Python Make Directory If Not Exists
Python provides various methods and modules to handle directories and files efficiently. One common requirement is to create a directory if it doesn’t already exist. In this article, we will explore different approaches to achieve this in Python and discuss best practices for handling directory creation.
Checking if a Directory Exists in Python:
Before creating a directory, it is essential to check if it already exists. Python offers multiple approaches to determine the existence of a directory.
Using the `os` Module to Check for the Existence of a Directory:
The `os` module in Python provides functions to interact with the operating system. To check if a directory exists, we can use the `os.path.exists()` function. This function takes a directory path as input and returns `True` if the directory exists; otherwise, it returns `False`.
“`python
import os
directory = ‘/path/to/directory’
if os.path.exists(directory):
print(“Directory already exists!”)
else:
print(“Directory does not exist!”)
“`
Creating a Directory in Python if It Doesn’t Already Exist:
If the directory doesn’t exist, we can create it using Python’s `os.makedirs()` function. This function recursively creates all the necessary directories to ensure the complete path exists.
“`python
import os
directory = ‘/path/to/directory’
if not os.path.exists(directory):
os.makedirs(directory)
print(“Directory created!”)
else:
print(“Directory already exists!”)
“`
Understanding the `os.path.exists()` Function:
The `os.path.exists()` function checks if a file or directory exists at the specified path. It returns `True` for both directories and files. To determine if the path represents a directory, we can use the `os.path.isdir()` function.
“`python
import os
path = ‘/path/to/directory’
if os.path.exists(path):
if os.path.isdir(path):
print(“Directory exists!”)
else:
print(“Path exists but is not a directory!”)
else:
print(“Directory does not exist!”)
“`
Making Use of the `os.makedirs()` Function to Create a Directory:
Python’s `os.makedirs()` function is a convenient way to create a directory and its parent directories if they don’t already exist. It automatically creates the entire directory tree specified in the given path.
“`python
import os
directory = ‘/path/to/directory’
os.makedirs(directory, exist_ok=True)
print(“Directory created!”)
“`
The `exist_ok=True` parameter ensures that the function doesn’t raise an exception if the directory already exists. It enables us to avoid handling exceptions explicitly.
Handling Exceptions When Creating a Directory:
In some cases, it might be necessary to handle exceptions manually when creating directories. The `os.makedirs()` function can raise an `OSError` if it encounters any issues during the directory creation process.
“`python
import os
directory = ‘/path/to/directory’
try:
os.makedirs(directory)
print(“Directory created!”)
except OSError as exception:
print(f”Failed to create directory: {exception}”)
“`
Using the `Path` Module from the `pathlib` Package:
Python’s `pathlib` module provides an object-oriented approach for handling paths. With the `Path` module, we can check directory existence and create directories using the `mkdir()` function.
“`python
from pathlib import Path
directory = Path(‘/path/to/directory’)
if not directory.exists():
directory.mkdir(parents=True)
print(“Directory created!”)
else:
print(“Directory already exists!”)
“`
Exploring the `os.mkdir()` Function for Directory Creation:
The `os.mkdir()` function is an alternative to `os.makedirs()` when we only need to create a single directory and not its parent directories. It raises an error if the parent directory doesn’t exist.
“`python
import os
directory = ‘/path/to/directory’
try:
os.mkdir(directory)
print(“Directory created!”)
except OSError as exception:
print(f”Failed to create directory: {exception}”)
“`
Recap and Best Practices for Making Directories in Python:
– To check if a directory exists, use `os.path.exists()` or `pathlib.Path.exists()`.
– To create a directory, use `os.makedirs()` or `pathlib.Path.mkdir()`. Enable `exist_ok=True` to avoid exceptions.
– Handle exceptions when necessary, especially when dealing with potential errors during directory creation.
– Use `os.mkdir()` to create a single directory if the parent directories already exist.
– Follow the best practice of checking and creating directories to ensure proper directory handling in your Python programs.
FAQs:
1. How can I create a directory if it doesn’t exist in Python?
You can use the `os.makedirs()` function or the `pathlib.Path.mkdir()` function to create a directory if it doesn’t exist. Both functions ensure that the entire directory tree is created if necessary.
2. What is the difference between `os.makedirs()` and `os.mkdir()`?
`os.makedirs()` creates the specified directory and its parent directories if they don’t already exist. In contrast, `os.mkdir()` only creates the specified directory and raises an error if the parent directory doesn’t exist.
3. How can I check if a directory exists in Python?
You can use the `os.path.exists()` function or the `pathlib.Path.exists()` function to check if a directory exists in Python. Both functions return `True` if the directory exists and `False` otherwise.
4. How can I handle exceptions when creating a directory in Python?
You can use a `try-except` block to handle exceptions that may occur while creating a directory. Catch the `OSError` exception and handle it according to your specific requirements.
5. Should I use `os.makedirs()` or `pathlib.Path.mkdir()` to create directories in Python?
Both functions serve the purpose of creating directories. Choose the one that suits your needs and coding style. It is recommended to use the `pathlib` module for new projects as it provides an object-oriented approach.
Create Directory If Not Exist In Python 😀
How To Check If A Folder Exists And Make It If Not In Python?
In the world of computer programming, organizing files and directories is an essential task. Often, you may find yourself needing to check if a folder exists and, if it doesn’t, create it programmatically. Python, being a versatile and powerful scripting language, provides a straightforward way to accomplish this task. In this article, we will explore different methods to check for the existence of a folder and create it if it doesn’t exist. So let’s dive in!
## Checking Folder Existence
Before creating a folder programmatically, it is crucial to ensure that it doesn’t already exist. Python provides various methods to perform this check.
### Method 1: os.path.exists()
One simple way to check if a folder exists is by using the `os.path.exists()` function. This function accepts a path as input and returns `True` if it exists and `False` otherwise.
“`python
import os
path = ‘/path/to/folder’ # Replace with actual folder path
if os.path.exists(path):
print(“Folder exists!”)
else:
print(“Folder doesn’t exist.”)
“`
### Method 2: os.path.isdir()
While `os.path.exists()` can check both files and directories, if you specifically want to verify the existence of a folder, you can use `os.path.isdir()`. This method returns `True` if the provided path corresponds to a directory, and `False` otherwise.
“`python
import os
path = ‘/path/to/folder’ # Replace with actual folder path
if os.path.isdir(path):
print(“Folder exists!”)
else:
print(“Folder doesn’t exist.”)
“`
### Method 3: pathlib.Path().is_dir()
With Python 3.4 onwards, the `pathlib` module provides an object-oriented approach to path manipulation. Using `Path().is_dir()` from `pathlib`, you can check if a folder exists.
“`python
import pathlib
path = pathlib.Path(‘/path/to/folder’) # Replace with actual folder path
if path.is_dir():
print(“Folder exists!”)
else:
print(“Folder doesn’t exist.”)
“`
## Creating a Folder Programmatically
Once you have ensured that the folder doesn’t exist, you can proceed to create it programmatically. Python offers various methods to accomplish this task.
### Method 1: os.makedirs()
The `os.makedirs()` function enables the creation of multiple folders along the provided path. It creates intermediate directories as needed.
“`python
import os
path = ‘/path/to/parent_folder/new_folder’ # Replace with actual folder path
os.makedirs(path, exist_ok=True)
“`
The `exist_ok=True` flag ensures that an exception is not raised if the folder already exists, preventing any unwanted errors.
### Method 2: pathlib.Path().mkdir()
The `pathlib` module also provides a convenient `Path().mkdir()` method to create a single directory. Similarly to `os.makedirs()`, it also handles the situation if the directory already exists.
“`python
import pathlib
path = pathlib.Path(‘/path/to/parent_folder/new_folder’) # Replace with actual folder path
path.mkdir(parents=True, exist_ok=True)
“`
Here, the `parents=True` flag ensures that any intermediate directories needed to create the new folder exist.
## Frequently Asked Questions
**Q1: Can I specify a relative path instead of an absolute path to check for folder existence?**
A1: Yes, you can use relative paths to check for folders. Python interprets relative paths based on the current working directory where the script is run.
**Q2: How can I create a folder with a specific name based on user input?**
A2: You can utilize Python’s `input()` function to prompt the user for input. The input can then be used to construct the folder name dynamically.
“`python
import os
folder_name = input(“Enter the desired folder name: “)
path = f’/path/to/parent_folder/{folder_name}’
os.makedirs(path, exist_ok=True)
“`
**Q3: What happens if I do not use `exist_ok=True` while creating a folder?**
A3: Without the `exist_ok=True` flag, if the folder you are trying to create already exists, a `FileExistsError` will be raised. Using the flag prevents this exception and allows for error-free execution.
**Q4: How can I handle any errors that occur during folder creation?**
A4: To handle exceptions that occur during folder creation, you can use a `try-except` block. By wrapping the folder creation code within a `try` block and specifying the appropriate exception in the `except` block, you can handle errors gracefully.
“`python
import os
path = ‘/path/to/folder’ # Replace with actual folder path
try:
os.makedirs(path, exist_ok=True)
print(“Folder successfully created!”)
except Exception as e:
print(f”Error occurred while creating the folder: {str(e)}”)
“`
In this example, any exception raised during folder creation will be caught, and a custom error message can be displayed.
Congratulations! You have learned different methods to check if a folder exists and make it if it doesn’t in Python. By utilizing functions from the `os` module and methods from the `pathlib` module, you can easily handle folder operations in your Python scripts. Keep experimenting and harnessing the power of Python to efficiently manage your files and directories!
Which Files Create Directory If Not Exist?
Creating directories is a crucial step when organizing and managing files on a computer or server. However, sometimes it becomes necessary to create directories programmatically, especially when dealing with large-scale file management tasks or automating processes. In such cases, it is essential to determine which files have the ability to create directories if they do not exist.
File creation operations typically rely on specific programming languages or command-line tools. Here, we will explore some of the most commonly used tools and methods that developers and system administrators utilize to create directories when necessary.
1. mkdir (Unix/Linux)
In Unix-based systems, the “mkdir” command is frequently used to create directories from the command line. By default, if a directory already exists with the specified name, the command will produce an error. However, the “-p” flag can be used to create parent directories if they don’t already exist. For example, running the command “mkdir -p /path/to/directory” will create the “directory” along with any missing parent directories.
2. os.makedirs (Python)
In Python, the “os.makedirs” function can be used to create directories. It creates all the intermediate directories as required. If a directory already exists, it throws an exception. Here is an example of creating a directory using Python:
“`python
import os
os.makedirs(‘/path/to/directory’, exist_ok=True)
“`
By setting the “exist_ok” parameter to “True,” Python will not raise an exception if the directory already exists.
3. FileSystem.CreateDirectory (C#)
In C#, the “FileSystem.CreateDirectory” method within the “System.IO” namespace is commonly employed to create directories. The method will create the specified directory if it does not already exist. If the path includes parent directories that are missing, the method will create those as well. Consider the following example:
“`csharp
using System.IO;
string directoryPath = “/path/to/directory”;
Directory.CreateDirectory(directoryPath);
“`
4. Files.createDirectories (Java)
In Java, the “Files.createDirectories” method from the “java.nio.file” package can be used to create directories. It creates directories along with any necessary parent directories. If the directory already exists, the method does nothing. Here is an example of creating a directory using Java:
“`java
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
Path directoryPath = Paths.get(“/path/to/directory”);
try {
Files.createDirectories(directoryPath);
} catch (IOException e) {
e.printStackTrace();
}
“`
5. PowerShell
PowerShell, a command-line shell and scripting language, provides the functionality to create directories using the “New-Item” cmdlet. By specifying the “-ItemType Directory” parameter, the command will create the directory if it does not already exist. Here is an example:
“`powershell
New-Item -ItemType Directory -Path “/path/to/directory” -Force
“`
FAQs:
Q1. Can I use these methods/tools to create directories on any operating system?
Yes, these methods/tools can be used on Unix/Linux, Windows, and Mac operating systems as they are compatible with various programming languages and command-line shells.
Q2. Is it necessary to have administrator privileges to create directories?
It depends on the access permissions of the parent directory. If the user has write permission in the parent directory, they can create a new directory within it. However, if the parent directory is restricted, like system directories, administrator privileges may be required.
