Js Heap Out Of Memory
The JavaScript Heap is the memory space used by JavaScript to store objects, variables, and function references. It plays a crucial role in the execution of JavaScript programs by managing memory allocation and deallocation. The heap is responsible for dynamically allocating memory for objects and variables and freeing up memory when it is no longer needed.
Factors leading to Heap Out of Memory
1. Memory Allocation in JavaScript:
JavaScript uses dynamic memory allocation, which means memory is allocated at runtime. This can lead to memory fragmentation and inefficient memory management if not handled properly.
2. Memory Management in JavaScript:
JavaScript relies on automatic garbage collection to free up memory. The garbage collector identifies unused objects and variables and deallocates their memory. However, if the garbage collector is not able to keep up with the rate of memory allocation, it can result in heap out of memory errors.
3. Large Data Structures and Objects:
When large data structures or objects are created in JavaScript, they consume a significant amount of memory. If these structures are not properly managed and deallocated when no longer needed, they can quickly fill up the heap and lead to heap out of memory errors.
Potential Causes of Heap Out of Memory Errors
1. Continuous Allocation without Proper Deallocation:
If objects and variables are continuously allocated without proper deallocation, the heap can become full over time, leading to heap out of memory errors. It is important to release memory resources when they are no longer needed.
2. Recursive Functions and Stack Overflow:
Recursive functions can cause stack overflow errors if the memory allocated for the function calls exceeds the available stack space. This can result in heap out of memory errors as well.
3. Inefficient Garbage Collection:
The garbage collector in JavaScript is responsible for freeing up memory by identifying and deallocating unused objects. If the garbage collector is not able to efficiently identify and collect garbage objects, it can lead to heap out of memory errors.
Handling Heap Out of Memory errors
1. Avoiding Memory Leaks:
Memory leaks occur when objects and variables are not properly deallocated, resulting in gradual memory consumption over time. To avoid memory leaks, ensure that all resources are released when they are no longer needed and avoid unnecessary object references.
2. Optimizing Memory Usage:
Optimizing memory usage involves minimizing memory allocations and releasing memory resources as soon as they are no longer needed. Use data structures and algorithms that are more memory-efficient and avoid unnecessary memory consumption.
3. Breaking Down Large Operations:
If you are dealing with large data structures or performing memory-intensive operations, consider breaking them down into smaller, more manageable chunks. This helps prevent the heap from being overwhelmed by a single large operation.
Tools and techniques to diagnose and troubleshoot Heap Out of Memory errors
1. Memory Profiling:
Memory profiling involves analyzing the memory usage of your JavaScript application to identify memory-intensive areas and potential memory leaks. Tools like Chrome DevTools provide memory profiling features to help diagnose and troubleshoot heap out of memory errors.
2. Heap Snapshots and Analysis:
Heap snapshots capture the current state of the heap, allowing you to analyze the objects and variables present in memory. By analyzing heap snapshots, you can identify memory leaks, inefficient memory usage, and areas of improvement.
3. Use of Performance Monitoring Tools:
Performance monitoring tools can help identify performance bottlenecks and memory usage patterns in your JavaScript application. These tools provide insights into memory usage, CPU usage, and other performance metrics, helping you diagnose and troubleshoot heap out of memory errors.
Best Practices for Preventing Heap Out of Memory errors
1. Managing Memory Usage in Loops:
When working with loops, be mindful of memory allocations within the loop body. Ensure that memory resources are properly managed and deallocated within each iteration to prevent excessive memory consumption.
2. Proper Resource Disposal:
Always release resources when they are no longer needed. This includes closing file handles, disposing of database connections, and removing event listeners. Failing to properly dispose of resources can lead to memory leaks and heap out of memory errors.
3. Regular Performance Monitoring and Optimization:
Regularly monitor the performance of your JavaScript application and optimize it for better memory usage. Use performance monitoring tools to identify areas of improvement and optimize memory usage to prevent heap out of memory errors.
JavaScript heap out of memory errors can be challenging to diagnose and troubleshoot. By understanding the factors leading to heap out of memory, implementing best practices to prevent them, and utilizing tools and techniques for diagnosis and troubleshooting, developers can effectively manage memory usage in their JavaScript applications and prevent heap out of memory errors.
Javascript Heap Out Of Memory Fixed
Keywords searched by users: js heap out of memory JavaScript heap out of memory npm, JavaScript heap out of memory Angular, JavaScript heap out of memory react, ineffective mark-compacts near heap limit allocation failed – javascript heap out of memory, JavaScript heap out of memory nextjs, reached heap limit allocation failed – javascript heap out of memory angular, JavaScript heap out of memory Ubuntu, Committing semi space failed Allocation failed – JavaScript heap out of memory
Categories: Top 49 Js Heap Out Of Memory
See more here: nhanvietluanvan.com
Javascript Heap Out Of Memory Npm
Introduction
When working with npm (Node Package Manager) for JavaScript development, you may come across a common issue known as the “JavaScript heap out of memory” error. This error can cause frustration and hinder the progress of your project. In this article, we will explore the causes behind this error, discuss potential solutions, and answer some frequently asked questions to help you effectively deal with this problem.
Understanding the JavaScript Heap
Before diving into the specifics of the error itself, it is important to understand the concept of the JavaScript heap. The heap is a memory space used by JavaScript to allocate and deallocate objects during runtime. It is where objects and variables are stored temporarily, and memory is managed dynamically.
The Out of Memory Error in npm
When the JavaScript heap is unable to allocate more memory to fulfill your program’s requirements, it leads to the “JavaScript heap out of memory” error. This error occurs primarily due to excessive memory consumption resulting from factors such as:
1. Large-scale data processing or manipulation: When dealing with large datasets, such as handling extensive arrays or processing complex JSON structures, memory usage can spike, pushing the heap to its limits.
2. Infinite loops or recursive functions: Poorly optimized code can result in infinite loops or recursive functions that consume excessive memory, causing the heap to run out of memory.
3. Memory leaks: Memory leaks occur when objects are not properly disposed of or references to objects are not released, leading to unused memory that accumulates over time and may eventually exceed the heap’s capacity.
