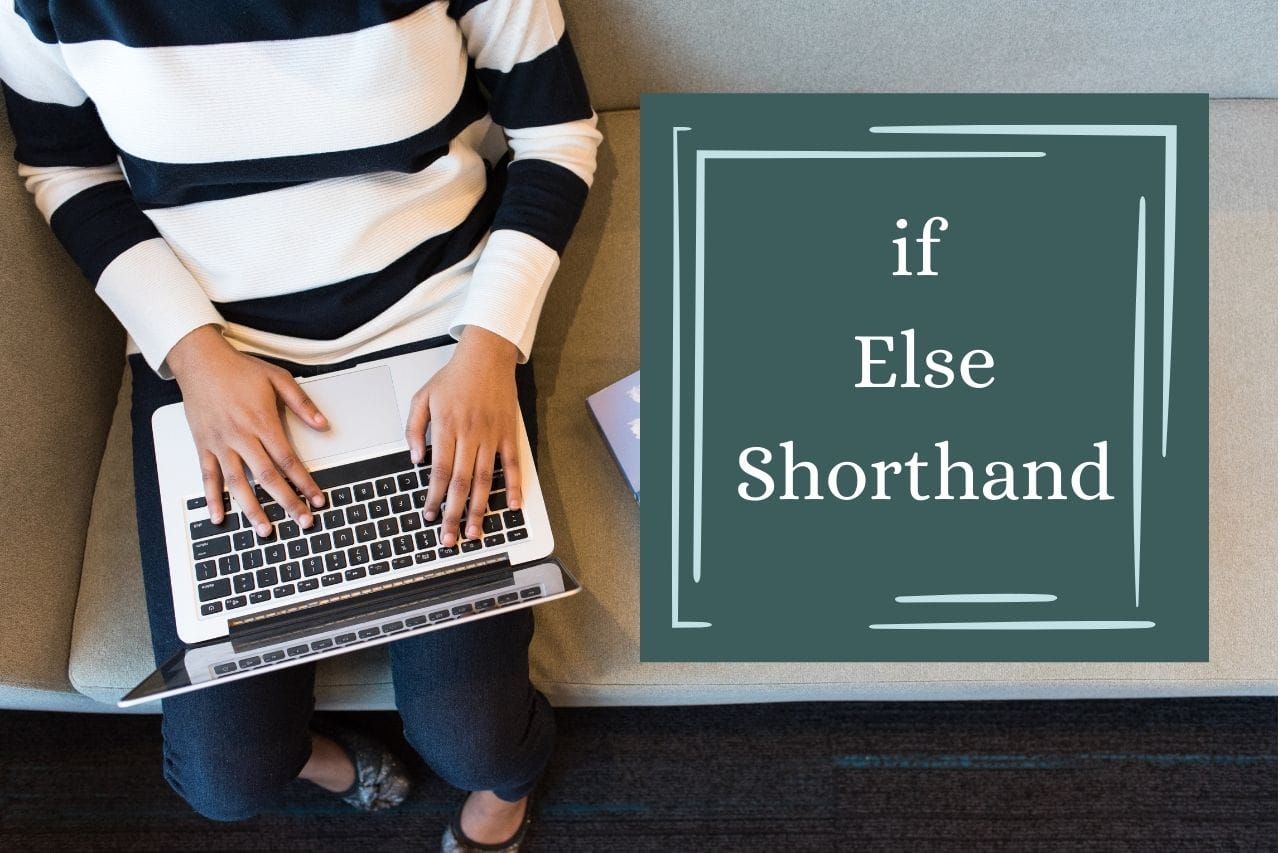


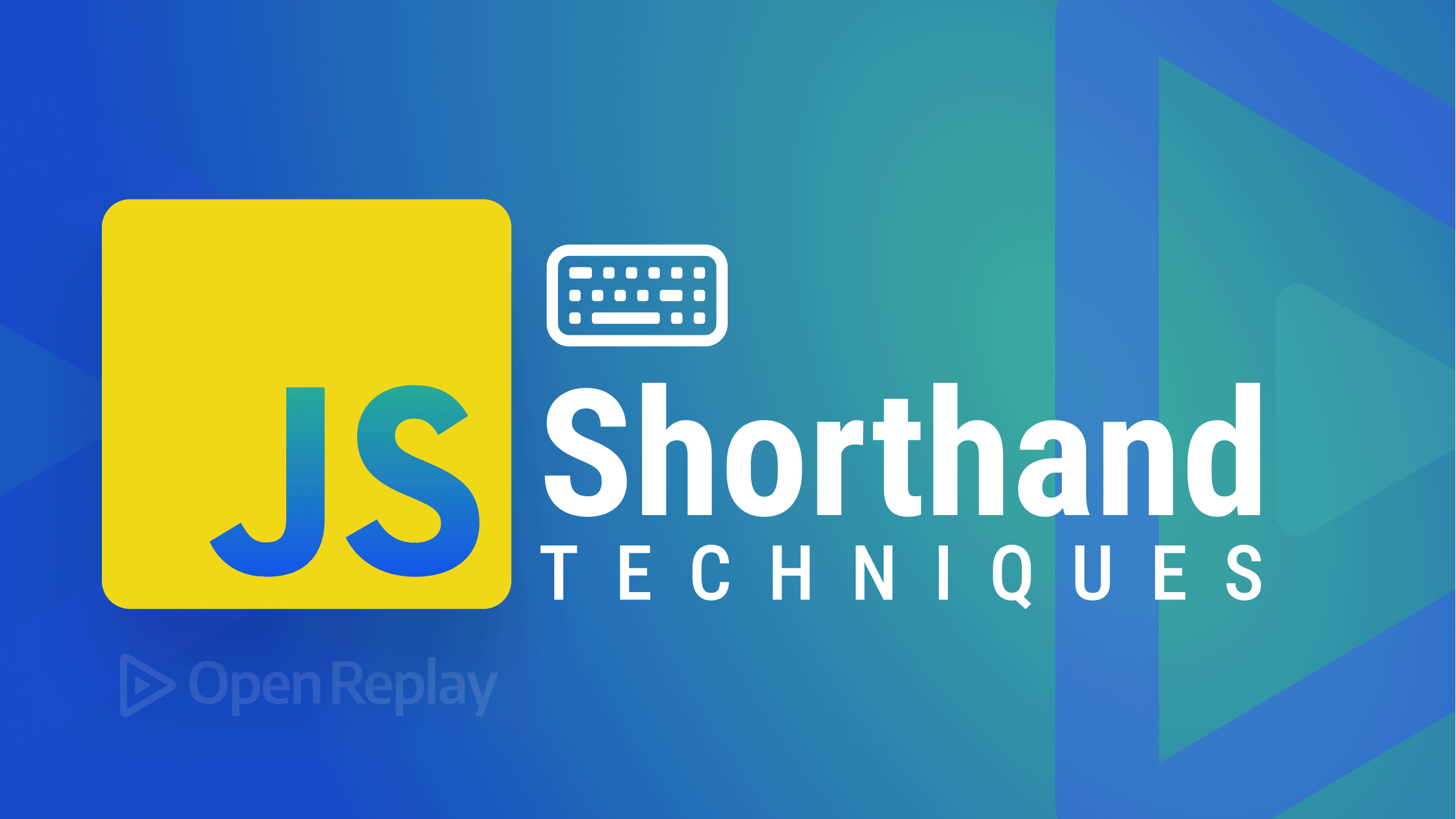
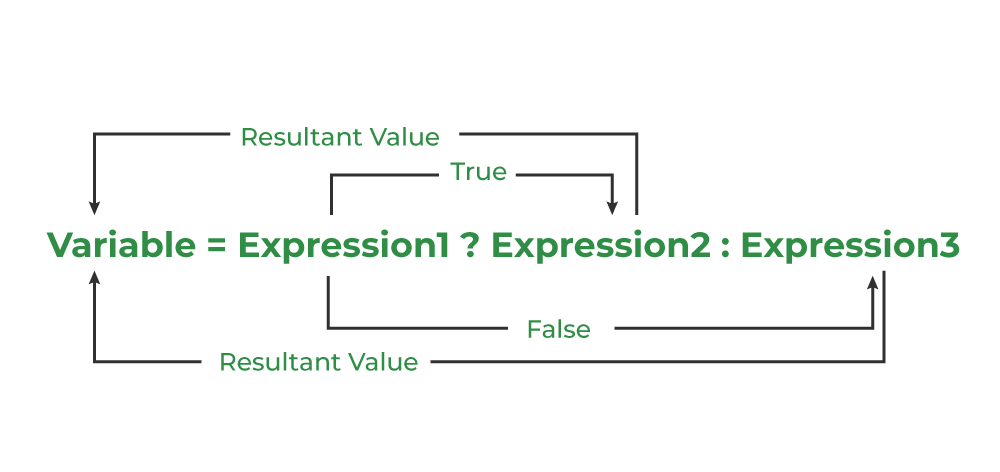
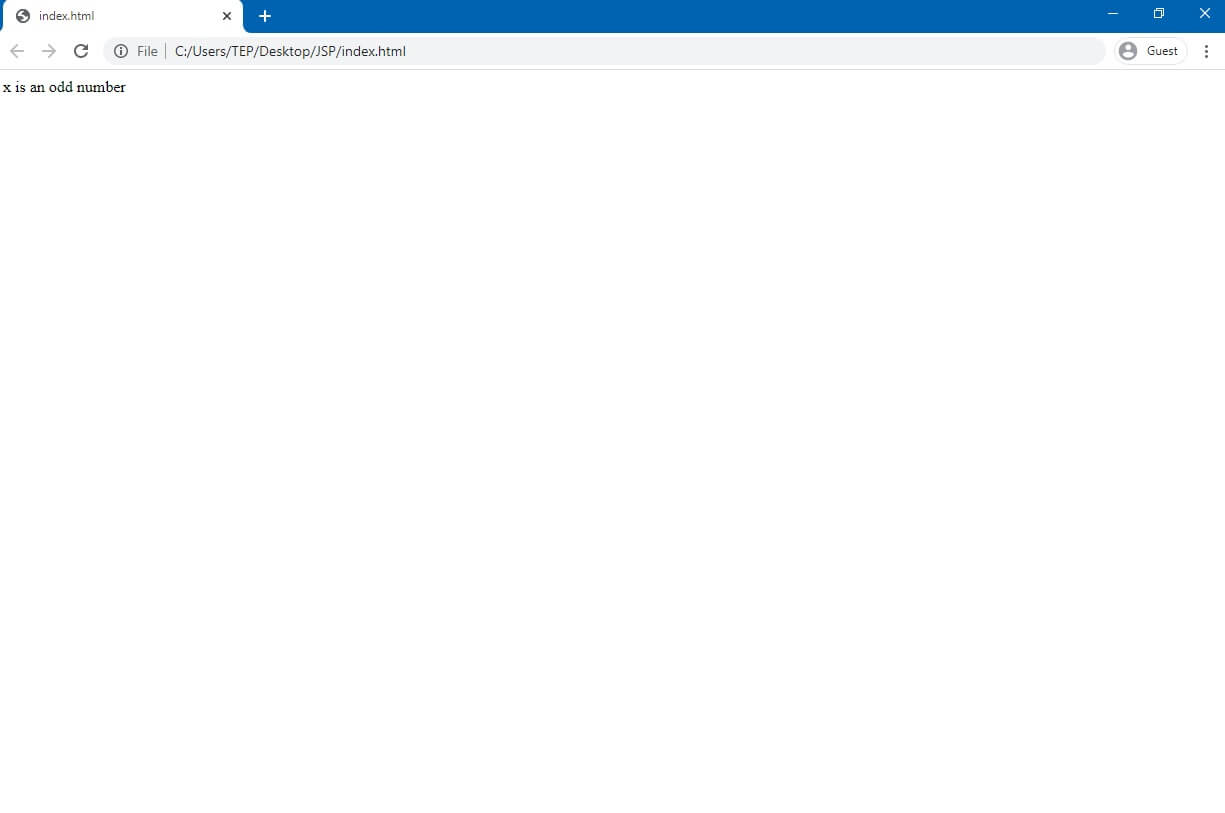