Q3. What happens if I try to create a directory that already exists?
In most cases, attempting to create a directory that already exists will result in an error or an exception. However, some methods/tools provide options to handle this situation gracefully, such as Python’s “os.makedirs” with the “exist_ok” parameter set to “True”.
Q4. How can I handle errors or exceptions when creating directories programmatically?
Most programming languages offer ways to handle errors and exceptions. For example, in Python, you can use the “try-except” block to catch any exceptions thrown during directory creation and handle them accordingly.
Q5. Are there any limitations on directory names when using these methods/tools?
Yes, there are certain limitations on directory names depending on the operating system and file system conventions. Special characters, reserved filenames, and length restrictions may apply.
In conclusion, several programming languages and command-line tools offer convenient methods to create directories when necessary. Understanding how to use these tools effectively will enhance your ability to automate file management tasks and improve overall organization. Remember to test your code thoroughly and handle any potential errors or exceptions to ensure smooth execution.
Keywords searched by users: python make directory if not exists Os mkdir if not exists, Path mkdir if not exists, Python create file if not exists, Python copy file auto create directory if not exist, Create folder if it doesn t exist Python, Python check directory exists if not create, Os mkdir exist ok, Check folder exist Python
Categories: Top 76 Python Make Directory If Not Exists
See more here: nhanvietluanvan.com
Os Mkdir If Not Exists
Creating directories is a common task in programming, particularly when dealing with file systems. In many cases, it is essential to ensure that a directory does not already exist before creating it. This is where the “mkdir if not exists” command comes into play. In this article, we will explore the functionality, usage, and benefits of the “os mkdir if not exists” command, along with some frequently asked questions.
What is “os mkdir if not exists”?
The “os mkdir if not exists” command is a feature provided by operating systems that allows programmers to create a directory only if it does not already exist. It serves as a convenient way to avoid errors and conflicts that may arise when attempting to create duplicate directories. This command is widely used across various programming languages, including Python, JavaScript, Ruby, and many more.
How does “os mkdir if not exists” work?
When the “os mkdir if not exists” command is used, the operating system checks whether the requested directory already exists. If the directory is not found, the system creates it; otherwise, it does nothing. This process ensures that multiple attempts to create the same directory do not result in errors or overwrite existing directories unintentionally.
Usage of “os mkdir if not exists”:
The syntax for using “os mkdir if not exists” depends on the programming language and operating system being used. However, the underlying principle remains the same.
In Python, the “os” module provides various functions to interact with the operating system, including directory creation. Let’s take a look at an example using Python:
“`python
import os
directory = “path/to/directory”
# Check if directory exists, if not create it
if not os.path.exists(directory):
os.makedirs(directory)
print(“Directory created successfully.”)
else:
print(“Directory already exists.”)
“`
In this example, the “os.path.exists(directory)” function is used to verify if the directory already exists. If it doesn’t exist, “os.makedirs(directory)” creates the directory. Otherwise, it displays a message indicating that the directory is already present.
Benefits of “os mkdir if not exists”:
1. Error Prevention: By utilizing “os mkdir if not exists,” programmers can avoid errors that might occur when trying to create a directory that already exists. This helps maintain the integrity of the file system and prevents accidental data loss.
2. Simplified Logic: Without the “os mkdir if not exists” command, developers would need to manually check for the existence of directories before creating them. This command simplifies the logic and reduces the number of lines of code required.
3. Improved Code Readability: Using the “os mkdir if not exists” command makes the code more concise and easier to read. It clearly communicates the intention of the programmer, enhancing code maintainability and reducing confusion for future developers who might work on the codebase.
FAQs:
Q: What is the alternative to “os mkdir if not exists”?
A: The alternative to “os mkdir if not exists” is to manually check if the directory already exists using conditional statements. However, this method is less efficient and more prone to errors, especially in complex directory structures.
Q: Are there any limitations to using “os mkdir if not exists”?
A: While “os mkdir if not exists” is widely supported, it is essential to check the documentation for specific programming languages and operating system versions to ensure compatibility.
Q: Can “os mkdir if not exists” be used to create nested directories?
A: Yes, “os mkdir if not exists” can be used to create nested directories. The command will create all the required directories within the specified path if they don’t exist.
Q: Does “os mkdir if not exists” work recursively?
A: Yes, the “os mkdir if not exists” command can create directories recursively. It will create all the necessary parent directories in the specified path that don’t exist.
Q: Are there any security considerations when using “os mkdir if not exists”?
A: It is important to ensure that appropriate permissions and security measures are in place when creating directories using “os mkdir if not exists.” This prevents unauthorized access and potential security breaches.
In conclusion, the “os mkdir if not exists” command simplifies the process of creating directories in programming languages across different operating systems. By preventing duplicate creations and reducing the risk of errors, it enhances code reliability and maintainability. Understanding the usage and benefits of this command can greatly streamline directory creation tasks in your programming endeavors.
Path Mkdir If Not Exists
In the programming world, creating directories (folders) is a common task that developers often encounter. In Python, the Path.mkdir() method allows you to create a new directory. However, before creating a directory, it is essential to check whether it already exists. The Path.mkdir if not exists functionality ensures that the directory is only created if it does not already exist. This article dives deep into the concept of Path.mkdir if not exists in Python, covering its usage, benefits, potential issues, and FAQs.
Understanding the Path.mkdir() Method
Before delving into Path.mkdir if not exists, it is crucial to understand the basic functionality of Path.mkdir() in Python. The Path.mkdir() method is part of the pathlib module, introduced in Python 3.4, which provides an object-oriented approach for working with file system paths. It allows you to create directories using the path specified.
Using Path.mkdir() is relatively straightforward. You instantiate a Path object with the desired directory path and call the mkdir() function on it. The method creates a new directory at the specified path.
Path.mkdir() vs. Path.mkdir if not exists
By default, the Path.mkdir() method raises a FileExistsError if the directory already exists. This can lead to exceptions and disrupt the program flow. However, using the Path.mkdir if not exists functionality helps you avoid these interruptions.
Path.mkdir if not exists checks if the directory already exists before attempting to create it. If the directory exists, the method simply does nothing. If the directory does not exist, it creates a new directory. This approach can be beneficial in scenarios where you want to create a directory only if it does not already exist, without causing any errors or interruptions.
Usage Example
To illustrate the usage of Path.mkdir if not exists, consider the following code snippet:
“`
from pathlib import Path
dir_path = Path(‘/path/to/directory/’)
# Create the directory if it does not exist
dir_path.mkdir(exist_ok=True)
“`
In the above example, a Path object is created with the desired directory path. The mkdir() method is then called with the exist_ok parameter set to True. Setting exist_ok to True ensures that the method behaves like Path.mkdir if not exists, creating the directory only if it doesn’t already exist.
Benefits of Path.mkdir if not exists
Using Path.mkdir if not exists offers several advantages:
1. Error Handling: Path.mkdir() raises an exception if the directory already exists, interrupting the program flow. In comparison, Path.mkdir if not exists gracefully handles the situation without interruptions.
2. Simplified Code: By using Path.mkdir if not exists, you can eliminate the need for explicit checks and conditionals to verify the existence of a directory. This results in cleaner and more concise code.
3. Idempotent Operations: The concept of idempotency states that performing an operation multiple times has the same result as performing it once. Path.mkdir if not exists adheres to this principle, ensuring that executing the method multiple times does not have any adverse effects. This property is particularly useful for automated scripts or when dealing with concurrent processes.
Potential Issues
While Path.mkdir if not exists offers a seamless solution for creating directories, there are a few considerations to keep in mind:
1. Permissions: The successful creation of a directory depends on the permissions of the parent directory. Ensure that the user running the code has appropriate permissions to create the directory.
2. Race Conditions: When multiple processes are creating or checking the existence of the same directory simultaneously, race conditions may occur. Although rare, this can lead to unpredictable behavior. Consider using locking mechanisms or other synchronization techniques to mitigate this issue.
FAQs
Q1. Is Path.mkdir if not exists limited to creating directories only?
No, it can be used to create directories, as well as subdirectories within an existing directory.
Q2. Should I always use Path.mkdir if not exists instead of Path.mkdir?
No, it depends on your specific use case. If you want your program to break or handle FileExistsError exceptions when a directory already exists, you can still use Path.mkdir(). Path.mkdir if not exists is primarily useful when you want to avoid interruptions and gracefully handle existing directories.
Q3. Can I specify the permissions for the newly created directory using Path.mkdir if not exists?
Yes, you can pass the desired permissions using the mode parameter of the mkdir() method. By default, it inherits the permissions of the parent directory.
Q4. Does Path.mkdir if not exists support relative paths?
Yes, it supports both absolute and relative paths. However, ensure that the relative paths are resolved correctly before invoking the method.
Q5. Which Python versions support Path.mkdir if not exists?
Path.mkdir if not exists is available in Python 3.4 and later versions.
In conclusion, Path.mkdir if not exists is a handy functionality in Python’s pathlib module that simplifies the creation of directories. It ensures that the directory is only created if it does not already exist, eliminating errors and interruptions. By understanding its usage, benefits, and potential considerations, developers can leverage Path.mkdir if not exists effectively in their code.
Python Create File If Not Exists
Creating a file in Python can be accomplished using the built-in `open()` function, which allows us to interact with files on the file system. By default, the `open()` function opens a file in write mode, which means that if the file does not exist, it will be created. However, it overwrites the contents of the file if it already exists. To overcome this limitation, we need to perform an additional check to ensure that the file does not exist before creating it.
One common approach is to use the `os.path` module to check if the file exists before creating it. The `os.path.exists()` function returns `True` if the file or directory exists, and `False` otherwise. Here’s an example of how we can use this function to create a file only if it does not exist:
“`python
import os
def create_file_if_not_exists(file_path):
if not os.path.exists(file_path):
with open(file_path, ‘w’) as file:
file.write(‘Hello, World!’)
print(‘File created successfully.’)
else:
print(‘File already exists.’)
“`
In the above code, we first check if the file exists using `os.path.exists()`. If it doesn’t exist, we create the file using `open()` in write mode and write some initial contents to it. Finally, we print a success message indicating whether the file was created or not.
Another approach is to use the `pathlib` module, which offers an object-oriented approach for working with file paths. The `Path` class from `pathlib` provides the `exists()` method, which can be used to check if a file or directory exists. Here’s how we can achieve file creation using `pathlib`:
“`python
from pathlib import Path
def create_file_if_not_exists(file_path):
path = Path(file_path)
if not path.exists():
path.touch()
print(‘File created successfully.’)
else:
print(‘File already exists.’)
“`
In the above example, we first create a `Path` object representing the file path. Then, we invoke the `exists()` method to determine if the file exists. If it does not exist, we create the file using the `touch()` method, and print an appropriate success message.
Apart from these basic approaches, there are several other variants and considerations when it comes to creating a file if it does not exist in Python. Let’s address some frequently asked questions to cover this topic comprehensively:
**1. How can I create a file in a specific directory?**
To create a file in a specific directory, you can provide the full path of the file when using the `open()` or `Path().touch()` methods. For example:
“`python
file_path = ‘/path/to/directory/file.txt’
# Using open()
with open(file_path, ‘w’) as file:
file.write(‘Hello, World!’)
# Using pathlib
path = Path(file_path)
path.touch()
“`
**2. How can I handle file creation errors?**
When creating a file, there might be scenarios where permission issues, disk full errors, or other exceptions occur. To handle such errors gracefully, you can enclose the file creation code within a try-except block and handle the specific exceptions accordingly.
“`python
try:
with open(file_path, ‘w’) as file:
file.write(‘Hello, World!’)
print(‘File created successfully.’)
except Exception as e:
print(f’File creation failed: {str(e)}’)
“`
**3. Can I create files with different file extensions?**
Yes, you can create files with any file extension by specifying the extension within the file name when providing the file path. For example:
“`python
# Creating a text file
file_path = ‘/path/to/file.txt’
# Creating an image file
file_path = ‘/path/to/file.png’
# Creating a JSON file
file_path = ‘/path/to/file.json’
“`
In conclusion, Python provides various ways to create a file if it does not already exist. By utilizing the `open()` function along with appropriate strategies like file existence checks using `os.path` or `pathlib`, developers can create files dynamically and handle different use cases effectively.
