Move A File In Python
Moving a file in Python involves relocating a file from one location to another within a file system. This operation is particularly useful when organizing files, creating backup copies, or performing other file management tasks. In Python, there are several ways to move a file, but one of the most commonly used methods is by utilizing the `shutil` module.
II. Checking if a File Exists
Before moving a file, it is essential to ensure that the file actually exists in the specified source location. This can be accomplished using the `os.path` module in Python. By combining the `os.path.exists()` function with the file path, you can verify if a file exists and proceed with the moving process accordingly.
III. Specifying the Source and Destination Paths
To move a file in Python, you need to specify both the source file path (the current location of the file) and the destination file path (the new location where the file should be moved). These paths can be absolute or relative.
For example, to move a file named “example.txt” from the source directory “C:/source” to the destination directory “C:/destination”, you would specify the source path as “C:/source/example.txt” and the destination path as “C:/destination/example.txt”.
IV. Using the `shutil` Module for File Movement
Python’s `shutil` module provides a convenient way to move files. The `shutil.move()` function can be used to perform the file movement operation. By passing the source path and the destination path as arguments to `shutil.move()`, the file will be moved from the source to the destination.
Example:
“`python
import shutil
source_path = ‘C:/source/example.txt’
destination_path = ‘C:/destination/example.txt’
shutil.move(source_path, destination_path)
“`
V. Handling Exceptions during File Movement
During the file movement process, errors can occur, such as insufficient permissions, file conflicts, or invalid file paths. To prevent your code from crashing, it is important to handle these exceptions using try-except blocks.
Example:
“`python
import shutil
try:
source_path = ‘C:/source/example.txt’
destination_path = ‘C:/destination/example.txt’
shutil.move(source_path, destination_path)
except shutil.Error as e:
print(f”Error: {e}”)
except Exception as e:
print(f”Error occurred while moving the file: {e}”)
“`
VI. Renaming a File while Moving
In Python, you can rename a file while moving it to a new location. By specifying a different filename in the destination path, you effectively rename the file during the move operation.
Example:
“`python
import shutil
source_path = ‘C:/source/example.txt’
destination_path = ‘C:/destination/new_name.txt’
shutil.move(source_path, destination_path)
“`
VII. Moving Multiple Files
If you need to move multiple files simultaneously, Python allows you to iterate through a list of source paths and perform the file movements iteratively using the `shutil.move()` function.
Example:
“`python
import shutil
source_paths = [‘C:/source/file1.txt’, ‘C:/source/file2.txt’, ‘C:/source/file3.txt’]
destination_path = ‘C:/destination/’
for source_path in source_paths:
shutil.move(source_path, destination_path)
“`
VIII. Moving a File to a Different Directory
Moving a file to a different directory in Python is similar to moving it within the same directory structure. The only difference is that you need to provide the complete destination path, including the new directory.
Example:
“`python
import shutil
source_path = ‘C:/source/example.txt’
destination_directory = ‘C:/new_directory/’
shutil.move(source_path, destination_directory)
“`
IX. Handling File Permissions and Attributes during Movement
When moving a file in Python, the file’s permissions and attributes are usually preserved in the destination location. However, depending on the operating system and file system, there might be exceptions. It is recommended to test the file movement operations on your specific platform to confirm the behavior.
FAQs:
Q1. Can I move a file to a different drive in Python?
Yes, you can move a file to a different drive by specifying the complete destination path, including the drive letter. For example, to move a file from drive C to drive D, you would specify the destination path as “D:/destination/example.txt”.
Q2. Can I move files between different operating systems using Python?
Yes, Python’s `shutil` module supports moving files between different operating systems, as long as the source and destination paths are valid and accessible on the target system.
Q3. What happens if the destination path already exists?
If the destination path already exists, the `shutil.move()` function will raise a `shutil.Error` exception. To handle this situation, you can either choose to rename the file or specify a different destination path.
Q4. Can I move folders using Python?
Yes, Python’s `shutil` module supports moving folders as well. The process is similar to moving files, but you need to provide the source and destination paths for the entire folder structure.
Q5. What happens if a file with the same name already exists in the destination location?
If a file with the same name already exists in the destination location, the `shutil.move()` function will raise a `shutil.Error` exception. To handle this situation, you can either choose to rename the file or specify a different destination path.
File Organizing With Python: Rename, Move, Copy \U0026 Delete Files And Folders
Keywords searched by users: move a file in python Move file Python, Shutil move file, Copy file Python, Move folder Python, Move file python windows, Move in python, Move multiple files to another directory python, Move all file in folder Python
Categories: Top 68 Move A File In Python
See more here: nhanvietluanvan.com
Move File Python
Python, being a versatile programming language, offers various functions and modules to manipulate files and perform file operations. One of the most common file operations is moving files from one location to another. In this article, we will explore the file movement functionality in Python, including the different methods and strategies you can use. So, if you’re ready to dive into the world of file handling in Python, let’s get started!
Moving Files in Python
Before we move forward, it’s important to understand the difference between moving a file and copying a file. When you move a file, it is essentially transferring the file from one directory to another, while removing it from the original location. On the other hand, copying a file creates a duplicate of the file in the desired destination directory, while retaining the original file in its original location. Though similar, both operations have their own use cases.
To start moving files in Python, we need to import the “shutil” module. The “shutil” module provides a high-level interface for handling files and directories. It offers various functions to perform file operations, including moving files. To import shutil, simply add the following line at the beginning of your Python script:
“`
import shutil
“`
Now, let’s discuss the different methods available to move files using Python.
1. Using shutil.move() Function:
The simplest way to move a file in Python is by using the “shutil.move()” function. This function takes two arguments: the source file path and the destination file path. Here’s an example:
“`python
import shutil
source_path = ‘/path/to/source/file.txt’
destination_path = ‘/path/to/destination/file.txt’
shutil.move(source_path, destination_path)
“`
In this example, the “shutil.move()” function moves the “file.txt” from the “source” directory to the “destination” directory.
2. Using os.rename() Function:
Another method to move files in Python is by using the “os.rename()” function. This function is part of the “os” module and allows us to rename or move files by providing the source and destination path. Here’s an example:
“`python
import os
source_path = ‘/path/to/source/file.txt’
destination_path = ‘/path/to/destination/file.txt’
os.rename(source_path, destination_path)
“`
The “os.rename()” function renames or moves the “file.txt” from the “source” directory to the “destination” directory.
3. Handling File Overwrites:
While moving a file, there might be situations where a file with the same name already exists in the destination directory. By default, both “shutil.move()” and “os.rename()” functions will raise an exception if such a situation occurs. However, to handle this and overwrite such files, we can pass the “overwrite=True” argument to the respective functions. Here’s an example:
“`python
import shutil
source_path = ‘/path/to/source/file.txt’
destination_path = ‘/path/to/destination/file.txt’
shutil.move(source_path, destination_path, overwrite=True)
“`
In this example, if a file with the same name already exists in the destination directory, it will be overwritten.
FAQs
Q1. Can I move multiple files using the shutil.move() function?
Yes, you can move multiple files in a single function call. Simply provide the source paths as a list and the destination directory. Here’s an example:
“`python
import shutil
source_paths = [‘/path/to/source/file1.txt’, ‘/path/to/source/file2.txt’]
destination_directory = ‘/path/to/destination/’
for source_path in source_paths:
shutil.move(source_path, destination_directory)
“`
In this example, both “file1.txt” and “file2.txt” will be moved to the “destination” directory.
Q2. Can I move files between different drives or partitions?
Yes, you can move files between different drives or partitions using the “shutil.move()” function. However, it is important to note that moving files between drives or partitions might take longer due to the physical transfer required.
Q3. How can I handle errors while moving files?
You can handle errors using error handling techniques such as try-except blocks. Both “shutil.move()” and “os.rename()” functions may raise exceptions if an error occurs while moving files. By wrapping the move function call inside a try-except block, you can catch and handle any errors gracefully.
Q4. Can I move directories instead of individual files?
Yes, both “shutil.move()” and “os.rename()” functions can be used to move directories. Simply provide the directory path as the source and destination paths.
Q5. Are there any limitations on file sizes or types while moving?
No, Python does not impose any limitations on file sizes or types while moving files. You can move files of any size or type using the “shutil.move()” or “os.rename()” functions.
Conclusion
In this article, we explored the various methods available in Python to move files from one location to another. We discussed the “shutil” module and its move function, as well as the “os” module with its rename function. We also covered handling file overwrites and provided answers to some common FAQs related to file movement in Python. With this knowledge, you can now efficiently handle file movement operations in your Python projects.
Shutil Move File
When it comes to programming and file manipulation, the Shutil library is a handy tool that provides several functions to work with files and directories. One of the most frequently used functions in the Shutil library is “move”, which allows users to easily move files from one location to another. In this article, we will explore the Shutil move file function in-depth and provide a thorough understanding of how to use it effectively.
What is the Shutil move file function?
The Shutil move file function is part of the Shutil library in Python, which provides a high-level interface for file and directory operations. Specifically, the move function is utilized to move files or directories from one location to another. It can be used within the same file system or across different file systems, effectively handling all necessary operations behind the scenes.
Syntax and Parameters:
The syntax of the Shutil move file function is as follows:
shutil.move(src, dst, copy_function=copy2)
The function takes in two parameters: src and dst, representing the source file or directory to be moved and the destination location, respectively. Additionally, an optional parameter called copy_function can be specified to define the method of copying files when moving across different file systems. By default, ‘copy2’ function is used for copying files.
Understanding the functionality:
The Shutil move file function performs a variety of operations depending on the source and destination provided. Let’s explore the possibilities:
1. Moving a file within the same file system:
When both the source and destination paths are within the same file system, the function simply renames the file or directory to the new location without creating a new copy. This operation is typically faster and more efficient than copying the entire file.
2. Moving a file across different file systems:
If the source and destination locations fall under different file systems, the Shutil move file function automatically copies the file using the copy_function specified. After the copy is completed successfully, the original file or directory is removed from the source path.
Handling exceptions and errors:
While using the Shutil move file function, it is essential to handle exceptions and errors that could potentially arise. Here are a few common exceptions and how to handle them:
– FileNotFoundError: This exception occurs when the source file or directory does not exist. It is recommended to add appropriate error handling to inform the user or take necessary actions accordingly.
