Python Printing Exception Message
Defining the concept of exceptions:
In Python, exceptions refer to events that occur during the execution of a program that disrupts the normal flow of the code. These exceptions are typically raised when an error or unexpected condition arises, and if not handled properly, they can cause the program to terminate abruptly.
Exploration of different types of exceptions in Python:
Python provides a wide range of built-in exceptions to cover various types of errors and unexpected conditions. Understanding these exceptions is crucial for effectively handling errors in your programs. Some common types of exceptions include:
1. SyntaxError: It occurs when the code violates Python’s syntax rules.
2. NameError: Raised when a variable or a name is not defined.
3. TypeError: Raised when an operation or function is applied to an object of inappropriate type.
4. ValueError: Occurs when a function receives an argument of correct type but with an invalid value.
5. ZeroDivisionError: Raised when attempting to divide a number by zero.
6. FileNotFoundError: Raised when a file or directory does not exist.
7. IndexError: Occurs when trying to access an index that is out of range.
8. KeyError: Raised when attempting to access a dictionary key that does not exist.
9. ImportError: Raised when a module or package cannot be imported.
10. AttributeError: Occurs when an attribute reference or assignment fails.
Handling exceptions using the try-except block:
To handle exceptions in Python, we use the try-except block. The code that might raise an exception is placed inside the try block, and the code to handle the exception is written inside the except block. Here’s the general syntax:
“`
try:
# code that might raise an exception
except ExceptionType:
# code to handle the exception
“`
Printing the exception message using the except block:
When an exception is raised, Python provides a helpful error message indicating the details of the exception. By default, this error message is printed to the console. However, you can also explicitly print the exception message using the except block. Here’s an example:
“`python
try:
# code that might raise an exception
except ExceptionType as e:
print(“Exception:”, e)
“`
In this example, the exception message is stored in the variable “e” using the “as” keyword, and then it is printed using the print() function.
Understanding the syntax of printing the exception message:
In Python, exception objects contain important information about the error that occurred. The common syntax for printing the exception message is:
“`python
print(“Exception:”, exc_type, “:”, exc_value)
“`
Here, the “exc_type” represents the type of the exception, and “exc_value” represents the detailed message associated with the exception. By printing these values, you can get a clear understanding of the exception that occurred.
Utilizing the traceback module to print the complete traceback information:
Sometimes, simply printing the exception message may not be sufficient for debugging complex issues. In such cases, the traceback module in Python can be used to print the complete traceback information. This includes the sequence of function calls and line numbers leading up to the exception. Here’s an example:
“`python
import traceback
try:
# code that might raise an exception
except ExceptionType as e:
traceback.print_exc()
“`
The traceback.print_exc() function prints the complete traceback information, helping you identify the exact location where the exception was raised.
Customizing exception messages for better error logging and debugging:
In certain scenarios, it is beneficial to customize exception messages to provide specific details or additional context about the error. This can greatly aid in error logging and debugging. To customize exception messages, you can create your own exception classes by inheriting from the built-in Exception class. Here’s an example:
“`python
class MyException(Exception):
def __init__(self, message):
self.message = message
try:
# code that might raise an exception
except ExceptionType as e:
raise MyException(“Custom message”) from e
“`
In this example, the custom exception class “MyException” is defined with an initializer that accepts a message parameter. When raising this exception, you can provide a custom message associated with the error.
FAQs:
Q1. Why is exception handling important in Python?
Exception handling allows us to gracefully handle errors and unexpected conditions in our programs. It prevents the program from crashing and gives us the ability to respond appropriately to errors.
Q2. How can I identify the name of an exception in Python?
To get the name of an exception, you can use the type() function and pass the exception object as an argument. For example, type(exception_name).__name__ will give you the name of the exception.
Q3. Can I catch multiple exceptions in a single try-except block?
Yes, it is possible to catch multiple exceptions in a single try-except block. You can separate the different exception types using commas. For instance:
“`python
try:
# code that might raise exceptions
except (ExceptionType1, ExceptionType2):
# code to handle these exceptions
“`
Q4. Is it necessary to specify the exception type in the except block?
No, it is not necessary to specify the exception type in the except block. If no exception type is specified, the except block will catch all types of exceptions.
Q5. How can I print the line number of an exception in Python?
By default, Python includes the line number in the traceback information when an exception occurs. When you print the complete traceback, it will include the line number where the exception was raised.
In conclusion, understanding how to print exception messages in Python is essential for effective error handling, debugging, and logging. By utilizing the try-except block, printing the exception message, and customizing exception classes, you can create robust and reliable Python programs capable of gracefully handling unexpected conditions.
Python Tutorial: Using Try/Except Blocks For Error Handling
Keywords searched by users: python printing exception message Python print exception message, Print line error python, Exception message Python, Python exception, Log exception Python, Get exception name python, Python try except print error, Custom exception Python
Categories: Top 50 Python Printing Exception Message
See more here: nhanvietluanvan.com
Python Print Exception Message
Understanding Python Exceptions:
Exceptions are special objects that represent an error that occurred during program execution. They help identify the issue by providing detailed information about the error. When an exception is raised, the program execution halts, and the control is transferred to the nearest exception handler that can handle the specific exception type. If no exception handler is found, the program terminates, and the error message is displayed.
Printing the Exception Message:
When an exception occurs, Python raises an error message describing the issue. To print the exception message, you can use the built-in print function along with the error object. For example, consider the following code snippet where we attempt to divide a number by zero:
“`python
try:
result = 10 / 0
except Exception as e:
print(“An error occurred:”, str(e))
“`
In this example, the division by zero raises a `ZeroDivisionError` exception. The exception message provides explicit information about the error. By printing the message using `print`, we get an output like: “An error occurred: division by zero.” This approach allows developers to have a better understanding of the nature of the exception and identify the source of the problem.
Exception Types:
Python provides a wide range of built-in exception types that represent different error scenarios. Some commonly encountered exception types include:
1. `ZeroDivisionError`: Raised when attempting to divide by zero.
2. `TypeError`: Arises when an operation is performed on incompatible data types.
3. `IndexError`: Raised when accessing an index out of range.
4. `ValueError`: Occurs when a function receives an argument of the correct type but an inappropriate value.
5. `KeyError`: Raised when a dictionary key is not found.
6. `FileNotFoundError`: Occurs when a file or directory is requested but cannot be found.
These are just a few examples of the numerous exception types available in Python. Each exception type allows for specialized handling of specific error cases, making it easier to pinpoint and resolve issues.
Exception Handling Techniques:
Python provides a powerful way to handle exceptions by using the `try-except` block. This block allows you to catch and handle exceptions that may occur during code execution. By enclosing the code that may raise an exception within a `try` block and specifying the specific exception type to catch in the corresponding `except` block, you can control how your program reacts to errors. Additionally, you can include multiple `except` blocks to handle different types of exceptions separately.
Consider the following example, where we divide two numbers provided by the user:
“`python
try:
num1 = int(input(“Enter the first number: “))
num2 = int(input(“Enter the second number: “))
result = num1 / num2
print(“Result:”, result)
except ValueError:
print(“Please enter valid numbers!”)
except ZeroDivisionError:
print(“Cannot divide by zero!”)
except Exception as e:
print(“An error occurred:”, str(e))
“`
In this code snippet, we use `try` to encapsulate the risky code that may raise exceptions and then handle specific exceptions in the respective `except` blocks. If a `ValueError` occurs (e.g., when the user enters a non-numeric value), the program displays the error message “Please enter valid numbers!” Likewise, if a `ZeroDivisionError` occurs, a corresponding error message “Cannot divide by zero!” is displayed. If an exception other than those explicitly specified occurs, it falls into the general `Exception` block, where the error message is printed.
Troubleshooting and FAQ:
Q1. What if I don’t handle exceptions in my code?
If an exception occurs, and you haven’t specified any exception handling, the program will terminate, and a traceback message containing detailed information about the exception will be displayed. This information includes the line numbers where the exception occurred, the code block involved, and the exception type along with the error message.
Q2. Can I create my own exception types?
Absolutely! Python allows developers to create their own custom exception types by subclassing the built-in `Exception` class or any of its subclasses. This feature is particularly useful when you want to raise specific exceptions for your custom application logic.
Q3. Can I have nested `try-except` blocks?
Yes, you can nest `try-except` blocks to handle exceptions at different levels of code execution. However, be cautious not to create overly complex nesting, as it can make your code harder to read and maintain.
Q4. How can I log exception messages rather than printing them?
While the built-in `print` function is handy for debugging purposes, it may not be the best option for logging exceptions in production code. Instead, you can utilize a logging framework like the `logging` module in Python. It offers more control and flexibility over handling exceptions and allows you to log messages to various outputs.
