Python Print Object Attributes
1. Introduction to object attributes in Python
In Python programming, object attributes are variables that are associated with a specific object. These attributes hold data that defines the state and behavior of the object. Object attributes are crucial for encapsulating data within an object and providing access to it through specific methods.
2. Understanding object attributes and their significance in Python programming
Object attributes play a vital role in object-oriented programming as they define the characteristics and behavior of an object. These attributes can be accessed and modified through class methods or instance methods. They provide a way to store and manipulate data within an object, making it easier to manage and organize complex programs.
3. The dot notation: accessing object attributes using the dot operator
In Python, object attributes can be accessed using the dot notation, which involves using the dot operator (.) followed by the name of the attribute. This allows you to directly interact with and manipulate the data stored in the attribute.
4. Accessing and printing a single object attribute using the dot notation
To access a single object attribute, you can simply use the dot notation. For example, if we have an object named “person” with an attribute “name,” we can access it as follows:
“`python
person.name
“`
To print the value of the attribute, you can use the print() function:
“`python
print(person.name)
“`
5. Accessing and printing multiple object attributes using the dot notation
You can also access and print multiple object attributes using the dot notation by separating the attribute names with dots. For example, if we have an object named “person” with attributes “name,” “age,” and “gender,” we can access and print them as follows:
“`python
print(person.name, person.age, person.gender)
“`
This will print the values of all the specified attributes.
6. Dealing with AttributeError when accessing non-existent or misspelled object attributes
When accessing object attributes using the dot notation, it is important to ensure that the attributes exist and are correctly spelled. Otherwise, you may encounter an AttributeError. This error occurs when an object does not have the specified attribute.
To handle this error, you can use a try-except block. For example:
“`python
try:
print(person.address)
except AttributeError:
print(“Address attribute does not exist.”)
“`
If the specified attribute does not exist, the code within the except block will be executed, preventing the program from crashing.
7. Using getattr() function to access object attributes dynamically
Python provides the getattr() function, which allows you to access object attributes dynamically. This means that you can provide the attribute name as a string and retrieve its value. The getattr() function takes two arguments – the object and the attribute name.
“`python
value = getattr(person, “name”)
print(value)
“`
In this example, the value of the “name” attribute of the “person” object is retrieved and printed.
8. Printing all object attributes using the __dict__ attribute
To print all the attributes of an object, you can use the __dict__ attribute. This attribute is a dictionary that contains the key-value pairs of all the object attributes. You can iterate over this dictionary and print the attribute names and their corresponding values.
“`python
for attribute, value in person.__dict__.items():
print(attribute, “:”, value)
“`
This will print the name and value of each attribute in the object.
9. Differentiating between instance attributes and class attributes in Python
In Python, there are two types of attributes – instance attributes and class attributes.
Instance attributes are specific to each individual object. They are defined within the constructor or methods of a class and are accessed using the dot notation on each object.
Class attributes, on the other hand, are shared among all the objects of a class. They are defined outside the constructor or methods of a class and are accessed using the class name followed by the dot notation.
10. Overriding the __str__() method to customize the printing of object attributes
By default, when you print an object, Python displays the object’s class name and memory address. However, you can customize the printing of object attributes by overriding the __str__() method. This method allows you to define a string representation of the object.
“`python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f”Person: {self.name}, Age: {self.age}”
person = Person(“John”, 25)
print(person)
“`
In this example, the __str__() method is overridden to return a formatted string containing the name and age of the person object. When the object is printed, the customized string representation will be displayed.
FAQs
Q: How can I print all the attributes of an object in Python?
A: You can use the __dict__ attribute of the object to access the key-value pairs of all the attributes. Then, you can iterate over this dictionary and print the attribute names and their corresponding values.
Q: Can I access object attributes dynamically in Python?
A: Yes, you can use the getattr() function to access object attributes dynamically. This allows you to provide the attribute name as a string and retrieve its value.
Q: What should I do if I encounter an AttributeError when accessing object attributes?
A: If you encounter an AttributeError, it means that the object does not have the specified attribute. To handle this error, you can use a try-except block to prevent the program from crashing.
Q: How can I differentiate between instance attributes and class attributes in Python?
A: Instance attributes are specific to each individual object and are accessed using the dot notation on each object. Class attributes are shared among all the objects of a class and are accessed using the class name followed by the dot notation.
Q: How can I customize the printing of object attributes in Python?
A: You can override the __str__() method of the object’s class. This method allows you to define a string representation of the object. When the object is printed, the customized string representation will be displayed.
Unit 7 – Classes: Printing Attributes From A Class – Python
Keywords searched by users: python print object attributes Python print attributes, Print all attributes of object Python, Python print object as JSON, Object attributes python, Get all attributes python object, Print class attributes Python, Show all attributes of an object Python, Python iterate class attributes
Categories: Top 45 Python Print Object Attributes
See more here: nhanvietluanvan.com
Python Print Attributes
Introduction
In Python, the print() function is a fundamental tool used for displaying output to the console. While it may seem straightforward, there are several attributes and techniques that can enhance the functionality and customization of the output. This article aims to provide a comprehensive understanding of the print attributes available in Python, delving into their usage, customization, and best practices.
Understanding the Basics
Before we explore the various attributes associated with the print() function, let’s familiarize ourselves with its basic usage. In its simplest form, print() allows us to display text or variables on the console. For example:
“`python
print(“Hello, World!”)
“`
Output:
“`
Hello, World!
“`
By default, print() automatically appends a newline character at the end, resulting in the text appearing on a new line. However, it is possible to change this behavior by utilizing the ‘end’ attribute.
The ‘end’ Attribute
The ‘end’ attribute specifies the character(s) to be appended at the end of the print statement. By default, it is set to ‘\n’, denoting a newline. Yet, it can be modified to achieve different results.
For instance, the following code uses an empty string as the ‘end’ attribute, preventing the newline character from being appended:
“`python
print(“Hello”, end=”)
print(“World!”)
“`
Output:
“`
HelloWorld!
“`
Using the ‘end’ attribute, users can control the format, whether it be appending a space, a tab, or any other desired character(s). This is particularly useful when we want to concatenate multiple print statements together.
The ‘sep’ Attribute
Another crucial attribute related to the print() function is ‘sep’. The ‘sep’ attribute defines the separator between two or more items that are passed to the print() function.
Consider the following example:
“`python
print(“Apple”, “Banana”, “Orange”, sep=’, ‘)
“`
Output:
“`
Apple, Banana, Orange
“`
By default, items passed to print() are separated by a single space. However, the ‘sep’ attribute allows us to customize the separator. In the example above, we specified ‘, ‘ as the separator, resulting in a comma-space combination between each item.
The ‘file’ Attribute
In addition to displaying output on the console, the print() function also supports output redirection. This can be achieved by utilizing the ‘file’ attribute, which allows users to specify the stream to which the output will be directed. By default, ‘file’ is set to ‘sys.stdout’, which represents the console output.
Here’s an example demonstrating the usage of the ‘file’ attribute to redirect output to a file:
“`python
with open(‘output.txt’, ‘w’) as f:
print(“This is redirected output!”, file=f)
“`
By specifying the file object ‘f’ as the value for ‘file’, the text “This is redirected output!” will be written to a file named ‘output.txt’ instead of appearing on the console.
Frequently Asked Questions (FAQs)
Q1. Can I format the output of the print() function?
Yes, Python provides various ways to format the output of the print() function. One commonly used method is by utilizing the string formatting syntax, such as using placeholders or f-strings. For instance:
“`python
name = “Alice”
age = 25
print(“My name is {} and I am {} years old.”.format(name, age))
“`
Output:
“`
My name is Alice and I am 25 years old.
“`
Q2. Can I print variables along with the text?
Certainly! You can easily include variables in your print statements by either using placeholders or f-strings. Here’s an example with placeholders:
“`python
x = 10
y = 20
print(“The value of x is {} and y is {}.”.format(x, y))
“`
Output:
“`
The value of x is 10 and y is 20.
“`
Q3. Can I print on the same line without a space between the items?
Yes, you can achieve this by customizing the ‘end’ attribute. Simply use an empty string (”) as the value of ‘end’ to avoid appending any character(s) between statements. For instance:
“`python
print(“Hello”, end=”)
print(“World!”)
“`
Output:
“`
HelloWorld!
“`
Q4. How can I print formatted numbers?
To format numbers according to specific requirements, you can make use of Python’s string formatting syntax. For example, to print a float with two decimal places, you can use the {:.2f} formatter:
“`python
pi = 3.141592653589793
print(“The value of pi is {:.2f}.”.format(pi))
“`
Output:
“`
The value of pi is 3.14.
“`
Conclusion
In this comprehensive guide, we have explored the various attributes associated with the print() function, such as ‘end’, ‘sep’, and ‘file’. We have learned how to modify the appearance of output, redirect it to different streams, and format the printed text. By leveraging these attributes effectively, Python developers can enhance the readability and usability of their programs.
Remember, the print() function is not limited to displaying simple text; it is a powerful tool that can greatly aid in debugging, data visualization, and general program output. Understanding and utilizing these attributes will undoubtedly take your Python programming skills to the next level.
Print All Attributes Of Object Python
Python is a versatile and powerful programming language that allows developers to create and manipulate objects easily. Objects are instances of classes, which are the fundamental building blocks of object-oriented programming. Each object has its own set of attributes, which define its characteristics and behavior.
At times, it becomes necessary to inspect an object and view all its attributes. Fortunately, Python provides several ways to achieve this. In this article, we will explore different methods to print all attributes of an object in Python.
Using the dir() function
The simplest way to get a list of all attributes of an object is by using the built-in dir() function. The dir() function returns a sorted list of attributes and methods of the given object. It includes all the attributes inherited from its class and any additional attributes defined specifically for the object.
