Python One Line For Loop
Python is widely known for its simplicity and readability. One of its powerful features is the ability to write for loops in a single line. This article will explain what a one-line for loop is, how to write one, and explore different variations and use cases. We will also discuss common errors and pitfalls to watch out for.
What is a one-line for loop in Python?
A one-line for loop, also known as a list comprehension, is a concise way to iterate over a sequence, apply some logic, and generate a new sequence in a single line of code. It combines the functionality of a traditional for loop and conditional statements, reducing the code length and increasing readability.
How to write a one-line for loop in Python?
To write a one-line for loop in Python, you typically use the following syntax:
“`
[expression for item in iterable]
“`
The expression is the operation you want to perform on each item, item represents the current element from the iterable, and iterable is the sequence you want to iterate over.
For example, let’s say we have a list of numbers and we want to square each one. We can write a one-line for loop like this:
“`python
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x**2 for x in numbers]
“`
The `squared_numbers` variable will now contain [1, 4, 9, 16, 25].
Using list comprehension in a one-line for loop
List comprehension allows you to generate a new list by applying an expression to each item in an iterable. It is a powerful feature that simplifies code and makes it more concise.
Consider the following example where we want to create a list of even numbers from 1 to 10:
“`python
even_numbers = [x for x in range(1, 11) if x % 2 == 0]
“`
The `even_numbers` variable will now contain [2, 4, 6, 8, 10].
Using if statements in a one-line for loop
You can also include conditional statements in a one-line for loop to filter elements based on certain criteria. For example, let’s say we want to create a list of numbers less than 5:
“`python
numbers = [1, 2, 3, 4, 5]
less_than_five = [x for x in numbers if x < 5]
```
The `less_than_five` variable will now contain [1, 2, 3, 4].
Nested one-line for loops in Python
Python allows you to nest for loops in a single line, which can be useful in certain scenarios. The outer loop executes once for each item in the outer iterable, while the inner loop executes for each item in the inner iterable. The resulting sequence will contain all combinations of the nested loops.
Let's take an example where we want to create a list of tuples representing all possible pairs of numbers from two separate lists:
```python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
pairs = [(x, y) for x in list1 for y in list2]
```
The `pairs` variable will now contain [(1, 4), (1, 5), (1, 6), (2, 4), (2, 5), (2, 6), (3, 4), (3, 5), (3, 6)].
Using multiple iterators in a one-line for loop
Python allows you to use multiple iterators in a one-line for loop, enabling you to iterate over multiple sequences simultaneously. This can be useful when you want to combine or compare elements from different sequences.
For example, let's say we have two lists and we want to create a list of tuples where each tuple contains corresponding elements from both lists:
```python
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
combined = [(x, y) for x, y in zip(list1, list2)]
```
The `combined` variable will now contain [(1, 'a'), (2, 'b'), (3, 'c')].
Common errors and pitfalls with one-line for loops in Python
While one-line for loops can be powerful and concise, they can also introduce some common errors and pitfalls. Here are a few things to keep in mind:
1. Incorrect indentation: Make sure you maintain proper indentation within the one-liner to avoid syntax errors.
2. Overusing one-liners: While one-liners can be helpful, they should not be used excessively. If a one-liner becomes too complex or difficult to understand, it's better to use a traditional for loop.
3. Lack of readability: One-liners may prioritize conciseness over readability. If code readability is more important to your specific use case, consider using a more explicit approach.
4. Limited debugging capabilities: Debugging one-liners can be challenging as you cannot easily step through each iteration. It's important to write clean and error-free code in the first place or convert your one-liner to a traditional for loop for better debugging options.
In conclusion, Python's one-line for loops, also known as list comprehensions, provide a concise and powerful way to iterate over sequences, apply conditions, and generate new sequences. They are a valuable tool for simplifying code and increasing readability. However, be cautious of potential errors and pitfalls and choose the most appropriate approach based on your specific use case. Happy coding!
Python Single Line If Else And For Loop | Python Shorthnds If Else And For Loop | All In One Code
Keywords searched by users: python one line for loop Python one line, Print for loop python one line, While one line python, 2 for in 1 line Python, Python lambda for loop, For if in one line Python, Python if-else one line, If else and for in one line Python
Categories: Top 19 Python One Line For Loop
See more here: nhanvietluanvan.com
Python One Line
In the realm of programming languages, Python stands tall as a versatile and powerful tool. Its simplicity, readability, and vast library ecosystem have made it the go-to language for both beginners and experts alike. One of Python’s most intriguing features is its ability to execute complex tasks in just a single line of code. In this article, we will explore the world of Python one-liners and how they can enhance your programming prowess.
Python’s Conciseness: Simplicity Meets Elegance
One-liners in Python are concise snippets of code that are written to perform a specific task in a remarkably short amount of space. They require a deep understanding of Python’s syntax and its built-in functions, but they can accomplish feats that would otherwise require dozens of lines of code.
What makes Python truly elegant in this regard is its emphasis on simplicity and readability. The language’s design philosophy, often referred to as the “Zen of Python,” encourages developers to write code that is easy to understand. This simplicity, combined with the powerful built-in functions and libraries, makes Python the ideal playground for crafting impactful one-liners.
Applications of Python One-liners
Python’s one-liners have a wide range of applications across different domains. They can be used for mathematical operations, data manipulation, web scraping, text processing, and much more. For instance, a one-liner can replace a lengthy for loop when performing computations on a list of numbers, or it can parse a web page and extract relevant information within seconds.
Furthermore, Python’s ease of integration with other technologies makes it an excellent choice for one-liners while working with databases, APIs, or even Internet of Things (IoT) devices. Regardless of the task at hand, Python’s compact and definitive nature ensures efficient and effective code execution.
Best Practices and Tips for Writing Python One-liners
Mastering the art of Python one-liners requires practice, but some best practices can help you along the way. Here are some essential tips to keep in mind:
1. Understand Python’s built-in functions and libraries: Familiarize yourself with Python’s extensive standard library and popular third-party packages like NumPy, Pandas, and BeautifulSoup. These libraries provide a multitude of functions that can simplify complex tasks.
2. Avoid excessive code chaining: While one-liners are meant to be concise, overloading them with excessive functions or operations may compromise readability. Strike a balance between simplicity and clarity.
3. Use list comprehensions and lambda functions: These powerful constructs allow you to generate iterable results and define anonymous functions directly within a one-liner, reducing the need for additional lines of code.
4. Be cautious with side effects: Some one-liners may have unintended consequences due to hidden side effects, such as modifying variables outside of their scope. Review your code carefully to avoid any unforeseen bugs.
One-liner FAQs
Q: Are one-liners always the best approach?
A: While one-liners can be extremely useful, they are not always the most appropriate choice. In some cases, writing more explicit code with proper documentation may be preferable, especially when collaborating with others or dealing with complex logic.
Q: Should I prioritize code readability or brevity?
A: Strive for a balance between the two. While brevity is one of the hallmarks of an effective one-liner, prioritizing readability ensures that your code remains maintainable and understandable in the long run.
Q: How can I debug a one-liner?
A: Debugging one-liners can be challenging due to their compact nature. Adding print statements or splitting the code into multiple lines can help identify issues. Additionally, using IPython or a debugger can provide deeper insights into the code execution.
Q: Where can I find examples and inspiration for one-liners?
A: Numerous online resources, books, and forums offer a plethora of Python one-liners. Websites like “Python One-Liners” or “Rosetta Code” showcase various examples across different problem domains, providing inspiration for your own endeavors.
Python one-liners represent the pinnacle of concise and impactful programming. Through their clever use, you can accomplish complex tasks with remarkable efficiency and elegance. Embrace the challenge, dive into Python’s vast ecosystem, and unleash your creativity through the simplicity of a single line of code.
Print For Loop Python One Line
Python, being one of the most popular programming languages, offers programmers a myriad of ways to accomplish tasks efficiently. When it comes to looping through sequences or performing repetitive tasks, the “for” loop is an invaluable tool in a Python developer’s arsenal. In this article, we will explore the power of a one-line “for” loop in Python, enabling you to write compact and elegant code.
Understanding the For Loop in Python
Before diving into the one-line implementation, let’s briefly review the basics of a standard “for” loop in Python. The “for” loop allows you to iterate over any iterable object, such as a list, tuple, string, or range. It follows a simple syntax:
“`python
for item in iterable:
# code block to be executed on each iteration
“`
In this syntax, the variable `item` represents the current item in the iteration, and `iterable` refers to the sequence being iterated over. The code block inside the loop is executed for each item in the sequence.
One Line For Loop Syntax
Python encourages concise and readable code, and the one-line “for” loop syntax embodies this philosophy. It allows you to collapse the entire loop, including the code block, into a single line. The syntax is as follows:
“`python
[expression for item in iterable]
“`
In this syntax, the `expression` can be any Python expression or function call that is executed for each item in the `iterable`. The result of each expression is collected and returned as a new iterable.
Examples of One Line For Loops
To demonstrate the power and versatility of the one-line “for” loop, let’s explore some examples:
1. Squaring Each Element in a List:
Suppose we have a list of numbers and want to create a new list containing the squares of each element. Using a one-line “for” loop, we can achieve this as follows:
“`python
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
“`
The resulting `squares` list will contain `[1, 4, 9, 16, 25]`.
2. Filtering Elements:
We can use a conditional statement within a one-line “for” loop to filter elements based on a given condition. For instance, let’s filter out all odd numbers from a list:
“`python
numbers = [1, 2, 3, 4, 5]
evens = [x for x in numbers if x % 2 == 0]
“`
The `evens` list will now contain only the even numbers `[2, 4]`.