In conclusion, understanding how to print exception messages in Python is crucial for effective debugging and troubleshooting. By harnessing the power of exception handling in Python, developers can gracefully recover from errors and enhance the resilience of their applications. Remember to study different exception types, implement appropriate error-handling strategies, and utilize effective logging techniques to build robust and reliable Python programs.
Print Line Error Python
Print statements play a significant role in displaying output or debugging statements during program execution in Python. They are used to print messages, variable values, or any other desired information on the console. The syntax of the print statement in Python 2.x is different from Python 3.x, which is the current version. Therefore, it is essential to understand the differences between the two versions when encountering print line errors.
One common cause of print line errors in Python is the wrong usage of parentheses. In Python 2.x, the print statement does not require parentheses around the argument being printed. For example, the print statement can be written as follows:
“`
print “Hello, World!”
“`
However, in Python 3.x, the print statement is a function, and therefore, it requires parentheses around the argument. The correct syntax for the same example would be:
“`
print(“Hello, World!”)
“`
Failure to include or exclude parentheses as per the Python version being used can result in a print line error.
Another potential source of such errors is the misuse of quotation marks. In Python, double quotes (“”) and single quotes (”) are both valid methods for enclosing strings. However, it is important to maintain consistency and ensure that the opening and closing quotes match. For instance, if a string is enclosed in double quotes, it must be closed with double quotes as well. Mixing different types of quotes within a print statement can lead to syntax errors, including print line errors.