Images related to the topic python make directory if not exists
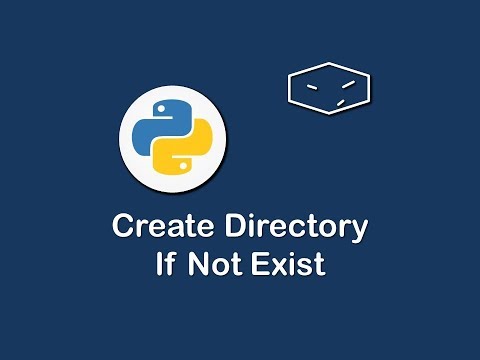
Found 19 images related to python make directory if not exists theme
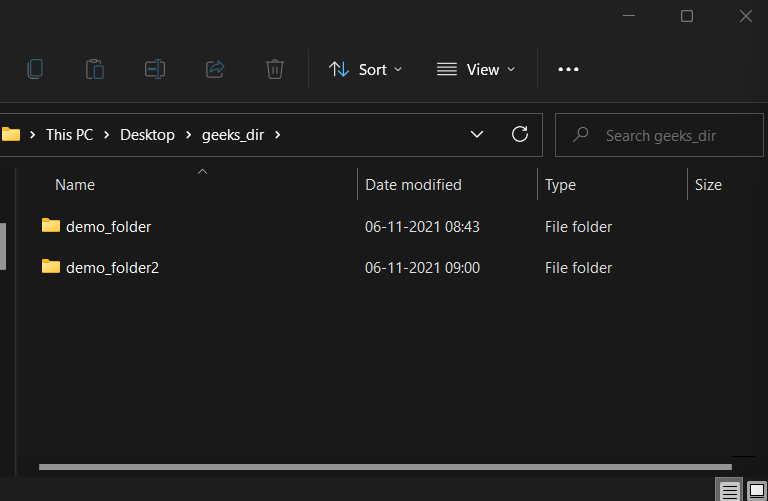
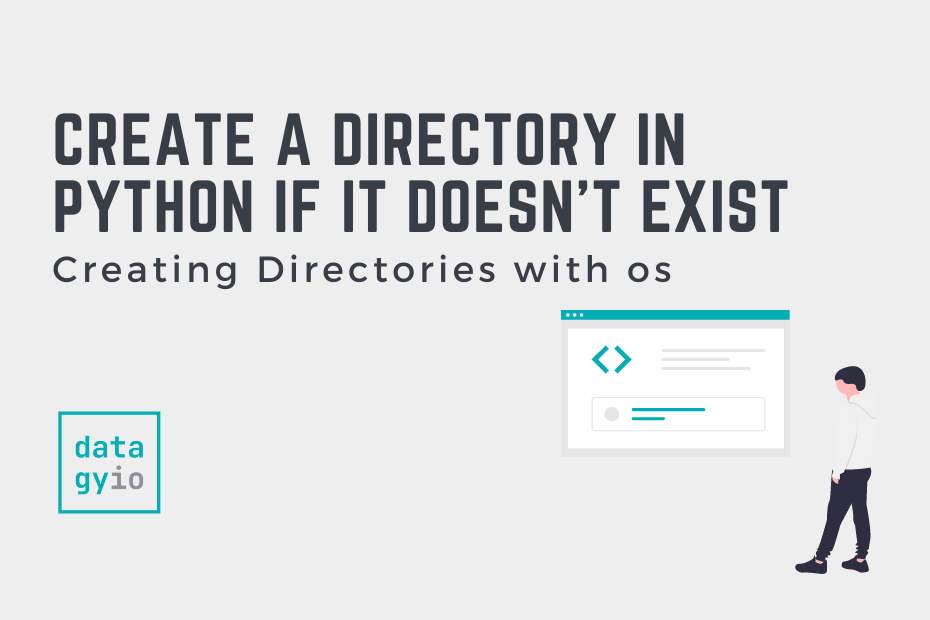
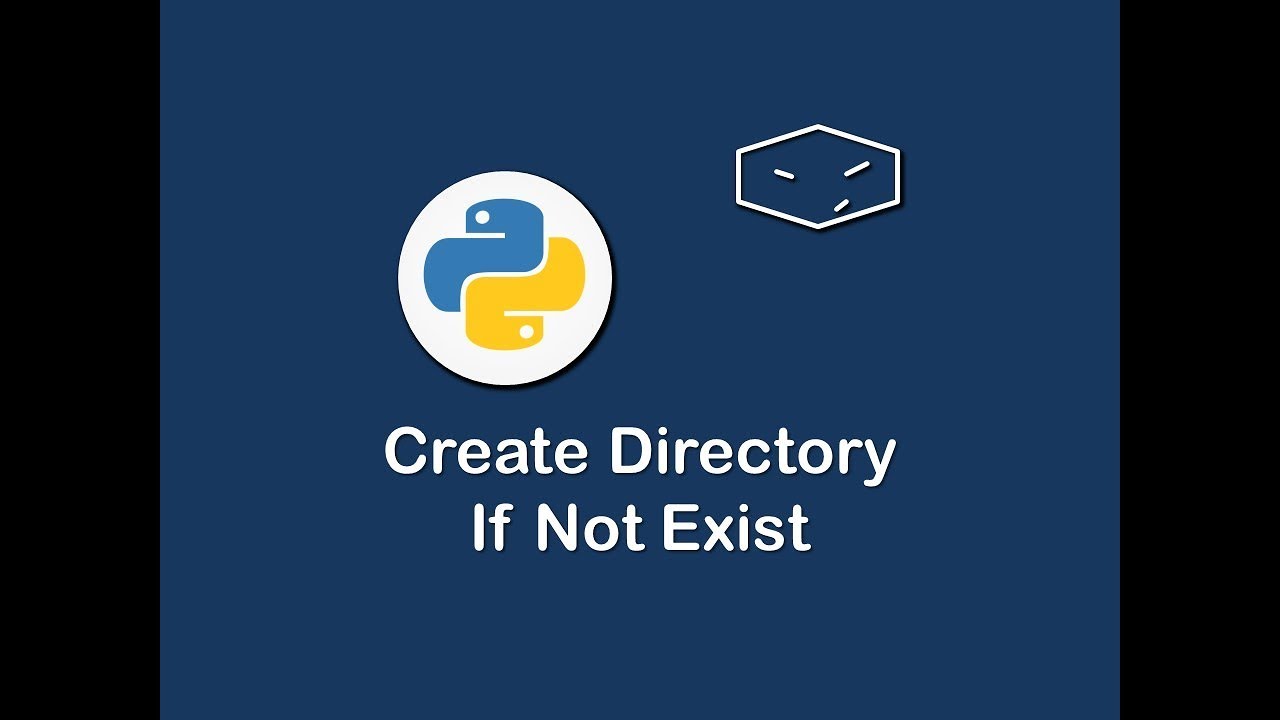
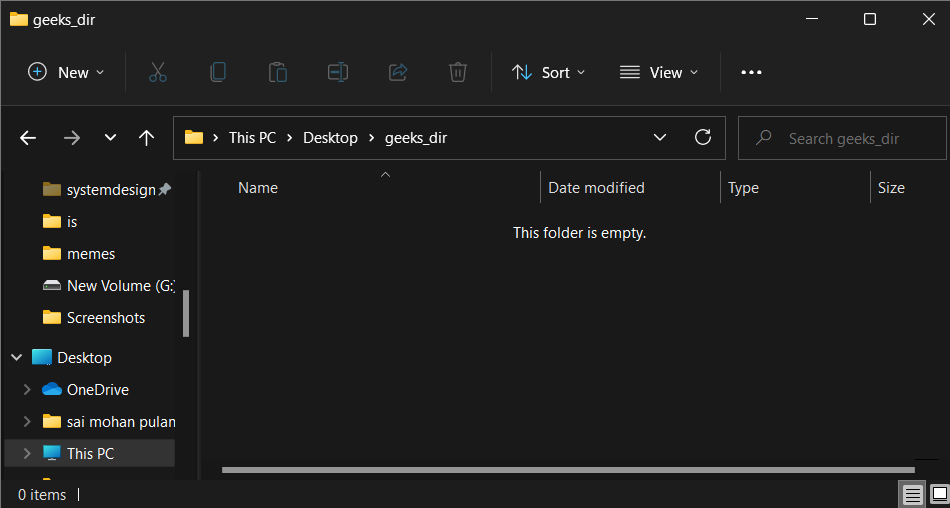
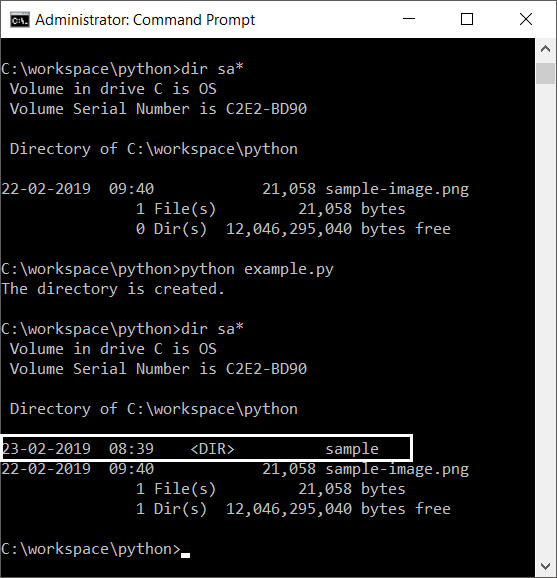
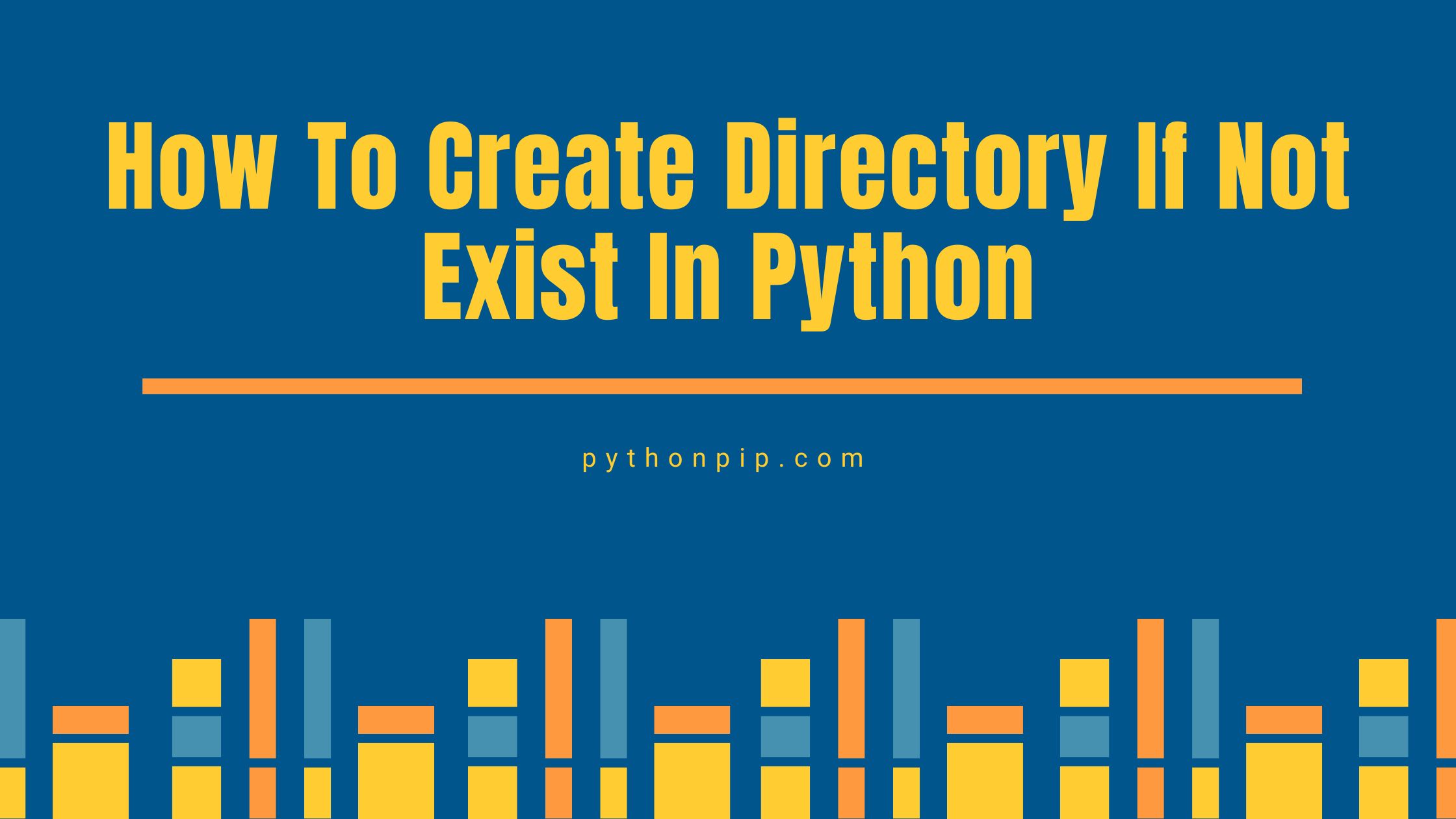

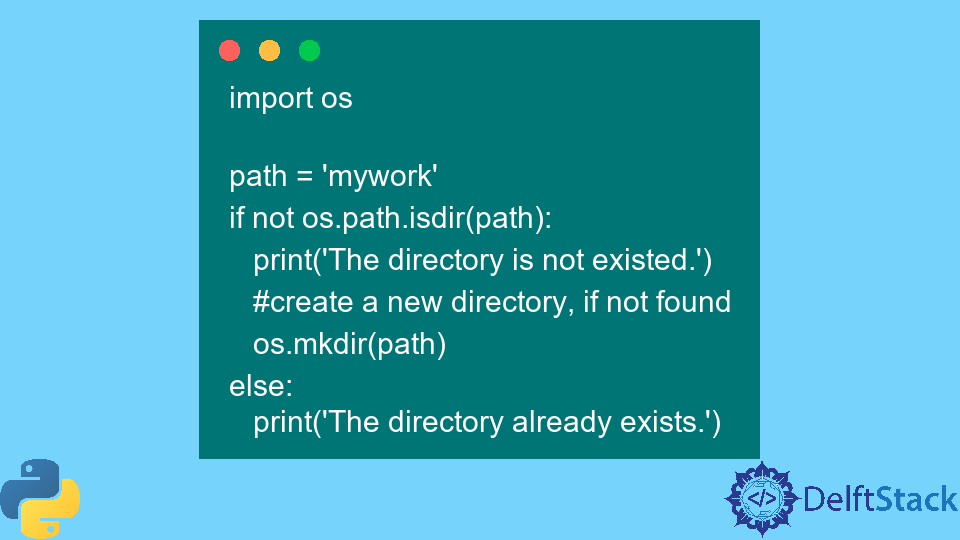
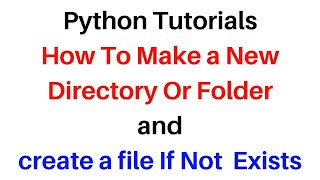
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/output-2.png)