3. Flattening a Nested List:
If you have a nested list and want to flatten it into a single list, you can achieve this with a one-line “for” loop combined with list comprehension:
“`python
nested_list = [[1, 2], [3, 4], [5, 6]]
flat_list = [x for sublist in nested_list for x in sublist]
“`
The resultant `flat_list` will be `[1, 2, 3, 4, 5, 6]`.
Advantages and Disadvantages of One Line For Loops
Using the one-line “for” loop syntax offers several benefits:
1. Concise Code: By collapsing the loop into a single line, the code becomes more readable, especially for simple and straightforward tasks.
2. Improved Performance: In some cases, a one-line “for” loop can offer better performance compared to traditional looping constructs.
3. Easier Maintenance: Compact code is often easier to understand and maintain, reducing the chances of bugs and improving code readability.
However, it is essential to weigh the advantages against the following limitations:
1. Reduced Readability: While the concise syntax is suitable for simple tasks, complex operations may result in less readable code. In such cases, it is better to use the traditional “for” loop syntax.
2. Limited Control Flow: One-line “for” loops are useful when each iteration is independent of previous iterations. If control flow requirements are more complicated, traditional “for” loops or other loop constructs should be used.
Frequently Asked Questions (FAQs):
Q1. Can I nest one-line “for” loops?