Indentation is another factor affecting the proper execution of print statements in Python. Python is heavily reliant on correct indentation to define the structure and scope of code blocks. A common error is improper indentation of print statements, which can result in a TabError or an IndentationError. To avoid this issue, ensure that the print statement is indented at the same level as the surrounding code.
Debugging print line errors can sometimes be challenging, especially for beginners. Here are a few tips to help identify and resolve such errors effectively:
1. Review the error message: When encountering a print line error, carefully read the error message displayed in the console. It often provides clues about the specific line of code where the error occurs and the nature of the error encountered.
2. Check for syntax errors: Examine the print statement for any obvious syntax mistakes, such as missing parentheses, incorrect quotes, or incorrect indentation. Often, rectifying these errors can fix the print line error.
3. Use print statements for debugging: If the print statement itself is causing the error, consider using other print statements to isolate the problem. By printing intermediate variables or values, you can narrow down the source of the error and identify which part of the code is causing the problem.
Frequently Asked Questions:
Q: Why am I getting an “invalid syntax” error while using the print statement?
A: There might be several reasons for receiving an “invalid syntax” error with the print statement. Firstly, ensure that you are using the correct syntax for the Python version you are using. If you are using Python 2.x, avoid using parentheses around the print statement. On the other hand, in Python 3.x, the print statement requires parentheses. Secondly, verify that the quotes used for string formatting are matching correctly.
Q: How can I print variables and strings together in a print statement?
A: To concatenate variables and strings within a print statement, use the concatenation operator (+) or format the string using the format() method. For example:
“`
name = “John”
age = 25
print(“My name is ” + name + ” and I am ” + str(age) + ” years old.”)
“`
or
“`
name = “John”