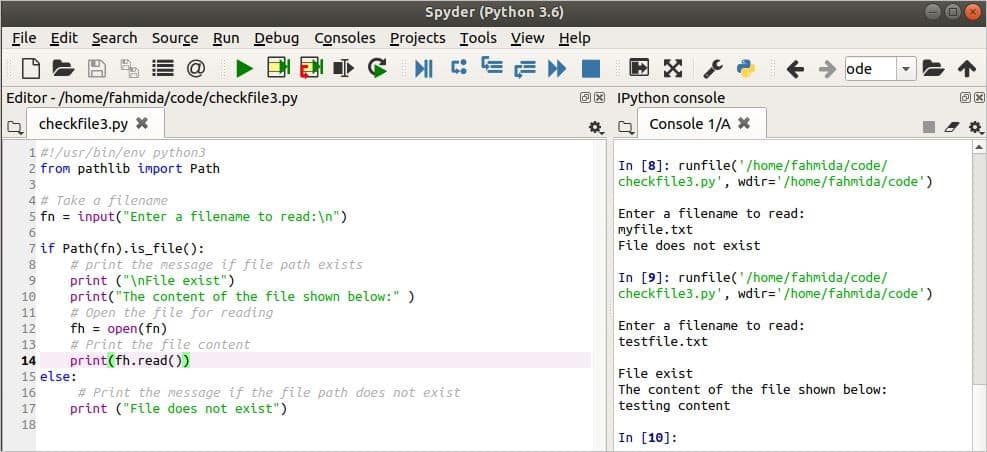
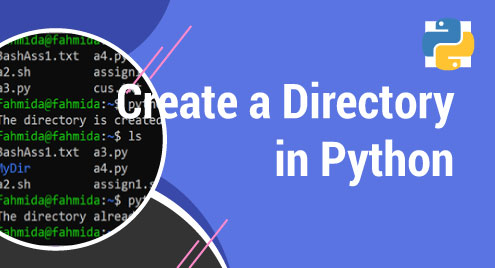
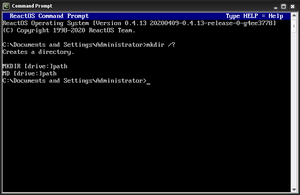
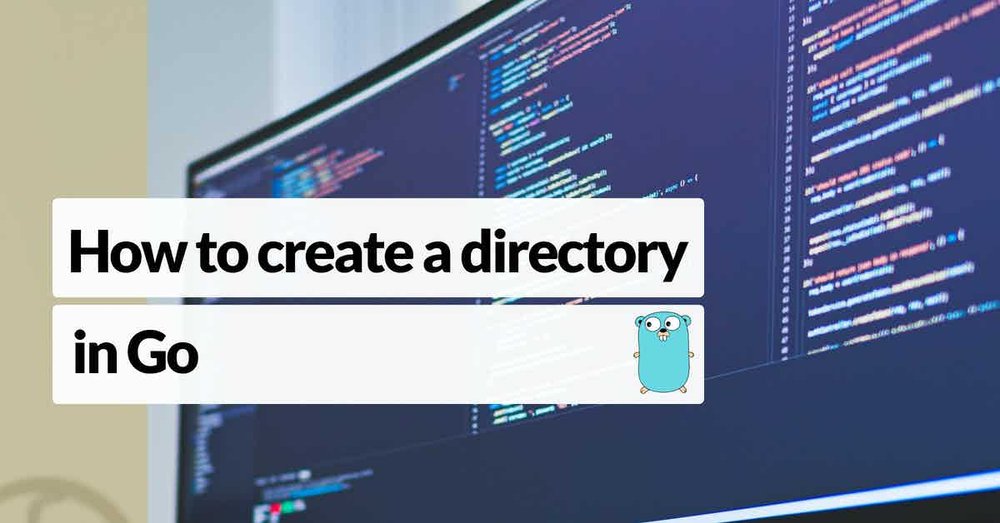
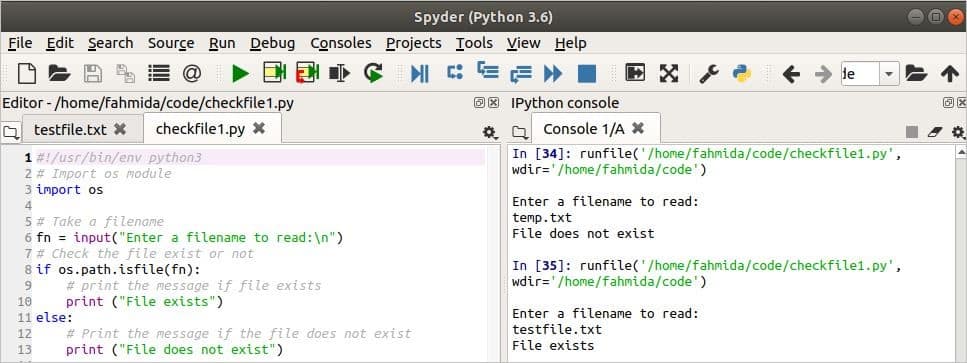

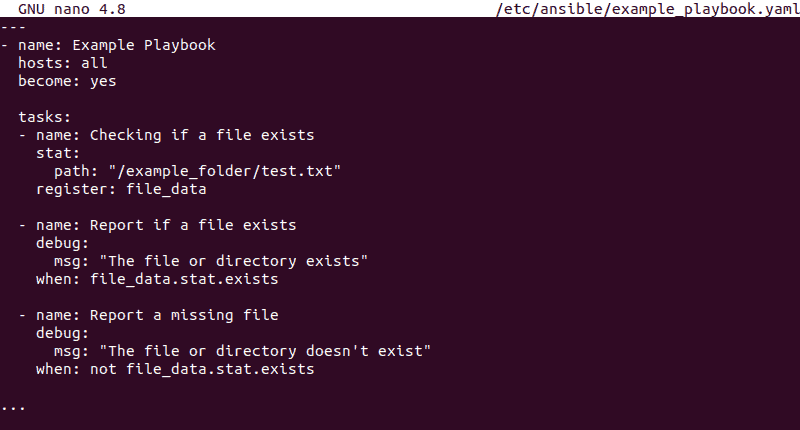
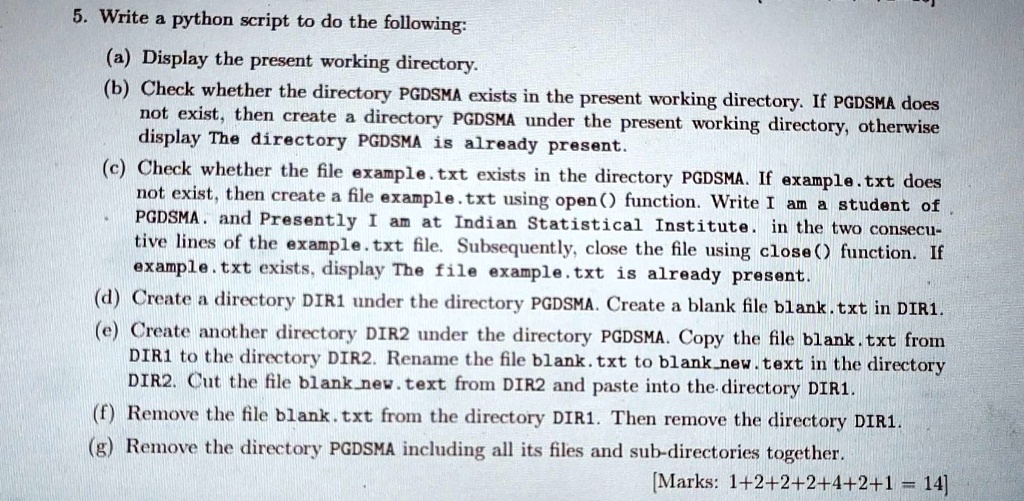
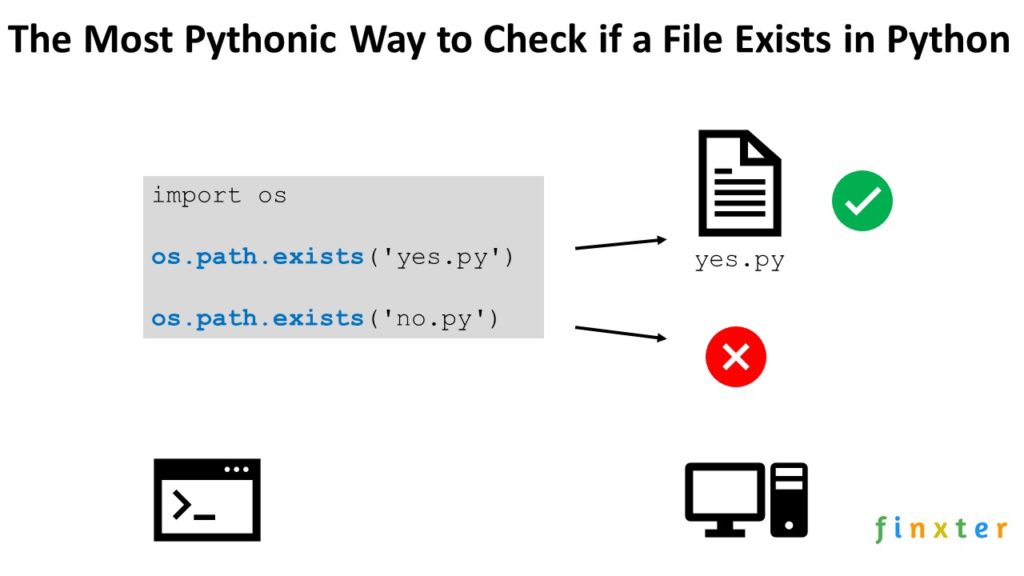
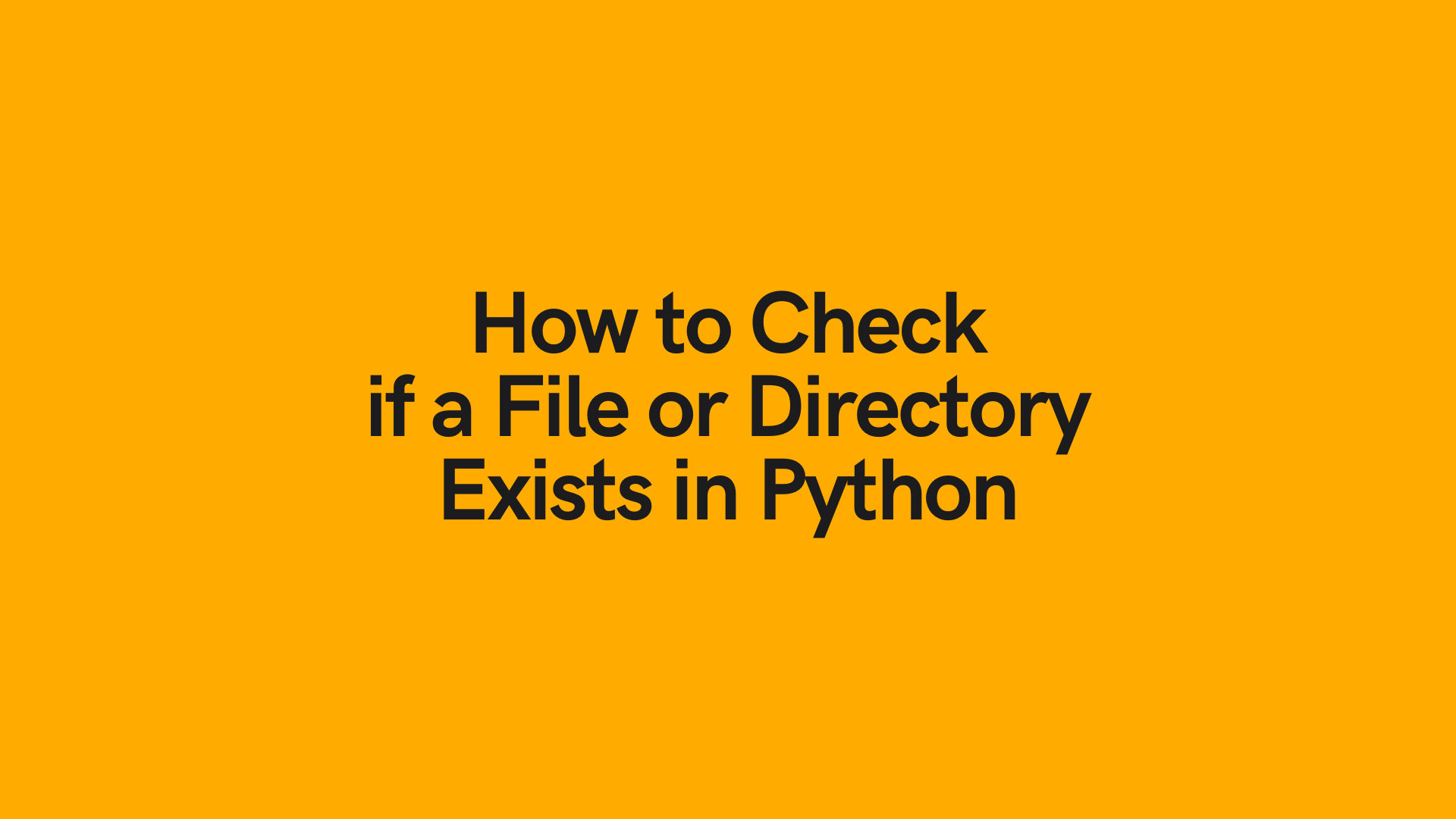
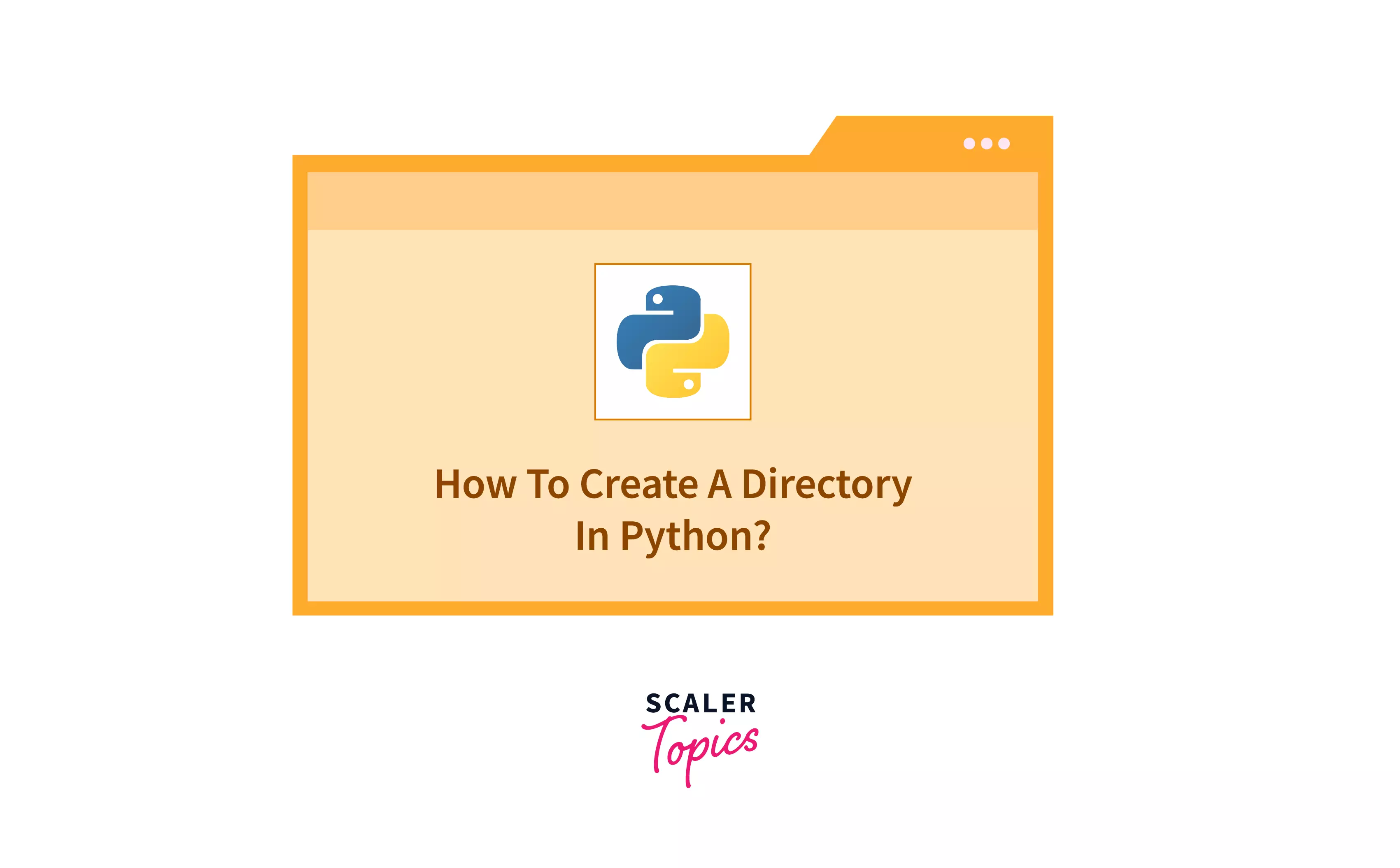
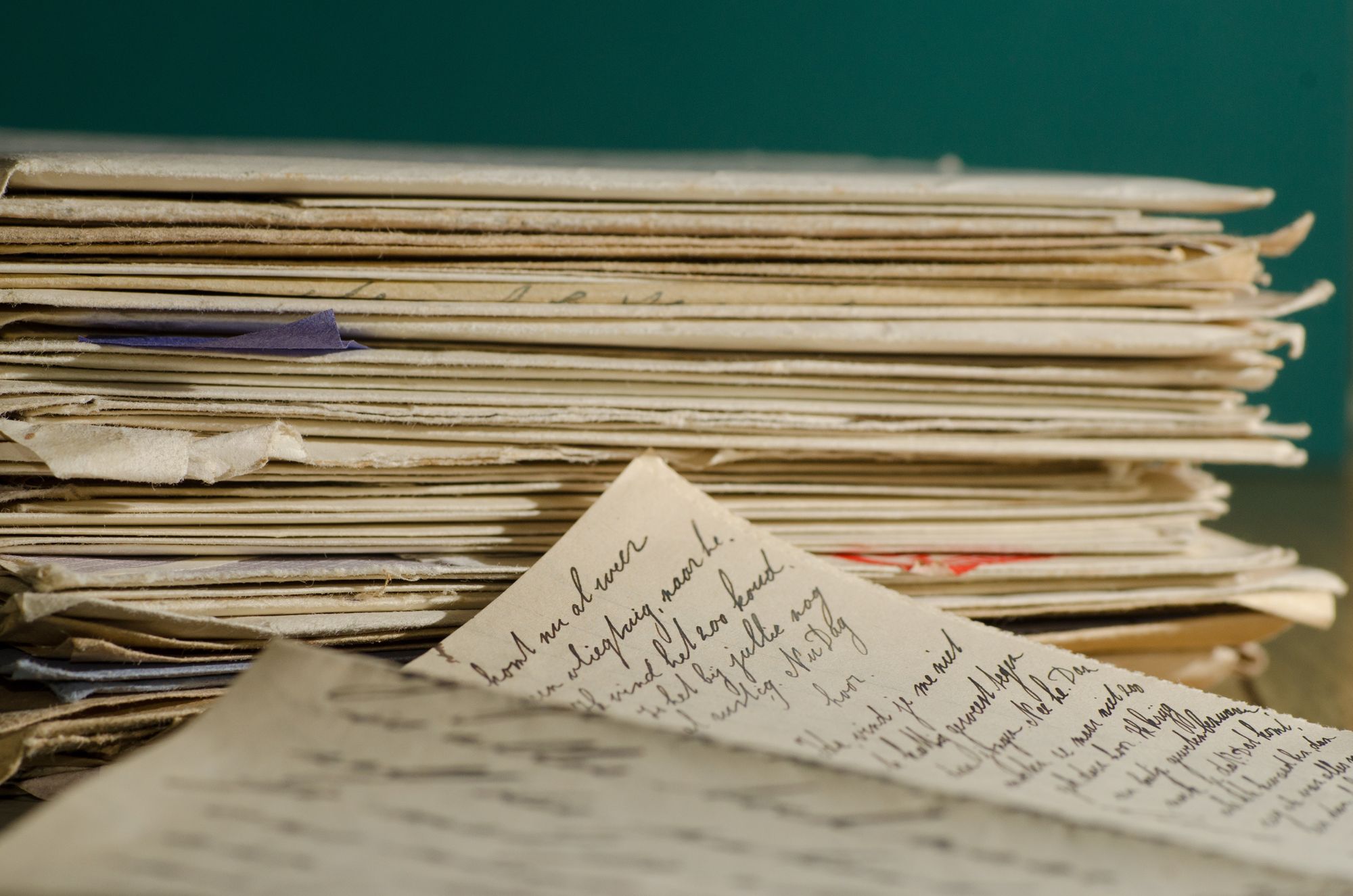
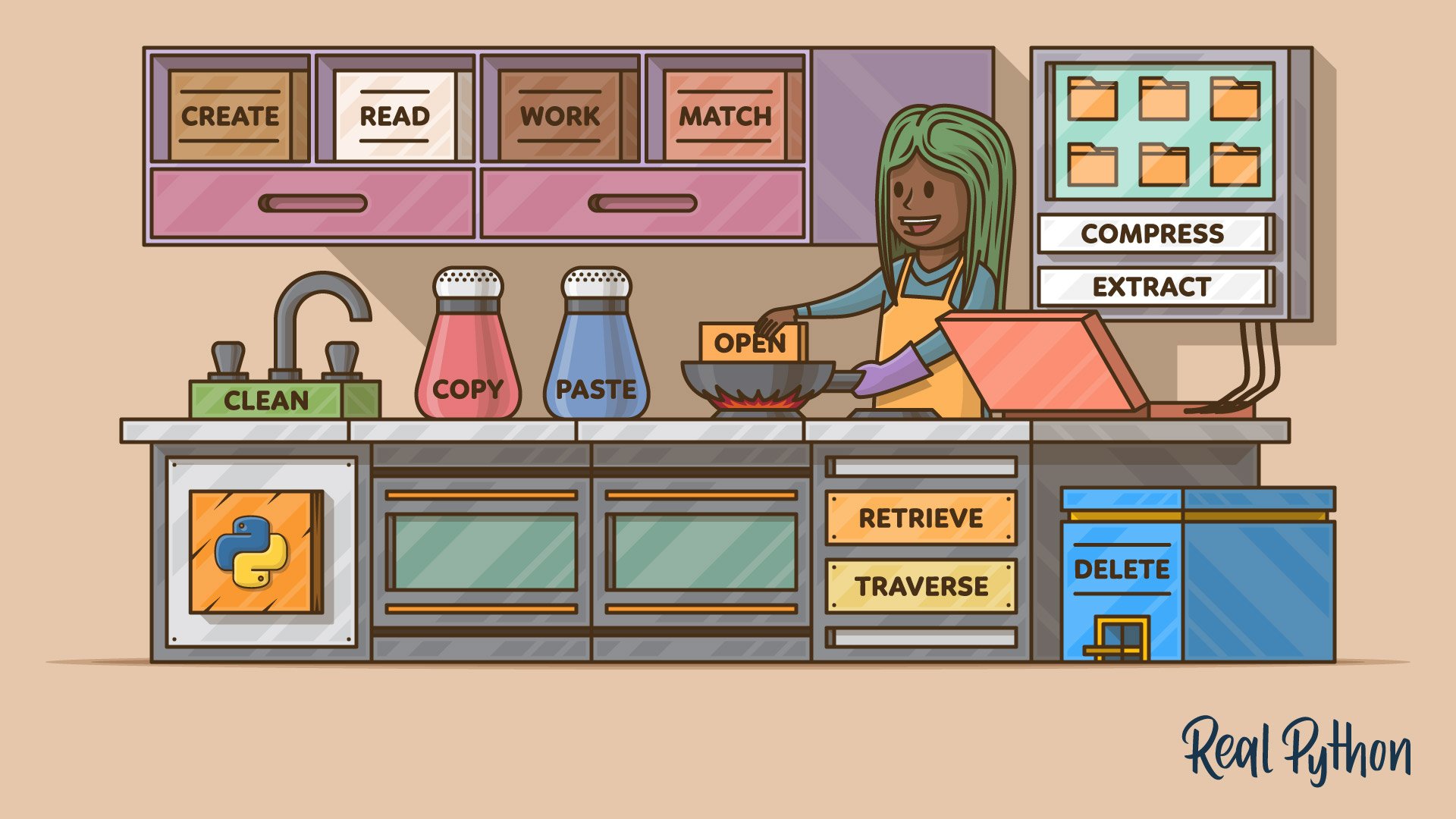
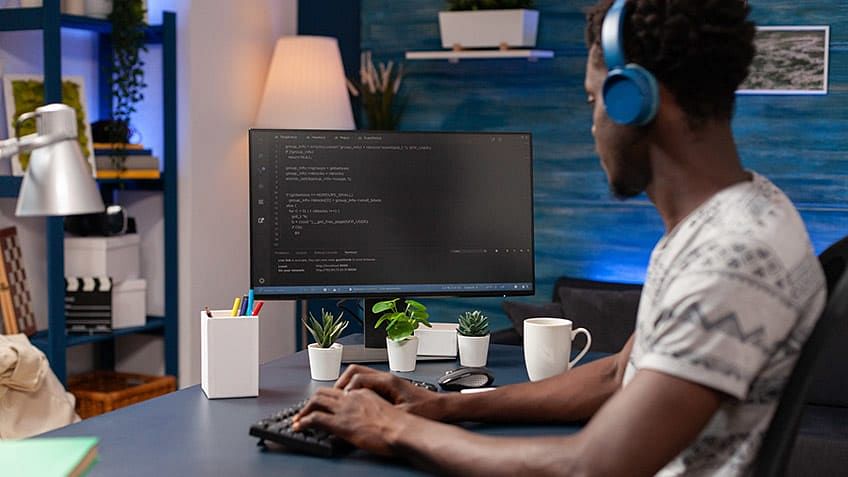