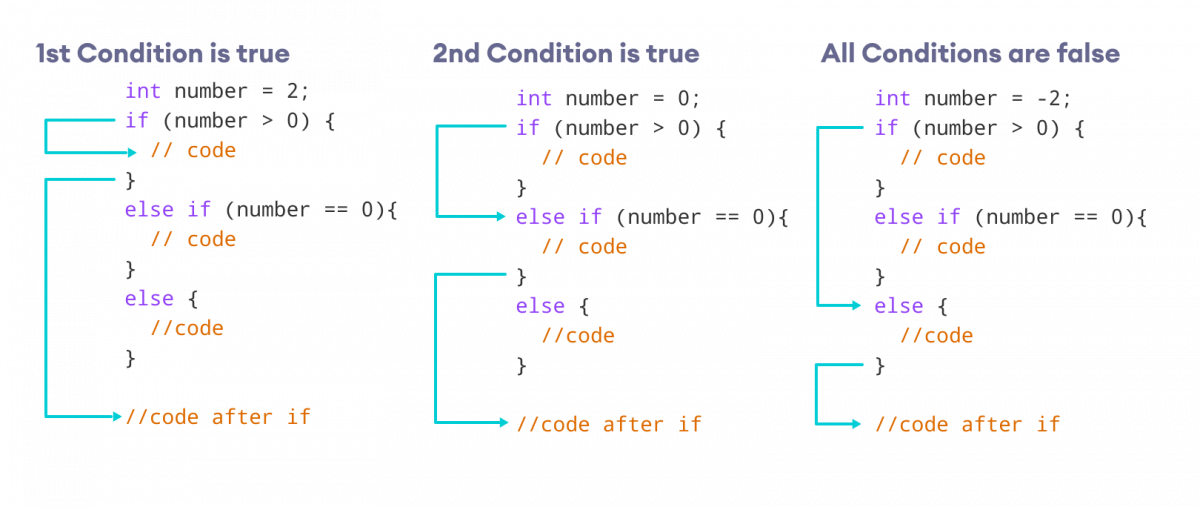
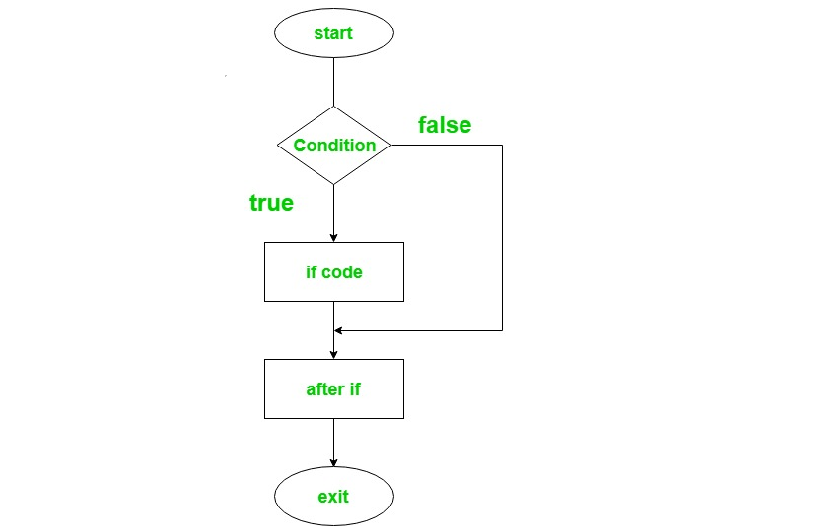
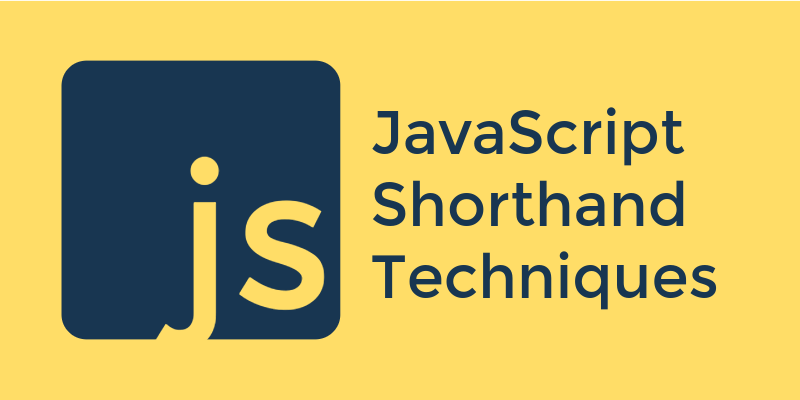
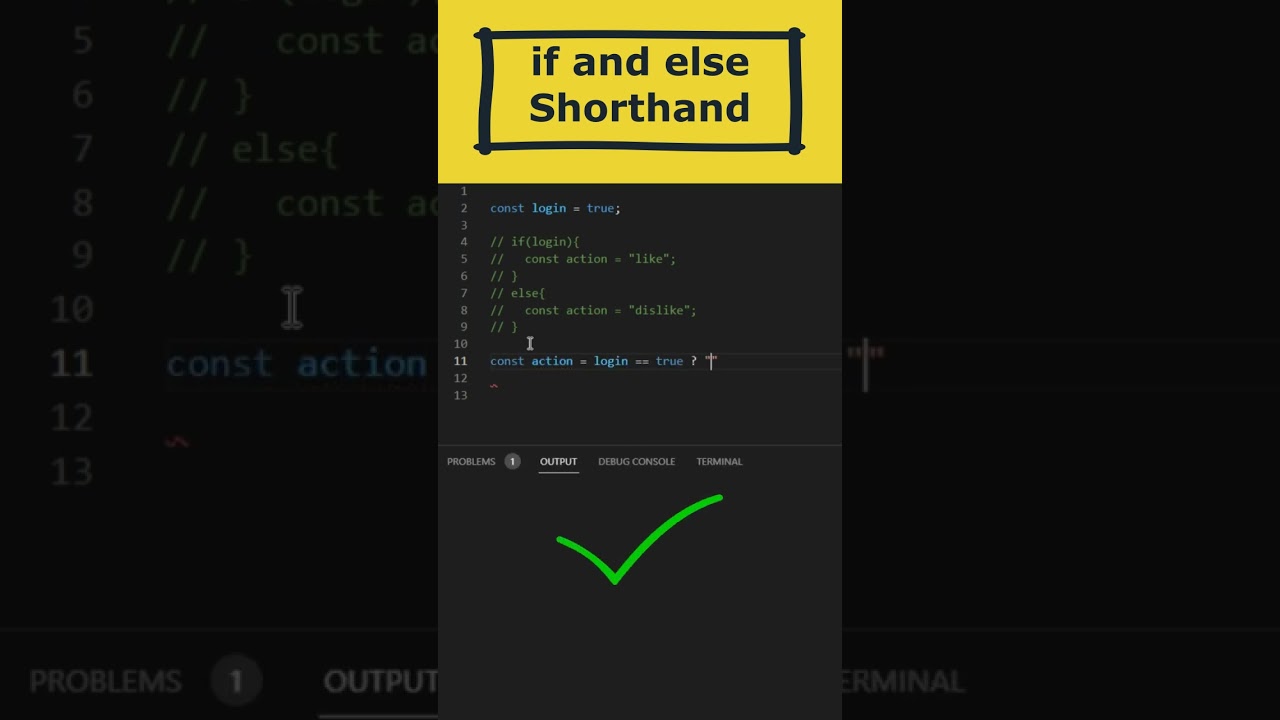


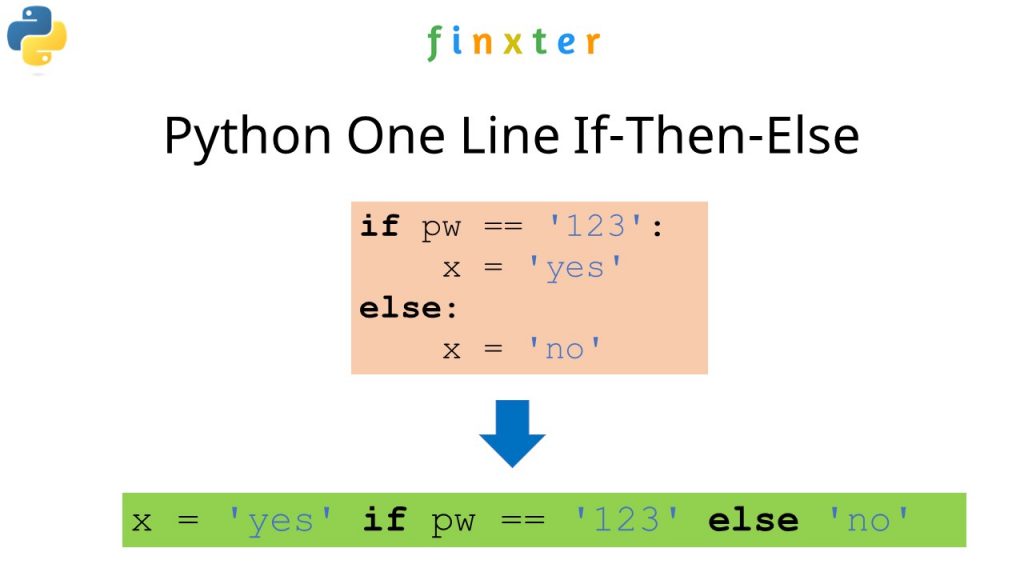