– PermissionError: This exception occurs when the user does not have sufficient permissions to access or modify the files or directories involved. By utilizing exception handling techniques like try-except blocks, the program can gracefully handle these situations and provide an optimal user experience.
FAQs:
Q: Can the Shutil move file function be used to move multiple files simultaneously?
A: Yes, the Shutil move file function can move multiple files at once by specifying the source and destination paths as lists or using wildcard characters. For example, shutil.move([‘file1.txt’, ‘file2.txt’], ‘destination_folder/’)
Q: Is it possible to overwrite existing files during the moving process?
A: By default, the Shutil move file function does not overwrite existing files in the destination location. If a file already exists, it raises a FileExistsError. To overwrite existing files, you can either handle the exception or utilize the ‘copy_function’ parameter to define different behavior.
Q: Can Shutil move file work across different operating systems?
A: Yes, the Shutil move file function is platform-independent and works seamlessly across different operating systems like Windows, Linux, or macOS.
Q: What happens if there is an error during the copy process?
A: In case an error occurs during the copy process (e.g., insufficient space or read/write errors), the Shutil move file function raises an error and does not delete the original file or directory from the source location.
Q: Does Shutil move file function preserve file metadata (e.g., timestamps)?
A: Yes, by default, the Shutil move file function preserves the original file metadata, including timestamps, unless explicitly specified otherwise.
In conclusion, the Shutil move file function is a powerful tool that simplifies the process of moving files and directories in Python. Whether you want to reorganize files, create backup functionality, or simply manage file locations efficiently, this function serves as a reliable choice. By grasping its syntax, parameters, and capabilities, you can harness the full potential of the Shutil library and optimize your file management processes.