Yes, it is possible to nest one-line “for” loops by using additional list comprehensions. However, nesting multiple loops may lead to less readable code, so it’s important to consider readability when using this technique.
Q2. Can I use an “else” statement in a one-line “for” loop?
No, the one-line “for” loop syntax does not support an “else” statement. If you require an “else” statement, you should resort to the traditional “for” loop syntax.
Q3. How can I avoid modifying the original iterable when using a one-line “for” loop?
Since the one-line “for” loop syntax returns a new iterable, the original iterable remains unchanged. Therefore, there is no need to worry about modifying the original sequence.
Q4. Are one-line “for” loops only suitable for small-scale operations?
One-line “for” loops are not restricted to small-scale operations. While they are often used for simple tasks, they can also handle complex operations and provide performance benefits.
In conclusion, the one-line “for” loop syntax in Python allows programmers to write elegant and compact code, reducing the chances of errors and improving code readability. However, it is essential to strike a balance between readability and complexity while utilizing this powerful functionality. By understanding the syntax and examples provided in this guide, you are now equipped to leverage the full potential of the one-line “for” loop in your Python projects.
Images related to the topic python one line for loop
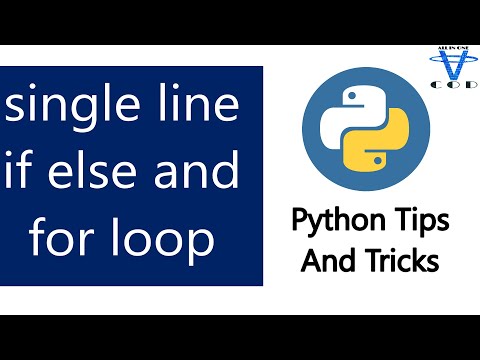
Found 34 images related to python one line for loop theme
