Python List Without Commas
Lists are an essential part of programming in Python. They allow you to store and manipulate collections of data in a flexible and efficient manner. In this article, we will explore the basic concepts of lists in Python, understand their essential components, and learn various techniques to manipulate and print lists without commas.
Exploring Basic Concepts of Lists in Python
In Python, a list is a collection of items enclosed within square brackets ([]). Lists can contain elements of different data types such as integers, strings, or even other lists. For example:
my_list = [1, 2, 3, “apple”, “banana”]
Here, we have a list containing integers (1, 2, 3) and strings (“apple”, “banana”).
Understanding the Essential Components of a List
To work effectively with lists, it is important to understand the essential components that make up a list. The two significant components of a list are indexing and length.
Indexing: Each element in a list is assigned a unique index starting from 0 for the first element. You can access individual elements of a list using their respective indices. For example:
my_list = [10, 20, 30, 40, 50]
print(my_list[2]) # Output: 30
Length: The length of a list refers to the total number of elements present in the list. You can determine the length of a list using the len() function. For example:
my_list = [1, 2, 3, 4, 5]
print(len(my_list)) # Output: 5
Manipulating Lists: Inserting, Appending, and Extending Elements
Lists offer several methods to manipulate their content. You can insert, append, or extend elements in a list.
Inserting Elements: The insert() method allows you to insert an element at a specific index within the list. For example:
my_list = [1, 2, 3, 4, 5]
my_list.insert(2, “apple”)
print(my_list) # Output: [1, 2, “apple”, 3, 4, 5]
Appending Elements: The append() method adds an element at the end of a list. For example:
my_list = [1, 2, 3, 4, 5]
my_list.append(“apple”)
print(my_list) # Output: [1, 2, 3, 4, 5, “apple”]
Extending Elements: The extend() method allows you to add multiple elements to the end of a list. For example:
my_list1 = [1, 2, 3]
my_list2 = [4, 5, 6]
my_list1.extend(my_list2)
print(my_list1) # Output: [1, 2, 3, 4, 5, 6]
Removing Elements from a List: Pop, Remove, and Delete Methods
To remove elements from a list, Python provides various methods such as pop(), remove(), and del.
Pop Method: The pop() method removes and returns an element from a specific index. For example:
my_list = [1, 2, 3, 4, 5]
removed_element = my_list.pop(2)
print(removed_element) # Output: 3
print(my_list) # Output: [1, 2, 4, 5]
Remove Method: The remove() method removes the first occurrence of a specific value from the list. For example:
my_list = [1, 2, 3, 4, 5]
my_list.remove(3)
print(my_list) # Output: [1, 2, 4, 5]
Delete Method: The del keyword deletes an element or a slice of elements using their indices. For example:
my_list = [1, 2, 3, 4, 5]
del my_list[2]
print(my_list) # Output: [1, 2, 4, 5]
Accessing and Modifying List Elements
In Python, you can access individual elements of a list using indexing. Similarly, you can modify the elements of a list by assigning new values to their corresponding indices. For example:
my_list = [10, 20, 30, 40, 50]
my_list[2] = 35
print(my_list) # Output: [10, 20, 35, 40, 50]
Slicing Lists: Creating Sublists
Lists support slicing, which allows you to extract a portion of a list. Slicing is done by specifying the start and end indices along with an optional step value. For example:
my_list = [1, 2, 3, 4, 5]
sub_list = my_list[1:4]
print(sub_list) # Output: [2, 3, 4]
Working with Nested Lists: A Multidimensional Approach
Python lists can also contain other lists, enabling you to create multidimensional lists. These nested lists offer a convenient way to store and access complex data structures. For example:
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(my_list[1][2]) # Output: 6
Sorting and Reversing Lists: Altering Element Order
Python provides built-in methods to sort and reverse the order of elements in a list.
Sorting a List: The sort() method arranges the elements of a list in ascending or descending order. For example:
my_list = [4, 2, 6, 1, 9, 7]
my_list.sort() # Ascending order
print(my_list) # Output: [1, 2, 4, 6, 7, 9]
Reversing a List: The reverse() method reverses the order of elements in a list. For example:
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
Using List Comprehensions to Create Lists Efficiently
List comprehensions provide a concise way to create lists based on existing lists or other iterable objects. They eliminate the need for explicit loops and conditional statements. For example:
my_list = [x for x in range(1, 10) if x % 2 == 0]
print(my_list) # Output: [2, 4, 6, 8]
Common Methods and Functions to Manipulate Python Lists without Commas
1. How to print list without brackets Python:
To print a list without brackets in Python, you can use the join() method to concatenate the elements of the list into a single string. For example:
my_list = [“apple”, “banana”, “cherry”]
result = ” “.join(my_list)
print(result) # Output: apple banana cherry
2. Remove square brackets from list Python:
To remove the square brackets from a list in Python, you can use slicing to slice the list and eliminate the brackets. For example:
my_list = [1, 2, 3, 4, 5]
result = str(my_list)[1:-1]
print(result) # Output: 1, 2, 3, 4, 5
3. Remove comma in List Python:
To remove the comma in a list in Python, you can use the join() method along with an empty string as the separator. For example:
my_list = [“apple”, “banana”, “cherry”]
result = “”.join(my_list)
print(result) # Output: applebananacherry
4. Print list Python:
To print a list in Python, you can simply use the print statement with the list as the argument. For example:
my_list = [1, 2, 3, 4, 5]
print(my_list) # Output: [1, 2, 3, 4, 5]
5. Array to string Java without brackets:
In Java, to convert an array to a string without brackets, you can use the Arrays.toString() method to convert the array to a string and then use the replace() method to remove the brackets. For example:
import java.util.Arrays;
int[] myArray = {1, 2, 3, 4, 5};
String result = Arrays.toString(myArray).replace(“[“, “”).replace(“]”, “”);
System.out.println(result); // Output: 1, 2, 3, 4, 5
6. How to print list in Python with brackets:
To print a list in Python with brackets, you can simply use the print statement with the list as the argument. The brackets will be automatically included. For example:
my_list = [1, 2, 3, 4, 5]
print(my_list) # Output: [1, 2, 3, 4, 5]
In conclusion, lists are fundamental data structures in Python that allow you to store and manipulate collections of data. They offer a wide range of methods and techniques to manage and modify their content. Additionally, we discussed various ways to manipulate and print lists without commas, providing flexibility and customization to suit your programming needs. With these concepts and techniques, you can efficiently work with lists in your Python programs.
Python Py Tutorial 2023 Remove Brackets And Commas From List Collection
Why Does My List Not Have Commas Python?
Python is a powerful programming language that offers a wide range of functionality, including the ability to work with lists. Lists are an essential component in Python as they allow us to store and manipulate collections of items. However, sometimes you might encounter a situation where your list does not have commas. In this article, we will explore why this might be the case and how to address it.
Understanding Lists in Python
Before diving into the specifics of why your list may not have commas, let’s refresh our understanding of lists in Python. A list is a mutable, ordered collection of items enclosed within square brackets []. Each item in a list is separated by a comma, allowing for easy differentiation between elements.
For example, consider the following list:
numbers = [1, 2, 3, 4, 5]
In this list, commas are used to separate each individual number. This makes it clear that we have a collection of distinct elements.
Why Doesn’t My List Have Commas?
If your list does not have commas, it is likely due to incorrect syntax or a misunderstanding of how lists work in Python. Here are some common reasons why your list might be missing commas:
1. Forgetting to include commas: The most common reason for missing commas in a list is simply forgetting to include them. It is crucial to ensure that each item in the list is separated by a comma. Forgetting to do so can lead to syntax errors.
2. Mixing elements without commas: Another common mistake is mixing elements without properly separating them. For instance, consider the following erroneous list:
mixed_list = [‘apple’ ‘banana’ ‘orange’]
In this example, the three fruits ‘apple,’ ‘banana,’ and ‘orange’ are not separated by commas. Consequently, Python treats it as one continuous string rather than individual elements.
3. Inappropriate use of quotation marks: If you are including strings in your list, it is essential to enclose them in quotation marks. Forgetting to add quotes or using inappropriate quotation marks can also lead to missing commas. For instance:
fruits = [apple, banana, orange]
In this example, the variable names ‘apple,’ ‘banana,’ and ‘orange’ should be enclosed in quotes to indicate that they are string elements. The lack of quotation marks here results in a syntax error.
4. Using incorrect data types: In Python, lists can contain various data types, including numbers, strings, and even other lists. However, mixing incompatible data types without appropriate conversions can lead to issues such as missing commas. Make sure to use the correct data type for each element within the list.
How to Fix Lists Without Commas
If you have identified that your list is missing commas, here are a few steps to help you rectify the issue:
1. Add commas between elements: Double-check your list and ensure that each element is separated by a comma. For example, if your list is:
numbers = [1 2 3 4 5]
Modify it to:
numbers = [1, 2, 3, 4, 5]
2. Enclose strings in quotes: If your list contains string elements, make sure to enclose them in appropriate quotes. For example:
fruits = [apple, banana, orange]
should be modified to:
fruits = [‘apple’, ‘banana’, ‘orange’]
3. Verify correct data types: Ensure that each element in the list has the appropriate data type. If you mix data types within the list, be sure to convert them as needed.
Frequently Asked Questions (FAQs)
Q1. Are commas necessary in Python lists?
Yes, commas are necessary in Python lists. They are used to separate individual elements, making it clear that the list contains distinct items.
Q2. What happens if commas are missing in a Python list?
If commas are missing in a Python list, it can lead to syntax errors and cause Python to interpret the list elements differently. Missing or misplaced commas can alter the intended structure of the list.