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/banner.webp)
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/all-fs-methods-1.png)
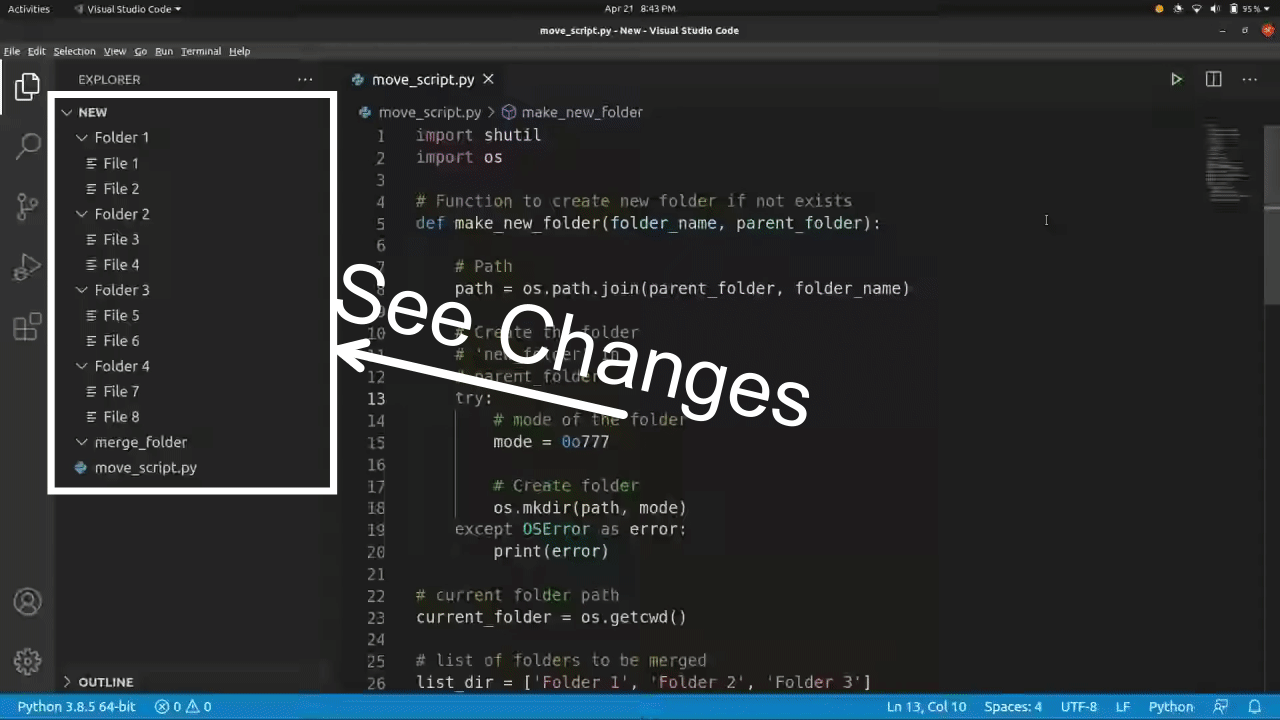

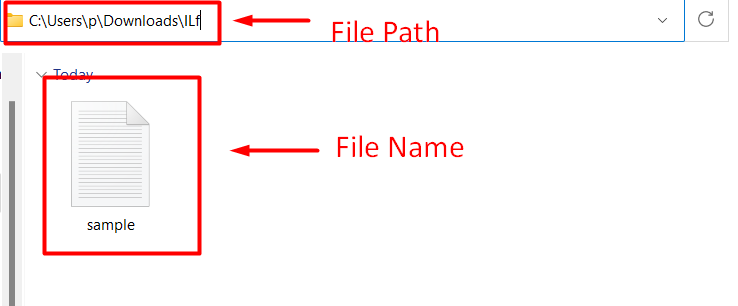
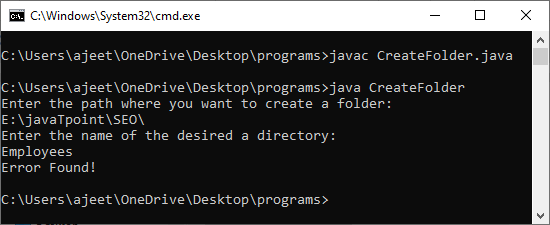
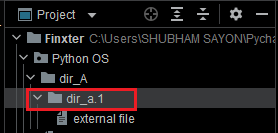
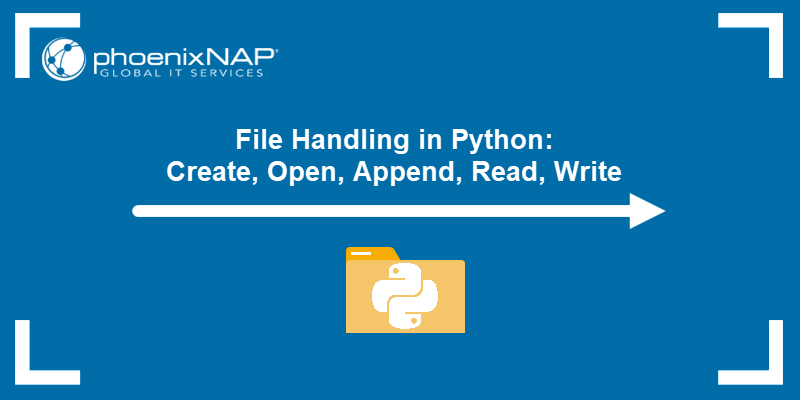
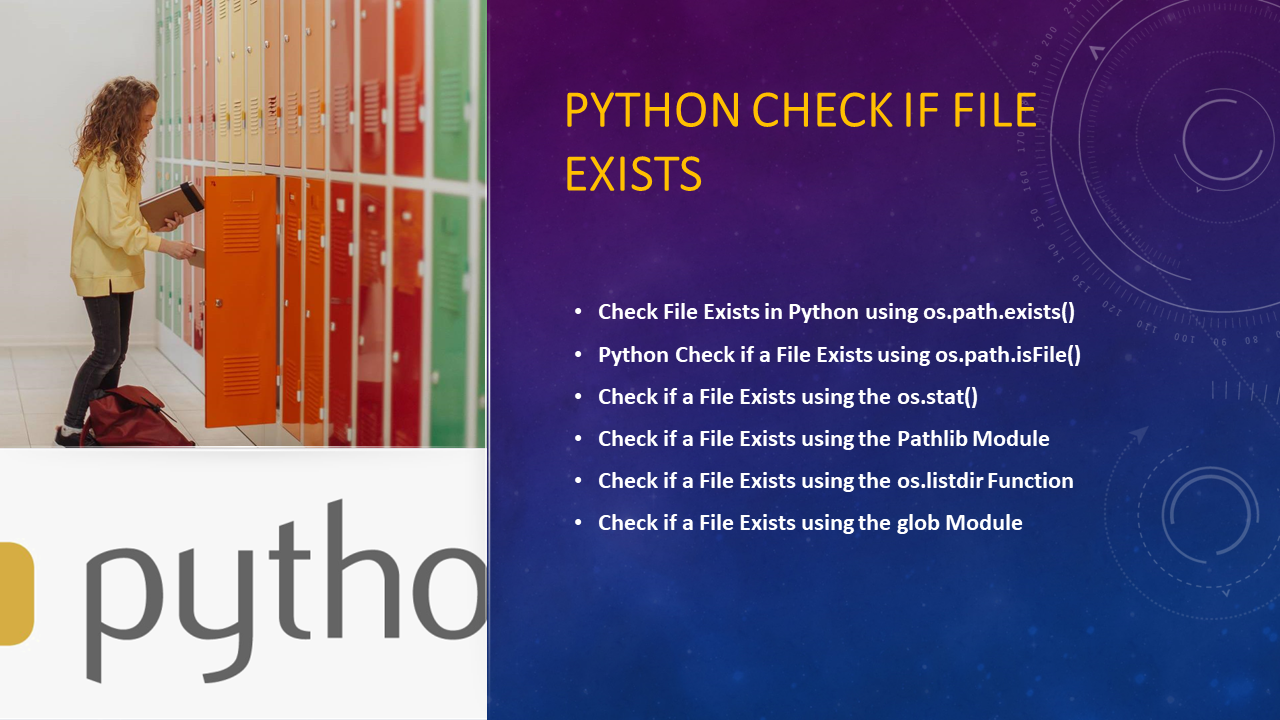
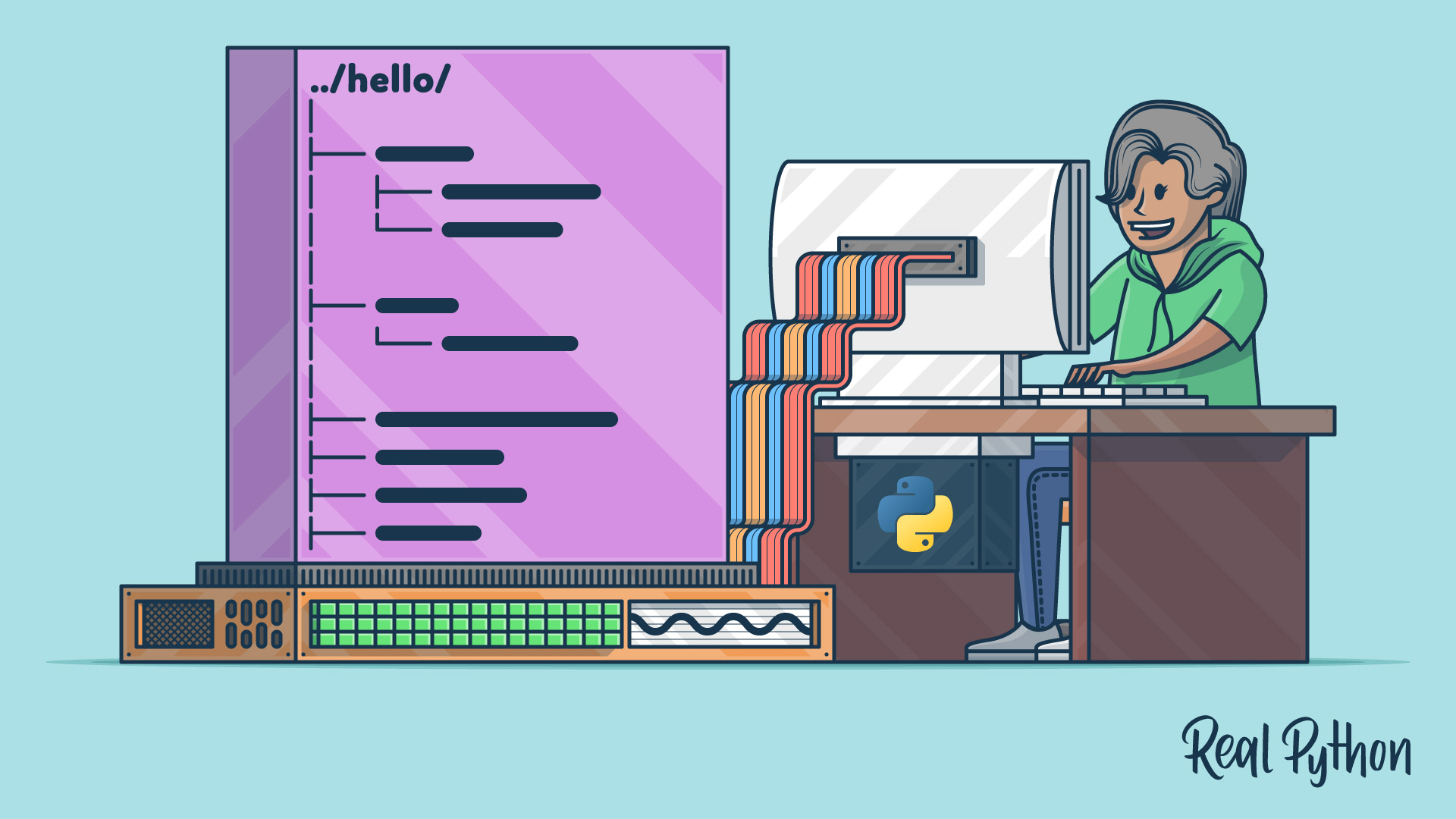
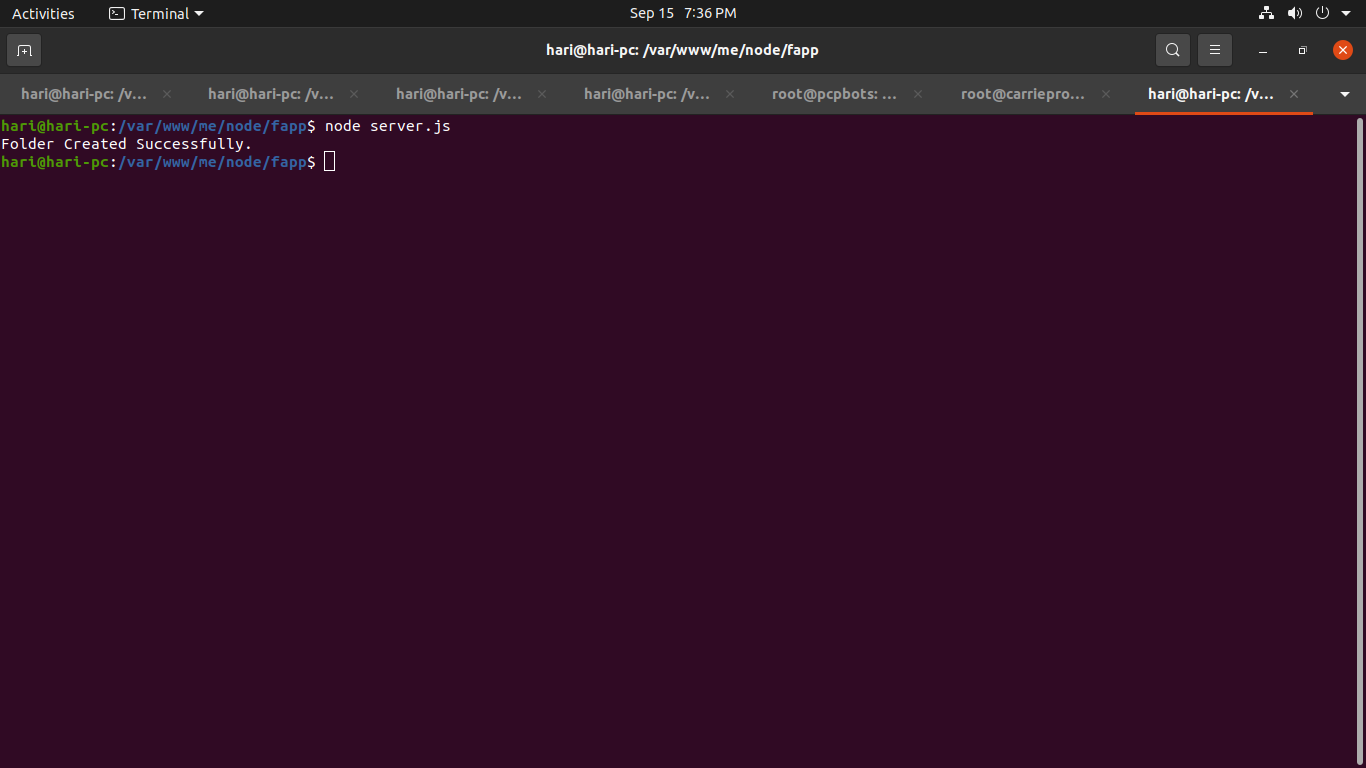
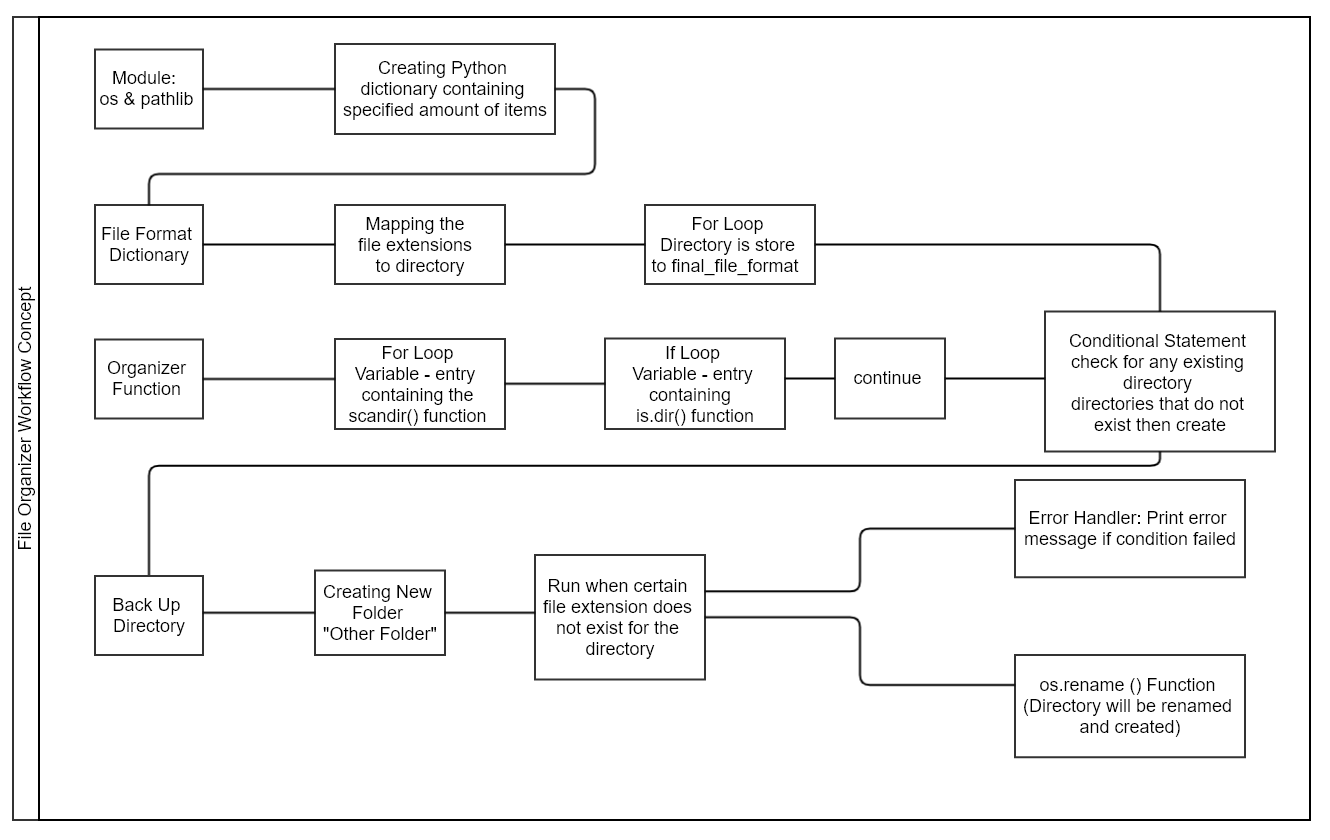
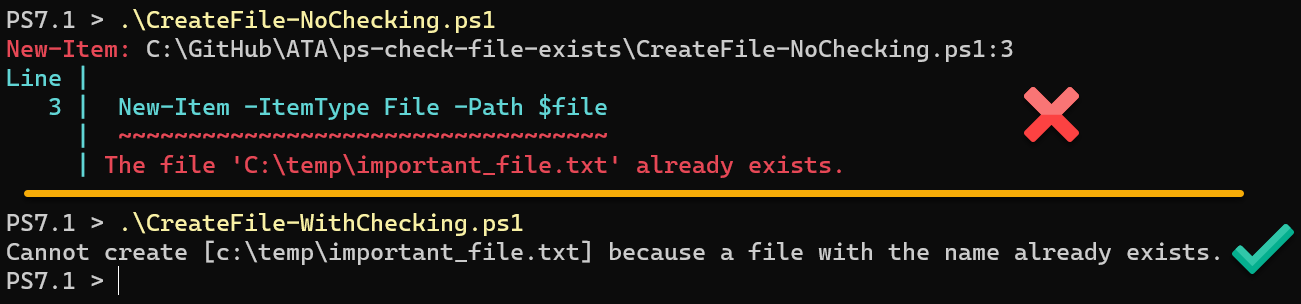