age = 25
print(“My name is {} and I am {} years old.”.format(name, age))
“`
Q: I am still unable to resolve the print line error. What should I do?
A: If you have tried the aforementioned troubleshooting steps and are still facing issues, consider seeking help from online programming communities or forums. Provide detailed information about the error, including the code snippet and the error message received. Knowledgeable individuals in these communities can guide you toward a solution.
In conclusion, understanding print line errors is crucial for Python programmers. By identifying common causes and following the tips provided in this article, you can effectively troubleshoot these errors. Remember to pay attention to syntax differences between Python versions, check quotation marks and indentation, and use print statements for debugging purposes.
Images related to the topic python printing exception message
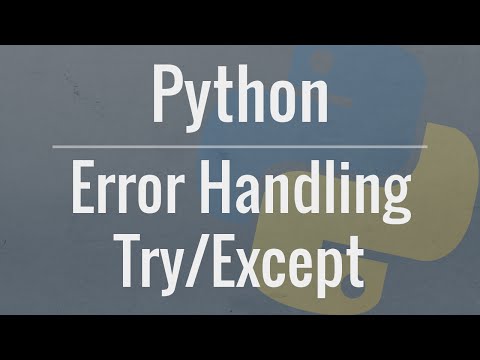
Found 21 images related to python printing exception message theme

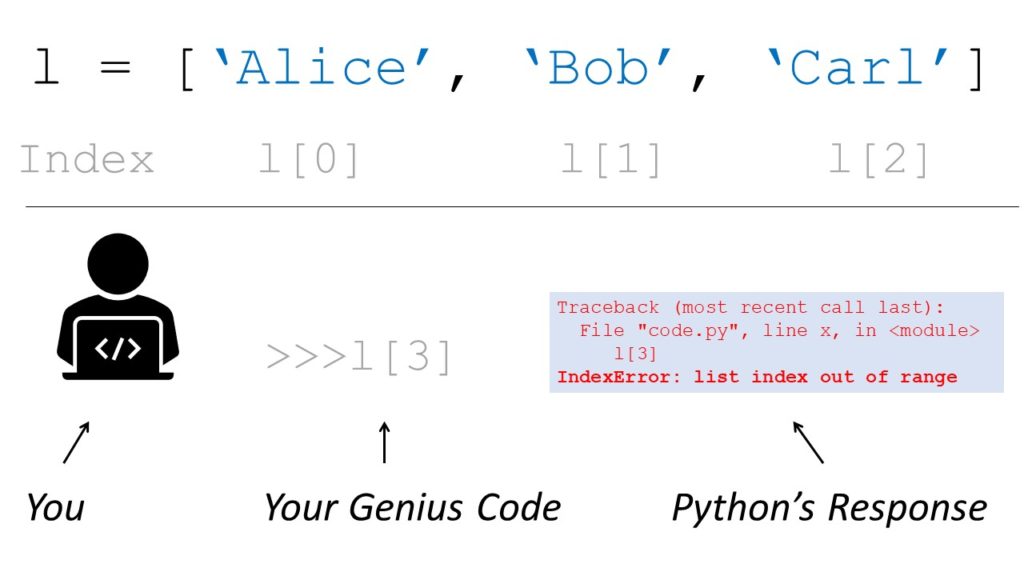