Q3. Can I have lists without commas in other programming languages?
The use of commas to separate list elements is specific to Python syntax. Other programming languages may have different conventions for working with lists. However, in most programming languages, some form of delimiter is used to separate items within a list-like data structure.
Q4. How do I identify if my list is missing commas?
If your list is missing commas, you may encounter syntax errors when running your Python code. Additionally, you can look for any irregularities in the structure of your list, such as elements merging together or unrecognizable output.
Q5. Can a list have other lists without commas?
Yes, a list can contain other lists within it. Each nested list would have its own set of commas to separate its individual elements. However, it is important to ensure that each comma is placed correctly to avoid syntax errors.
In conclusion, commas are vital when working with lists in Python. Incorrect usage of commas can result in syntax errors and alter the intended structure of the list. By understanding the reasons behind missing commas, you can identify and rectify the issue to ensure your Python code operates as expected. Remember to always double-check your code and be mindful of the correct syntax and data types when working with lists.
How To Print Out A List Without Brackets And Commas Python?
Printing out a list in Python is a common task, but sometimes the default formatting can be inconvenient. By default, Python uses brackets and commas to represent the elements of a list. However, there are situations where we might prefer a cleaner and more concise representation. In this article, we will explore different ways to print out a list without brackets and commas in Python.
Method 1: Using a for loop and the end parameter in the print function
The simplest approach to print out a list without brackets and commas is by using a for loop and the end parameter in the print function. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
for element in my_list:
print(element, end=’ ‘)
“`
Output:
“`
1 2 3 4 5
“`
In this method, we iterate over each element of the list using a for loop. Then, we print each element followed by a space character. By setting the `end` parameter of the print function to a space character (‘ ’), we ensure that each element is printed on the same line with a space separating them.
Method 2: Using the join() method
Another way to print out a list without brackets and commas is by using the join() method. The join() method is a string method that concatenates all elements of a list into a single string. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(‘ ‘.join(map(str, my_list)))
“`
Output:
“`
1 2 3 4 5
“`
In this method, we use the map() function to convert each element of the list to a string, as the join() method requires all elements to be strings. Then, we use the ‘ ‘.join() syntax to concatenate all elements with a space character as the separator.
Method 3: Using the * operator and the print function
Python provides the * operator to unpack elements from a list or any iterable. We can use this operator along with the print function to print out a list without brackets and commas. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(*my_list)
“`
Output:
“`
1 2 3 4 5
“`
In this method, we use the * operator before the list name in the print function. It tells Python to unpack the elements of the list and print them as separate arguments, effectively printing them without brackets and commas.
FAQs:
Q1. Can I use these methods to print out a list of strings?
Answer: Yes, these methods work for lists containing any type of elements, including strings, integers, floats, or even custom objects.
Q2. What happens if the list is empty?
Answer: If the list is empty, all the methods will result in no output.
Q3. Is there a way to preserve the original list formatting while printing?
Answer: Yes, if you want to preserve the original formatting of the list, you can convert it to a string using the str() function and print it without any modifications.
Q4. Can I use these methods to print out a nested list?
Answer: Yes, these methods can be used to print out nested lists as well. However, the output might not be easy to read, especially when the nesting level is deep.
Q5. Are there any other ways to print out a list without brackets and commas in Python?
Answer: Yes, there are several other approaches you can explore to achieve the same result. Some other methods involve using list comprehensions, string formatting syntax, or even external libraries.
In conclusion, printing out a list without brackets and commas in Python can be accomplished using various methods, such as using a for loop and the end parameter, the join() method, or the * operator. Each method has its own advantages and can be tailored to suit different use cases. By choosing the right method, you can ensure a clean and concise representation of your lists in Python.
Keywords searched by users: python list without commas How to print list without brackets Python, Remove square brackets from list Python, Remove comma in List Python, Print list Python, Array to string java without brackets, How to print list in python with brackets
Categories: Top 48 Python List Without Commas
See more here: nhanvietluanvan.com
How To Print List Without Brackets Python
Printing a list without brackets is a common requirement in Python programming. While printing a list with brackets is the default behavior of the print() function, there are several techniques you can use to remove the brackets and present the list elements in a more readable format.
In this article, we will explore different methods to print a list without brackets, giving you the flexibility to choose the approach that suits your needs. We will also answer some frequently asked questions regarding this topic.
Method 1: Using the join() function
One way to remove the brackets while printing a list is by using the join() function. The join() function takes a separator string as an argument and concatenates all the elements of the list into a single string. By specifying an empty string as the separator, the elements are concatenated without any delimiter.
Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
print(‘ ‘.join(my_list))
“`
Output:
“`
apple banana cherry
“`
Method 2: Using the str() function and slicing
Another technique to print a list without brackets is by converting each element of the list into a string and concatenating them using the + operator. By slicing the resulting string, we can remove the brackets.
Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
print(str(my_list)[1:-1])
“`
Output:
“`
‘apple’, ‘banana’, ‘cherry’
“`
Method 3: Using the * operator and sep parameter
The print() function in Python provides a sep parameter that specifies the separator between the elements while printing. By setting the sep parameter to an empty string, the default separator (which is a space) is overridden, resulting in the removal of brackets.
Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
print(*my_list, sep=”)
“`
Output:
“`
applebananacherry
“`
Method 4: Using a loop
If you prefer a more traditional approach, you can iterate over the list and print each element using a loop. By specifying an empty string as the end parameter in the print() function, each element is printed on the same line.
Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
for element in my_list:
print(element, end=”)
“`
Output:
“`
applebananacherry
“`
FAQs
Q1: Why should I print a list without brackets?
A1: Printing a list without brackets can make the output more readable, especially when dealing with large lists or when the brackets are unnecessary for the context in which the list is presented.
Q2: Can I remove the brackets permanently from a list?
A2: No. The brackets are an essential part of the list’s syntax and are required for proper representation and interpretation of the list in Python. Removing them permanently would alter the structure of the list.
Q3: How do I print a list without any delimiter between elements?
A3: You can use the join() function with an empty string as the separator, or the * operator with the sep parameter set to an empty string in the print() function to print a list without any delimiter between elements.
Q4: Are there any limitations to these methods?
A4: The methods described in this article work perfectly for most cases. However, it’s important to note that they assume the list elements are convertible to strings. If the elements are of complex types, you may need to customize the approach accordingly.
Q5: Can I use these methods to print lists of numbers or other data types?
A5: Absolutely! These methods can be used to print lists of any data type, including numbers, strings, or custom objects. Just make sure the elements are formatted appropriately for the desired output.
In conclusion, Python offers multiple ways to print a list without brackets. Whether you prefer using the join() function, slicing, manipulating the print() function’s parameters, or employing loops, you now have the tools to present your lists in a way that suits your specific requirements.
Remove Square Brackets From List Python
Python is a versatile programming language widely used for web development, data analysis, and automation tasks. One of its most powerful features is its ability to work with different data structures, such as lists. Lists in Python are ordered collections of items, enclosed in square brackets and separated by commas. However, in some cases, you may find the need to remove the square brackets from a list and work with the items individually. In this article, we will explore various methods to remove square brackets from a list in Python, along with some frequently asked questions.
Method 1: Using String Manipulation Techniques
One straightforward approach to remove square brackets from a list is by converting the list into its string representation and then manipulating that string. Here’s an example:
“`
my_list = [1, 2, 3, 4, 5]
list_string = str(my_list)
list_string = list_string.replace(“[“, “”).replace(“]”, “”)
new_list = list_string.split(“, “)
print(new_list)
“`
In this method, we first convert the list `my_list` into a string using the `str()` function. Then, we use the `replace()` method to remove the opening and closing square brackets. After that, we split the string based on the comma and space (“, “) to obtain a new list without the square brackets.
Method 2: Utilizing List Comprehension
Another efficient way to remove square brackets from a list is by using list comprehension. List comprehension offers a concise and elegant way to manipulate lists in Python. Here’s an example of how to utilize list comprehension to achieve this:
“`
my_list = [1, 2, 3, 4, 5]
new_list = [item for item in my_list]
print(new_list)
“`
In this method, we create a new list `new_list` using list comprehension, where we assign each item in `my_list` to the variable `item`. The resulting list will not have the square brackets. Although this method might seem redundant, it can be useful when performing additional computations or transformations on the list elements.
Method 3: Removing Square Brackets from List Output
If you wish to remove square brackets from the output of a list, you can employ the `join()` method to concatenate the elements of the list into a string. Here’s an example:
“`
my_list = [1, 2, 3, 4, 5]
output_string = ‘, ‘.join(map(str, my_list))
print(output_string)
“`
In this method, we use the `map()` function to convert each item in the original list to a string. Then, we use the `join()` method to concatenate the elements with a comma and space separator. The resulting output will be a string without any square brackets.
Frequently Asked Questions (FAQs):
Q: Can I remove square brackets using the `str()` function alone?
A: No, the `str()` function will convert the list into a string but will retain the square brackets. Additional string manipulation methods, like `replace()`, are required to remove them.
Q: Why would I want to remove square brackets from a list?
A: There can be various reasons for removing square brackets from a list. It could be for aesthetic purposes when displaying the list’s output, or when you want to work with the list’s individual elements separately.
Q: Can I remove square brackets from a nested list?
A: Yes, the methods mentioned above will work for nested lists as well. However, you will need to apply them recursively if you want to remove square brackets from all levels of nesting.
Q: Does removing square brackets change the data type of the list?
A: No, removing square brackets does not change the data type of the list. The list remains a list; it’s just the representation of the list that changes.
In conclusion, Python provides multiple ways to remove square brackets from a list. Whether it’s through string manipulation techniques, list comprehension, or manipulating the list’s output, you can customize your solution based on your specific requirements. By being familiar with these methods, you can enhance your Python programming skills and effectively work with lists in various scenarios.