Solutions for JavaScript Heap Out of Memory Error
Now that we understand the potential causes behind the error, let’s explore some solutions to tackle the “JavaScript heap out of memory” problem in npm:
1. Adjusting memory allocation: The simplest solution is to allocate more memory to the heap by modifying the Node.js command. Adding the “–max-old-space-size” flag followed by a desired memory limit (e.g., “–max-old-space-size=4096”) increases the available heap memory.
2. Optimizing code and reducing memory usage: Refactoring your code to improve efficiency can help reduce memory consumption and prevent heap overflow. Techniques like implementing pagination, using streaming libraries, or optimizing loops and data structures can significantly reduce memory usage.
3. Using stream-based processing: Instead of loading an entire dataset into memory, consider using streaming libraries and techniques to process data incrementally, reducing the memory footprint.
4. Identifying and fixing memory leaks: Using memory profiling tools like Node.js’s built-in “heapdump” module or third-party libraries like “heapdump” or “memwatch-next” can help identify memory leaks. Once you find the leak, fixing it involves properly disposing of objects and releasing references to prevent memory accumulation.
5. Splitting large tasks: For operations involving large datasets or heavy computations, consider splitting the tasks into smaller, manageable chunks. This approach allows the garbage collector to free up memory during smaller intervals, preventing heap exhaustion.
Frequently Asked Questions (FAQs)
1. How can I check the current memory usage of my Node.js application?
You can use the “process.memoryUsage()” method in Node.js to get the current memory usage of your application. It returns an object containing the heap total, heap used, and other memory statistics.
2. I’ve increased the memory limit, but the error still persists. What should I do?
If increasing the memory limit does not solve the issue, double-check your code for potential memory leaks, optimize any resource-intensive operations, and ensure that you are not inadvertently allocating large amounts of memory.
3. Can I allocate unlimited memory to the JavaScript heap?
No, allocating unlimited memory is not recommended. It is best to analyze your code and allocate only the necessary memory to prevent unnecessary memory consumption.
4. Is restarting my application a solution to the out of memory error?
While restarting your application may temporarily alleviate the issue, it is not a permanent solution. Resolve underlying causes such as memory leaks or excessive memory usage to prevent the error from reoccurring.
Conclusion
The “JavaScript heap out of memory” error is a common challenge faced by developers working with npm and JavaScript. By understanding the causes behind this error and implementing the suggested solutions discussed in this article, you can effectively manage and prevent this issue in your projects. Remember to optimize your code, monitor memory usage, and address any memory leaks to ensure smooth execution of your JavaScript applications.
Javascript Heap Out Of Memory Angular
Introduction:
One of the most common challenges faced by developers working with Angular is encountering the “JavaScript heap out of memory” error. This issue can be frustrating and can lead to performance degradation, particularly when dealing with large applications or complex components. In this article, we will dive deep into the causes of this error, discuss its impact on an Angular application, and explore effective solutions to overcome it.
Understanding the JavaScript Heap:
The JavaScript heap is a region in the memory where JavaScript objects are allocated and managed. It is responsible for storing variables, objects, and function calls during the execution of a JavaScript program. In Angular applications, the heap is used extensively to store and manage the application’s components, services, and other runtime data.
Common Causes of JavaScript Heap Out of Memory Error:
1. Memory Leaks:
Memory leaks occur when objects or data are not properly released from the memory, leading to unnecessary memory consumption. In Angular, these leaks can be caused by improper subscription management, circular references, or long-lived objects that are not garbage collected.
2. Inefficient Data Handling:
Poorly optimized code that handles large data sets can quickly exhaust the JavaScript heap, particularly when performing operations like sorting, filtering, or mapping on arrays with thousands of elements.
3. Recursive Functions:
Recursive functions, if not implemented correctly or with proper exit conditions, can cause the heap to run out of memory. These function calls stack up and consume excessive memory resources.
Impact of JavaScript Heap Out of Memory Error on an Angular Application:
When the JavaScript heap runs out of memory, it can result in a wide range of issues, including:
1. Slow Application Performance:
The application may become sluggish, unresponsive, or experience significant response delays. This can have a negative impact on user experience and overall satisfaction.
2. Crashes and Unhandled Exceptions:
Out of memory errors can lead to unexpected crashes or unhandled exceptions, resulting in the application abruptly terminating or showing error messages to users.
3. Frequent Garbage Collection:
When the heap is under pressure, the garbage collector starts working more frequently to free up memory. This excessive garbage collection can cause the application to pause intermittently, known as “hiccups,” leading to a jarring user experience.
Solutions to Resolve JavaScript Heap Out of Memory Error in Angular:
1. Optimize Memory Consumption:
Ensure efficient memory usage by identifying and fixing memory leaks. Review the codebase and verify that subscriptions are properly managed, resources are released when no longer needed, and circular references are avoided. Use tools like the Angular DevTools or Chrome DevTools to detect memory leaks and optimize memory consumption.
2. Employ Lazy Loading and Code Splitting:
Angular allows lazy loading and code splitting, which load only the required components or modules when needed. This helps minimize the initial memory footprint of the application, alleviating the pressure on the JavaScript heap.
3. Implement Pagination or Virtual Scrolling:
When working with large datasets, consider implementing pagination or virtual scrolling to load and display data incrementally. This prevents loading the entire dataset at once, reducing memory usage and improving performance.
4. Optimize Recursive Functions:
Review and optimize recursive functions to ensure they have proper exit conditions and avoid unnecessary memory consumption. Tail recursion techniques can be employed to avoid stack buildup and optimize memory usage.
5. Increase Heap Size:
If the application requires more memory to function properly, the default JavaScript heap size can be increased. This can be done by specifying the maximum heap size using command-line arguments or environment variables. However, note that this solution should be used with caution, as increasing the heap size may lead to other issues if the underlying cause of the out of memory error is not addressed.
FAQs:
Q1. How can I check if my Angular application is suffering from a heap out of memory issue?
A. You can monitor memory consumption using browser developer tools such as Chrome DevTools. Look for excessive memory usage or frequent garbage collection pauses.