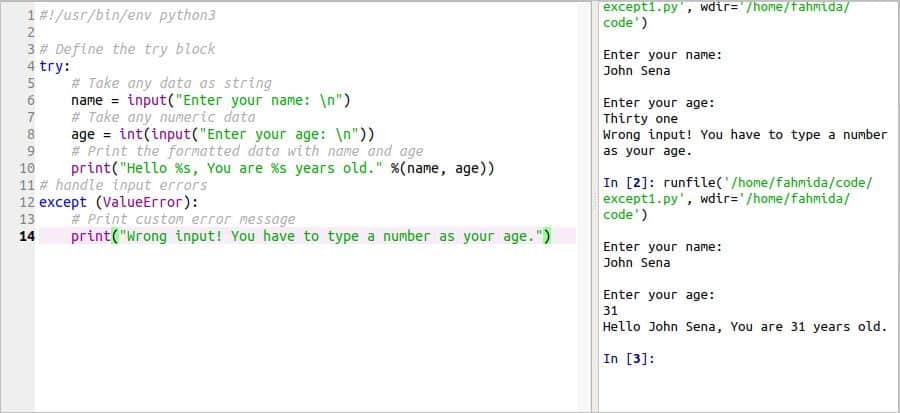
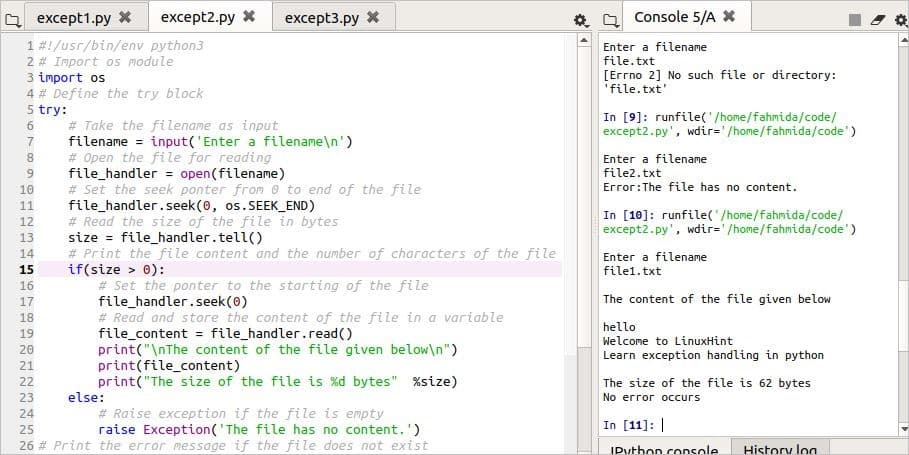
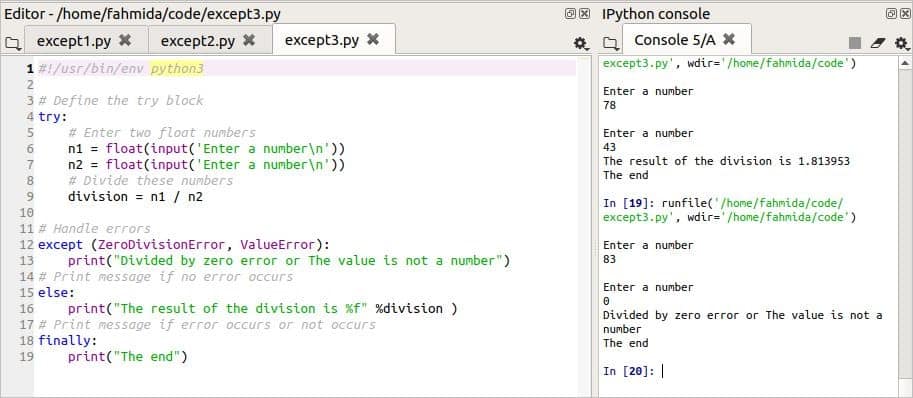
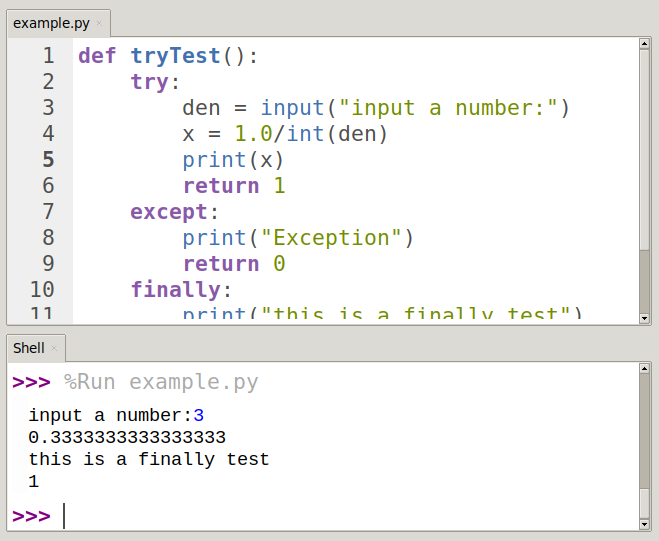

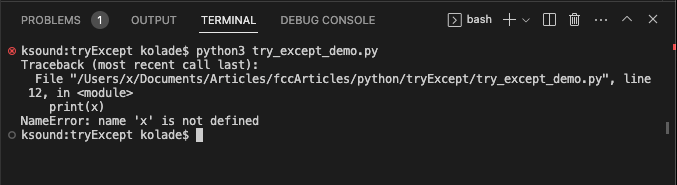
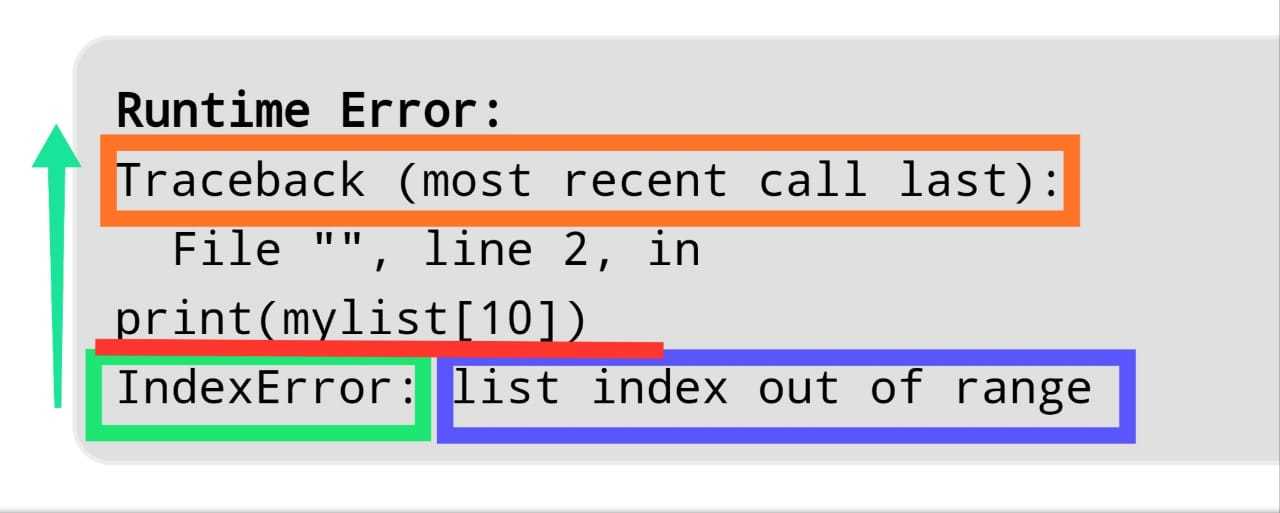
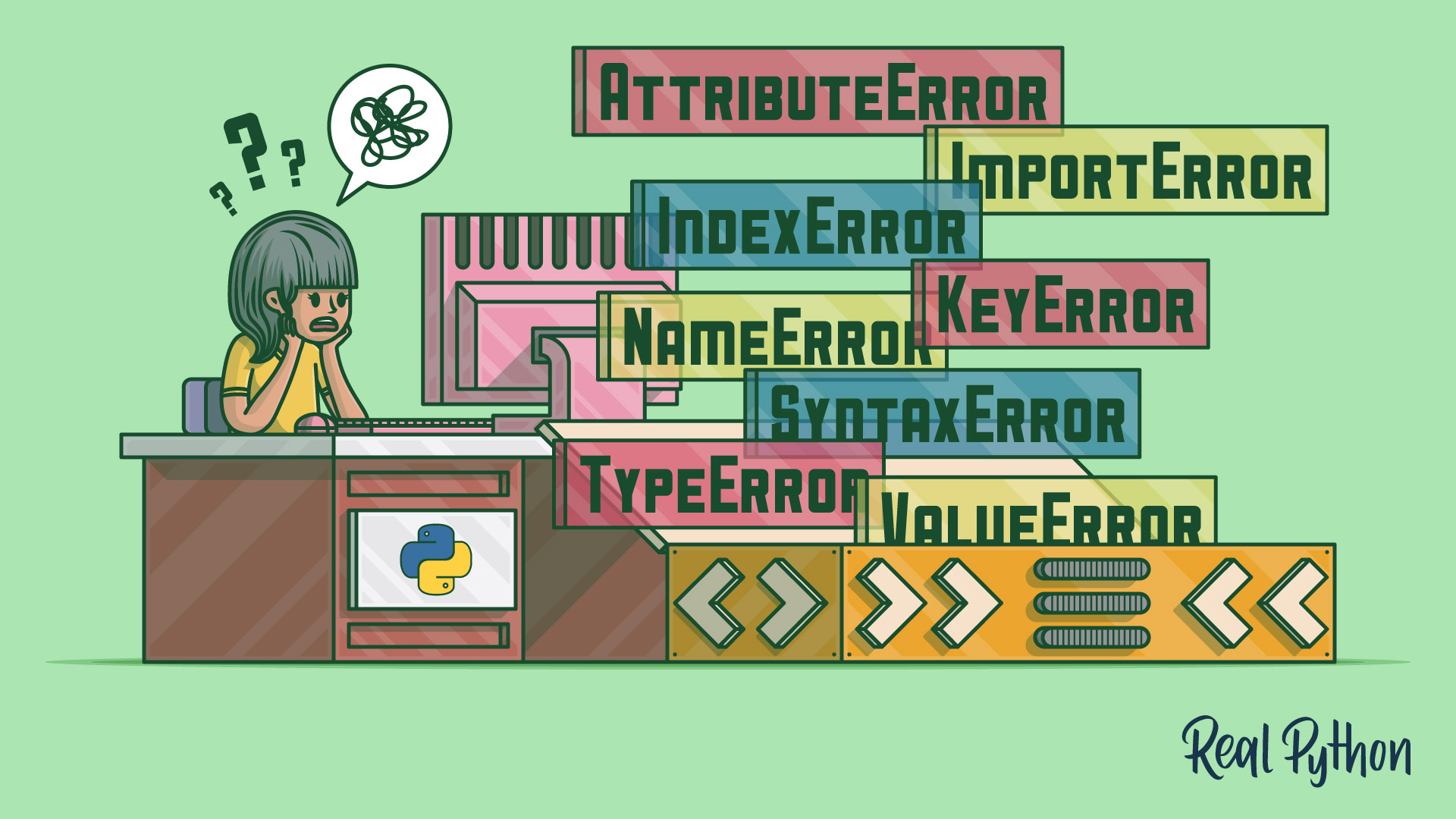
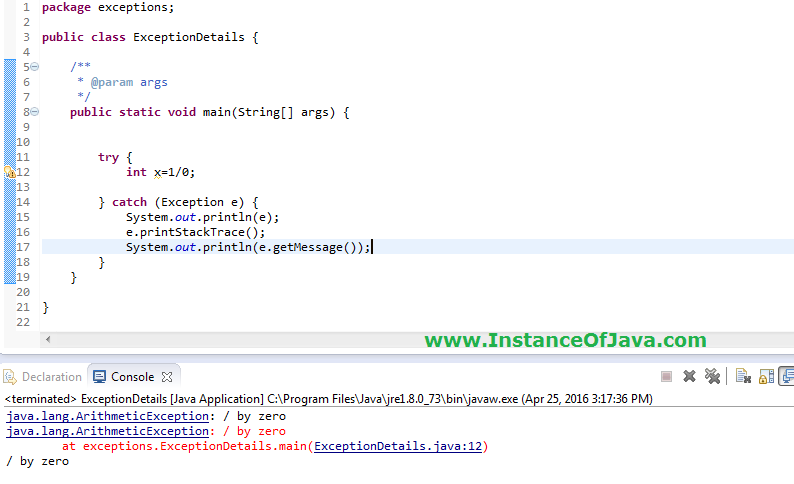
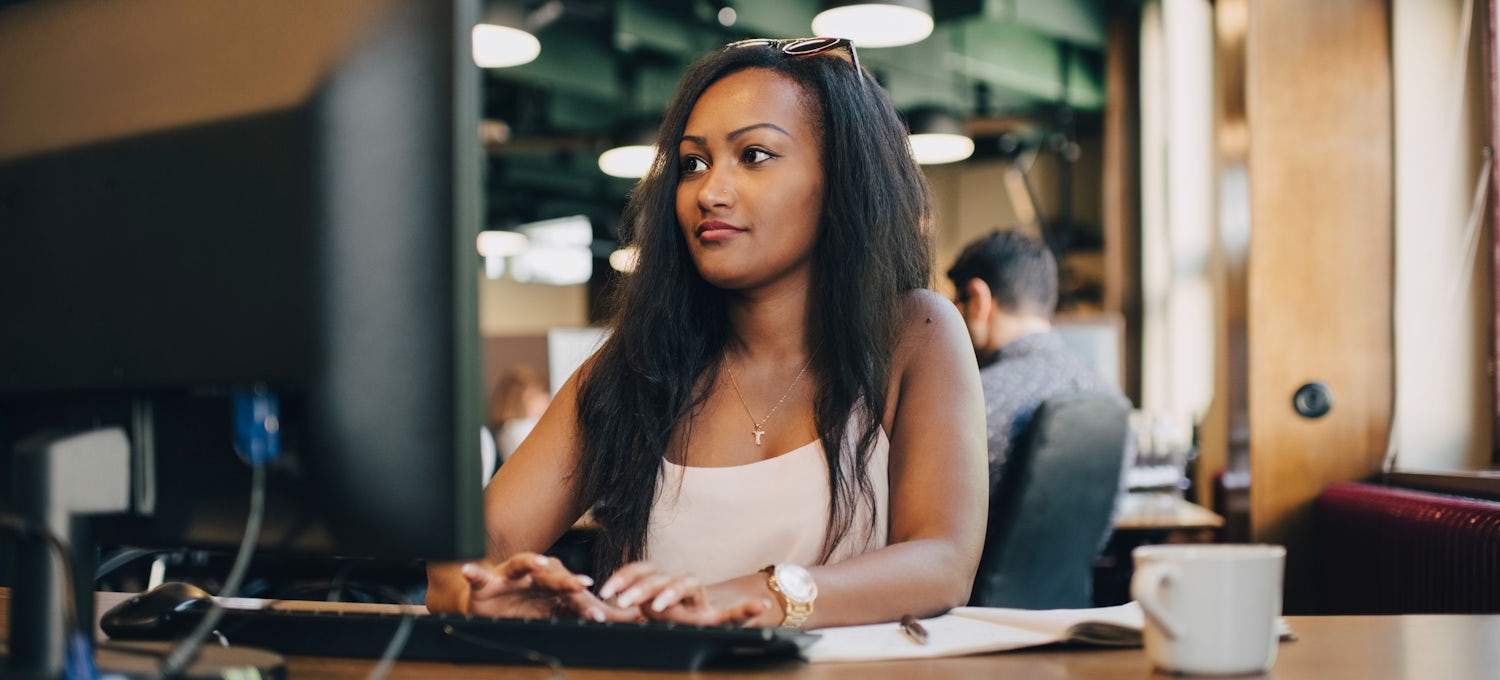


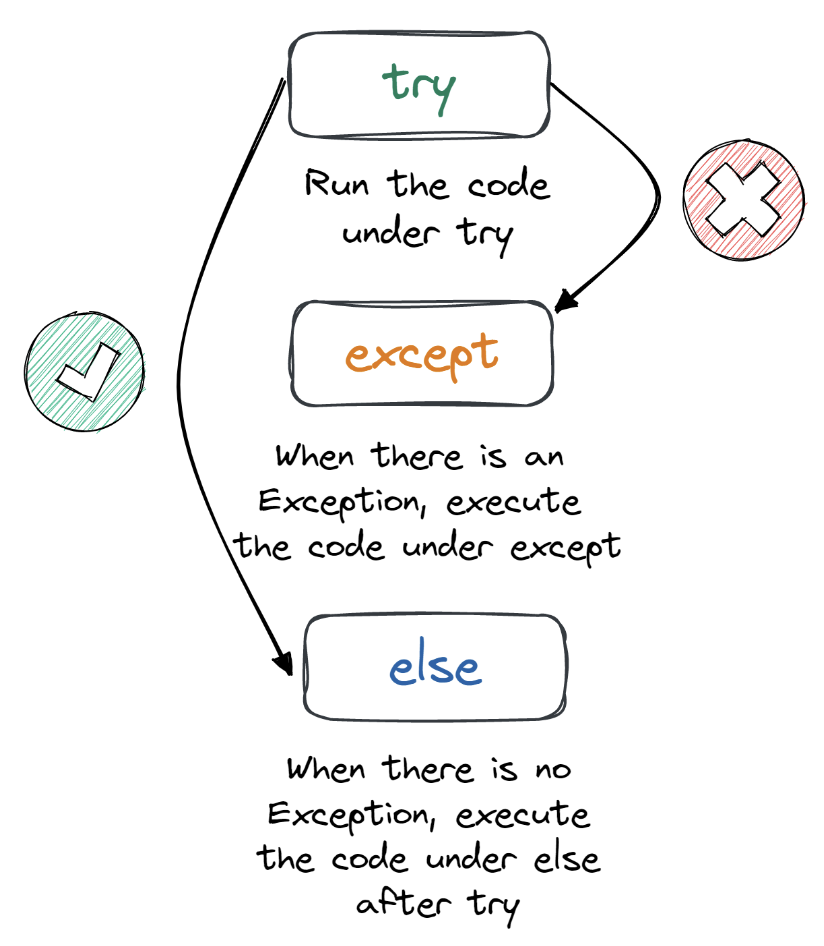

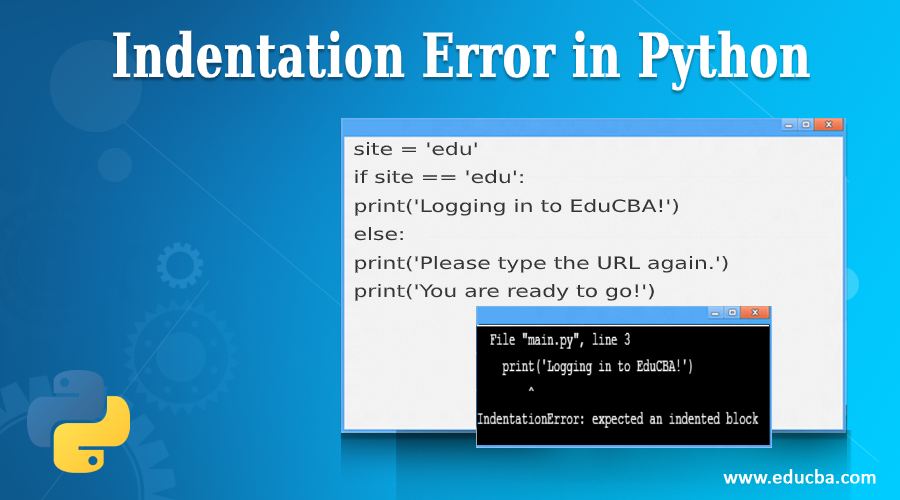
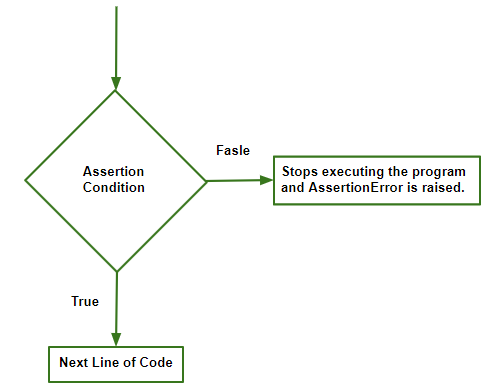
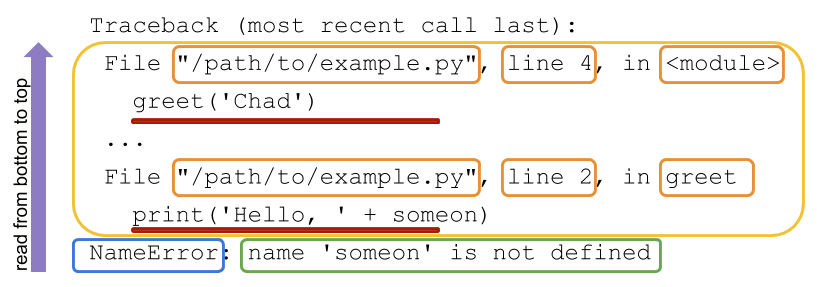
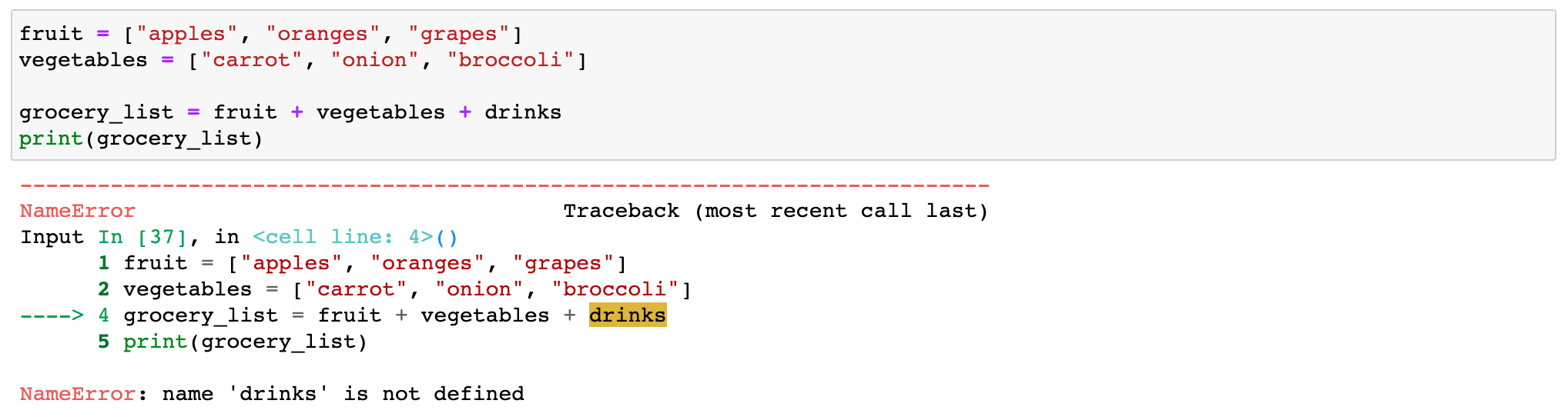
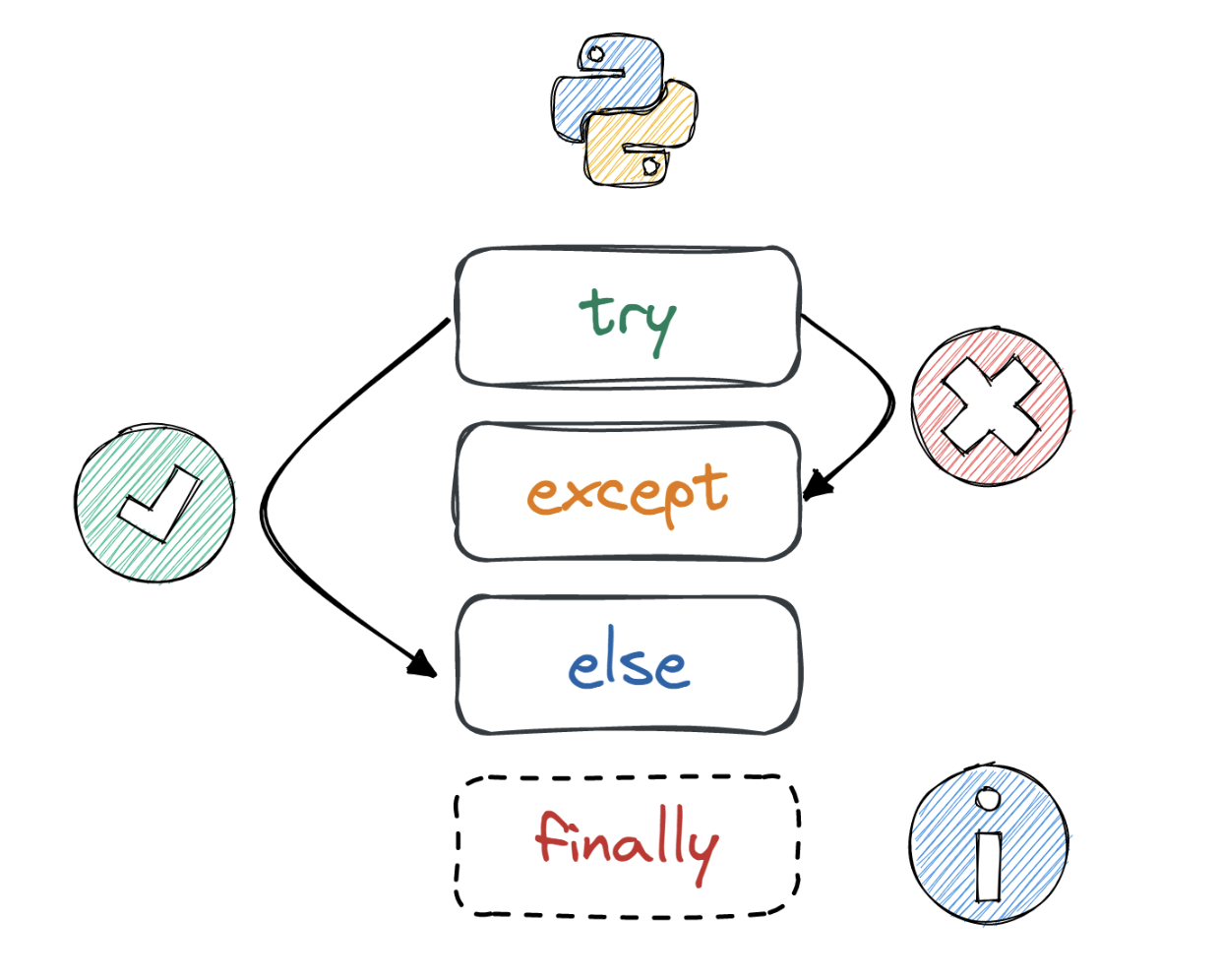
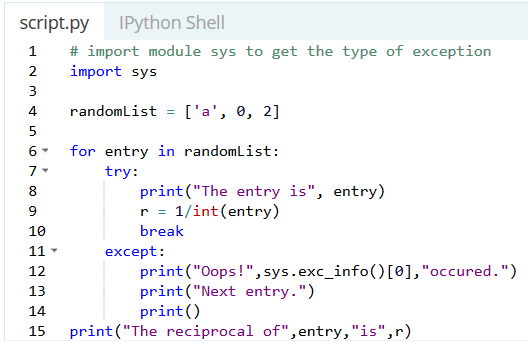

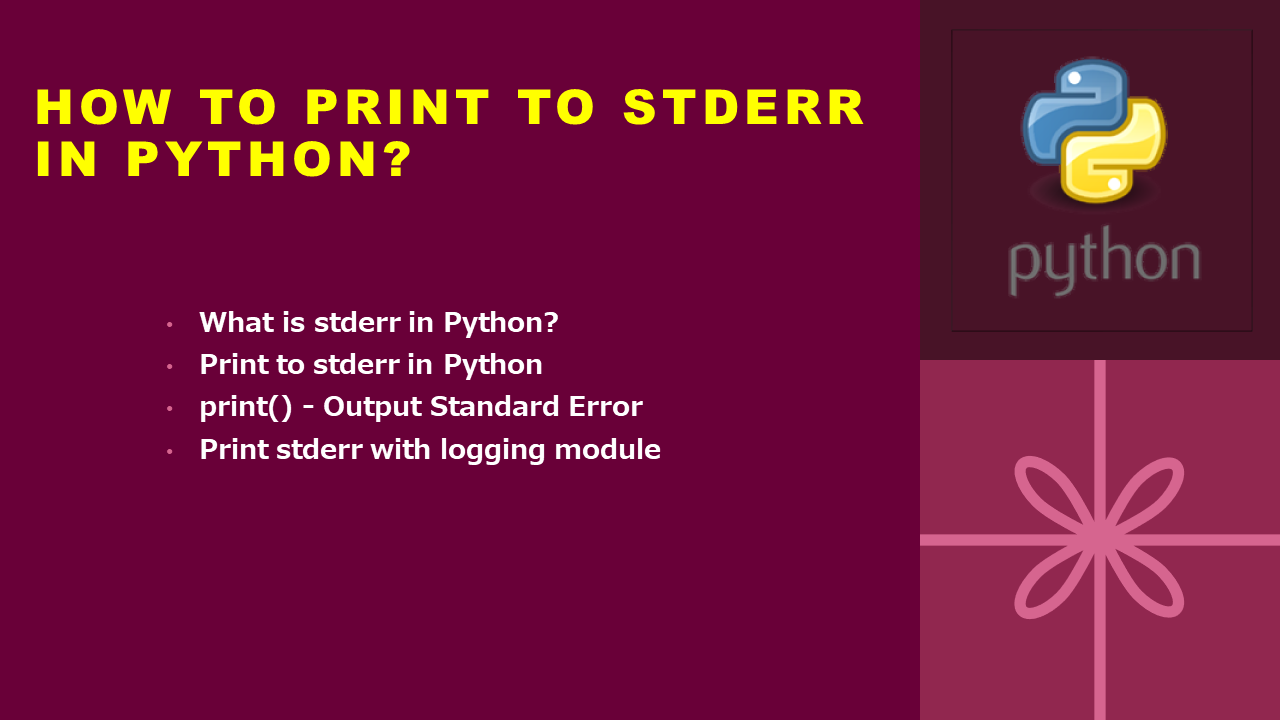

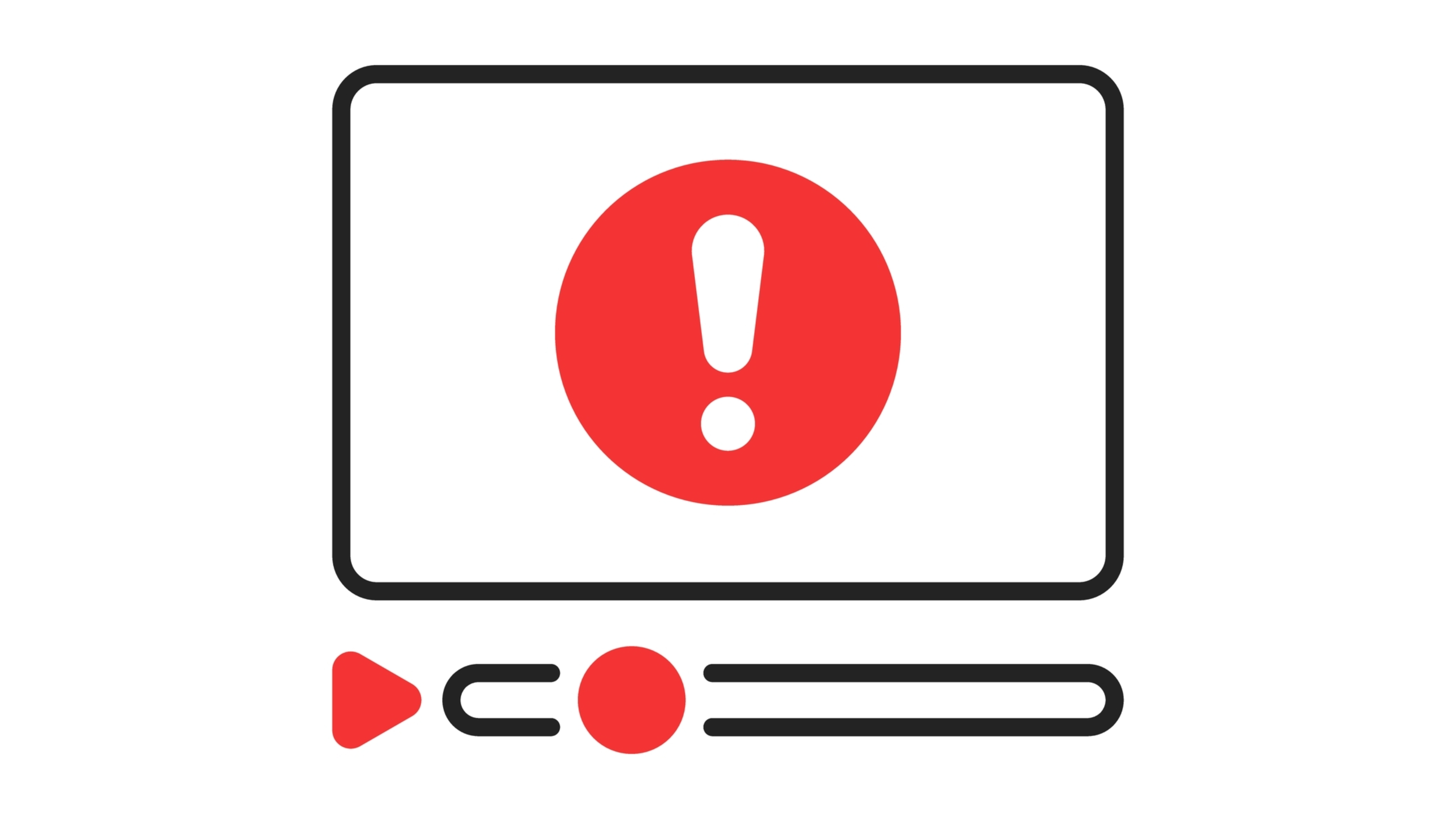
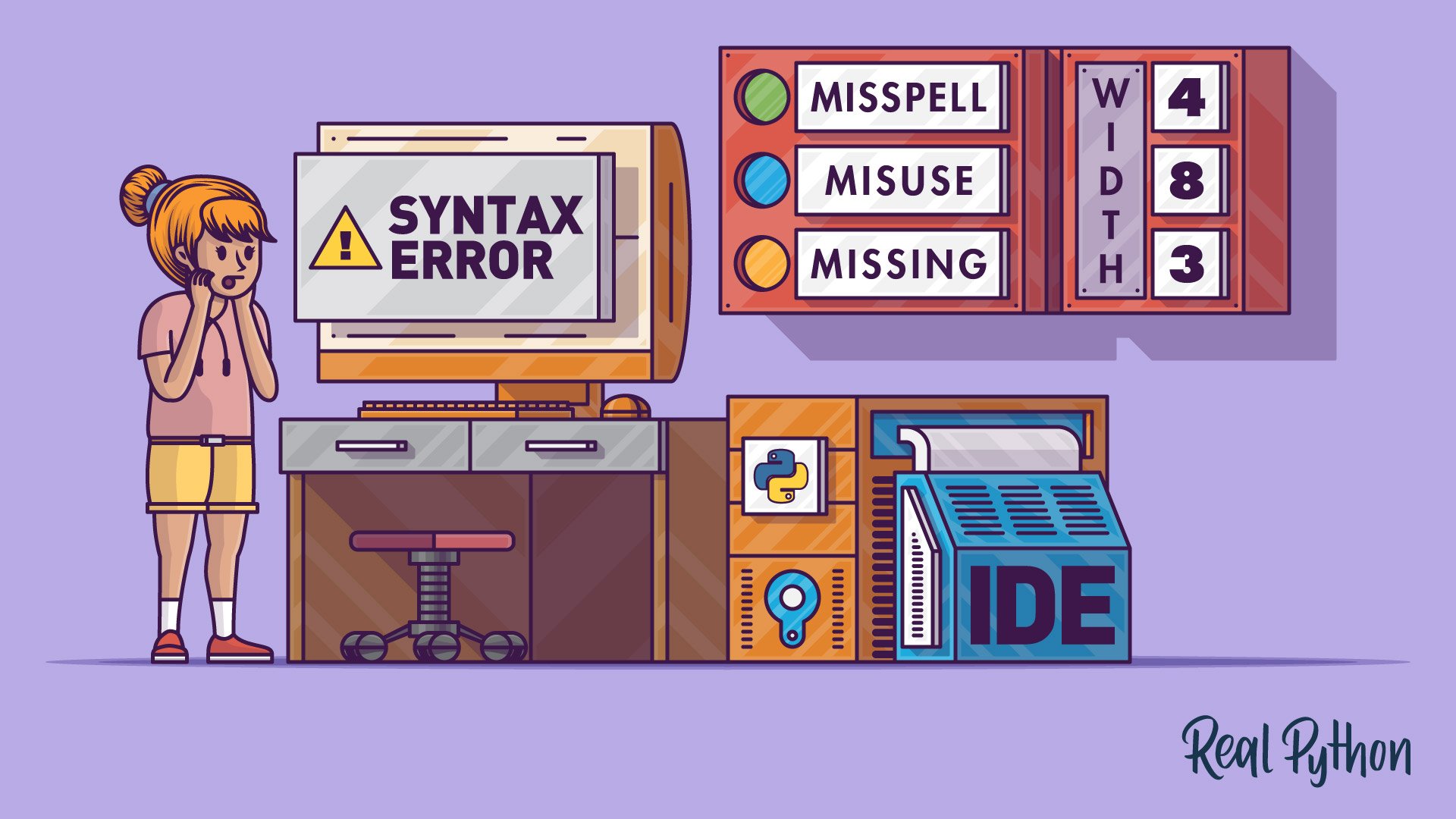



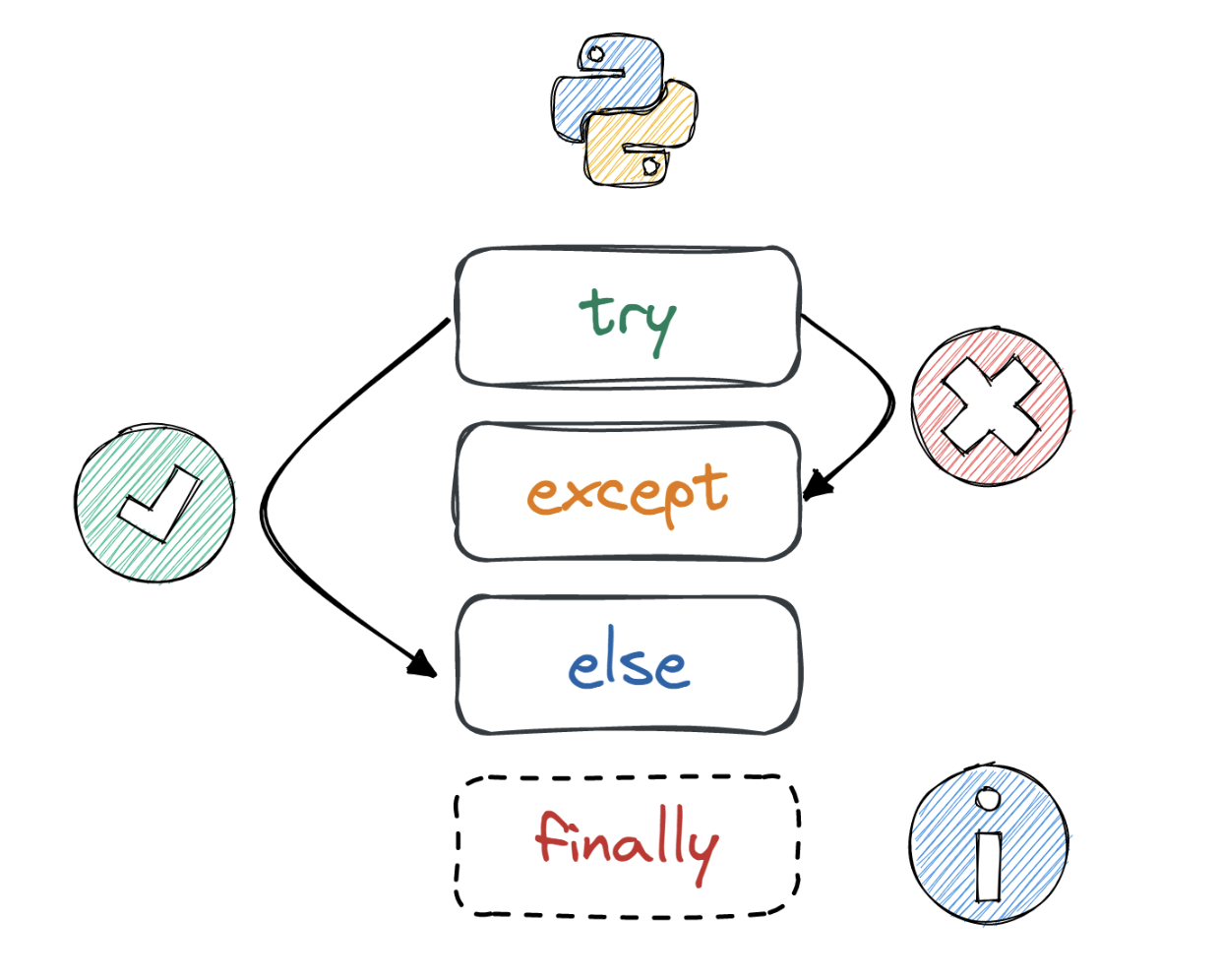
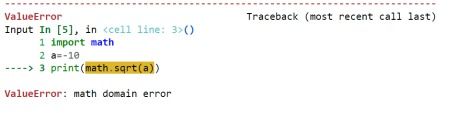

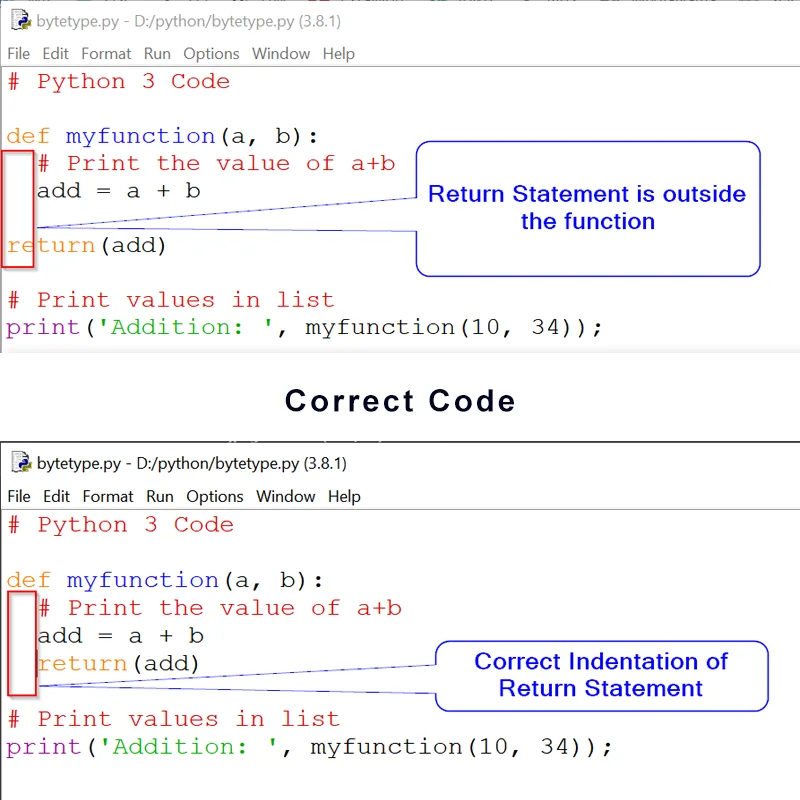
![Python IndexError: List Index Out of Range [Easy Fix] – Be on the Right Side of Change Python Indexerror: List Index Out Of Range [Easy Fix] – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2020/05/image-74.png)