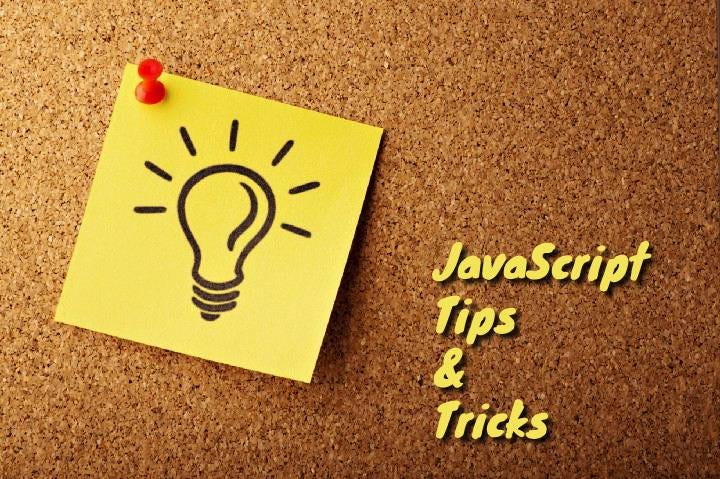
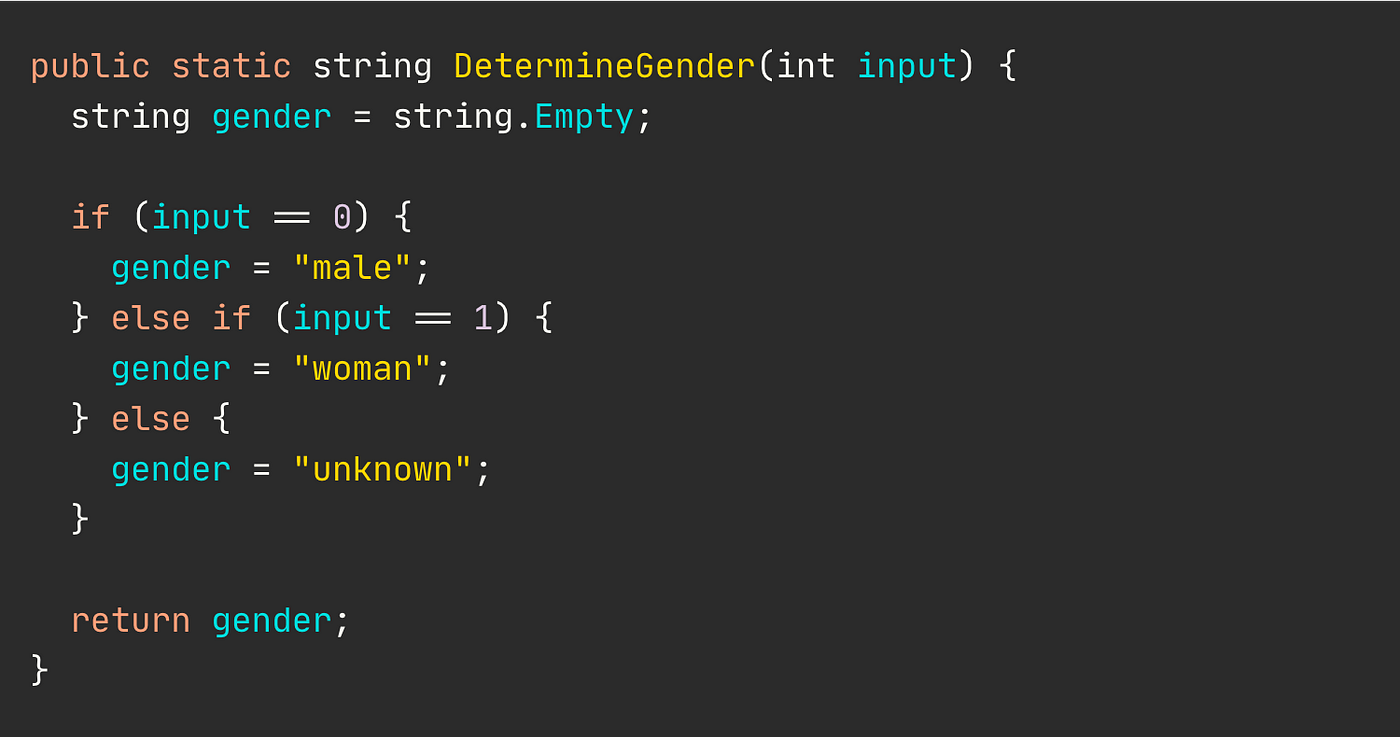

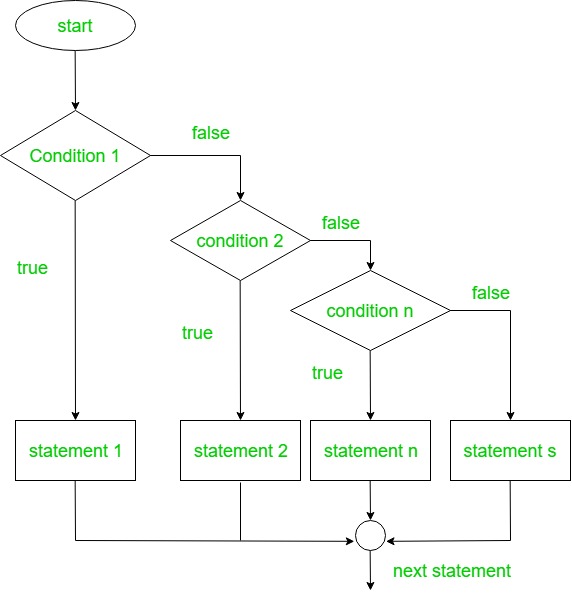
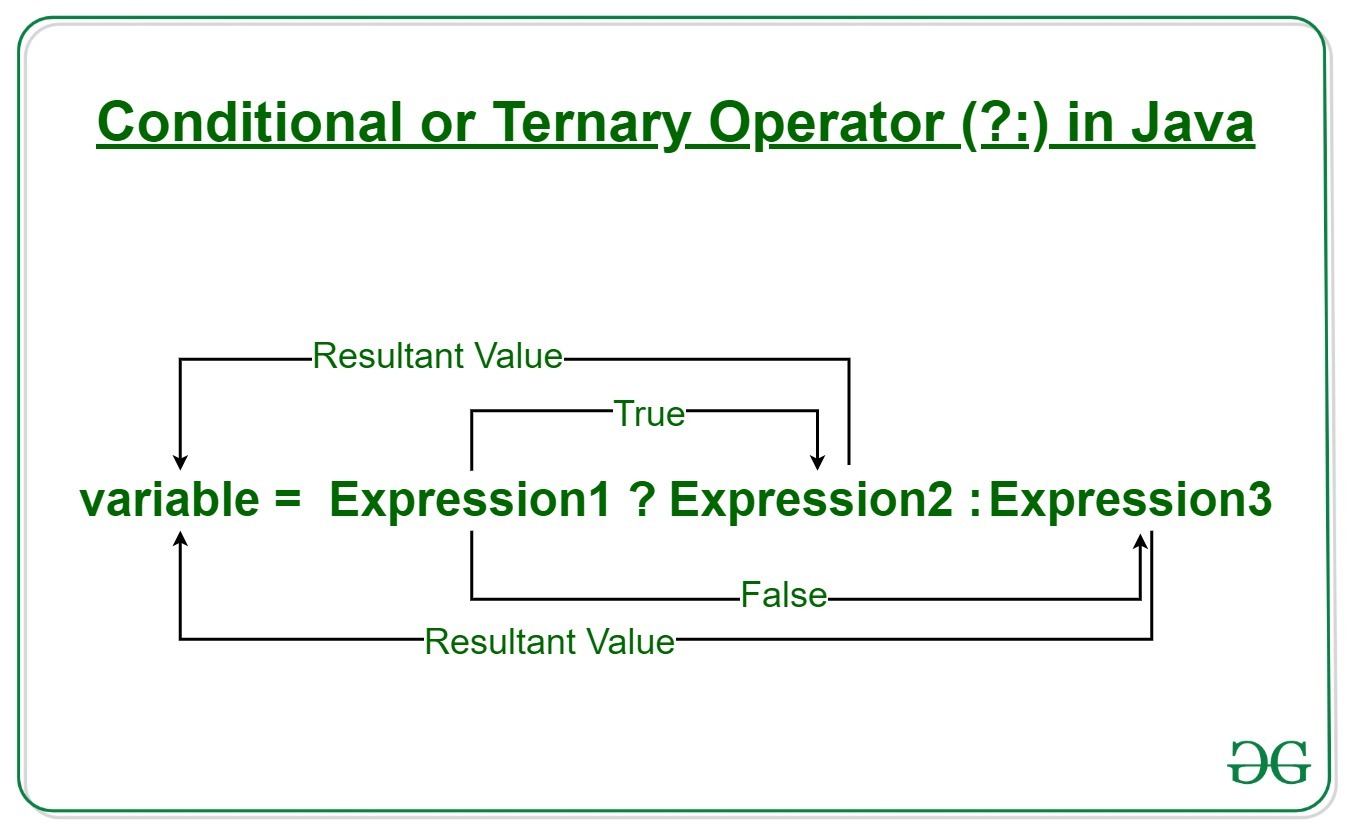