Images related to the topic move a file in python
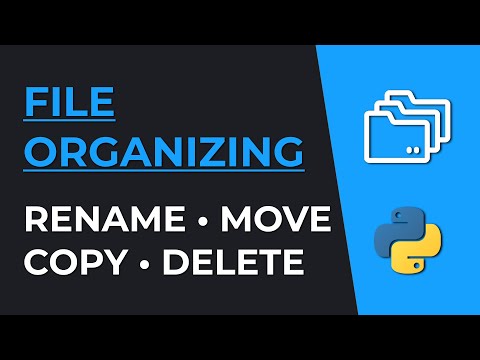
Found 29 images related to move a file in python theme
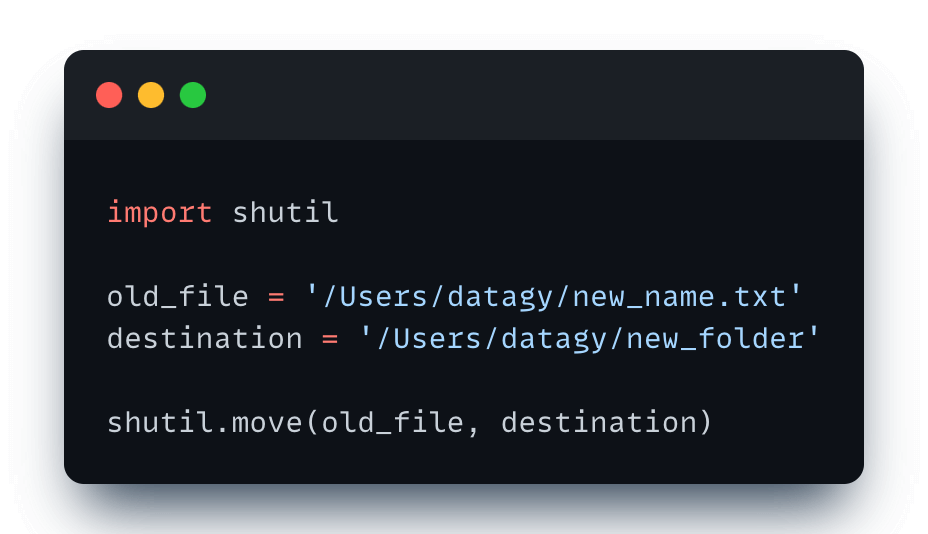
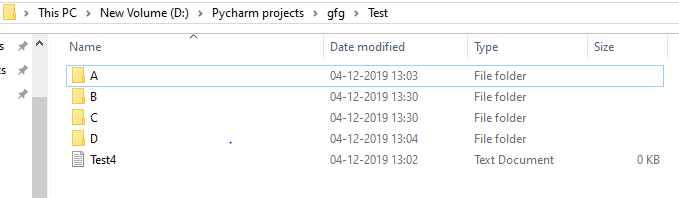
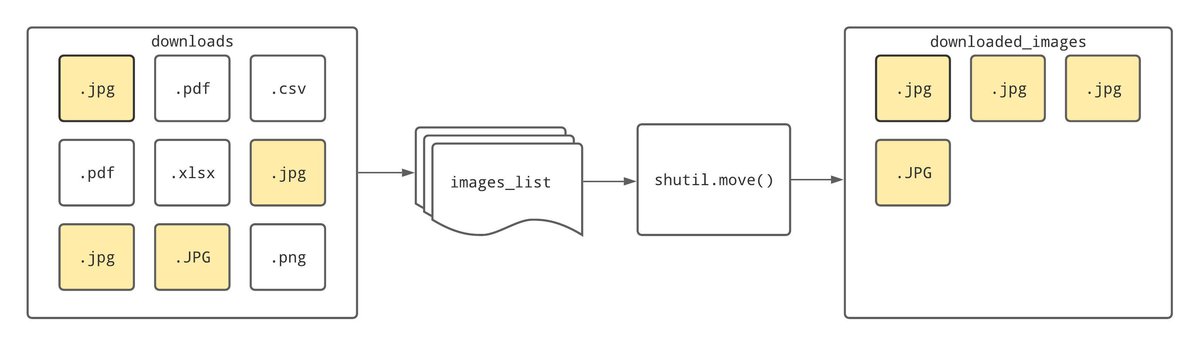
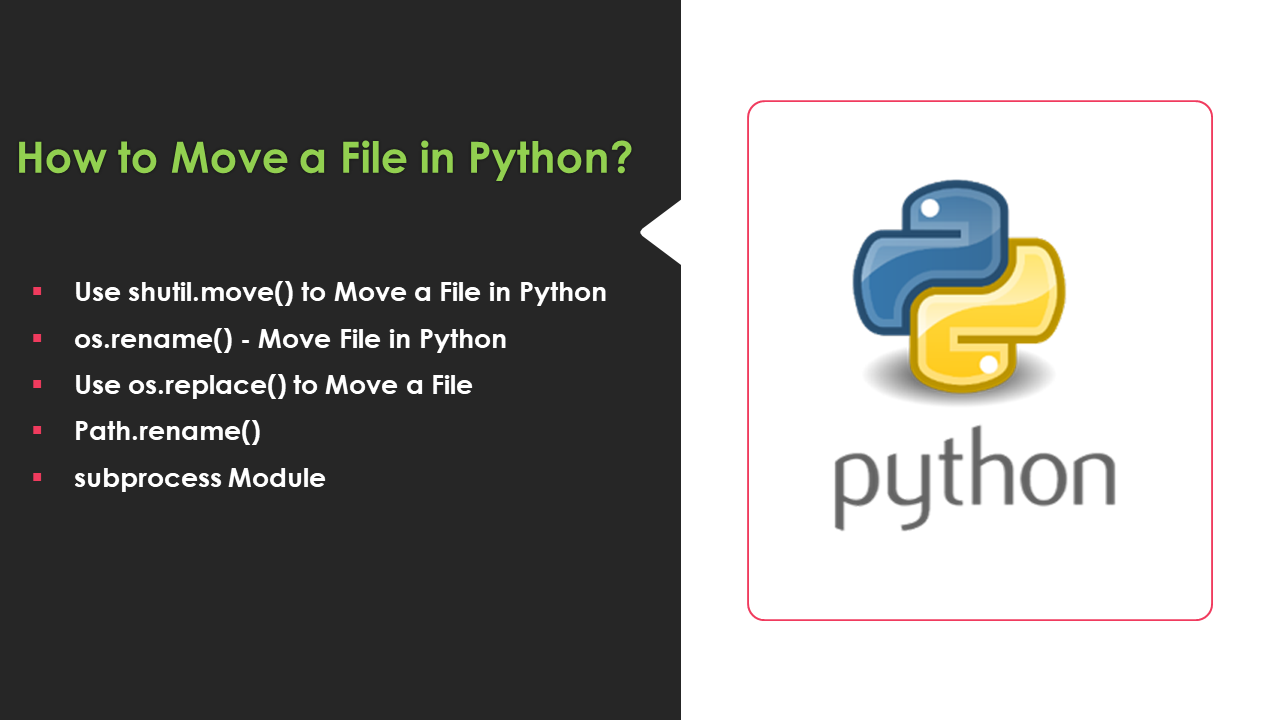
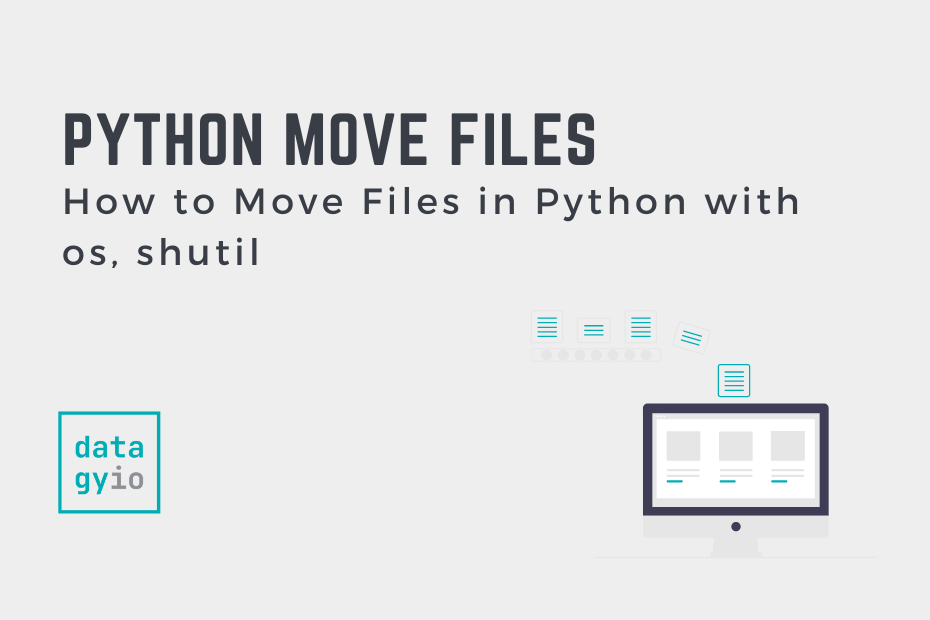
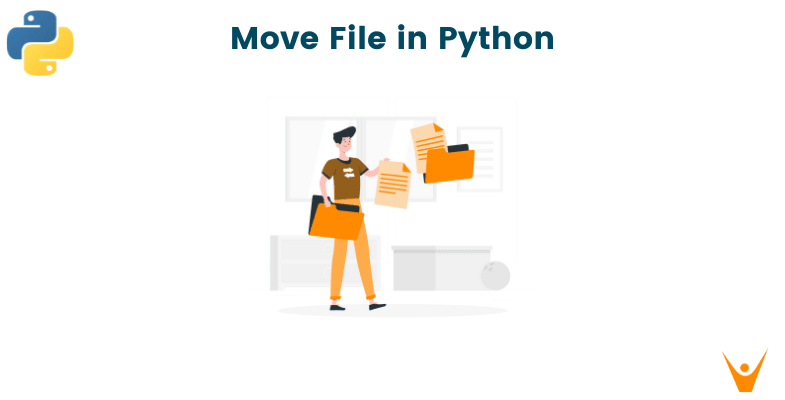
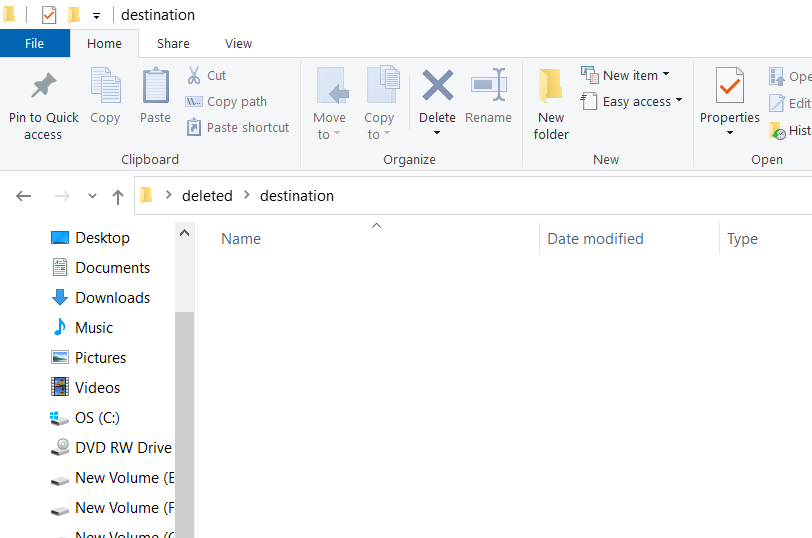

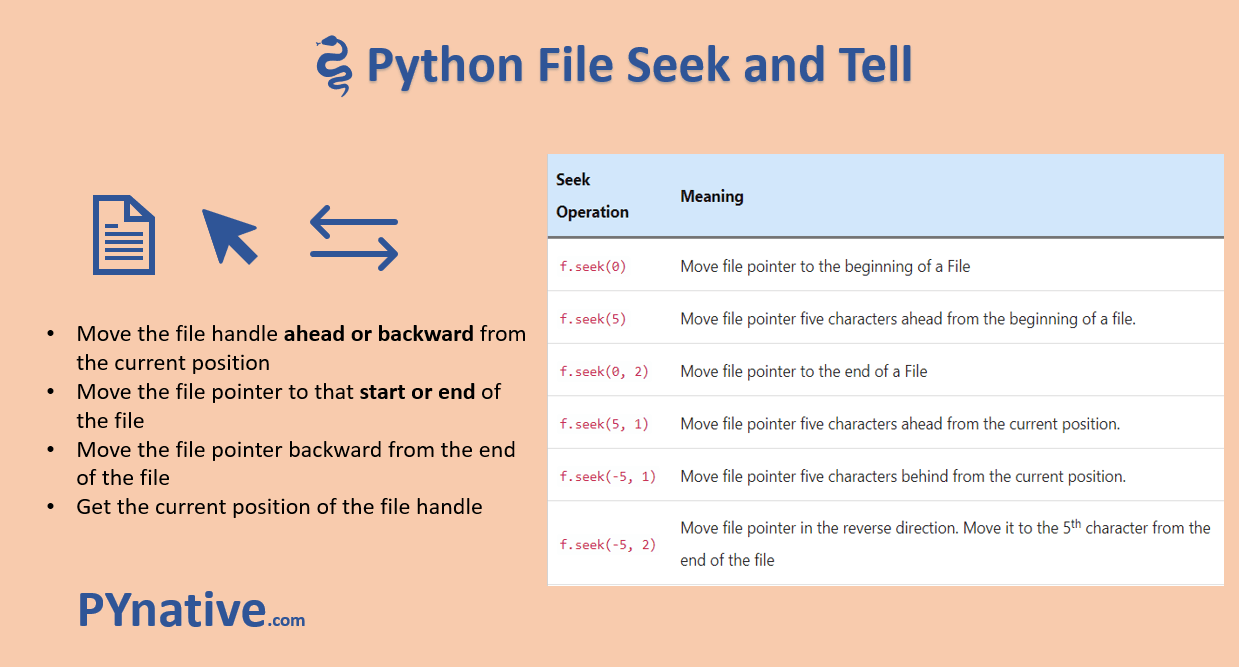
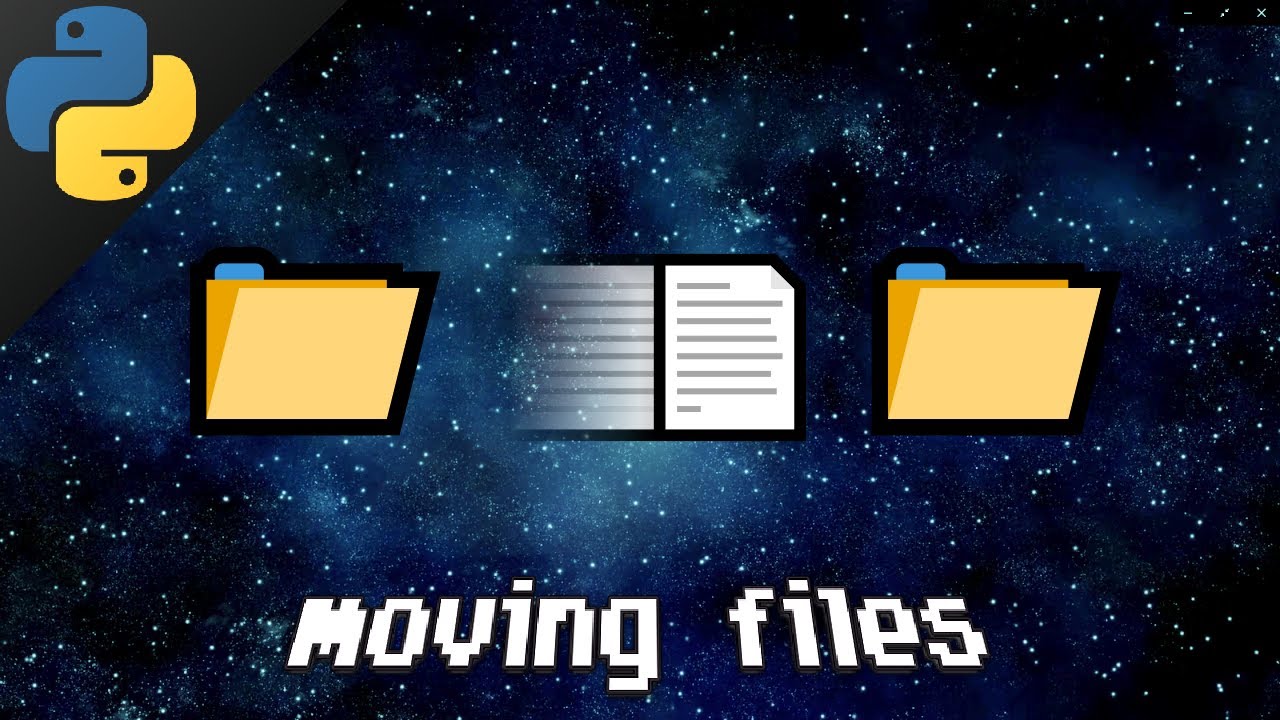
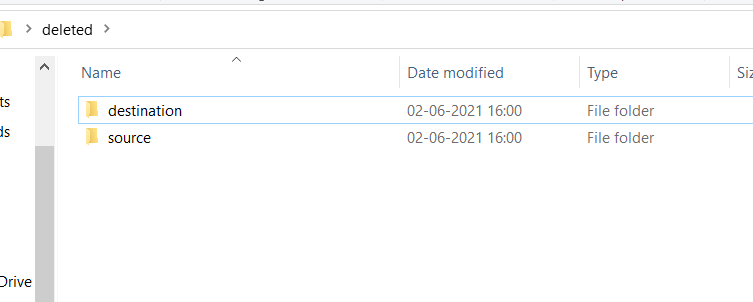
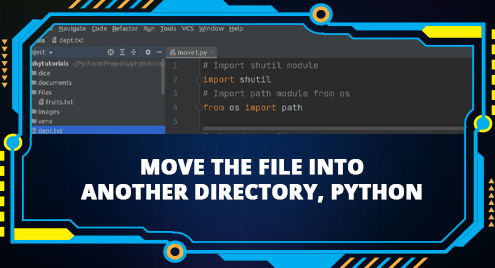