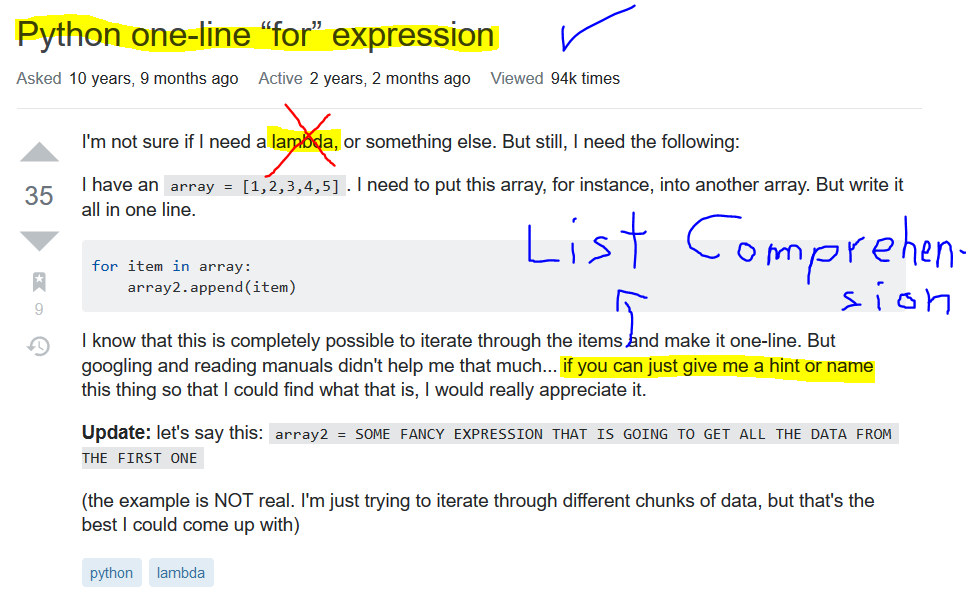
![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2020/05/listcomp-1024x576.jpg)
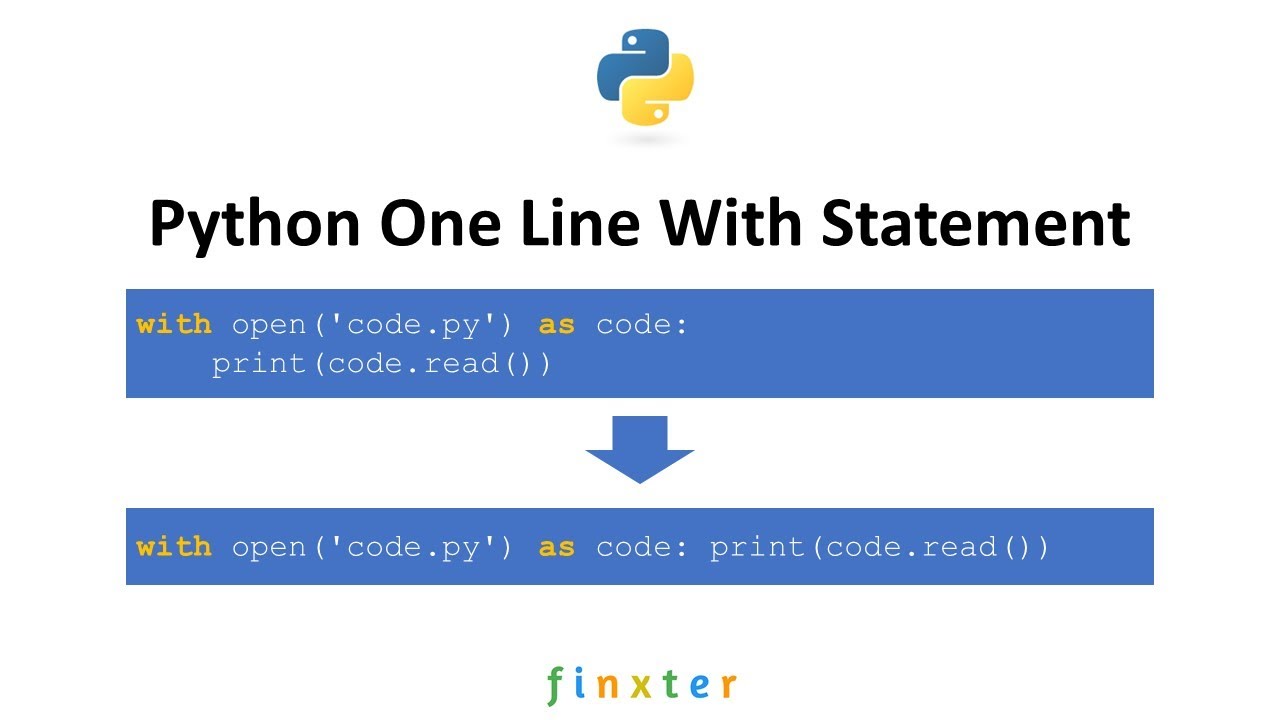
![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2019/12/grafik.png)

![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://i.ytimg.com/vi/M6XNZ40lRFQ/maxresdefault.jpg)
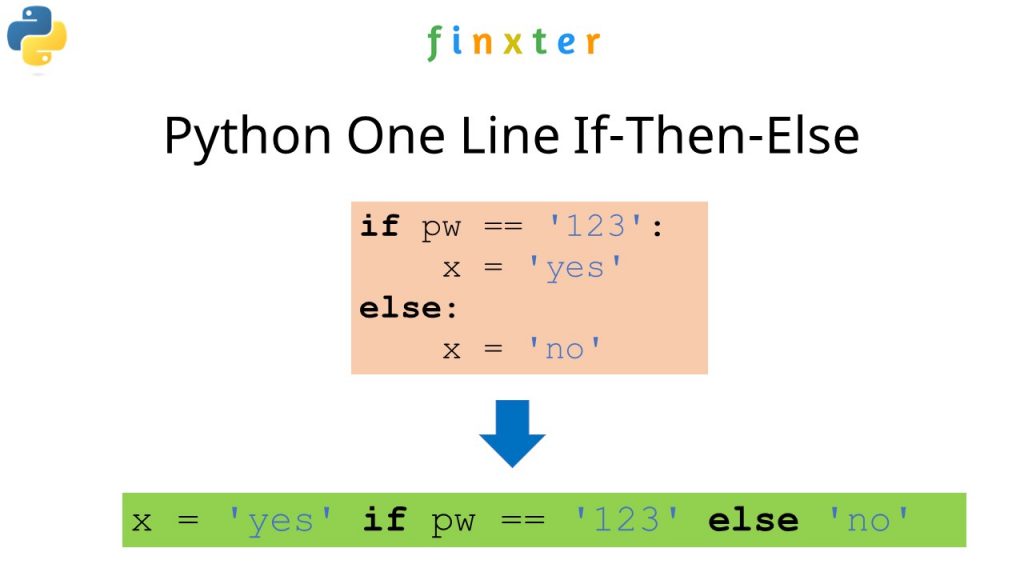

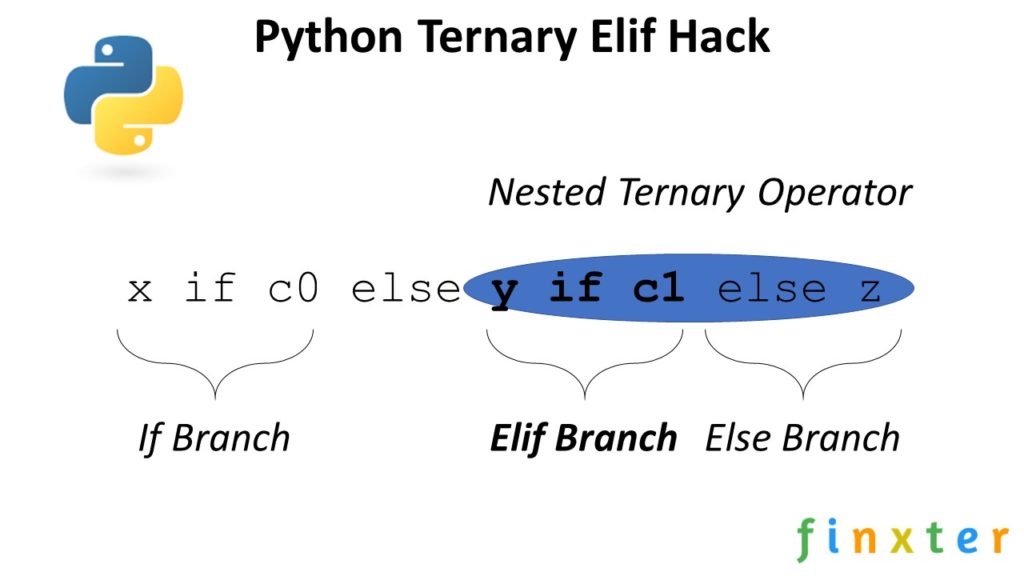

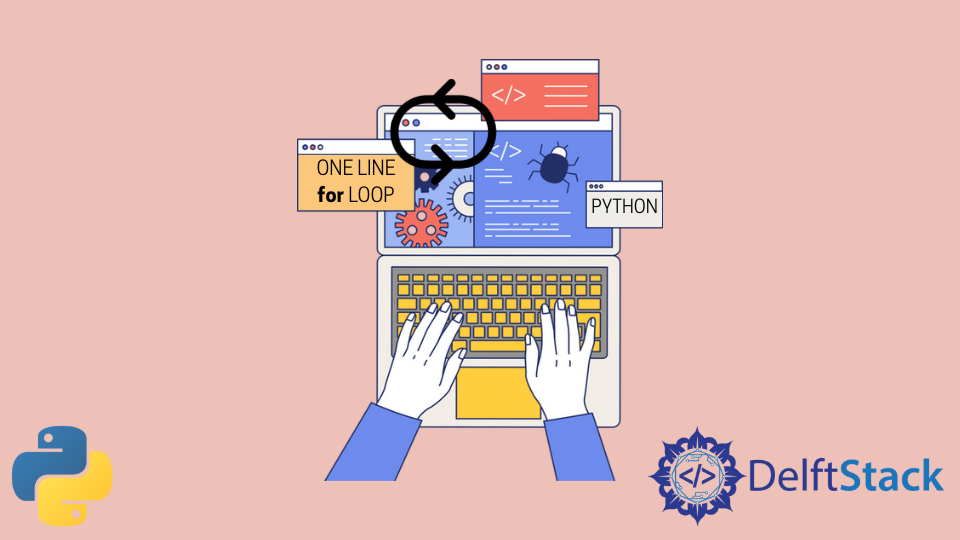

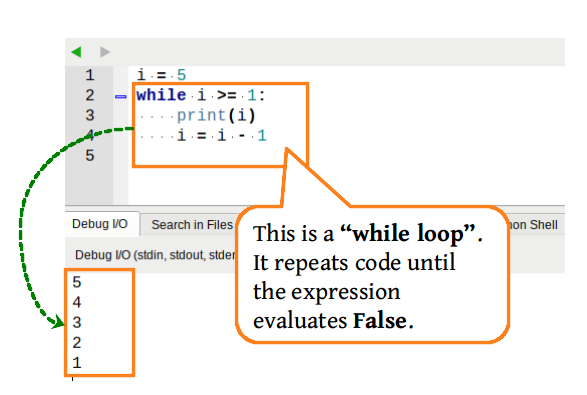
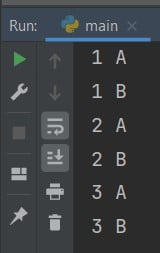
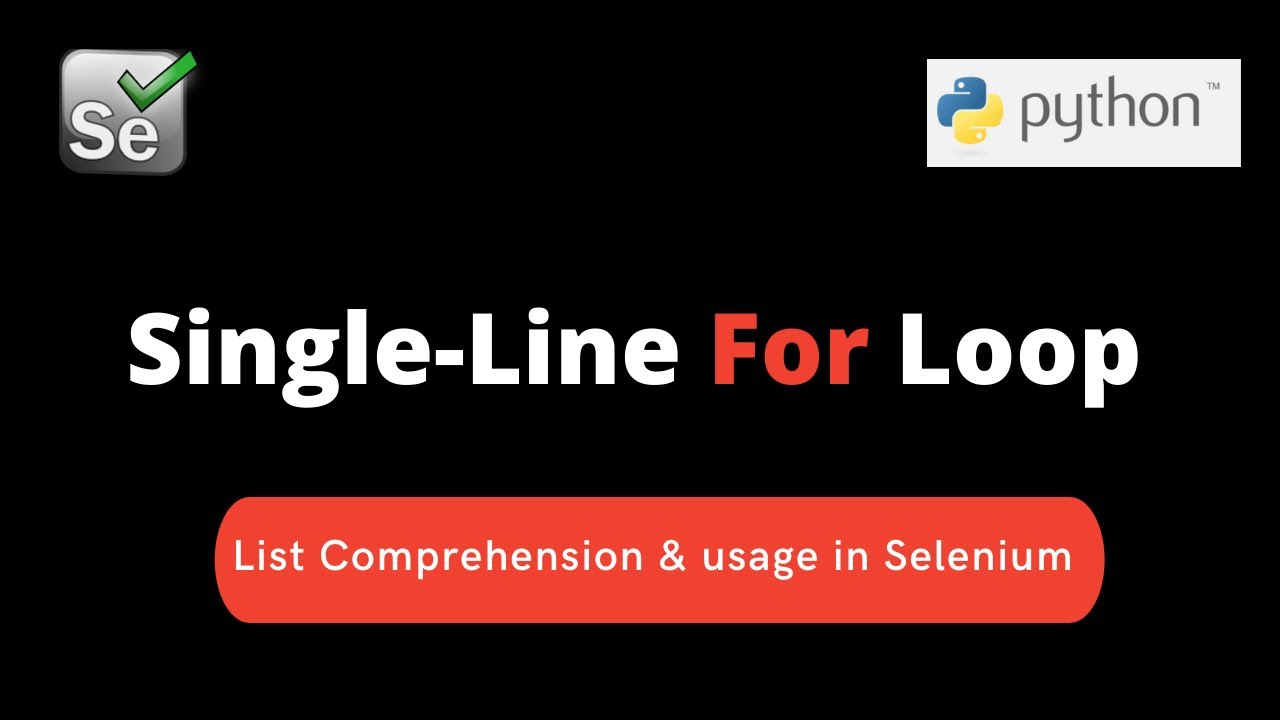

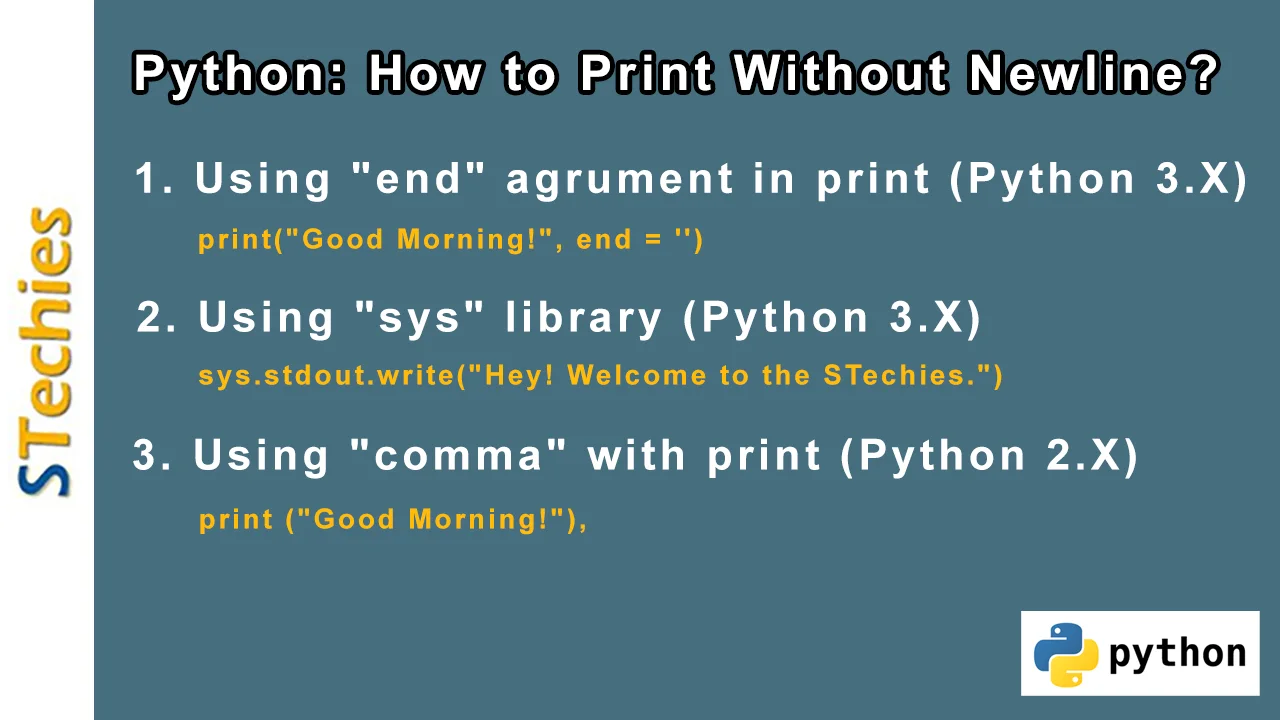
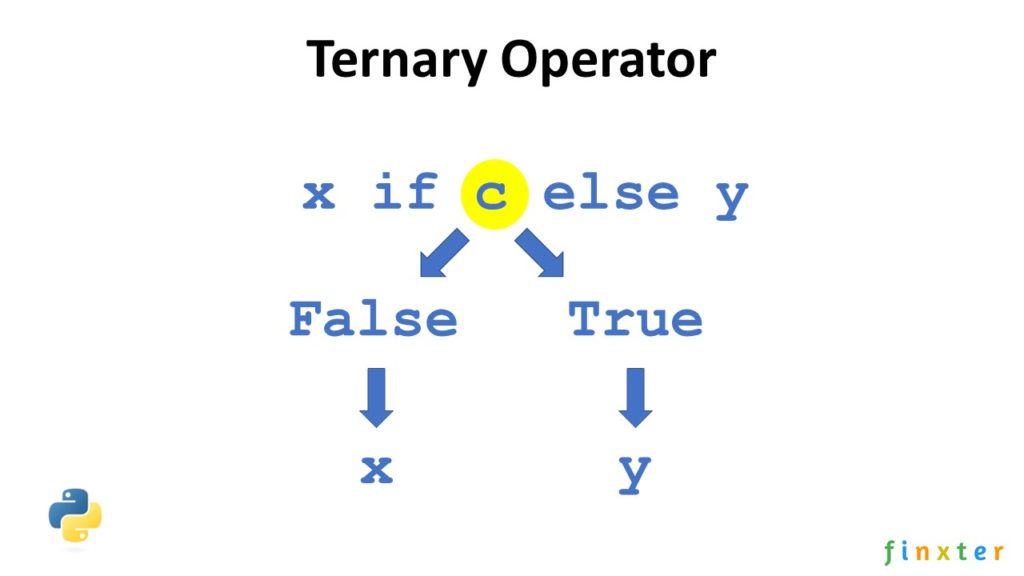
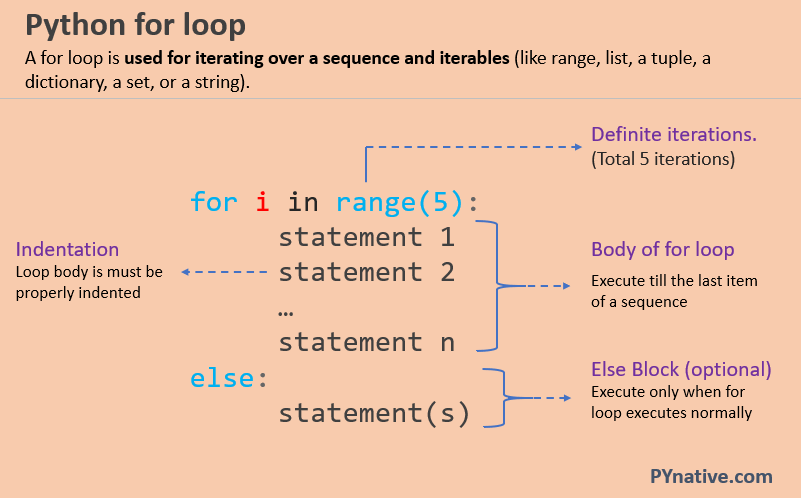
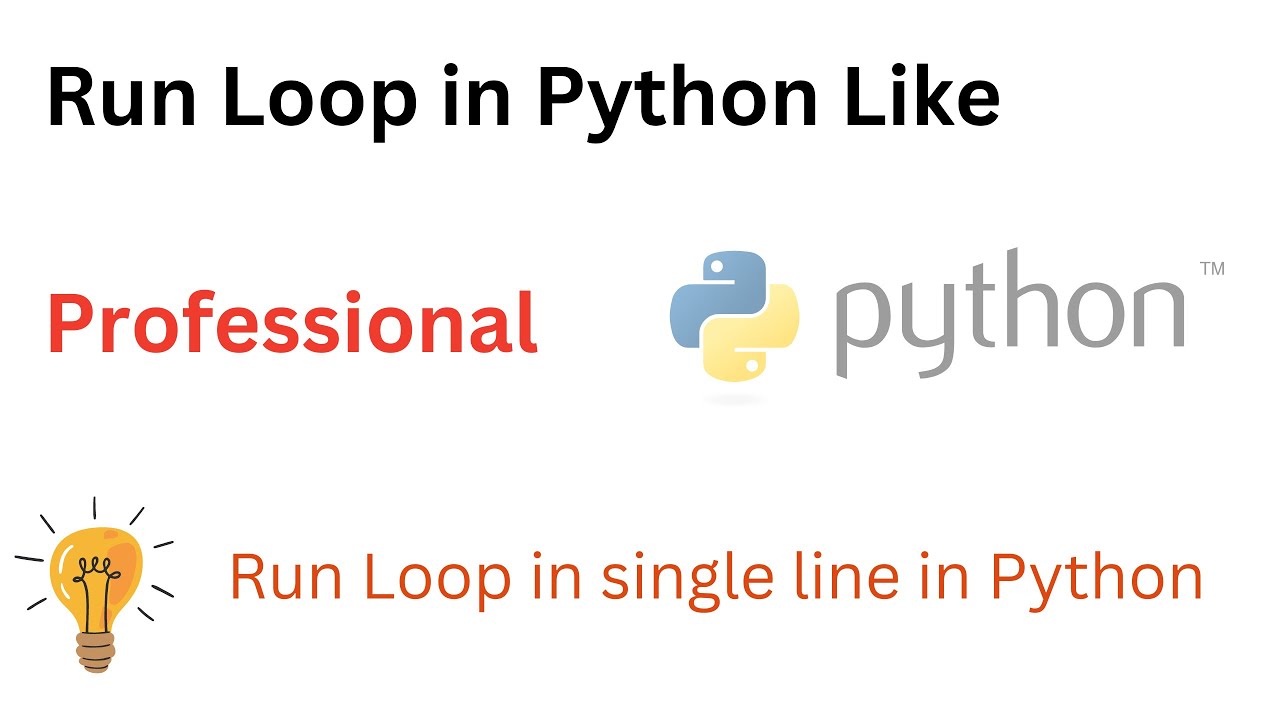