![FileExistsError: [Errno 17] File exists in Python [Solved] | bobbyhadz Fileexistserror: [Errno 17] File Exists In Python [Solved] | Bobbyhadz](https://bobbyhadz.com/images/blog/python-fileexistserror-errno-17-file-exists/directory-already-exists.webp)


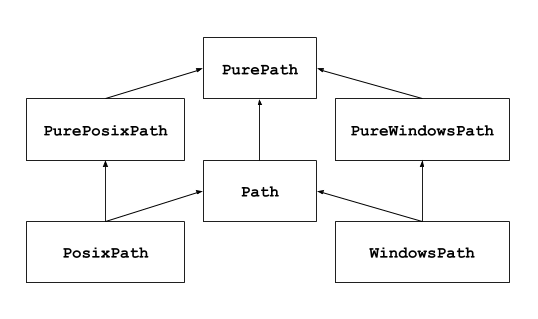
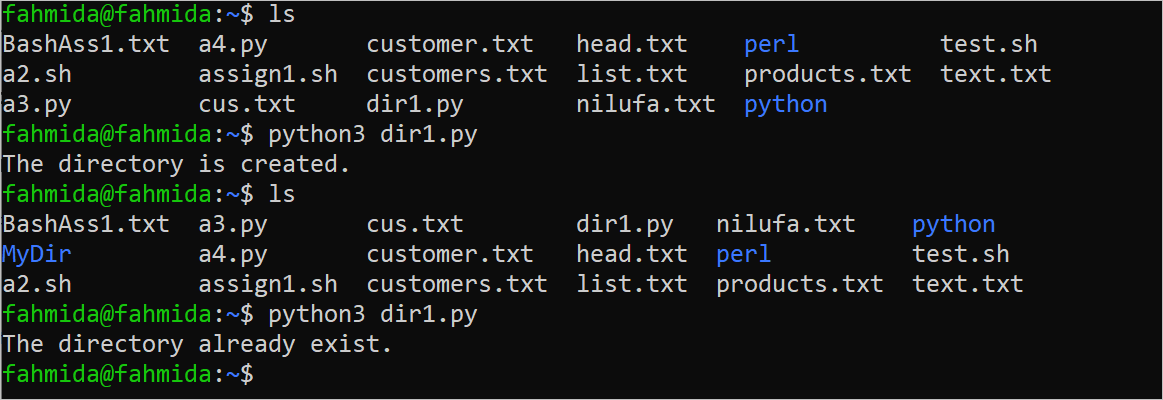
![Create File in Python [4 Ways] – PYnative Create File In Python [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/created-files.png)
![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-1.png)