Here is an example:
“`python
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
my_car = Car(“Tesla”, “Model S”, 2022)
print(dir(my_car))
“`
Output:
“`
[‘__class__’, ‘__delattr__’, ‘__dir__’, ‘__doc__’, ‘__eq__’, ‘__format__’, ‘__ge__’, ‘__getattribute__’, ‘__gt__’, ‘__hash__’, ‘__init__’, ‘__init_subclass__’, ‘__le__’, ‘__lt__’, ‘__ne__’, ‘__new__’, ‘__reduce__’, ‘__reduce_ex__’, ‘__repr__’, ‘__setattr__’, ‘__sizeof__’, ‘__str__’, ‘__subclasshook__’, ‘make’, ‘model’, ‘year’]
“`
As seen in the output, the dir() function gives a list of all attributes, including the special attributes provided by Python itself. These special attributes usually start and end with double underscores (also known as “dunder” methods).
Using the vars() function
Another method to access an object’s attributes is by using the built-in vars() function. The vars() function returns a dictionary of the object’s attributes and their corresponding values.
Here is an example:
“`python
class Person:
def __init__(self, name, age, occupation):
self.name = name
self.age = age
self.occupation = occupation
person = Person(“John Doe”, 30, “Software Engineer”)
print(vars(person))
“`
Output:
“`
{‘name’: ‘John Doe’, ‘age’: 30, ‘occupation’: ‘Software Engineer’}
“`
In the output, we can see a dictionary containing the attributes and their values. This method is useful when you want to access the attribute values for further processing.
Using the getattr() function
The getattr() function is used to retrieve an attribute value of an object based on its name. By iterating over all possible attribute names, we can effectively print all attributes of an object.
Here is an example:
“`python
class Book:
def __init__(self, title, author, genre):
self.title = title
self.author = author
self.genre = genre
book = Book(“To Kill a Mockingbird”, “Harper Lee”, “Fiction”)
for attr_name in dir(book):
if not attr_name.startswith(“__”):
attr_value = getattr(book, attr_name)
print(attr_name, “:”, attr_value)
“`
Output:
“`
author : Harper Lee
genre : Fiction
title : To Kill a Mockingbird
“`
As shown in the output, this approach prints each attribute name and its corresponding value. However, it excludes the special attributes starting with double underscores.
Using a custom function
If none of the above methods suit your requirements, you can create a custom function to print all attributes of an object. This approach allows you to define the output format and filter attributes based on certain criteria.
Here is an example:
“`python
class Laptop:
def __init__(self, brand, model, price):
self.brand = brand
self.model = model
self.price = price
def print_attributes(obj):
for attr_name in dir(obj):
if not attr_name.startswith(“__”):
attr_value = getattr(obj, attr_name)
print(attr_name, “:”, attr_value)
laptop = Laptop(“Dell”, “XPS 13”, 1599)
print_attributes(laptop)
“`
Output:
“`
brand : Dell
model : XPS 13
price : 1599
“`
In this example, the custom function `print_attributes()` is defined to print all attributes of an object. You can modify this function to fit your specific needs.
FAQs
1. Can I print only the attributes specific to an object and exclude attributes inherited from its class?
Yes, the `dir()` function provides a list of all attributes, including those inherited from the class. You can filter out inherited attributes based on their class using `object.__class__`.
2. How can I print the attributes of built-in Python objects like lists and dictionaries?
Built-in Python objects have their own set of attributes and methods. You can use the `dir()` or `vars()` function to access these attributes.
3. Can I print the attributes of an object defined in a module or package without importing them?
No, you need to import the module or package containing the object before accessing its attributes. Alternatively, you can use the `inspect` module to dynamically retrieve information about objects without importing them.
In conclusion, Python provides several convenient ways to print all attributes of an object. Depending on your requirements, you can choose between built-in functions such as `dir()` and `vars()`, or create a custom function. Understanding an object’s attributes aids in effective debugging, testing, and overall object-oriented programming.
Python Print Object As Json
To print objects as JSON in Python, we can take advantage of the built-in JSON module. This module provides functions that enable us to convert Python objects into JSON strings and vice versa. The first step is to import the JSON module by using the following line of code:
“`python
import json
“`
Once the JSON module is imported, we can proceed to serialize Python objects into JSON. Serialization is the process of converting an object into a format that can be stored or transmitted. To achieve this, the JSON module provides the `json.dumps()` function, which takes in a Python object and returns a JSON string. Let’s consider a simple example:
“`python
person = {
“name”: “John Doe”,
“age”: 30,
“city”: “New York”
}
json_string = json.dumps(person)
print(json_string)
“`
In the above code snippet, we have a Python dictionary named `person` that contains information about an individual. We use the `json.dumps()` function to convert the `person` dictionary into a JSON string, which is then printed using the `print()` function. The output of this code will be:
“`
{“name”: “John Doe”, “age”: 30, “city”: “New York”}
“`
The Python dictionary has been successfully serialized into a JSON string. Note that the keys in the JSON string are enclosed in double quotes, as per the JSON specification.
Besides dictionaries, Python objects such as lists, tuples, and simple data types like integers and strings can also be serialized using `json.dumps()`. However, certain Python objects, known as complex objects, cannot be directly serialized into JSON. These objects include custom classes, complex data structures like sets, and objects that contain properties that are not serializable (e.g., functions or methods).
In order to serialize complex objects, we can define our own JSON encoder class and inherit from the `json.JSONEncoder` class provided by the JSON module. This allows us to define how the complex objects should be converted into JSON. Here’s an example:
“`python
class Person:
def __init__(self, name, age, city):
self.name = name
self.age = age
self.city = city
person = Person(“John Doe”, 30, “New York”)
class PersonEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Person):
return {“name”: obj.name, “age”: obj.age, “city”: obj.city}
return super().default(obj)
json_string = json.dumps(person, cls=PersonEncoder)
print(json_string)
“`
In this code snippet, we define a custom class `Person` with attributes `name`, `age`, and `city`. We then instantiate an instance of the `Person` class named `person`. To correctly serialize the `person` object, we have to define a custom encoder class `PersonEncoder` that extends `json.JSONEncoder` and overrides its `default()` method. Within this method, we check if the object being serialized is of type `Person` and return a dictionary representation of the object’s attributes. Finally, we pass the `PersonEncoder` class to the `json.dumps()` function using the `cls` parameter to indicate that the custom encoder should be used.
This approach allows us to customize the serialization process for complex objects and ensures they can be properly converted into JSON strings.
FAQs:
Q: Can Python print nested objects as JSON?
A: Yes, Python can print nested objects as JSON. The JSON module handles the serialization of nested objects automatically. When you serialize an object that contains other objects, such as dictionaries within lists or nested dictionaries, the JSON module will handle the serialization recursively, converting the entire structure into a valid JSON string.
Q: What happens if a Python object cannot be serialized into JSON?
A: If a Python object cannot be serialized into JSON due to it being a complex object or containing non-serializable properties, a `TypeError` will be raised. To overcome this, you can define a custom encoder class, as shown earlier, to provide instructions on how to handle the serialization of those specific objects.
Q: How can I pretty-print the JSON output in Python?
A: The JSON module provides a `json.dumps()` function parameter called `indent` that allows you to specify the number of spaces for indentation. By setting `indent` to a positive integer value, you can easily format the JSON string for better readability. For example: `json.dumps(data, indent=4)`.
Q: Can Python deserialize a JSON string back into a Python object?
A: Yes, the JSON module in Python can deserialize JSON strings back into Python objects using the `json.loads()` function. This function takes in a JSON string and returns a Python object. It is the inverse operation of serialization, effectively translating a JSON string into its corresponding Python object.
In summary, Python’s JSON module provides an easy way to print objects as JSON strings. By utilizing the `json.dumps()` function, we can serialize Python objects into JSON. For more complex objects, custom encoder classes can be defined to handle their serialization. Python’s ability to seamlessly convert between Python objects and JSON strings is a valuable feature for working with data interchange and communication between different systems.