Remove Comma In List Python
If you have ever worked with lists in Python, you might have come across situations where you need to remove a comma from a list. The Python programming language provides simple and efficient ways to achieve this. In this article, we will explore different methods to remove a comma in a list using Python, along with some related concepts.
When dealing with lists, it is essential to understand what they are in Python. A list is a versatile data structure that can hold multiple values of any type. Elements in a list are enclosed within square brackets and separated by commas. By default, each element is separated by a comma, which sometimes needs to be removed for specific use cases.
Method 1: Using the join() function
One of the most straightforward ways to remove a comma from a list is to use the join() function. This function takes a list as an argument and concatenates its elements into a single string. You can specify the separator you want to use between the elements by passing it as an argument to the join() function. To remove the comma, you can use an empty string as the separator. Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
result = ”.join(my_list)
print(result)
“`
Output:
“`
applebananacherry
“`
In the above example, the join() function concatenates the elements of the list `my_list` without any separator, effectively removing the comma.
Method 2: Using string manipulation
Another approach to remove a comma from a list is by using string manipulation techniques. You can convert the list into a string representation and replace the comma with an empty string. Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
result = str(my_list).replace(‘,’, ”)
print(result)
“`
Output:
“`
[‘apple’ ‘banana’ ‘cherry’]
“`
In the above example, the list `my_list` is converted into a string using the `str()` function. Then, using the `replace()` function, we replace the comma with an empty string. This approach can be useful if you want to keep the square brackets around the elements.