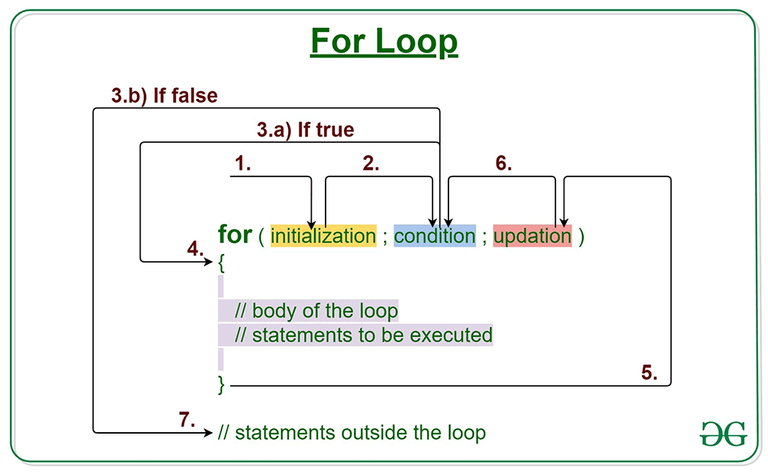
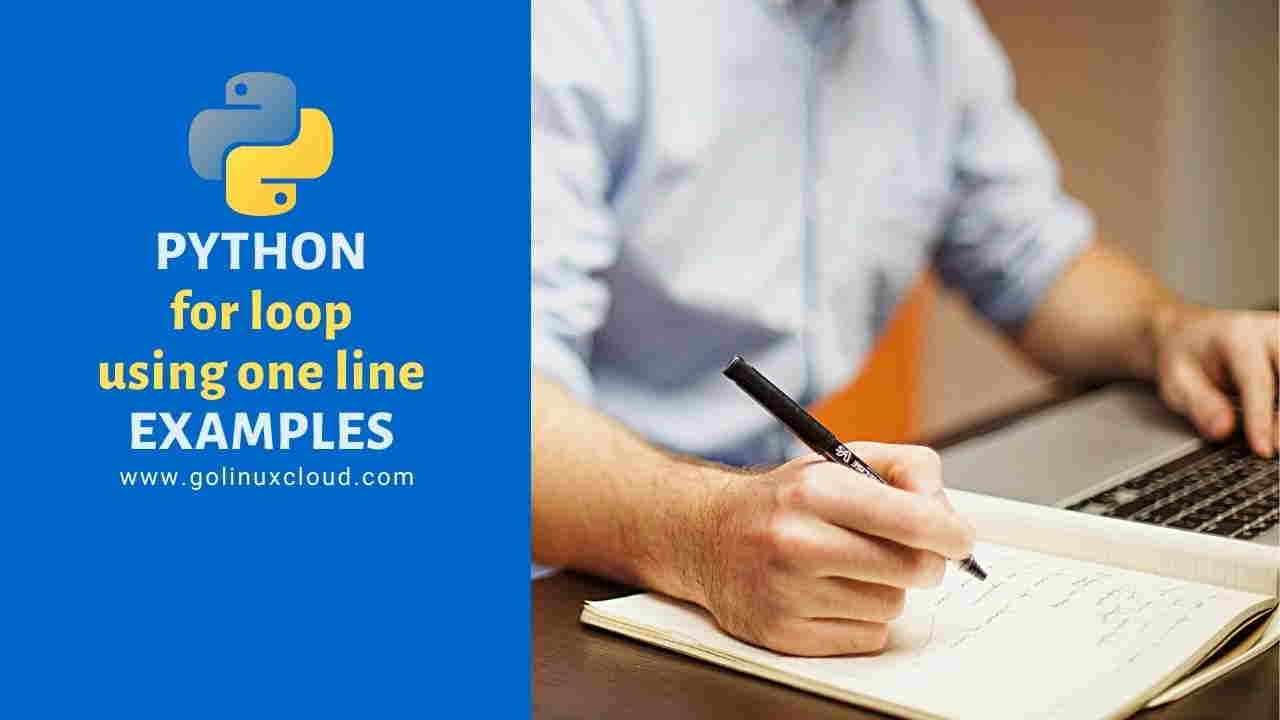

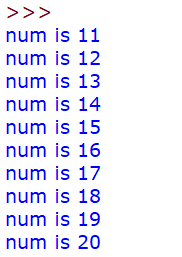

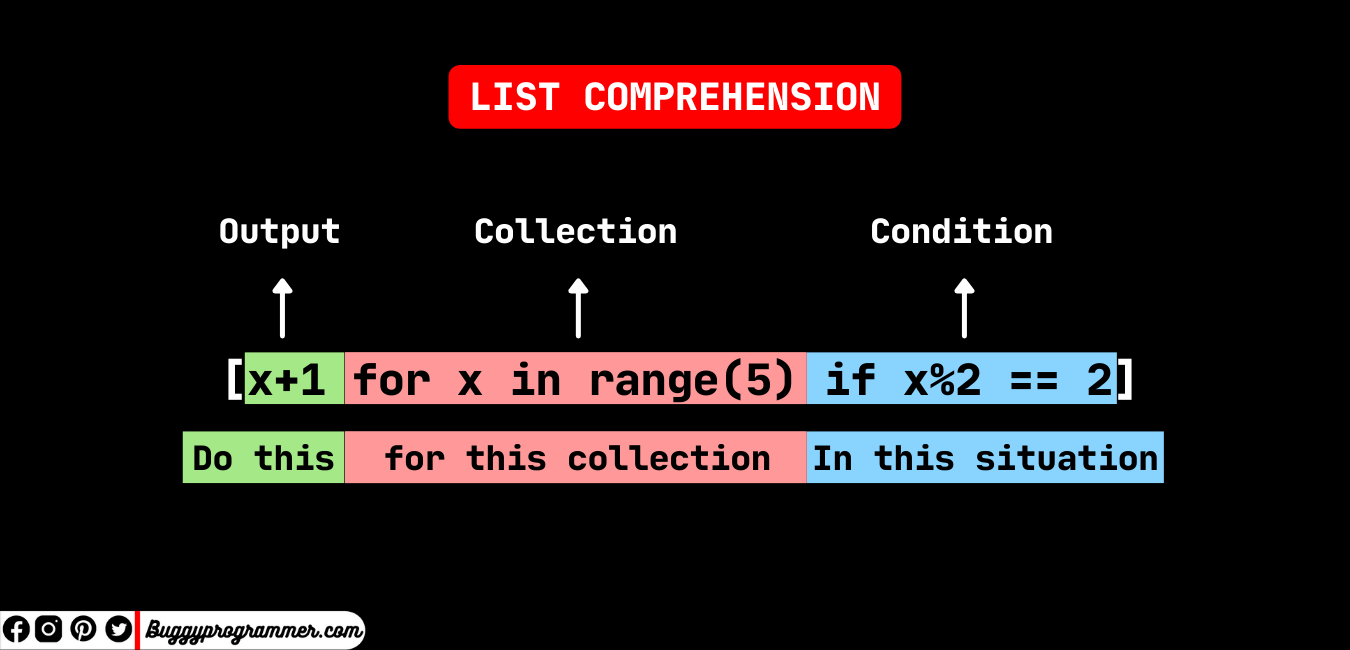

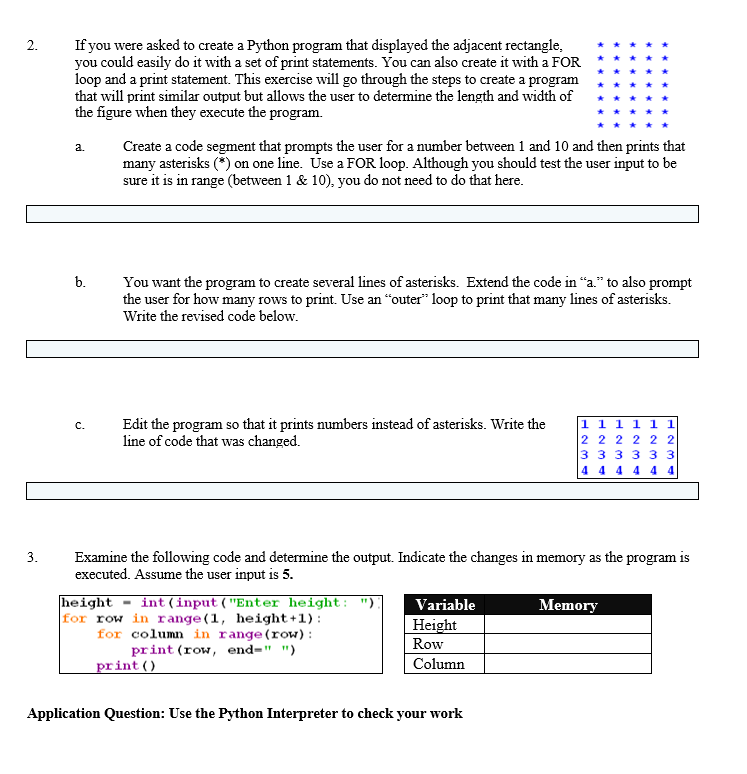
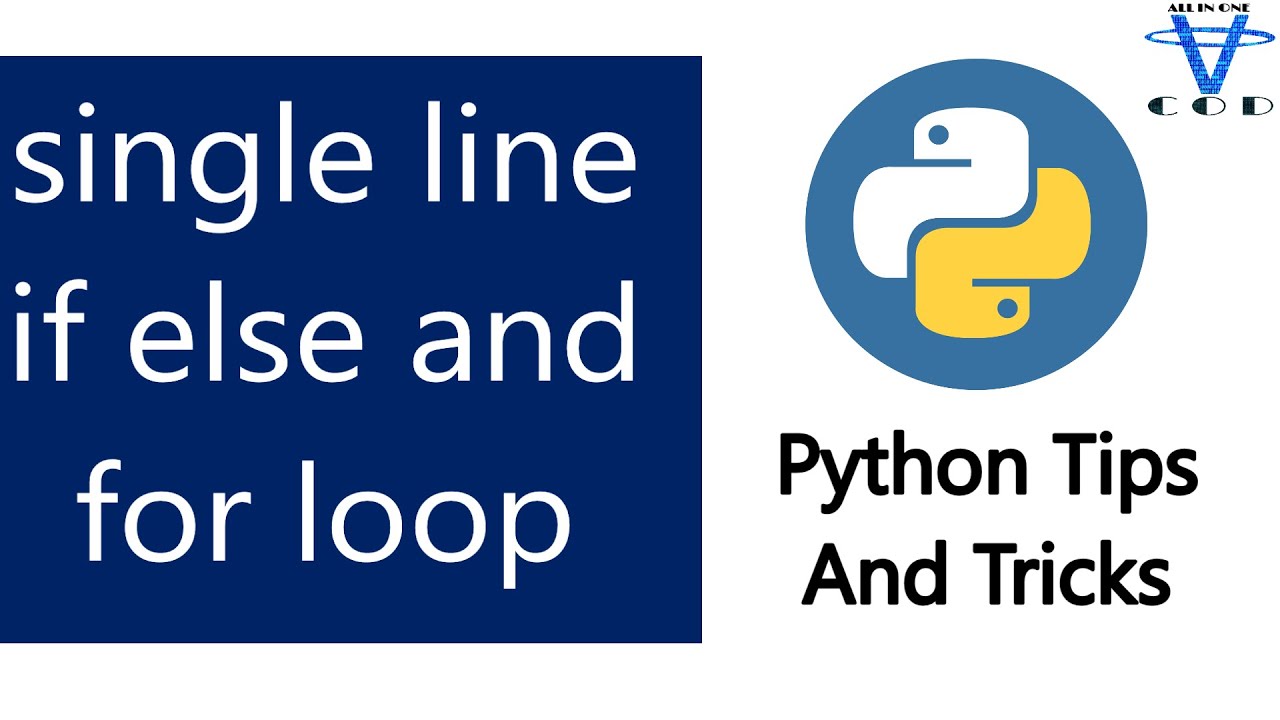
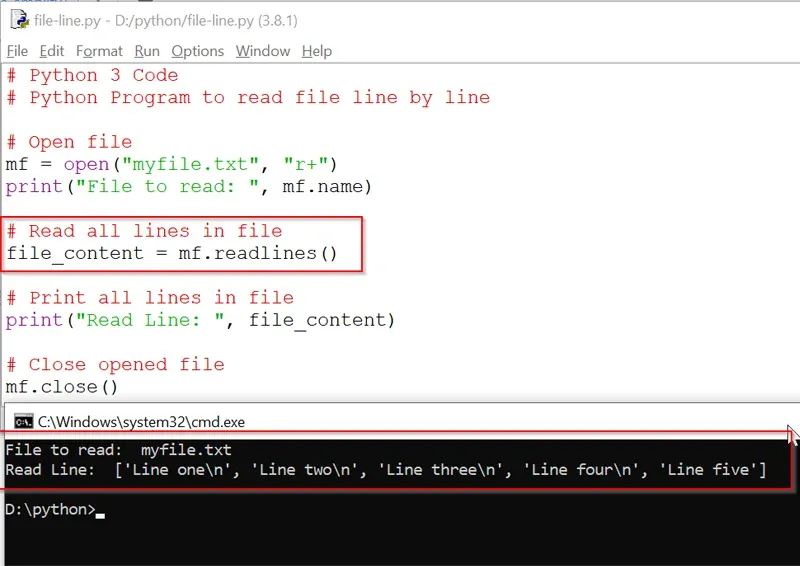
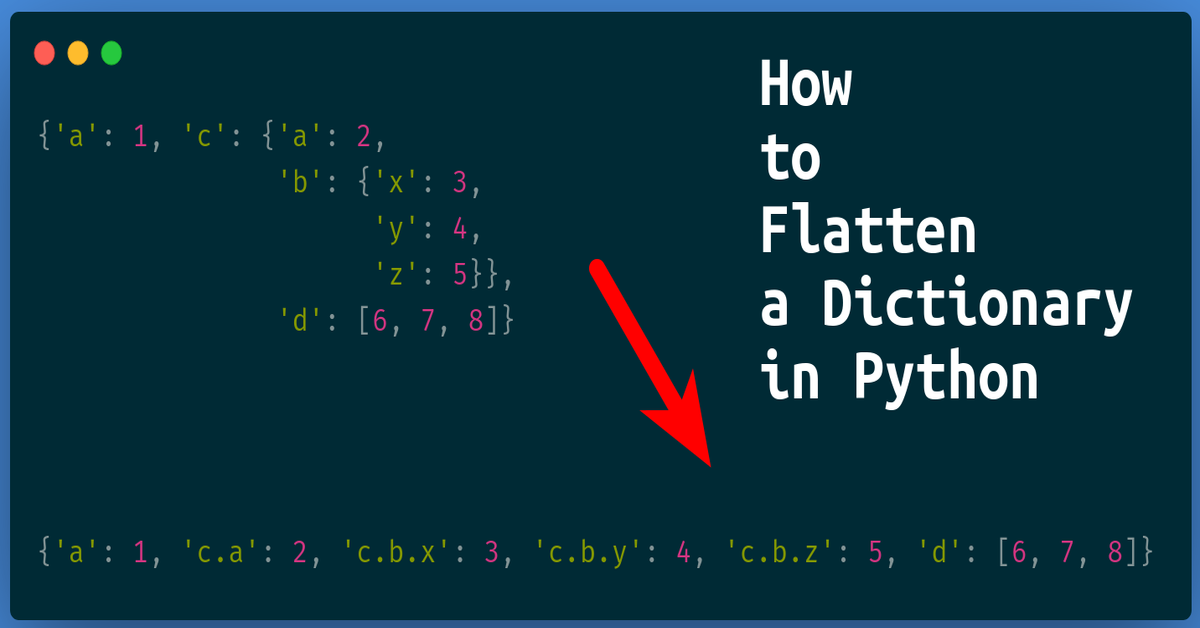
![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://i.ytimg.com/vi/9qsq2Vf48W8/maxresdefault.jpg)
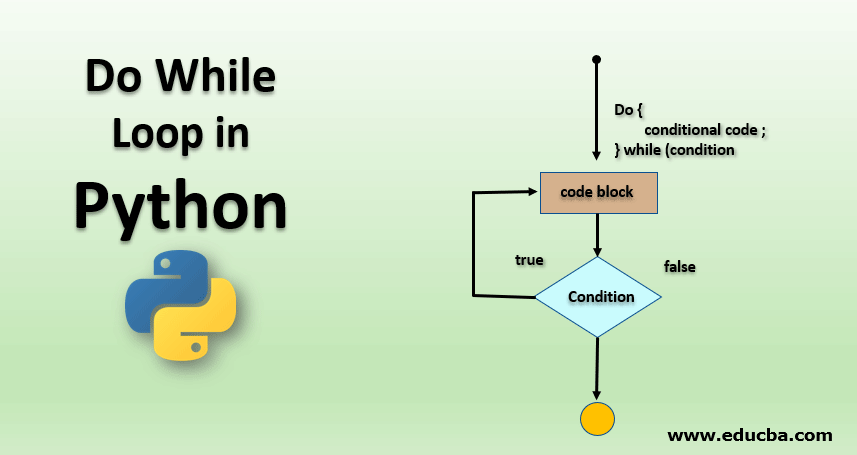
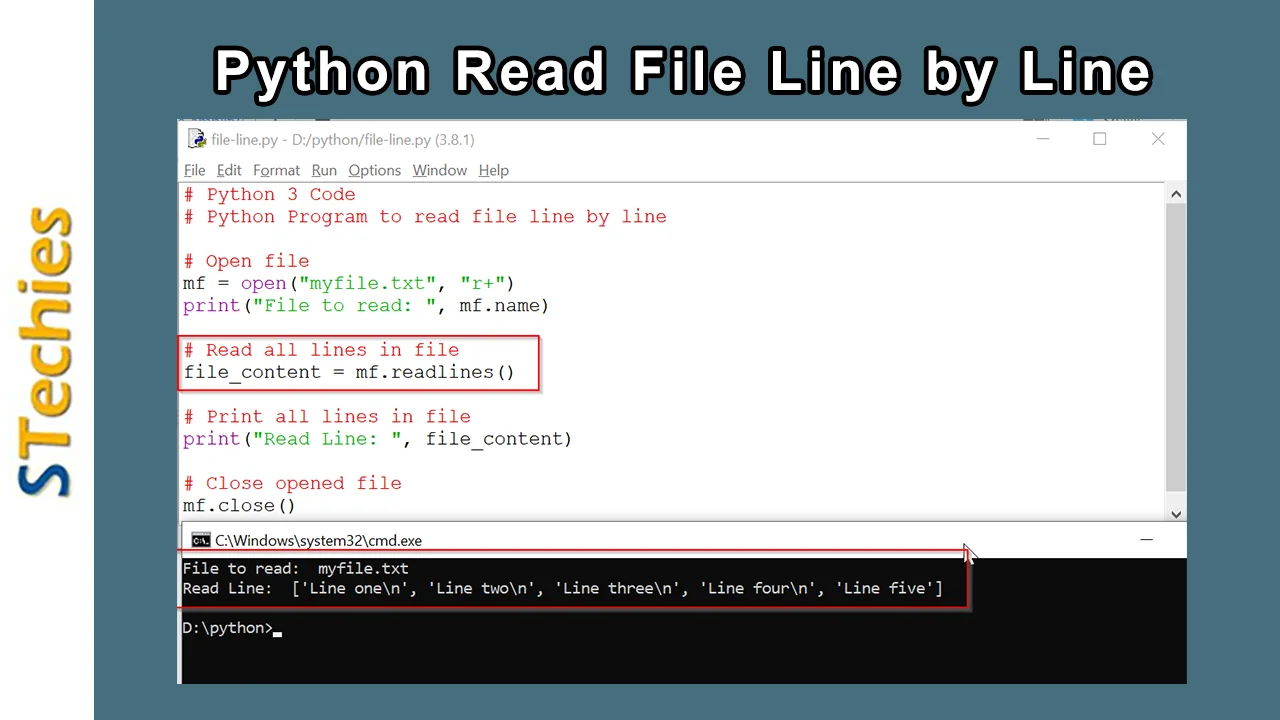
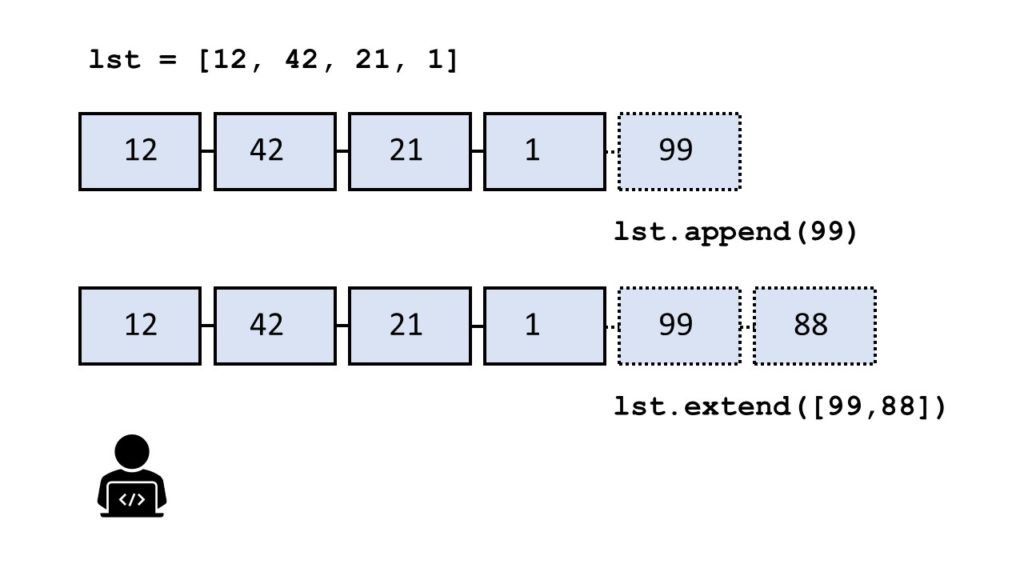
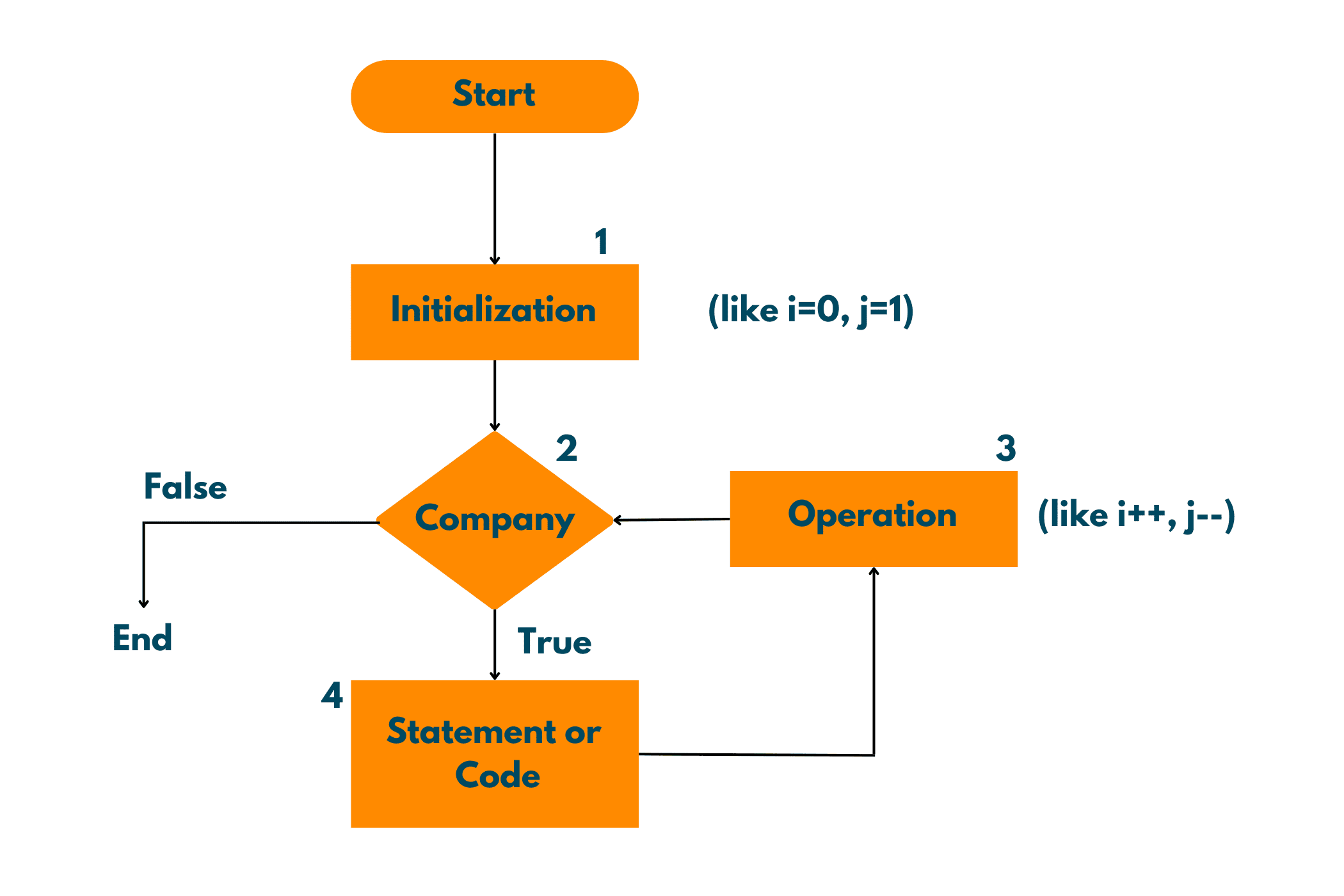
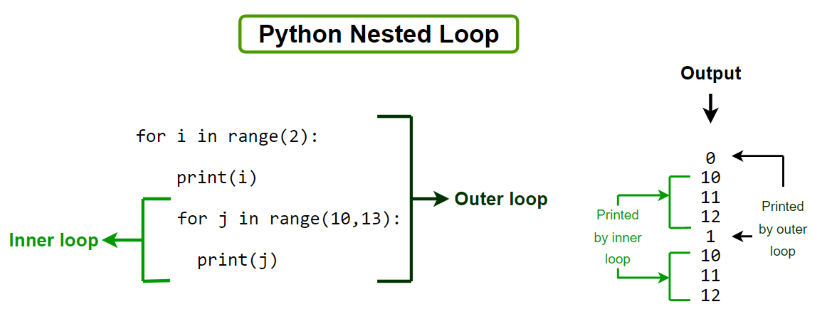
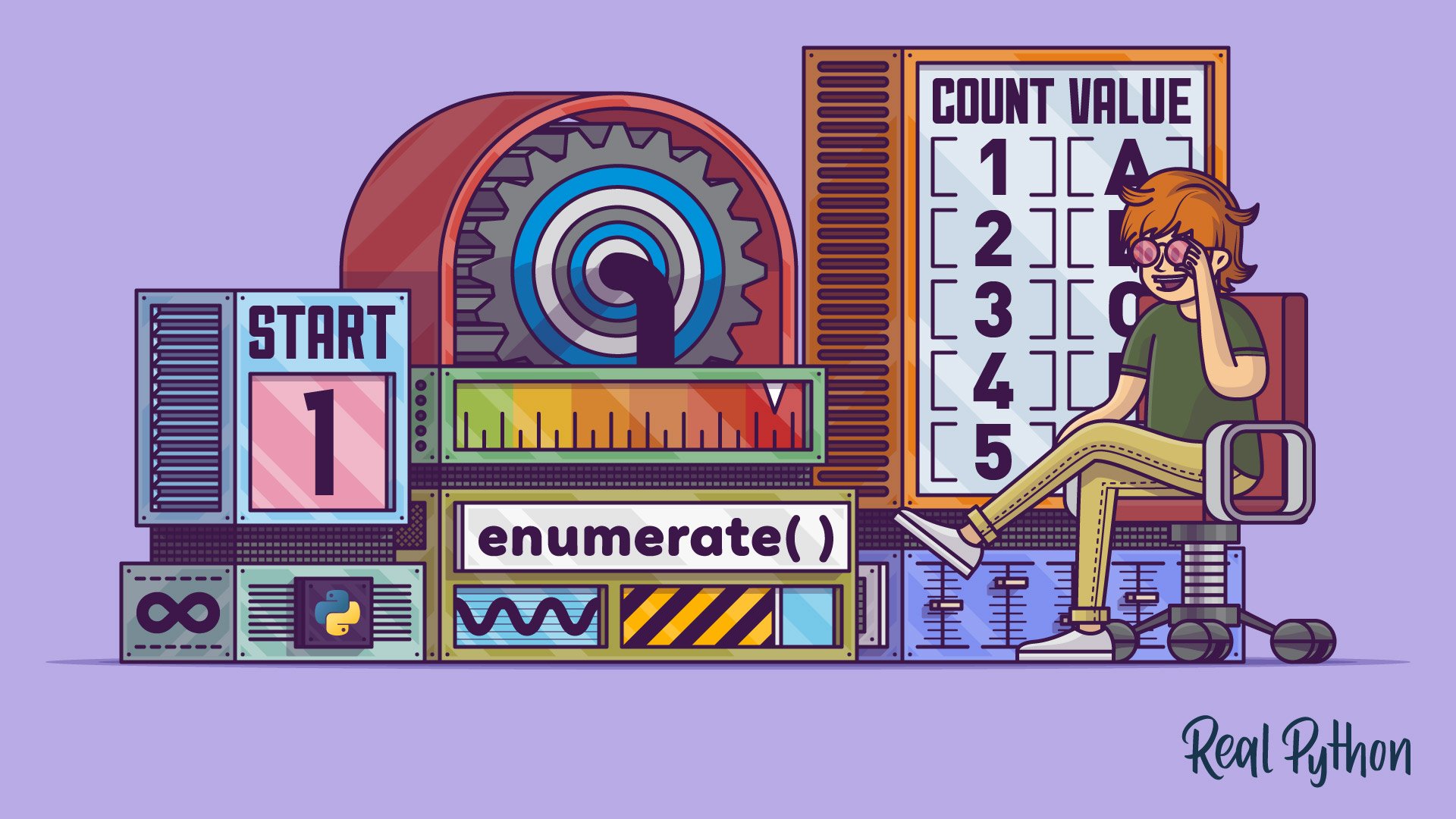
![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2023/01/image-224.png)