Images related to the topic python print object attributes
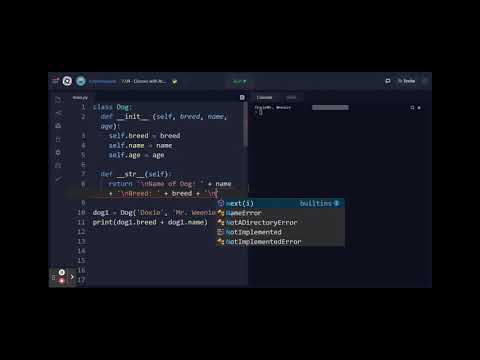
Found 6 images related to python print object attributes theme
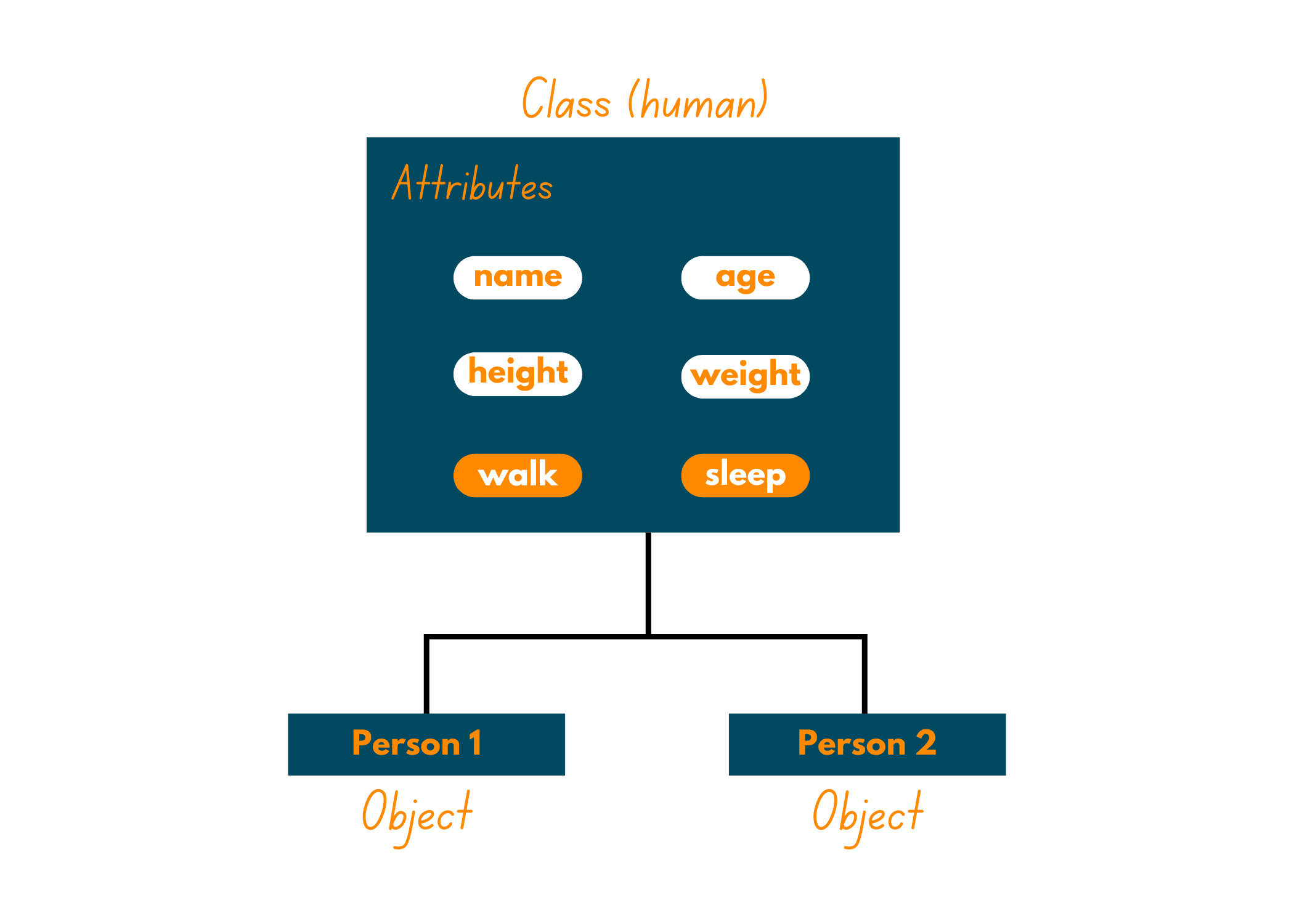
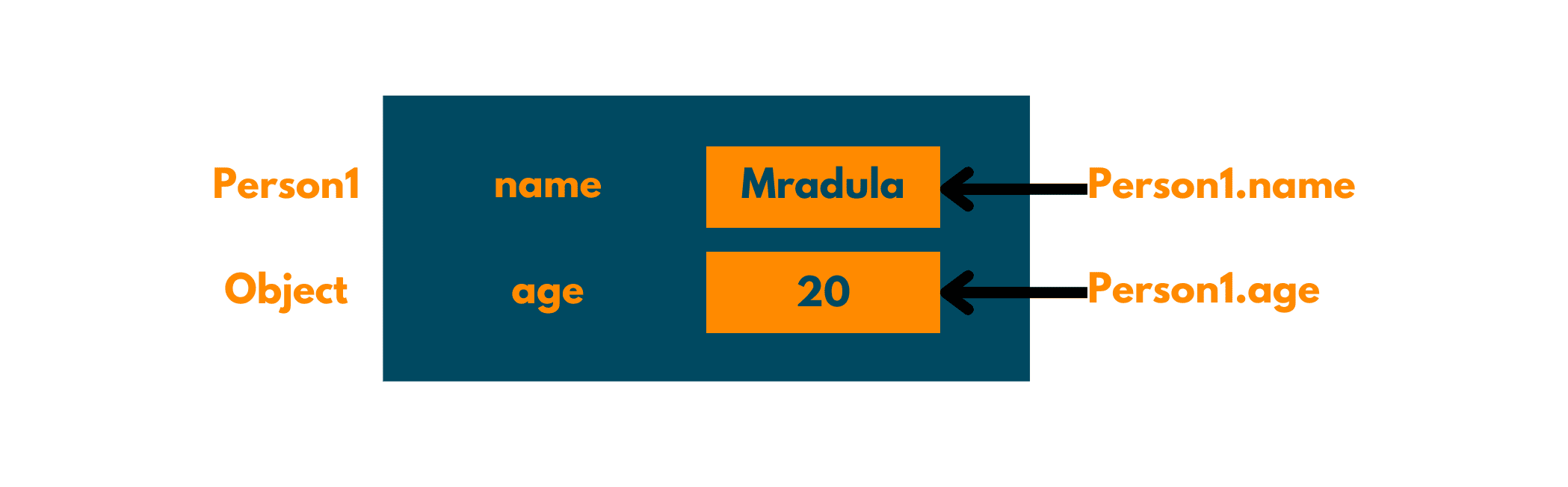
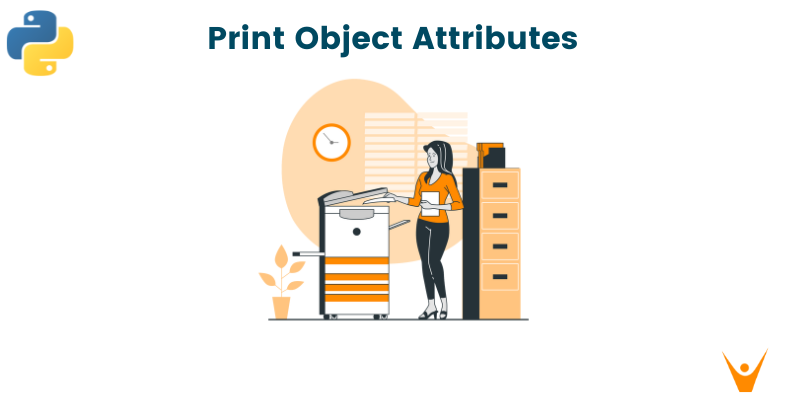

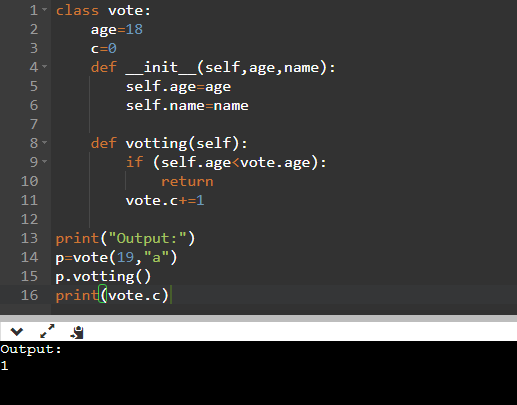

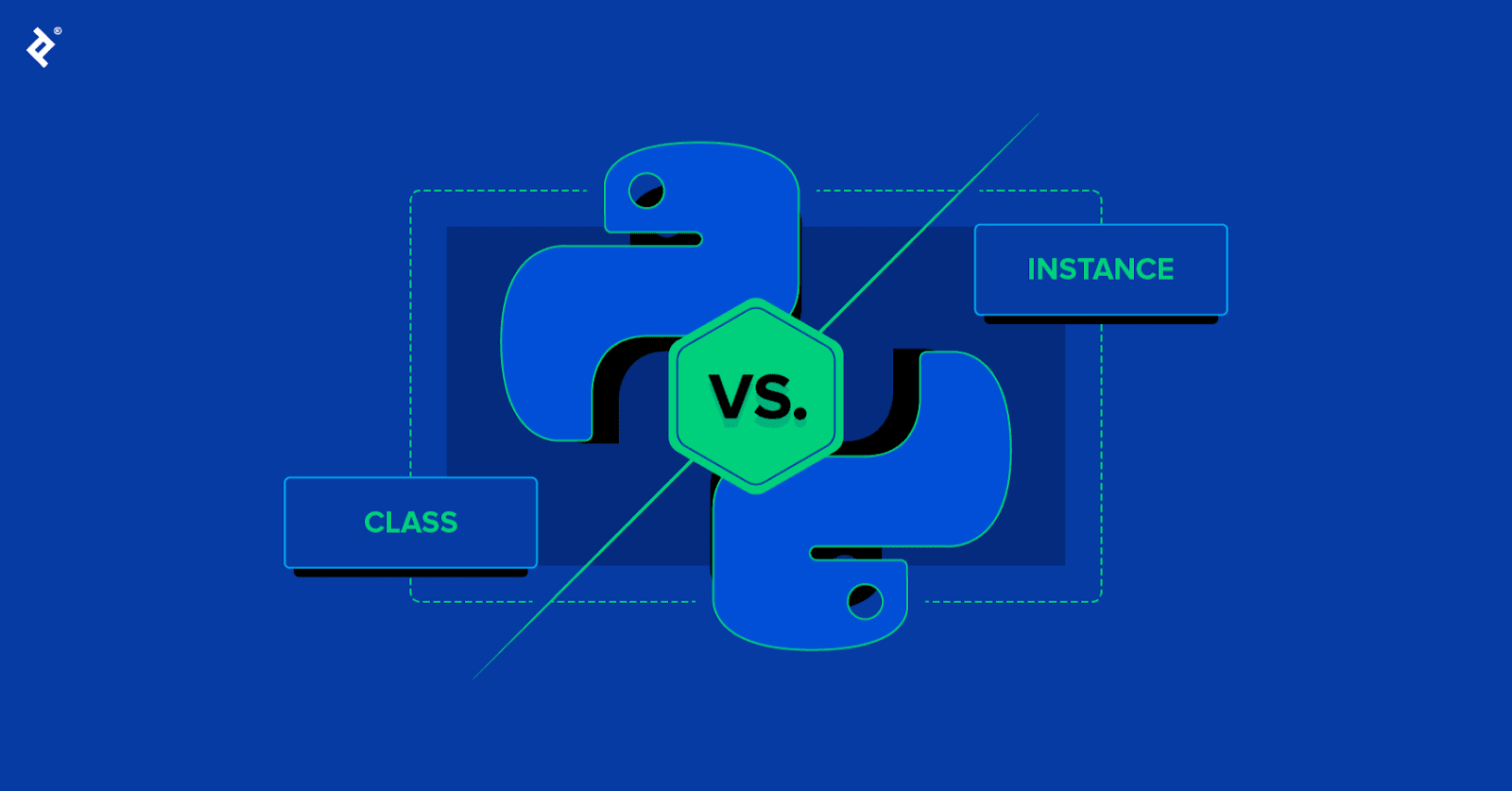