Frequently Asked Questions (FAQs):
Q1. Can I remove the comma only between specific elements in the list?
Yes, you can remove the comma only between specific elements in the list by using these methods. Before applying any of the methods mentioned above, you can manipulate the list or its elements to meet your requirements.
Q2. Can I remove the comma from a nested list?
Yes, the aforementioned methods can also be used to remove the comma from a nested list. You need to apply the methods to each nested list separately.
Q3. How can I remove the comma without altering the original list?
The methods explained here return a modified string representation of the list. If you want to keep the original list intact, you can assign the modified string to a new variable or use it as per your requirement, without affecting the original list.
Q4. Can I remove the comma from a list of integers or other non-string elements?
Yes, the methods mentioned above can be used to remove the comma from lists containing any type of element, including integers, floats, and non-string objects.
Q5. Are there any other methods to remove the comma from a list?
While the join() function and string manipulation are commonly used approaches, there may be alternate methods available. However, these two methods are efficient and widely used due to their simplicity and effectiveness.
In conclusion, removing a comma from a list in Python is a simple task that can be accomplished using the join() function or string manipulation techniques. Understanding these methods enables you to manipulate list elements in a way that suits your specific requirements. By employing the techniques discussed in this article, you can become more proficient in working with lists and easily remove commas when necessary.
Images related to the topic python list without commas
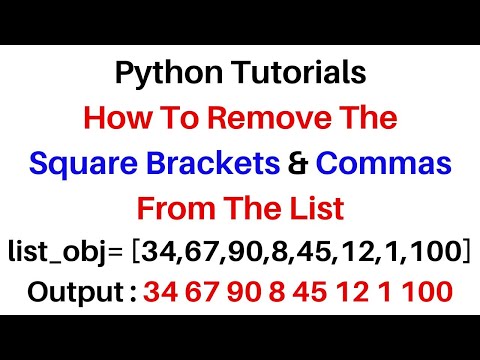
Found 28 images related to python list without commas theme