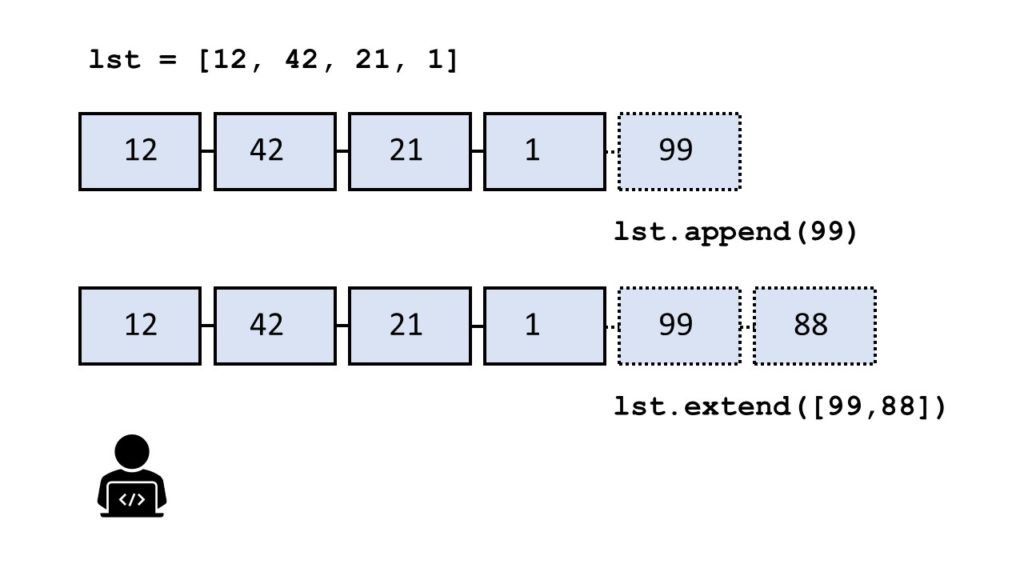

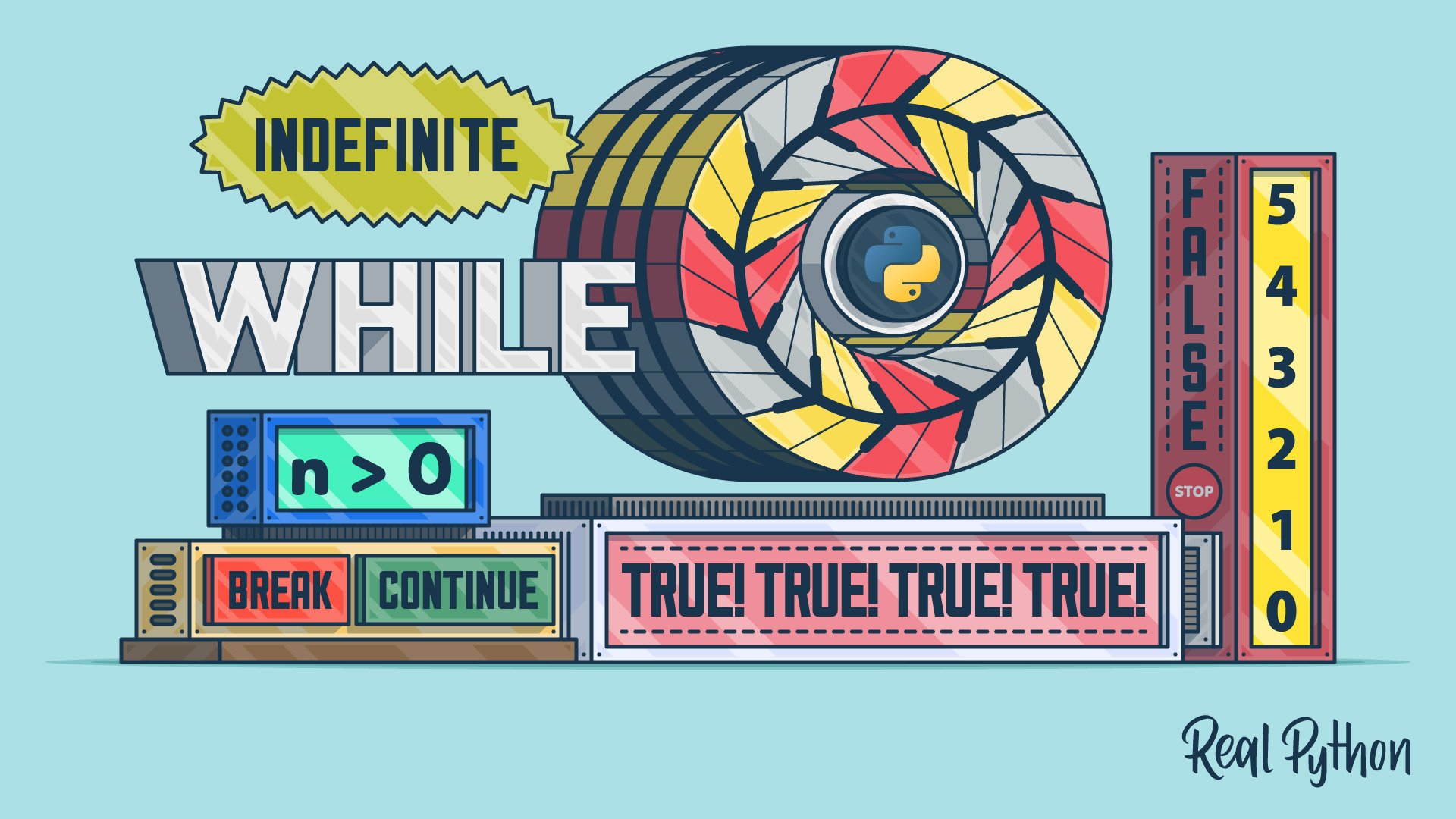
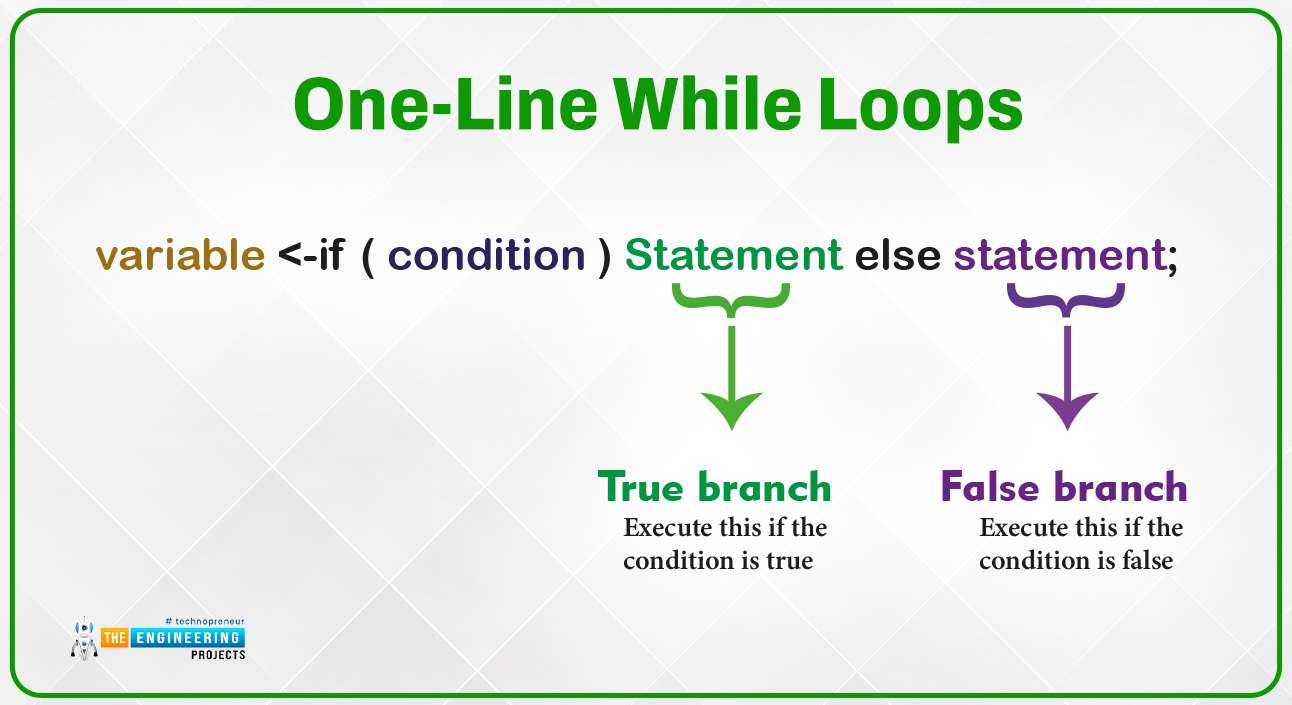

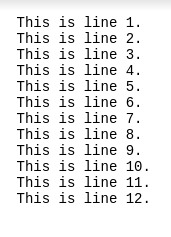
Article link: python one line for loop.
Learn more about the topic python one line for loop.
- Python One Line For Loop [A Simple Tutorial] – Finxter
- One Line for Loop in Python | Delft Stack
- Python for loop in one line explained with easy examples
- Python one-line “for” expression – lambda – Stack Overflow
- Python one line for loop tutorial – sebhastian
- One Line for Loop in Python – Its Linux FOSS
- 4 Ways To Write One-Liner For Loops in Python
- Python One Line for Loops [Tutorial] – Treehouse Blog
- Loops Condition in One line with Python – Level Up Coding
See more: https://nhanvietluanvan.com/luat-hoc