Q2. How can I detect memory leaks in my Angular application?
A. Tools like Angular DevTools or Chrome DevTools’ memory profiling feature can help identify memory leaks by tracking object allocations and deallocations.
Q3. Are there any best practices to prevent memory leaks in Angular?
A. Yes, some best practices include properly unsubscribing from subscriptions, avoiding circular references, using ngOnDestroy to release resources, and handling long-lived objects judiciously.
Q4. Is it recommended to manually adjust the heap size for an Angular application?
A. Manually adjusting the heap size should be seen as a last resort. It is highly recommended to optimize the application code and resolve memory leaks before considering increasing the heap size.
Conclusion:
The “JavaScript heap out of memory” error can significantly affect the performance of an Angular application. By understanding the causes and implementing appropriate solutions, developers can mitigate this issue. Optimizing memory consumption, lazy loading, employing pagination, and optimizing recursive functions are just a few techniques to consider when tackling this error. By following best practices and utilizing proper monitoring and profiling tools, developers can ensure optimal memory utilization and deliver high-performing Angular applications.
Javascript Heap Out Of Memory React
React is a popular JavaScript library used for building user interfaces. It allows developers to create reusable UI components and efficiently update and render them as the application’s state changes. Despite its numerous benefits, React applications can sometimes encounter a common issue known as “JavaScript heap out of memory.” In this article, we will explore what this error means, its causes, and potential solutions, providing comprehensive insights into this topic.
Understanding JavaScript Heap Out of Memory
In JavaScript, the heap is a region of memory where objects are allocated and deallocated. When a React application runs, it uses the JavaScript heap to store all the objects used in the application, including variables, arrays, and objects.
However, the JavaScript heap has a limited size, which can be a problem when dealing with large applications or handling memory-intensive operations. When the heap runs out of memory, it results in a “JavaScript heap out of memory” error. This error signifies that the allocated heap size is insufficient to accommodate all the objects required by the application, leading to performance issues or even crashes.
Causes of JavaScript Heap Out of Memory in React
The JavaScript heap out of memory error in React can be caused by several factors. Some common causes include:
1. Memory Leaks: Memory leaks occur when objects are not properly released and continue to occupy memory even when they are no longer needed. In React, this can happen when components are not unmounted correctly or when references to objects are not properly removed. Over time, these memory leaks accumulate and consume a significant amount of memory, eventually leading to an out of memory error.
2. Large Object Sizes: React applications that handle massive amounts of data or render complex UI components may require larger object sizes in the heap. If the heap size is not adjusted accordingly, it can quickly exhaust the available memory and trigger the out of memory error.
3. Recursive Calls: Recursive calls, where a function repeatedly calls itself, can also result in the JavaScript heap running out of memory. If the recursion is not correctly controlled or terminated, it can create a stack overflow, consuming excessive memory and ultimately causing the error.
Solutions to JavaScript Heap Out of Memory Errors
To address JavaScript heap out of memory errors in a React application, several solutions can be explored:
1. Increase Heap Memory: One solution is to increase the heap memory available to the application. This can be done by setting a higher limit for the JavaScript heap size using the “–max-old-space-size” flag when running the application. For example:
“`
node –max-old-space-size=4096
“`
This increases the heap size to 4GB. However, it is important to note that excessively increasing heap size can lead to other performance issues, such as slower garbage collection. Therefore, it is crucial to find the right balance.
2. Optimize Component Unmounting: Properly managing component unmounting is essential to prevent memory leaks. Ensure that components are correctly unmounted when no longer needed by using lifecycle methods such as componentWillUnmount(). This ensures that unused objects are released from memory, preventing unnecessary memory consumption.
3. Memory Management Techniques: Implementing memory management techniques can help reduce memory consumption. For instance, using libraries like React’s useMemo() or useCallback() can memoize variables or functions, reducing unnecessary re-computations and optimizing memory usage.
4. Chunking Large Objects: When dealing with large objects, break them down into smaller chunks or load them lazily. By dividing the data or UI components into manageable pieces, you can reduce the memory footprint and prevent out of memory errors.
5. Limit Recursive Calls: If your application relies on recursion, make sure to control the depth and conditions of recursion. Set proper termination conditions to avoid infinite loops, which can rapidly exhaust memory. You can also consider converting recursive algorithms into iterative ones, reducing memory consumption.
FAQs
1. How can I check the current heap memory size in a React application?
You can use the performance.memory API provided by modern browsers. Access the memory object using the window.performance.memory object and inspect its properties. The “usedJSHeapSize” property indicates the current heap memory usage.
2. Can I completely avoid JavaScript heap out of memory errors?
While it is challenging to completely eliminate the possibility of such errors, following memory management best practices and optimizing your application’s codebase can significantly reduce the occurrences of heap memory issues.
3. Are there any tools available to debug memory leaks in React?
Yes, there are various tools available that can help identify and troubleshoot memory leaks in React applications. Some popular tools include Chrome DevTools, React’s Profiler API, and third-party tools like React Developer Tools or HeapSnapShot.
4. Is garbage collection affected by increasing the JavaScript heap size?
Garbage collection can be slower with larger heap sizes. As the heap size increases, garbage collection takes more time to traverse and mark objects that are no longer needed. It is important to find a balance between heap size and garbage collection efficiency.
Conclusion
JavaScript heap out of memory errors in React can be a challenging issue to tackle. By understanding the causes and implementing appropriate solutions, developers can optimize their React applications to reduce memory consumption and prevent out of memory errors. Applying strategies such as increasing heap memory, optimizing component unmounting, and implementing memory management techniques can ensure a more stable and performant React application. Remember to closely monitor memory usage and leverage debugging tools to identify and resolve memory-related issues efficiently.