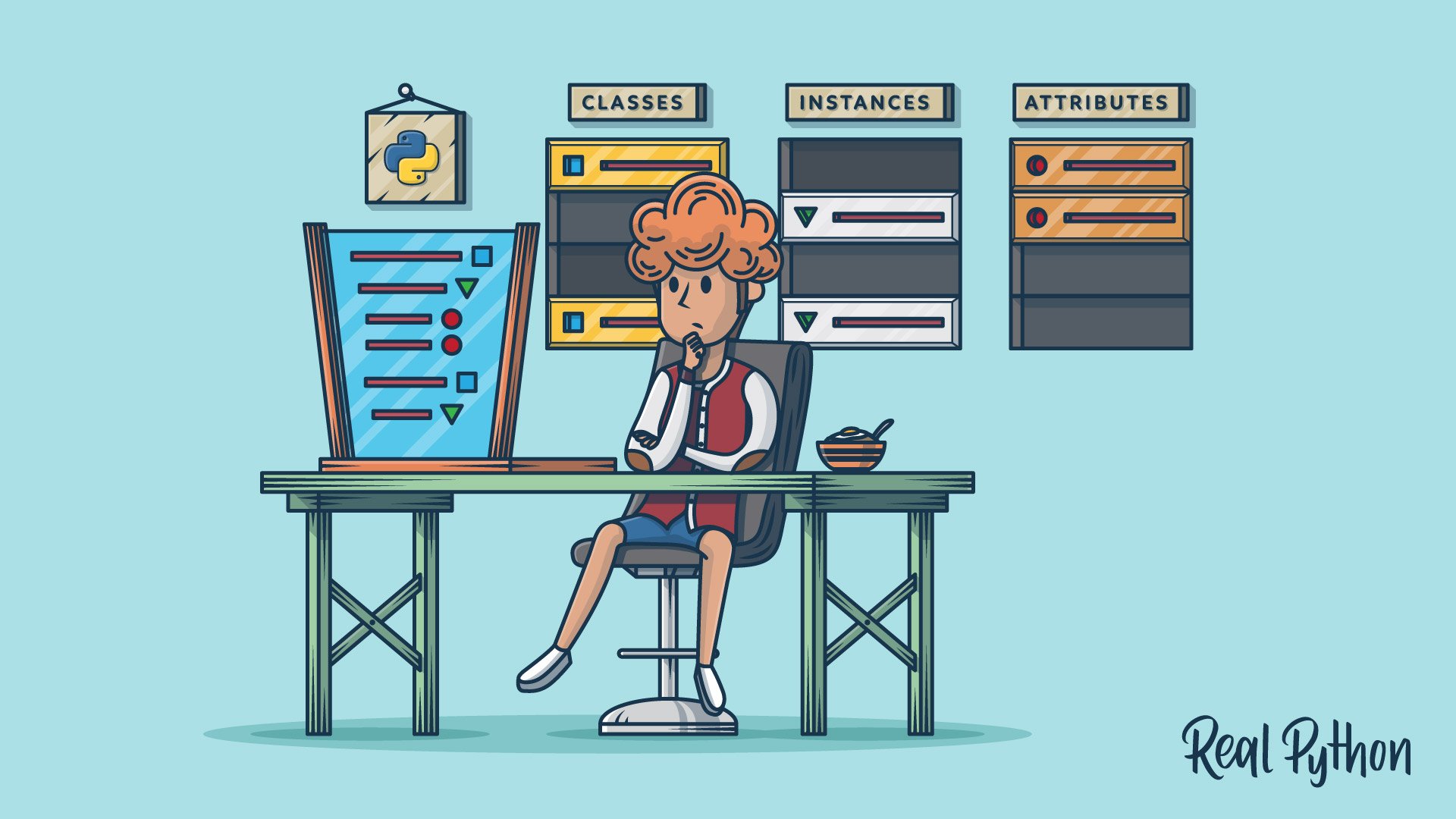
![FIXED] AttributeError: 'NoneType' object has no attribute 'something' – Be on the Right Side of Change Fixed] Attributeerror: 'Nonetype' Object Has No Attribute 'Something' – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2021/12/AttributeError-1024x576.png)
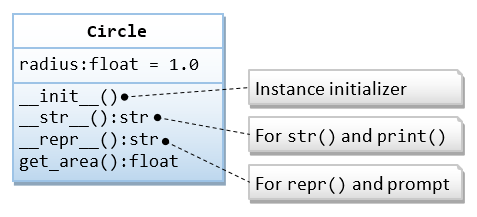

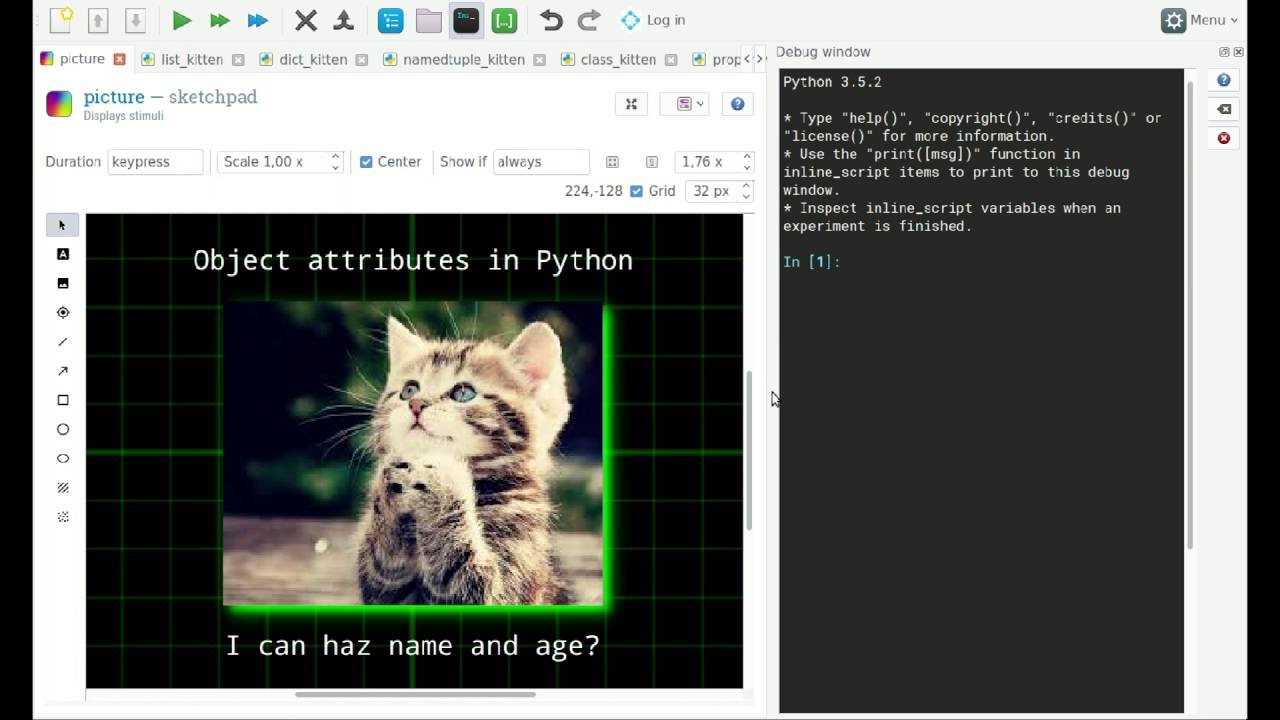