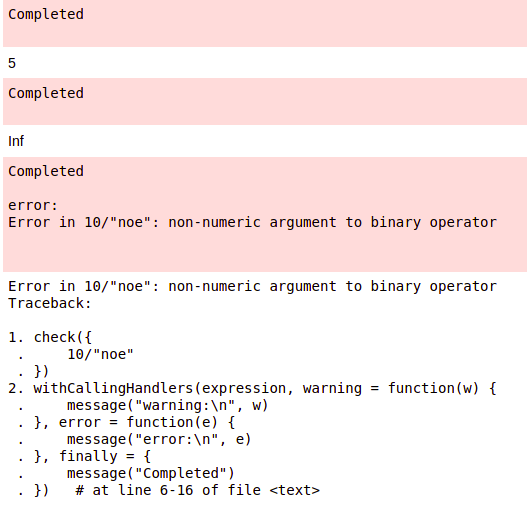
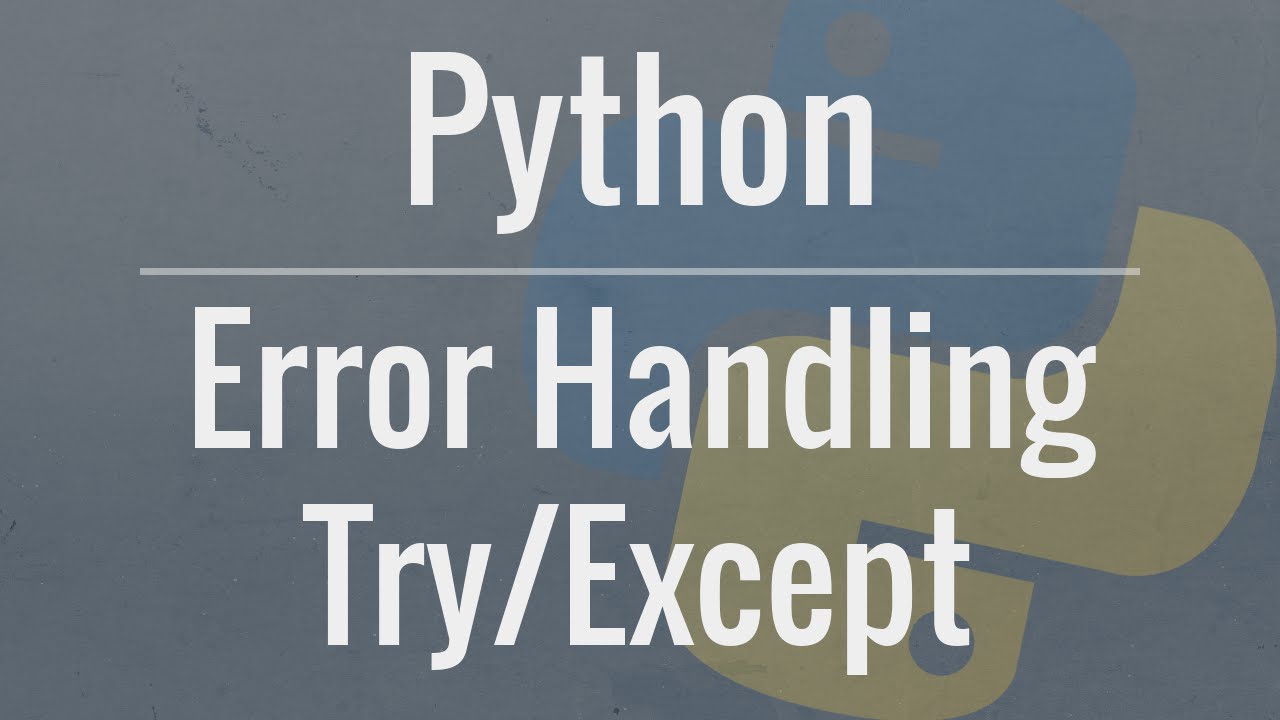
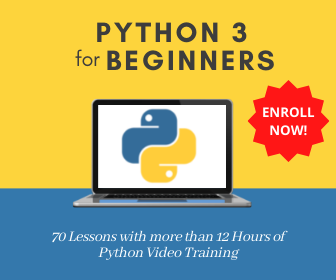
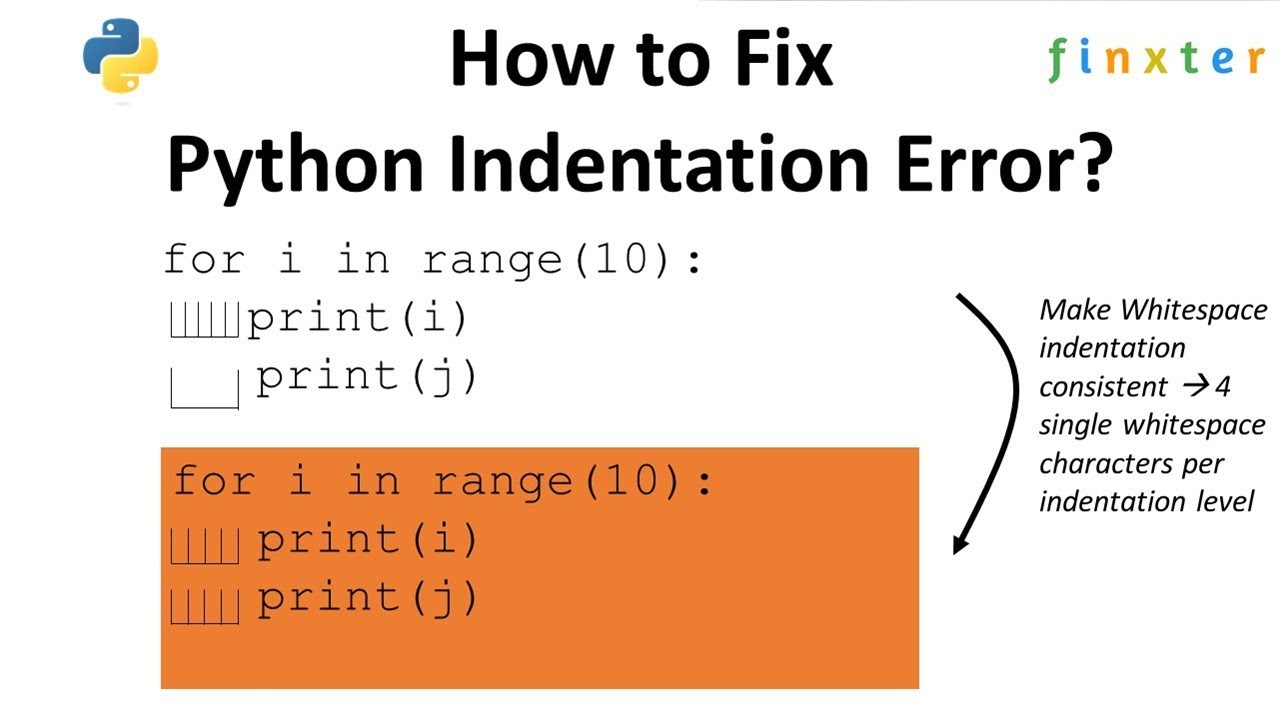
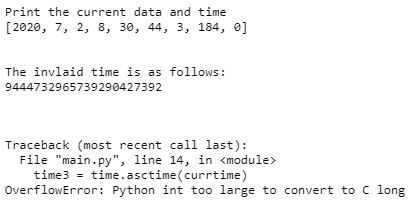
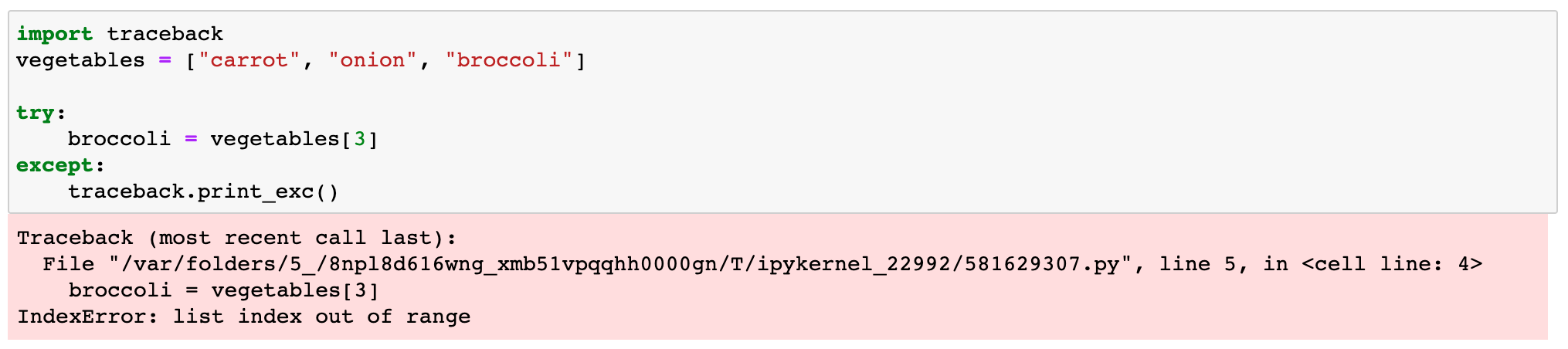
Article link: python printing exception message.
Learn more about the topic python printing exception message.
- python exception message capturing – Stack Overflow
- How to Catch and Print Exception Messages in Python – Finxter
- How can I print an exception message in Python? – Gitnux Blog
- Python Print Exception – How to Try-Except-Print an Error
- How to Catch and Print the Exception Messages in Python
- Python Print Exception Message – Linux Hint
- How do I print an exception in Python? – W3docs
- Python Print exception message – Tutorial – By EyeHunts
- 8. Errors and Exceptions — Python 3.11.4 documentation
- How to Catch and Print Exception Messages in Python?
See more: https://nhanvietluanvan.com/luat-hoc