Images related to the topic js heap out of memory
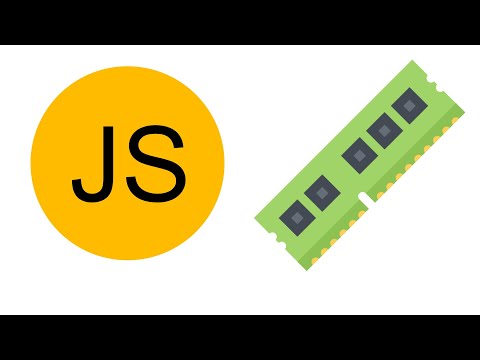
Found 31 images related to js heap out of memory theme

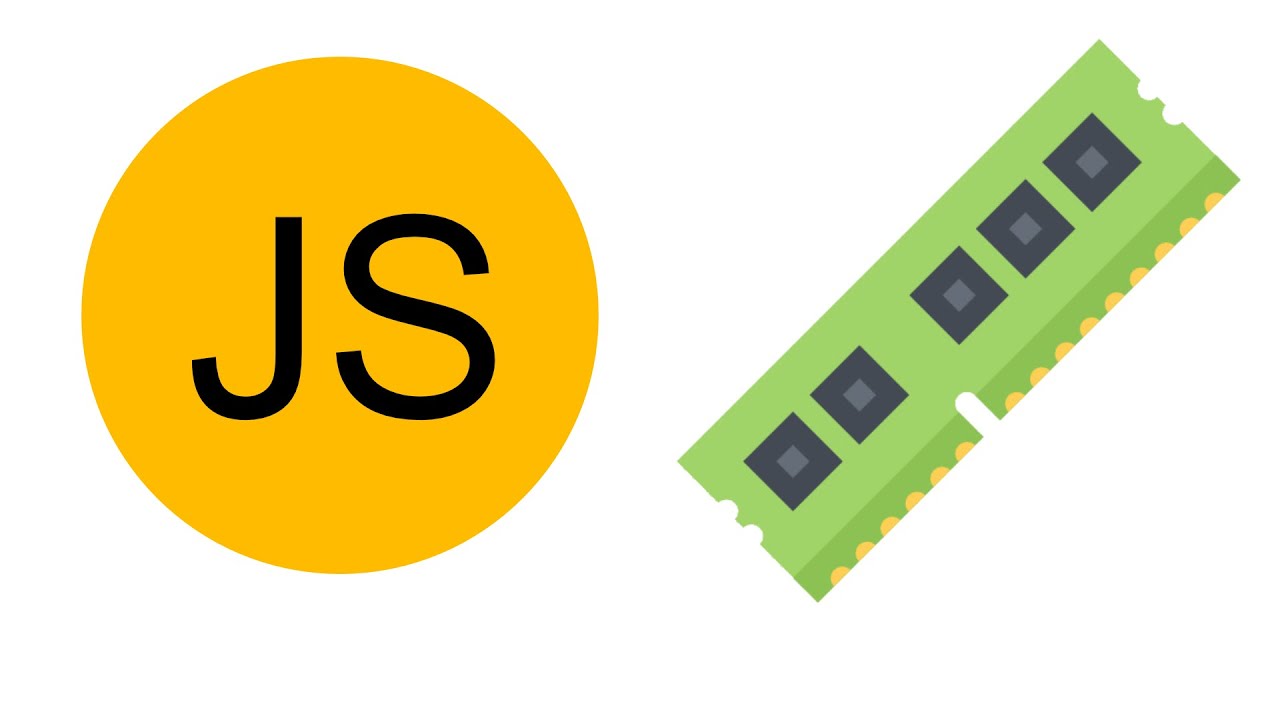
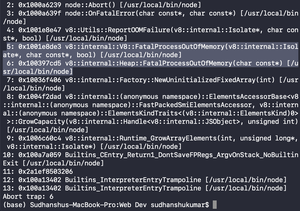

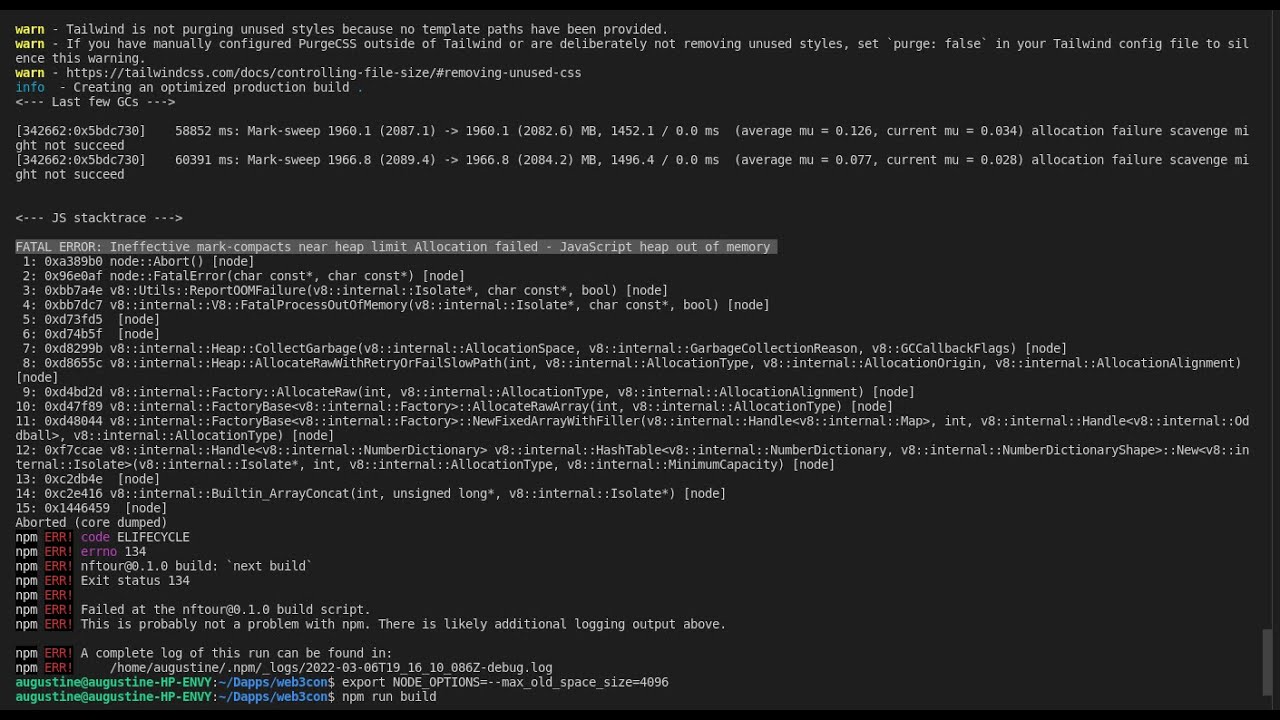