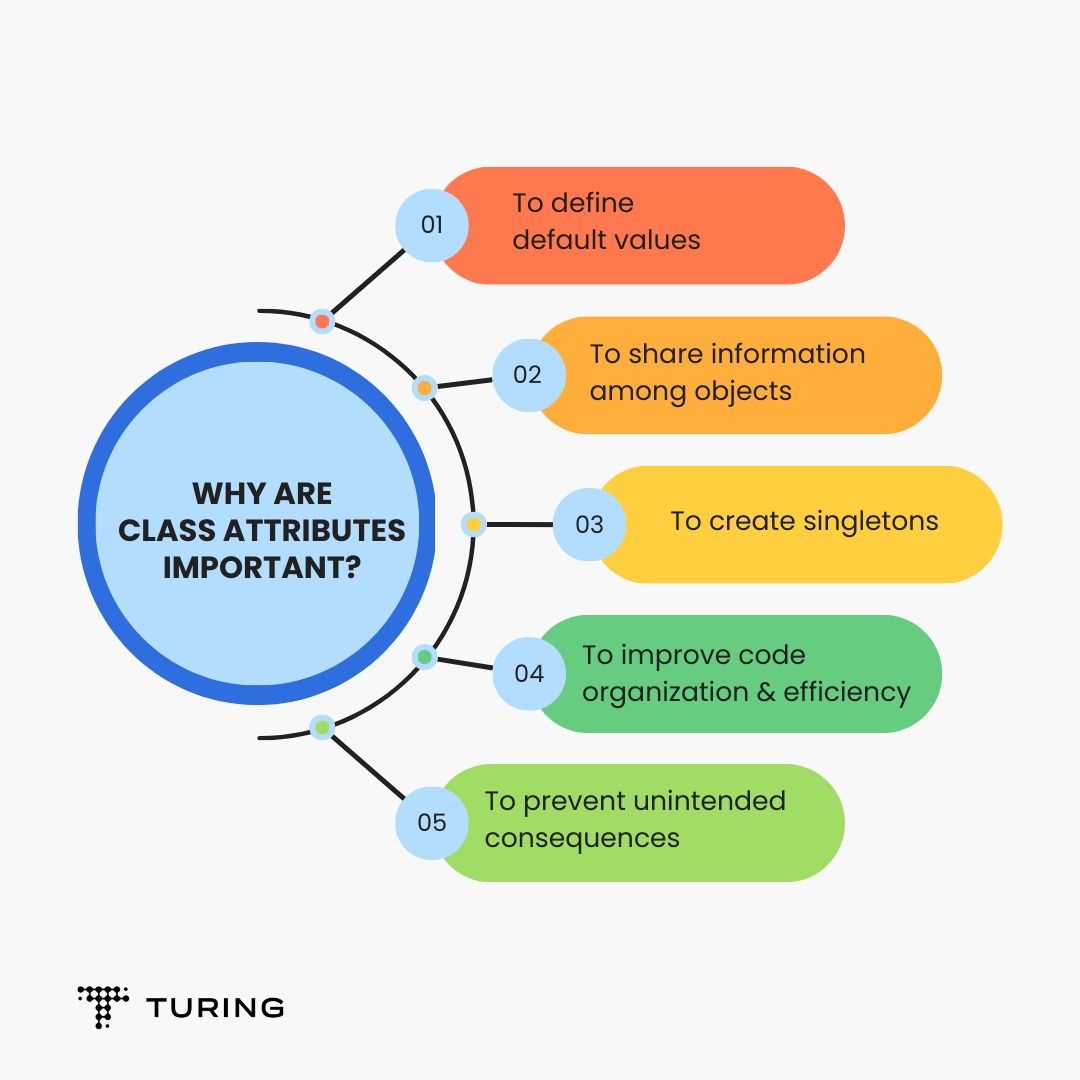
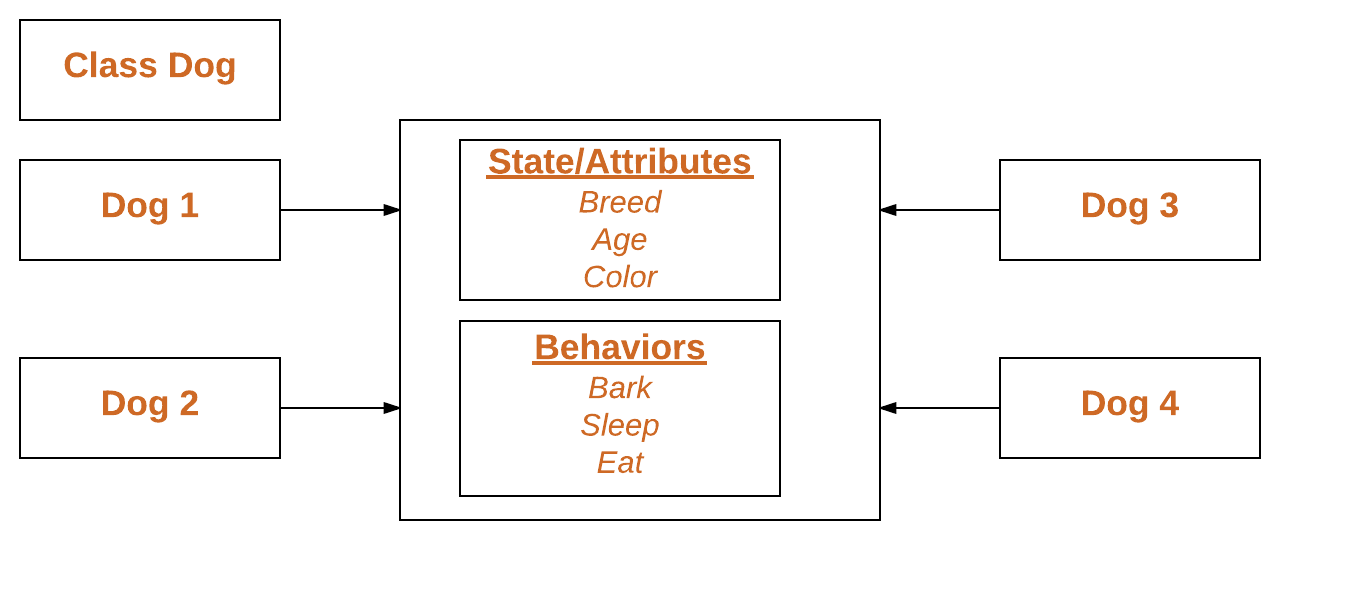

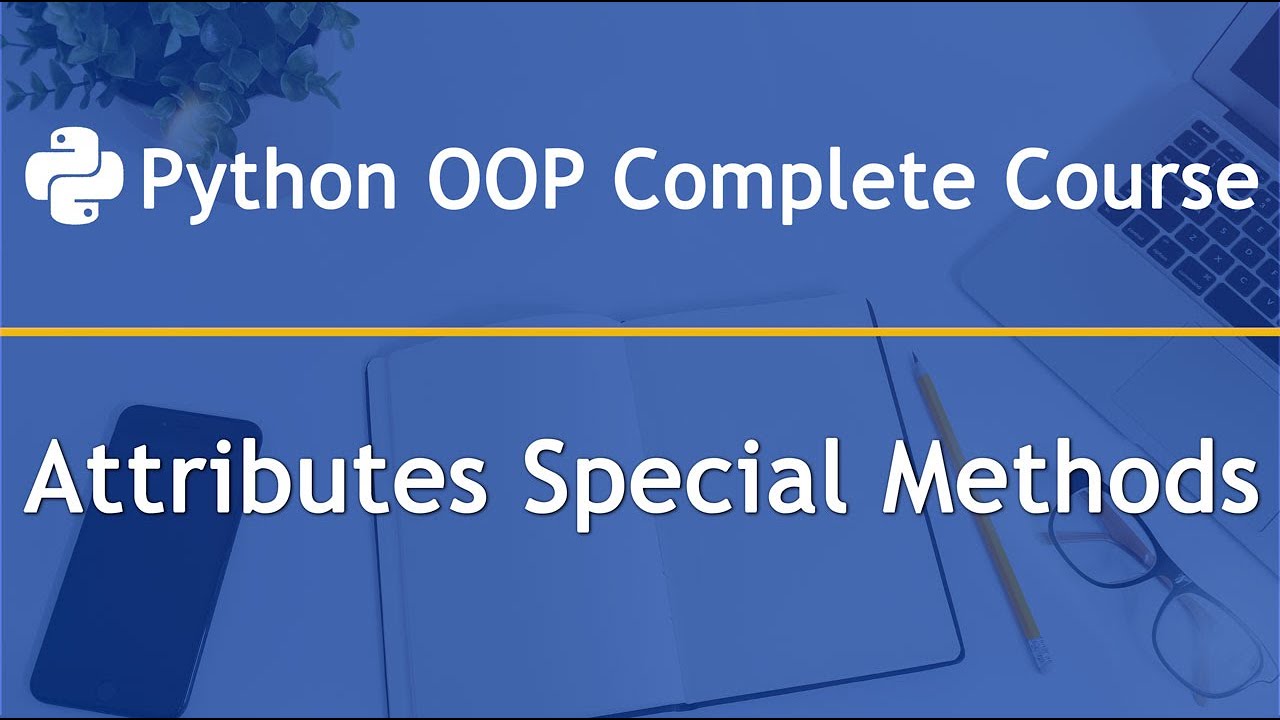
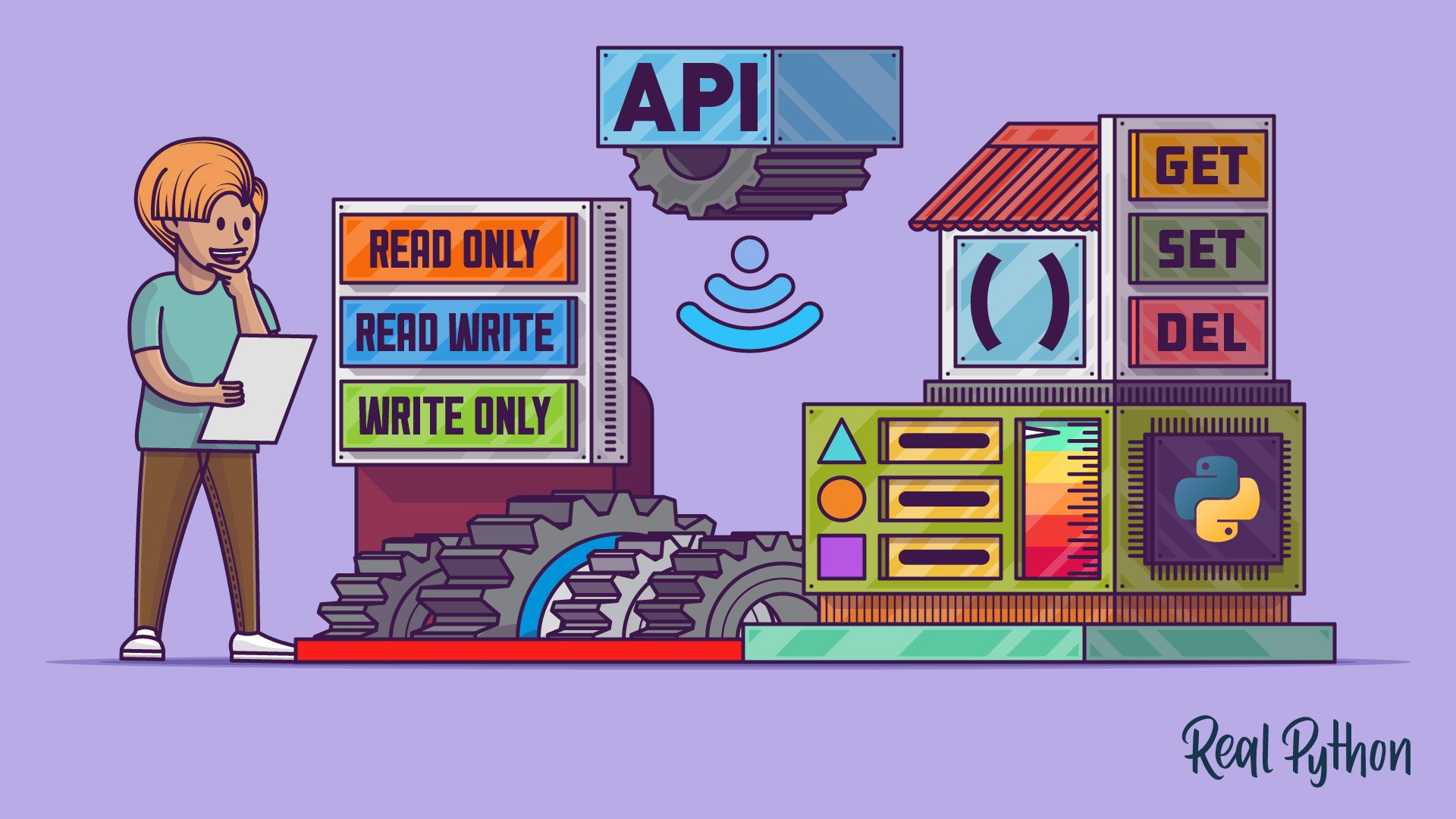


![Python Classes and Objects [Guide] – PYnative Python Classes And Objects [Guide] – Pynative](https://pynative.com/wp-content/uploads/2021/08/class_and_objects.jpg)
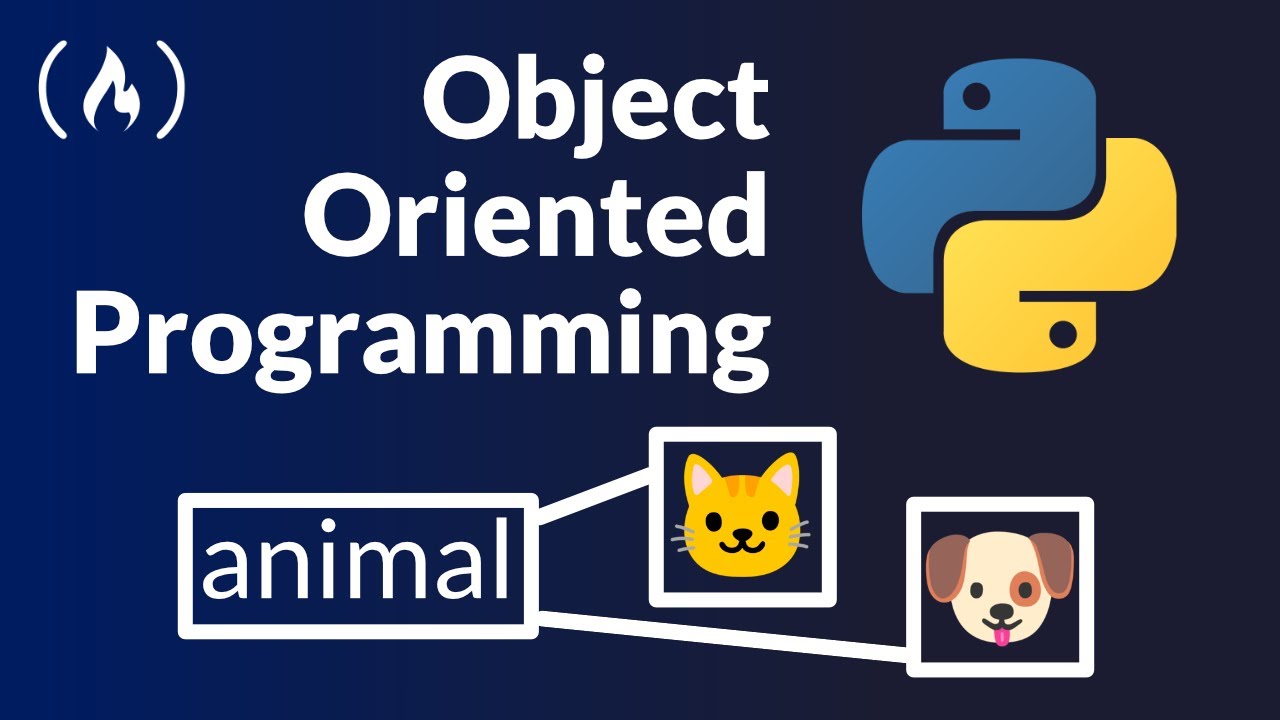



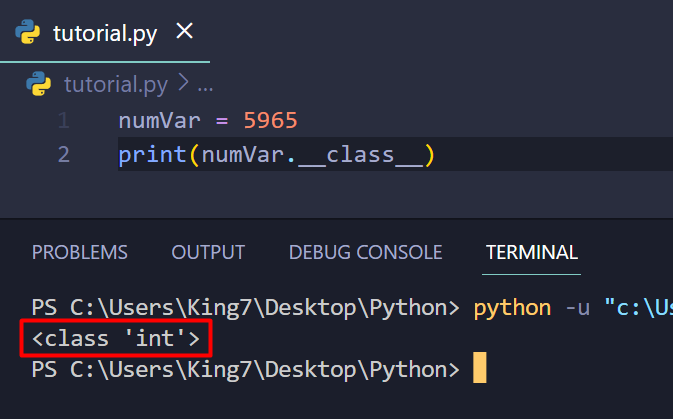