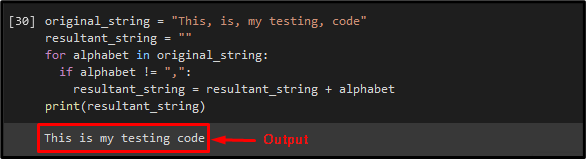


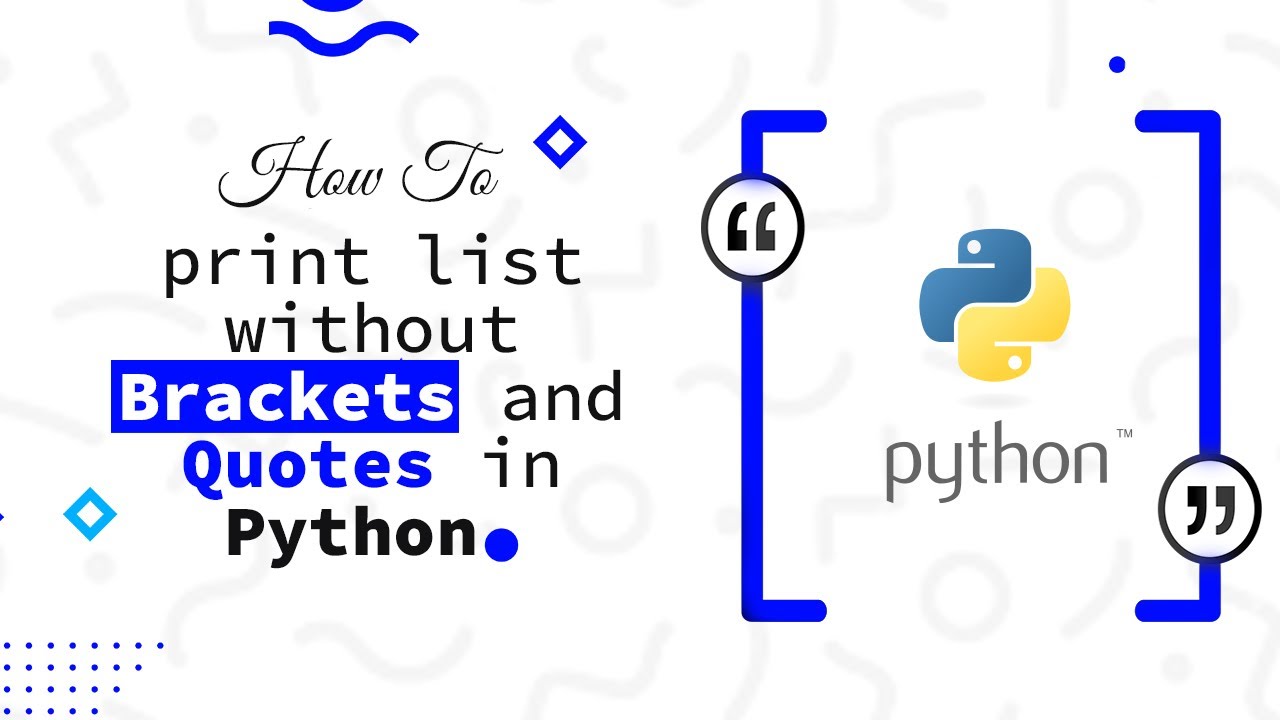
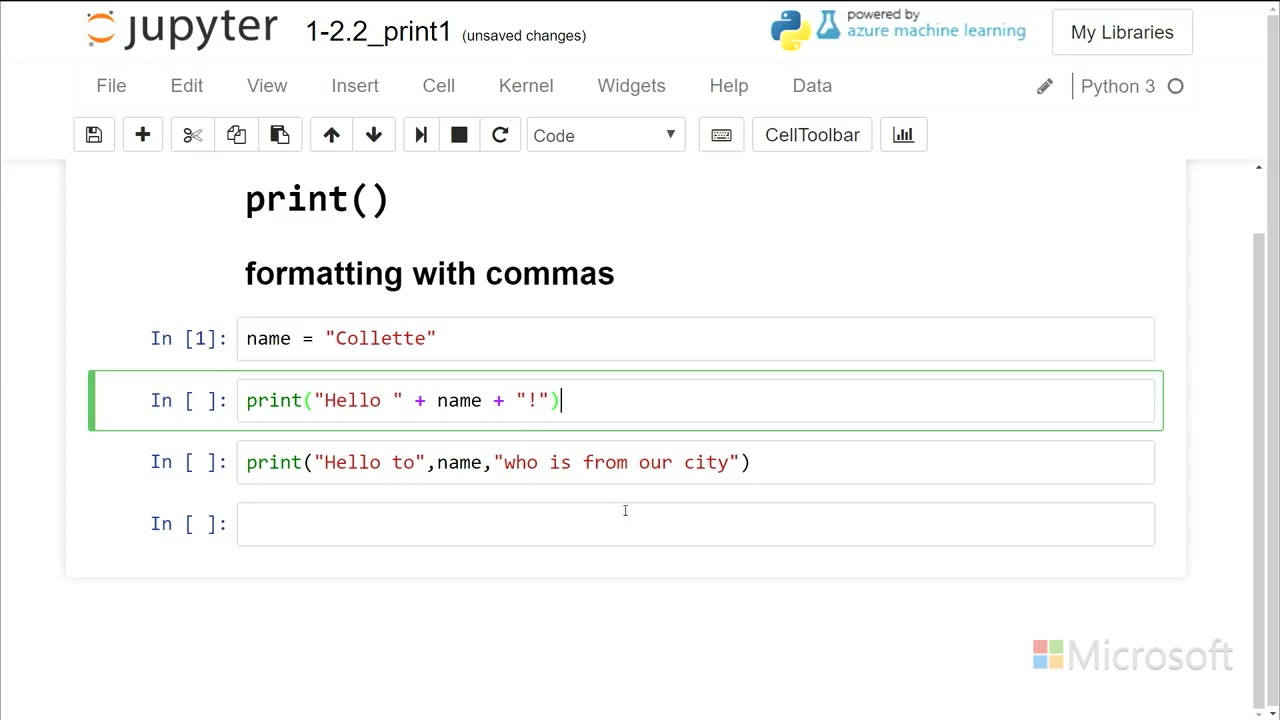
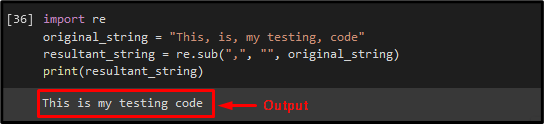
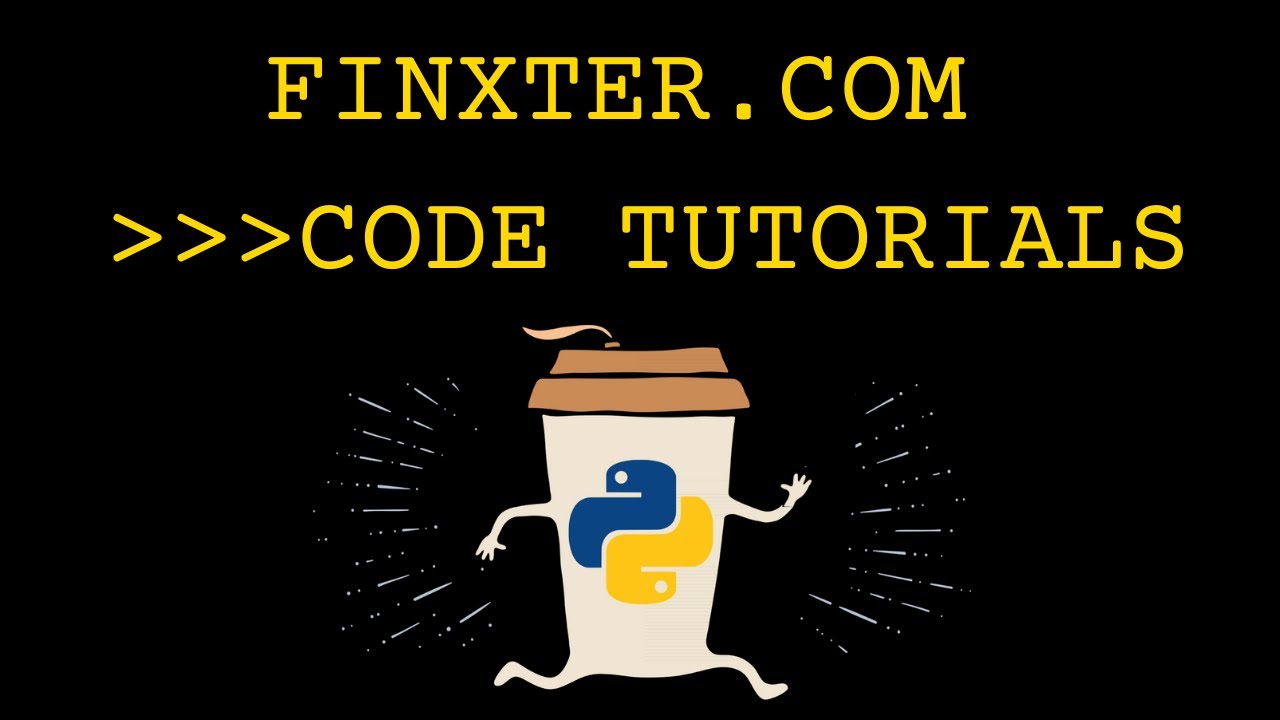
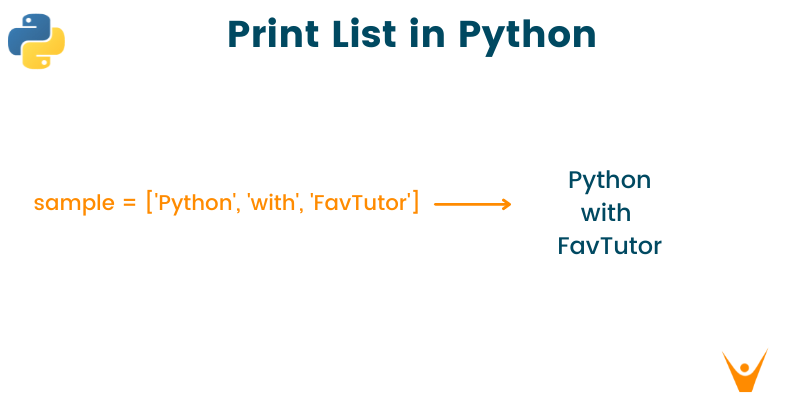
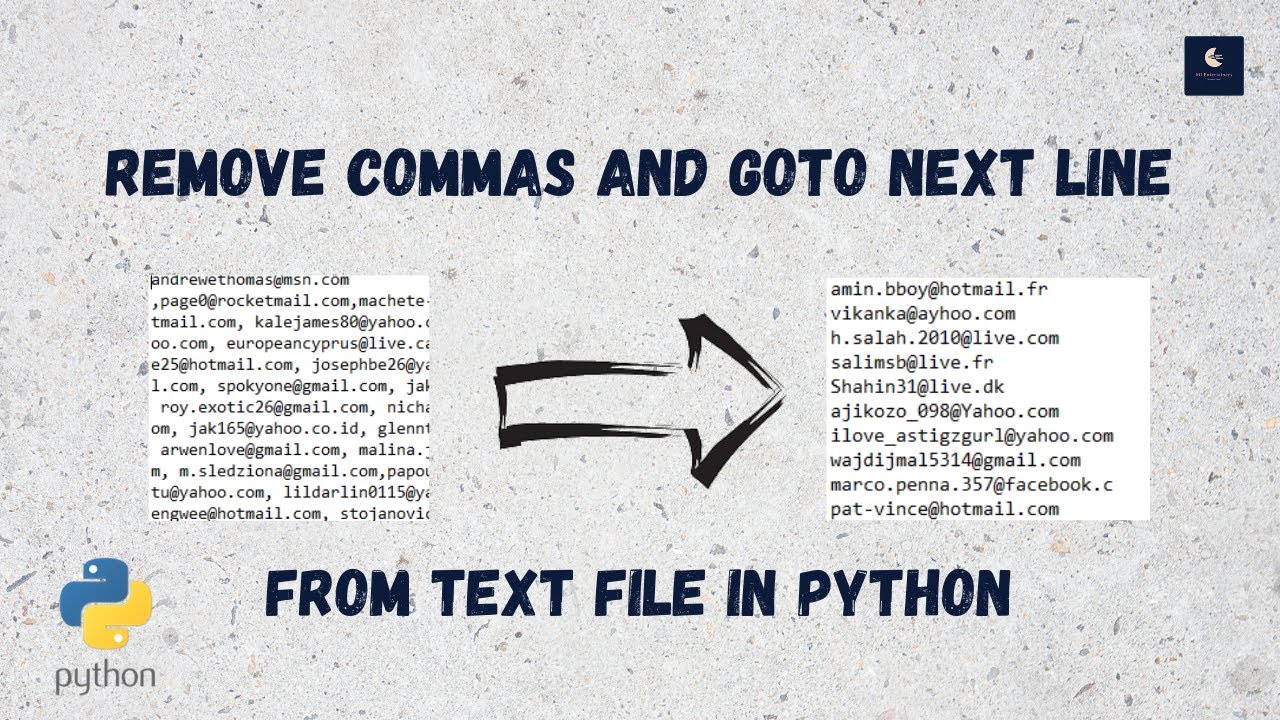
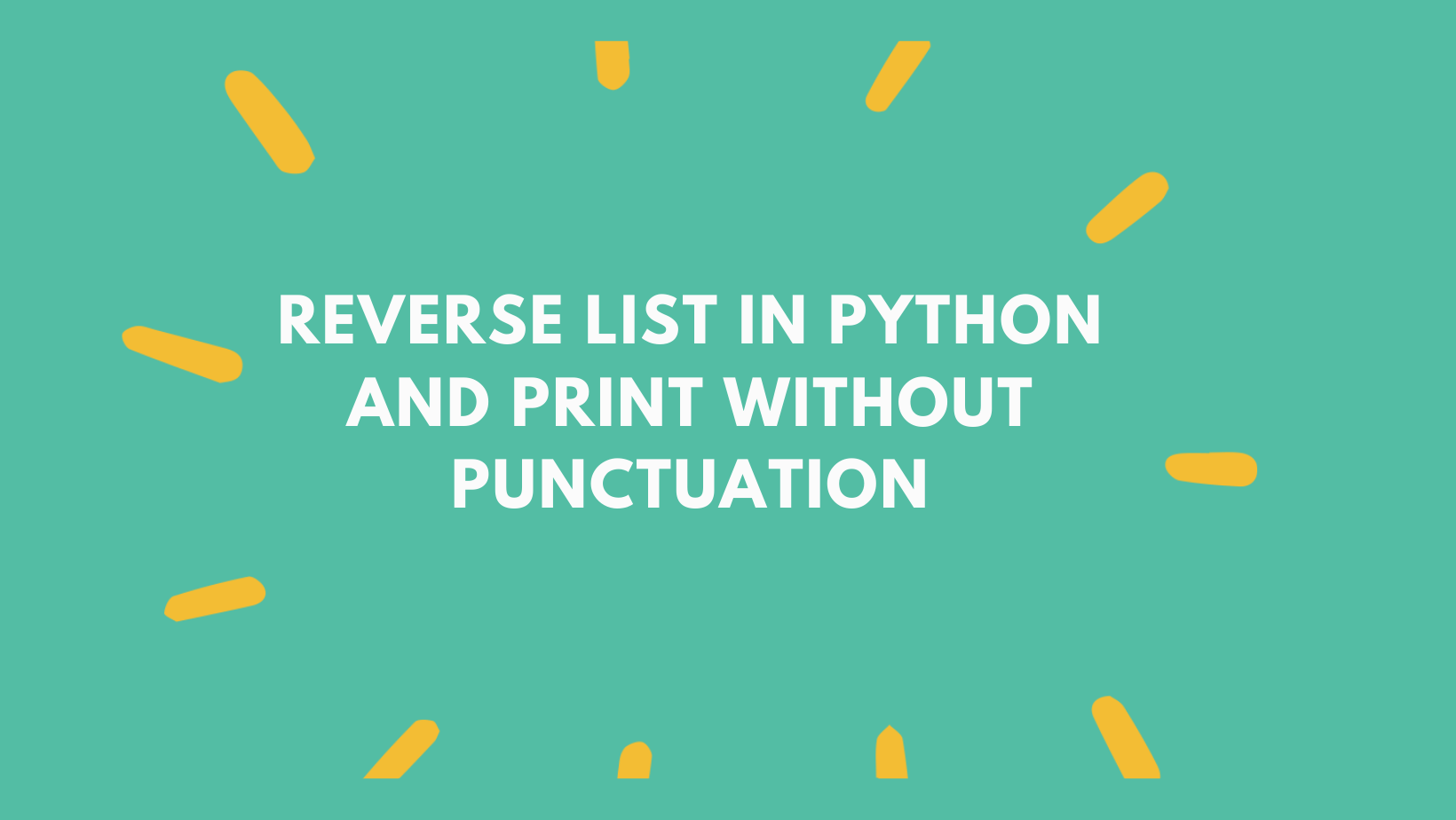
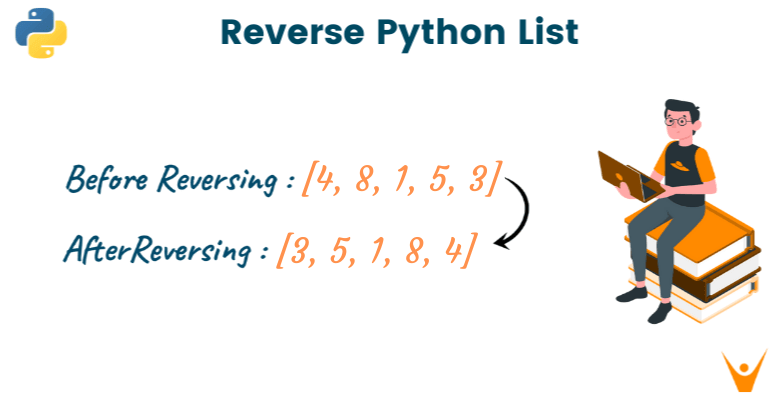
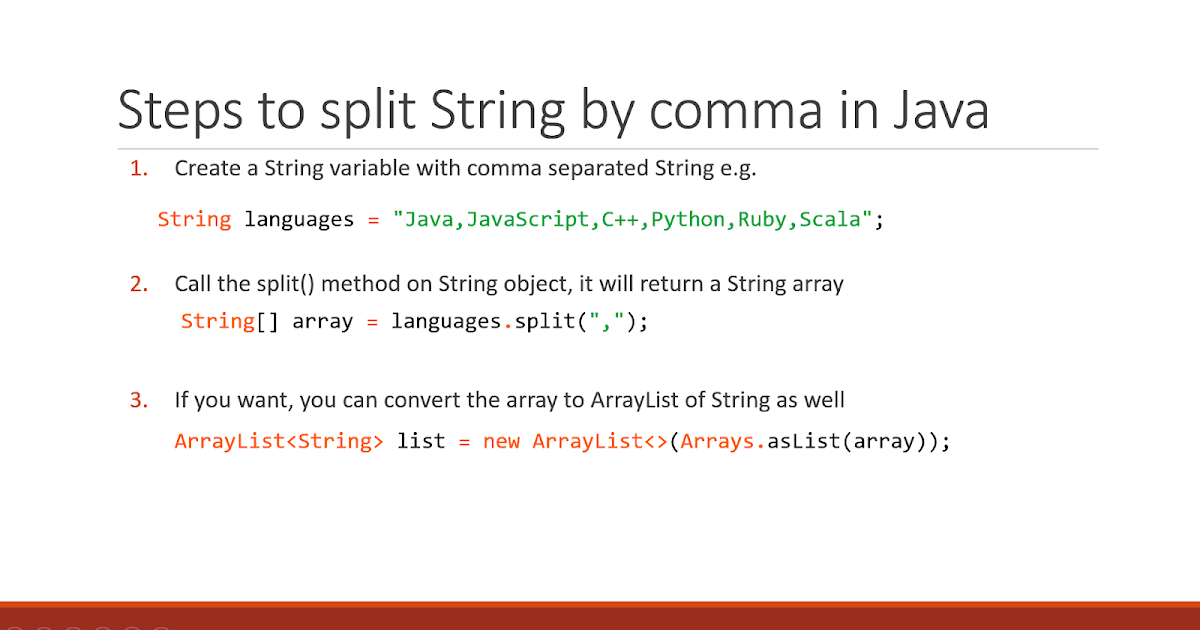


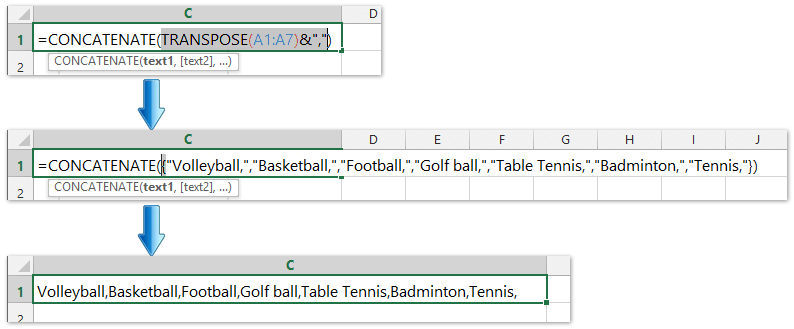
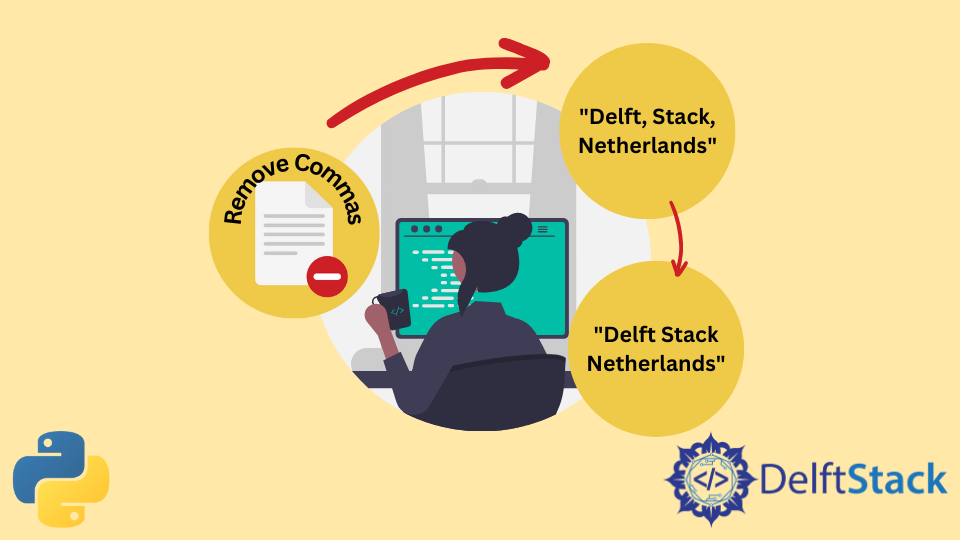
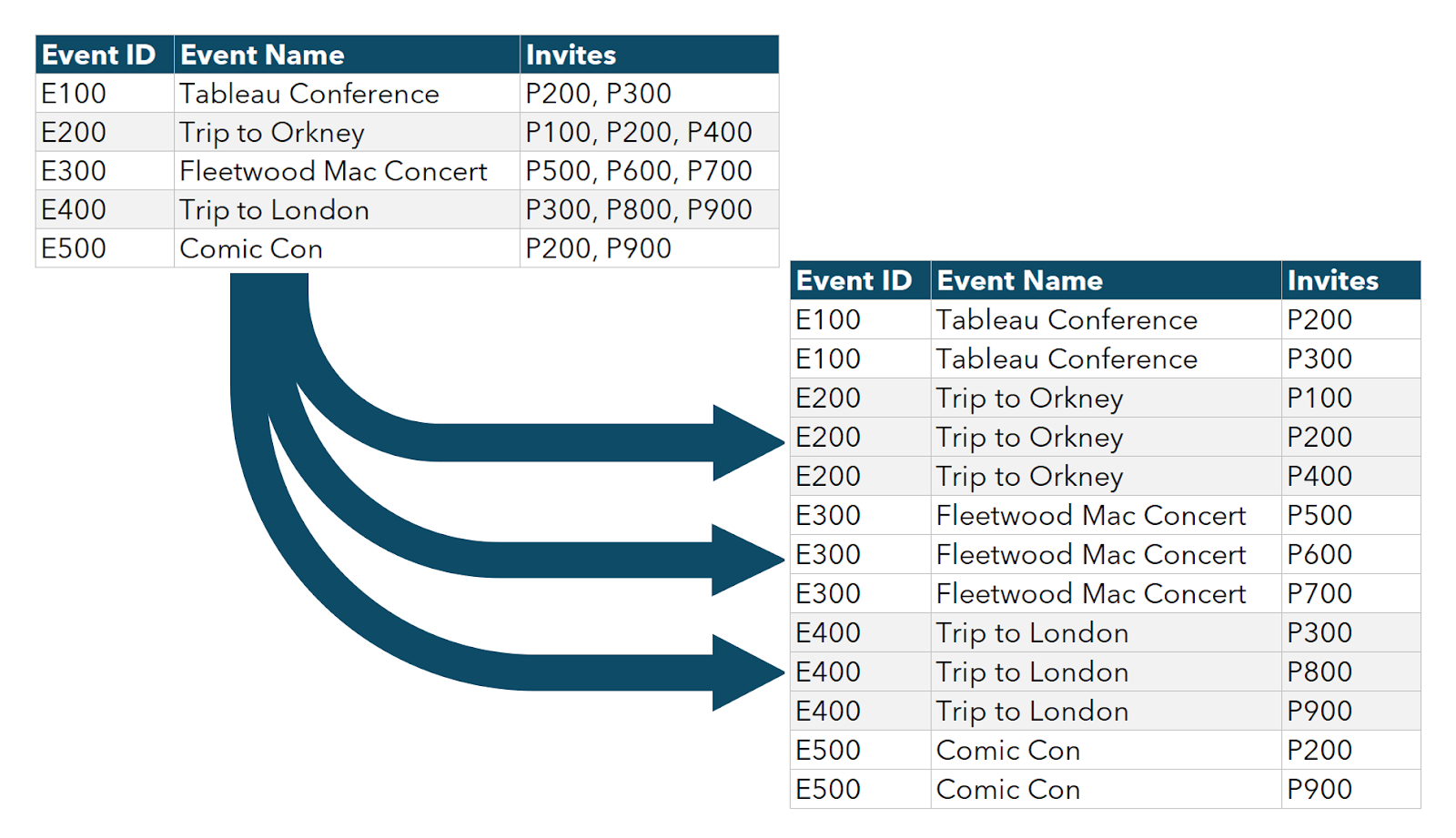
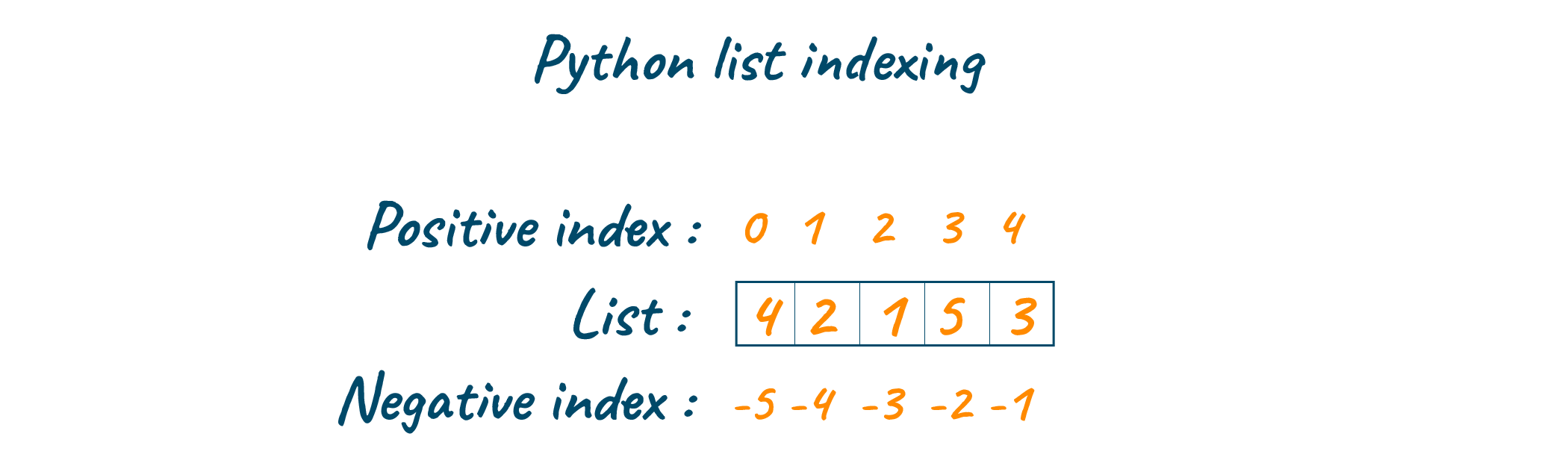

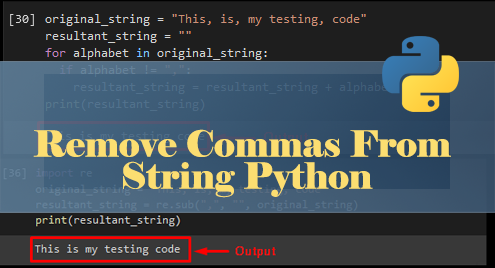
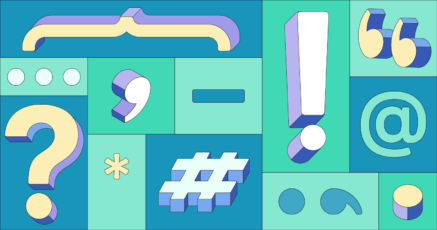
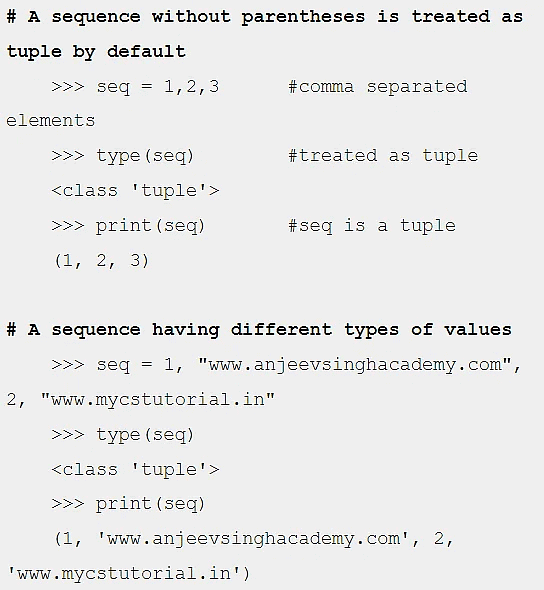


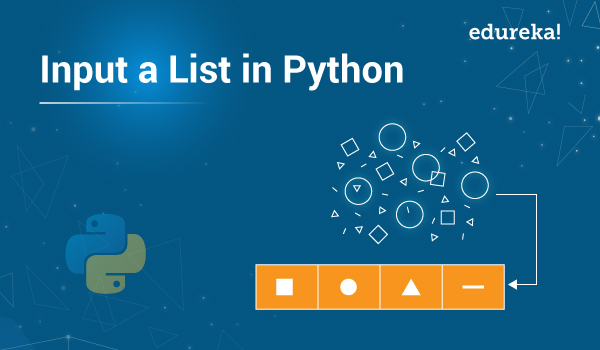


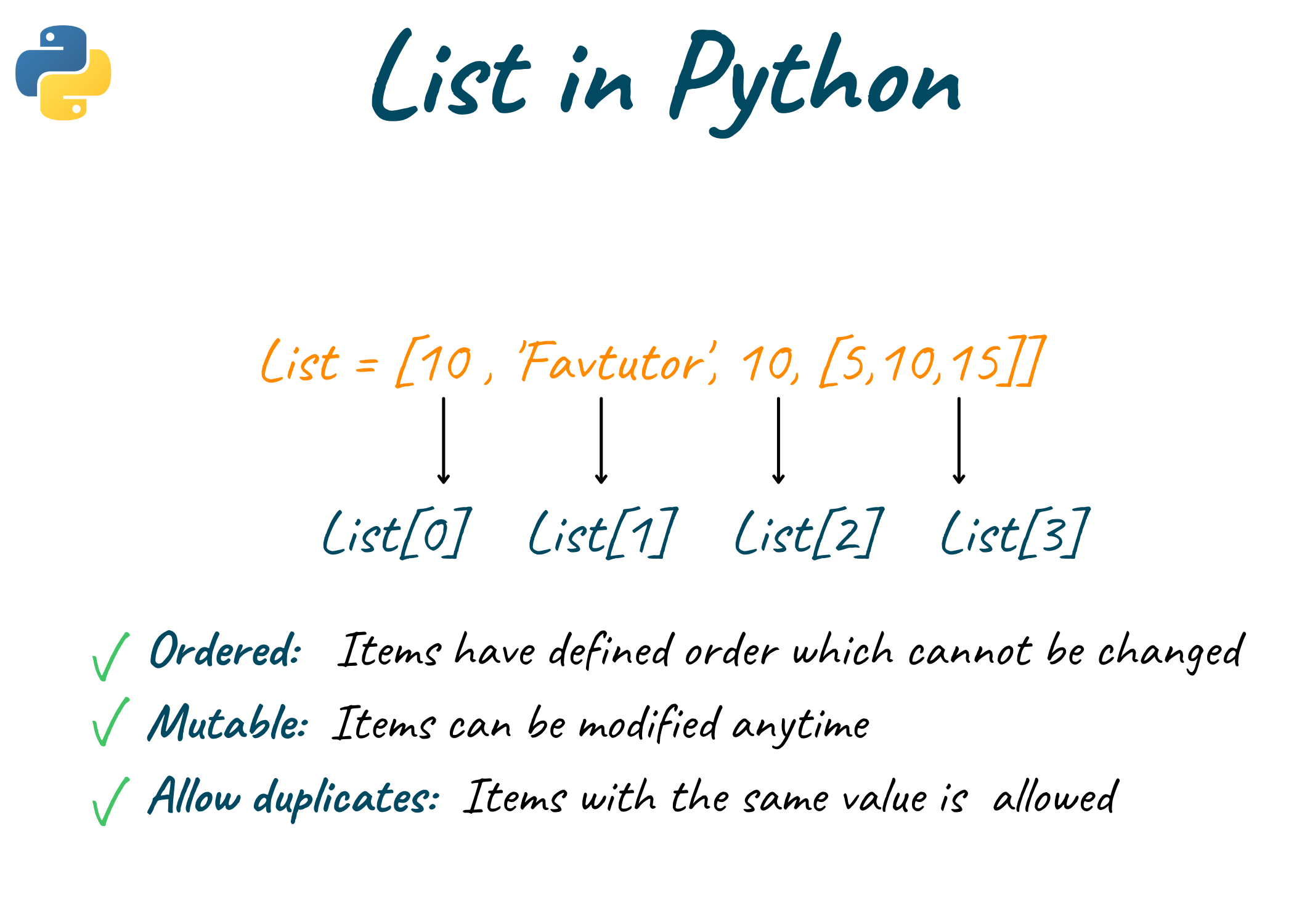

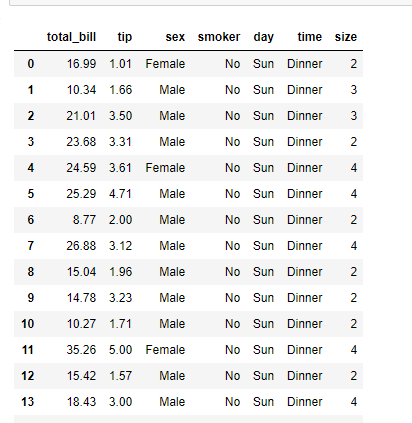
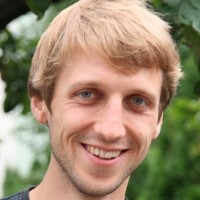

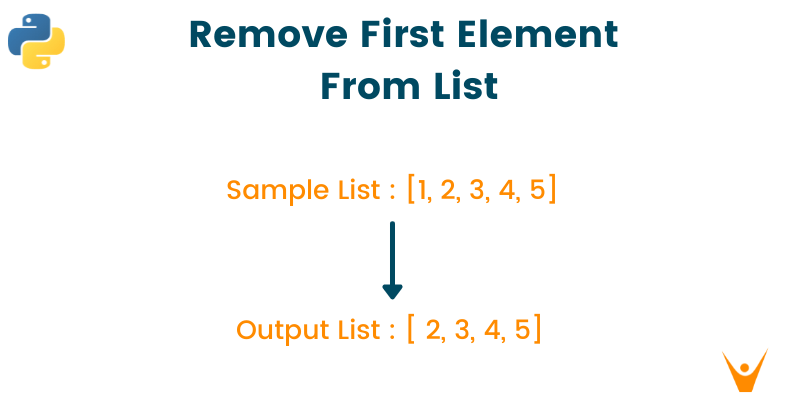

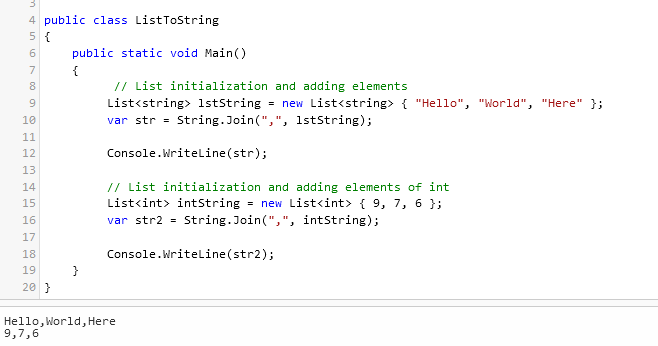
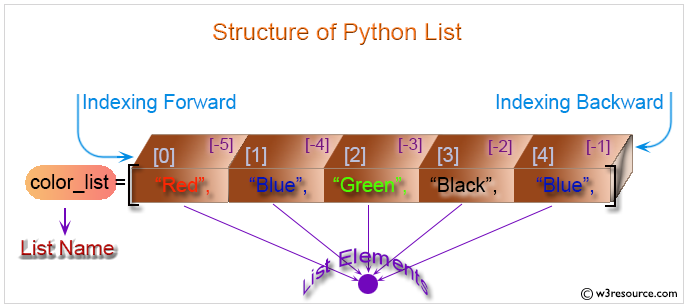