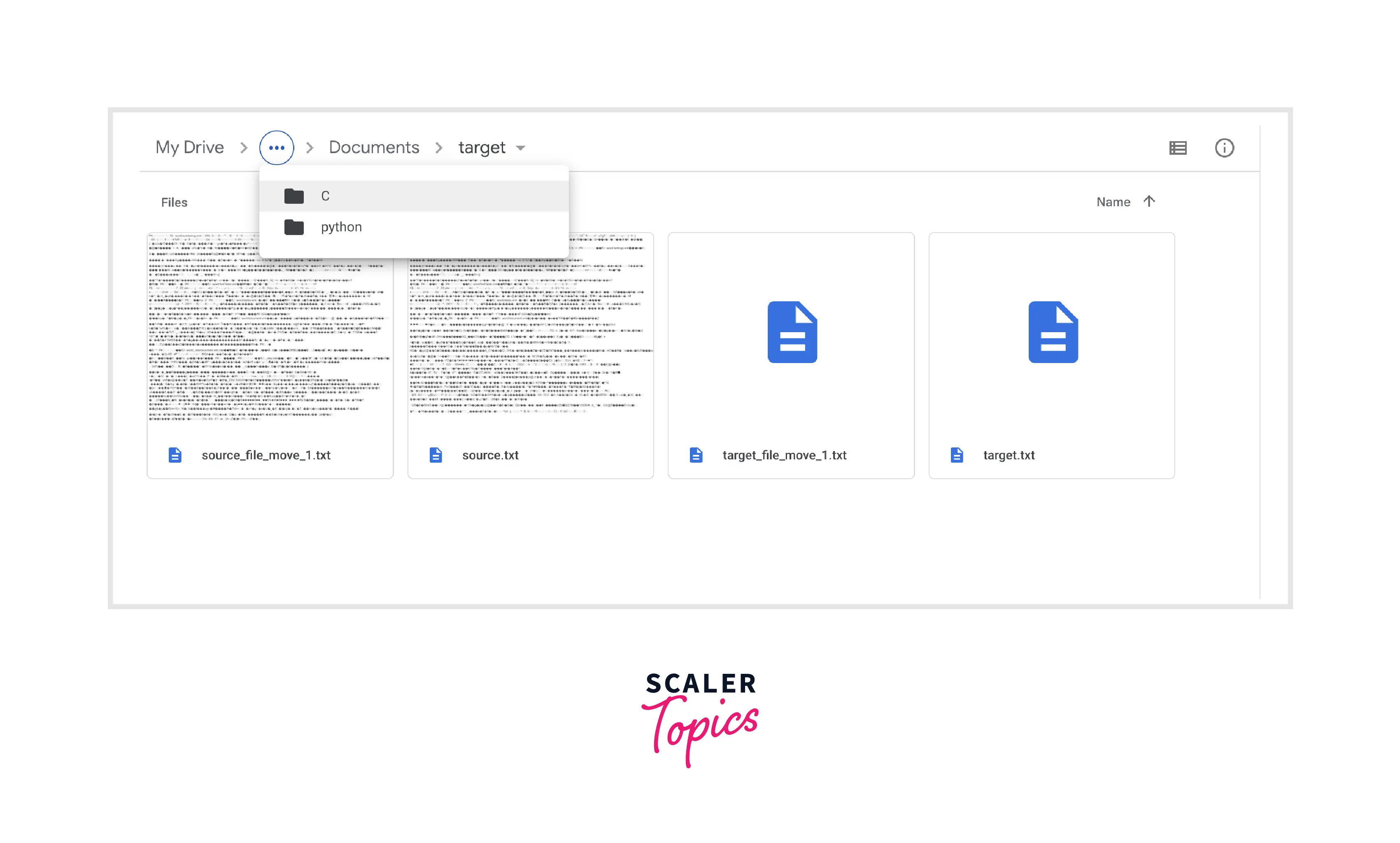
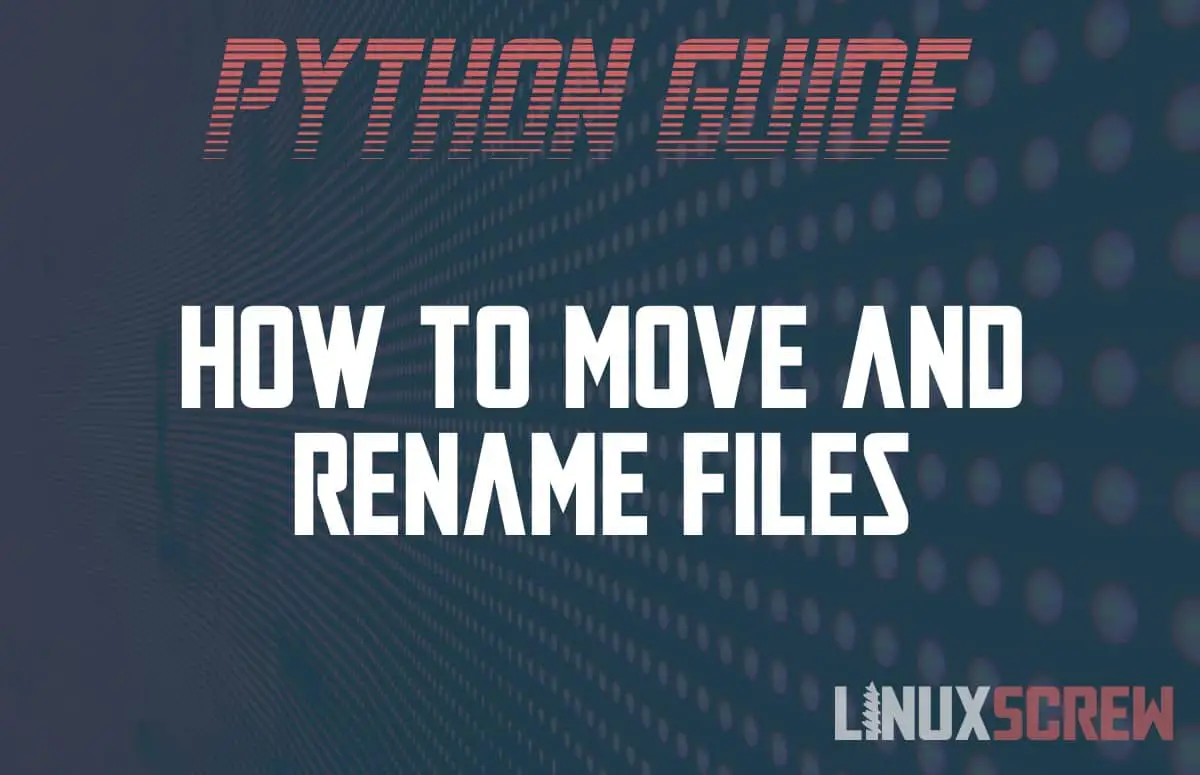
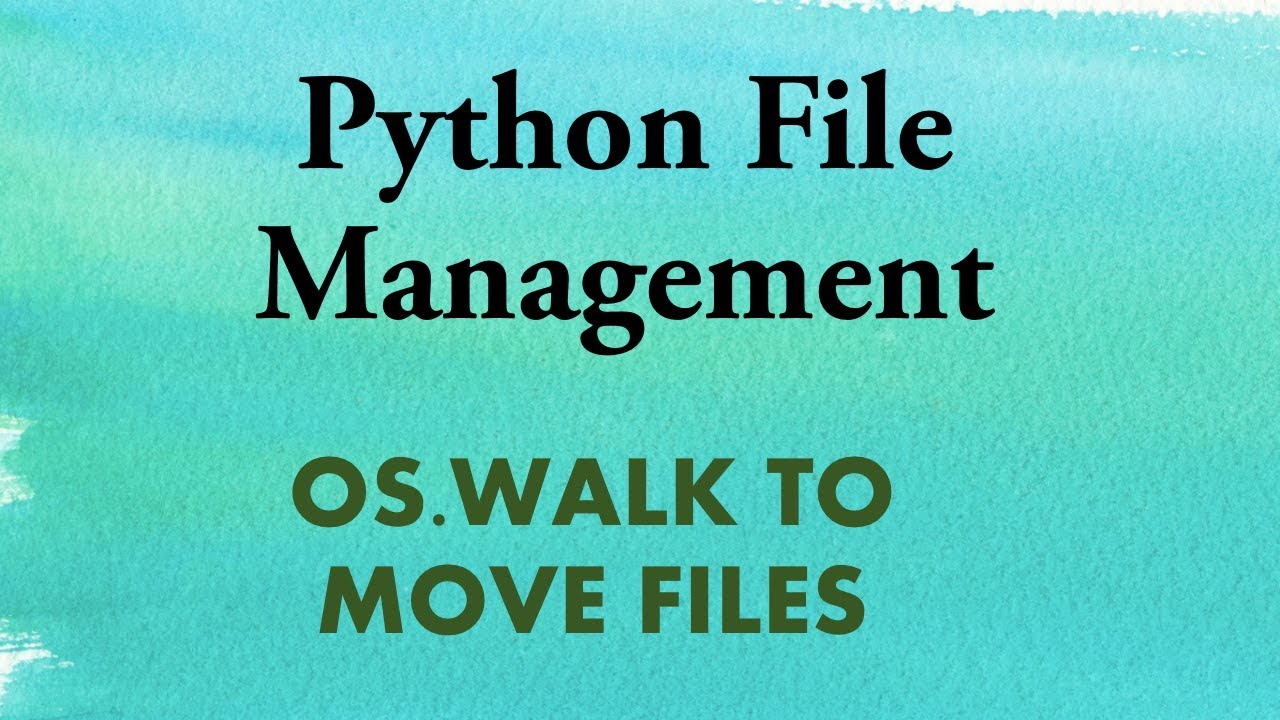
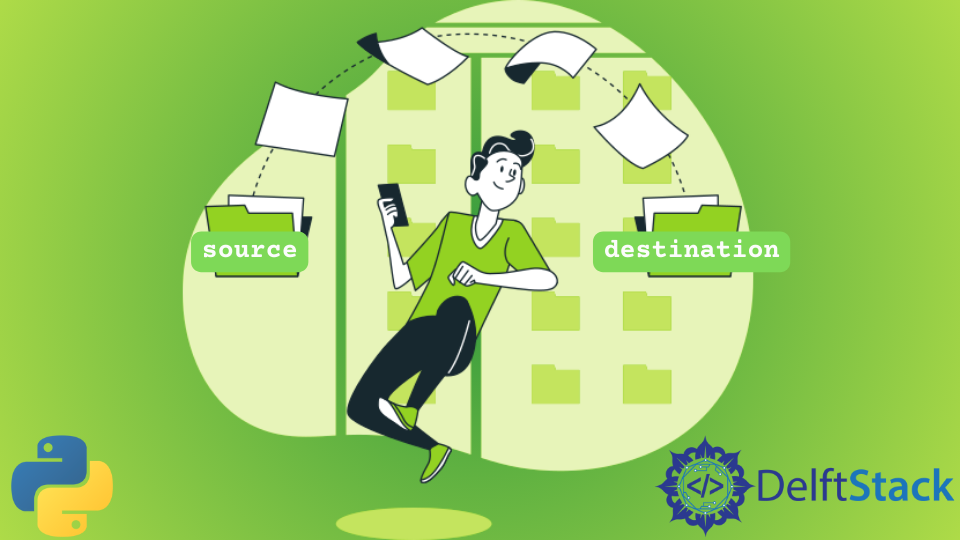
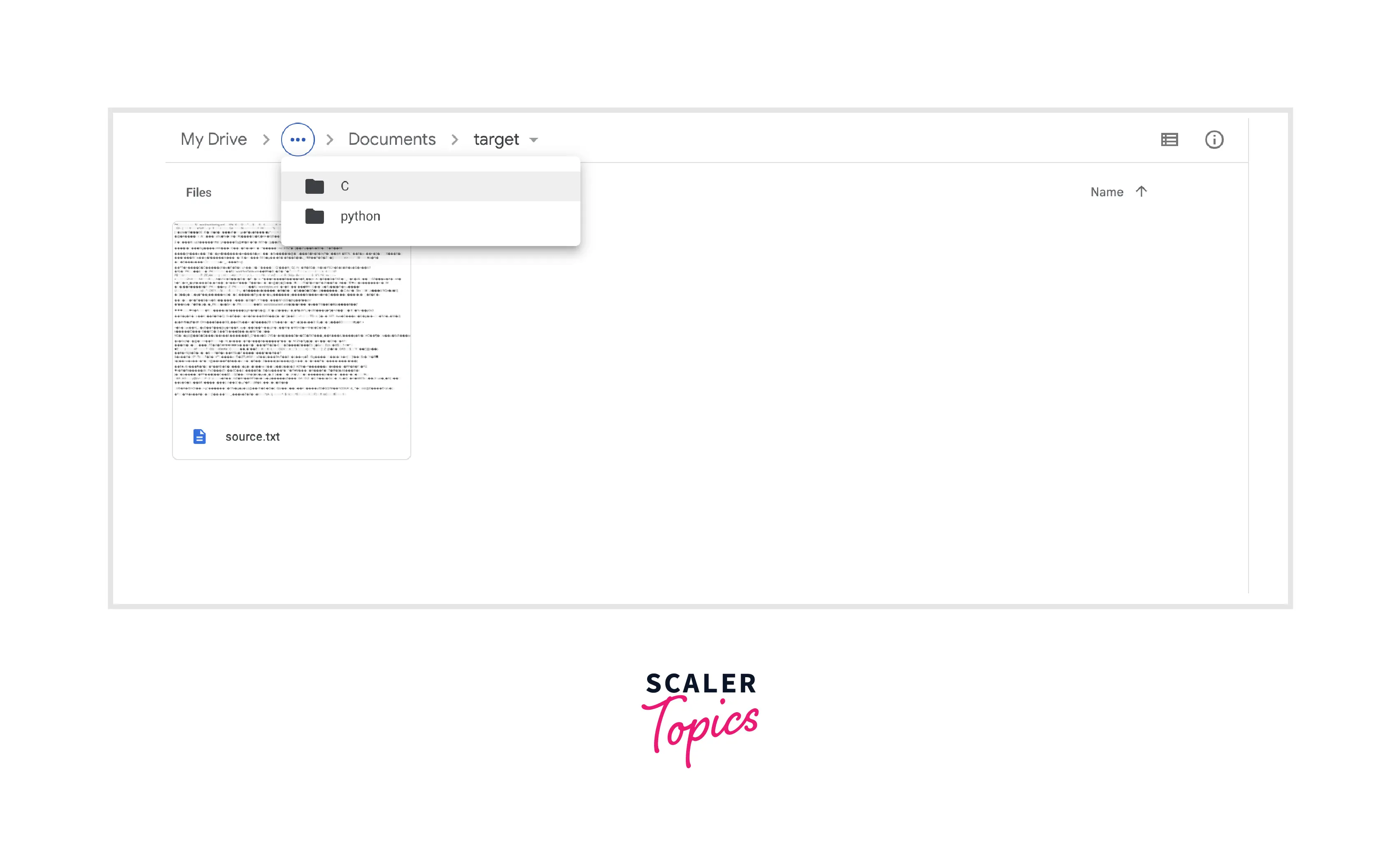
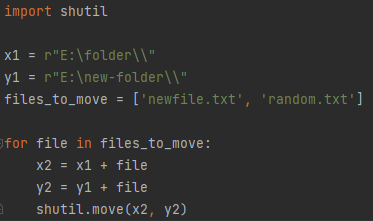

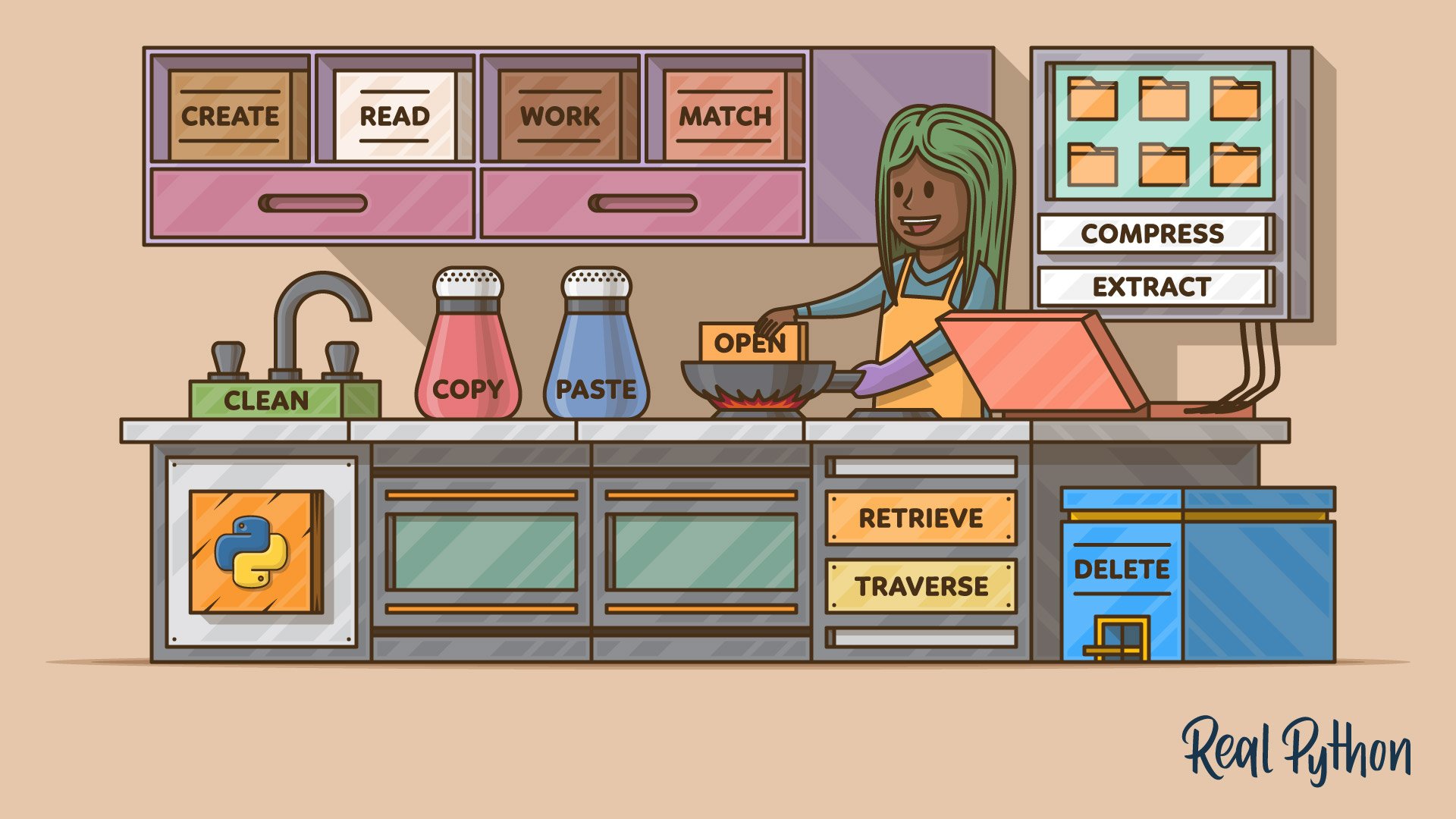
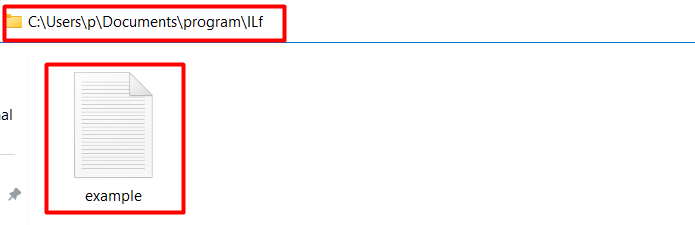
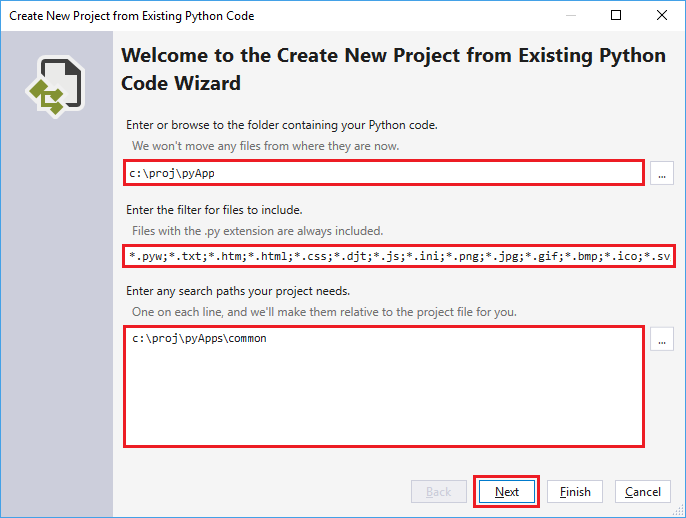
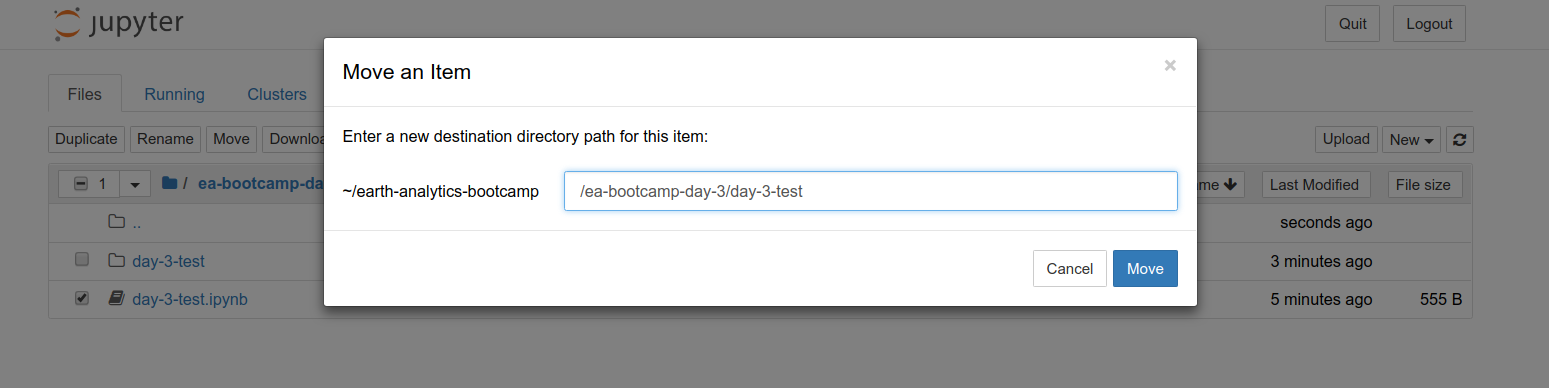
![Python Copy Files and Directories [10 Ways] – PYnative Python Copy Files And Directories [10 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/python-copy-files.png)
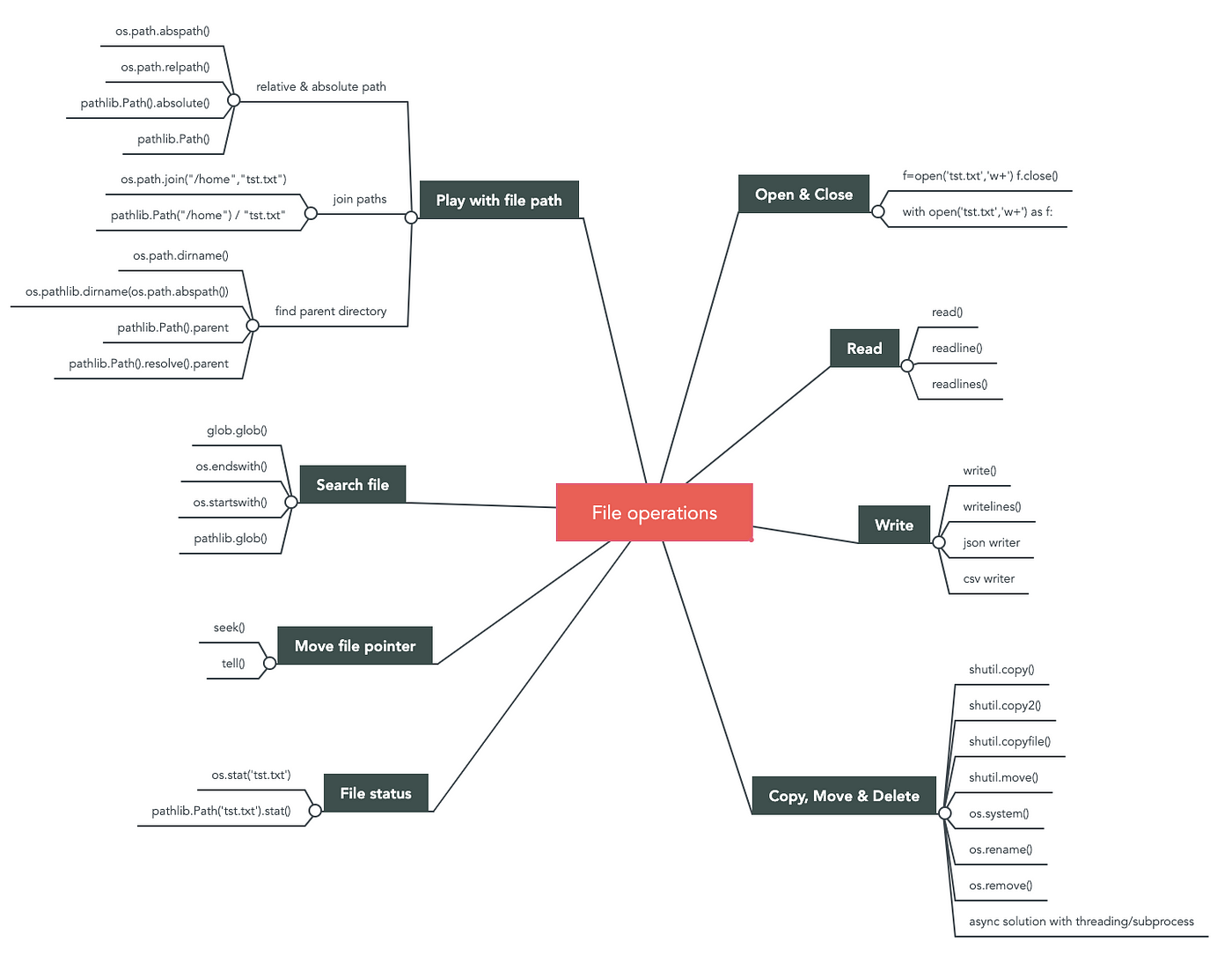
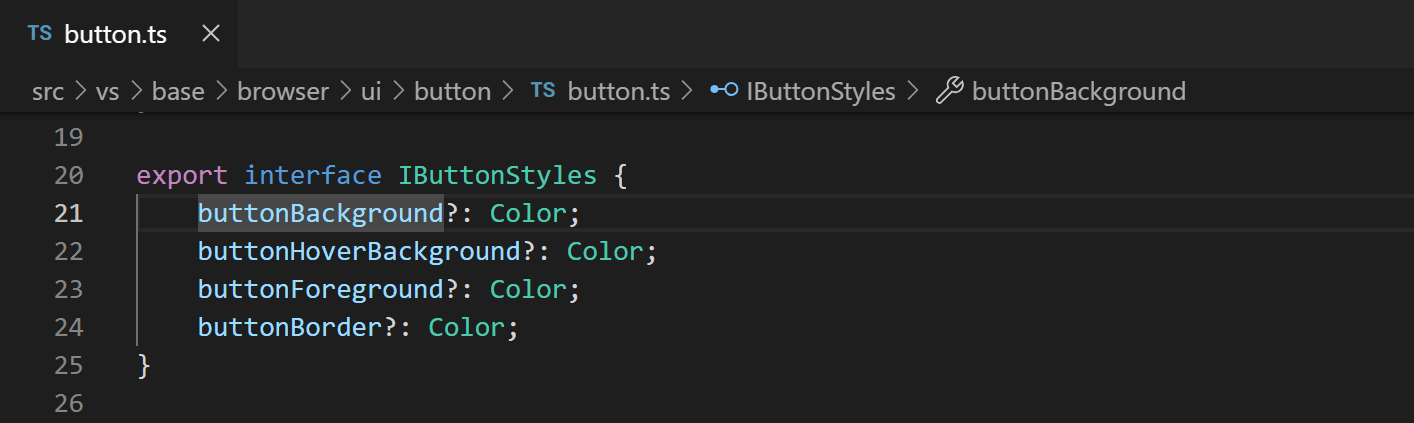

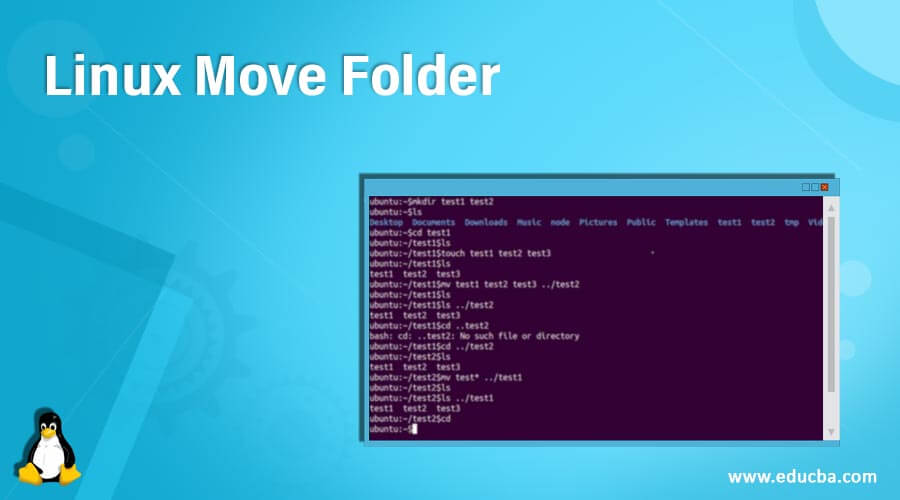