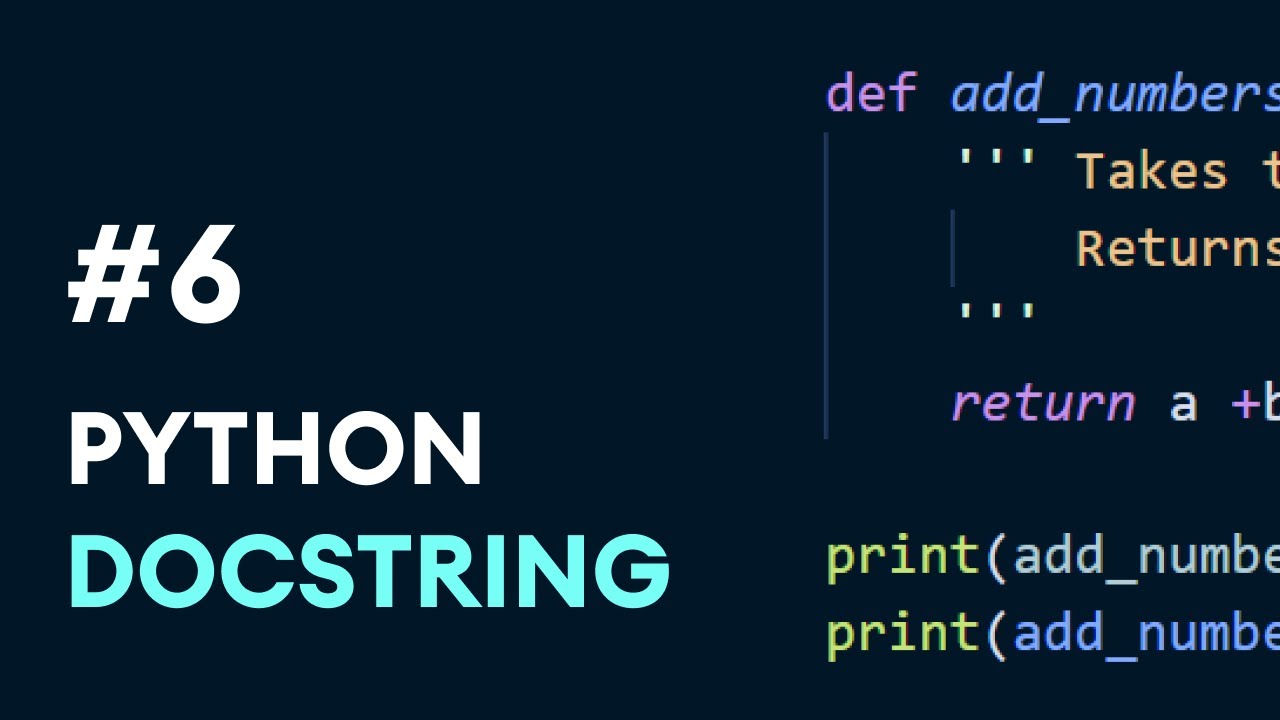
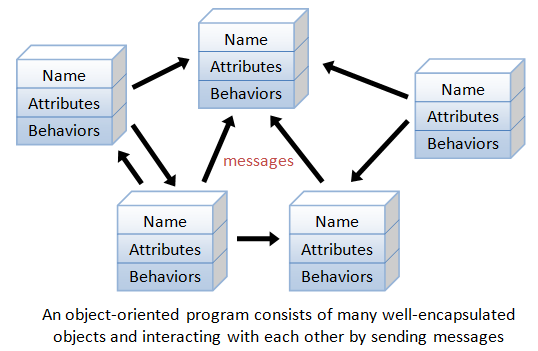
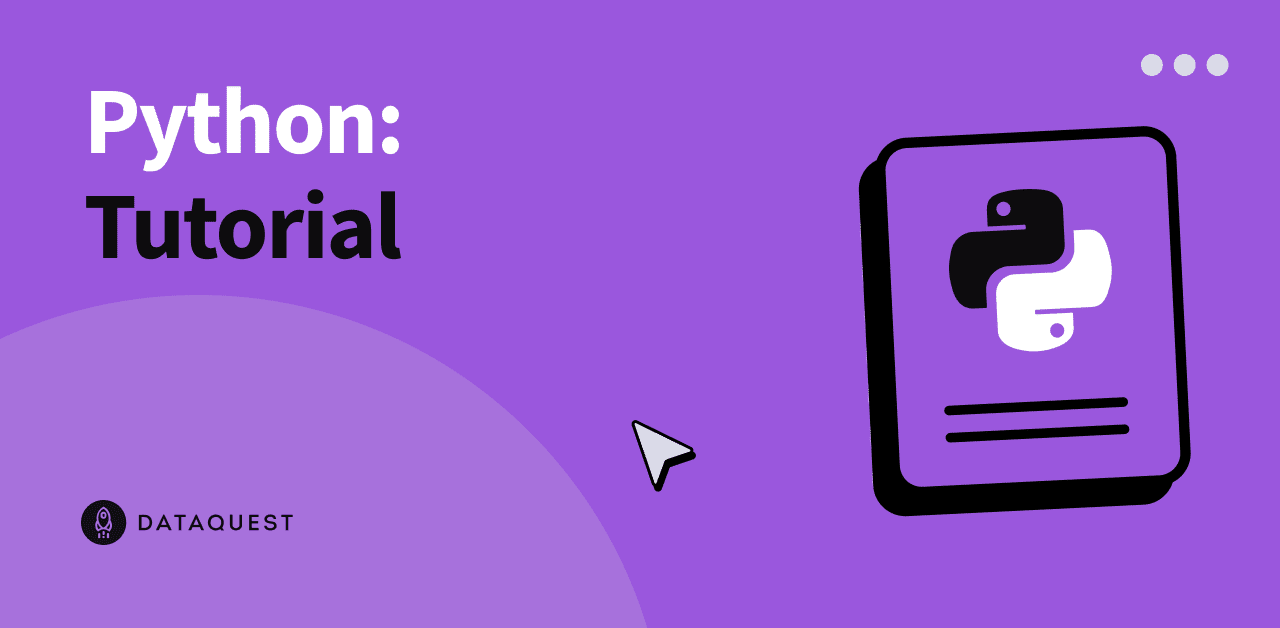

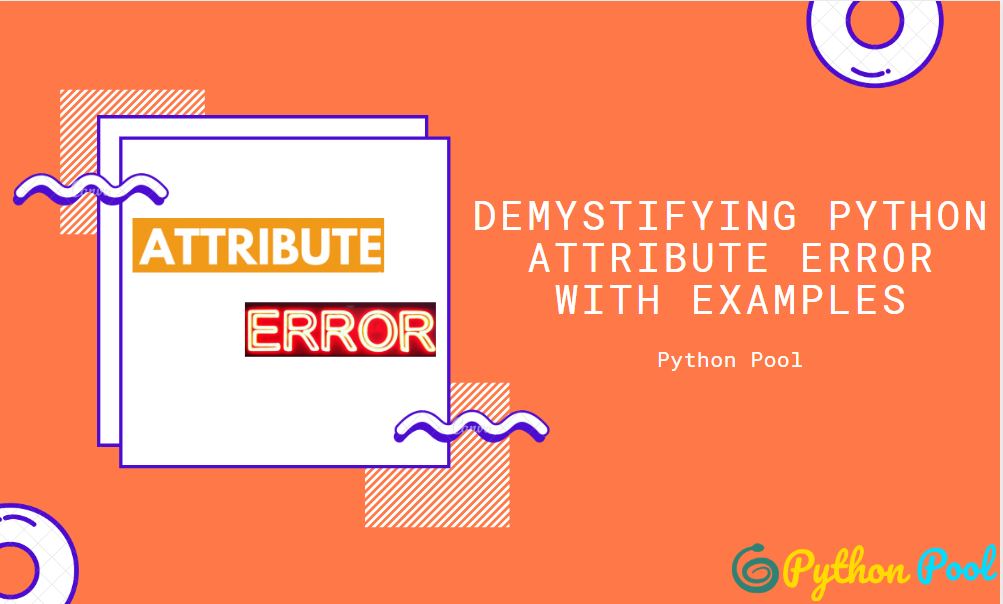
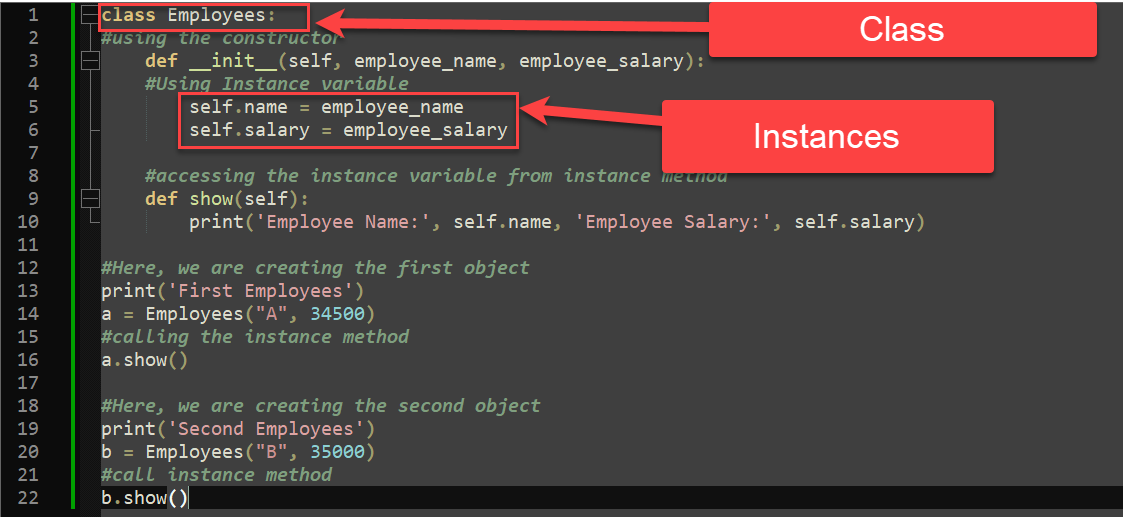
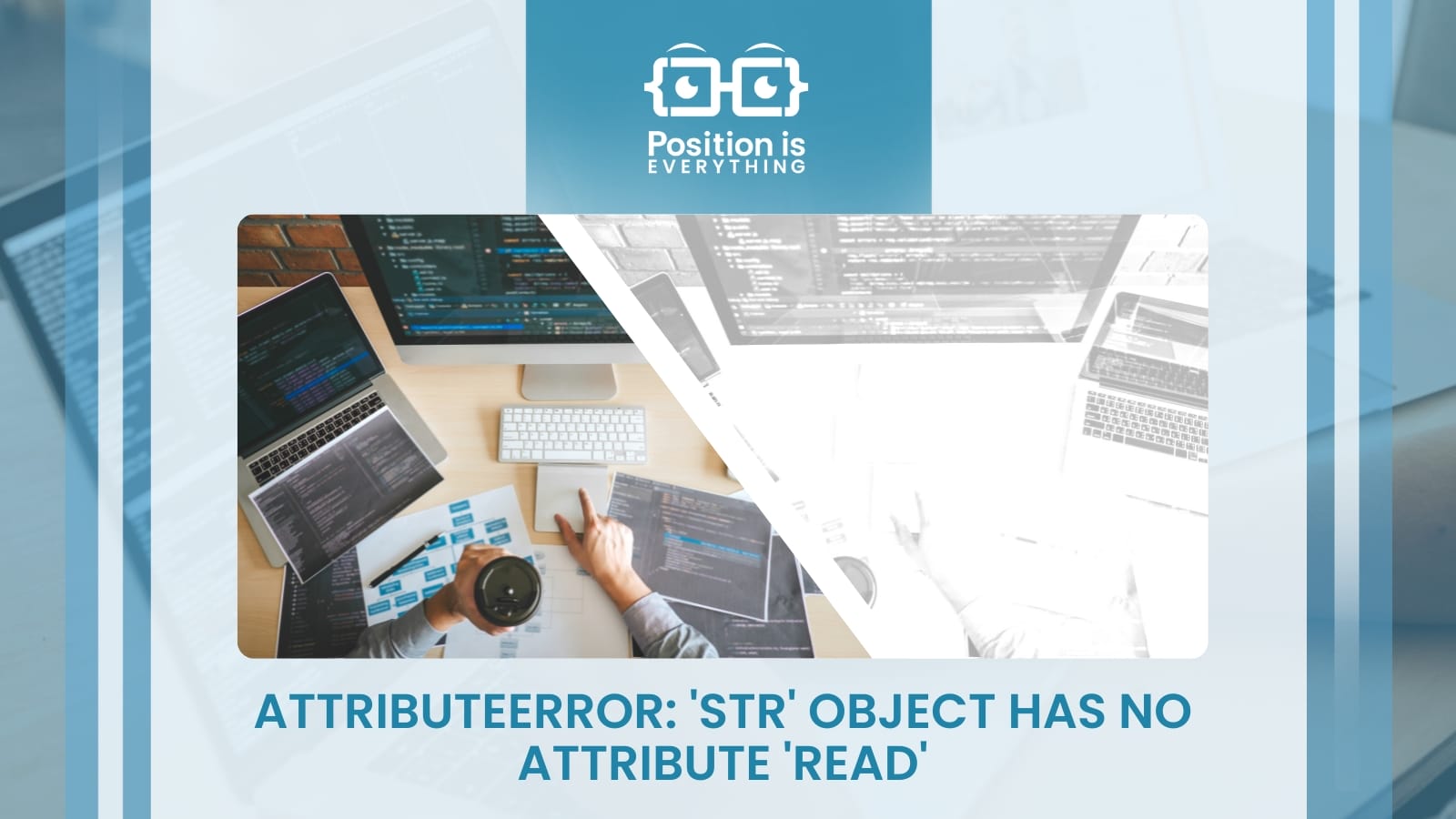
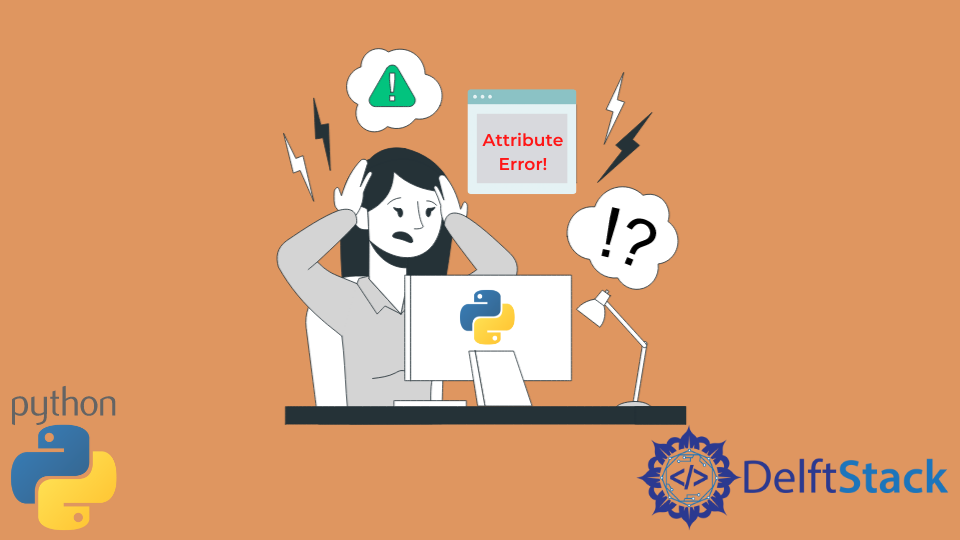
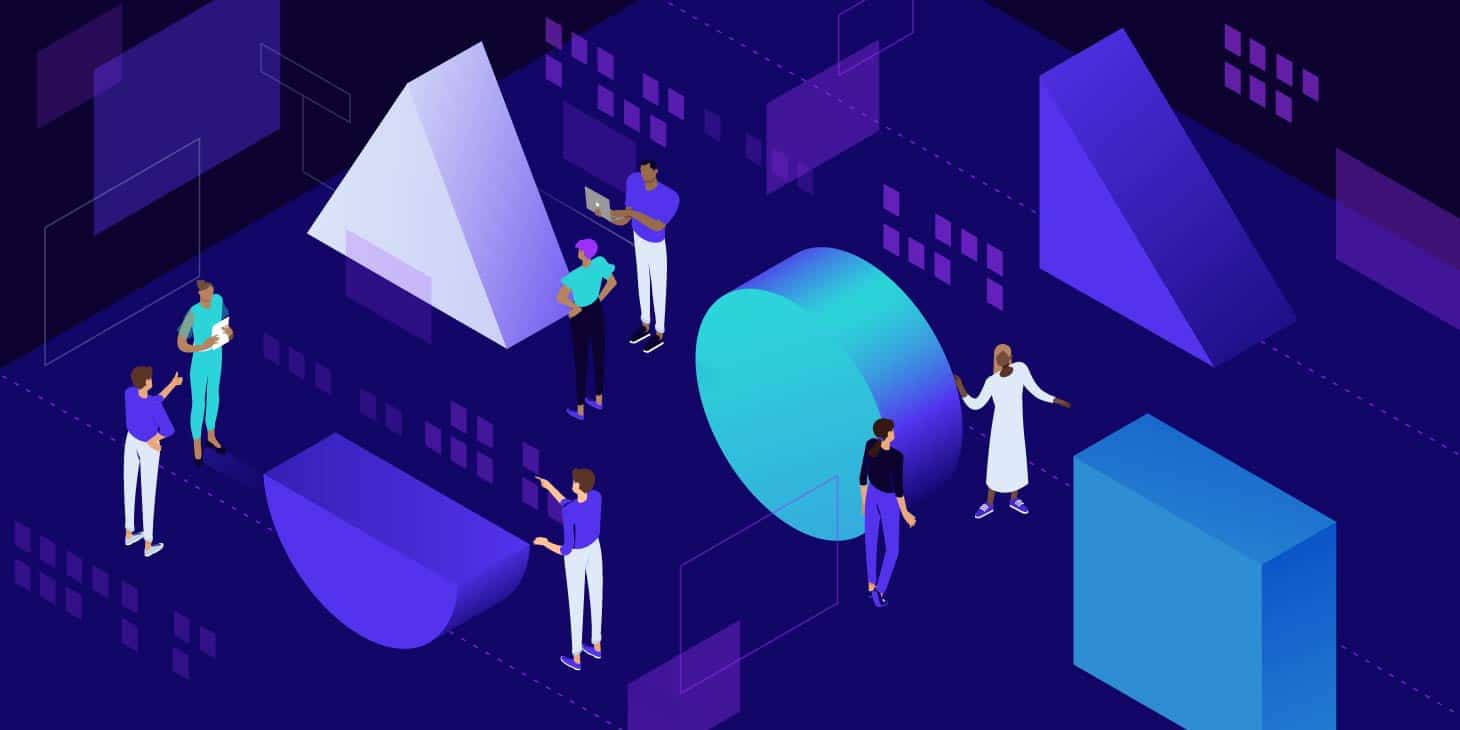
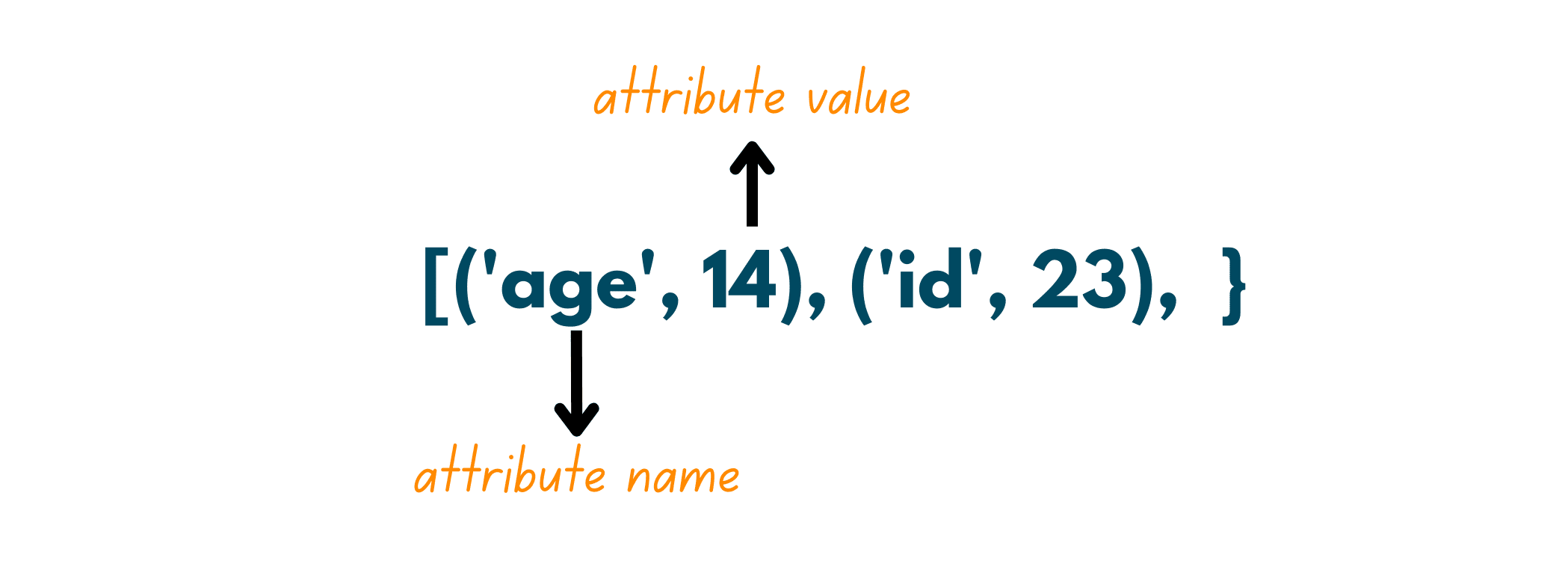