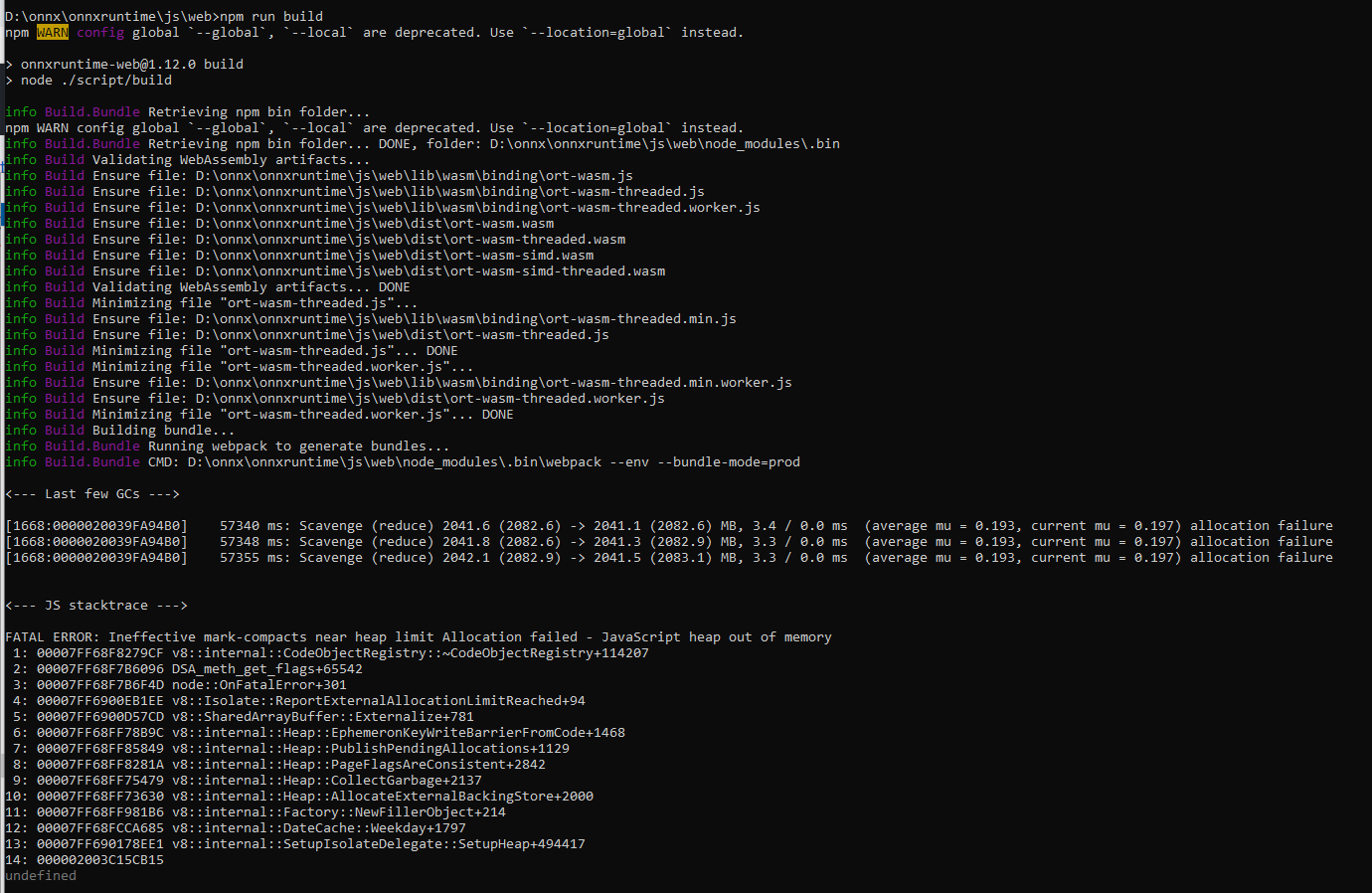
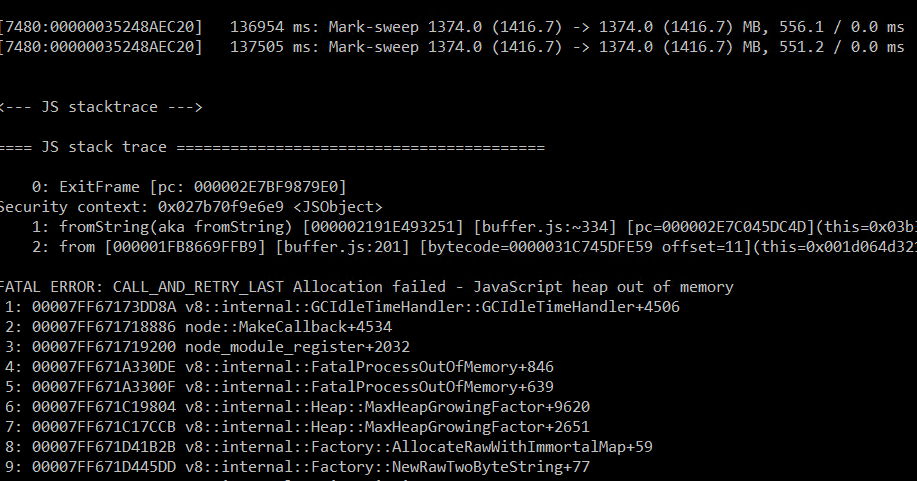




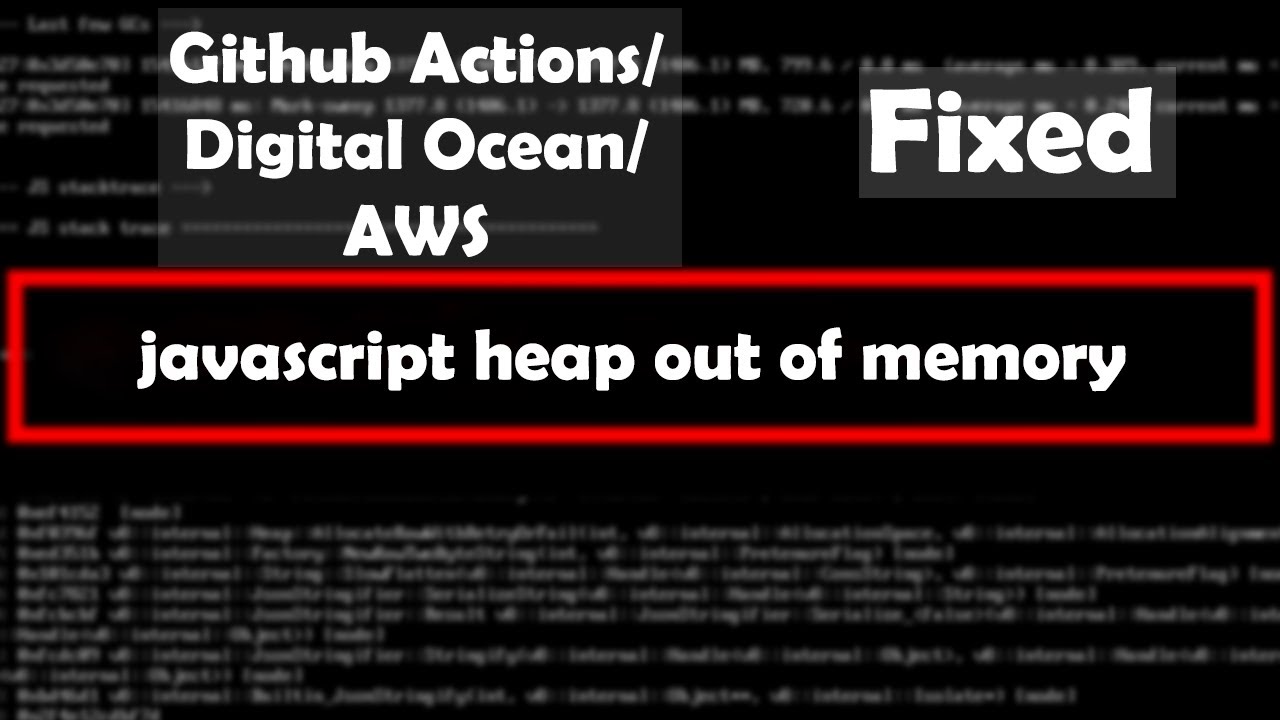


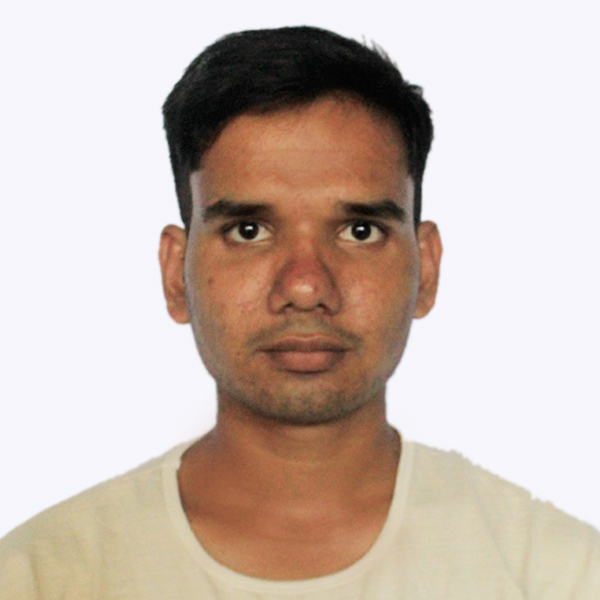

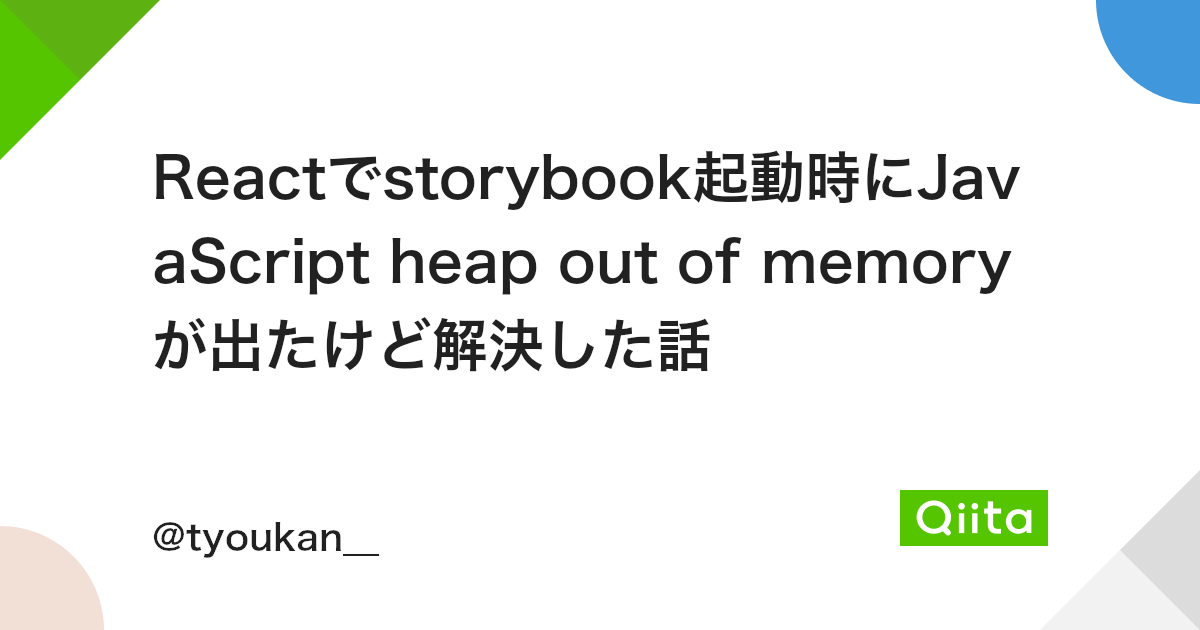

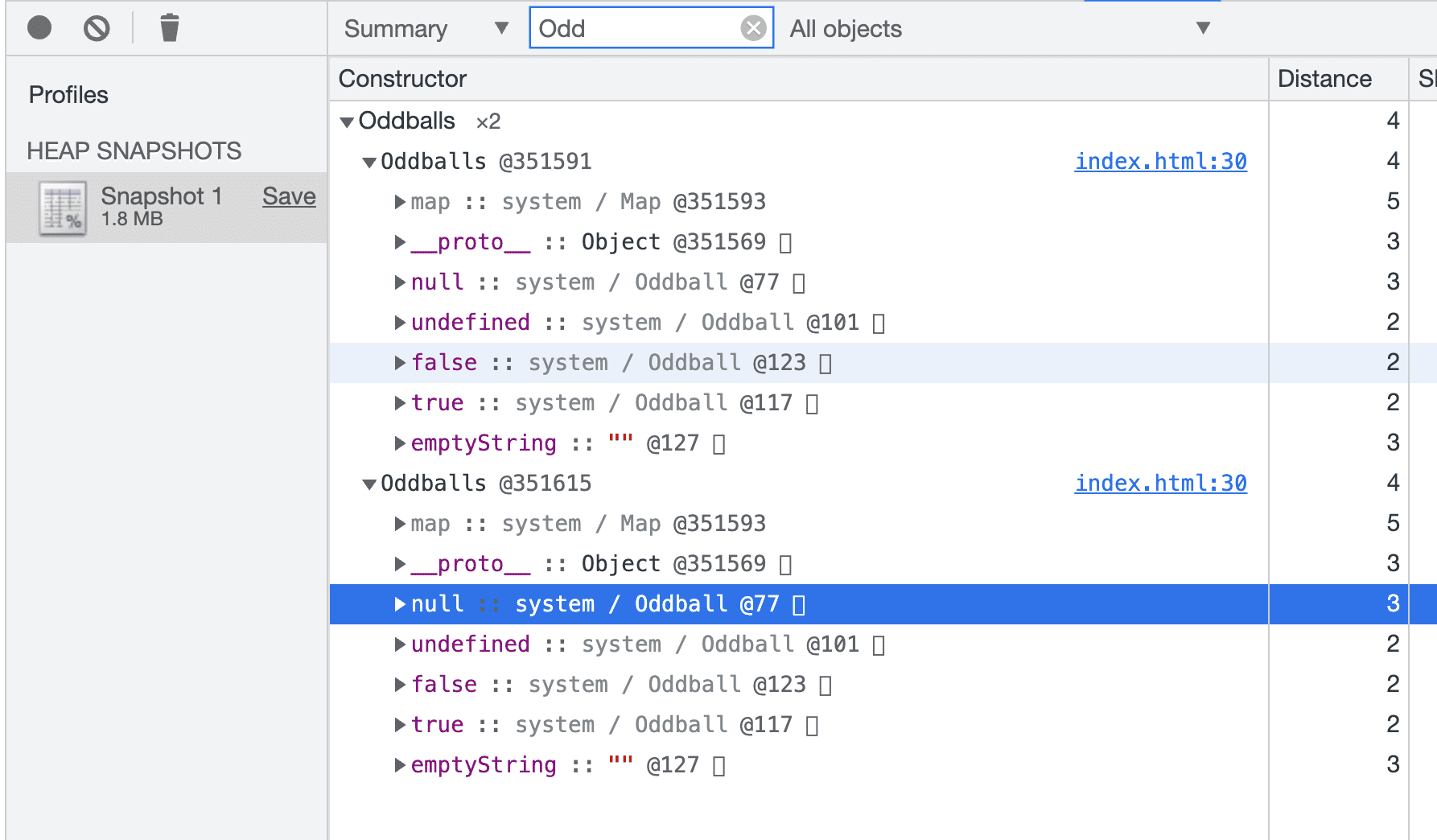