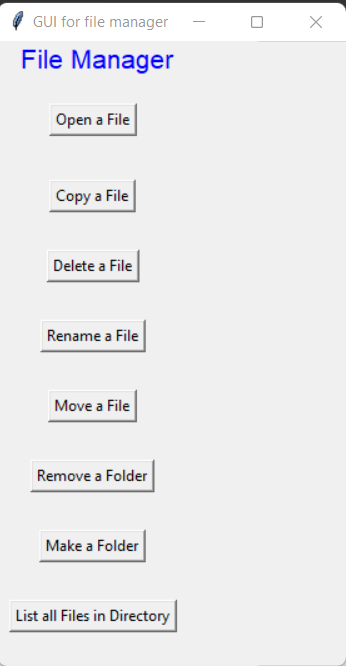
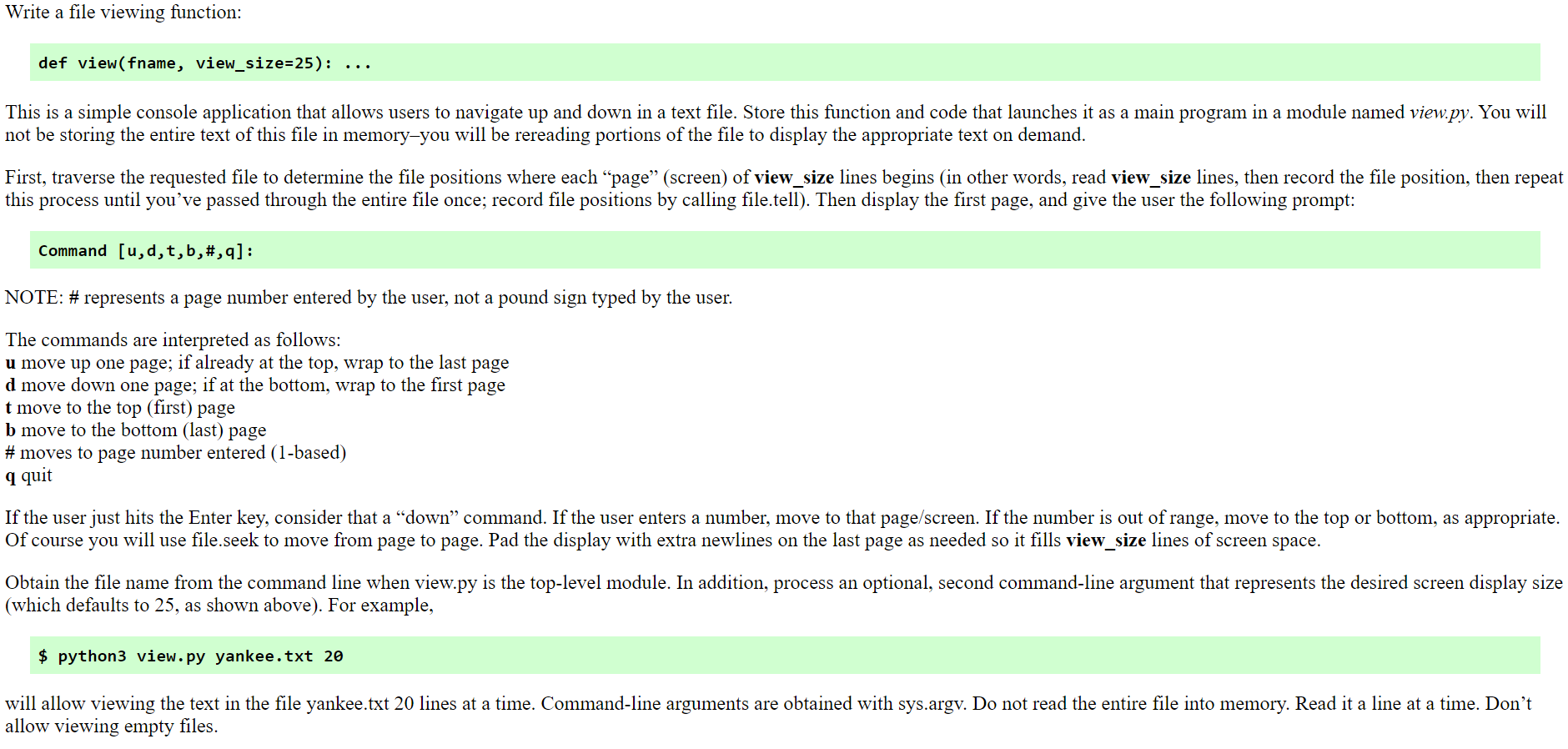
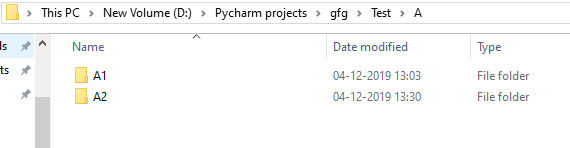

![FileNotFoundError: [Errno 2] No such file or directory [Fix] | bobbyhadz Filenotfounderror: [Errno 2] No Such File Or Directory [Fix] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-filenotfounderror-no-such-file-or-directory/move-file-next-to-your-python-script.webp)
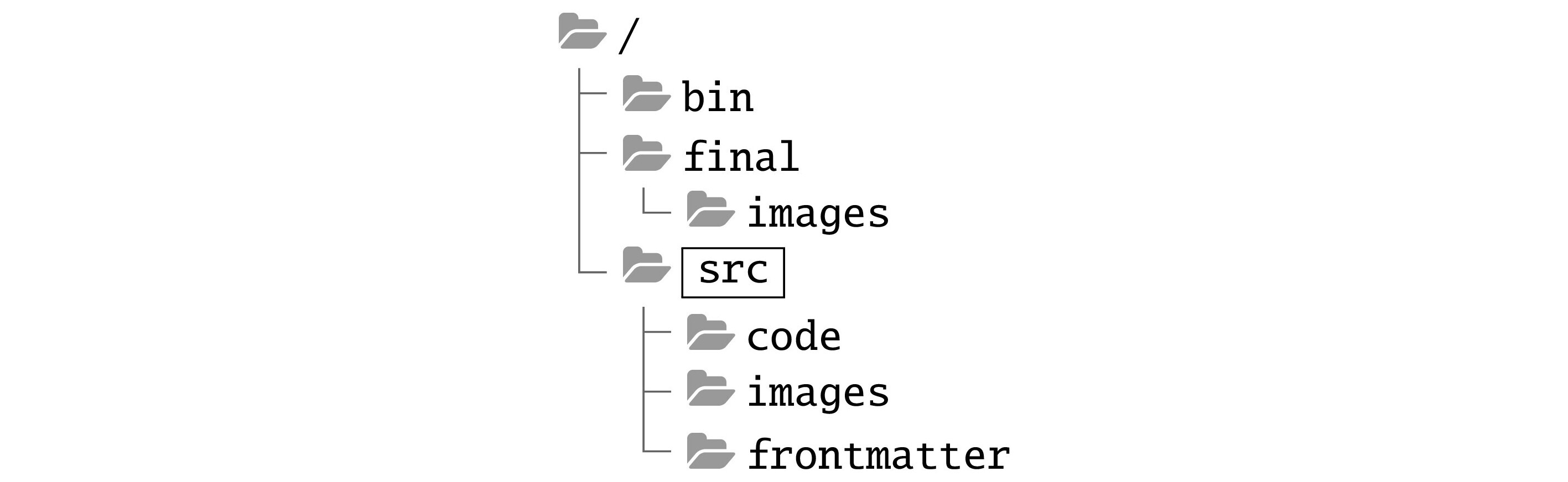
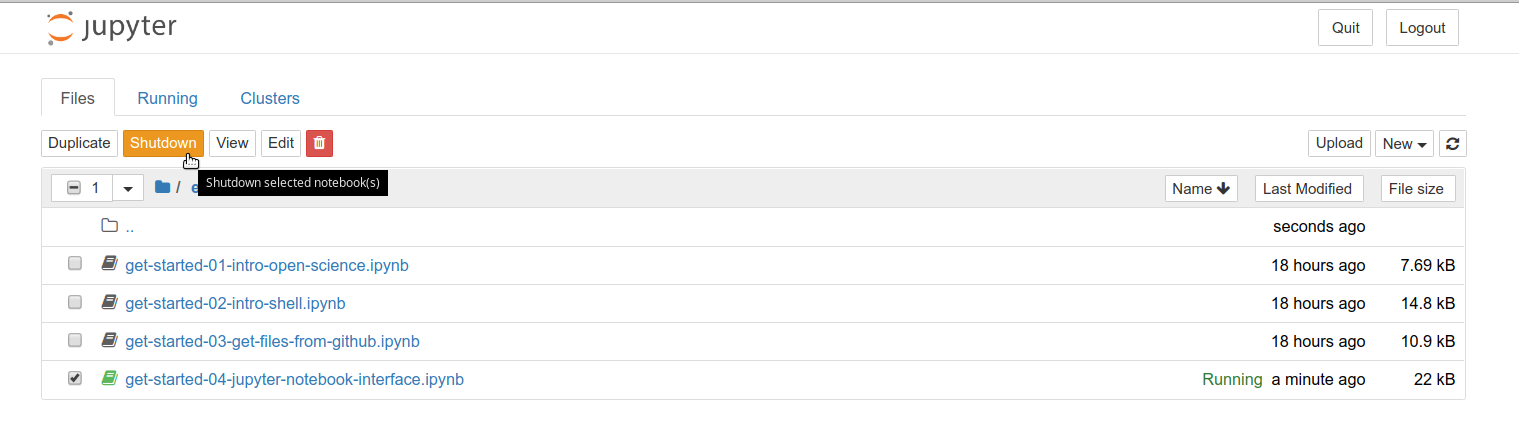
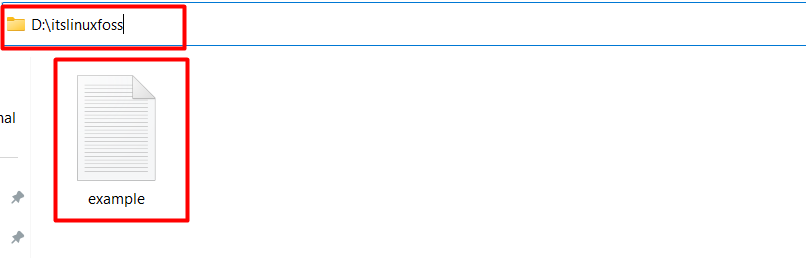



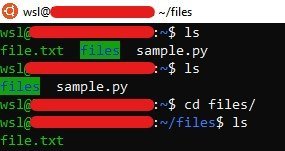
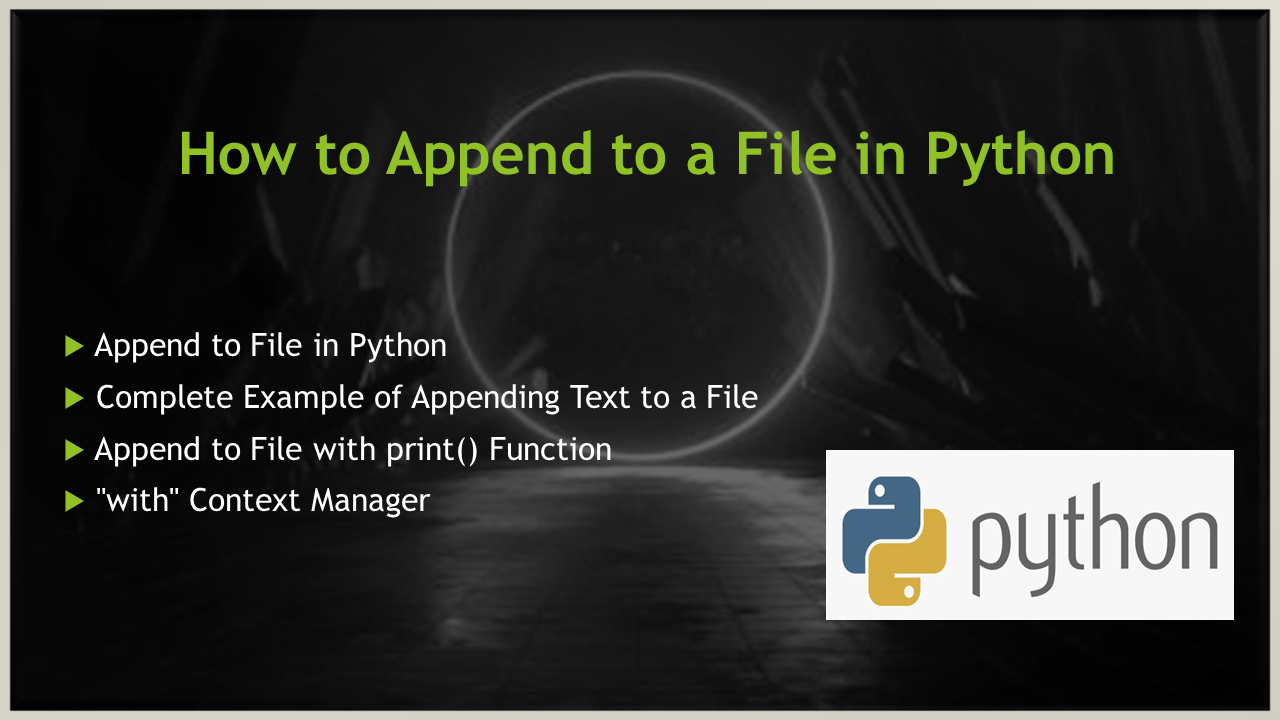
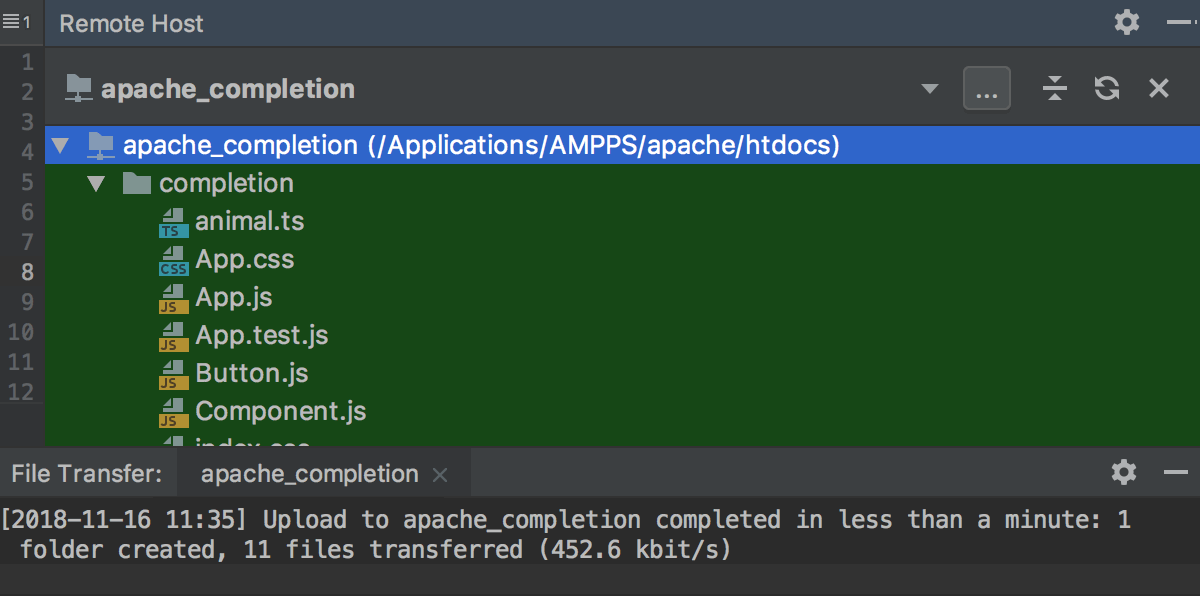

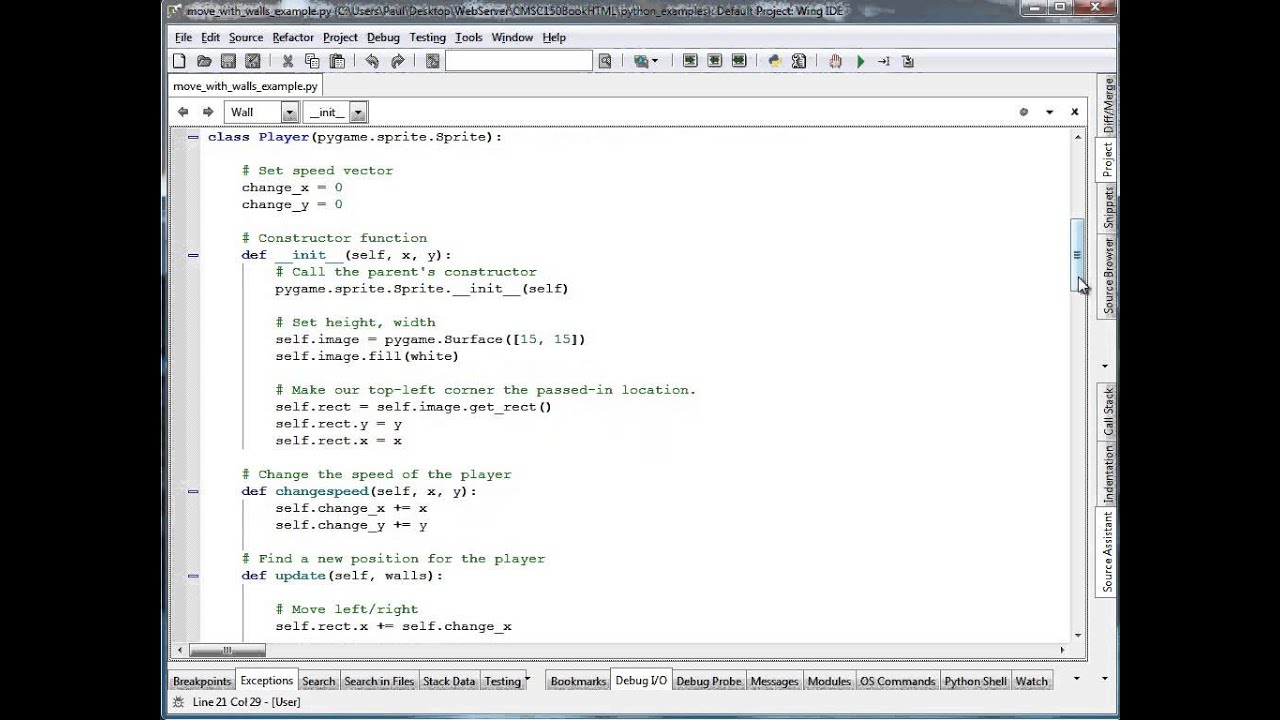