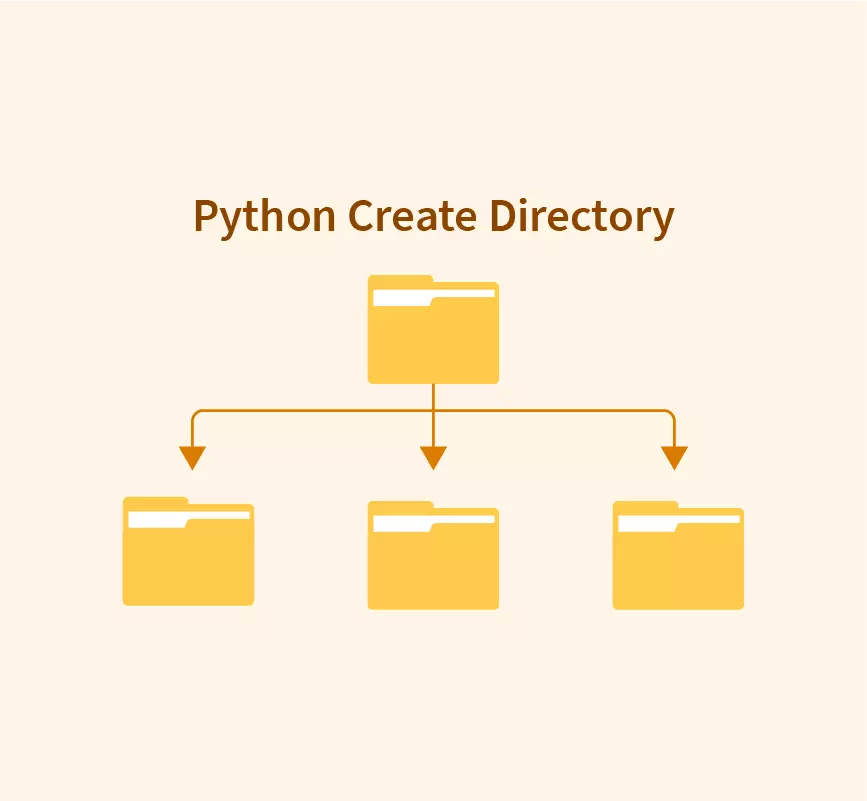
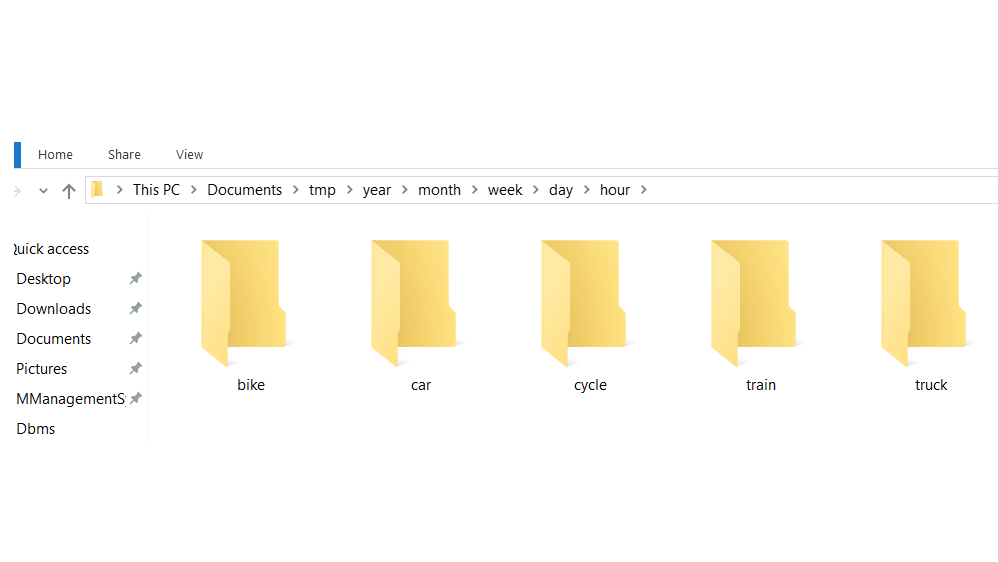
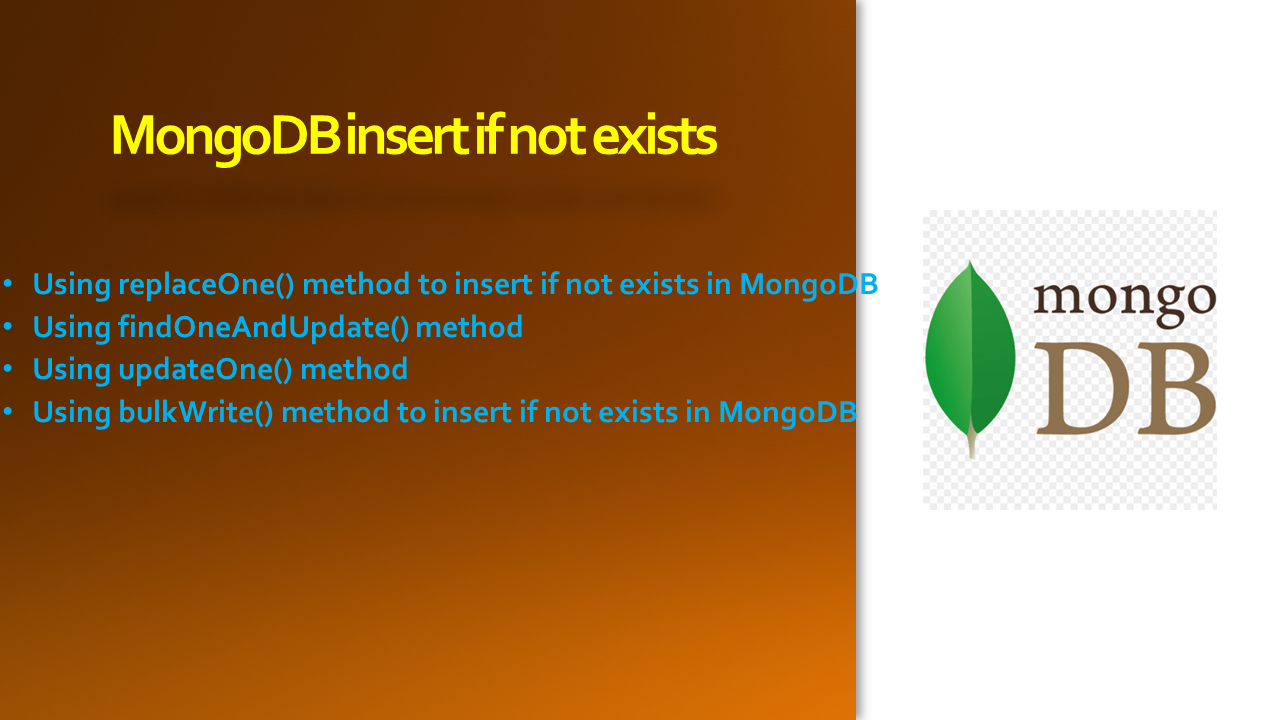
Article link: python make directory if not exists.
Learn more about the topic python make directory if not exists.
- How can I create a directory if it does not exist using Python
- How to Create Directory If Not Exists in Python – AppDividend
- How can I create a directory in Python using ‘mkdir if not exists’?
- How do I create a directory, and any missing parent directories?
- How to Create Directory If it Does Not Exist using Python?
- Python: Check if a File or Directory Exists – GeeksforGeeks
- Create a directory if it does not exist and then create the files in that …
- Python Directory and Files Management – Programiz
- Python: Create a Directory if it Doesn’t Exist – Datagy
- Create directory if it does not exist in Python
- Create Directory if not Exists in Python – Programming Basic
- Python Create Directory If Not Exists Top 17 Latest Posts
- How To Create a Directory If Not Exist In Python – pythonpip.com
See more: https://nhanvietluanvan.com/luat-hoc