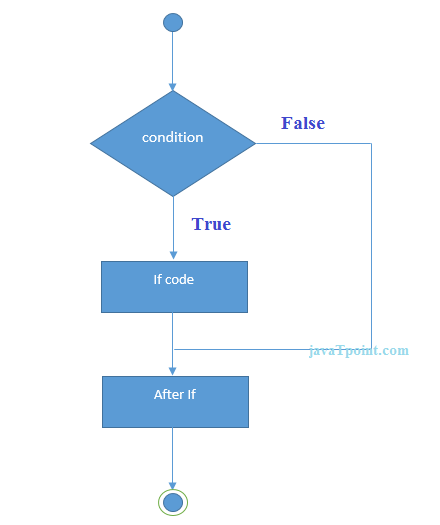



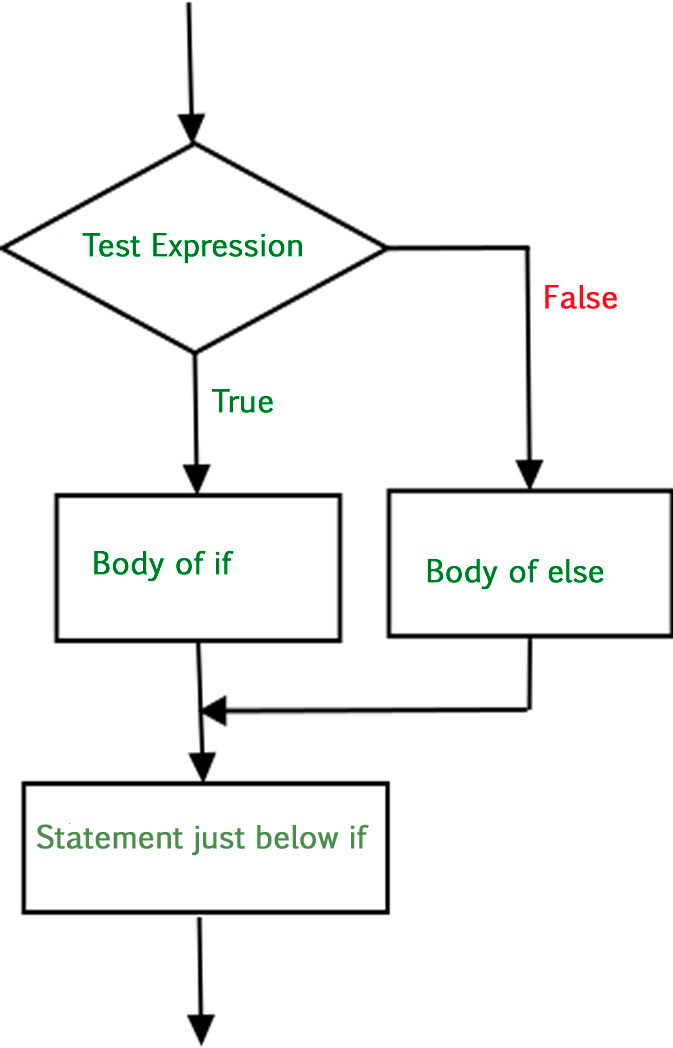

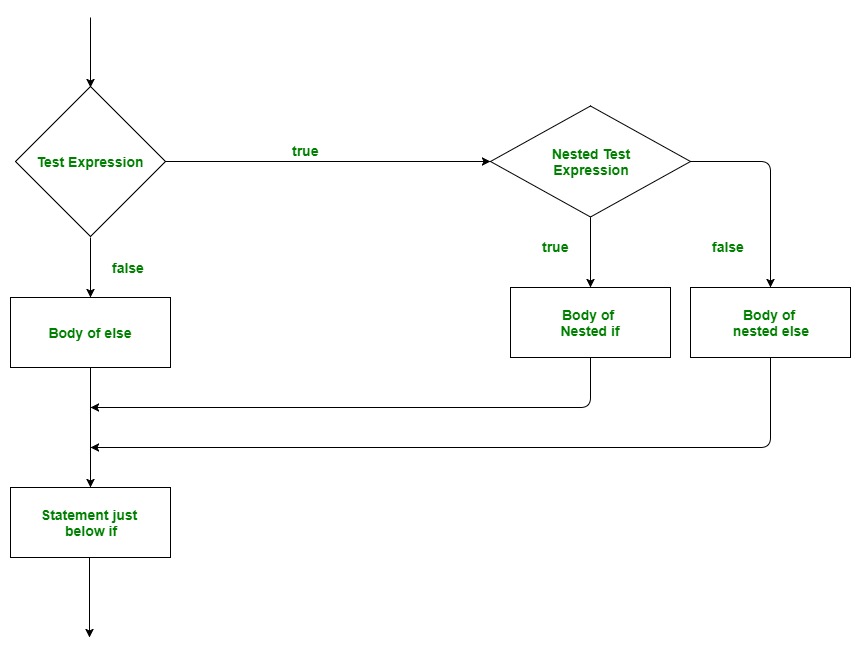
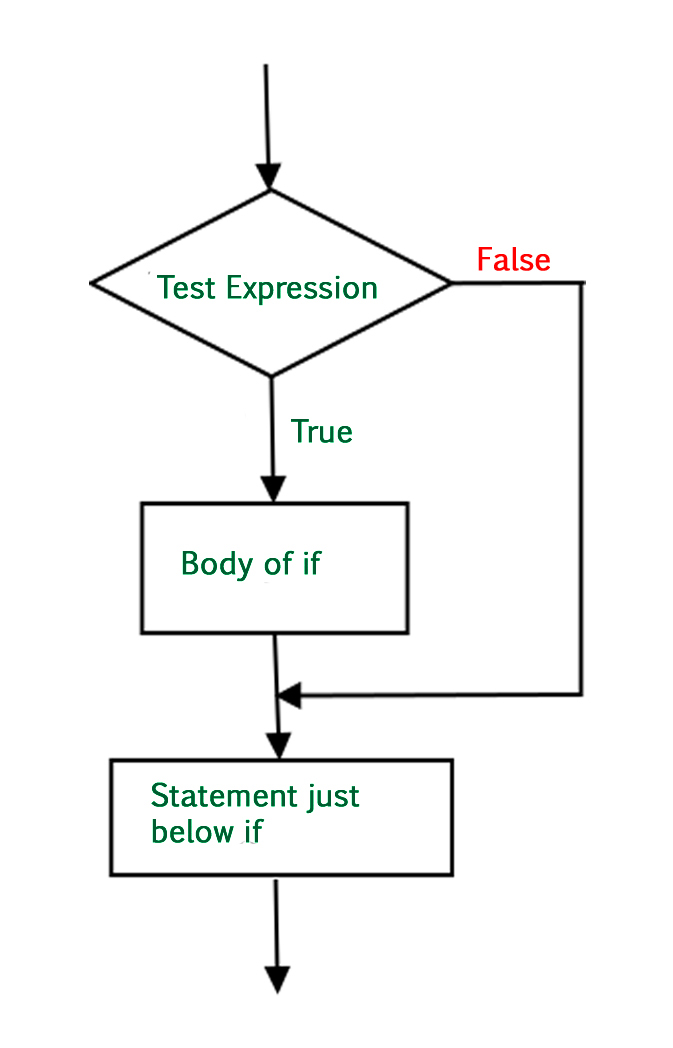


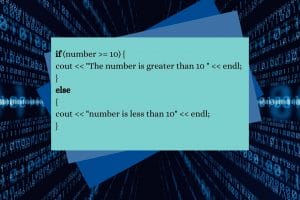
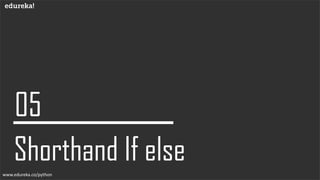
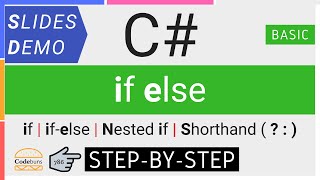
Article link: js else if shorthand.
Learn more about the topic js else if shorthand.
- shortHand if else-if and else statement – Stack Overflow
- How to use shorthand for if/else statement in JavaScript
- Conditional (ternary) operator – JavaScript – MDN Web Docs
- Javascript shorthand for if/else statements (ternary operator …
- JavaScript if else shorthand | Example code
- How To Write Conditional Statements in JavaScript
- JavaScript if shorthand without the else – Daily Dev Tips
- JavaScript If-Else and If-Then – JS Conditional Statements
See more: https://nhanvietluanvan.com/luat-hoc/