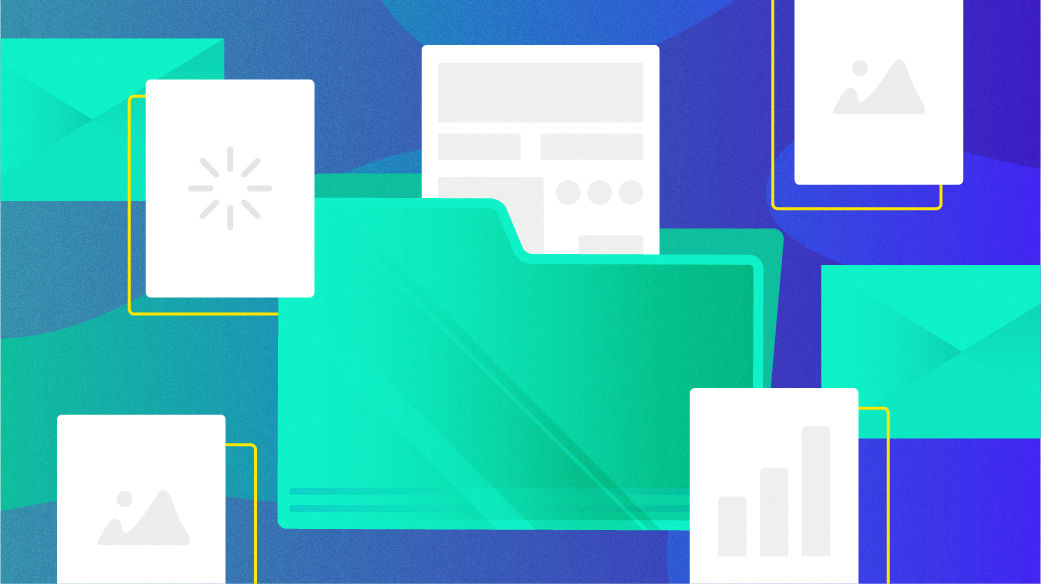
Article link: move a file in python.
Learn more about the topic move a file in python.
- How to Move Files in Python (os, shutil) – Datagy
- How do I move a file in Python? – Stack Overflow
- How to Move Files in Python: Single and Multiple File Examples
- Move Files Or Directories in Python – PYnative
- 3 Ways to Move File in Python (Shutil, OS & Pathlib modules)
- How to Move a File in Python? – Spark By {Examples}
- How to Move a File or Directory in Python (with examples)
- How to move all files from one directory to another using Python
- How to move a file from one folder to another using Python
See more: https://nhanvietluanvan.com/luat-hoc