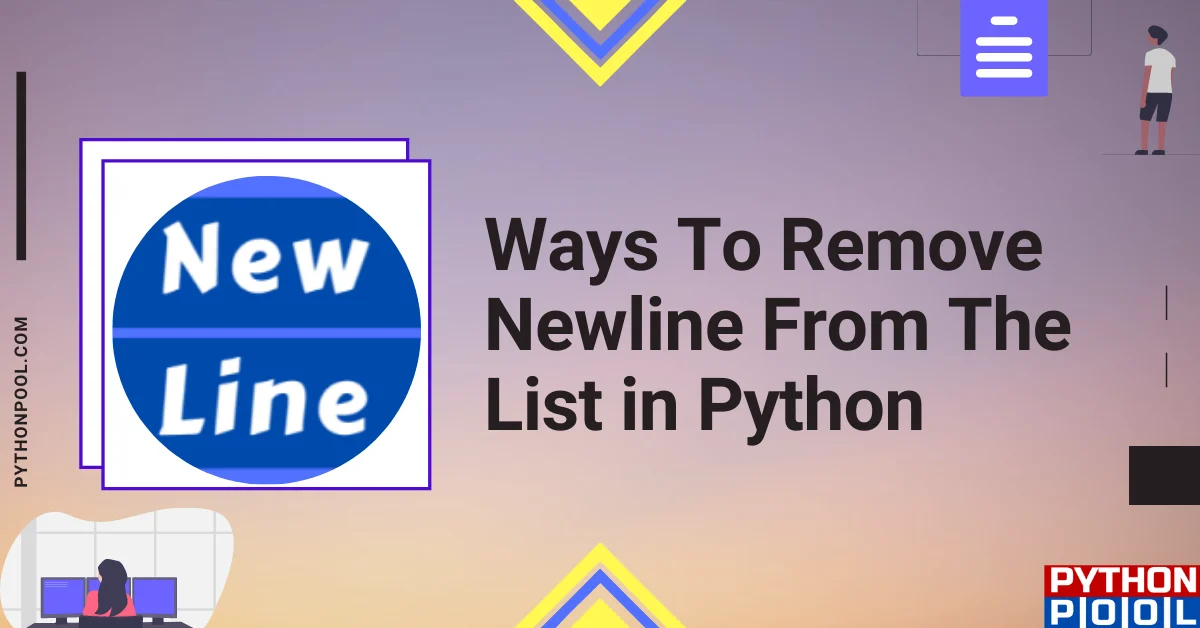

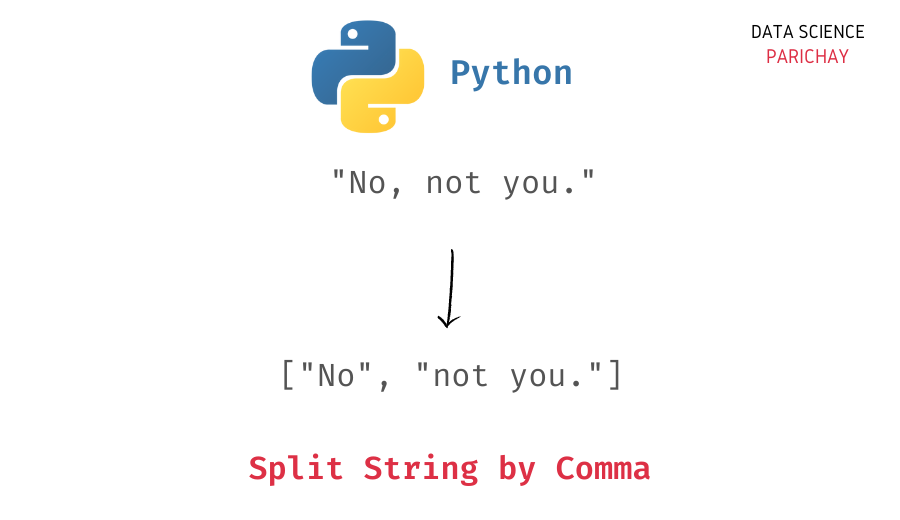
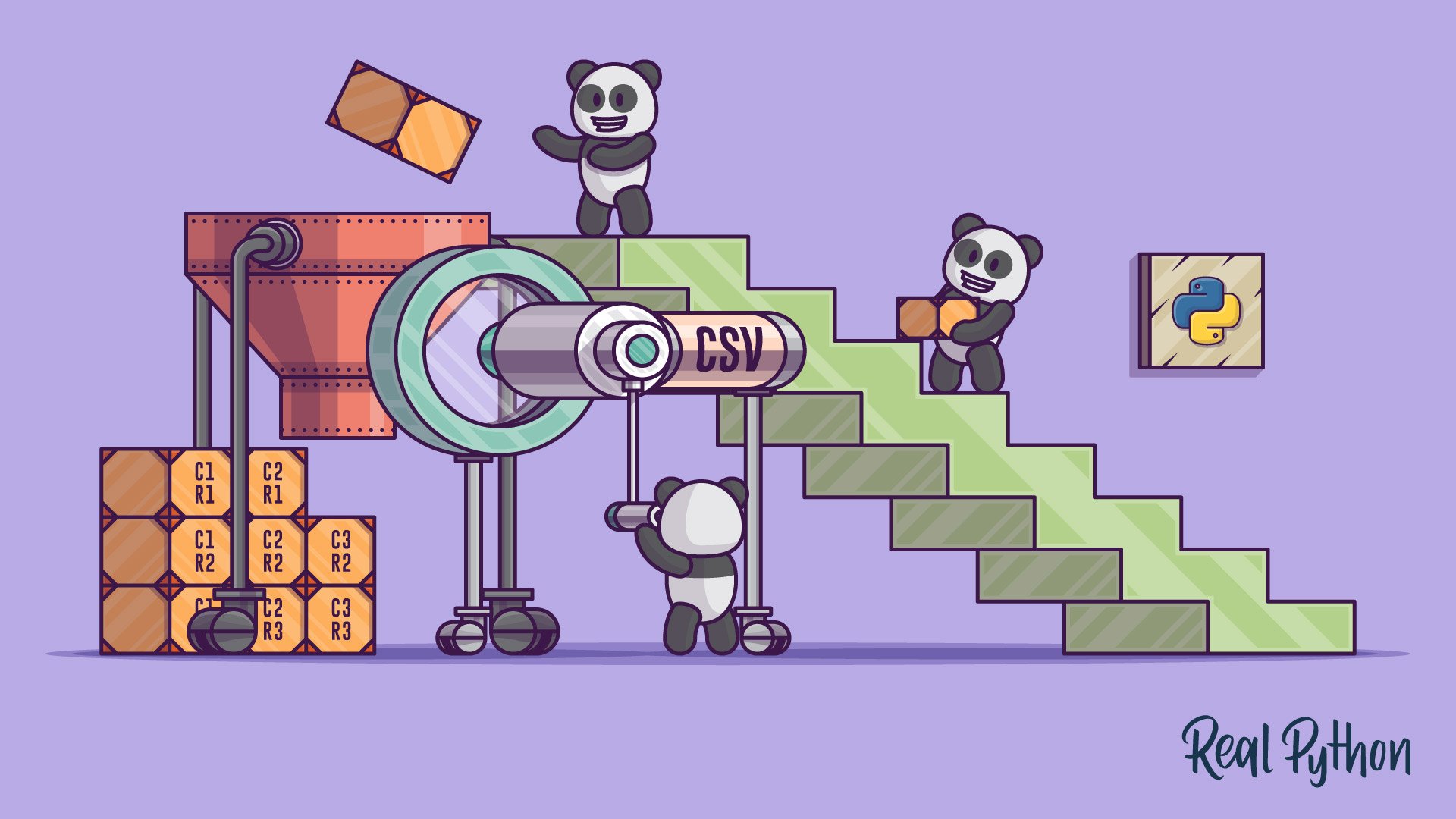
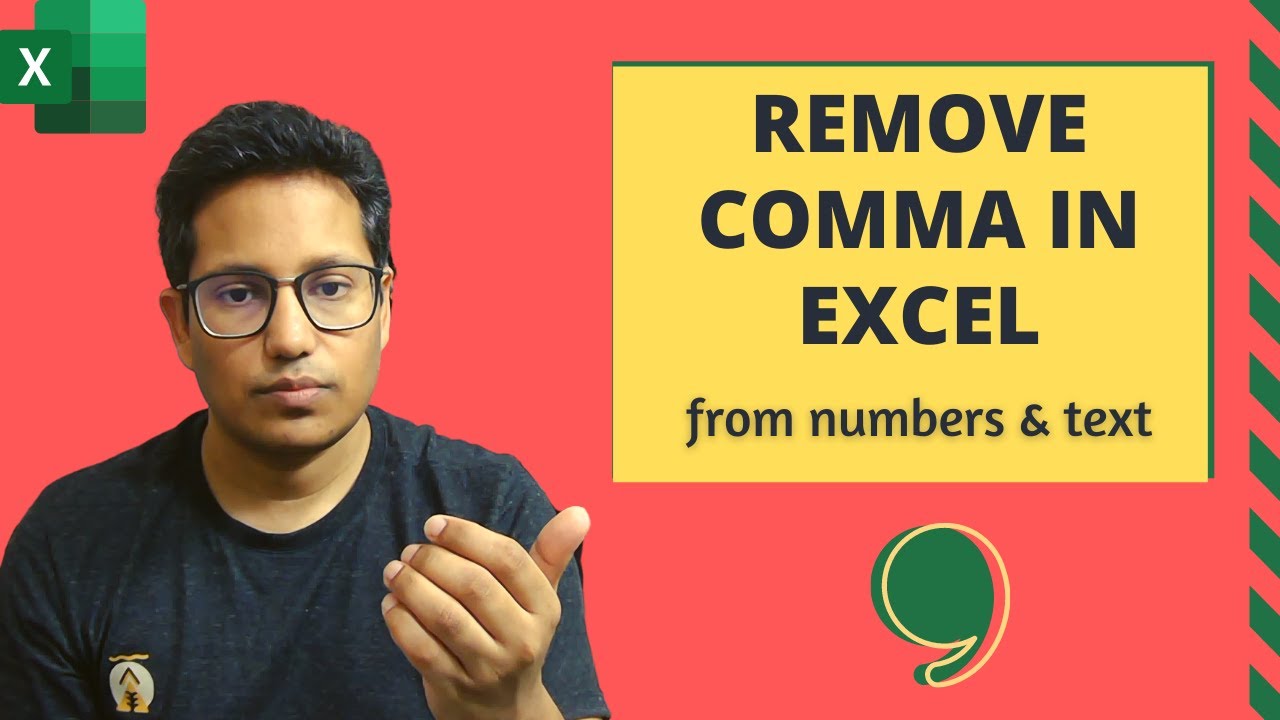
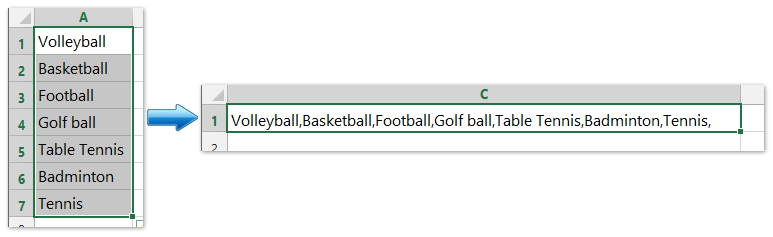
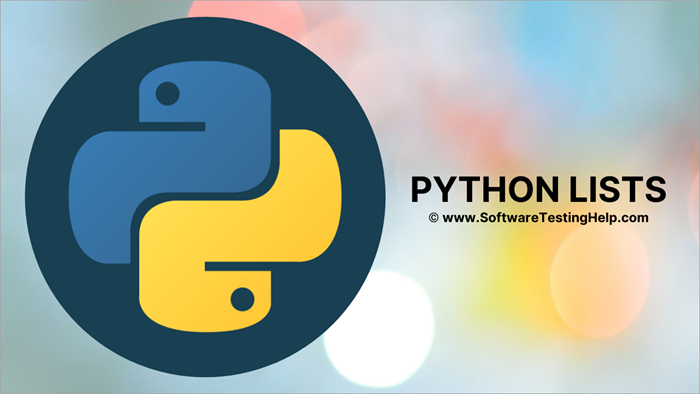
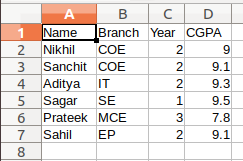


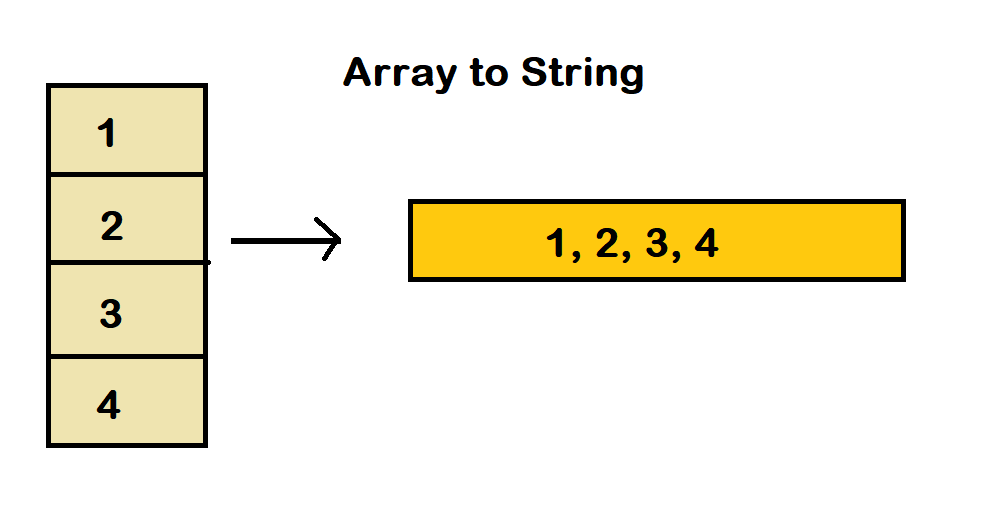
Article link: python list without commas.
Learn more about the topic python list without commas.
- Print a List without the Commas and Brackets in Python
- python – convert a list to an array, out put has no comma – Stack Overflow
- How to Print a List Without Brackets in Python – Javatpoint
- How to print a list with integers without the brackets, commas …
- How to Print a List Without Square Brackets in Python – Entechin
- Issue 43180: Lists without commas? – Python tracker
- How to Print a List Without Brackets in Python – Javatpoint
- How to print a list without brackets and commas in Python
See more: https://nhanvietluanvan.com/luat-hoc