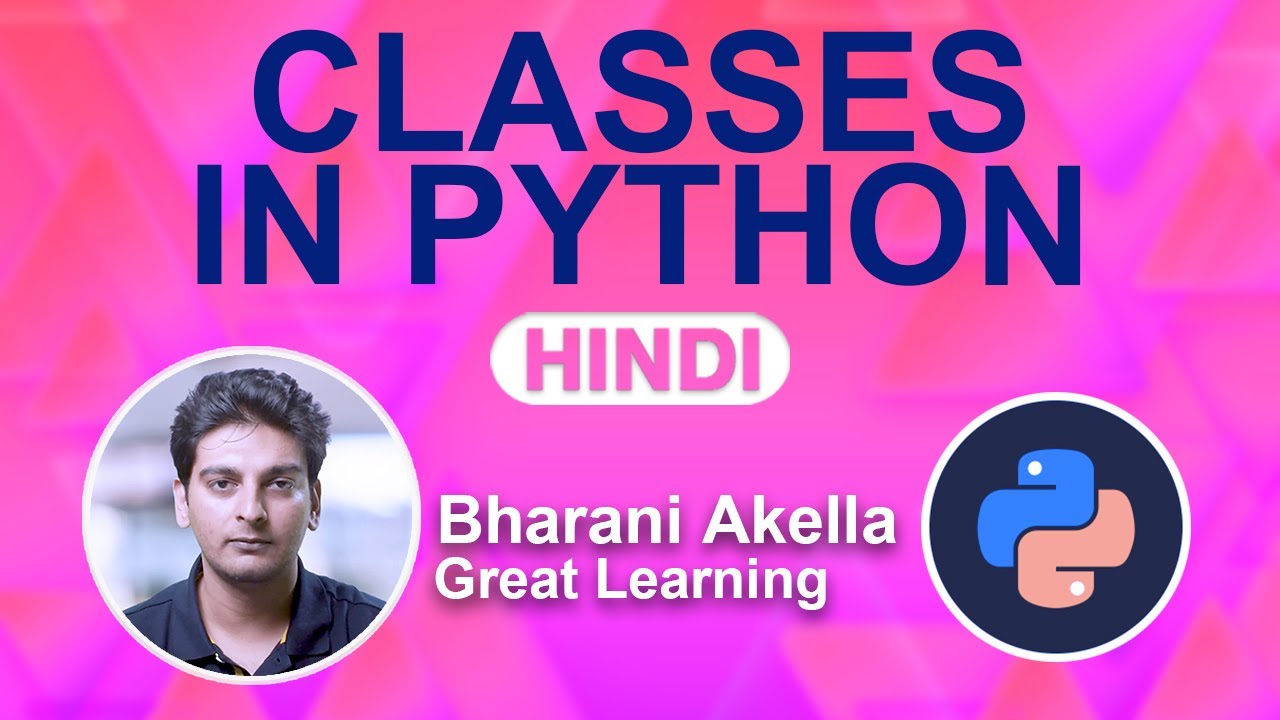
Article link: python print object attributes.
Learn more about the topic python print object attributes.
- Python: Print an Object’s Attributes – Datagy
- How to Print Object Attributes in Python? (with Code) – FavTutor
- Print Object Properties and Values in Python?
- Is there a built-in function to print all the current properties and …
- Object Oriented Programming Python
- Print Object’s Attributes in Python | Delft Stack
- How to Print Object Attributes in Python? – Its Linux FOSS
- How to print all the attributes of an object in Python – Adam Smith
- How to get all Attributes of an Object in Python – bobbyhadz
- How to get all object attributes in Python – CodeSpeedy
See more: https://nhanvietluanvan.com/luat-hoc