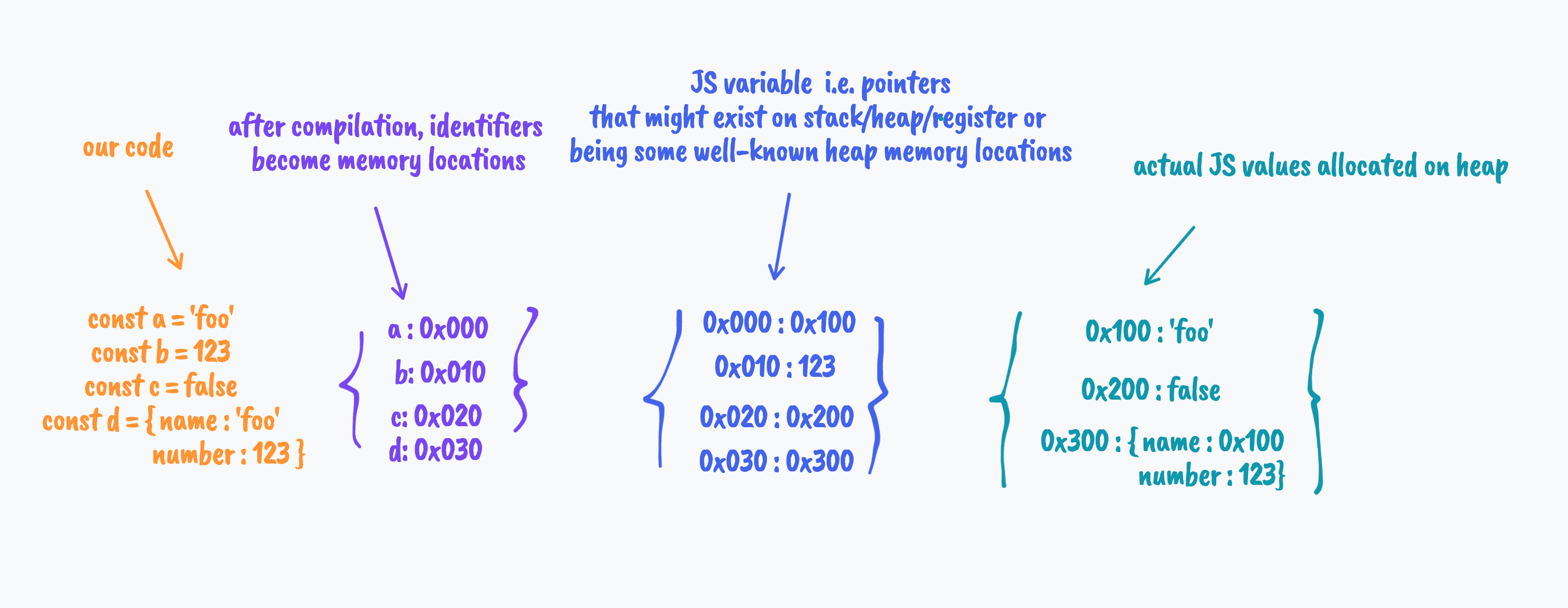

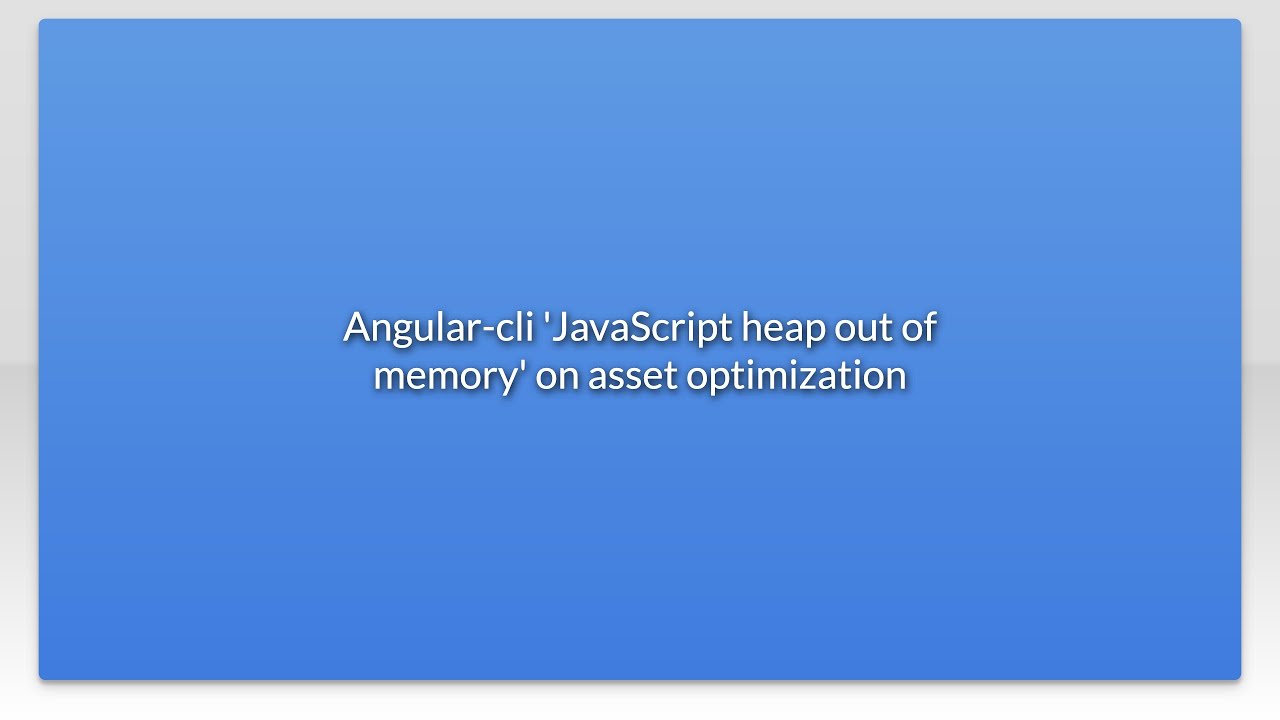

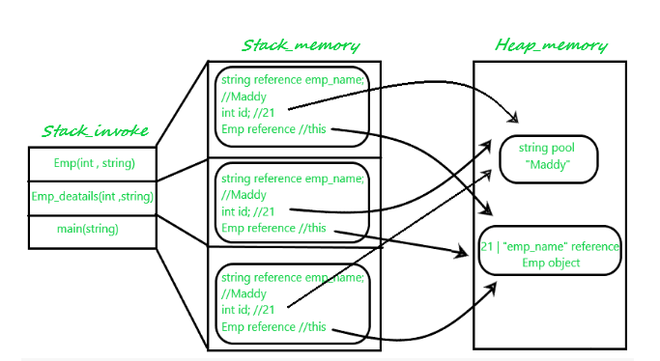


Article link: js heap out of memory.
Learn more about the topic js heap out of memory.
- How to Fix JavaScript Heap Out of Memory Error – MakeUseOf
- Node.js heap out of memory – javascript – Stack Overflow
- Solved: How to Fix “JavaScript Heap Out of Memory Error”
- JavaScript heap out of memory – Snyk Support
- JavaScript Heap out of memory error [Solved] – bobbyhadz
- How to solve JavaScript heap out of memory error – sebhastian
- JavaScript Heap Out Of Memory Error | Felix Gerschau
- JavaScript heap out of memory – PhantomBuster
- JavaScript Heap Out of Memory – Position Is Everything
- How to Solve Heap Out Of Memory Error in JavaScript?
See more: https://nhanvietluanvan.com/luat-hoc/